azure_cosmosdb
Module azure_cosmosdb
API
Declarations
Definitions
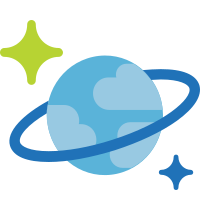
ballerinax/azure_cosmosdb Ballerina library
Overview
It provides the capability to connect to Azure Cosmos DB and execute CRUD (Create, Read, Update, and Delete) operations for databases and containers, to execute SQL queries to query containers, etc. In addition, it allows the special features provided by Cosmos DB such as operations on JavaScript language-integrated queries, management of users and permissions, etc.
This module supports Azure Cosmos DB(SQL) API version 2018-12-31
.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Microsoft Account with Azure Subscription
- Create an Azure Cosmos DB account
- Obtain tokens
- Go to your Azure Cosmos DB account and click Keys.
- Copy the URI and PRIMARY KEY in the Read-write Keys tab.
Quickstart
To use the Azure Cosmos DB connector in your Ballerina application, update the .bal file as follows:
Step 1 - Import connector
Import the ballerinax/azure_cosmosdb
module into the Ballerina project.
import ballerinax/azure_cosmosdb as cosmosdb;
Step 2 - Create a new connector instance
You can now add the connection configuration with the Master-Token
or Resource-Token
, and the resource URI to the
Cosmos DB Account.
cosmosdb:ConnectionConfig configuration = { baseUrl: <URI>, primaryKeyOrResourceToken: <PRIMARY_KEY> }; cosmosdb:DataPlaneClient azureCosmosClient = check new (configuration);
Step 3 - Invoke connector operation
-
Create a document
Once you follow the above steps. you can create a new document inside the Cosmos container as shown below. Cosmos DB is designed to store and query JSON-like documents. Therefore, the document you create must be of theJSON
type. It also needs a unique ID to identify it.. In this example, the document ID ismy_document
record {|string id; json...;|} document = { id: "my_document", "FirstName": "Alan", "FamilyName": "Turing", "Parents": [{ "FamilyName": "Turing", "FirstName": "Julius" }, { "FamilyName": "Stoney", "FirstName": "Ethel" }], "gender": 0 }; int valueOfPartitionKey = 0; cosmosdb:Document documentResult = azureCosmosClient-> createDocument("my_database", "my_container", document, valueOfPartitionKey);
Note:
- This document is created inside an already existing container with ID my_container and the container was created inside a database with ID my_document.
- As this container have selected path /gender as the partition key path. The document you create should include that path with a valid value.
- The new document to create is represented as
record {|string id; json...;|}
. The json... represent key-value pairs where the values are of typeboolean, int, float, decimal, string, json[], or map<json>
- Use
bal run
command to compile and run the Ballerina program
Clients
azure_cosmosdb: DataPlaneClient
This is the Azure Cosmos DB SQL API, a fast NoSQL database servic offers rich querying over diverse data, helps deliver configurable and reliable performance, is globally distributed, and enables rapid development.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create an Azure Cosmos DB account
and obtain tokens following this guide.
init (ConnectionConfig cosmosdbConfig, ClientConfiguration httpClientConfig)
- cosmosdbConfig ConnectionConfig - Configurations required to initialize the
Client
endpoint
- httpClientConfig ClientConfiguration {} - HTTP configuration
createDocument
function createDocument(string databaseId, string containerId, record { id string, json... } document, int|float|decimal|string partitionKey, DocumentCreateOptions? documentCreateOptions) returns Document|Error
Creates a document.
Parameters
- databaseId string - ID of the database to which the container belongs to
- containerId string - ID of the container where, document is created
- document record { id string, json... } - A JSON document to be saved in the database
- documentCreateOptions DocumentCreateOptions? (default ()) - The
cosmos_db:DocumentCreateOptions
which can be used to add additional capabilities to the request
Return Type
replaceDocument
function replaceDocument(string databaseId, string containerId, record { id string, json... } document, int|float|decimal|string partitionKey, DocumentReplaceOptions? documentReplaceOptions) returns Document|Error
Replaces a document.
Parameters
- databaseId string - ID of the database to which the container belongs to
- containerId string - ID of the container which contains the existing document
- document record { id string, json... } - A JSON document which will replace the existing document
- documentReplaceOptions DocumentReplaceOptions? (default ()) - The
cosmos_db:DocumentReplaceOptions
which can be used to add additional capabilities to the request
Return Type
getDocument
function getDocument(string databaseId, string containerId, string documentId, int|float|decimal|string partitionKey, ResourceReadOptions? resourceReadOptions) returns Document|Error
Gets information about a document.
Parameters
- databaseId string - ID of the database to which the container belongs to
- containerId string - ID of the container which contains the document
- documentId string - ID of the document
- resourceReadOptions ResourceReadOptions? (default ()) - The
cosmos_db:ResourceReadOptions
which can be used to add additional capabilities to the request
Return Type
getDocumentList
function getDocumentList(string databaseId, string containerId, DocumentListOptions? documentListOptions) returns stream<Document, error?>|Error
Lists information of all the documents .
Parameters
- databaseId string - ID of the database to which the container belongs to
- containerId string - ID of the container which contains the document
- documentListOptions DocumentListOptions? (default ()) - The
cosmos_db:DocumentListOptions
which can be used to add additional capabilities to the request
Return Type
deleteDocument
function deleteDocument(string databaseId, string containerId, string documentId, int|float|decimal|string partitionKey, ResourceDeleteOptions? resourceDeleteOptions) returns DeleteResponse|Error
Deletes a document.
Parameters
- databaseId string - ID of the database to which the container belongs to
- containerId string - ID of the container which contains the document
- documentId string - ID of the document
- resourceDeleteOptions ResourceDeleteOptions? (default ()) - The
cosmos_db:ResourceDeleteOptions
which can be used to add additional capabilities to the request
Return Type
- DeleteResponse|Error - If successful, returns
cosmos_db:DeleteResponse
. Else returnscosmos_db:Error
.
queryDocuments
function queryDocuments(string databaseId, string containerId, string sqlQuery, ResourceQueryOptions? resourceQueryOptions) returns stream<Document, error?>|Error
Queries documents.
Parameters
- databaseId string - ID of the database to which the container belongs to
- containerId string - ID of the container to query
- sqlQuery string - A string containing the SQL query
- resourceQueryOptions ResourceQueryOptions? (default ()) - The
cosmos_db:ResourceQueryOptions
which can be used to add additional capabilities to the request
Return Type
createStoredProcedure
function createStoredProcedure(string databaseId, string containerId, string storedProcedureId, string storedProcedure) returns StoredProcedure|Error
Creates a new stored procedure.
Parameters
- databaseId string - ID of the database to which the container belongs to
- containerId string - ID of the container where, stored procedure will be created
- storedProcedureId string - A unique ID for the newly created stored procedure
- storedProcedure string - A JavaScript function represented as a string
Return Type
- StoredProcedure|Error - If successful, returns a
cosmos_db:StoredProcedure
. Else returnscosmos_db:Error
.
replaceStoredProcedure
function replaceStoredProcedure(string databaseId, string containerId, string storedProcedureId, string storedProcedure) returns StoredProcedure|Error
Replaces a stored procedure with new one.
Parameters
- databaseId string - ID of the database to which the container belongs to
- containerId string - ID of the container which contains the existing stored procedures
- storedProcedureId string - The ID of the stored procedure to be replaced
- storedProcedure string - A JavaScript function which will replace the existing one
Return Type
- StoredProcedure|Error - If successful, returns a
cosmos_db:StoredProcedure
. Else returnscosmos_db:Error
.
listStoredProcedures
function listStoredProcedures(string databaseId, string containerId, ResourceReadOptions? resourceReadOptions) returns stream<StoredProcedure, error?>|Error
Lists information of all stored procedures.
Parameters
- databaseId string - ID of the database to which the container belongs to
- containerId string - ID of the container which contains the stored procedures
- resourceReadOptions ResourceReadOptions? (default ()) - The
cosmos_db:ResourceReadOptions
which can be used to add additional capabilities to the request
Return Type
- stream<StoredProcedure, error?>|Error - If successful, returns a
stream<cosmos_db:StoredProcedure, error>
. Else returnscosmos_db:Error
.
deleteStoredProcedure
function deleteStoredProcedure(string databaseId, string containerId, string storedProcedureId, ResourceDeleteOptions? resourceDeleteOptions) returns DeleteResponse|Error
Deletes a stored procedure.
Parameters
- databaseId string - ID of the database to which the container belongs to
- containerId string - ID of the container which contains the stored procedure
- storedProcedureId string - ID of the stored procedure to delete
- resourceDeleteOptions ResourceDeleteOptions? (default ()) - The
cosmos_db:ResourceDeleteOptions
which can be used to add additional capabilities to the request
Return Type
- DeleteResponse|Error - If successful, returns
cosmos_db:DeleteResponse
. Else returnscosmos_db:Error
.
executeStoredProcedure
function executeStoredProcedure(string databaseId, string containerId, string storedProcedureId, StoredProcedureExecuteOptions storedProcedureExecuteOptions) returns json|Error
Executes a stored procedure.
Parameters
- databaseId string - ID of the database to which the container belongs to
- containerId string - ID of the container which contains the stored procedure
- storedProcedureId string - ID of the stored procedure to execute
- storedProcedureExecuteOptions StoredProcedureExecuteOptions - A record
cosmos_db:StoredProcedureExecuteOptions
to specify the additional parameters
Return Type
- json|Error - If successful, returns
json
with the output from the executed function. Else returnscosmos_db:Error
.
azure_cosmosdb: ManagementClient
This is the Azure Cosmos DB SQL API, a fast NoSQL database servic offers rich querying over diverse data, helps deliver configurable and reliable performance, is globally distributed, and enables rapid development.
Constructor
Gets invoked to initialize the connector
.
The HTTP client initialization requires setting the API credentials.
Create an Azure Cosmos DB account
and obtain tokens following this guide.
init (ConnectionConfig azureConfig, ClientConfiguration httpClientConfig)
- azureConfig ConnectionConfig -
- httpClientConfig ClientConfiguration {} - HTTP configuration
createDatabase
function createDatabase(string databaseId, (int|record {| maxThroughput int |})? throughputOption) returns Database|Error
Creates a database.
Parameters
- databaseId string - ID of the new database. Must be a unique value.
Return Type
createDatabaseIfNotExist
function createDatabaseIfNotExist(string databaseId, (int|record {| maxThroughput int |})? throughputOption) returns Database?|Error
Creates a database only if the specified database ID does not exist already.
Parameters
- databaseId string - ID of the new database. Must be a unique value.
Return Type
getDatabase
function getDatabase(string databaseId, ResourceReadOptions? resourceReadOptions) returns Database|Error
Gets information of a given database.
Parameters
- databaseId string - ID of the database
- resourceReadOptions ResourceReadOptions? (default ()) - The
cosmos_db:ResourceReadOptions
which can be used to add additional capabilities to the request
Return Type
listDatabases
Lists information of all databases.
Return Type
deleteDatabase
function deleteDatabase(string databaseId, ResourceDeleteOptions? resourceDeleteOptions) returns DeleteResponse|Error
Deletes a given database.
Parameters
- databaseId string - ID of the database to delete
- resourceDeleteOptions ResourceDeleteOptions? (default ()) - The
cosmos_db:ResourceDeleteOptions
which can be used to add additional capabilities to the request
Return Type
- DeleteResponse|Error - If successful, returns
cosmos_db:DeleteResponse
. Else returnscosmos_db:Error
.
createContainer
function createContainer(string databaseId, string containerId, PartitionKey partitionKey, IndexingPolicy? indexingPolicy, (int|record {| maxThroughput int |})? throughputOption) returns Container|Error
Creates a container inside the given database.
Parameters
- databaseId string - ID of the database to which the container belongs to
- containerId string - ID of the new container. Must be a unique value.
- partitionKey PartitionKey - A record
cosmos_db:PartitionKey
- indexingPolicy IndexingPolicy? (default ()) - A record
cosmos_db:IndexingPolicy
.
Return Type
createContainerIfNotExist
function createContainerIfNotExist(string databaseId, string containerId, PartitionKey partitionKey, IndexingPolicy? indexingPolicy, (int|record {| maxThroughput int |})? throughputOption) returns Container?|Error
Creates a container only if the specified container ID does not exist already.
Parameters
- databaseId string - ID of the database to which the container belongs to
- containerId string - ID of the new container
- partitionKey PartitionKey - A record of
cosmos_db:PartitionKey
- indexingPolicy IndexingPolicy? (default ()) - A record of
cosmos_db:IndexingPolicy
.
Return Type
getContainer
function getContainer(string databaseId, string containerId, ResourceReadOptions? resourceReadOptions) returns Container|Error
Gets information about a container.
Parameters
- databaseId string - ID of the database to which the container belongs to
- containerId string - ID of the container
- resourceReadOptions ResourceReadOptions? (default ()) - The
cosmos_db:ResourceReadOptions
which can be used to add additional capabilities to the request
Return Type
listContainers
Lists information of all containers.
Parameters
- databaseId string - ID of the database to which the containers belongs to
Return Type
deleteContainer
function deleteContainer(string databaseId, string containerId, ResourceDeleteOptions? resourceDeleteOptions) returns DeleteResponse|Error
Deletes a container.
Parameters
- databaseId string - ID of the database to which the container belongs to
- containerId string - ID of the container to delete
- resourceDeleteOptions ResourceDeleteOptions? (default ()) - The
cosmos_db:ResourceDeleteOptions
which can be used to add additional capabilities to the request
Return Type
- DeleteResponse|Error - If successful, returns
cosmos_db:DeleteResponse
. Else returnscosmos_db:Error
.
listPartitionKeyRanges
function listPartitionKeyRanges(string databaseId, string containerId) returns stream<PartitionKeyRange, error?>|Error
Retrieves a list of partition key ranges for the container.
Parameters
- databaseId string - ID of the database to which the container belongs to
- containerId string - ID of the container where the partition key ranges are related to
Return Type
- stream<PartitionKeyRange, error?>|Error - If successful, returns
stream<cosmos_db:PartitionKeyRange, error>
. Else returnscosmos_db:Error
.
createUserDefinedFunction
function createUserDefinedFunction(string databaseId, string containerId, string userDefinedFunctionId, string userDefinedFunction) returns UserDefinedFunction|Error
Creates a new User Defined Function.
Parameters
- databaseId string - ID of the database to which the container belongs to
- containerId string - ID of the container where, User Defined Function is created
- userDefinedFunctionId string - A unique ID for the newly created User Defined Function
- userDefinedFunction string - A JavaScript function represented as a string
Return Type
- UserDefinedFunction|Error - If successful, returns a
cosmos_db:UserDefinedFunction
. Else returnscosmos_db:Error
.
replaceUserDefinedFunction
function replaceUserDefinedFunction(string databaseId, string containerId, string userDefinedFunctionId, string userDefinedFunction) returns UserDefinedFunction|Error
Replaces an existing User Defined Function.
Parameters
- databaseId string - ID of the database to which the container belongs to
- containerId string - ID of the container which contains the existing User Defined Function
- userDefinedFunctionId string - The ID of the User Defined Function to replace
- userDefinedFunction string - A JavaScript function represented as a string
Return Type
- UserDefinedFunction|Error - If successful, returns a
cosmos_db:UserDefinedFunction
. Else returnscosmos_db:Error
.
listUserDefinedFunctions
function listUserDefinedFunctions(string databaseId, string containerId, ResourceReadOptions? resourceReadOptions) returns stream<UserDefinedFunction, error?>|Error
Gets a list of existing user defined functions.
Parameters
- databaseId string - ID of the database to which the container belongs to
- containerId string - ID of the container which contains the user defined functions
- resourceReadOptions ResourceReadOptions? (default ()) - The
cosmos_db:ResourceReadOptions
which can be used to add additional capabilities to the request
Return Type
- stream<UserDefinedFunction, error?>|Error - If successful, returns a
stream<cosmos_db:UserDefinedFunction, error>
. Else returnscosmos_db:Error
.
deleteUserDefinedFunction
function deleteUserDefinedFunction(string databaseId, string containerId, string userDefinedFunctionid, ResourceDeleteOptions? resourceDeleteOptions) returns DeleteResponse|Error
Deletes an existing User Defined Function.
Parameters
- databaseId string - ID of the database to which the container belongs to
- containerId string - ID of the container which contains the User Defined Function
- userDefinedFunctionid string - ID of UDF to delete
- resourceDeleteOptions ResourceDeleteOptions? (default ()) - The
cosmos_db:ResourceDeleteOptions
which can be used to add additional capabilities to the request
Return Type
- DeleteResponse|Error - If successful, returns
cosmos_db:DeleteResponse
. Else returnscosmos_db:Error
.
createTrigger
function createTrigger(string databaseId, string containerId, string triggerId, string trigger, TriggerOperation triggerOperation, TriggerType triggerType) returns Trigger|Error
Creates a trigger.
Parameters
- databaseId string - ID of the database to which the container belongs to
- containerId string - ID of the container where trigger is created
- triggerId string - A unique ID for the newly created trigger
- trigger string - A JavaScript function represented as a string
- triggerOperation TriggerOperation - The specific operation in which trigger will be executed can be
All
,Create
,Replace
orDelete
- triggerType TriggerType - The instance in which trigger will be executed
Pre
orPost
Return Type
replaceTrigger
function replaceTrigger(string databaseId, string containerId, string triggerId, string trigger, TriggerOperation triggerOperation, TriggerType triggerType) returns Trigger|Error
Replaces an existing trigger.
Parameters
- databaseId string - ID of the database to which the container belongs to
- containerId string - ID of the container which contains the trigger
- triggerId string - The ID of the trigger to be replaced
- trigger string - A JavaScript function represented as a string
- triggerOperation TriggerOperation - The specific operation in which trigger will be executed
- triggerType TriggerType - The instance in which trigger will be executed
Pre
orPost
Return Type
listTriggers
function listTriggers(string databaseId, string containerId, ResourceReadOptions? resourceReadOptions) returns stream<Trigger, error?>|Error
Lists existing triggers.
Parameters
- databaseId string - ID of the database to which the container belongs to
- containerId string - ID of the container which contains the triggers
- resourceReadOptions ResourceReadOptions? (default ()) - The
cosmos_db:ResourceReadOptions
which can be used to add additional capabilities to the request
Return Type
deleteTrigger
function deleteTrigger(string databaseId, string containerId, string triggerId, ResourceDeleteOptions? resourceDeleteOptions) returns DeleteResponse|Error
Deletes an existing trigger.
Parameters
- databaseId string - ID of the database to which the container belongs to
- containerId string - ID of the container which contains the trigger
- triggerId string - ID of the trigger to be deleted
- resourceDeleteOptions ResourceDeleteOptions? (default ()) - The
cosmos_db:ResourceDeleteOptions
which can be used to add additional capabilities to the request
Return Type
- DeleteResponse|Error - If successful, returns
cosmos_db:DeleteResponse
. Else returnscosmos_db:Error
.
createUser
Creates a user for a given database.
Parameters
- databaseId string - ID of the database where the user is created.
- userId string - ID of the new user. Must be a unique value.
replaceUserId
Replaces the ID of an existing user.
Parameters
- databaseId string - ID of the database to which, the existing user belongs to
- userId string - Old ID of the user
- newUserId string - New ID for the user
getUser
function getUser(string databaseId, string userId, ResourceReadOptions? resourceReadOptions) returns User|Error
Gets information of a user.
Parameters
- databaseId string - ID of the database to which, the user belongs to
- userId string - ID of user
- resourceReadOptions ResourceReadOptions? (default ()) - The
cosmos_db:ResourceReadOptions
which can be used to add additional capabilities to the request
listUsers
function listUsers(string databaseId, ResourceReadOptions? resourceReadOptions) returns stream<User, error?>|Error
Lists users of a specific database.
Parameters
- databaseId string - ID of the database to which, the user belongs to
- resourceReadOptions ResourceReadOptions? (default ()) - The
cosmos_db:ResourceReadOptions
which can be used to add additional capabilities to the request
Return Type
deleteUser
function deleteUser(string databaseId, string userId, ResourceDeleteOptions? resourceDeleteOptions) returns DeleteResponse|Error
Deletes a user.
Parameters
- databaseId string - ID of the database to which, the user belongs to
- userId string - ID of the user to delete
- resourceDeleteOptions ResourceDeleteOptions? (default ()) - The
cosmos_db:ResourceDeleteOptions
which can be used to add additional capabilities to the request
Return Type
- DeleteResponse|Error - If successful, returns
cosmos_db:DeleteResponse
. Else returnscosmos_db:Error
.
createPermission
function createPermission(string databaseId, string userId, string permissionId, PermisssionMode permissionMode, string resourcePath, int? validityPeriodInSeconds) returns Permission|Error
Creates a permission for a user.
Parameters
- databaseId string - ID of the database to which, the user belongs to
- userId string - ID of user to which, the permission is granted. Must be a unique value.
- permissionId string - A unique ID for the newly created permission
- permissionMode PermisssionMode - The mode to which the permission is scoped
- resourcePath string - The resource this permission is allowing the user to access
- validityPeriodInSeconds int? (default ()) - Validity period of the permission in seconds.
Return Type
- Permission|Error - If successful, returns a
cosmos_db:Permission
. Else returnscosmos_db:Error
.
replacePermission
function replacePermission(string databaseId, string userId, string permissionId, PermisssionMode permissionMode, string resourcePath, int? validityPeriodInSeconds) returns Permission|Error
Replaces an existing permission.
Parameters
- databaseId string - ID of the database where the user is created
- userId string - ID of user to which, the permission is granted
- permissionId string - The ID of the permission to be replaced
- permissionMode PermisssionMode - The mode to which the permission is scoped
- resourcePath string - The resource this permission is allowing the user to access
- validityPeriodInSeconds int? (default ()) - Validity period of the permission in seconds.
Return Type
- Permission|Error - If successful, returns a
cosmos_db:Permission
. Else returnscosmos_db:Error
.
getPermission
function getPermission(string databaseId, string userId, string permissionId, ResourceReadOptions? resourceReadOptions) returns Permission|Error
Gets information of a permission.
Parameters
- databaseId string - ID of the database where the user is created
- userId string - ID of user to which, the permission is granted
- permissionId string - ID of the permission
- resourceReadOptions ResourceReadOptions? (default ()) - The
cosmos_db:ResourceReadOptions
which can be used to add additional capabilities to the request
Return Type
- Permission|Error - If successful, returns a
cosmos_db:Permission
. Else returnscosmos_db:Error
.
listPermissions
function listPermissions(string databaseId, string userId, ResourceReadOptions? resourceReadOptions) returns stream<Permission, error?>|Error
Lists permissions belong to a user.
Parameters
- databaseId string - ID of the database where the user is created
- userId string - ID of user to which, the permissions are granted
- resourceReadOptions ResourceReadOptions? (default ()) - The
cosmos_db:ResourceReadOptions
which can be used to add additional capabilities to the request
Return Type
- stream<Permission, error?>|Error - If successful, returns a
stream<cosmos_db:Permission, error>
. Else returnscosmos_db:Error
.
deletePermission
function deletePermission(string databaseId, string userId, string permissionId, ResourceDeleteOptions? resourceDeleteOptions) returns DeleteResponse|Error
Deletes a permission belongs to a user.
Parameters
- databaseId string - ID of the database where the user is created
- userId string - ID of user to which, the permission is granted
- permissionId string - ID of the permission to delete
- resourceDeleteOptions ResourceDeleteOptions? (default ()) - The
cosmos_db:ResourceDeleteOptions
which can be used to add additional capabilities to the request
Return Type
- DeleteResponse|Error - If successful, returns
cosmos_db:DeleteResponse
. Else returnscosmos_db:Error
.
replaceOffer
Replaces an existing offer.
Parameters
- offer Offer - A record
cosmos_db:Offer
getOffer
function getOffer(string offerId, ResourceReadOptions? resourceReadOptions) returns Offer|Error
Gets information about an offer.
Parameters
- offerId string - The ID of the offer
- resourceReadOptions ResourceReadOptions? (default ()) - The
cosmos_db:ResourceReadOptions
which can be used to add additional capabilities to the request
listOffers
function listOffers(ResourceReadOptions? resourceReadOptions) returns stream<Offer, error?>|Error
Lists information of offers.
Parameters
- resourceReadOptions ResourceReadOptions? (default ()) - The
cosmos_db:ResourceReadOptions
which can be used to add additional capabilities to the request
Return Type
queryOffer
function queryOffer(string sqlQuery, ResourceQueryOptions? resourceQueryOptions) returns stream<Offer, error?>|Error
Performs queries on offer resources.
Parameters
- sqlQuery string - A string value containing SQL query
- resourceQueryOptions ResourceQueryOptions? (default ()) - The
cosmos_db:ResourceQueryOptions
which can be used to add additional capabilities to the request
Constants
Enums
azure_cosmosdb: ChangeFeedOption
Incremental changes to documents within the collection.
Members
azure_cosmosdb: ConsistencyLevel
The Consistency Level Override for document create and update.
Members
azure_cosmosdb: IndexDataType
Datatype for which the indexing behavior is applied to.
Members
azure_cosmosdb: IndexingDirective
Whether to include or exclude the document in indexing.
Members
azure_cosmosdb: IndexingMode
Mode of indexing for the container.
Members
azure_cosmosdb: IndexType
Type of an Index.
Members
azure_cosmosdb: OfferType
Performance levels for a specific throughput level.
They depend on the Cosmos DB region which the container
belongs to and partitioning nature of the container (ie: single partitioned or multiple partitioned).
Members
Invalid
for V2
, user-defined throughput levelsazure_cosmosdb: OfferVersion
Specific version for a given offer.
Members
azure_cosmosdb: PermisssionMode
Access mode for the resource.
Members
azure_cosmosdb: TriggerOperation
Type of operation that invokes the trigger.
Members
azure_cosmosdb: TriggerType
When the trigger is fired.
Members
Records
azure_cosmosdb: Commons
Common elements representing information.
Fields
- resourceId string? - A unique identifier which is used internally for placement and navigation of the resource
- selfReference string? - A unique addressable URI for the resource
- eTag string? - Resource etag for the resource retrieved
- sessionToken string? - Session token of the request
azure_cosmosdb: ConnectionConfig
Configuration parameters to create Azure Cosmos DB client.
Fields
- baseUrl string - Base URL of the Azure Cosmos DB account
- primaryKeyOrResourceToken string - The token used to make the request call authorized
azure_cosmosdb: Container
Parameters representing information about a container.
Fields
- id string - User generated unique ID for the container
- Fields Included from *Commons
- indexingPolicy IndexingPolicy - Record of type
IndexingPolicy
- partitionKey PartitionKey - Record of type
PartitionKey
azure_cosmosdb: Database
Parameters representing information about a database.
Fields
- id string - User generated unique ID for the database
- Fields Included from *Commons
azure_cosmosdb: DeleteResponse
Metadata headers which will return for a delete request.
Fields
- sessionToken string - Session token from the response
azure_cosmosdb: Document
Parameters representing information about a document.
Fields
- id string - User generated unique ID for the document
- Fields Included from *Commons
- documentBody map<json> - The document reprsented as a map of json
azure_cosmosdb: DocumentCreateOptions
Optional parameters which can be passed to the function when creating a document.
Fields
- indexingDirective IndexingDirective? - The option whether to include the document in the index
- Allowed values are
Include
orExclude
.
- Allowed values are
- isUpsertRequest boolean(default false) - A boolean value which specify whether the request is an upsert request
azure_cosmosdb: DocumentListOptions
Optional parameters which can be passed to the function when listing information about the documents.
Fields
- consistancyLevel ConsistencyLevel? - The consistency level override
- Allowed values are
Strong
,Bounded
,Session
orEventual
. - The override must be the same or weaker than the Cosmos DB account’s configured consistency level.
- Allowed values are
- sessionToken string? - Echo the latest read value of
session token header
to acquire session level consistency
- changeFeedOption ChangeFeedOption? - Must be set to
Incremental feed
or omitted otherwise
- partitionKeyRangeId string? - The partition key range ID for reading data
azure_cosmosdb: DocumentReplaceOptions
Optional parameters which can be passed to the function when replacing a document.
Fields
- indexingDirective IndexingDirective? - The option whether to include the document in the index
- Allowed values are 'Include' or 'Exclude'.
azure_cosmosdb: ExcludedPath
Parameters representing excluded path type.
Fields
- path string - Path that is excluded from indexing
azure_cosmosdb: HttpDetail
Extra HTTP status code detail of an error.
Fields
- status int - The HTTP status code of the error
azure_cosmosdb: IncludedPath
Parameters representing included path type.
Fields
- path string - Path to which the indexing behavior applies to
- indexes Index[](default []) - Array of type
Index
, representing index values
azure_cosmosdb: Index
Parameters representing an index.
Fields
- kind IndexType(default HASH) - Type of index
- Can be
Hash
,Range
orSpatial
.
- Can be
- dataType IndexDataType(default STRING) - Datatype for which the indexing behavior is applied to
- Can be
String
,Number
,Point
,Polygon
orLineString
.
- Can be
- precision int(default MAX_PRECISION) - Precision of the index
- Can be either set to -1 for maximum precision or between 1-8 for
Number
, and 1-100 forString
- Not applicable for
Point
,Polygon
, andLineString
data types. Default is -1.
- Can be either set to -1 for maximum precision or between 1-8 for
azure_cosmosdb: IndexingPolicy
Parameters necessary to create an indexing policy when creating a container.
Fields
- indexingMode IndexingMode? - Mode of indexing. Can be
consistent
ornone
- automatic boolean(default true) - Specify whether indexing is done automatically or not
- Must be
true
if indexing must be automatic andfalse
otherwise.
- Must be
- includedPaths IncludedPath[]? - Array of type
IncludedPath
representing included paths
- excludedPaths ExcludedPath[]? - Array of type
IncludedPath
representing excluded paths
azure_cosmosdb: Offer
Parameters representing an offer.
Fields
- id string - User generated unique ID for the offer
- Fields Included from *Commons
- offerVersion OfferVersion - Offer version
- This value can be
V1
for pre-defined throughput levels andV2
for user-defined throughput levels
- This value can be
- offerType OfferType - Performance level for V1 offer version, allows
S1
,S2
andS3
.
- content map<json> - Information about the offer
- For
V2
offers, it contains the throughput of the collection.
- For
- resourceResourceId string - The resource id(_rid) of the collection
- resourceSelfLink string - The self-link(_self) of the collection
azure_cosmosdb: PartitionKey
Parameters representing a partition key.
Fields
- paths string[](default []) - Array of paths using which, data within the collection can be partitioned. The array must contain only a single value.
- kindreadonly string(default PARTITIONING_ALGORITHM_TYPE_HASH) - Algorithm used for partitioning
- Only Hash is supported.
- keyVersion PartitionKeyVersion(default PARTITION_KEY_VERSION_1) - Version of partition key. Default is 1. To use a large partition key, set the version to 2.
azure_cosmosdb: PartitionKeyRange
Parameters representing a partition key range.
Fields
- id string - ID for the partition key range
- Fields Included from *Commons
- minInclusive string - Minimum partition key hash value for the partition key range
- maxExclusive string - Maximum partition key hash value for the partition key range
azure_cosmosdb: Permission
Parameters representing a permission.
Fields
- id string - User generated unique ID for the permission
- Fields Included from *Commons
- permissionMode PermisssionMode - Access mode for the resource, Should be
All
orRead
- resourcePath string - Full addressable path of the resource associated with the permission
- token string - System generated
Resource-Token
for the particular resource and user
azure_cosmosdb: ResourceDeleteOptions
Optional parameters which can be passed to the function when deleting other resources in Cosmos DB.
Fields
- sessionToken string? - Echo the latest read value of
sessionToken
to acquire session level consistency
azure_cosmosdb: ResourceQueryOptions
Optional parameters which can be passed to the function when querying containers.
Fields
- consistancyLevel ConsistencyLevel? - The consistency level override
- Allowed values are
Strong
,Bounded
,Session
orEventual
. - The override must be the same or weaker than the account’s configured consistency level.
- Allowed values are
- sessionToken string? - Echo the latest read value of
sessionToken
to acquire session level consistency
- enableCrossPartition boolean(default true) - Boolean value specifying whether to allow cross partitioning <br/> Default is
true
where, it allows to query across all logical partitions.
azure_cosmosdb: ResourceReadOptions
Optional parameters which can be passed to the function when reading the information about other resources in Cosmos DB such as Containers, StoredProcedures, Triggers, User Defined Functions, etc.
Fields
- consistancyLevel ConsistencyLevel? - The consistency level override
- Allowed values are
Strong
,Bounded
,Session
orEventual
. - The override must be the same or weaker than the account’s configured consistency level.
- Allowed values are
- sessionToken string? - Echo the latest read value of
sessionToken
to acquire session level consistency
azure_cosmosdb: StoredProcedure
Parameters representing a stored procedure.
Fields
- id string - User generated unique ID for the stored procedure
- Fields Included from *Commons
- storedProcedure string - A JavaScript function, respresented as a string
azure_cosmosdb: StoredProcedureExecuteOptions
The options which can be passed for execution of stored procedures.
Fields
- parameters string[](default []) - An array of parameters which has values match the function parameters of a stored procedure
azure_cosmosdb: Trigger
Parameters representing a trigger.
Fields
- id string - User generated unique ID for the trigger
- Fields Included from *Commons
- triggerFunction string - A JavaScript function, respresented as a string
- triggerOperation TriggerOperation(default ALL) - Type of operation that invokes the trigger
- Can be
All
,Create
,Replace
orDelete
.
- Can be
- triggerType TriggerType(default PRE) - When the trigger is fired,
Pre
orPost
azure_cosmosdb: User
Parameters representing a user.
Fields
- id string - User generated unique ID for the user
- Fields Included from *Commons
- permissions string - A system generated property that specifies the addressable path of the permissions resource
azure_cosmosdb: UserDefinedFunction
Parameters representing a user defined function.
Fields
- id string - User generated unique ID for the user defined function
- Fields Included from *Commons
- userDefinedFunction string - A JavaScript function, respresented as a string
Errors
azure_cosmosdb: DbOperationError
The payload access errors where the error detail contains the HTTP status.
azure_cosmosdb: Error
The union of all types of errors in the connector.
azure_cosmosdb: InputValidationError
The errors which occur when providing an invalid value.
azure_cosmosdb: PayloadValidationError
The errors which will come from the Azure API call itself.
Union types
azure_cosmosdb: PartitionKeyVersion
PartitionKeyVersion
Version of the partition key.
Import
import ballerinax/azure_cosmosdb;
Metadata
Released date: over 3 years ago
Version: 2.1.0
License: Apache-2.0
Compatibility
Platform: java11
Ballerina version: slbeta6
Pull count
Total: 6743
Current verison: 25
Weekly downloads
Keywords
IT Operations/Databases
Cost/Paid
Vendor/Microsoft
Contributors