twilio
Modules
twilio
twilio.listenerModule twilio
API
Definitions
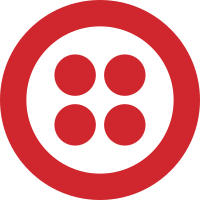
ballerinax/twilio Ballerina library
Overview
The Twilio API provides capability to access its platform for communications. These APIs connects the software layer and communications networks around the world to allow users to call and message anyone, globally.
This module supports Twilio Basic API version 2010-04-01.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Twilio account.
- Obtain tokens as follows:
-
Create a Twilio account.
-
Obtain a Twilio phone number.
!!! Tip
If you use a trail account, you may need to verify your recipient phone numbers before having any communication with them.
-
Obtain a Twilio Account Auth Token.
-
If you want to use WhatsApp service, Configure your Twilio phone number to use WhatsApp services. For instructions, see Twilio Documentation - Manage and Configure Your WhatsApp-enabled Twilio Numbers.
-
Configure the connector with obtained tokens.
Quickstart
To use the Twilio connector in your Ballerina application, update the .bal file as follows:
Step 1 - Import connector
Import the Twilio module to your Ballerina program as follows. You can use configurable variables to provide the necessary credentials.
import ballerinax/twilio;
Step 2 - Create a new connector instance
To create a new connector instance, add a configuration as follows:
configurable string accountSId = ?; configurable string authToken = ?; twilio:ConnectionConfig twilioConfig = { auth: { accountSId: accountSId, authToken: authToken } }; twilio:Client twilioClient = new (twilioConfig);
Step 3 - Invoke connector operation
- Invoke the connector operation using the client as follows:
public function main() returns error? { twilio:Account response = check twilioClient->getAccountDetails(); }
- Use
bal run
command to compile and run the Ballerina program.
Clients
twilio: Client
The Twilio API provides capability to access its platform for communications. These APIs connects the software layer and communications networks around the world to allow users to call and message anyone, globally.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Twilio account and obtain account SID and auth token at console
or else follow this guide for more details.
init (ConnectionConfig twilioConfig, ClientConfiguration httpClientConfig)
- twilioConfig ConnectionConfig - Twilio connection configuration
- httpClientConfig ClientConfiguration {} -
getAccountDetails
Gets account details of the given account-sid.
sendSms
function sendSms(string fromNo, string toNo, string message, string? statusCallbackUrl) returns SmsResponse|error
Sends SMS from the given account-sid.
Parameters
- fromNo string - Mobile number which the SMS should be sent from
- toNo string - Mobile number which the SMS should be received to
- message string - Message body of the SMS
- statusCallbackUrl string? (default ()) - (optional) Callback URL where the status callback events are needed to be dispatched
Return Type
- SmsResponse|error - A programmable SMS response object or else an error
getMessage
function getMessage(string messageSid) returns MessageResourceResponse|error
Gets the relevant message from a given message-sid.
Parameters
- messageSid string - Message-sid of a relevant message
Return Type
- MessageResourceResponse|error - A message resource response record or else an error
sendWhatsAppMessage
function sendWhatsAppMessage(string fromNo, string toNo, string message) returns WhatsAppResponse|error
Sends WhatsApp message from the given Sender ID of the account.
Parameters
- fromNo string - Mobile number from which the WhatsApp message should be sent
- toNo string - Mobile number by which the WhatsApp message should be received
- message string - Message body of the WhatsApp message
Return Type
- WhatsAppResponse|error - A whatsAppResponse object or else an error
makeVoiceCall
function makeVoiceCall(string fromNo, string toNo, VoiceCallInput voiceCallInput, StatusCallback? statusCallback) returns VoiceCallResponse|error
Makes a voice call from the given account-sid.
Parameters
- fromNo string - Mobile number which the voice call should be sent from
- toNo string - Mobile number which the voice call should be received to
- voiceCallInput VoiceCallInput - What should be heard when the other party picks up the phone (a Url that returns TwiML Voice instructions or inline message. example: "http://demo.twilio.com/docs/voice.xml" or "Thank you for calling")
- statusCallback StatusCallback? (default ()) - (optional) StatusCallback record which contains the callback url and the events whose status needs to be delivered
Return Type
- VoiceCallResponse|error - A voiceCallresponse object with basic details or else an error
Constants
Enums
twilio: VoiceCallInputType
Represents voice call input types.
Members
Records
twilio: Account
Represents Twilio account.
Fields
- sid string(default "") - Unique identifier of the account
- name string(default "") - The name of the account
- status string(default "") - The status of the account (active, suspended, closed)
- 'type string(default "") -
- createdDate string(default "") - The date that this account was created
- updatedDate string(default "") - The date that this account was last updated
twilio: APIKeyBasedAuthentication
Twilio API Key Based Authentication
Fields
- accountSId string - Twilio account SID
- apiKey string - Twilio API key SID
- apiSecret string - Twilio API key Secret
twilio: AuthyAppDetailsResponse
Represents Authy app details response.
Fields
- appId string(default "") - Unique identifier of the Authy app
- name string(default "") - Name of the Authy app
- plan string(default "") - The subscribed plan
- isSmsEnabled boolean(default false) - Status of whether SMS is enabled
- isPhoneCallsEnabled boolean(default false) - Status of whether phone call is enabled
- isOnetouchEnabled boolean(default false) - Status of whether one touch is enabled
- message string(default "") - A messaging indicating the result of the operation
- isSuccess boolean(default false) - Is the request was success or not
twilio: AuthyOtpResponse
Represents Authy OTP response.
Fields
- message string(default "") - A messaging indicating the result of the operation
- cellphone string(default "") - Phone number used to send the message or call
- isIgnored boolean(default false) - True if we detected an Authy or SDK enabled app installed
- isSuccess boolean(default false) - Is the request was success or not
twilio: AuthyOtpVerifyResponse
Represents Authy OTP verify response.
Fields
- message string(default "") - A messaging indicating the result of the operation
- token string(default "") - Either "is valid" or "is invalid"
- isSuccess boolean(default false) - Is the OTP was valid or not
twilio: AuthyUserAddResponse
Represents Authy user adding response.
Fields
- userId string(default "") - Unique identifier of the user
- message string(default "") - A messaging indicating the result of the operation
- isSuccess boolean(default false) - Is the request was success or not
twilio: AuthyUserDeleteResponse
Represents Authy user delete response.
Fields
- message string(default "") - A messaging indicating the result of the operation
- isSuccess boolean(default false) - Is the request was success or not
twilio: AuthyUserSecretResponse
Represents Authy user secret response.
Fields
- issuer string(default "") - Name of the Authy name
- label string(default "") - A custom label given by the user. If not, the name of the authy app
- qrCodeUrl string(default "") - URL for the generated qr code
- isSuccess boolean(default false) - Is the request was success or not
twilio: AuthyUserStatusResponse
Represents Authy user status response.
Fields
- userId string(default "") - Unique identifier of the user
- isConfirmed boolean(default false) - Is user confirmed or not
- isRegistered boolean(default false) - Is user registered or not
- countryCode string(default "") - Country code of user
- phoneNumber string(default "") - Phone number of user
- isAccountDisabled boolean(default false) - Is account disabled or not
- message string(default "") - A messaging indicating the result of the operation
- isSuccess boolean(default false) - Is the request was success or not
twilio: ConnectionConfig
Twilio Configuration.
Fields
- auth TokenBasedAuthentication|APIKeyBasedAuthentication - Twilio authentication configuration
twilio: MessageResourceResponse
Represents the message resource in the Twilio Rest API.
Fields
- body string(default "") - The message body
- numSegments string(default "") - The number of segments, which make up the complete message
- direction string(default "") - The direction of the message (inbound, outbound-api, outbound-call, outbound-reply)
- fromNumber string(default "") - The phone number from which the message sent
- toNumber string(default "") - The phone number to which the message received
- dateUpdated string(default "") - The date and time at which this resource was last updated
- price string(default "") - The price amount of the message
- errorMessage string(default "") - The description of the error_code if the message status is failed or undelivered
- uri string(default "") - The URI of the resource relative to https://api.twilio.com
- accountSid string(default "") - The unique identifier of the account, which sent the message
- numMedia string(default "") - The number of associated media files
- status string(default "") - The status of the message
- messagingServiceSid string(default "") - The SID of the Messaging Service used with the message. The value is null if a Messaging Service was not used
- sid string(default "") - The unique string that created to identify the message resource
- dateSent string(default "") - The date and time in GMT that the resource was sent specified in RFC 2822 format
- dateCreated string(default "") - The date and time in GMT that the resource was created specified in RFC 2822 format
- errorCode string(default "") - The error code returned if your message status is failed or undelivered
- priceUnit string(default "") - The currency in which price is measured, in ISO 4127 format
- apiVersion string(default "") - The API version used to process the message
- subresourceUris json(default {}) - A list of related resources identified by their URIs relative to https://api.twilio.com
twilio: SmsResponse
Represents Twilio SMS response.
Fields
- sid string(default "") - Unique identifier of the account
- dateCreated string(default "") - The date and time at which this resource was created
- dateUpdated string(default "") - The date and time at which this resource was last updated
- dateSent string(default "") - The date and time at which the outgoing message was sent
- accountSid string(default "") - The unique identifier of the account, which sent the message
- toNumber string(default "") - The phone number to which the message was sent
- fromNumber string(default "") - The phone number from which the message was sent
- body string(default "") - The text of the message to be sent
- status string(default "") - Status of the voice call (queued, failed, sent, delivered, undelivered)
- direction string(default "") - The direction of the message (inbound, outbound-api, outbound-call, outbound-reply)
- apiVersion string(default "") - The API version, which is used to process the message
- price string(default "") - The price amount of the SMS
- priceUnit string(default "") - The price currency
- uri string(default "") - The URI of the resource relative to https://api.twilio.com
- numSegments string(default "") - The number of segments, which make up the complete message
twilio: StatusCallback
Represents the StatusCallback record for registering status change callback URL for Twilio Voice status change events.
Fields
- url string - Callback URL where the status changes needs to be delivered.
- method string - HTTP method in which the event payload needs to be delivered
- events string[]? - Interested list of status change events.
twilio: TokenBasedAuthentication
Twilio Token Based Authentication
Fields
- accountSId string - Twilio account SID
- authToken string - The authentication token of the account
twilio: VoiceCallInput
Represents voice call message input options.
Fields
- userInputType VoiceCallInputType - Whether the userInput is a URL or inline message
- userInput string - A Twiml URL or an inline Message that what should be heard when the other party picks up the phone
twilio: VoiceCallResponse
Represents Twilio voice call response.
Fields
- sid string(default "") - Unique identifier of the account
- status string(default "") - Status of the voice call (queued, initiated, ringing, answered, completed)
- price string(default "") - The price amount of the call
- priceUnit string(default "") - The price currency
twilio: WhatsAppResponse
Represents the Twilio WhatsApp message response. More details of the message format is accessible from https://www.twilio.com/docs/sms/api/message-resource#create-a-message-resource
Fields
- sid string(default "") - Unique identifier of the account
- dateCreated string(default "") - The date and time at which this resource was created
- dateUpdated string(default "") - The date and time at which this resource was last updated
- dateSent string(default "") - The date and time at which the outgoing message was sent
- accountSid string(default "") - The unique identifier of the account, which sent the message
- toNumber string(default "") - The phone number to which the message was sent
- fromNumber string(default "") - The phone number from which the message was sent
- messageServiceSid string(default "") - The SID of the Messaging Service, which will be associated with the message
- body string(default "") - The text of the message to be sent
- status string(default "") - Status of the voice call (queued, failed, sent, delivered, undelivered)
- numSegments string(default "") - The number of segments, which make up the complete message
- numMedia string(default "") - The number of associated media files
- direction string(default "") - The direction of the message (inbound, outbound-api, outbound-call, outbound-reply)
- apiVersion string(default "") - The API version used to process the message
- price string(default "") - The price of the SMS (This is set to null for WhatsApp messages)
- priceUnit string(default "") - The currency of the price (This is set to null for WhatsApp messages)
- errorCode string(default "") - The error code returned if the message status is failed or undelivered
- errorMessage string(default "") - The description of the error_code if the message status is failed or undelivered
- uri string(default "") - The URI of the resource relative to https://api.twilio.com
- subresourceUris json(default {}) - A list of related resources identified by their URIs relative to https://api.twilio.com
Errors
twilio: Error
Represents the Twilio module related error.
twilio: TwilioError
Represents the Twilio error. This will be returned if an error occurred on Twilio operations.
Import
import ballerinax/twilio;
Metadata
Released date: over 3 years ago
Version: 2.1.0
License: Apache-2.0
Compatibility
Platform: java11
Ballerina version: slbeta6
Pull count
Total: 2260128
Current verison: 610
Weekly downloads
Keywords
Communication/Phone & SMS
Cost/Paid
Contributors