salesforce.einstein
Module salesforce.einstein
API
Definitions
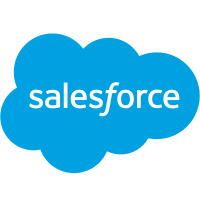
ballerinax/salesforce.einstein Ballerina library
Overview
This is a generated connector for Einstein Vision and Einstein Language API OpenAPI specification.
Allows you to access the Einstein Vision and Einstein Language services via the standard REST API calls. Use the APIs to programmatically work with datasets, labels, examples, models, and predictions.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Einstein API Account.
- Obtain tokens by following this guide.
Quickstart
To use the Salesfore Einstein Vision and Einstein Language connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/salesforce.einstein
module into the Ballerina project.
import ballerinax/salesforce.einstein;
Step 2 - Create a new connector instance
You can now make the connection configuration using the <ACCESS_TOKEN>.
einstein:ClientConfig clientConfig = { auth : { token: `<ACCESS_TOKEN>` } }; einstein:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example to list datasets using the connector.
public function main() { einstein:DatasetList|error result = baseClient->listDatasets(); if (result is einstein:DatasetList) { log:printInfo(result.toString()); } else { log:printInfo(result.toString()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
salesforce.einstein: Client
This is a generated connector for Einstein Vision and Einstein Language API OpenAPI specification. Allows you to access the Einstein Vision and Einstein Language services via the standard REST API calls. Use the APIs to programmatically work with datasets, labels, examples, models, and predictions.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Einstein API Account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.einstein.ai" - URL of the target service
getApiUsage
function getApiUsage() returns ApiUsageList|error
Get API Isage
Return Type
- ApiUsageList|error - api usage
listLanguageDatasets
function listLanguageDatasets(string offset, string count, boolean global) returns DatasetList|error
Get All Langugae Datasets
Parameters
- offset string (default "0") - Index of the dataset from which you want to start paging
- count string (default "25") - Number of datsets to return. Maximum valid value is 25. If you specify a number greater than 25, the call returns 25 datasets.
- global boolean (default false) - If true, returns all global datasets. Global datasets are public datasets that Salesforce provides.
Return Type
- DatasetList|error - Success
uploadDatasetAsync
function uploadDatasetAsync(DatasetsUploadBody payload) returns Dataset|error
Create a Dataset From a File Asynchronously
Parameters
- payload DatasetsUploadBody - Create dataset payload
uploadDatasetSync
function uploadDatasetSync(UploadSyncBody payload) returns Dataset|error
Create a Dataset From a File Synchronously
Parameters
- payload UploadSyncBody - Create dataset payload
getLanguageDataset
Get a Language Dataset
Parameters
- datasetId string - Dataset Id
deleteLanguageDataset
function deleteLanguageDataset(string datasetId) returns DeletionResponse|error
Delete a Langugage Dataset
Parameters
- datasetId string - Dataset Id
Return Type
- DeletionResponse|error - Success
getLanguageExamples
function getLanguageExamples(string datasetId, string offset, string count, string? 'source) returns ExampleList|error
Get All Language Examples
Parameters
- datasetId string - Dataset Id
- offset string (default "0") - Index of the example from which you want to start paging.
- count string (default "100") - Number of examples to return.
- 'source string? (default ()) - return examples that were created in the dataset as feedback
Return Type
- ExampleList|error - Success
getTrainedLanguageModels
function getTrainedLanguageModels(string datasetId, string offset, string count) returns ModelList|error
Get All Language Models
Parameters
- datasetId string - Dataset Id
- offset string (default "0") - Index of the model from which you want to start paging.
- count string (default "100") - Number of models to return.
updateLanguageDatasetAsync
function updateLanguageDatasetAsync(string datasetId, DatasetidUploadBody payload) returns Dataset|error
Create Examples From a File
getLanguageDatasetDeletionStatus
function getLanguageDatasetDeletionStatus(string id) returns DeletionResponse|error
Get Deletion Status of Language Dataset
Parameters
- id string - Deletion Id
Return Type
- DeletionResponse|error - deletion status
getExamplesByLabel
function getExamplesByLabel(string? labelId, string offset, string count) returns ExampleList|error
Get All Examples for Label
Parameters
- labelId string? (default ()) - Label Id
- offset string (default "0") - Index of the example from which you want to start paging.
- count string (default "100") - Number of examples to return.
Return Type
- ExampleList|error - Success
provideLanguageFeedbackExample
function provideLanguageFeedbackExample(LanguageFeedbackBody payload) returns Example|error
Create a Language Feedback Example
Parameters
- payload LanguageFeedbackBody - Create dataset feedback payload
getIntentPrediction
function getIntentPrediction(IntentPredictRequest payload) returns IntentPredictResponse|error
Prediction for Intent
Parameters
- payload IntentPredictRequest - Intent prediction payload
Return Type
- IntentPredictResponse|error - Prediction Result
getTrainedLanguageModelMetrics
Get Language Model Metrics
Parameters
- modelId string - Model Id
deleteLanguageModel
function deleteLanguageModel(string modelId) returns DeletionResponse|error
Delete a Language Model
Parameters
- modelId string - Model Id
Return Type
- DeletionResponse|error - Deletion submitted
getTrainedLanguageModelLearningCurve
function getTrainedLanguageModelLearningCurve(string modelId, string offset, string count) returns LearningCurveList|error
Get Language Model Learning Curve
Parameters
- modelId string - Model ID
- offset string (default "0") - Index of the epoch from which you want to start paging
- count string (default "25") - Number of epoch to return. Maximum valid value is 25.
Return Type
- LearningCurveList|error - Learning Curve
retrainLanguageDataset
function retrainLanguageDataset(LanguageRetrainBody payload) returns TrainResponse|error
Retrain a Language Dataset
Parameters
- payload LanguageRetrainBody - Retrain language dataset payload.
Return Type
- TrainResponse|error - Training Status
getSentimentPrediction
function getSentimentPrediction(SentimentPredictRequest payload) returns SentimentPredictResponse|error
Get Sentiment Prediction
Parameters
- payload SentimentPredictRequest - Sentiment prediction payload
Return Type
- SentimentPredictResponse|error - Prediction Result
trainLanguageDataset
function trainLanguageDataset(LanguageTrainBody payload) returns TrainResponse|error
Train a Langugage Dataset
Parameters
- payload LanguageTrainBody - Train dataset payload
Return Type
- TrainResponse|error - Training Status
getLanguageTrainStatusAndProgress
function getLanguageTrainStatusAndProgress(string modelId) returns TrainResponse|error
Get Language Training Status
Parameters
- modelId string - Model ID
Return Type
- TrainResponse|error - Training Status
generateTokenV2
function generateTokenV2(Oauth2TokenBody payload) returns GenerateAccessTokenResponse|error
Generate an OAuth Token
Parameters
- payload Oauth2TokenBody - Generate token payload
Return Type
- GenerateAccessTokenResponse|error - access token result
revokeRefreshTokenV2
Delete a Refresh Token
Parameters
- token string - the token to revoke
addFeedbackExamples
function addFeedbackExamples(VisionBulkfeedbackBody payload) returns Dataset|error
Create Feedback Examples From a Zip File
Parameters
- payload VisionBulkfeedbackBody - Create feedback payload.
listVisionDatasets
function listVisionDatasets(string offset, string count, boolean global) returns DatasetList|error
Get All Vision Datasets
Parameters
- offset string (default "0") - Index of the dataset from which you want to start paging
- count string (default "25") - Number of datsets to return. Maximum valid value is 25. If you specify a number greater than 25, the call returns 25 datasets.
- global boolean (default false) - If true, returns all global datasets. Global datasets are public datasets that Salesforce provides.
Return Type
- DatasetList|error - Success
createDataset
function createDataset(VisionDatasetsBody payload) returns Dataset|error
Create a Dataset
Parameters
- payload VisionDatasetsBody - Create dataset payload
uploadVisionDatasetAsync
function uploadVisionDatasetAsync(DatasetsUploadBody1 payload) returns Dataset|error
Create a Dataset From a Zip File Asynchronously
Parameters
- payload DatasetsUploadBody1 - Create dataset payload
uploadVisionDatasetSync
function uploadVisionDatasetSync(UploadSyncBody1 payload) returns Dataset|error
Create a Dataset From a Zip File Synchronously
Parameters
- payload UploadSyncBody1 - Create dataset payload
getVisionDataset
Get a Vision Dataset
Parameters
- datasetId string - Dataset Id
deleteVisionDataset
function deleteVisionDataset(string datasetId) returns DeletionResponse|error
Delete a Vision Dataset
Parameters
- datasetId string - Dataset Id
Return Type
- DeletionResponse|error - Success
getVisionDatasetExamples
function getVisionDatasetExamples(string datasetId, string offset, string count, string? 'source) returns ExampleList|error
Get All Vision Dataset Examples
Parameters
- datasetId string - Dataset Id
- offset string (default "0") - Index of the example from which you want to start paging.
- count string (default "100") - Number of examples to return.
- 'source string? (default ()) - return examples that were created in the dataset as feedback
Return Type
- ExampleList|error - Success
addExample
function addExample(string datasetId, DatasetidExamplesBody payload) returns Example|error
Create an Example
Parameters
- datasetId string - Dataset Id
- payload DatasetidExamplesBody - Create dataset example payload
getTrainedVisionModels
function getTrainedVisionModels(string datasetId, string offset, string count) returns ModelList|error
Get All Vision Models
Parameters
- datasetId string - Dataset Id
- offset string (default "0") - Index of the model from which you want to start paging.
- count string (default "100") - Number of models to return.
updateVisionDatasetAsync
function updateVisionDatasetAsync(string datasetId, DatasetidUploadBody1 payload) returns Dataset|error
Create Examples From a Zip File
Parameters
- datasetId string - Dataset Id
- payload DatasetidUploadBody1 - Create dataset example payload
getVisionDatasetDeleteionStatus
function getVisionDatasetDeleteionStatus(string id) returns DeletionResponse|error
Get Deletion Status of Vision Dataset
Parameters
- id string - Deletion Id
Return Type
- DeletionResponse|error - deletion status
detectImage
function detectImage(ObjectDetectionRequest payload) returns ObjectDetectionResponse|error
Detection with Image File
Parameters
- payload ObjectDetectionRequest - Object detection payload
Return Type
- ObjectDetectionResponse|error - Detection Result
getVisionExamplesByLabel
function getVisionExamplesByLabel(string? labelId, string offset, string count) returns ExampleList|error
Get All Vision Examples for Label
Parameters
- labelId string? (default ()) - Label Id
- offset string (default "0") - Index of the example from which you want to start paging.
- count string (default "100") - Number of examples to return.
Return Type
- ExampleList|error - Success
provideVisionFeedbackExample
function provideVisionFeedbackExample(VisionFeedbackBody payload) returns Example|error
Create a Vision Feedback Example
Parameters
- payload VisionFeedbackBody - Create dataset feedback payload
getTrainedVisionModelMetrics
Get Vision Model Metrics
Parameters
- modelId string - Model ID
deleteVisionModel
function deleteVisionModel(string modelId) returns DeletionResponse|error
Delete a Vision Model
Parameters
- modelId string - Model ID
Return Type
- DeletionResponse|error - Deletion submitted
getTrainedVisionModelLearningCurve
function getTrainedVisionModelLearningCurve(string modelId, string offset, string count) returns LearningCurveList|error
Get Vision Model Learning Curve
Parameters
- modelId string - Model ID
- offset string (default "0") - Index of the epoch from which you want to start paging
- count string (default "25") - Number of epoch to return. Maximum valid value is 25.
Return Type
- LearningCurveList|error - Learning Curve
ocrMultipart
function ocrMultipart(VisionOcrBody payload) returns OCRPredictResponse|error
Detect Text
Parameters
- payload VisionOcrBody - Detect OCR prediction payload.
Return Type
- OCRPredictResponse|error - OCR Result
getVisionPredict
function getVisionPredict(ImageClassificationRequest payload) returns ImageClassificationResponse|error
Get Language Prediction
Parameters
- payload ImageClassificationRequest - Image prediction payload.
Return Type
- ImageClassificationResponse|error - Prediction Result
retrainVisionDataset
function retrainVisionDataset(VisionRetrainBody payload) returns TrainResponse|error
Retrain a Vision Dataset
Parameters
- payload VisionRetrainBody - Retrain vision dataset payload.
Return Type
- TrainResponse|error - Training Status
trainVisionDataset
function trainVisionDataset(VisionTrainBody payload) returns TrainResponse|error
Train a Vision Dataset
Parameters
- payload VisionTrainBody - Train dataset payload
Return Type
- TrainResponse|error - Training Status
getVisionTrainStatusAndProgress
function getVisionTrainStatusAndProgress(string modelId) returns TrainResponse|error
Get Vision Training Status
Parameters
- modelId string - Model ID
Return Type
- TrainResponse|error - Training Status
Records
salesforce.einstein: ApiUsage
Fields
- endsAt string? -
- id string? -
- licenseId string? -
- 'object string? -
- organizationId string? -
- planData PlanData[]? -
- predictionsMax int? -
- predictionsUsed int? -
- startsAt string? -
salesforce.einstein: ApiUsageList
Fields
- data ApiUsage[]? -
- 'object string? -
salesforce.einstein: Attributes
Contains additional attributes related to the task parameter. If the task parameter is table, the row and column IDs for the detected text are returned. If the task parameter is contact, the detected entity tags will be returned.
Fields
- cellLocation CellLocation? - Location information
- language string? - Language of the key and value. Defaults to English. Only English is currently supported. Returned only when the task parameter value is form.
- pageNumber string? - Page that contains the identified text. The model always returns 1, except when you send in a multi-page PDF.
- tag string? - Entity that the model predicts for the detected text.
- value EntityObject? - Contains the detected text of the data that was entered in the form field. For example, in a driver's license, the value might be 09/13/1999 for issue date. Returned only when the task parameter value is form
salesforce.einstein: BoundingBox
Contains the coordinates for the bounding box that encloses the detected text.
Fields
- maxX int? - X-coordinate of the left side of the bounding box. The origin of the coordinate system is the top-left of the image. Number of pixels from the left edge of the image.
- maxY int? - Y-coordinate of the top of the bounding box. Number of pixels from the top edge of the image.
- minX int? - X-coordinate of the right side of the bounding box. Number of pixels from the left edge of the image.
- minY int? - Y-coordinate of the bottom of the bounding box. Number of pixels from the top edge of the image.
salesforce.einstein: CellLocation
Location information
Fields
- colIndex int? - Index of the column that contains the detected text.
- rowIndex int? - Index of the row that contains the detected text.
salesforce.einstein: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
salesforce.einstein: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
salesforce.einstein: Dataset
Fields
- available boolean? -
- createdAt string? - Date and time that the dataset was created.
- id int -
- labelSummary LabelSummary? - Contains the labels array that contains all the labels for the dataset.
- language string? - Dataset language.
- name string -
- numOfDuplicates int? - Number of duplicate images. This number includes duplicates in the .zip file from which the dataset was created plus the number of duplicate images from subsequent PUT calls to add images to the dataset.
- 'object string? - Object returned; in this case, dataset.
- statusMsg string? -
- totalExamples int? - Total number of examples in the dataset.
- totalLabels int? - Total number of labels in the dataset.
- 'type string? -
- updatedAt string? -
salesforce.einstein: DatasetidExamplesBody
Create dataset example payload
Fields
- data string? - Location of the local image file to upload.
- labelId int? - ID of the label to add to the example.
- name string? - Name of the example. Maximum length is 180 characters.
salesforce.einstein: DatasetidUploadBody
Dataset upload payload
Fields
- data string? - Path to the .csv, .tsv, or .json file on a local drive.
- 'type string? - URL of the .csv, .tsv, or .json file.
salesforce.einstein: DatasetidUploadBody1
Create dataset example payload
Fields
- data string? - Location of the local image file to upload.
- path string? - URL of the .zip file.
salesforce.einstein: DatasetList
Fields
- data Dataset[]? -
- 'object string? -
salesforce.einstein: DatasetsUploadBody
Create dataset payload
Fields
- data string? - Path to the .csv, .tsv, or .json file on the local drive (FilePart).
- name string? - Name of the dataset. Optional. If this parameter is omitted, the dataset name is derived from the file name.
- path string? - URL of the .csv, .tsv, or .json file.
- 'type string? - Type of dataset data.
salesforce.einstein: DatasetsUploadBody1
Create dataset payload
Fields
- data string? - Path to the .zip file on the local drive (FilePart).
- name string? - Name of the dataset. Optional. If this parameter is omitted, the dataset name is derived from the .zip file name.
- path string? - URL of the .zip file.
- 'type string? - Type of dataset data.
salesforce.einstein: DeletionResponse
Fields
- deletedObjectId string? -
- id string? -
- message string? -
- 'object string? -
- organizationId string? -
- progress decimal? -
- status string? -
- 'type string? -
salesforce.einstein: DetectionResult
label
Fields
- boundingBox BoundingBox? - Contains the coordinates for the bounding box that encloses the detected text.
- label string? - Probability lable for the input.
- probability float? - Probability value for the input. Values are between 0�1.
salesforce.einstein: EntityObject
Contains the detected text of the data that was entered in the form field. For example, in a driver's license, the value might be 09/13/1999 for issue date. Returned only when the task parameter value is form
Fields
- boundingBox BoundingBox? - Contains the coordinates for the bounding box that encloses the detected text.
- entity string? - Entity
- text string? - The data value for the specified key. For example, For example, in a driver's license, if key text is 4a iss, the value text might be 09/13/1999.
salesforce.einstein: Example
Fields
- createdAt string? - Date and time that the example was created.
- id int - ID of the example.
- label Label? - Contains information about the label with which the example is associated.
- location string? - URL of the image in the dataset. This is a temporary URL that expires in 30 minutes. This URL can be used to display images that were uploaded to a dataset in a UI.
- name string - Name of the example.
- 'object string? - Object returned; in this case, example.
salesforce.einstein: ExampleList
Fields
- data Example[]? -
- 'object string? -
salesforce.einstein: GenerateAccessTokenResponse
Fields
- access_token string? -
- expires_in string? -
- refresh_token string? -
- token_type string? -
salesforce.einstein: ImageClassificationRequest
Image prediction payload.
Fields
- modelId string - ID of the model that makes the prediction.
- numResults int? - Number of probabilities to return.
- sampleBase64Content string? - The image contained in a base64 string.
- sampleId string? - String that you can pass in to tag the prediction. Optional. Can be any value, and is returned in the response.
- sampleLocation string? - URL of the image file.
salesforce.einstein: ImageClassificationResponse
Fields
- 'object string? -
- probabilities LabelResult[]? -
- sampleId string? - Value passed in when the prediction call was made. Returned only if the sampleId request parameter is provided.
salesforce.einstein: IntentPredictRequest
Fields
- document string - Text for which you want to return an intent prediction.
- modelId string - ID of the model that makes the prediction. The model must have been created from a dataset with a type of text-sentiment.
- numResults int? - Number of probabilities to return.
- sampleId string? - String that you can pass in to tag the prediction. Optional. Can be any value, and is returned in the response.
salesforce.einstein: IntentPredictResponse
Fields
- 'object string? -
- probabilities LabelResult[]? -
- sampleId string? - Value passed in when the prediction call was made. Returned only if the sampleId request parameter is provided.
salesforce.einstein: Label
Contains information about the label with which the example is associated.
Fields
- datasetId int - ID of the dataset that the label belongs to.
- id int? - ID of the label.
- name string - Name of the label.
- numExamples int? - Number of examples that have the label.
salesforce.einstein: LabelResult
label
Fields
- label string? - Probability lable for the input.
- probability float? - Probability value for the input. Values are between 0�1.
salesforce.einstein: LabelSummary
Contains the labels array that contains all the labels for the dataset.
Fields
- labels Label[]? - Contains information about the label with which the example is associated.
salesforce.einstein: LanguageFeedbackBody
Create dataset feedback payload
Fields
- document string? - Intent or sentiment string to add to the dataset.
- expectedLabel string? - Correct label for the example. Must be a label that exists in the dataset.
- modelId string? - ID of the model that misclassified the image. The feedback example is added to the dataset associated with this model.
- name string? - Name of the example. Optional. Maximum length is 180 characters.
salesforce.einstein: LanguageRetrainBody
Retrain dataset payload
Fields
- algorithm string? - Algorithm used for train
- epochs int? - Number of training iterations for the neural network. Optional.
- learningRate float? - N/A for intent or sentiment models.
- modelId string? - ID of the model to be updated from the training.
- trainParams V2LanguageTrainParams? - JSON that contains parameters that specify how the model is created
salesforce.einstein: LanguageTrainBody
Train dataset payload
Fields
- algorithm string? - Algorithm used for train
- datasetId int? - ID of the dataset to train.
- epochs int? - Number of training iterations for the neural network. Optional.
- learningRate decimal? - N/A for intent or sentiment models.
- name string? - Name of the model. Maximum length is 180 characters.
- trainParams V2LanguageTrainParams? - JSON that contains parameters that specify how the model is created
salesforce.einstein: LearningCurve
Fields
- epoch record {}? - Epoch to which the metrics correspond.
- epochResults record {}? - Prediction results for the set of data used to test the model during training.
- metricsData record {}? - Model metrics values.
- 'object string? - Object returned; in this case, learningcurve.
salesforce.einstein: LearningCurveList
Fields
- data LearningCurve[]? -
- 'object string? -
salesforce.einstein: Metrics
Fields
- algorithm string? -
- createdAt string? - Date and time that the model was created.
- id string? - Model Id
- language string? -
- metricsData record {}? - Model metrics values.
- 'object string? -
salesforce.einstein: Model
Fields
- algorithm string? - Algorithm used to create the model. Returned only when the modelType is image-detection.
- createdAt string? - Date and time that the model was created.
- datasetId int - ID of the dataset trained to create the model.
- datasetVersionId int - Not available yet
- failureMsg string? - Reason the dataset training failed. Returned only if the training status is FAILED.
- language string? - Model language inherited from the dataset language. For image datasets, default is N/A. For text datasets, default is en_US.
- modelId string - ID of the model. Contains letters and numbers.
- modelType string? - Type of data from which the model was created.
- name string - Name of the model.
- 'object string? - Object returned; in this case, model.
- progress decimal - How far the dataset training has progressed. Values are between 0�1.
- status string - Status of the model.
- updatedAt string? - Date and time that the model was last updated.
salesforce.einstein: ModelList
Fields
- data Model[]? -
- 'object string? -
salesforce.einstein: Oauth2TokenBody
Generate token payload
Fields
- assertion string? - encrypted payload to identify yourself
- grant_type string? - specify the authentication method desired
- refresh_token string? - The refresh token you created previously.
- scope string? - set to
offline
to generate a refresh token
- valid_for int? - Number of seconds until the access token expires. Default is 60 seconds. Maximum value is 30 days
salesforce.einstein: ObjectDetectionRequest
Object detection payload
Fields
- modelId string - ID of the model that makes the detection.
- sampleBase64Content string? - The image contained in a base64 string.
- sampleId string? - String that you can pass in to tag the prediction. Optional. Can be any value, and is returned in the response.
- sampleLocation string? - URL of the image file.
salesforce.einstein: ObjectDetectionResponse
Fields
- 'object string? -
- probabilities DetectionResult[]? -
- sampleId string? - Value passed in when the prediction call was made. Returned only if the sampleId request parameter is provided.
salesforce.einstein: OCRPredictResponse
Fields
- 'object string? -
- probabilities OCRResult[]? -
- sampleId string? - Same value as request parameter. Returned only if the sampleId request parameter is provided.
- task string? - Same value as request parameter. Returns text if the request parameter isn't supplied.
salesforce.einstein: OCRResult
Array of probabilities for the prediction.
Fields
- attributes Attributes? - Contains additional attributes related to the task parameter. If the task parameter is table, the row and column IDs for the detected text are returned. If the task parameter is contact, the detected entity tags will be returned.
- boundingBox BoundingBox? - Contains the coordinates for the bounding box that encloses the detected text.
- label string? - Content of the detected text.
- probability float? - Probability value for the input. Values are between 0�1.
salesforce.einstein: PlanData
Fields
- amount int? -
- plan string? -
- 'source string? -
salesforce.einstein: PredictionErrorResponse
Fields
- message string? -
- 'object string? -
salesforce.einstein: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
salesforce.einstein: SentimentPredictRequest
Sentiment predict payload
Fields
- document string - Text for which you want to return a sentiment prediction.
- modelId string - ID of the model that makes the prediction. The model must have been created from a dataset with a type of text-sentiment.
- numResults int? - Number of probabilities to return.
- sampleId string? - String that you can pass in to tag the prediction. Optional. Can be any value, and is returned in the response.
salesforce.einstein: SentimentPredictResponse
Fields
- 'object string? -
- probabilities LabelResult[]? -
- sampleId string? - Value passed in when the prediction call was made. Returned only if the sampleId request parameter is provided.
salesforce.einstein: TrainResponse
Fields
- algorithm string? - Algorithm used to create the model. Returned only when the modelType is image-detection.
- createdAt string? - Date and time that the model was created.
- datasetId int - ID of the dataset trained to create the model.
- datasetVersionId int - Not available yet
- epochs int? - Number of epochs used during training.
- failureMsg string? - Reason the dataset training failed. Returned only if the training status is FAILED.
- language string - Model language inherited from the dataset language. For image datasets, default is N/A. For text datasets, default is en_US.
- learningRate decimal? - Learning rate used during training.
- modelId string - ID of the model. Contains letters and numbers.
- modelType string? - Type of data from which the model was created.
- name string - Name of the model.
- 'object string? - Object returned; in this case, training.
- progress decimal - How far the dataset training has progressed. Values are between 0�1.
- queuePosition int? - Where the training job is in the queue. This field appears in the response only if the status is QUEUED.
- status string - Status of the model.
- trainParams string? - Training parameters passed into the request.
- trainStats string? - Returns null when you train a dataset. Training statistics are returned when the status is SUCCEEDED or FAILED.
- updatedAt string? - Date and time that the model was last updated.
salesforce.einstein: UploadSyncBody
Create dataset payload
Fields
- data string? - Path to the .csv, .tsv, or .json file on the local drive (FilePart).
- name string? - Name of the dataset. Optional. If this parameter is omitted, the dataset name is derived from the file name.
- path string? - URL of the .csv, .tsv, or .json file.
- 'type string? - Type of dataset data.
salesforce.einstein: UploadSyncBody1
Create dataset payload
Fields
- data string? - Path to the .zip file on the local drive (FilePart).
- name string? - Name of the dataset. Optional. If this parameter is omitted, the dataset name is derived from the .zip file name.
- path string? - URL of the .zip file.
- 'type string? - Type of dataset data.
salesforce.einstein: V2LanguageTrainParams
JSON that contains parameters that specify how the model is created
Fields
- trainSplitRatio float? - Lets you specify the ratio of data used to train the dataset and the data used to test the model.
- withFeedback boolean? - Lets you specify that feedback examples are included in the data to be trained to create the model.
- withGlobalDatasetId int? - Lets you specify that a global dataset is used in addition to the specified dataset to create the model.
salesforce.einstein: V2VisionTrainParams
JSON that contains parameters that specify how the model is created
Fields
- trainSplitRatio float? - Lets you specify the ratio of data used to train the dataset and the data used to test the model.
- withFeedback boolean? - Lets you specify that feedback examples are included in the data to be trained to create the model.
- withGlobalDatasetId int? - Lets you specify that a global dataset is used in addition to the specified dataset to create the model.
salesforce.einstein: VisionBulkfeedbackBody
Create feedback payload.
Fields
- data string? - Local .zip file to upload. The maximum .zip file size you can upload from a local drive is 50 MB.
- modelId string? - ID of the model that misclassified the images. The feedback examples are added to the dataset associated with this model.
salesforce.einstein: VisionDatasetsBody
Create dataset payload
Fields
- labels string? - Optional comma-separated list of labels. If specified, creates the labels in the dataset. Maximum number of labels per dataset is 250.
- name string? - Name of the dataset. Maximum length is 180 characters.
- 'type string? - Type of dataset data
salesforce.einstein: VisionFeedbackBody
Create dataset feedback payload
Fields
- data string? - Local image file to upload.
- expectedLabel string? - Correct label for the example. Must be a label that exists in the dataset.
- modelId string? - ID of the model that misclassified the image. The feedback example is added to the dataset associated with this model.
- name string? - Name of the example. Optional. Maximum length is 180 characters.
salesforce.einstein: VisionOcrBody
Detect OCR prediction payload.
Fields
- modelId string? - ID of the model that makes the prediction. Valid values are OCRModel and tabulatev2.
- sampleContent string? - Binary content of image file uploaded as multipart/form-data. Optional.
- sampleId string? - String that you can pass in to tag the prediction. Optional. Can be any value, and is returned in the response.
- sampleLocation string? - URL of the image file. Use this parameter when sending in a file from a web location. Optional.
- task string? - Optional. Designates the type of data in the image. Default is text. Valid values: contact, table, and text.
salesforce.einstein: VisionRetrainBody
Retrain dataset payload
Fields
- algorithm string? - Specifies the algorithm used to train the dataset. Optional. Use this parameter only when training a dataset with a type of image-detection. Valid values are object-detection-v1 and retail-execution.
- epochs int? - Number of training iterations for the neural network. Optional.
- learningRate float? - Specifies how much the gradient affects the optimization of the model at each time step. Optional.
- modelId string? - ID of the model to be updated from the training.
- trainParams V2VisionTrainParams? - JSON that contains parameters that specify how the model is created
salesforce.einstein: VisionTrainBody
Train dataset payload
Fields
- algorithm string? - Specifies the algorithm used to train the dataset. Optional. Use this parameter only when training a dataset with a type of image-detection. Valid values are object-detection-v1 and retail-execution.
- datasetId int? - ID of the dataset to train.
- epochs int? - Number of training iterations for the neural network. Optional.
- learningRate decimal? - Specifies how much the gradient affects the optimization of the model at each time step. Optional.
- name string? - Name of the model. Maximum length is 180 characters.
- trainParams V2VisionTrainParams? - JSON that contains parameters that specify how the model is created
Import
import ballerinax/salesforce.einstein;
Metadata
Released date: about 2 years ago
Version: 1.3.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 9
Current verison: 7
Weekly downloads
Keywords
Business Intelligence/Analytics
Cost/Freemium
Contributors