twilio
Module twilio
API
Definitions
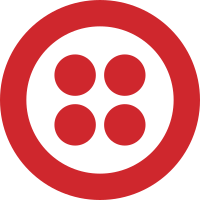
ballerinax/twilio Ballerina library
Overview
Twilio is a cloud communications platform that allows software developers to programmatically make and receive phone calls, send and receive text messages, and perform other communication functions using its web service APIs.
The Ballerina Twilio connector supports the Twilio Basic API version 2010-04-01, enabling users to leverage these communication capabilities within their Ballerina applications.
Setup guide
Before using the ballerinax-twilio connector you must have access to Twilio API, If you do not have access to Twilio API please complete the following steps:
Step 1: Create a Twilio account
Creating a Twilio account can be done by visiting Twilio and clicking the "Try Twilio for Free" button.
Step 2: Obtain a Twilio phone number
All trial projects can provision a free trial phone number for testing. Here's how to get started.
Notice: Trial project phone number selection may be limited. You must upgrade your Twilio project to provision more than one phone number, or to provision a number that is not available to trial projects.
- Access the Buy a Number page in the Console.
- Enter the criteria for the phone number you need, and then click Search.
- Country: Select the desired country from the drop-down menu.
- Number or Location: Select the desired option to search by digits/phrases, or a specific City or Region.
- Capabilities: Select your service needs for this number.
- Click Buy to purchase a phone number for your current project or sub-account.
Notice: Many countries require identity documentation for Phone Number compliance. Requests to provision phone numbers with these regulations will be required to select or add the required documentation after clicking Buy in Console. To see which countries and phone number types are affected by these requirements, please see twilio's Phone Number Regulations site.
Step 3: Obtain a Twilio account Sid with auth token
Twilio uses two credentials to determine which account an API request is coming from: The account Sid, which acts as a username
, and the Auth Token which acts as a password
. You can find your account Sid and auth token in your Twilio console.
Your account's Auth Token is hidden by default. Click show to display the token, and hide to conceal it again. For further information click here
Quickstart
To use the twilio
connector in your Ballerina application, modify the .bal
file as follows:
Step 1 - Import the module
Import the Twilio module into your Ballerina program as shown below:
import ballerinax/twilio;
Step 2 - Create a new connector instance
To create a new connector instance, add a configuration as follows (You can use configurable variables to provide the necessary credentials):
configurable string accountSid = ?; configurable string authToken = ?; twilio:ConnectionConfig twilioConfig = { auth: { username: accountSid, password: authToken } }; twilio:Client twilio = check new (twilioConfig);
Step 3 - Invoke the connector operation
Invoke the sending SMS operation using the client as shown below:
public function main() returns error? { twilio:CreateMessageRequest messageRequest = { To: "+XXXXXXXXXXX", // Phone number that you want to send the message to From: "+XXXXXXXXXXX", // Twilio phone number Body: "Hello from Ballerina" }; twilio:Message response = check twilio->createMessage(messageRequest); // Print the status of the message from the response io:println("Message Status: ", response?.status); }
Step 4: Run the Ballerina application
bal run
Examples
The Twilio connector comes equipped with examples that demonstrate its usage across various scenarios. These examples are conveniently organized into three distinct groups based on the functionalities they showcase. For a more hands-on experience and a deeper understanding of these capabilities, we encourage you to experiment with the provided examples in your development environment.
- Account management
- Create a sub-account - Create a subaccount under a Twilio account
- Fetch an account - Get details of a Twilio account
- Fetch balance - Get the balance of a Twilio account
- List accounts - List all subaccounts under a Twilio account
- Update an account - Update the name of a Twilio account
- Call management
- Make a call - Make a call to a phone number via a Twilio
- Fetch call log - Get details of a call made via a Twilio
- List call logs - Get details of all calls made via a Twilio
- Delete a call log - Delete the log of a call made via Twilio
- Message management
- Send an SMS message - Send an SMS to a phone number via a Twilio
- Send a Whatsapp message - Send a Whatsapp message to a phone number via a Twilio
- List message logs - Get details of all messages sent via a Twilio
- Fetch a message log - Get details of a message sent via a Twilio
- Delete a message log - Delete a message log via a Twilio
Clients
twilio: Client
This is the public Twilio REST API.
Constructor
Gets invoked to initialize the connector
.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.twilio.com" - URL of the target service
listAccount
function listAccount(string? friendlyName, Account_enum_status? status, int? pageSize, int? page, string? pageToken) returns ListAccountResponse|error
Retrieves a collection of Accounts belonging to the account used to make the request
Parameters
- friendlyName string? (default ()) - Only return the Account resources with friendly names that exactly match this name.
- status Account_enum_status? (default ()) - Only return Account resources with the given status. Can be
closed
,suspended
oractive
.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
- ListAccountResponse|error - OK
createAccount
function createAccount(CreateAccountRequest payload) returns Account|error
Create a new Twilio Subaccount from the account making the request
Parameters
- payload CreateAccountRequest - The
CreateAccountRequest
record should be used as a payload to create a Subaccount.
fetchAccount
Fetch the account specified by the provided Account Sid
Parameters
- sid string - The Account Sid that uniquely identifies the account to fetch
updateAccount
function updateAccount(string sid, UpdateAccountRequest payload) returns Account|error
Modify the properties of a given Account
Parameters
- sid string - The Account Sid that uniquely identifies the account to update
- payload UpdateAccountRequest - The
UpdateAccountRequest
record should be used as a payload to update an Account.
listAddress
function listAddress(string? customerName, string? friendlyName, string? isoCountry, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListAddressResponse|error
List Address
Parameters
- customerName string? (default ()) - The
customer_name
of the Address resources to read.
- friendlyName string? (default ()) - The string that identifies the Address resources to read.
- isoCountry string? (default ()) - The ISO country code of the Address resources to read.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
- ListAddressResponse|error - OK
createAddress
function createAddress(CreateAddressRequest payload, string? accountSid) returns Address|error
Create Address
Parameters
- payload CreateAddressRequest - The
CreateAddressRequest
record should be used as a payload to create a new Address resource.
fetchAddress
Fetch Address
Parameters
- sid string - The Twilio-provided string that uniquely identifies the Address resource to fetch.
updateAddress
function updateAddress(string sid, UpdateAddressRequest payload, string? accountSid) returns Address|error
Update Address
Parameters
- sid string - The Twilio-provided string that uniquely identifies the Address resource to update.
- payload UpdateAddressRequest - The
UpdateAddressRequest
record should be used as a payload to update an Address resource.
deleteAddress
Delete Address
Parameters
- sid string - The Twilio-provided string that uniquely identifies the Address resource to delete.
listApplication
function listApplication(string? friendlyName, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListApplicationResponse|error
Retrieve a list of applications representing an application within the requesting account
Parameters
- friendlyName string? (default ()) - The string that identifies the Application resources to read.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
createApplication
function createApplication(CreateApplicationRequest payload, string? accountSid) returns Application|error
Create a new application within your account
Parameters
- payload CreateApplicationRequest - The
CreateApplicationRequest
record should be used as a payload to create a new Application resource.
Return Type
- Application|error - Created
fetchApplication
function fetchApplication(string sid, string? accountSid) returns Application|error
Fetch the application specified by the provided sid
Parameters
- sid string - The Twilio-provided string that uniquely identifies the Application resource to fetch.
Return Type
- Application|error - OK
updateApplication
function updateApplication(string sid, UpdateApplicationRequest payload, string? accountSid) returns Application|error
Updates the application's properties
Parameters
- sid string - The Twilio-provided string that uniquely identifies the Application resource to update.
- payload UpdateApplicationRequest - The
UpdateApplicationRequest
record should be used as a payload to update an Application resource.
Return Type
- Application|error - OK
deleteApplication
Delete the application by the specified application sid
Parameters
- sid string - The Twilio-provided string that uniquely identifies the Application resource to delete.
fetchAuthorizedConnectApp
function fetchAuthorizedConnectApp(string connectAppSid, string? accountSid) returns Authorized_connect_app|error
Fetch an instance of an authorized-connect-app
Parameters
- connectAppSid string - The SID of the Connect App to fetch.
Return Type
listAuthorizedConnectApp
function listAuthorizedConnectApp(int? pageSize, int? page, string? pageToken, string? accountSid) returns ListAuthorizedConnectAppResponse|error
Retrieve a list of authorized-connect-apps belonging to the account used to make the request
Parameters
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
listAvailablePhoneNumberCountry
function listAvailablePhoneNumberCountry(int? pageSize, int? page, string? pageToken, string? accountSid) returns ListAvailablePhoneNumberCountryResponse|error
List contries that have available for phone numbers
Parameters
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
fetchAvailablePhoneNumberCountry
function fetchAvailablePhoneNumberCountry(string countryCode, string? accountSid) returns Available_phone_number_country|error
Fetch an available phone number by country code
Parameters
- countryCode string - The ISO-3166-1 country code of the country to fetch available phone numbers information about.
Return Type
listAvailablePhoneNumberLocal
function listAvailablePhoneNumberLocal(string countryCode, int? areaCode, string? contains, boolean? smsEnabled, boolean? mmsEnabled, boolean? voiceEnabled, boolean? excludeAllAddressRequired, boolean? excludeLocalAddressRequired, boolean? excludeForeignAddressRequired, boolean? beta, string? nearNumber, string? nearLatLong, int? distance, string? inPostalCode, string? inRegion, string? inRateCenter, string? inLata, string? inLocality, boolean? faxEnabled, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListAvailablePhoneNumberLocalResponse|error
List available phone numbers (local)
Parameters
- countryCode string - The ISO-3166-1 country code of the country from which to read phone numbers.
- areaCode int? (default ()) - The area code of the phone numbers to read. Applies to only phone numbers in the US and Canada.
- smsEnabled boolean? (default ()) - Whether the phone numbers can receive text messages. Can be:
true
orfalse
.
- mmsEnabled boolean? (default ()) - Whether the phone numbers can receive MMS messages. Can be:
true
orfalse
.
- voiceEnabled boolean? (default ()) - Whether the phone numbers can receive calls. Can be:
true
orfalse
.
- beta boolean? (default ()) - Whether to read phone numbers that are new to the Twilio platform. Can be:
true
orfalse
and the default istrue
.
- nearNumber string? (default ()) - Given a phone number, find a geographically close number within
distance
miles. Distance defaults to 25 miles. Applies to only phone numbers in the US and Canada.
- nearLatLong string? (default ()) - Given a latitude/longitude pair
lat,long
find geographically close numbers withindistance
miles. Applies to only phone numbers in the US and Canada.
- distance int? (default ()) - The search radius, in miles, for a
near_
query. Can be up to500
and the default is25
. Applies to only phone numbers in the US and Canada.
- inPostalCode string? (default ()) - Limit results to a particular postal code. Given a phone number, search within the same postal code as that number. Applies to only phone numbers in the US and Canada.
- inRegion string? (default ()) - Limit results to a particular region, state, or province. Given a phone number, search within the same region as that number. Applies to only phone numbers in the US and Canada.
- inRateCenter string? (default ()) - Limit results to a specific rate center, or given a phone number search within the same rate center as that number. Requires
in_lata
to be set as well. Applies to only phone numbers in the US and Canada.
- inLocality string? (default ()) - Limit results to a particular locality or city. Given a phone number, search within the same Locality as that number.
- faxEnabled boolean? (default ()) - Whether the phone numbers can receive faxes. Can be:
true
orfalse
.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
listAvailablePhoneNumberMachineToMachine
function listAvailablePhoneNumberMachineToMachine(string countryCode, int? areaCode, string? contains, boolean? smsEnabled, boolean? mmsEnabled, boolean? voiceEnabled, boolean? excludeAllAddressRequired, boolean? excludeLocalAddressRequired, boolean? excludeForeignAddressRequired, boolean? beta, string? nearNumber, string? nearLatLong, int? distance, string? inPostalCode, string? inRegion, string? inRateCenter, string? inLata, string? inLocality, boolean? faxEnabled, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListAvailablePhoneNumberMachineToMachineResponse|error
List available phone numbers (MachineToMachine)
Parameters
- countryCode string - The ISO-3166-1 country code of the country from which to read phone numbers.
- areaCode int? (default ()) - The area code of the phone numbers to read. Applies to only phone numbers in the US and Canada.
- smsEnabled boolean? (default ()) - Whether the phone numbers can receive text messages. Can be:
true
orfalse
.
- mmsEnabled boolean? (default ()) - Whether the phone numbers can receive MMS messages. Can be:
true
orfalse
.
- voiceEnabled boolean? (default ()) - Whether the phone numbers can receive calls. Can be:
true
orfalse
.
- beta boolean? (default ()) - Whether to read phone numbers that are new to the Twilio platform. Can be:
true
orfalse
and the default istrue
.
- nearNumber string? (default ()) - Given a phone number, find a geographically close number within
distance
miles. Distance defaults to 25 miles. Applies to only phone numbers in the US and Canada.
- nearLatLong string? (default ()) - Given a latitude/longitude pair
lat,long
find geographically close numbers withindistance
miles. Applies to only phone numbers in the US and Canada.
- distance int? (default ()) - The search radius, in miles, for a
near_
query. Can be up to500
and the default is25
. Applies to only phone numbers in the US and Canada.
- inPostalCode string? (default ()) - Limit results to a particular postal code. Given a phone number, search within the same postal code as that number. Applies to only phone numbers in the US and Canada.
- inRegion string? (default ()) - Limit results to a particular region, state, or province. Given a phone number, search within the same region as that number. Applies to only phone numbers in the US and Canada.
- inRateCenter string? (default ()) - Limit results to a specific rate center, or given a phone number search within the same rate center as that number. Requires
in_lata
to be set as well. Applies to only phone numbers in the US and Canada.
- inLocality string? (default ()) - Limit results to a particular locality or city. Given a phone number, search within the same Locality as that number.
- faxEnabled boolean? (default ()) - Whether the phone numbers can receive faxes. Can be:
true
orfalse
.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
listAvailablePhoneNumberMobile
function listAvailablePhoneNumberMobile(string countryCode, int? areaCode, string? contains, boolean? smsEnabled, boolean? mmsEnabled, boolean? voiceEnabled, boolean? excludeAllAddressRequired, boolean? excludeLocalAddressRequired, boolean? excludeForeignAddressRequired, boolean? beta, string? nearNumber, string? nearLatLong, int? distance, string? inPostalCode, string? inRegion, string? inRateCenter, string? inLata, string? inLocality, boolean? faxEnabled, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListAvailablePhoneNumberMobileResponse|error
List available phone numbers (Mobile)
Parameters
- countryCode string - The ISO-3166-1 country code of the country from which to read phone numbers.
- areaCode int? (default ()) - The area code of the phone numbers to read. Applies to only phone numbers in the US and Canada.
- smsEnabled boolean? (default ()) - Whether the phone numbers can receive text messages. Can be:
true
orfalse
.
- mmsEnabled boolean? (default ()) - Whether the phone numbers can receive MMS messages. Can be:
true
orfalse
.
- voiceEnabled boolean? (default ()) - Whether the phone numbers can receive calls. Can be:
true
orfalse
.
- beta boolean? (default ()) - Whether to read phone numbers that are new to the Twilio platform. Can be:
true
orfalse
and the default istrue
.
- nearNumber string? (default ()) - Given a phone number, find a geographically close number within
distance
miles. Distance defaults to 25 miles. Applies to only phone numbers in the US and Canada.
- nearLatLong string? (default ()) - Given a latitude/longitude pair
lat,long
find geographically close numbers withindistance
miles. Applies to only phone numbers in the US and Canada.
- distance int? (default ()) - The search radius, in miles, for a
near_
query. Can be up to500
and the default is25
. Applies to only phone numbers in the US and Canada.
- inPostalCode string? (default ()) - Limit results to a particular postal code. Given a phone number, search within the same postal code as that number. Applies to only phone numbers in the US and Canada.
- inRegion string? (default ()) - Limit results to a particular region, state, or province. Given a phone number, search within the same region as that number. Applies to only phone numbers in the US and Canada.
- inRateCenter string? (default ()) - Limit results to a specific rate center, or given a phone number search within the same rate center as that number. Requires
in_lata
to be set as well. Applies to only phone numbers in the US and Canada.
- inLocality string? (default ()) - Limit results to a particular locality or city. Given a phone number, search within the same Locality as that number.
- faxEnabled boolean? (default ()) - Whether the phone numbers can receive faxes. Can be:
true
orfalse
.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
listAvailablePhoneNumberNational
function listAvailablePhoneNumberNational(string countryCode, int? areaCode, string? contains, boolean? smsEnabled, boolean? mmsEnabled, boolean? voiceEnabled, boolean? excludeAllAddressRequired, boolean? excludeLocalAddressRequired, boolean? excludeForeignAddressRequired, boolean? beta, string? nearNumber, string? nearLatLong, int? distance, string? inPostalCode, string? inRegion, string? inRateCenter, string? inLata, string? inLocality, boolean? faxEnabled, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListAvailablePhoneNumberNationalResponse|error
List available phone numbers (National)
Parameters
- countryCode string - The ISO-3166-1 country code of the country from which to read phone numbers.
- areaCode int? (default ()) - The area code of the phone numbers to read. Applies to only phone numbers in the US and Canada.
- smsEnabled boolean? (default ()) - Whether the phone numbers can receive text messages. Can be:
true
orfalse
.
- mmsEnabled boolean? (default ()) - Whether the phone numbers can receive MMS messages. Can be:
true
orfalse
.
- voiceEnabled boolean? (default ()) - Whether the phone numbers can receive calls. Can be:
true
orfalse
.
- beta boolean? (default ()) - Whether to read phone numbers that are new to the Twilio platform. Can be:
true
orfalse
and the default istrue
.
- nearNumber string? (default ()) - Given a phone number, find a geographically close number within
distance
miles. Distance defaults to 25 miles. Applies to only phone numbers in the US and Canada.
- nearLatLong string? (default ()) - Given a latitude/longitude pair
lat,long
find geographically close numbers withindistance
miles. Applies to only phone numbers in the US and Canada.
- distance int? (default ()) - The search radius, in miles, for a
near_
query. Can be up to500
and the default is25
. Applies to only phone numbers in the US and Canada.
- inPostalCode string? (default ()) - Limit results to a particular postal code. Given a phone number, search within the same postal code as that number. Applies to only phone numbers in the US and Canada.
- inRegion string? (default ()) - Limit results to a particular region, state, or province. Given a phone number, search within the same region as that number. Applies to only phone numbers in the US and Canada.
- inRateCenter string? (default ()) - Limit results to a specific rate center, or given a phone number search within the same rate center as that number. Requires
in_lata
to be set as well. Applies to only phone numbers in the US and Canada.
- inLocality string? (default ()) - Limit results to a particular locality or city. Given a phone number, search within the same Locality as that number.
- faxEnabled boolean? (default ()) - Whether the phone numbers can receive faxes. Can be:
true
orfalse
.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
listAvailablePhoneNumberSharedCost
function listAvailablePhoneNumberSharedCost(string countryCode, int? areaCode, string? contains, boolean? smsEnabled, boolean? mmsEnabled, boolean? voiceEnabled, boolean? excludeAllAddressRequired, boolean? excludeLocalAddressRequired, boolean? excludeForeignAddressRequired, boolean? beta, string? nearNumber, string? nearLatLong, int? distance, string? inPostalCode, string? inRegion, string? inRateCenter, string? inLata, string? inLocality, boolean? faxEnabled, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListAvailablePhoneNumberSharedCostResponse|error
List available phone numbers (SharedCost)
Parameters
- countryCode string - The ISO-3166-1 country code of the country from which to read phone numbers.
- areaCode int? (default ()) - The area code of the phone numbers to read. Applies to only phone numbers in the US and Canada.
- smsEnabled boolean? (default ()) - Whether the phone numbers can receive text messages. Can be:
true
orfalse
.
- mmsEnabled boolean? (default ()) - Whether the phone numbers can receive MMS messages. Can be:
true
orfalse
.
- voiceEnabled boolean? (default ()) - Whether the phone numbers can receive calls. Can be:
true
orfalse
.
- beta boolean? (default ()) - Whether to read phone numbers that are new to the Twilio platform. Can be:
true
orfalse
and the default istrue
.
- nearNumber string? (default ()) - Given a phone number, find a geographically close number within
distance
miles. Distance defaults to 25 miles. Applies to only phone numbers in the US and Canada.
- nearLatLong string? (default ()) - Given a latitude/longitude pair
lat,long
find geographically close numbers withindistance
miles. Applies to only phone numbers in the US and Canada.
- distance int? (default ()) - The search radius, in miles, for a
near_
query. Can be up to500
and the default is25
. Applies to only phone numbers in the US and Canada.
- inPostalCode string? (default ()) - Limit results to a particular postal code. Given a phone number, search within the same postal code as that number. Applies to only phone numbers in the US and Canada.
- inRegion string? (default ()) - Limit results to a particular region, state, or province. Given a phone number, search within the same region as that number. Applies to only phone numbers in the US and Canada.
- inRateCenter string? (default ()) - Limit results to a specific rate center, or given a phone number search within the same rate center as that number. Requires
in_lata
to be set as well. Applies to only phone numbers in the US and Canada.
- inLocality string? (default ()) - Limit results to a particular locality or city. Given a phone number, search within the same Locality as that number.
- faxEnabled boolean? (default ()) - Whether the phone numbers can receive faxes. Can be:
true
orfalse
.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
listAvailablePhoneNumberTollFree
function listAvailablePhoneNumberTollFree(string countryCode, int? areaCode, string? contains, boolean? smsEnabled, boolean? mmsEnabled, boolean? voiceEnabled, boolean? excludeAllAddressRequired, boolean? excludeLocalAddressRequired, boolean? excludeForeignAddressRequired, boolean? beta, string? nearNumber, string? nearLatLong, int? distance, string? inPostalCode, string? inRegion, string? inRateCenter, string? inLata, string? inLocality, boolean? faxEnabled, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListAvailablePhoneNumberTollFreeResponse|error
List available phone numbers (TollFree)
Parameters
- countryCode string - The ISO-3166-1 country code of the country from which to read phone numbers.
- areaCode int? (default ()) - The area code of the phone numbers to read. Applies to only phone numbers in the US and Canada.
- smsEnabled boolean? (default ()) - Whether the phone numbers can receive text messages. Can be:
true
orfalse
.
- mmsEnabled boolean? (default ()) - Whether the phone numbers can receive MMS messages. Can be:
true
orfalse
.
- voiceEnabled boolean? (default ()) - Whether the phone numbers can receive calls. Can be:
true
orfalse
.
- beta boolean? (default ()) - Whether to read phone numbers that are new to the Twilio platform. Can be:
true
orfalse
and the default istrue
.
- nearNumber string? (default ()) - Given a phone number, find a geographically close number within
distance
miles. Distance defaults to 25 miles. Applies to only phone numbers in the US and Canada.
- nearLatLong string? (default ()) - Given a latitude/longitude pair
lat,long
find geographically close numbers withindistance
miles. Applies to only phone numbers in the US and Canada.
- distance int? (default ()) - The search radius, in miles, for a
near_
query. Can be up to500
and the default is25
. Applies to only phone numbers in the US and Canada.
- inPostalCode string? (default ()) - Limit results to a particular postal code. Given a phone number, search within the same postal code as that number. Applies to only phone numbers in the US and Canada.
- inRegion string? (default ()) - Limit results to a particular region, state, or province. Given a phone number, search within the same region as that number. Applies to only phone numbers in the US and Canada.
- inRateCenter string? (default ()) - Limit results to a specific rate center, or given a phone number search within the same rate center as that number. Requires
in_lata
to be set as well. Applies to only phone numbers in the US and Canada.
- inLocality string? (default ()) - Limit results to a particular locality or city. Given a phone number, search within the same Locality as that number.
- faxEnabled boolean? (default ()) - Whether the phone numbers can receive faxes. Can be:
true
orfalse
.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
listAvailablePhoneNumberVoip
function listAvailablePhoneNumberVoip(string countryCode, int? areaCode, string? contains, boolean? smsEnabled, boolean? mmsEnabled, boolean? voiceEnabled, boolean? excludeAllAddressRequired, boolean? excludeLocalAddressRequired, boolean? excludeForeignAddressRequired, boolean? beta, string? nearNumber, string? nearLatLong, int? distance, string? inPostalCode, string? inRegion, string? inRateCenter, string? inLata, string? inLocality, boolean? faxEnabled, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListAvailablePhoneNumberVoipResponse|error
List available phone numbers (Voip)
Parameters
- countryCode string - The ISO-3166-1 country code of the country from which to read phone numbers.
- areaCode int? (default ()) - The area code of the phone numbers to read. Applies to only phone numbers in the US and Canada.
- smsEnabled boolean? (default ()) - Whether the phone numbers can receive text messages. Can be:
true
orfalse
.
- mmsEnabled boolean? (default ()) - Whether the phone numbers can receive MMS messages. Can be:
true
orfalse
.
- voiceEnabled boolean? (default ()) - Whether the phone numbers can receive calls. Can be:
true
orfalse
.
- beta boolean? (default ()) - Whether to read phone numbers that are new to the Twilio platform. Can be:
true
orfalse
and the default istrue
.
- nearNumber string? (default ()) - Given a phone number, find a geographically close number within
distance
miles. Distance defaults to 25 miles. Applies to only phone numbers in the US and Canada.
- nearLatLong string? (default ()) - Given a latitude/longitude pair
lat,long
find geographically close numbers withindistance
miles. Applies to only phone numbers in the US and Canada.
- distance int? (default ()) - The search radius, in miles, for a
near_
query. Can be up to500
and the default is25
. Applies to only phone numbers in the US and Canada.
- inPostalCode string? (default ()) - Limit results to a particular postal code. Given a phone number, search within the same postal code as that number. Applies to only phone numbers in the US and Canada.
- inRegion string? (default ()) - Limit results to a particular region, state, or province. Given a phone number, search within the same region as that number. Applies to only phone numbers in the US and Canada.
- inRateCenter string? (default ()) - Limit results to a specific rate center, or given a phone number search within the same rate center as that number. Requires
in_lata
to be set as well. Applies to only phone numbers in the US and Canada.
- inLocality string? (default ()) - Limit results to a particular locality or city. Given a phone number, search within the same Locality as that number.
- faxEnabled boolean? (default ()) - Whether the phone numbers can receive faxes. Can be:
true
orfalse
.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
fetchBalance
Fetch the balance for an Account based on Account Sid. Balance changes may not be reflected immediately. Child accounts do not contain balance information
Parameters
- accountSid string? (default ()) - The unique SID identifier of the Account.
listCall
function listCall(string? to, string? 'from, string? parentCallSid, Call_enum_status? status, string? startTime, string? startedOnOrBefore, string? startedOnOrAfter, string? endTime, string? endedOnOrBefore, string? endedOnOrAfter, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListCallResponse|error
Retrieves a collection of calls made to and from your account
Parameters
- to string? (default ()) - Only show calls made to this phone number, SIP address, Client identifier or SIM SID.
- 'from string? (default ()) - Only include calls from this phone number, SIP address, Client identifier or SIM SID.
- parentCallSid string? (default ()) - Only include calls spawned by calls with this SID.
- status Call_enum_status? (default ()) - The status of the calls to include. Can be:
queued
,ringing
,in-progress
,canceled
,completed
,failed
,busy
, orno-answer
.
- startTime string? (default ()) - Only include calls that started on this date. Specify a date as
YYYY-MM-DD
in GMT, for example:2009-07-06
, to read only calls that started on this date. You can also specify an inequality, such asStartTime<=YYYY-MM-DD
, to read calls that started on or before midnight of this date, andStartTime>=YYYY-MM-DD
to read calls that started on or after midnight of this date.
- startedOnOrBefore string? (default ()) - Only include calls that started on this date. Specify a date as
YYYY-MM-DD
in GMT, for example:2009-07-06
, to read only calls that started on this date. You can also specify an inequality, such asStartTime<=YYYY-MM-DD
, to read calls that started on or before midnight of this date, andStartTime>=YYYY-MM-DD
to read calls that started on or after midnight of this date.
- startedOnOrAfter string? (default ()) - Only include calls that started on this date. Specify a date as
YYYY-MM-DD
in GMT, for example:2009-07-06
, to read only calls that started on this date. You can also specify an inequality, such asStartTime<=YYYY-MM-DD
, to read calls that started on or before midnight of this date, andStartTime>=YYYY-MM-DD
to read calls that started on or after midnight of this date.
- endTime string? (default ()) - Only include calls that ended on this date. Specify a date as
YYYY-MM-DD
in GMT, for example:2009-07-06
, to read only calls that ended on this date. You can also specify an inequality, such asEndTime<=YYYY-MM-DD
, to read calls that ended on or before midnight of this date, andEndTime>=YYYY-MM-DD
to read calls that ended on or after midnight of this date.
- endedOnOrBefore string? (default ()) - Only include calls that ended on this date. Specify a date as
YYYY-MM-DD
in GMT, for example:2009-07-06
, to read only calls that ended on this date. You can also specify an inequality, such asEndTime<=YYYY-MM-DD
, to read calls that ended on or before midnight of this date, andEndTime>=YYYY-MM-DD
to read calls that ended on or after midnight of this date.
- endedOnOrAfter string? (default ()) - Only include calls that ended on this date. Specify a date as
YYYY-MM-DD
in GMT, for example:2009-07-06
, to read only calls that ended on this date. You can also specify an inequality, such asEndTime<=YYYY-MM-DD
, to read calls that ended on or before midnight of this date, andEndTime>=YYYY-MM-DD
to read calls that ended on or after midnight of this date.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
- ListCallResponse|error - OK
createCall
function createCall(CreateCallRequest payload, string? accountSid) returns Call|error
Create a new outgoing call to phones, SIP-enabled endpoints or Twilio Client connections
Parameters
- payload CreateCallRequest - The
CrateCallRequest
record should be used as a payload to create a call.
fetchCall
Fetch the call specified by the provided Call SID
Parameters
- sid string - The SID of the Call resource to fetch.
updateCall
function updateCall(string sid, UpdateCallRequest payload, string? accountSid) returns Call|error
Initiates a call redirect or terminates a call
Parameters
- sid string - The Twilio-provided string that uniquely identifies the Call resource to update
- payload UpdateCallRequest - The
UpdateCallRequest
record should be used as a payload to update a call.
deleteCall
Delete a Call record from your account. Once the record is deleted, it will no longer appear in the API and Account Portal logs.
Parameters
- sid string - The Twilio-provided Call SID that uniquely identifies the Call resource to delete
listCallEvent
function listCallEvent(string callSid, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListCallEventResponse|error
Retrieve a list of all events for a call.
Parameters
- callSid string - The unique SID identifier of the Call.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
- accountSid string? (default ()) - The unique SID identifier of the Account.
Return Type
fetchCallFeedback
function fetchCallFeedback(string callSid, string? accountSid) returns CallCall_feedback|error
Fetch a Feedback resource from a call
Parameters
- callSid string - The call sid that uniquely identifies the call
Return Type
- CallCall_feedback|error - OK
updateCallFeedback
function updateCallFeedback(string callSid, UpdateCallFeedbackRequest payload, string? accountSid) returns CallCall_feedback|error
Update a Feedback resource for a call
Parameters
- callSid string - The call sid that uniquely identifies the call
- payload UpdateCallFeedbackRequest - The
UpdateCallFeedbackRequest
record should be used as a payload to update a feedback.
Return Type
- CallCall_feedback|error - OK
createCallFeedbackSummary
function createCallFeedbackSummary(CreateCallFeedbackSummaryRequest payload, string? accountSid) returns CallCall_feedback_summary|error
Create a FeedbackSummary resource for a call
Parameters
- payload CreateCallFeedbackSummaryRequest - The
CreateCallFeedbackSummaryRequest
record should be used as a payload to create a feedback summary.
Return Type
- CallCall_feedback_summary|error - Created
fetchCallFeedbackSummary
function fetchCallFeedbackSummary(string sid, string? accountSid) returns CallCall_feedback_summary|error
Fetch a FeedbackSummary resource from a call
Parameters
- sid string - A 34 character string that uniquely identifies this resource.
Return Type
deleteCallFeedbackSummary
Delete a FeedbackSummary resource from a call
Parameters
- sid string - A 34 character string that uniquely identifies this resource.
fetchCallNotification
function fetchCallNotification(string callSid, string sid, string? accountSid) returns CallCall_notificationInstance|error
Fetch call notifications for a call
Parameters
- sid string - The Twilio-provided string that uniquely identifies the Call Notification resource to fetch.
Return Type
listCallNotification
function listCallNotification(string callSid, int? log, string? messageDate, string? loggedAtOrBefore, string? loggedAtOrAfter, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListCallNotificationResponse|error
List Call Notification associated with a Call
Parameters
- log int? (default ()) - Only read notifications of the specified log level. Can be:
0
to read only ERROR notifications or1
to read only WARNING notifications. By default, all notifications are read.
- messageDate string? (default ()) - Only show notifications for the specified date, formatted as
YYYY-MM-DD
. You can also specify an inequality, such as<=YYYY-MM-DD
for messages logged at or before midnight on a date, or>=YYYY-MM-DD
for messages logged at or after midnight on a date.
- loggedAtOrBefore string? (default ()) - Only show notifications for the specified date, formatted as
YYYY-MM-DD
. You can also specify an inequality, such as<=YYYY-MM-DD
for messages logged at or before midnight on a date, or>=YYYY-MM-DD
for messages logged at or after midnight on a date.
- loggedAtOrAfter string? (default ()) - Only show notifications for the specified date, formatted as
YYYY-MM-DD
. You can also specify an inequality, such as<=YYYY-MM-DD
for messages logged at or before midnight on a date, or>=YYYY-MM-DD
for messages logged at or after midnight on a date.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
listCallRecording
function listCallRecording(string callSid, string? dateCreated, string? dateCreatedOnOrBefore, string? dateCreatedOnOrAfter, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListCallRecordingResponse|error
Retrieve a list of recordings belonging to the call used to make the request
Parameters
- dateCreated string? (default ()) - The
date_created
value, specified asYYYY-MM-DD
, of the resources to read. You can also specify inequality:DateCreated<=YYYY-MM-DD
will return recordings generated at or before midnight on a given date, andDateCreated>=YYYY-MM-DD
returns recordings generated at or after midnight on a date.
- dateCreatedOnOrBefore string? (default ()) - The
date_created
value, specified asYYYY-MM-DD
, of the resources to read. You can also specify inequality:DateCreated<=YYYY-MM-DD
will return recordings generated at or before midnight on a given date, andDateCreated>=YYYY-MM-DD
returns recordings generated at or after midnight on a date.
- dateCreatedOnOrAfter string? (default ()) - The
date_created
value, specified asYYYY-MM-DD
, of the resources to read. You can also specify inequality:DateCreated<=YYYY-MM-DD
will return recordings generated at or before midnight on a given date, andDateCreated>=YYYY-MM-DD
returns recordings generated at or after midnight on a date.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
createCallRecording
function createCallRecording(string callSid, CreateCallRecordingRequest payload, string? accountSid) returns CallCall_recording|error
Create a recording for the call
Parameters
- payload CreateCallRecordingRequest - The
CreateCallRecordingRequest
record should be used as a payload to create a recording.
Return Type
- CallCall_recording|error - Created
fetchCallRecording
function fetchCallRecording(string callSid, string sid, string? accountSid) returns CallCall_recording|error
Fetch an instance of a recording for a call
Parameters
- sid string - The Twilio-provided string that uniquely identifies the Recording resource to fetch.
Return Type
- CallCall_recording|error - OK
updateCallRecording
function updateCallRecording(string callSid, string sid, UpdateCallRecordingRequest payload, string? accountSid) returns CallCall_recording|error
Changes the status of the recording to paused, stopped, or in-progress. Note: Pass Twilio.CURRENT
instead of recording sid to reference current active recording.
Parameters
- sid string - The Twilio-provided string that uniquely identifies the Recording resource to update.
- payload UpdateCallRecordingRequest - The
UpdateCallRecordingRequest
record should be used as a payload to update a recording.
Return Type
- CallCall_recording|error - OK
deleteCallRecording
Delete a recording from your account
Parameters
- sid string - The Twilio-provided string that uniquely identifies the Recording resource to delete.
fetchConference
function fetchConference(string sid, string? accountSid) returns Conference|error
Fetch an instance of a conference
Parameters
- sid string - The Twilio-provided string that uniquely identifies the Conference resource to fetch
Return Type
- Conference|error - OK
updateConference
function updateConference(string sid, UpdateConferenceRequest payload, string? accountSid) returns Conference|error
Update a conference
Parameters
- sid string - The Twilio-provided string that uniquely identifies the Conference resource to update
- payload UpdateConferenceRequest - The
UpdateConferenceRequest
record should be used as a payload to update a conference.
Return Type
- Conference|error - OK
listConference
function listConference(string? dateCreated, string? dateCreatedOnOrBefore, string? dateCreatedOnOrAfter, string? dateUpdated, string? dateUpdatedOnOrBefore, string? dateUpdatedOnOrAfter, string? friendlyName, Conference_enum_status? status, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListConferenceResponse|error
Retrieve a list of conferences belonging to the account used to make the request
Parameters
- dateCreated string? (default ()) - The
date_created
value, specified asYYYY-MM-DD
, of the resources to read. To read conferences that started on or before midnight on a date, use<=YYYY-MM-DD
, and to specify conferences that started on or after midnight on a date, use>=YYYY-MM-DD
.
- dateCreatedOnOrBefore string? (default ()) - The
date_created
value, specified asYYYY-MM-DD
, of the resources to read. To read conferences that started on or before midnight on a date, use<=YYYY-MM-DD
, and to specify conferences that started on or after midnight on a date, use>=YYYY-MM-DD
.
- dateCreatedOnOrAfter string? (default ()) - The
date_created
value, specified asYYYY-MM-DD
, of the resources to read. To read conferences that started on or before midnight on a date, use<=YYYY-MM-DD
, and to specify conferences that started on or after midnight on a date, use>=YYYY-MM-DD
.
- dateUpdated string? (default ()) - The
date_updated
value, specified asYYYY-MM-DD
, of the resources to read. To read conferences that were last updated on or before midnight on a date, use<=YYYY-MM-DD
, and to specify conferences that were last updated on or after midnight on a given date, use>=YYYY-MM-DD
.
- dateUpdatedOnOrBefore string? (default ()) - The
date_updated
value, specified asYYYY-MM-DD
, of the resources to read. To read conferences that were last updated on or before midnight on a date, use<=YYYY-MM-DD
, and to specify conferences that were last updated on or after midnight on a given date, use>=YYYY-MM-DD
.
- dateUpdatedOnOrAfter string? (default ()) - The
date_updated
value, specified asYYYY-MM-DD
, of the resources to read. To read conferences that were last updated on or before midnight on a date, use<=YYYY-MM-DD
, and to specify conferences that were last updated on or after midnight on a given date, use>=YYYY-MM-DD
.
- friendlyName string? (default ()) - The string that identifies the Conference resources to read.
- status Conference_enum_status? (default ()) - The status of the resources to read. Can be:
init
,in-progress
, orcompleted
.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
fetchConferenceRecording
function fetchConferenceRecording(string conferenceSid, string sid, string? accountSid) returns ConferenceConference_recording|error
Fetch an instance of a recording for a call
Parameters
- conferenceSid string - The Conference SID that identifies the conference associated with the recording to fetch.
- sid string - The Twilio-provided string that uniquely identifies the Conference Recording resource to fetch.
Return Type
updateConferenceRecording
function updateConferenceRecording(string conferenceSid, string sid, UpdateConferenceRecordingRequest payload, string? accountSid) returns ConferenceConference_recording|error
Changes the status of the recording to paused, stopped, or in-progress. Note: To use Twilio.CURRENT
, pass it as recording sid.
Parameters
- conferenceSid string - The Conference SID that identifies the conference associated with the recording to update.
- sid string - The Twilio-provided string that uniquely identifies the Conference Recording resource to update. Use
Twilio.CURRENT
to reference the current active recording.
- payload UpdateConferenceRecordingRequest - The
UpdateConferenceRecordingRequest
record should be used as a payload to update a recording.
Return Type
deleteConferenceRecording
function deleteConferenceRecording(string conferenceSid, string sid, string? accountSid) returns Response|error
Delete a recording from your account
Parameters
- conferenceSid string - The Conference SID that identifies the conference associated with the recording to delete.
- sid string - The Twilio-provided string that uniquely identifies the Conference Recording resource to delete.
listConferenceRecording
function listConferenceRecording(string conferenceSid, string? dateCreated, string? dateCreatedOnOrBefore, string? dateCreatedOnOrAfter, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListConferenceRecordingResponse|error
Retrieve a list of recordings belonging to the call used to make the request
Parameters
- conferenceSid string - The Conference SID that identifies the conference associated with the recording to read.
- dateCreated string? (default ()) - The
date_created
value, specified asYYYY-MM-DD
, of the resources to read. You can also specify inequality:DateCreated<=YYYY-MM-DD
will return recordings generated at or before midnight on a given date, andDateCreated>=YYYY-MM-DD
returns recordings generated at or after midnight on a date.
- dateCreatedOnOrBefore string? (default ()) - The
date_created
value, specified asYYYY-MM-DD
, of the resources to read. You can also specify inequality:DateCreated<=YYYY-MM-DD
will return recordings generated at or before midnight on a given date, andDateCreated>=YYYY-MM-DD
returns recordings generated at or after midnight on a date.
- dateCreatedOnOrAfter string? (default ()) - The
date_created
value, specified asYYYY-MM-DD
, of the resources to read. You can also specify inequality:DateCreated<=YYYY-MM-DD
will return recordings generated at or before midnight on a given date, andDateCreated>=YYYY-MM-DD
returns recordings generated at or after midnight on a date.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
fetchConnectApp
function fetchConnectApp(string sid, string? accountSid) returns Connect_app|error
Fetch an instance of a connect-app
Parameters
- sid string - The Twilio-provided string that uniquely identifies the ConnectApp resource to fetch.
Return Type
- Connect_app|error - OK
updateConnectApp
function updateConnectApp(string sid, UpdateConnectAppRequest payload, string? accountSid) returns Connect_app|error
Update a connect-app with the specified parameters
Parameters
- sid string - The Twilio-provided string that uniquely identifies the ConnectApp resource to update.
- payload UpdateConnectAppRequest - The
UpdateConnectAppRequest
record should be used as a payload to update a connect-app.
Return Type
- Connect_app|error - OK
deleteConnectApp
Delete an instance of a connect-app
Parameters
- sid string - The Twilio-provided string that uniquely identifies the ConnectApp resource to fetch.
listConnectApp
function listConnectApp(int? pageSize, int? page, string? pageToken, string? accountSid) returns ListConnectAppResponse|error
Retrieve a list of connect-apps belonging to the account used to make the request
Parameters
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
listDependentPhoneNumber
function listDependentPhoneNumber(string addressSid, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListDependentPhoneNumberResponse|error
List dependent phone numbers for an address
Parameters
- addressSid string - The SID of the Address resource associated with the phone number.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
fetchIncomingPhoneNumber
function fetchIncomingPhoneNumber(string sid, string? accountSid) returns Incoming_phone_number|error
Fetch an incoming-phone-number belonging to the account used to make the request.
Parameters
- sid string - The Twilio-provided string that uniquely identifies the IncomingPhoneNumber resource to fetch.
Return Type
updateIncomingPhoneNumber
function updateIncomingPhoneNumber(string sid, UpdateIncomingPhoneNumberRequest payload, string? accountSid) returns Incoming_phone_number|error
Update an incoming-phone-number instance.
Parameters
- sid string - The Twilio-provided string that uniquely identifies the IncomingPhoneNumber resource to update.
- payload UpdateIncomingPhoneNumberRequest - The
UpdateIncomingPhoneNumberRequest
record should be used as a payload to update a phone number.
- accountSid string? (default ()) - The SID of the Account that created the IncomingPhoneNumber resource to update. For more information, see Exchanging Numbers Between Subaccounts.
Return Type
deleteIncomingPhoneNumber
Delete a phone-numbers belonging to the account used to make the request.
Parameters
- sid string - The Twilio-provided string that uniquely identifies the IncomingPhoneNumber resource to delete.
listIncomingPhoneNumber
function listIncomingPhoneNumber(boolean? beta, string? friendlyName, string? phoneNumber, string? origin, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListIncomingPhoneNumberResponse|error
Retrieve a list of incoming-phone-numbers belonging to the account used to make the request.
Parameters
- beta boolean? (default ()) - Whether to include phone numbers new to the Twilio platform. Can be:
true
orfalse
and the default istrue
.
- friendlyName string? (default ()) - A string that identifies the IncomingPhoneNumber resources to read.
- phoneNumber string? (default ()) - The phone numbers of the IncomingPhoneNumber resources to read. You can specify partial numbers and use '*' as a wildcard for any digit.
- origin string? (default ()) - Whether to include phone numbers based on their origin. Can be:
twilio
orhosted
. By default, phone numbers of all origin are included.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
createIncomingPhoneNumber
function createIncomingPhoneNumber(CreateIncomingPhoneNumberRequest payload, string? accountSid) returns Incoming_phone_number|error
Purchase a phone-number for the account.
Parameters
- payload CreateIncomingPhoneNumberRequest - The
CreateIncomingPhoneNumberRequest
record should be used as a payload to purchase a phone number.
Return Type
- Incoming_phone_number|error - Created
fetchIncomingPhoneNumberAssignedAddOn
function fetchIncomingPhoneNumberAssignedAddOn(string resourceSid, string sid, string? accountSid) returns Incoming_phone_numberIncoming_phone_number_assigned_add_on|error
Fetch an instance of an Add-on installation currently assigned to this Number.
Parameters
- resourceSid string - The SID of the Phone Number to which the Add-on is assigned.
- sid string - The Twilio-provided string that uniquely identifies the resource to fetch.
Return Type
deleteIncomingPhoneNumberAssignedAddOn
function deleteIncomingPhoneNumberAssignedAddOn(string resourceSid, string sid, string? accountSid) returns Response|error
Remove the assignment of an Add-on installation from the Number specified.
Parameters
- resourceSid string - The SID of the Phone Number to which the Add-on is assigned.
- sid string - The Twilio-provided string that uniquely identifies the resource to delete.
listIncomingPhoneNumberAssignedAddOn
function listIncomingPhoneNumberAssignedAddOn(string resourceSid, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListIncomingPhoneNumberAssignedAddOnResponse|error
Retrieve a list of Add-on installations currently assigned to this Number.
Parameters
- resourceSid string - The SID of the Phone Number to which the Add-on is assigned.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
createIncomingPhoneNumberAssignedAddOn
function createIncomingPhoneNumberAssignedAddOn(string resourceSid, CreateIncomingPhoneNumberAssignedAddOnRequest payload, string? accountSid) returns Incoming_phone_numberIncoming_phone_number_assigned_add_on|error
Assign an Add-on installation to the Number specified.
Parameters
- resourceSid string - The SID of the Phone Number to assign the Add-on.
- payload CreateIncomingPhoneNumberAssignedAddOnRequest - The
CreateIncomingPhoneNumberAssignedAddOnRequest
record should be used as a payload to crete an incoming phone number assigned add-on.
Return Type
fetchIncomingPhoneNumberAssignedAddOnExtension
function fetchIncomingPhoneNumberAssignedAddOnExtension(string resourceSid, string assignedAddOnSid, string sid, string? accountSid) returns Incoming_phone_numberIncoming_phone_number_assigned_add_onIncoming_phone_number_assigned_add_on_extension|error
Fetch an instance of an Extension for the Assigned Add-on.
Parameters
- resourceSid string - The SID of the Phone Number to which the Add-on is assigned.
- assignedAddOnSid string - The SID that uniquely identifies the assigned Add-on installation.
- sid string - The Twilio-provided string that uniquely identifies the resource to fetch.
Return Type
listIncomingPhoneNumberAssignedAddOnExtension
function listIncomingPhoneNumberAssignedAddOnExtension(string resourceSid, string assignedAddOnSid, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListIncomingPhoneNumberAssignedAddOnExtensionResponse|error
Retrieve a list of Extensions for the Assigned Add-on.
Parameters
- resourceSid string - The SID of the Phone Number to which the Add-on is assigned.
- assignedAddOnSid string - The SID that uniquely identifies the assigned Add-on installation.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
listIncomingPhoneNumberLocal
function listIncomingPhoneNumberLocal(boolean? beta, string? friendlyName, string? phoneNumber, string? origin, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListIncomingPhoneNumberLocalResponse|error
List incoming-phone-numbers local for an address
Parameters
- beta boolean? (default ()) - Whether to include phone numbers new to the Twilio platform. Can be:
true
orfalse
and the default istrue
.
- friendlyName string? (default ()) - A string that identifies the resources to read.
- phoneNumber string? (default ()) - The phone numbers of the IncomingPhoneNumber resources to read. You can specify partial numbers and use '*' as a wildcard for any digit.
- origin string? (default ()) - Whether to include phone numbers based on their origin. Can be:
twilio
orhosted
. By default, phone numbers of all origin are included.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
createIncomingPhoneNumberLocal
function createIncomingPhoneNumberLocal(CreateIncomingPhoneNumberLocalRequest payload, string? accountSid) returns Incoming_phone_numberIncoming_phone_number_local|error
Create a phone-number for the account.
Parameters
- payload CreateIncomingPhoneNumberLocalRequest - The
CreateIncomingPhoneNumberLocalRequest
record should be used as a payload to purchase a phone number.
Return Type
listIncomingPhoneNumberMobile
function listIncomingPhoneNumberMobile(boolean? beta, string? friendlyName, string? phoneNumber, string? origin, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListIncomingPhoneNumberMobileResponse|error
List incoming-phone-numbers mobile for an address
Parameters
- beta boolean? (default ()) - Whether to include phone numbers new to the Twilio platform. Can be:
true
orfalse
and the default istrue
.
- friendlyName string? (default ()) - A string that identifies the resources to read.
- phoneNumber string? (default ()) - The phone numbers of the IncomingPhoneNumber resources to read. You can specify partial numbers and use '*' as a wildcard for any digit.
- origin string? (default ()) - Whether to include phone numbers based on their origin. Can be:
twilio
orhosted
. By default, phone numbers of all origin are included.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
createIncomingPhoneNumberMobile
function createIncomingPhoneNumberMobile(CreateIncomingPhoneNumberMobileRequest payload, string? accountSid) returns Incoming_phone_numberIncoming_phone_number_mobile|error
Create a phone-number for the account.
Parameters
- payload CreateIncomingPhoneNumberMobileRequest - The
CreateIncomingPhoneNumberMobileRequest
record should be used as a payload to purchase a phone number.
Return Type
listIncomingPhoneNumberTollFree
function listIncomingPhoneNumberTollFree(boolean? beta, string? friendlyName, string? phoneNumber, string? origin, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListIncomingPhoneNumberTollFreeResponse|error
List incoming-phone-numbers troll-free for an address
Parameters
- beta boolean? (default ()) - Whether to include phone numbers new to the Twilio platform. Can be:
true
orfalse
and the default istrue
.
- friendlyName string? (default ()) - A string that identifies the resources to read.
- phoneNumber string? (default ()) - The phone numbers of the IncomingPhoneNumber resources to read. You can specify partial numbers and use '*' as a wildcard for any digit.
- origin string? (default ()) - Whether to include phone numbers based on their origin. Can be:
twilio
orhosted
. By default, phone numbers of all origin are included.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
createIncomingPhoneNumberTollFree
function createIncomingPhoneNumberTollFree(CreateIncomingPhoneNumberTollFreeRequest payload, string? accountSid) returns Incoming_phone_numberIncoming_phone_number_toll_free|error
Create a phone-number for the account.
Parameters
- payload CreateIncomingPhoneNumberTollFreeRequest - The
CreateIncomingPhoneNumberTollFreeRequest
record should be used as a payload to purchase a phone number.
Return Type
fetchKey
Fetch key resource
Parameters
- sid string - The Twilio-provided string that uniquely identifies the Key resource to fetch.
updateKey
function updateKey(string sid, UpdateKeyRequest payload, string? accountSid) returns Key|error
Update a Key with the specified parameters
Parameters
- sid string - The Twilio-provided string that uniquely identifies the Key resource to update.
- payload UpdateKeyRequest - The
UpdateKeyRequest
record should be used as a payload to update a key.
deleteKey
Delete a Key resource
Parameters
- sid string - The Twilio-provided string that uniquely identifies the Key resource to delete.
listKey
function listKey(int? pageSize, int? page, string? pageToken, string? accountSid) returns ListKeyResponse|error
List of Keys resources.
Parameters
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
- ListKeyResponse|error - OK
createNewKey
function createNewKey(CreateNewKeyRequest payload, string? accountSid) returns New_key|error
Create a Key for the account.
Parameters
- payload CreateNewKeyRequest - The
CreateKeyRequest
record should be used as a payload to create a key.
fetchMedia
function fetchMedia(string messageSid, string sid, string? accountSid) returns MessageMedia|error
Fetch a single Media resource associated with a specific Message resource
Parameters
- messageSid string - The SID of the Message resource that is associated with the Media resource.
- sid string - The Twilio-provided string that uniquely identifies the Media resource to fetch.
Return Type
- MessageMedia|error - OK
deleteMedia
Delete the Media resource.
Parameters
- messageSid string - The SID of the Message resource that is associated with the Media resource.
- sid string - The unique identifier of the to-be-deleted Media resource.
listMedia
function listMedia(string messageSid, string? dateCreated, string? dateCreatedOnOrBefore, string? dateCreatedOnOrAfter, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListMediaResponse|error
Read a list of Media resources associated with a specific Message resource
Parameters
- messageSid string - The SID of the Message resource that is associated with the Media resources.
- dateCreated string? (default ()) - Only include Media resources that were created on this date. Specify a date as
YYYY-MM-DD
in GMT, for example:2009-07-06
, to read Media that were created on this date. You can also specify an inequality, such asStartTime<=YYYY-MM-DD
, to read Media that were created on or before midnight of this date, andStartTime>=YYYY-MM-DD
to read Media that were created on or after midnight of this date.
- dateCreatedOnOrBefore string? (default ()) - Only include Media resources that were created on this date. Specify a date as
YYYY-MM-DD
in GMT, for example:2009-07-06
, to read Media that were created on this date. You can also specify an inequality, such asStartTime<=YYYY-MM-DD
, to read Media that were created on or before midnight of this date, andStartTime>=YYYY-MM-DD
to read Media that were created on or after midnight of this date.
- dateCreatedOnOrAfter string? (default ()) - Only include Media resources that were created on this date. Specify a date as
YYYY-MM-DD
in GMT, for example:2009-07-06
, to read Media that were created on this date. You can also specify an inequality, such asStartTime<=YYYY-MM-DD
, to read Media that were created on or before midnight of this date, andStartTime>=YYYY-MM-DD
to read Media that were created on or after midnight of this date.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
- ListMediaResponse|error - OK
fetchMember
function fetchMember(string queueSid, string callSid, string? accountSid) returns QueueMember|error
Fetch a specific member from the queue
Parameters
- queueSid string - The SID of the Queue in which to find the members to fetch.
Return Type
- QueueMember|error - OK
updateMember
function updateMember(string queueSid, string callSid, UpdateMemberRequest payload, string? accountSid) returns QueueMember|error
Dequeue a member from a queue and have the member's call begin executing the TwiML document at that URL
Parameters
- queueSid string - The SID of the Queue in which to find the members to update.
- payload UpdateMemberRequest - The
UpdateMemberRequest
record should be used as a payload to update a member.
Return Type
- QueueMember|error - OK
listMember
function listMember(string queueSid, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListMemberResponse|error
Retrieve the members of the queue
Parameters
- queueSid string - The SID of the Queue in which to find the members
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
- ListMemberResponse|error - OK
listMessage
function listMessage(string? to, string? 'from, string? dateSent, string? dateSentOnOrBefore, string? dateSentOnOrAfter, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListMessageResponse|error
Retrieve a list of Message resources associated with a Twilio Account
Parameters
- to string? (default ()) - Filter by recipient. For example: Set this
to
parameter to+15558881111
to retrieve a list of Message resources withto
properties of+15558881111
- 'from string? (default ()) - Filter by sender. For example: Set this
from
parameter to+15552229999
to retrieve a list of Message resources withfrom
properties of+15552229999
- dateSent string? (default ()) - Filter by Message
sent_date
. Accepts GMT dates in the following formats:YYYY-MM-DD
(to find Messages with a specificsent_date
),<=YYYY-MM-DD
(to find Messages withsent_date
s on and before a specific date), and>=YYYY-MM-DD
(to find Messages withsent_dates
on and after a specific date).
- dateSentOnOrBefore string? (default ()) - Filter by Message
sent_date
. Accepts GMT dates in the following formats:YYYY-MM-DD
(to find Messages with a specificsent_date
),<=YYYY-MM-DD
(to find Messages withsent_date
s on and before a specific date), and>=YYYY-MM-DD
(to find Messages withsent_dates
on and after a specific date).
- dateSentOnOrAfter string? (default ()) - Filter by Message
sent_date
. Accepts GMT dates in the following formats:YYYY-MM-DD
(to find Messages with a specificsent_date
),<=YYYY-MM-DD
(to find Messages withsent_date
s on and before a specific date), and>=YYYY-MM-DD
(to find Messages withsent_dates
on and after a specific date).
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
- ListMessageResponse|error - OK
createMessage
function createMessage(CreateMessageRequest payload, string? accountSid) returns Message|error
Send a message
Parameters
- payload CreateMessageRequest - The
CreateMessageRequest
record should be used as a payload to send a message.
fetchMessage
Fetch a specific Message
Parameters
- sid string - The SID of the Message resource to be fetched
updateMessage
function updateMessage(string sid, UpdateMessageRequest payload, string? accountSid) returns Message|error
Update a Message resource (used to redact Message body
text and to cancel not-yet-sent messages)
Parameters
- sid string - The SID of the Message resource to be updated.
- payload UpdateMessageRequest - The
UpdateMessageRequest
record should be used as a payload to update a message.
deleteMessage
Deletes a Message resource from your account
Parameters
- sid string - The SID of the Message resource you wish to delete
createMessageFeedback
function createMessageFeedback(string messageSid, CreateMessageFeedbackRequest payload, string? accountSid) returns MessageMessage_feedback|error
Create Message Feedback to confirm a tracked user action was performed by the recipient of the associated Message
Parameters
- messageSid string - The SID of the Message resource for which to create MessageFeedback.
- payload CreateMessageFeedbackRequest - The
CreateMessageFeedbackRequest
record should be used as a payload to create MessageFeedback.
Return Type
- MessageMessage_feedback|error - Created
listSigningKey
function listSigningKey(int? pageSize, int? page, string? pageToken, string? accountSid) returns ListSigningKeyResponse|error
Fetch a specific MessageFeedback
Parameters
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
createNewSigningKey
function createNewSigningKey(CreateNewSigningKeyRequest payload, string? accountSid) returns New_signing_key|error
Create a new Signing Key for the account making the request.
Parameters
- payload CreateNewSigningKeyRequest - The
CreateNewSigningKeyRequest
record should be used as a payload to create a signing key.
Return Type
- New_signing_key|error - Created
fetchNotification
function fetchNotification(string sid, string? accountSid) returns NotificationInstance|error
Fetch a notification belonging to the account used to make the request
Parameters
- sid string - The Twilio-provided string that uniquely identifies the Notification resource to fetch.
Return Type
listNotification
function listNotification(int? log, string? messageDate, string? loggedAtOrBefore, string? loggedAtOrAfter, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListNotificationResponse|error
Retrieve a list of notifications belonging to the account used to make the request
Parameters
- log int? (default ()) - Only read notifications of the specified log level. Can be:
0
to read only ERROR notifications or1
to read only WARNING notifications. By default, all notifications are read.
- messageDate string? (default ()) - Only show notifications for the specified date, formatted as
YYYY-MM-DD
. You can also specify an inequality, such as<=YYYY-MM-DD
for messages logged at or before midnight on a date, or>=YYYY-MM-DD
for messages logged at or after midnight on a date.
- loggedAtOrBefore string? (default ()) - Only show notifications for the specified date, formatted as
YYYY-MM-DD
. You can also specify an inequality, such as<=YYYY-MM-DD
for messages logged at or before midnight on a date, or>=YYYY-MM-DD
for messages logged at or after midnight on a date.
- loggedAtOrAfter string? (default ()) - Only show notifications for the specified date, formatted as
YYYY-MM-DD
. You can also specify an inequality, such as<=YYYY-MM-DD
for messages logged at or before midnight on a date, or>=YYYY-MM-DD
for messages logged at or after midnight on a date.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
fetchOutgoingCallerId
function fetchOutgoingCallerId(string sid, string? accountSid) returns Outgoing_caller_id|error
Fetch an outgoing-caller-id belonging to the account used to make the request
Parameters
- sid string - The Twilio-provided string that uniquely identifies the OutgoingCallerId resource to fetch.
Return Type
- Outgoing_caller_id|error - OK
updateOutgoingCallerId
function updateOutgoingCallerId(string sid, UpdateOutgoingCallerIdRequest payload, string? accountSid) returns Outgoing_caller_id|error
Updates the caller-id
Parameters
- sid string - The Twilio-provided string that uniquely identifies the OutgoingCallerId resource to update.
- payload UpdateOutgoingCallerIdRequest - The
UpdateOutgoingCallerIdRequest
record should be used as a payload to update an outgoing caller id.
Return Type
- Outgoing_caller_id|error - OK
deleteOutgoingCallerId
Delete the caller-id specified from the account
Parameters
- sid string - The Twilio-provided string that uniquely identifies the OutgoingCallerId resource to delete.
listOutgoingCallerId
function listOutgoingCallerId(string? phoneNumber, string? friendlyName, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListOutgoingCallerIdResponse|error
Retrieve a list of outgoing-caller-ids belonging to the account used to make the request
Parameters
- phoneNumber string? (default ()) - The phone number of the OutgoingCallerId resources to read.
- friendlyName string? (default ()) - The string that identifies the OutgoingCallerId resources to read.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
createValidationRequest
function createValidationRequest(CreateValidationRequest payload, string? accountSid) returns Validation_request|error
Create a new outgoing-caller-id for the account.
Parameters
- payload CreateValidationRequest - The
CreateOutgoingCallerIdRequest
record should be used as a payload to create an outgoing caller id.
Return Type
- Validation_request|error - Created
fetchParticipant
function fetchParticipant(string conferenceSid, string callSid, string? accountSid) returns ConferenceParticipant|error
Fetch an instance of a participant
Return Type
updateParticipant
function updateParticipant(string conferenceSid, string callSid, UpdateParticipantRequest payload, string? accountSid) returns ConferenceParticipant|error
Update the properties of the participant
Parameters
- conferenceSid string - The SID of the conference with the participant to update.
- payload UpdateParticipantRequest - The
UpdateParticipantRequest
record should be used as a payload to update a participant.
Return Type
deleteParticipant
function deleteParticipant(string conferenceSid, string callSid, string? accountSid) returns Response|error
Kick a participant from a given conference
listParticipant
function listParticipant(string conferenceSid, boolean? muted, boolean? hold, boolean? coaching, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListParticipantResponse|error
Retrieve a list of participants belonging to the account used to make the request
Parameters
- conferenceSid string - The SID of the conference with the participants to read.
- muted boolean? (default ()) - Whether to return only participants that are muted. Can be:
true
orfalse
.
- hold boolean? (default ()) - Whether to return only participants that are on hold. Can be:
true
orfalse
.
- coaching boolean? (default ()) - Whether to return only participants who are coaching another call. Can be:
true
orfalse
.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
createParticipant
function createParticipant(string conferenceSid, CreateParticipantRequest payload, string? accountSid) returns ConferenceParticipant|error
Create a participant in a conference
Parameters
- conferenceSid string - The SID of the participant's conference.
- payload CreateParticipantRequest - The
CreateParticipantRequest
record should be used as a payload to create a participant.
Return Type
- ConferenceParticipant|error - Created
createPayments
function createPayments(string callSid, CreatePaymentsRequest payload, string? accountSid) returns CallPayments|error
create an instance of payments. This will start a new payments session
Parameters
- callSid string - The SID of the call that will create the resource. Call leg associated with this sid is expected to provide payment information thru DTMF.
- payload CreatePaymentsRequest - The
CreatePaymentsRequest
record should be used as a payload to create a payments resource.
Return Type
- CallPayments|error - Created
updatePayments
function updatePayments(string callSid, string sid, UpdatePaymentsRequest payload, string? accountSid) returns CallPayments|error
update an instance of payments with different phases of payment flows.
Parameters
- callSid string - The SID of the call that will update the resource. This should be the same call sid that was used to create payments resource.
- sid string - The SID of Payments session that needs to be updated.
- payload UpdatePaymentsRequest - The
UpdatePaymentsRequest
record should be used as a payload to update a payments resource.
Return Type
- CallPayments|error - Accepted
fetchQueue
Fetch an instance of a queue identified by the QueueSid
Parameters
- sid string - The Twilio-provided string that uniquely identifies the Queue resource to fetch
updateQueue
function updateQueue(string sid, UpdateQueueRequest payload, string? accountSid) returns Queue|error
Update the queue with the new parameters
Parameters
- sid string - The Twilio-provided string that uniquely identifies the Queue resource to update
- payload UpdateQueueRequest - The
UpdateQueueRequest
record should be used as a payload to update a queue.
deleteQueue
Remove an empty queue
Parameters
- sid string - The Twilio-provided string that uniquely identifies the Queue resource to delete
listQueue
function listQueue(int? pageSize, int? page, string? pageToken, string? accountSid) returns ListQueueResponse|error
Retrieve a list of queues belonging to the account used to make the request
Parameters
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
- ListQueueResponse|error - OK
createQueue
function createQueue(CreateQueueRequest payload, string? accountSid) returns Queue|error
Create a queue
Parameters
- payload CreateQueueRequest - The
CreateQueueRequest
record should be used as a payload to create a queue.
fetchRecording
function fetchRecording(string sid, boolean? includeSoftDeleted, string? accountSid) returns Recording|error
Fetch an instance of a recording
Parameters
- sid string - The Twilio-provided string that uniquely identifies the Recording resource to fetch.
- includeSoftDeleted boolean? (default ()) - A boolean parameter indicating whether to retrieve soft deleted recordings or not. Recordings metadata are kept after deletion for a retention period of 40 days.
deleteRecording
Delete a recording from your account
Parameters
- sid string - The Twilio-provided string that uniquely identifies the Recording resource to delete.
listRecording
function listRecording(string? dateCreated, string? dateCreatedOnOrBefore, string? dateCreatedOnOrAfter, string? callSid, string? conferenceSid, boolean? includeSoftDeleted, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListRecordingResponse|error
Retrieve a list of recordings belonging to the account used to make the request
Parameters
- dateCreated string? (default ()) - Only include recordings that were created on this date. Specify a date as
YYYY-MM-DD
in GMT, for example:2009-07-06
, to read recordings that were created on this date. You can also specify an inequality, such asDateCreated<=YYYY-MM-DD
, to read recordings that were created on or before midnight of this date, andDateCreated>=YYYY-MM-DD
to read recordings that were created on or after midnight of this date.
- dateCreatedOnOrBefore string? (default ()) - Only include recordings that were created on this date. Specify a date as
YYYY-MM-DD
in GMT, for example:2009-07-06
, to read recordings that were created on this date. You can also specify an inequality, such asDateCreated<=YYYY-MM-DD
, to read recordings that were created on or before midnight of this date, andDateCreated>=YYYY-MM-DD
to read recordings that were created on or after midnight of this date.
- dateCreatedOnOrAfter string? (default ()) - Only include recordings that were created on this date. Specify a date as
YYYY-MM-DD
in GMT, for example:2009-07-06
, to read recordings that were created on this date. You can also specify an inequality, such asDateCreated<=YYYY-MM-DD
, to read recordings that were created on or before midnight of this date, andDateCreated>=YYYY-MM-DD
to read recordings that were created on or after midnight of this date.
- conferenceSid string? (default ()) - The Conference SID that identifies the conference associated with the recording to read.
- includeSoftDeleted boolean? (default ()) - A boolean parameter indicating whether to retrieve soft deleted recordings or not. Recordings metadata are kept after deletion for a retention period of 40 days.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
fetchRecordingAddOnResult
function fetchRecordingAddOnResult(string referenceSid, string sid, string? accountSid) returns RecordingRecording_add_on_result|error
Fetch an instance of an AddOnResult
Parameters
- referenceSid string - The SID of the recording to which the result to fetch belongs.
- sid string - The Twilio-provided string that uniquely identifies the Recording AddOnResult resource to fetch.
Return Type
deleteRecordingAddOnResult
function deleteRecordingAddOnResult(string referenceSid, string sid, string? accountSid) returns Response|error
Delete a result and purge all associated Payloads
Parameters
- referenceSid string - The SID of the recording to which the result to delete belongs.
- sid string - The Twilio-provided string that uniquely identifies the Recording AddOnResult resource to delete.
listRecordingAddOnResult
function listRecordingAddOnResult(string referenceSid, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListRecordingAddOnResultResponse|error
Retrieve a list of results belonging to the recording
Parameters
- referenceSid string - The SID of the recording to which the result to read belongs.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
fetchRecordingAddOnResultPayload
function fetchRecordingAddOnResultPayload(string referenceSid, string addOnResultSid, string sid, string? accountSid) returns RecordingRecording_add_on_resultRecording_add_on_result_payload|error
Fetch an instance of a result payload
Parameters
- referenceSid string - The SID of the recording to which the AddOnResult resource that contains the payload to fetch belongs.
- addOnResultSid string - The SID of the AddOnResult to which the payload to fetch belongs.
- sid string - The Twilio-provided string that uniquely identifies the Recording AddOnResult Payload resource to fetch.
Return Type
deleteRecordingAddOnResultPayload
function deleteRecordingAddOnResultPayload(string referenceSid, string addOnResultSid, string sid, string? accountSid) returns Response|error
Delete a payload from the result along with all associated Data
Parameters
- referenceSid string - The SID of the recording to which the AddOnResult resource that contains the payloads to delete belongs.
- addOnResultSid string - The SID of the AddOnResult to which the payloads to delete belongs.
- sid string - The Twilio-provided string that uniquely identifies the Recording AddOnResult Payload resource to delete.
listRecordingAddOnResultPayload
function listRecordingAddOnResultPayload(string referenceSid, string addOnResultSid, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListRecordingAddOnResultPayloadResponse|error
Retrieve a list of payloads belonging to the AddOnResult
Parameters
- referenceSid string - The SID of the recording to which the AddOnResult resource that contains the payloads to read belongs.
- addOnResultSid string - The SID of the AddOnResult to which the payloads to read belongs.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
fetchRecordingTranscription
function fetchRecordingTranscription(string recordingSid, string sid, string? accountSid) returns RecordingRecording_transcription|error
Fetch an instance of a recording transcription
Parameters
- sid string - The Twilio-provided string that uniquely identifies the Transcription resource to fetch.
Return Type
deleteRecordingTranscription
function deleteRecordingTranscription(string recordingSid, string sid, string? accountSid) returns Response|error
Delete a transcription from your account
Parameters
- sid string - The Twilio-provided string that uniquely identifies the Transcription resource to delete.
listRecordingTranscription
function listRecordingTranscription(string recordingSid, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListRecordingTranscriptionResponse|error
Retrieve a list of transcriptions belonging to the recording
Parameters
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
fetchShortCode
function fetchShortCode(string sid, string? accountSid) returns Short_code|error
Fetch an instance of a short code
Parameters
- sid string - The Twilio-provided string that uniquely identifies the ShortCode resource to fetch
Return Type
- Short_code|error - OK
updateShortCode
function updateShortCode(string sid, UpdateShortCodeRequest payload, string? accountSid) returns Short_code|error
Update a short code with the following parameters
Parameters
- sid string - The Twilio-provided string that uniquely identifies the ShortCode resource to update.
- payload UpdateShortCodeRequest - The
UpdateShortCodeRequest
record should be used as a payload to update a short code.
Return Type
- Short_code|error - OK
listShortCode
function listShortCode(string? friendlyName, string? shortCode, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListShortCodeResponse|error
Retrieve a list of short-codes belonging to the account used to make the request
Parameters
- friendlyName string? (default ()) - The string that identifies the ShortCode resources to read.
- shortCode string? (default ()) - Only show the ShortCode resources that match this pattern. You can specify partial numbers and use '*' as a wildcard for any digit.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
fetchSigningKey
function fetchSigningKey(string sid, string? accountSid) returns Signing_key|error
Create a short code
Parameters
- sid string - The Twilio-provided string that uniquely identifies the ShortCode resource to update.
Return Type
- Signing_key|error - OK
updateSigningKey
function updateSigningKey(string sid, UpdateSigningKeyRequest payload, string? accountSid) returns Signing_key|error
Update a signing key with the following parameters
Parameters
- sid string - The Twilio-provided string that uniquely identifies the SigningKey resource to update.
- payload UpdateSigningKeyRequest - The
UpdateSigningKeyRequest
record should be used as a payload to update a signing key.
Return Type
- Signing_key|error - OK
deleteSigningKey
Delete a signing key from the account
Parameters
- sid string - The Twilio-provided string that uniquely identifies the SigningKey resource to delete.
listSipAuthCallsCredentialListMapping
function listSipAuthCallsCredentialListMapping(string domainSid, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListSipAuthCallsCredentialListMappingResponse|error
Retrieve a list of credential list mappings belonging to the domain used in the request
Parameters
- domainSid string - The SID of the SIP domain that contains the resources to read.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
createSipAuthCallsCredentialListMapping
function createSipAuthCallsCredentialListMapping(string domainSid, CreateSipAuthCallsCredentialListMappingRequest payload, string? accountSid) returns SipSip_domainSip_authSip_auth_callsSip_auth_calls_credential_list_mapping|error
Create a new credential list mapping resource
Parameters
- domainSid string - The SID of the SIP domain that will contain the new resource.
- payload CreateSipAuthCallsCredentialListMappingRequest - The
CreateSipAuthCallsCredentialListMappingRequest
record should be used as a payload to create a credential list mapping.
Return Type
fetchSipAuthCallsCredentialListMapping
function fetchSipAuthCallsCredentialListMapping(string domainSid, string sid, string? accountSid) returns SipSip_domainSip_authSip_auth_callsSip_auth_calls_credential_list_mapping|error
Fetch a specific instance of a credential list mapping
Parameters
- domainSid string - The SID of the SIP domain that contains the resource to fetch.
- sid string - The Twilio-provided string that uniquely identifies the CredentialListMapping resource to fetch.
deleteSipAuthCallsCredentialListMapping
function deleteSipAuthCallsCredentialListMapping(string domainSid, string sid, string? accountSid) returns Response|error
Delete a credential list mapping from the requested domain
Parameters
- domainSid string - The SID of the SIP domain that contains the resource to delete.
- sid string - The Twilio-provided string that uniquely identifies the CredentialListMapping resource to delete.
listSipAuthCallsIpAccessControlListMapping
function listSipAuthCallsIpAccessControlListMapping(string domainSid, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListSipAuthCallsIpAccessControlListMappingResponse|error
Retrieve a list of IP Access Control List mappings belonging to the domain used in the request
Parameters
- domainSid string - The SID of the SIP domain that contains the resources to read.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
createSipAuthCallsIpAccessControlListMapping
function createSipAuthCallsIpAccessControlListMapping(string domainSid, CreateSipAuthCallsIpAccessControlListMappingRequest payload, string? accountSid) returns SipSip_domainSip_authSip_auth_callsSip_auth_calls_ip_access_control_list_mapping|error
Create a new IP Access Control List mapping
Parameters
- domainSid string - The SID of the SIP domain that will contain the new resource.
- payload CreateSipAuthCallsIpAccessControlListMappingRequest - The
CreateSipAuthCallsIpAccessControlListMappingRequest
record should be used as a payload to create a IP Access Control List mapping.
Return Type
fetchSipAuthCallsIpAccessControlListMapping
function fetchSipAuthCallsIpAccessControlListMapping(string domainSid, string sid, string? accountSid) returns SipSip_domainSip_authSip_auth_callsSip_auth_calls_ip_access_control_list_mapping|error
Fetch a specific instance of an IP Access Control List mapping
Parameters
- domainSid string - The SID of the SIP domain that contains the resource to fetch.
- sid string - The Twilio-provided string that uniquely identifies the IpAccessControlListMapping resource to fetch.
Return Type
deleteSipAuthCallsIpAccessControlListMapping
function deleteSipAuthCallsIpAccessControlListMapping(string domainSid, string sid, string? accountSid) returns Response|error
Delete an IP Access Control List mapping from the requested domain
Parameters
- domainSid string - The SID of the SIP domain that contains the resources to delete.
- sid string - The Twilio-provided string that uniquely identifies the IpAccessControlListMapping resource to delete.
listSipAuthRegistrationsCredentialListMapping
function listSipAuthRegistrationsCredentialListMapping(string domainSid, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListSipAuthRegistrationsCredentialListMappingResponse|error
Retrieve a list of credential list mappings belonging to the domain used in the request
Parameters
- domainSid string - The SID of the SIP domain that contains the resources to read.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
createSipAuthRegistrationsCredentialListMapping
function createSipAuthRegistrationsCredentialListMapping(string domainSid, CreateSipAuthRegistrationsCredentialListMappingRequest payload, string? accountSid) returns SipSip_domainSip_authSip_auth_registrationsSip_auth_registrations_credential_list_mapping|error
Create a new credential list mapping resource
Parameters
- domainSid string - The SID of the SIP domain that will contain the new resource.
- payload CreateSipAuthRegistrationsCredentialListMappingRequest - The
CreateSipAuthRegistrationsCredentialListMappingRequest
record should be used as a payload to create a credential list mapping.
Return Type
fetchSipAuthRegistrationsCredentialListMapping
function fetchSipAuthRegistrationsCredentialListMapping(string domainSid, string sid, string? accountSid) returns SipSip_domainSip_authSip_auth_registrationsSip_auth_registrations_credential_list_mapping|error
Fetch a specific instance of a credential list mapping
Parameters
- domainSid string - The SID of the SIP domain that contains the resource to fetch.
- sid string - The Twilio-provided string that uniquely identifies the CredentialListMapping resource to fetch.
Return Type
deleteSipAuthRegistrationsCredentialListMapping
function deleteSipAuthRegistrationsCredentialListMapping(string domainSid, string sid, string? accountSid) returns Response|error
Delete a credential list mapping from the requested domain
Parameters
- domainSid string - The SID of the SIP domain that contains the resources to delete.
- sid string - The Twilio-provided string that uniquely identifies the CredentialListMapping resource to delete.
listSipCredential
function listSipCredential(string credentialListSid, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListSipCredentialResponse|error
Retrieve a list of credentials.
Parameters
- credentialListSid string - The unique id that identifies the credential list that contains the desired credentials.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
- accountSid string? (default ()) - The unique id of the Account that is responsible for this resource.
Return Type
createSipCredential
function createSipCredential(string credentialListSid, CreateSipCredentialRequest payload, string? accountSid) returns SipSip_credential_listSip_credential|error
Create a new credential resource.
Parameters
- credentialListSid string - The unique id that identifies the credential list to include the created credential.
- payload CreateSipCredentialRequest - The
CreateSipCredentialRequest
record should be used as a payload to create a credential.
- accountSid string? (default ()) - The unique id of the Account that is responsible for this resource.
Return Type
- SipSip_credential_listSip_credential|error - Created
fetchSipCredential
function fetchSipCredential(string credentialListSid, string sid, string? accountSid) returns SipSip_credential_listSip_credential|error
Fetch a single credential.
Parameters
- credentialListSid string - The unique id that identifies the credential list that contains the desired credential.
- sid string - The unique id that identifies the resource to fetch.
- accountSid string? (default ()) - The unique id of the Account that is responsible for this resource.
Return Type
updateSipCredential
function updateSipCredential(string credentialListSid, string sid, UpdateSipCredentialRequest payload, string? accountSid) returns SipSip_credential_listSip_credential|error
Update a credential resource.
Parameters
- credentialListSid string - The unique id that identifies the credential list that includes this credential.
- sid string - The unique id that identifies the resource to update.
- payload UpdateSipCredentialRequest - The
UpdateSipCredentialRequest
record should be used as a payload to update a credential.
- accountSid string? (default ()) - The unique id of the Account that is responsible for this resource.
Return Type
deleteSipCredential
function deleteSipCredential(string credentialListSid, string sid, string? accountSid) returns Response|error
Delete a credential resource.
Parameters
- credentialListSid string - The unique id that identifies the credential list that contains the desired credentials.
- sid string - The unique id that identifies the resource to delete.
- accountSid string? (default ()) - The unique id of the Account that is responsible for this resource.
listSipCredentialList
function listSipCredentialList(int? pageSize, int? page, string? pageToken, string? accountSid) returns ListSipCredentialListResponse|error
Get All Credential Lists
Parameters
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
- accountSid string? (default ()) - The unique id of the Account that is responsible for this resource.
Return Type
createSipCredentialList
function createSipCredentialList(CreateSipCredentialListRequest payload, string? accountSid) returns SipSip_credential_list|error
Create a Credential List
Parameters
- payload CreateSipCredentialListRequest - The
CreateSipCredentialListRequest
record should be used as a payload to create a credential list.
- accountSid string? (default ()) - The unique id of the Account that is responsible for this resource.
Return Type
- SipSip_credential_list|error - Created
fetchSipCredentialList
function fetchSipCredentialList(string sid, string? accountSid) returns SipSip_credential_list|error
Get a Credential List
Parameters
- sid string - The credential list Sid that uniquely identifies this resource.
- accountSid string? (default ()) - The unique id of the Account that is responsible for this resource.
Return Type
updateSipCredentialList
function updateSipCredentialList(string sid, UpdateSipCredentialListRequest payload, string? accountSid) returns SipSip_credential_list|error
Update a Credential List
Parameters
- sid string - The credential list Sid that uniquely identifies this resource.
- payload UpdateSipCredentialListRequest - The
UpdateSipCredentialListRequest
record should be used as a payload to update a credential list.
- accountSid string? (default ()) - The unique id of the Account that is responsible for this resource.
Return Type
deleteSipCredentialList
Delete a Credential List
Parameters
- sid string - The credential list Sid that uniquely identifies this resource.
- accountSid string? (default ()) - The unique id of the Account that is responsible for this resource.
listSipCredentialListMapping
function listSipCredentialListMapping(string domainSid, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListSipCredentialListMappingResponse|error
Read multiple CredentialListMapping resources from an account.
Parameters
- domainSid string - A 34 character string that uniquely identifies the SIP Domain that includes the resource to read.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
createSipCredentialListMapping
function createSipCredentialListMapping(string domainSid, CreateSipCredentialListMappingRequest payload, string? accountSid) returns SipSip_domainSip_credential_list_mapping|error
Create a CredentialListMapping resource for an account.
Parameters
- domainSid string - A 34 character string that uniquely identifies the SIP Domain for which the CredentialList resource will be mapped.
- payload CreateSipCredentialListMappingRequest - The
CreateSipCredentialListMappingRequest
record should be used as a payload to create a CredentialListMapping resource.
Return Type
fetchSipCredentialListMapping
function fetchSipCredentialListMapping(string domainSid, string sid, string? accountSid) returns SipSip_domainSip_credential_list_mapping|error
Fetch a single CredentialListMapping resource from an account.
Parameters
- domainSid string - A 34 character string that uniquely identifies the SIP Domain that includes the resource to fetch.
- sid string - A 34 character string that uniquely identifies the resource to fetch.
Return Type
deleteSipCredentialListMapping
function deleteSipCredentialListMapping(string domainSid, string sid, string? accountSid) returns Response|error
Delete a CredentialListMapping resource from an account.
Parameters
- domainSid string - A 34 character string that uniquely identifies the SIP Domain that includes the resource to delete.
- sid string - A 34 character string that uniquely identifies the resource to delete.
listSipDomain
function listSipDomain(int? pageSize, int? page, string? pageToken, string? accountSid) returns ListSipDomainResponse|error
Retrieve a list of domains belonging to the account used to make the request
Parameters
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
createSipDomain
function createSipDomain(CreateSipDomainRequest payload, string? accountSid) returns SipSip_domain|error
Create a new Domain
Parameters
- payload CreateSipDomainRequest - The
CreateSipDomainRequest
record should be used as a payload to create a domain.
Return Type
- SipSip_domain|error - Created
fetchSipDomain
function fetchSipDomain(string sid, string? accountSid) returns SipSip_domain|error
Fetch an instance of a Domain
Parameters
- sid string - The Twilio-provided string that uniquely identifies the SipDomain resource to fetch.
Return Type
- SipSip_domain|error - OK
updateSipDomain
function updateSipDomain(string sid, UpdateSipDomainRequest payload, string? accountSid) returns SipSip_domain|error
Update the attributes of a domain
Parameters
- sid string - The Twilio-provided string that uniquely identifies the SipDomain resource to update.
- payload UpdateSipDomainRequest - The
UpdateSipDomainRequest
record should be used as a payload to update a domain.
Return Type
- SipSip_domain|error - OK
deleteSipDomain
Delete an instance of a Domain
Parameters
- sid string - The Twilio-provided string that uniquely identifies the SipDomain resource to delete.
listSipIpAccessControlList
function listSipIpAccessControlList(int? pageSize, int? page, string? pageToken, string? accountSid) returns ListSipIpAccessControlListResponse|error
Retrieve a list of IpAccessControlLists that belong to the account used to make the request
Parameters
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
createSipIpAccessControlList
function createSipIpAccessControlList(CreateSipIpAccessControlListRequest payload, string? accountSid) returns SipSip_ip_access_control_list|error
Create a new IpAccessControlList resource
Parameters
- payload CreateSipIpAccessControlListRequest - The
CreateSipIpAccessControlListRequest
record should be used as a payload to create an IpAccessControlList.
Return Type
- SipSip_ip_access_control_list|error - Created
fetchSipIpAccessControlList
function fetchSipIpAccessControlList(string sid, string? accountSid) returns SipSip_ip_access_control_list|error
Fetch a specific instance of an IpAccessControlList
Parameters
- sid string - A 34 character string that uniquely identifies the resource to fetch.
Return Type
updateSipIpAccessControlList
function updateSipIpAccessControlList(string sid, UpdateSipIpAccessControlListRequest payload, string? accountSid) returns SipSip_ip_access_control_list|error
Rename an IpAccessControlList
Parameters
- sid string - A 34 character string that uniquely identifies the resource to udpate.
- payload UpdateSipIpAccessControlListRequest - The
UpdateSipIpAccessControlListRequest
record should be used as a payload to update an IpAccessControlList.
Return Type
deleteSipIpAccessControlList
Delete an IpAccessControlList from the requested account
Parameters
- sid string - A 34 character string that uniquely identifies the resource to delete.
fetchSipIpAccessControlListMapping
function fetchSipIpAccessControlListMapping(string domainSid, string sid, string? accountSid) returns SipSip_domainSip_ip_access_control_list_mapping|error
Fetch an IpAccessControlListMapping resource.
Parameters
- domainSid string - A 34 character string that uniquely identifies the SIP domain.
- sid string - A 34 character string that uniquely identifies the resource to fetch.
- accountSid string? (default ()) - The unique id of the Account that is responsible for this resource.
Return Type
deleteSipIpAccessControlListMapping
function deleteSipIpAccessControlListMapping(string domainSid, string sid, string? accountSid) returns Response|error
Delete an IpAccessControlListMapping resource.
Parameters
- domainSid string - A 34 character string that uniquely identifies the SIP domain.
- sid string - A 34 character string that uniquely identifies the resource to delete.
- accountSid string? (default ()) - The unique id of the Account that is responsible for this resource.
listSipIpAccessControlListMapping
function listSipIpAccessControlListMapping(string domainSid, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListSipIpAccessControlListMappingResponse|error
Retrieve a list of IpAccessControlListMapping resources.
Parameters
- domainSid string - A 34 character string that uniquely identifies the SIP domain.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
- accountSid string? (default ()) - The unique id of the Account that is responsible for this resource.
Return Type
createSipIpAccessControlListMapping
function createSipIpAccessControlListMapping(string domainSid, CreateSipIpAccessControlListMappingRequest payload, string? accountSid) returns SipSip_domainSip_ip_access_control_list_mapping|error
Create a new IpAccessControlListMapping resource.
Parameters
- domainSid string - A 34 character string that uniquely identifies the SIP domain.
- payload CreateSipIpAccessControlListMappingRequest - The
CreateSipIpAccessControlListMappingRequest
record should be used as a payload to create an IpAccessControlListMapping resource.
- accountSid string? (default ()) - The unique id of the Account that is responsible for this resource.
Return Type
listSipIpAddress
function listSipIpAddress(string ipAccessControlListSid, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListSipIpAddressResponse|error
Read multiple IpAddress resources.
Parameters
- ipAccessControlListSid string - The IpAccessControlList Sid that identifies the IpAddress resources to read.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
createSipIpAddress
function createSipIpAddress(string ipAccessControlListSid, CreateSipIpAddressRequest payload, string? accountSid) returns SipSip_ip_access_control_listSip_ip_address|error
Create a new IpAddress resource.
Parameters
- ipAccessControlListSid string - The IpAccessControlList Sid with which to associate the created IpAddress resource.
- payload CreateSipIpAddressRequest - The
CreateSipIpAddressRequest
record should be used as a payload to create an IpAddress resource.
Return Type
fetchSipIpAddress
function fetchSipIpAddress(string ipAccessControlListSid, string sid, string? accountSid) returns SipSip_ip_access_control_listSip_ip_address|error
Read one IpAddress resource.
Parameters
- ipAccessControlListSid string - The IpAccessControlList Sid that identifies the IpAddress resources to fetch.
- sid string - A 34 character string that uniquely identifies the IpAddress resource to fetch.
Return Type
updateSipIpAddress
function updateSipIpAddress(string ipAccessControlListSid, string sid, UpdateSipIpAddressRequest payload, string? accountSid) returns SipSip_ip_access_control_listSip_ip_address|error
Update an IpAddress resource.
Parameters
- ipAccessControlListSid string - The IpAccessControlList Sid that identifies the IpAddress resources to update.
- sid string - A 34 character string that identifies the IpAddress resource to update.
- payload UpdateSipIpAddressRequest - The
UpdateSipIpAddressRequest
record should be used as a payload to update an IpAddress resource.
Return Type
deleteSipIpAddress
function deleteSipIpAddress(string ipAccessControlListSid, string sid, string? accountSid) returns Response|error
Delete an IpAddress resource.
Parameters
- ipAccessControlListSid string - The IpAccessControlList Sid that identifies the IpAddress resources to delete.
- sid string - A 34 character string that uniquely identifies the resource to delete.
createSiprec
function createSiprec(string callSid, CreateSiprecRequest payload, string? accountSid) returns CallSiprec|error
Create a Siprec
Parameters
- payload CreateSiprecRequest - The
CreateSiprecRequest
record should be used as a payload to create a Siprec.
Return Type
- CallSiprec|error - Created
updateSiprec
function updateSiprec(string callSid, string sid, UpdateSiprecRequest payload, string? accountSid) returns CallSiprec|error
Stop a Siprec using either the SID of the Siprec resource or the name
used when creating the resource
Parameters
- sid string - The SID of the Siprec resource, or the
name
used when creating the resource.
- payload UpdateSiprecRequest - The
UpdateSiprecRequest
record should be used as a payload to update a Siprec.
Return Type
- CallSiprec|error - OK
createStream
function createStream(string callSid, CreateStreamRequest payload, string? accountSid) returns CallStream|error
Create a Stream
Parameters
- payload CreateStreamRequest - The
CreateStreamRequest
record should be used as a payload to create a Stream.
Return Type
- CallStream|error - Created
updateStream
function updateStream(string callSid, string sid, UpdateStreamRequest payload, string? accountSid) returns CallStream|error
Stop a Stream using either the SID of the Stream resource or the name
used when creating the resource
Parameters
- sid string - The SID of the Stream resource, or the
name
used when creating the resource
- payload UpdateStreamRequest - The
UpdateStreamRequest
record should be used as a payload to update a Stream.
Return Type
- CallStream|error - OK
createToken
function createToken(CreateTokenRequest payload, string? accountSid) returns Token|error
Create a new token for ICE servers
Parameters
- payload CreateTokenRequest - The
CreateTokenRequest
record should be used as a payload to create a token.
fetchTranscription
function fetchTranscription(string sid, string? accountSid) returns Transcription|error
Fetch an instance of a Transcription
Parameters
- sid string - The Twilio-provided string that uniquely identifies the Transcription resource to fetch.
Return Type
- Transcription|error - OK
deleteTranscription
Delete a transcription from the account used to make the request
Parameters
- sid string - The Twilio-provided string that uniquely identifies the Transcription resource to delete.
listTranscription
function listTranscription(int? pageSize, int? page, string? pageToken, string? accountSid) returns ListTranscriptionResponse|error
Retrieve a list of transcriptions belonging to the account used to make the request
Parameters
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
listUsageRecord
function listUsageRecord(Usage_record_enum_category? category, string? startDate, string? endDate, boolean? includeSubaccounts, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListUsageRecordResponse|error
Retrieve a list of usage-records belonging to the account used to make the request
Parameters
- category Usage_record_enum_category? (default ()) - The usage category of the UsageRecord resources to read. Only UsageRecord resources in the specified category are retrieved.
- startDate string? (default ()) - Only include usage that has occurred on or after this date. Specify the date in GMT and format as
YYYY-MM-DD
. You can also specify offsets from the current date, such as:-30days
, which will set the start date to be 30 days before the current date.
- endDate string? (default ()) - Only include usage that occurred on or before this date. Specify the date in GMT and format as
YYYY-MM-DD
. You can also specify offsets from the current date, such as:+30days
, which will set the end date to 30 days from the current date.
- includeSubaccounts boolean? (default ()) - Whether to include usage from the master account and all its subaccounts. Can be:
true
(the default) to include usage from the master account and all subaccounts orfalse
to retrieve usage from only the specified account.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
listUsageRecordAllTime
function listUsageRecordAllTime(Usage_record_all_time_enum_category? category, string? startDate, string? endDate, boolean? includeSubaccounts, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListUsageRecordAllTimeResponse|error
Retrieve a list of usage-records belonging to the account used to make the request
Parameters
- category Usage_record_all_time_enum_category? (default ()) - The usage category of the UsageRecord resources to read. Only UsageRecord resources in the specified category are retrieved.
- startDate string? (default ()) - Only include usage that has occurred on or after this date. Specify the date in GMT and format as
YYYY-MM-DD
. You can also specify offsets from the current date, such as:-30days
, which will set the start date to be 30 days before the current date.
- endDate string? (default ()) - Only include usage that occurred on or before this date. Specify the date in GMT and format as
YYYY-MM-DD
. You can also specify offsets from the current date, such as:+30days
, which will set the end date to 30 days from the current date.
- includeSubaccounts boolean? (default ()) - Whether to include usage from the master account and all its subaccounts. Can be:
true
(the default) to include usage from the master account and all subaccounts orfalse
to retrieve usage from only the specified account.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
listUsageRecordDaily
function listUsageRecordDaily(Usage_record_daily_enum_category? category, string? startDate, string? endDate, boolean? includeSubaccounts, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListUsageRecordDailyResponse|error
Retrieve a list of daily usage-records belonging to the account used to make the request
Parameters
- category Usage_record_daily_enum_category? (default ()) - The usage category of the UsageRecord resources to read. Only UsageRecord resources in the specified category are retrieved.
- startDate string? (default ()) - Only include usage that has occurred on or after this date. Specify the date in GMT and format as
YYYY-MM-DD
. You can also specify offsets from the current date, such as:-30days
, which will set the start date to be 30 days before the current date.
- endDate string? (default ()) - Only include usage that occurred on or before this date. Specify the date in GMT and format as
YYYY-MM-DD
. You can also specify offsets from the current date, such as:+30days
, which will set the end date to 30 days from the current date.
- includeSubaccounts boolean? (default ()) - Whether to include usage from the master account and all its subaccounts. Can be:
true
(the default) to include usage from the master account and all subaccounts orfalse
to retrieve usage from only the specified account.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
listUsageRecordLastMonth
function listUsageRecordLastMonth(Usage_record_last_month_enum_category? category, string? startDate, string? endDate, boolean? includeSubaccounts, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListUsageRecordLastMonthResponse|error
Retrieve a list of last month's usage-records belonging to the account used to make the request
Parameters
- category Usage_record_last_month_enum_category? (default ()) - The usage category of the UsageRecord resources to read. Only UsageRecord resources in the specified category are retrieved.
- startDate string? (default ()) - Only include usage that has occurred on or after this date. Specify the date in GMT and format as
YYYY-MM-DD
. You can also specify offsets from the current date, such as:-30days
, which will set the start date to be 30 days before the current date.
- endDate string? (default ()) - Only include usage that occurred on or before this date. Specify the date in GMT and format as
YYYY-MM-DD
. You can also specify offsets from the current date, such as:+30days
, which will set the end date to 30 days from the current date.
- includeSubaccounts boolean? (default ()) - Whether to include usage from the master account and all its subaccounts. Can be:
true
(the default) to include usage from the master account and all subaccounts orfalse
to retrieve usage from only the specified account.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
listUsageRecordMonthly
function listUsageRecordMonthly(Usage_record_monthly_enum_category? category, string? startDate, string? endDate, boolean? includeSubaccounts, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListUsageRecordMonthlyResponse|error
Retrieve a list of monthly usage-records belonging to the account used to make the request
Parameters
- category Usage_record_monthly_enum_category? (default ()) - The usage category of the UsageRecord resources to read. Only UsageRecord resources in the specified category are retrieved.
- startDate string? (default ()) - Only include usage that has occurred on or after this date. Specify the date in GMT and format as
YYYY-MM-DD
. You can also specify offsets from the current date, such as:-30days
, which will set the start date to be 30 days before the current date.
- endDate string? (default ()) - Only include usage that occurred on or before this date. Specify the date in GMT and format as
YYYY-MM-DD
. You can also specify offsets from the current date, such as:+30days
, which will set the end date to 30 days from the current date.
- includeSubaccounts boolean? (default ()) - Whether to include usage from the master account and all its subaccounts. Can be:
true
(the default) to include usage from the master account and all subaccounts orfalse
to retrieve usage from only the specified account.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
listUsageRecordThisMonth
function listUsageRecordThisMonth(Usage_record_this_month_enum_category? category, string? startDate, string? endDate, boolean? includeSubaccounts, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListUsageRecordThisMonthResponse|error
Retrieve a list of this month's usage-records belonging to the account used to make the request
Parameters
- category Usage_record_this_month_enum_category? (default ()) - The usage category of the UsageRecord resources to read. Only UsageRecord resources in the specified category are retrieved.
- startDate string? (default ()) - Only include usage that has occurred on or after this date. Specify the date in GMT and format as
YYYY-MM-DD
. You can also specify offsets from the current date, such as:-30days
, which will set the start date to be 30 days before the current date.
- endDate string? (default ()) - Only include usage that occurred on or before this date. Specify the date in GMT and format as
YYYY-MM-DD
. You can also specify offsets from the current date, such as:+30days
, which will set the end date to 30 days from the current date.
- includeSubaccounts boolean? (default ()) - Whether to include usage from the master account and all its subaccounts. Can be:
true
(the default) to include usage from the master account and all subaccounts orfalse
to retrieve usage from only the specified account.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
listUsageRecordToday
function listUsageRecordToday(Usage_record_today_enum_category? category, string? startDate, string? endDate, boolean? includeSubaccounts, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListUsageRecordTodayResponse|error
Retrieve a list of today's usage-records belonging to the account used to make the request
Parameters
- category Usage_record_today_enum_category? (default ()) - The usage category of the UsageRecord resources to read. Only UsageRecord resources in the specified category are retrieved.
- startDate string? (default ()) - Only include usage that has occurred on or after this date. Specify the date in GMT and format as
YYYY-MM-DD
. You can also specify offsets from the current date, such as:-30days
, which will set the start date to be 30 days before the current date.
- endDate string? (default ()) - Only include usage that occurred on or before this date. Specify the date in GMT and format as
YYYY-MM-DD
. You can also specify offsets from the current date, such as:+30days
, which will set the end date to 30 days from the current date.
- includeSubaccounts boolean? (default ()) - Whether to include usage from the master account and all its subaccounts. Can be:
true
(the default) to include usage from the master account and all subaccounts orfalse
to retrieve usage from only the specified account.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
listUsageRecordYearly
function listUsageRecordYearly(Usage_record_yearly_enum_category? category, string? startDate, string? endDate, boolean? includeSubaccounts, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListUsageRecordYearlyResponse|error
Retrieve a list of yearly usage-records belonging to the account used to make the request
Parameters
- category Usage_record_yearly_enum_category? (default ()) - The usage category of the UsageRecord resources to read. Only UsageRecord resources in the specified category are retrieved.
- startDate string? (default ()) - Only include usage that has occurred on or after this date. Specify the date in GMT and format as
YYYY-MM-DD
. You can also specify offsets from the current date, such as:-30days
, which will set the start date to be 30 days before the current date.
- endDate string? (default ()) - Only include usage that occurred on or before this date. Specify the date in GMT and format as
YYYY-MM-DD
. You can also specify offsets from the current date, such as:+30days
, which will set the end date to 30 days from the current date.
- includeSubaccounts boolean? (default ()) - Whether to include usage from the master account and all its subaccounts. Can be:
true
(the default) to include usage from the master account and all subaccounts orfalse
to retrieve usage from only the specified account.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
listUsageRecordYesterday
function listUsageRecordYesterday(Usage_record_yesterday_enum_category? category, string? startDate, string? endDate, boolean? includeSubaccounts, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListUsageRecordYesterdayResponse|error
Retrieve a list of yesterday's usage-records belonging to the account used to make the request
Parameters
- category Usage_record_yesterday_enum_category? (default ()) - The usage category of the UsageRecord resources to read. Only UsageRecord resources in the specified category are retrieved.
- startDate string? (default ()) - Only include usage that has occurred on or after this date. Specify the date in GMT and format as
YYYY-MM-DD
. You can also specify offsets from the current date, such as:-30days
, which will set the start date to be 30 days before the current date.
- endDate string? (default ()) - Only include usage that occurred on or before this date. Specify the date in GMT and format as
YYYY-MM-DD
. You can also specify offsets from the current date, such as:+30days
, which will set the end date to 30 days from the current date.
- includeSubaccounts boolean? (default ()) - Whether to include usage from the master account and all its subaccounts. Can be:
true
(the default) to include usage from the master account and all subaccounts orfalse
to retrieve usage from only the specified account.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
fetchUsageTrigger
function fetchUsageTrigger(string sid, string? accountSid) returns UsageUsage_trigger|error
Fetch and instance of a usage-trigger
Parameters
- sid string - The Twilio-provided string that uniquely identifies the UsageTrigger resource to fetch.
Return Type
- UsageUsage_trigger|error - OK
updateUsageTrigger
function updateUsageTrigger(string sid, UpdateUsageTriggerRequest payload, string? accountSid) returns UsageUsage_trigger|error
Update an instance of a usage trigger
Parameters
- sid string - The Twilio-provided string that uniquely identifies the UsageTrigger resource to update.
- payload UpdateUsageTriggerRequest - The
UpdateUsageTriggerRequest
record should be used as a payload to update a UsageTrigger resource.
Return Type
- UsageUsage_trigger|error - OK
deleteUsageTrigger
Delete a usage-trigger from the account used to make the request
Parameters
- sid string - The Twilio-provided string that uniquely identifies the UsageTrigger resource to delete.
listUsageTrigger
function listUsageTrigger(Usage_trigger_enum_recurring? recurring, Usage_trigger_enum_trigger_field? triggerBy, Usage_trigger_enum_usage_category? usageCategory, int? pageSize, int? page, string? pageToken, string? accountSid) returns ListUsageTriggerResponse|error
Retrieve a list of usage-triggers belonging to the account used to make the request
Parameters
- recurring Usage_trigger_enum_recurring? (default ()) - The frequency of recurring UsageTriggers to read. Can be:
daily
,monthly
, oryearly
to read recurring UsageTriggers. An empty value or a value ofalltime
reads non-recurring UsageTriggers.
- triggerBy Usage_trigger_enum_trigger_field? (default ()) - The trigger field of the UsageTriggers to read. Can be:
count
,usage
, orprice
as described in the UsageRecords documentation.
- usageCategory Usage_trigger_enum_usage_category? (default ()) - The usage category of the UsageTriggers to read. Must be a supported usage categories.
- pageSize int? (default ()) - How many resources to return in each list page. The default is 50, and the maximum is 1000.
- page int? (default ()) - The page index. This value is simply for client state.
- pageToken string? (default ()) - The page token. This is provided by the API.
Return Type
createUsageTrigger
function createUsageTrigger(CreateUsageTriggerRequest payload, string? accountSid) returns UsageUsage_trigger|error
Create a new UsageTrigger
Parameters
- payload CreateUsageTriggerRequest - The
CreateUsageTriggerRequest
record should be used as a payload to create a UsageTrigger.
Return Type
- UsageUsage_trigger|error - Created
createUserDefinedMessage
function createUserDefinedMessage(string callSid, CreateUserDefinedMessageRequest payload, string? accountSid) returns CallUser_defined_message|error
Create a new User Defined Message for the given Call SID.
Parameters
- payload CreateUserDefinedMessageRequest - The
CreateUserDefinedMessageRequest
record should be used as a payload to create a User Defined Message.
Return Type
- CallUser_defined_message|error - Created
createUserDefinedMessageSubscription
function createUserDefinedMessageSubscription(string callSid, CreateUserDefinedMessageSubscriptionRequest payload, string? accountSid) returns CallUser_defined_message_subscription|error
Subscribe to User Defined Messages for a given Call SID.
Parameters
- payload CreateUserDefinedMessageSubscriptionRequest - The
CreateUserDefinedMessageSubscriptionRequest
record should be used as a payload to create a User Defined Message Subscription.
Return Type
- CallUser_defined_message_subscription|error - Created
deleteUserDefinedMessageSubscription
function deleteUserDefinedMessageSubscription(string callSid, string sid, string? accountSid) returns Response|error
Delete a specific User Defined Message Subscription.
Records
twilio: Account
Fields
- auth_token string? - The authorization token for this account. This token should be kept a secret, so no sharing.
- date_created string? - The date that this account was created, in GMT in RFC 2822 format
- date_updated string? - The date that this account was last updated, in GMT in RFC 2822 format.
- friendly_name string? - A human readable description of this account, up to 64 characters long. By default the FriendlyName is your email address.
- owner_account_sid string? - The unique 34 character id that represents the parent of this account. The OwnerAccountSid of a parent account is it's own sid.
- sid string? - A 34 character string that uniquely identifies this resource.
- status Account_enum_status? -
- subresource_uris record {}? - A Map of various subresources available for the given Account Instance
- 'type Account_enum_type? -
- uri string? - The URI for this resource, relative to
https://api.twilio.com
twilio: Address
Fields
- city string? - The city in which the address is located.
- customer_name string? - The name associated with the address.This property has a maximum length of 16 4-byte characters, or 21 3-byte characters.
- friendly_name string? - The string that you assigned to describe the resource.
- iso_country string? - The ISO country code of the address.
- postal_code string? - The postal code of the address.
- region string? - The state or region of the address.
- sid string? - The unique string that that we created to identify the Address resource.
- street string? - The number and street address of the address.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
- emergency_enabled boolean? - Whether emergency calling has been enabled on this number.
- validated boolean? - Whether the address has been validated to comply with local regulation. In countries that require valid addresses, an invalid address will not be accepted.
true
indicates the Address has been validated.false
indicate the country doesn't require validation or the Address is not valid.
- verified boolean? - Whether the address has been verified to comply with regulation. In countries that require valid addresses, an invalid address will not be accepted.
true
indicates the Address has been verified.false
indicate the country doesn't require verified or the Address is not valid.
- street_secondary string? - The additional number and street address of the address.
twilio: AddressDependent_phone_number
Fields
- sid string? - The unique string that that we created to identify the DependentPhoneNumber resource.
- friendly_name string? - The string that you assigned to describe the resource.
- voice_url string? - The URL we call when the phone number receives a call. The
voice_url
will not be used if avoice_application_sid
or atrunk_sid
is set.
- voice_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
voice_url
. Can be:GET
orPOST
.
- voice_fallback_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
voice_fallback_url
. Can be:GET
orPOST
.
- voice_fallback_url string? - The URL that we call when an error occurs retrieving or executing the TwiML requested by
url
.
- voice_caller_id_lookup boolean? - Whether we look up the caller's caller-ID name from the CNAM database. Can be:
true
orfalse
. Caller ID lookups can cost $0.01 each.
- sms_fallback_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
sms_fallback_url
. Can be:GET
orPOST
.
- sms_fallback_url string? - The URL that we call when an error occurs while retrieving or executing the TwiML from
sms_url
.
- sms_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
sms_url
. Can be:GET
orPOST
.
- sms_url string? - The URL we call when the phone number receives an incoming SMS message.
- address_requirements Dependent_phone_number_enum_address_requirement? -
- capabilities anydata? - The set of Boolean properties that indicates whether a phone number can receive calls or messages. Capabilities are
Voice
,SMS
, andMMS
and each capability can be:true
orfalse
.
- status_callback string? - The URL we call using the
status_callback_method
to send status information to your application.
- status_callback_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
status_callback
. Can be:GET
orPOST
.
- api_version string? - The API version used to start a new TwiML session.
- sms_application_sid string? - The SID of the application that handles SMS messages sent to the phone number. If an
sms_application_sid
is present, we ignore allsms_*_url
values and use those of the application.
- voice_application_sid string? - The SID of the application that handles calls to the phone number. If a
voice_application_sid
is present, we ignore all of the voice urls and use those set on the application. Setting avoice_application_sid
will automatically delete yourtrunk_sid
and vice versa.
- trunk_sid string? - The SID of the Trunk that handles calls to the phone number. If a
trunk_sid
is present, we ignore all of the voice urls and voice applications and use those set on the Trunk. Setting atrunk_sid
will automatically delete yourvoice_application_sid
and vice versa.
- emergency_status Dependent_phone_number_enum_emergency_status? -
- emergency_address_sid string? - The SID of the emergency address configuration that we use for emergency calling from the phone number.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
twilio: Application
Fields
- api_version string? - The API version used to start a new TwiML session.
- friendly_name string? - The string that you assigned to describe the resource.
- message_status_callback string? - The URL we call using a POST method to send message status information to your application.
- sid string? - The unique string that that we created to identify the Application resource.
- sms_fallback_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
sms_fallback_url
. Can be:GET
orPOST
.
- sms_fallback_url string? - The URL that we call when an error occurs while retrieving or executing the TwiML from
sms_url
.
- sms_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
sms_url
. Can be:GET
orPOST
.
- sms_status_callback string? - The URL we call using a POST method to send status information to your application about SMS messages that refer to the application.
- sms_url string? - The URL we call when the phone number receives an incoming SMS message.
- status_callback string? - The URL we call using the
status_callback_method
to send status information to your application.
- status_callback_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
status_callback
. Can be:GET
orPOST
.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
- voice_caller_id_lookup boolean? - Whether we look up the caller's caller-ID name from the CNAM database (additional charges apply). Can be:
true
orfalse
.
- voice_fallback_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
voice_fallback_url
. Can be:GET
orPOST
.
- voice_fallback_url string? - The URL that we call when an error occurs retrieving or executing the TwiML requested by
url
.
- voice_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
voice_url
. Can be:GET
orPOST
.
- voice_url string? - The URL we call when the phone number assigned to this application receives a call.
- public_application_connect_enabled boolean? - Whether to allow other Twilio accounts to dial this applicaton using Dial verb. Can be:
true
orfalse
.
twilio: Authorized_connect_app
Fields
- connect_app_company_name string? - The company name set for the Connect App.
- connect_app_description string? - A detailed description of the Connect App.
- connect_app_friendly_name string? - The name of the Connect App.
- connect_app_homepage_url string? - The public URL for the Connect App.
- connect_app_sid string? - The SID that we assigned to the Connect App.
- permissions Authorized_connect_app_enum_permission[]? - The set of permissions that you authorized for the Connect App. Can be:
get-all
orpost-all
.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
twilio: Available_phone_number_country
Fields
- country_code string? - The ISO-3166-1 country code of the country.
- country string? - The name of the country.
- uri string? - The URI of the Country resource, relative to
https://api.twilio.com
.
- beta boolean? - Whether all phone numbers available in the country are new to the Twilio platform.
true
if they are andfalse
if all numbers are not in the Twilio Phone Number Beta program.
- subresource_uris record {}? - A list of related AvailablePhoneNumber resources identified by their URIs relative to
https://api.twilio.com
.
twilio: Available_phone_number_local
Fields
- friendly_name string? - A formatted version of the phone number.
- locality string? - The locality or city of this phone number's location.
- rate_center string? - The rate center of this phone number. Available for only phone numbers from the US and Canada.
- latitude decimal? - The latitude of this phone number's location. Available for only phone numbers from the US and Canada.
- longitude decimal? - The longitude of this phone number's location. Available for only phone numbers from the US and Canada.
- region string? - The two-letter state or province abbreviation of this phone number's location. Available for only phone numbers from the US and Canada.
- postal_code string? - The postal or ZIP code of this phone number's location. Available for only phone numbers from the US and Canada.
- iso_country string? - The ISO country code of this phone number.
- address_requirements string? - The type of Address resource the phone number requires. Can be:
none
,any
,local
, orforeign
.none
means no address is required.any
means an address is required, but it can be anywhere in the world.local
means an address in the phone number's country is required.foreign
means an address outside of the phone number's country is required.
- beta boolean? - Whether the phone number is new to the Twilio platform. Can be:
true
orfalse
.
- capabilities Available_phone_number_local_capabilities? - The set of Boolean properties that indicate whether a phone number can receive calls or messages. Capabilities are:
Voice
,SMS
, andMMS
and each capability can be:true
orfalse
.
twilio: Available_phone_number_local_capabilities
The set of Boolean properties that indicate whether a phone number can receive calls or messages. Capabilities are: Voice
, SMS
, and MMS
and each capability can be: true
or false
.
Fields
- mms boolean? - Whether the phone number can send and receive MMS messages. Can be:
true
orfalse
.
- sms boolean? - Whether the phone number can send and receive SMS messages. Can be:
true
orfalse
.
- voice boolean? - Whether the phone number can receive voice calls. Can be:
true
orfalse
.
- fax boolean? - Whether the phone number can receive faxes. Can be:
true
orfalse
.
twilio: Available_phone_number_machine_to_machine
Fields
- friendly_name string? - A formatted version of the phone number.
- locality string? - The locality or city of this phone number's location.
- rate_center string? - The rate center of this phone number. Available for only phone numbers from the US and Canada.
- latitude decimal? - The latitude of this phone number's location. Available for only phone numbers from the US and Canada.
- longitude decimal? - The longitude of this phone number's location. Available for only phone numbers from the US and Canada.
- region string? - The two-letter state or province abbreviation of this phone number's location. Available for only phone numbers from the US and Canada.
- postal_code string? - The postal or ZIP code of this phone number's location. Available for only phone numbers from the US and Canada.
- iso_country string? - The ISO country code of this phone number.
- address_requirements string? - The type of Address resource the phone number requires. Can be:
none
,any
,local
, orforeign
.none
means no address is required.any
means an address is required, but it can be anywhere in the world.local
means an address in the phone number's country is required.foreign
means an address outside of the phone number's country is required.
- beta boolean? - Whether the phone number is new to the Twilio platform. Can be:
true
orfalse
.
- capabilities Available_phone_number_local_capabilities? - The set of Boolean properties that indicate whether a phone number can receive calls or messages. Capabilities are:
Voice
,SMS
, andMMS
and each capability can be:true
orfalse
.
twilio: Available_phone_number_mobile
Fields
- friendly_name string? - A formatted version of the phone number.
- locality string? - The locality or city of this phone number's location.
- rate_center string? - The rate center of this phone number. Available for only phone numbers from the US and Canada.
- latitude decimal? - The latitude of this phone number's location. Available for only phone numbers from the US and Canada.
- longitude decimal? - The longitude of this phone number's location. Available for only phone numbers from the US and Canada.
- region string? - The two-letter state or province abbreviation of this phone number's location. Available for only phone numbers from the US and Canada.
- postal_code string? - The postal or ZIP code of this phone number's location. Available for only phone numbers from the US and Canada.
- iso_country string? - The ISO country code of this phone number.
- address_requirements string? - The type of Address resource the phone number requires. Can be:
none
,any
,local
, orforeign
.none
means no address is required.any
means an address is required, but it can be anywhere in the world.local
means an address in the phone number's country is required.foreign
means an address outside of the phone number's country is required.
- beta boolean? - Whether the phone number is new to the Twilio platform. Can be:
true
orfalse
.
- capabilities Available_phone_number_local_capabilities? - The set of Boolean properties that indicate whether a phone number can receive calls or messages. Capabilities are:
Voice
,SMS
, andMMS
and each capability can be:true
orfalse
.
twilio: Available_phone_number_national
Fields
- friendly_name string? - A formatted version of the phone number.
- locality string? - The locality or city of this phone number's location.
- rate_center string? - The rate center of this phone number. Available for only phone numbers from the US and Canada.
- latitude decimal? - The latitude of this phone number's location. Available for only phone numbers from the US and Canada.
- longitude decimal? - The longitude of this phone number's location. Available for only phone numbers from the US and Canada.
- region string? - The two-letter state or province abbreviation of this phone number's location. Available for only phone numbers from the US and Canada.
- postal_code string? - The postal or ZIP code of this phone number's location. Available for only phone numbers from the US and Canada.
- iso_country string? - The ISO country code of this phone number.
- address_requirements string? - The type of Address resource the phone number requires. Can be:
none
,any
,local
, orforeign
.none
means no address is required.any
means an address is required, but it can be anywhere in the world.local
means an address in the phone number's country is required.foreign
means an address outside of the phone number's country is required.
- beta boolean? - Whether the phone number is new to the Twilio platform. Can be:
true
orfalse
.
- capabilities Available_phone_number_local_capabilities? - The set of Boolean properties that indicate whether a phone number can receive calls or messages. Capabilities are:
Voice
,SMS
, andMMS
and each capability can be:true
orfalse
.
twilio: Available_phone_number_shared_cost
Fields
- friendly_name string? - A formatted version of the phone number.
- locality string? - The locality or city of this phone number's location.
- rate_center string? - The rate center of this phone number. Available for only phone numbers from the US and Canada.
- latitude decimal? - The latitude of this phone number's location. Available for only phone numbers from the US and Canada.
- longitude decimal? - The longitude of this phone number's location. Available for only phone numbers from the US and Canada.
- region string? - The two-letter state or province abbreviation of this phone number's location. Available for only phone numbers from the US and Canada.
- postal_code string? - The postal or ZIP code of this phone number's location. Available for only phone numbers from the US and Canada.
- iso_country string? - The ISO country code of this phone number.
- address_requirements string? - The type of Address resource the phone number requires. Can be:
none
,any
,local
, orforeign
.none
means no address is required.any
means an address is required, but it can be anywhere in the world.local
means an address in the phone number's country is required.foreign
means an address outside of the phone number's country is required.
- beta boolean? - Whether the phone number is new to the Twilio platform. Can be:
true
orfalse
.
- capabilities Available_phone_number_local_capabilities? - The set of Boolean properties that indicate whether a phone number can receive calls or messages. Capabilities are:
Voice
,SMS
, andMMS
and each capability can be:true
orfalse
.
twilio: Available_phone_number_toll_free
Fields
- friendly_name string? - A formatted version of the phone number.
- locality string? - The locality or city of this phone number's location.
- rate_center string? - The rate center of this phone number. Available for only phone numbers from the US and Canada.
- latitude decimal? - The latitude of this phone number's location. Available for only phone numbers from the US and Canada.
- longitude decimal? - The longitude of this phone number's location. Available for only phone numbers from the US and Canada.
- region string? - The two-letter state or province abbreviation of this phone number's location. Available for only phone numbers from the US and Canada.
- postal_code string? - The postal or ZIP code of this phone number's location. Available for only phone numbers from the US and Canada.
- iso_country string? - The ISO country code of this phone number.
- address_requirements string? - The type of Address resource the phone number requires. Can be:
none
,any
,local
, orforeign
.none
means no address is required.any
means an address is required, but it can be anywhere in the world.local
means an address in the phone number's country is required.foreign
means an address outside of the phone number's country is required.
- beta boolean? - Whether the phone number is new to the Twilio platform. Can be:
true
orfalse
.
- capabilities Available_phone_number_local_capabilities? - The set of Boolean properties that indicate whether a phone number can receive calls or messages. Capabilities are:
Voice
,SMS
, andMMS
and each capability can be:true
orfalse
.
twilio: Available_phone_number_voip
Fields
- friendly_name string? - A formatted version of the phone number.
- locality string? - The locality or city of this phone number's location.
- rate_center string? - The rate center of this phone number. Available for only phone numbers from the US and Canada.
- latitude decimal? - The latitude of this phone number's location. Available for only phone numbers from the US and Canada.
- longitude decimal? - The longitude of this phone number's location. Available for only phone numbers from the US and Canada.
- region string? - The two-letter state or province abbreviation of this phone number's location. Available for only phone numbers from the US and Canada.
- postal_code string? - The postal or ZIP code of this phone number's location. Available for only phone numbers from the US and Canada.
- iso_country string? - The ISO country code of this phone number.
- address_requirements string? - The type of Address resource the phone number requires. Can be:
none
,any
,local
, orforeign
.none
means no address is required.any
means an address is required, but it can be anywhere in the world.local
means an address in the phone number's country is required.foreign
means an address outside of the phone number's country is required.
- beta boolean? - Whether the phone number is new to the Twilio platform. Can be:
true
orfalse
.
- capabilities Available_phone_number_local_capabilities? - The set of Boolean properties that indicate whether a phone number can receive calls or messages. Capabilities are:
Voice
,SMS
, andMMS
and each capability can be:true
orfalse
.
twilio: Balance
Fields
- account_sid string? - The unique SID identifier of the Account.
- balance string? - The balance of the Account, in units specified by the unit parameter. Balance changes may not be reflected immediately. Child accounts do not contain balance information
- currency string? - The units of currency for the account balance
twilio: Call
Fields
- sid string? - The unique string that we created to identify this Call resource.
- parent_call_sid string? - The SID that identifies the call that created this leg.
- phone_number_sid string? - If the call was inbound, this is the SID of the IncomingPhoneNumber resource that received the call. If the call was outbound, it is the SID of the OutgoingCallerId resource from which the call was placed.
- status Call_enum_status? -
- duration string? - The length of the call in seconds. This value is empty for busy, failed, unanswered, or ongoing calls.
- price string? - The charge for this call, in the currency associated with the account. Populated after the call is completed. May not be immediately available.
- direction string? - A string describing the direction of the call. Can be:
inbound
for inbound calls,outbound-api
for calls initiated via the REST API oroutbound-dial
for calls initiated by a<Dial>
verb. Using Elastic SIP Trunking, the values can betrunking-terminating
for outgoing calls from your communications infrastructure to the PSTN ortrunking-originating
for incoming calls to your communications infrastructure from the PSTN.
- answered_by string? - Either
human
ormachine
if this call was initiated with answering machine detection. Empty otherwise.
- api_version string? - The API version used to create the call.
- forwarded_from string? - The forwarding phone number if this call was an incoming call forwarded from another number (depends on carrier supporting forwarding). Otherwise, empty.
- group_sid string? - The Group SID associated with this call. If no Group is associated with the call, the field is empty.
- caller_name string? - The caller's name if this call was an incoming call to a phone number with caller ID Lookup enabled. Otherwise, empty.
- queue_time string? - The wait time in milliseconds before the call is placed.
- trunk_sid string? - The unique identifier of the trunk resource that was used for this call. The field is empty if the call was not made using a SIP trunk or if the call is not terminated.
- uri string? - The URI of this resource, relative to
https://api.twilio.com
.
- subresource_uris record {}? - A list of subresources available to this call, identified by their URIs relative to
https://api.twilio.com
.
twilio: CallCall_event
Fields
- request anydata? - Contains a dictionary representing the request of the call.
- response anydata? - Contains a dictionary representing the call response, including a list of the call events.
twilio: CallCall_feedback
Fields
- issues Call_feedback_enum_issues[]? - A list of issues experienced during the call. The issues can be:
imperfect-audio
,dropped-call
,incorrect-caller-id
,post-dial-delay
,digits-not-captured
,audio-latency
,unsolicited-call
, orone-way-audio
.
- quality_score int? -
1
to5
quality score where1
represents imperfect experience and5
represents a perfect call.
- sid string? - A 34 character string that uniquely identifies this resource.
twilio: CallCall_feedback_summary
Fields
- call_count int? - The total number of calls.
- call_feedback_count int? - The total number of calls with a feedback entry.
- end_date string? - The last date for which feedback entries are included in this Feedback Summary, formatted as
YYYY-MM-DD
and specified in UTC.
- include_subaccounts boolean? - Whether the feedback summary includes subaccounts;
true
if it does, otherwisefalse
.
- issues anydata[]? - A list of issues experienced during the call. The issues can be:
imperfect-audio
,dropped-call
,incorrect-caller-id
,post-dial-delay
,digits-not-captured
,audio-latency
, orone-way-audio
.
- quality_score_average decimal? - The average QualityScore of the feedback entries.
- quality_score_median decimal? - The median QualityScore of the feedback entries.
- quality_score_standard_deviation decimal? - The standard deviation of the quality scores.
- sid string? - A 34 character string that uniquely identifies this resource.
- start_date string? - The first date for which feedback entries are included in this feedback summary, formatted as
YYYY-MM-DD
and specified in UTC.
- status Call_feedback_summary_enum_status? -
twilio: CallCall_notification
Fields
- api_version string? - The API version used to create the Call Notification resource.
- error_code string? - A unique error code for the error condition that is described in our Error Dictionary.
- log string? - An integer log level that corresponds to the type of notification:
0
is ERROR,1
is WARNING.
- message_text string? - The text of the notification.
- more_info string? - The URL for more information about the error condition. This value is a page in our Error Dictionary.
- request_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method used to generate the notification. If the notification was generated during a phone call, this is the HTTP Method used to request the resource on your server. If the notification was generated by your use of our REST API, this is the HTTP method used to call the resource on our servers.
- request_url string? - The URL of the resource that generated the notification. If the notification was generated during a phone call, this is the URL of the resource on your server that caused the notification. If the notification was generated by your use of our REST API, this is the URL of the resource you called.
- sid string? - The unique string that that we created to identify the Call Notification resource.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
twilio: CallCall_notificationInstance
Fields
- api_version string? - The API version used to create the Call Notification resource.
- error_code string? - A unique error code for the error condition that is described in our Error Dictionary.
- log string? - An integer log level that corresponds to the type of notification:
0
is ERROR,1
is WARNING.
- message_text string? - The text of the notification.
- more_info string? - The URL for more information about the error condition. This value is a page in our Error Dictionary.
- request_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method used to generate the notification. If the notification was generated during a phone call, this is the HTTP Method used to request the resource on your server. If the notification was generated by your use of our REST API, this is the HTTP method used to call the resource on our servers.
- request_url string? - The URL of the resource that generated the notification. If the notification was generated during a phone call, this is the URL of the resource on your server that caused the notification. If the notification was generated by your use of our REST API, this is the URL of the resource you called.
- request_variables string? - The HTTP GET or POST variables we sent to your server. However, if the notification was generated by our REST API, this contains the HTTP POST or PUT variables you sent to our API.
- response_body string? - The HTTP body returned by your server.
- response_headers string? - The HTTP headers returned by your server.
- sid string? - The unique string that that we created to identify the Call Notification resource.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
twilio: CallCall_recording
Fields
- api_version string? - The API version used to make the recording.
- conference_sid string? - The Conference SID that identifies the conference associated with the recording, if a conference recording.
- duration string? - The length of the recording in seconds.
- sid string? - The unique string that that we created to identify the Recording resource.
- price decimal? - The one-time cost of creating the recording in the
price_unit
currency.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
- encryption_details anydata? - How to decrypt the recording if it was encrypted using Call Recording Encryption feature.
- price_unit string? - The currency used in the
price
property. Example:USD
.
- status Call_recording_enum_status? -
- channels int? - The number of channels in the final recording file. Can be:
1
, or2
. Separating a two leg call into two separate channels of the recording file is supported in Dial and Outbound Rest API record options.
- 'source Call_recording_enum_source? -
- error_code int? - The error code that describes why the recording is
absent
. The error code is described in our Error Dictionary. This value is null if the recordingstatus
is notabsent
.
- track string? - The recorded track. Can be:
inbound
,outbound
, orboth
.
twilio: CallPayments
Fields
- sid string? - The SID of the Payments resource.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
twilio: CallSiprec
Fields
- sid string? - The SID of the Siprec resource.
- name string? - The user-specified name of this Siprec, if one was given when the Siprec was created. This may be used to stop the Siprec.
- status Siprec_enum_status? -
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
twilio: CallStream
Fields
- sid string? - The SID of the Stream resource.
- name string? - The user-specified name of this Stream, if one was given when the Stream was created. This may be used to stop the Stream.
- status Stream_enum_status? -
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
twilio: CallUser_defined_message
Fields
- sid string? - The SID that uniquely identifies this User Defined Message.
- date_created string? - The date that this User Defined Message was created, given in RFC 2822 format.
twilio: CallUser_defined_message_subscription
Fields
- sid string? - The SID that uniquely identifies this User Defined Message Subscription.
- date_created string? - The date that this User Defined Message Subscription was created, given in RFC 2822 format.
- uri string? - The URI of the User Defined Message Subscription Resource, relative to
https://api.twilio.com
.
twilio: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
twilio: Conference
Fields
- api_version string? - The API version used to create this conference.
- friendly_name string? - A string that you assigned to describe this conference room. Maxiumum length is 128 characters.
- region string? - A string that represents the Twilio Region where the conference audio was mixed. May be
us1
,ie1
,de1
,sg1
,br1
,au1
, andjp1
. Basic conference audio will always be mixed inus1
. Global Conference audio will be mixed nearest to the majority of participants.
- sid string? - The unique string that that we created to identify this Conference resource.
- status Conference_enum_status? -
- uri string? - The URI of this resource, relative to
https://api.twilio.com
.
- subresource_uris record {}? - A list of related resources identified by their URIs relative to
https://api.twilio.com
.
- reason_conference_ended Conference_enum_reason_conference_ended? -
- call_sid_ending_conference string? - The call SID that caused the conference to end.
twilio: ConferenceConference_recording
Fields
- api_version string? - The API version used to create the recording.
- conference_sid string? - The Conference SID that identifies the conference associated with the recording.
- duration string? - The length of the recording in seconds.
- sid string? - The unique string that that we created to identify the Conference Recording resource.
- price string? - The one-time cost of creating the recording in the
price_unit
currency.
- price_unit string? - The currency used in the
price
property. Example:USD
.
- status Conference_recording_enum_status? -
- channels int? - The number of channels in the final recording file. Can be:
1
, or2
. Separating a two leg call into two separate channels of the recording file is supported in Dial and Outbound Rest API record options.
- 'source Conference_recording_enum_source? -
- error_code int? - The error code that describes why the recording is
absent
. The error code is described in our Error Dictionary. This value is null if the recordingstatus
is notabsent
.
- encryption_details anydata? - How to decrypt the recording if it was encrypted using Call Recording Encryption feature.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
twilio: ConferenceParticipant
Fields
- label string? - The user-specified label of this participant, if one was given when the participant was created. This may be used to fetch, update or delete the participant.
- call_sid_to_coach string? - The SID of the participant who is being
coached
. The participant being coached is the only participant who can hear the participant who iscoaching
.
- coaching boolean? - Whether the participant is coaching another call. Can be:
true
orfalse
. If not present, defaults tofalse
unlesscall_sid_to_coach
is defined. Iftrue
,call_sid_to_coach
must be defined.
- conference_sid string? - The SID of the conference the participant is in.
- end_conference_on_exit boolean? - Whether the conference ends when the participant leaves. Can be:
true
orfalse
and the default isfalse
. Iftrue
, the conference ends and all other participants drop out when the participant leaves.
- muted boolean? - Whether the participant is muted. Can be
true
orfalse
.
- hold boolean? - Whether the participant is on hold. Can be
true
orfalse
.
- start_conference_on_enter boolean? - Whether the conference starts when the participant joins the conference, if it has not already started. Can be:
true
orfalse
and the default istrue
. Iffalse
and the conference has not started, the participant is muted and hears background music until another participant starts the conference.
- status Participant_enum_status? -
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
twilio: Connect_app
Fields
- authorize_redirect_url string? - The URL we redirect the user to after we authenticate the user and obtain authorization to access the Connect App.
- company_name string? - The company name set for the Connect App.
- deauthorize_callback_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
deauthorize_callback_url
.
- deauthorize_callback_url string? - The URL we call using the
deauthorize_callback_method
to de-authorize the Connect App.
- description string? - The description of the Connect App.
- friendly_name string? - The string that you assigned to describe the resource.
- homepage_url string? - The public URL where users can obtain more information about this Connect App.
- permissions Connect_app_enum_permission[]? - The set of permissions that your ConnectApp requests.
- sid string? - The unique string that that we created to identify the ConnectApp resource.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
twilio: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth CredentialsConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
twilio: CreateAccountRequest
Fields
- FriendlyName string? - A human readable description of the account to create, defaults to
SubAccount Created at {YYYY-MM-DD HH:MM meridian}
twilio: CreateAddressRequest
Fields
- CustomerName string - The name to associate with the new address.
- Street string - The number and street address of the new address.
- City string - The city of the new address.
- Region string - The state or region of the new address.
- PostalCode string - The postal code of the new address.
- IsoCountry string - The ISO country code of the new address.
- FriendlyName string? - A descriptive string that you create to describe the new address. It can be up to 64 characters long.
- EmergencyEnabled boolean? - Whether to enable emergency calling on the new address. Can be:
true
orfalse
.
- AutoCorrectAddress boolean? - Whether we should automatically correct the address. Can be:
true
orfalse
and the default istrue
. If empty ortrue
, we will correct the address you provide if necessary. Iffalse
, we won't alter the address you provide.
- StreetSecondary string? - The additional number and street address of the address.
twilio: CreateApplicationRequest
Fields
- ApiVersion string? - The API version to use to start a new TwiML session. Can be:
2010-04-01
or2008-08-01
. The default value is the account's default API version.
- VoiceUrl string? - The URL we should call when the phone number assigned to this application receives a call.
- VoiceMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
voice_url
. Can be:GET
orPOST
.
- VoiceFallbackUrl string? - The URL that we should call when an error occurs retrieving or executing the TwiML requested by
url
.
- VoiceFallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
voice_fallback_url
. Can be:GET
orPOST
.
- StatusCallback string? - The URL we should call using the
status_callback_method
to send status information to your application.
- StatusCallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
status_callback
. Can be:GET
orPOST
.
- VoiceCallerIdLookup boolean? - Whether we should look up the caller's caller-ID name from the CNAM database (additional charges apply). Can be:
true
orfalse
.
- SmsUrl string? - The URL we should call when the phone number receives an incoming SMS message.
- SmsMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
sms_url
. Can be:GET
orPOST
.
- SmsFallbackUrl string? - The URL that we should call when an error occurs while retrieving or executing the TwiML from
sms_url
.
- SmsFallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
sms_fallback_url
. Can be:GET
orPOST
.
- SmsStatusCallback string? - The URL we should call using a POST method to send status information about SMS messages sent by the application.
- MessageStatusCallback string? - The URL we should call using a POST method to send message status information to your application.
- FriendlyName string? - A descriptive string that you create to describe the new application. It can be up to 64 characters long.
- PublicApplicationConnectEnabled boolean? - Whether to allow other Twilio accounts to dial this applicaton using Dial verb. Can be:
true
orfalse
.
twilio: CreateCallFeedbackSummaryRequest
Fields
- StartDate string - Only include feedback given on or after this date. Format is
YYYY-MM-DD
and specified in UTC.
- EndDate string - Only include feedback given on or before this date. Format is
YYYY-MM-DD
and specified in UTC.
- IncludeSubaccounts boolean? - Whether to also include Feedback resources from all subaccounts.
true
includes feedback from all subaccounts andfalse
, the default, includes feedback from only the specified account.
- StatusCallback string? - The URL that we will request when the feedback summary is complete.
- StatusCallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method (
GET
orPOST
) we use to make the request to theStatusCallback
URL.
twilio: CreateCallRecordingRequest
Fields
- RecordingStatusCallbackEvent string[]? - The recording status events on which we should call the
recording_status_callback
URL. Can be:in-progress
,completed
andabsent
and the default iscompleted
. Separate multiple event values with a space.
- RecordingStatusCallback string? - The URL we should call using the
recording_status_callback_method
on each recording event specified inrecording_status_callback_event
. For more information, see RecordingStatusCallback parameters.
- RecordingStatusCallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
recording_status_callback
. Can be:GET
orPOST
and the default isPOST
.
- Trim string? - Whether to trim any leading and trailing silence in the recording. Can be:
trim-silence
ordo-not-trim
and the default isdo-not-trim
.trim-silence
trims the silence from the beginning and end of the recording anddo-not-trim
does not.
- RecordingChannels string? - The number of channels used in the recording. Can be:
mono
ordual
and the default ismono
.mono
records all parties of the call into one channel.dual
records each party of a 2-party call into separate channels.
- RecordingTrack string? - The audio track to record for the call. Can be:
inbound
,outbound
orboth
. The default isboth
.inbound
records the audio that is received by Twilio.outbound
records the audio that is generated from Twilio.both
records the audio that is received and generated by Twilio.
twilio: CreateCallRequest
Fields
- To string - The phone number, SIP address, or client identifier to call.
- From string - The phone number or client identifier to use as the caller id. If using a phone number, it must be a Twilio number or a Verified outgoing caller id for your account. If the
to
parameter is a phone number,From
must also be a phone number.
- Method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use when calling the
url
parameter's value. Can be:GET
orPOST
and the default isPOST
. If anapplication_sid
parameter is present, this parameter is ignored.
- FallbackUrl string? - The URL that we call using the
fallback_method
if an error occurs when requesting or executing the TwiML aturl
. If anapplication_sid
parameter is present, this parameter is ignored.
- FallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method that we should use to request the
fallback_url
. Can be:GET
orPOST
and the default isPOST
. If anapplication_sid
parameter is present, this parameter is ignored.
- StatusCallback string? - The URL we should call using the
status_callback_method
to send status information to your application. If nostatus_callback_event
is specified, we will send thecompleted
status. If anapplication_sid
parameter is present, this parameter is ignored. URLs must contain a valid hostname (underscores are not permitted).
- StatusCallbackEvent string[]? - The call progress events that we will send to the
status_callback
URL. Can be:initiated
,ringing
,answered
, andcompleted
. If no event is specified, we send thecompleted
status. If you want to receive multiple events, specify each one in a separatestatus_callback_event
parameter. See the code sample for monitoring call progress. If anapplication_sid
is present, this parameter is ignored.
- StatusCallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use when calling the
status_callback
URL. Can be:GET
orPOST
and the default isPOST
. If anapplication_sid
parameter is present, this parameter is ignored.
- SendDigits string? - A string of keys to dial after connecting to the number, maximum of 32 digits. Valid digits in the string include: any digit (
0
-9
), '#
', '*
' and 'w
', to insert a half second pause. For example, if you connected to a company phone number and wanted to pause for one second, and then dial extension 1234 followed by the pound key, the value of this parameter would beww1234#
. Remember to URL-encode this string, since the '#
' character has special meaning in a URL. If bothSendDigits
andMachineDetection
parameters are provided, thenMachineDetection
will be ignored.
- Timeout int? - The integer number of seconds that we should allow the phone to ring before assuming there is no answer. The default is
60
seconds and the maximum is600
seconds. For some call flows, we will add a 5-second buffer to the timeout value you provide. For this reason, a timeout value of 10 seconds could result in an actual timeout closer to 15 seconds. You can set this to a short time, such as15
seconds, to hang up before reaching an answering machine or voicemail.
- Record boolean? - Whether to record the call. Can be
true
to record the phone call, orfalse
to not. The default isfalse
. Therecording_url
is sent to thestatus_callback
URL.
- RecordingChannels string? - The number of channels in the final recording. Can be:
mono
ordual
. The default ismono
.mono
records both legs of the call in a single channel of the recording file.dual
records each leg to a separate channel of the recording file. The first channel of a dual-channel recording contains the parent call and the second channel contains the child call.
- RecordingStatusCallback string? - The URL that we call when the recording is available to be accessed.
- RecordingStatusCallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use when calling the
recording_status_callback
URL. Can be:GET
orPOST
and the default isPOST
.
- SipAuthUsername string? - The username used to authenticate the caller making a SIP call.
- SipAuthPassword string? - The password required to authenticate the user account specified in
sip_auth_username
.
- MachineDetection string? - Whether to detect if a human, answering machine, or fax has picked up the call. Can be:
Enable
orDetectMessageEnd
. UseEnable
if you would like us to returnAnsweredBy
as soon as the called party is identified. UseDetectMessageEnd
, if you would like to leave a message on an answering machine. Ifsend_digits
is provided, this parameter is ignored. For more information, see Answering Machine Detection.
- MachineDetectionTimeout int? - The number of seconds that we should attempt to detect an answering machine before timing out and sending a voice request with
AnsweredBy
ofunknown
. The default timeout is 30 seconds.
- RecordingStatusCallbackEvent string[]? - The recording status events that will trigger calls to the URL specified in
recording_status_callback
. Can be:in-progress
,completed
andabsent
. Defaults tocompleted
. Separate multiple values with a space.
- Trim string? - Whether to trim any leading and trailing silence from the recording. Can be:
trim-silence
ordo-not-trim
and the default istrim-silence
.
- CallerId string? - The phone number, SIP address, or Client identifier that made this call. Phone numbers are in E.164 format (e.g., +16175551212). SIP addresses are formatted as
name@company.com
.
- MachineDetectionSpeechThreshold int? - The number of milliseconds that is used as the measuring stick for the length of the speech activity, where durations lower than this value will be interpreted as a human and longer than this value as a machine. Possible Values: 1000-6000. Default: 2400.
- MachineDetectionSpeechEndThreshold int? - The number of milliseconds of silence after speech activity at which point the speech activity is considered complete. Possible Values: 500-5000. Default: 1200.
- MachineDetectionSilenceTimeout int? - The number of milliseconds of initial silence after which an
unknown
AnsweredBy result will be returned. Possible Values: 2000-10000. Default: 5000.
- AsyncAmd string? - Select whether to perform answering machine detection in the background. Default, blocks the execution of the call until Answering Machine Detection is completed. Can be:
true
orfalse
.
- AsyncAmdStatusCallback string? - The URL that we should call using the
async_amd_status_callback_method
to notify customer application whether the call was answered by human, machine or fax.
- AsyncAmdStatusCallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use when calling the
async_amd_status_callback
URL. Can be:GET
orPOST
and the default isPOST
.
- Byoc string? - The SID of a BYOC (Bring Your Own Carrier) trunk to route this call with. Note that
byoc
is only meaningful whento
is a phone number; it will otherwise be ignored. (Beta)
- CallReason string? - The Reason for the outgoing call. Use it to specify the purpose of the call that is presented on the called party's phone. (Branded Calls Beta)
- CallToken string? - A token string needed to invoke a forwarded call. A call_token is generated when an incoming call is received on a Twilio number. Pass an incoming call's call_token value to a forwarded call via the call_token parameter when creating a new call. A forwarded call should bear the same CallerID of the original incoming call.
- RecordingTrack string? - The audio track to record for the call. Can be:
inbound
,outbound
orboth
. The default isboth
.inbound
records the audio that is received by Twilio.outbound
records the audio that is generated from Twilio.both
records the audio that is received and generated by Twilio.
- TimeLimit int? - The maximum duration of the call in seconds. Constraints depend on account and configuration.
- Url string? - The absolute URL that returns the TwiML instructions for the call. We will call this URL using the
method
when the call connects. For more information, see the Url Parameter section in Making Calls.
- Twiml string? - TwiML instructions for the call Twilio will use without fetching Twiml from url parameter. If both
twiml
andurl
are provided thentwiml
parameter will be ignored. Max 4000 characters.
- ApplicationSid string? - The SID of the Application resource that will handle the call, if the call will be handled by an application.
twilio: CreateIncomingPhoneNumberAssignedAddOnRequest
Fields
- InstalledAddOnSid string - The SID that identifies the Add-on installation.
twilio: CreateIncomingPhoneNumberLocalRequest
Fields
- ApiVersion string? - The API version to use for incoming calls made to the new phone number. The default is
2010-04-01
.
- FriendlyName string? - A descriptive string that you created to describe the new phone number. It can be up to 64 characters long. By default, this is a formatted version of the phone number.
- SmsApplicationSid string? - The SID of the application that should handle SMS messages sent to the new phone number. If an
sms_application_sid
is present, we ignore all of thesms_*_url
urls and use those set on the application.
- SmsFallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method that we should use to call
sms_fallback_url
. Can be:GET
orPOST
and defaults toPOST
.
- SmsFallbackUrl string? - The URL that we should call when an error occurs while requesting or executing the TwiML defined by
sms_url
.
- SmsMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method that we should use to call
sms_url
. Can be:GET
orPOST
and defaults toPOST
.
- SmsUrl string? - The URL we should call when the new phone number receives an incoming SMS message.
- StatusCallback string? - The URL we should call using the
status_callback_method
to send status information to your application.
- StatusCallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
status_callback
. Can be:GET
orPOST
and defaults toPOST
.
- VoiceApplicationSid string? - The SID of the application we should use to handle calls to the new phone number. If a
voice_application_sid
is present, we ignore all of the voice urls and use only those set on the application. Setting avoice_application_sid
will automatically delete yourtrunk_sid
and vice versa.
- VoiceCallerIdLookup boolean? - Whether to lookup the caller's name from the CNAM database and post it to your app. Can be:
true
orfalse
and defaults tofalse
.
- VoiceFallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method that we should use to call
voice_fallback_url
. Can be:GET
orPOST
and defaults toPOST
.
- VoiceFallbackUrl string? - The URL that we should call when an error occurs retrieving or executing the TwiML requested by
url
.
- VoiceMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method that we should use to call
voice_url
. Can be:GET
orPOST
and defaults toPOST
.
- VoiceUrl string? - The URL that we should call to answer a call to the new phone number. The
voice_url
will not be called if avoice_application_sid
or atrunk_sid
is set.
- IdentitySid string? - The SID of the Identity resource that we should associate with the new phone number. Some regions require an identity to meet local regulations.
- AddressSid string? - The SID of the Address resource we should associate with the new phone number. Some regions require addresses to meet local regulations.
- EmergencyStatus Incoming_phone_number_local_enum_emergency_status? -
- EmergencyAddressSid string? - The SID of the emergency address configuration to use for emergency calling from the new phone number.
- TrunkSid string? - The SID of the Trunk we should use to handle calls to the new phone number. If a
trunk_sid
is present, we ignore all of the voice urls and voice applications and use only those set on the Trunk. Setting atrunk_sid
will automatically delete yourvoice_application_sid
and vice versa.
- VoiceReceiveMode Incoming_phone_number_local_enum_voice_receive_mode? -
- BundleSid string? - The SID of the Bundle resource that you associate with the phone number. Some regions require a Bundle to meet local Regulations.
twilio: CreateIncomingPhoneNumberMobileRequest
Fields
- ApiVersion string? - The API version to use for incoming calls made to the new phone number. The default is
2010-04-01
.
- FriendlyName string? - A descriptive string that you created to describe the new phone number. It can be up to 64 characters long. By default, the is a formatted version of the phone number.
- SmsApplicationSid string? - The SID of the application that should handle SMS messages sent to the new phone number. If an
sms_application_sid
is present, we ignore all of thesms_*_url
urls and use those of the application.
- SmsFallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method that we should use to call
sms_fallback_url
. Can be:GET
orPOST
and defaults toPOST
.
- SmsFallbackUrl string? - The URL that we should call when an error occurs while requesting or executing the TwiML defined by
sms_url
.
- SmsMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method that we should use to call
sms_url
. Can be:GET
orPOST
and defaults toPOST
.
- SmsUrl string? - The URL we should call when the new phone number receives an incoming SMS message.
- StatusCallback string? - The URL we should call using the
status_callback_method
to send status information to your application.
- StatusCallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
status_callback
. Can be:GET
orPOST
and defaults toPOST
.
- VoiceApplicationSid string? - The SID of the application we should use to handle calls to the new phone number. If a
voice_application_sid
is present, we ignore all of the voice urls and use only those set on the application. Setting avoice_application_sid
will automatically delete yourtrunk_sid
and vice versa.
- VoiceCallerIdLookup boolean? - Whether to lookup the caller's name from the CNAM database and post it to your app. Can be:
true
orfalse
and defaults tofalse
.
- VoiceFallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method that we should use to call
voice_fallback_url
. Can be:GET
orPOST
and defaults toPOST
.
- VoiceFallbackUrl string? - The URL that we should call when an error occurs retrieving or executing the TwiML requested by
url
.
- VoiceMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method that we should use to call
voice_url
. Can be:GET
orPOST
and defaults toPOST
.
- VoiceUrl string? - The URL that we should call to answer a call to the new phone number. The
voice_url
will not be called if avoice_application_sid
or atrunk_sid
is set.
- IdentitySid string? - The SID of the Identity resource that we should associate with the new phone number. Some regions require an identity to meet local regulations.
- AddressSid string? - The SID of the Address resource we should associate with the new phone number. Some regions require addresses to meet local regulations.
- EmergencyStatus Incoming_phone_number_mobile_enum_emergency_status? -
- EmergencyAddressSid string? - The SID of the emergency address configuration to use for emergency calling from the new phone number.
- TrunkSid string? - The SID of the Trunk we should use to handle calls to the new phone number. If a
trunk_sid
is present, we ignore all of the voice urls and voice applications and use only those set on the Trunk. Setting atrunk_sid
will automatically delete yourvoice_application_sid
and vice versa.
- VoiceReceiveMode Incoming_phone_number_mobile_enum_voice_receive_mode? -
- BundleSid string? - The SID of the Bundle resource that you associate with the phone number. Some regions require a Bundle to meet local Regulations.
twilio: CreateIncomingPhoneNumberRequest
Fields
- ApiVersion string? - The API version to use for incoming calls made to the new phone number. The default is
2010-04-01
.
- FriendlyName string? - A descriptive string that you created to describe the new phone number. It can be up to 64 characters long. By default, this is a formatted version of the new phone number.
- SmsApplicationSid string? - The SID of the application that should handle SMS messages sent to the new phone number. If an
sms_application_sid
is present, we ignore all of thesms_*_url
urls and use those set on the application.
- SmsFallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method that we should use to call
sms_fallback_url
. Can be:GET
orPOST
and defaults toPOST
.
- SmsFallbackUrl string? - The URL that we should call when an error occurs while requesting or executing the TwiML defined by
sms_url
.
- SmsMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method that we should use to call
sms_url
. Can be:GET
orPOST
and defaults toPOST
.
- SmsUrl string? - The URL we should call when the new phone number receives an incoming SMS message.
- StatusCallback string? - The URL we should call using the
status_callback_method
to send status information to your application.
- StatusCallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
status_callback
. Can be:GET
orPOST
and defaults toPOST
.
- VoiceApplicationSid string? - The SID of the application we should use to handle calls to the new phone number. If a
voice_application_sid
is present, we ignore all of the voice urls and use only those set on the application. Setting avoice_application_sid
will automatically delete yourtrunk_sid
and vice versa.
- VoiceCallerIdLookup boolean? - Whether to lookup the caller's name from the CNAM database and post it to your app. Can be:
true
orfalse
and defaults tofalse
.
- VoiceFallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method that we should use to call
voice_fallback_url
. Can be:GET
orPOST
and defaults toPOST
.
- VoiceFallbackUrl string? - The URL that we should call when an error occurs retrieving or executing the TwiML requested by
url
.
- VoiceMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method that we should use to call
voice_url
. Can be:GET
orPOST
and defaults toPOST
.
- VoiceUrl string? - The URL that we should call to answer a call to the new phone number. The
voice_url
will not be called if avoice_application_sid
or atrunk_sid
is set.
- EmergencyStatus Incoming_phone_number_enum_emergency_status? -
- EmergencyAddressSid string? - The SID of the emergency address configuration to use for emergency calling from the new phone number.
- TrunkSid string? - The SID of the Trunk we should use to handle calls to the new phone number. If a
trunk_sid
is present, we ignore all of the voice urls and voice applications and use only those set on the Trunk. Setting atrunk_sid
will automatically delete yourvoice_application_sid
and vice versa.
- IdentitySid string? - The SID of the Identity resource that we should associate with the new phone number. Some regions require an identity to meet local regulations.
- AddressSid string? - The SID of the Address resource we should associate with the new phone number. Some regions require addresses to meet local regulations.
- VoiceReceiveMode Incoming_phone_number_enum_voice_receive_mode? -
- BundleSid string? - The SID of the Bundle resource that you associate with the phone number. Some regions require a Bundle to meet local Regulations.
- AreaCode string? - The desired area code for your new incoming phone number. Can be any three-digit, US or Canada area code. We will provision an available phone number within this area code for you. You must provide an
area_code
or aphone_number
. (US and Canada only).
twilio: CreateIncomingPhoneNumberTollFreeRequest
Fields
- ApiVersion string? - The API version to use for incoming calls made to the new phone number. The default is
2010-04-01
.
- FriendlyName string? - A descriptive string that you created to describe the new phone number. It can be up to 64 characters long. By default, this is a formatted version of the phone number.
- SmsApplicationSid string? - The SID of the application that should handle SMS messages sent to the new phone number. If an
sms_application_sid
is present, we ignore allsms_*_url
values and use those of the application.
- SmsFallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method that we should use to call
sms_fallback_url
. Can be:GET
orPOST
and defaults toPOST
.
- SmsFallbackUrl string? - The URL that we should call when an error occurs while requesting or executing the TwiML defined by
sms_url
.
- SmsMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method that we should use to call
sms_url
. Can be:GET
orPOST
and defaults toPOST
.
- SmsUrl string? - The URL we should call when the new phone number receives an incoming SMS message.
- StatusCallback string? - The URL we should call using the
status_callback_method
to send status information to your application.
- StatusCallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
status_callback
. Can be:GET
orPOST
and defaults toPOST
.
- VoiceApplicationSid string? - The SID of the application we should use to handle calls to the new phone number. If a
voice_application_sid
is present, we ignore all of the voice urls and use those set on the application. Setting avoice_application_sid
will automatically delete yourtrunk_sid
and vice versa.
- VoiceCallerIdLookup boolean? - Whether to lookup the caller's name from the CNAM database and post it to your app. Can be:
true
orfalse
and defaults tofalse
.
- VoiceFallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method that we should use to call
voice_fallback_url
. Can be:GET
orPOST
and defaults toPOST
.
- VoiceFallbackUrl string? - The URL that we should call when an error occurs retrieving or executing the TwiML requested by
url
.
- VoiceMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method that we should use to call
voice_url
. Can be:GET
orPOST
and defaults toPOST
.
- VoiceUrl string? - The URL that we should call to answer a call to the new phone number. The
voice_url
will not be called if avoice_application_sid
or atrunk_sid
is set.
- IdentitySid string? - The SID of the Identity resource that we should associate with the new phone number. Some regions require an Identity to meet local regulations.
- AddressSid string? - The SID of the Address resource we should associate with the new phone number. Some regions require addresses to meet local regulations.
- EmergencyStatus Incoming_phone_number_toll_free_enum_emergency_status? -
- EmergencyAddressSid string? - The SID of the emergency address configuration to use for emergency calling from the new phone number.
- TrunkSid string? - The SID of the Trunk we should use to handle calls to the new phone number. If a
trunk_sid
is present, we ignore all of the voice urls and voice applications and use only those set on the Trunk. Setting atrunk_sid
will automatically delete yourvoice_application_sid
and vice versa.
- VoiceReceiveMode Incoming_phone_number_toll_free_enum_voice_receive_mode? -
- BundleSid string? - The SID of the Bundle resource that you associate with the phone number. Some regions require a Bundle to meet local Regulations.
twilio: CreateMessageFeedbackRequest
Fields
- Outcome Message_feedback_enum_outcome? -
twilio: CreateMessageRequest
Fields
- To string - The recipient's phone number in E.164 format (for SMS/MMS) or channel address, e.g.
whatsapp:+15552229999
.
- StatusCallback string? - The URL of the endpoint to which Twilio sends Message status callback requests. URL must contain a valid hostname and underscores are not allowed. If you include this parameter with the
messaging_service_sid
, Twilio uses this URL instead of the Status Callback URL of the Messaging Service.
- ApplicationSid string? - The SID of the associated TwiML Application. If this parameter is provided, the
status_callback
parameter of this request is ignored; Message status callback requests are sent to the TwiML App'smessage_status_callback
URL.
- MaxPrice decimal? - The maximum price in US dollars that you are willing to pay for this Message's delivery. The value can have up to four decimal places. When the
max_price
parameter is provided, the cost of a message is checked before it is sent. If the cost exceedsmax_price
, the message is not sent and the Messagestatus
isfailed
.
- ProvideFeedback boolean? - Boolean indicating whether or not you intend to provide delivery confirmation feedback to Twilio (used in conjunction with the Message Feedback subresource). Default value is
false
.
- Attempt int? - Total number of attempts made (including this request) to send the message regardless of the provider used
- ValidityPeriod int? - The maximum length in seconds that the Message can remain in Twilio's outgoing message queue. If a queued Message exceeds the
validity_period
, the Message is not sent. Accepted values are integers from1
to14400
. Default value is14400
. Avalidity_period
greater than5
is recommended. Learn more about the validity period
- ForceDelivery boolean? - Reserved
- ContentRetention Message_enum_content_retention? -
- AddressRetention Message_enum_address_retention? -
- SmartEncoded boolean? - Whether to detect Unicode characters that have a similar GSM-7 character and replace them. Can be:
true
orfalse
.
- PersistentAction string[]? - Rich actions for non-SMS/MMS channels. Used for sending location in WhatsApp messages.
- ShortenUrls boolean? - For Messaging Services with Link Shortening configured only: A Boolean indicating whether or not Twilio should shorten links in the
body
of the Message. Default value isfalse
. Iftrue
, themessaging_service_sid
parameter must also be provided.
- ScheduleType Message_enum_schedule_type? -
- SendAt string? - The time that Twilio will send the message. Must be in ISO 8601 format.
- SendAsMms boolean? - If set to
true
, Twilio delivers the message as a single MMS message, regardless of the presence of media.
- ContentVariables string? - For Content Editor/API only: Key-value pairs of Template variables and their substitution values.
content_sid
parameter must also be provided. If values are not defined in thecontent_variables
parameter, the Template's default placeholder values are used.
- RiskCheck Message_enum_risk_check? -
- From string? - The sender's Twilio phone number (in E.164 format), alphanumeric sender ID, Wireless SIM, short code, or channel address (e.g.,
whatsapp:+15554449999
). The value of thefrom
parameter must be a sender that is hosted within Twilio and belongs to the Account creating the Message. If you are usingmessaging_service_sid
, this parameter can be empty (Twilio assigns afrom
value from the Messaging Service's Sender Pool) or you can provide a specific sender from your Sender Pool.
- MessagingServiceSid string? - The SID of the Messaging Service you want to associate with the Message. When this parameter is provided and the
from
parameter is omitted, Twilio selects the optimal sender from the Messaging Service's Sender Pool. You may also provide afrom
parameter if you want to use a specific Sender from the Sender Pool.
- Body string? - The text content of the outgoing message. Can be up to 1,600 characters in length. SMS only: If the
body
contains more than 160 GSM-7 characters (or 70 UCS-2 characters), the message is segmented and charged accordingly. For longbody
text, consider using the send_as_mms parameter.
- MediaUrl string[]? - The URL of media to include in the Message content.
jpeg
,jpg
,gif
, andpng
file types are fully supported by Twilio and content is formatted for delivery on destination devices. The media size limit is 5 MB for supported file types (jpeg
,jpg
,png
,gif
) and 500 KB for other types of accepted media. To send more than one image in the message, provide multiplemedia_url
parameters in the POST request. You can include up to tenmedia_url
parameters per message. International and carrier limits apply.
- ContentSid string? - For Content Editor/API only: The SID of the Content Template to be used with the Message, e.g.,
HXXXXXXXXXXXXXXXXXXXXXXXXXXXXX
. If this parameter is not provided, a Content Template is not used. Find the SID in the Console on the Content Editor page. For Content API users, the SID is found in Twilio's response when creating the Template or by fetching your Templates.
twilio: CreateNewKeyRequest
Fields
- FriendlyName string? - A descriptive string that you create to describe the resource. It can be up to 64 characters long.
twilio: CreateNewSigningKeyRequest
Fields
- FriendlyName string? - A descriptive string that you create to describe the resource. It can be up to 64 characters long.
twilio: CreateParticipantRequest
Fields
- From string - The phone number, Client identifier, or username portion of SIP address that made this call. Phone numbers are in E.164 format (e.g., +16175551212). Client identifiers are formatted
client:name
. If using a phone number, it must be a Twilio number or a Verified outgoing caller id for your account. If theto
parameter is a phone number,from
must also be a phone number. Ifto
is sip address, this value offrom
should be a username portion to be used to populate the P-Asserted-Identity header that is passed to the SIP endpoint.
- To string - The phone number, SIP address, or Client identifier that received this call. Phone numbers are in E.164 format (e.g., +16175551212). SIP addresses are formatted as
sip:name@company.com
. Client identifiers are formattedclient:name
. Custom parameters may also be specified.
- StatusCallback string? - The URL we should call using the
status_callback_method
to send status information to your application.
- StatusCallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
status_callback
. Can be:GET
andPOST
and defaults toPOST
.
- StatusCallbackEvent string[]? - The conference state changes that should generate a call to
status_callback
. Can be:initiated
,ringing
,answered
, andcompleted
. Separate multiple values with a space. The default value iscompleted
.
- Label string? - A label for this participant. If one is supplied, it may subsequently be used to fetch, update or delete the participant.
- Timeout int? - The number of seconds that we should allow the phone to ring before assuming there is no answer. Can be an integer between
5
and600
, inclusive. The default value is60
. We always add a 5-second timeout buffer to outgoing calls, so value of 10 would result in an actual timeout that was closer to 15 seconds.
- Record boolean? - Whether to record the participant and their conferences, including the time between conferences. Can be
true
orfalse
and the default isfalse
.
- Muted boolean? - Whether the agent is muted in the conference. Can be
true
orfalse
and the default isfalse
.
- Beep string? - Whether to play a notification beep to the conference when the participant joins. Can be:
true
,false
,onEnter
, oronExit
. The default value istrue
.
- StartConferenceOnEnter boolean? - Whether to start the conference when the participant joins, if it has not already started. Can be:
true
orfalse
and the default istrue
. Iffalse
and the conference has not started, the participant is muted and hears background music until another participant starts the conference.
- EndConferenceOnExit boolean? - Whether to end the conference when the participant leaves. Can be:
true
orfalse
and defaults tofalse
.
- WaitUrl string? - The URL we should call using the
wait_method
for the music to play while participants are waiting for the conference to start. The default value is the URL of our standard hold music. Learn more about hold music.
- WaitMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
wait_url
. Can beGET
orPOST
and the default isPOST
. When using a static audio file, this should beGET
so that we can cache the file.
- EarlyMedia boolean? - Whether to allow an agent to hear the state of the outbound call, including ringing or disconnect messages. Can be:
true
orfalse
and defaults totrue
.
- MaxParticipants int? - The maximum number of participants in the conference. Can be a positive integer from
2
to250
. The default value is250
.
- ConferenceRecord string? - Whether to record the conference the participant is joining. Can be:
true
,false
,record-from-start
, anddo-not-record
. The default value isfalse
.
- ConferenceTrim string? - Whether to trim leading and trailing silence from the conference recording. Can be:
trim-silence
ordo-not-trim
and defaults totrim-silence
.
- ConferenceStatusCallback string? - The URL we should call using the
conference_status_callback_method
when the conference events inconference_status_callback_event
occur. Only the value set by the first participant to join the conference is used. Subsequentconference_status_callback
values are ignored.
- ConferenceStatusCallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
conference_status_callback
. Can be:GET
orPOST
and defaults toPOST
.
- ConferenceStatusCallbackEvent string[]? - The conference state changes that should generate a call to
conference_status_callback
. Can be:start
,end
,join
,leave
,mute
,hold
,modify
,speaker
, andannouncement
. Separate multiple values with a space. Defaults tostart end
.
- RecordingChannels string? - The recording channels for the final recording. Can be:
mono
ordual
and the default ismono
.
- RecordingStatusCallback string? - The URL that we should call using the
recording_status_callback_method
when the recording status changes.
- RecordingStatusCallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use when we call
recording_status_callback
. Can be:GET
orPOST
and defaults toPOST
.
- SipAuthUsername string? - The SIP username used for authentication.
- SipAuthPassword string? - The SIP password for authentication.
- ConferenceRecordingStatusCallback string? - The URL we should call using the
conference_recording_status_callback_method
when the conference recording is available.
- ConferenceRecordingStatusCallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
conference_recording_status_callback
. Can be:GET
orPOST
and defaults toPOST
.
- RecordingStatusCallbackEvent string[]? - The recording state changes that should generate a call to
recording_status_callback
. Can be:started
,in-progress
,paused
,resumed
,stopped
,completed
,failed
, andabsent
. Separate multiple values with a space, ex:'in-progress completed failed'
.
- ConferenceRecordingStatusCallbackEvent string[]? - The conference recording state changes that generate a call to
conference_recording_status_callback
. Can be:in-progress
,completed
,failed
, andabsent
. Separate multiple values with a space, ex:'in-progress completed failed'
- Coaching boolean? - Whether the participant is coaching another call. Can be:
true
orfalse
. If not present, defaults tofalse
unlesscall_sid_to_coach
is defined. Iftrue
,call_sid_to_coach
must be defined.
- CallSidToCoach string? - The SID of the participant who is being
coached
. The participant being coached is the only participant who can hear the participant who iscoaching
.
- JitterBufferSize string? - Jitter buffer size for the connecting participant. Twilio will use this setting to apply Jitter Buffer before participant's audio is mixed into the conference. Can be:
off
,small
,medium
, andlarge
. Default tolarge
.
- Byoc string? - The SID of a BYOC (Bring Your Own Carrier) trunk to route this call with. Note that
byoc
is only meaningful whento
is a phone number; it will otherwise be ignored. (Beta)
- CallerId string? - The phone number, Client identifier, or username portion of SIP address that made this call. Phone numbers are in E.164 format (e.g., +16175551212). Client identifiers are formatted
client:name
. If using a phone number, it must be a Twilio number or a Verified outgoing caller id for your account. If theto
parameter is a phone number,callerId
must also be a phone number. Ifto
is sip address, this value ofcallerId
should be a username portion to be used to populate the From header that is passed to the SIP endpoint.
- CallReason string? - The Reason for the outgoing call. Use it to specify the purpose of the call that is presented on the called party's phone. (Branded Calls Beta)
- RecordingTrack string? - The audio track to record for the call. Can be:
inbound
,outbound
orboth
. The default isboth
.inbound
records the audio that is received by Twilio.outbound
records the audio that is sent from Twilio.both
records the audio that is received and sent by Twilio.
- TimeLimit int? - The maximum duration of the call in seconds. Constraints depend on account and configuration.
- MachineDetection string? - Whether to detect if a human, answering machine, or fax has picked up the call. Can be:
Enable
orDetectMessageEnd
. UseEnable
if you would like us to returnAnsweredBy
as soon as the called party is identified. UseDetectMessageEnd
, if you would like to leave a message on an answering machine. For more information, see Answering Machine Detection.
- MachineDetectionTimeout int? - The number of seconds that we should attempt to detect an answering machine before timing out and sending a voice request with
AnsweredBy
ofunknown
. The default timeout is 30 seconds.
- MachineDetectionSpeechThreshold int? - The number of milliseconds that is used as the measuring stick for the length of the speech activity, where durations lower than this value will be interpreted as a human and longer than this value as a machine. Possible Values: 1000-6000. Default: 2400.
- MachineDetectionSpeechEndThreshold int? - The number of milliseconds of silence after speech activity at which point the speech activity is considered complete. Possible Values: 500-5000. Default: 1200.
- MachineDetectionSilenceTimeout int? - The number of milliseconds of initial silence after which an
unknown
AnsweredBy result will be returned. Possible Values: 2000-10000. Default: 5000.
- AmdStatusCallback string? - The URL that we should call using the
amd_status_callback_method
to notify customer application whether the call was answered by human, machine or fax.
- AmdStatusCallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use when calling the
amd_status_callback
URL. Can be:GET
orPOST
and the default isPOST
.
- Trim string? - Whether to trim any leading and trailing silence from the participant recording. Can be:
trim-silence
ordo-not-trim
and the default istrim-silence
.
- CallToken string? - A token string needed to invoke a forwarded call. A call_token is generated when an incoming call is received on a Twilio number. Pass an incoming call's call_token value to a forwarded call via the call_token parameter when creating a new call. A forwarded call should bear the same CallerID of the original incoming call.
twilio: CreatePaymentsRequest
Fields
- IdempotencyKey string - A unique token that will be used to ensure that multiple API calls with the same information do not result in multiple transactions. This should be a unique string value per API call and can be a randomly generated.
- StatusCallback string - Provide an absolute or relative URL to receive status updates regarding your Pay session. Read more about the expected StatusCallback values
- BankAccountType Payments_enum_bank_account_type? -
- ChargeAmount decimal? - A positive decimal value less than 1,000,000 to charge against the credit card or bank account. Default currency can be overwritten with
currency
field. Leave blank or set to 0 to tokenize.
- Description string? - The description can be used to provide more details regarding the transaction. This information is submitted along with the payment details to the Payment Connector which are then posted on the transactions.
- Input string? - A list of inputs that should be accepted. Currently only
dtmf
is supported. All digits captured during a pay session are redacted from the logs.
- MinPostalCodeLength int? - A positive integer that is used to validate the length of the
PostalCode
inputted by the user. User must enter this many digits.
- Parameter anydata? - A single-level JSON object used to pass custom parameters to payment processors. (Required for ACH payments). The information that has to be included here depends on the <Pay> Connector. Read more.
- PaymentConnector string? - This is the unique name corresponding to the Pay Connector installed in the Twilio Add-ons. Learn more about <Pay> Connectors. The default value is
Default
.
- PaymentMethod Payments_enum_payment_method? -
- PostalCode boolean? - Indicates whether the credit card postal code (zip code) is a required piece of payment information that must be provided by the caller. The default is
true
.
- SecurityCode boolean? - Indicates whether the credit card security code is a required piece of payment information that must be provided by the caller. The default is
true
.
- Timeout int? - The number of seconds that <Pay> should wait for the caller to press a digit between each subsequent digit, after the first one, before moving on to validate the digits captured. The default is
5
, maximum is600
.
- TokenType Payments_enum_token_type? -
- ValidCardTypes string? - Credit card types separated by space that Pay should accept. The default value is
visa mastercard amex
twilio: CreateQueueRequest
Fields
- FriendlyName string - A descriptive string that you created to describe this resource. It can be up to 64 characters long.
- MaxSize int? - The maximum number of calls allowed to be in the queue. The default is 1000. The maximum is 5000.
twilio: CreateSipAuthCallsCredentialListMappingRequest
Fields
- CredentialListSid string - The SID of the CredentialList resource to map to the SIP domain.
twilio: CreateSipAuthCallsIpAccessControlListMappingRequest
Fields
- IpAccessControlListSid string - The SID of the IpAccessControlList resource to map to the SIP domain.
twilio: CreateSipAuthRegistrationsCredentialListMappingRequest
Fields
- CredentialListSid string - The SID of the CredentialList resource to map to the SIP domain.
twilio: CreateSipCredentialListMappingRequest
Fields
- CredentialListSid string - A 34 character string that uniquely identifies the CredentialList resource to map to the SIP domain.
twilio: CreateSipCredentialListRequest
Fields
- FriendlyName string - A human readable descriptive text that describes the CredentialList, up to 64 characters long.
twilio: CreateSipCredentialRequest
Fields
- Username string - The username that will be passed when authenticating SIP requests. The username should be sent in response to Twilio's challenge of the initial INVITE. It can be up to 32 characters long.
- Password string - The password that the username will use when authenticating SIP requests. The password must be a minimum of 12 characters, contain at least 1 digit, and have mixed case. (eg
IWasAtSignal2018
)
twilio: CreateSipDomainRequest
Fields
- DomainName string - The unique address you reserve on Twilio to which you route your SIP traffic. Domain names can contain letters, digits, and "-" and must end with
sip.twilio.com
.
- FriendlyName string? - A descriptive string that you created to describe the resource. It can be up to 64 characters long.
- VoiceUrl string? - The URL we should when the domain receives a call.
- VoiceMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
voice_url
. Can be:GET
orPOST
.
- VoiceFallbackUrl string? - The URL that we should call when an error occurs while retrieving or executing the TwiML from
voice_url
.
- VoiceFallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
voice_fallback_url
. Can be:GET
orPOST
.
- VoiceStatusCallbackUrl string? - The URL that we should call to pass status parameters (such as call ended) to your application.
- VoiceStatusCallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
voice_status_callback_url
. Can be:GET
orPOST
.
- SipRegistration boolean? - Whether to allow SIP Endpoints to register with the domain to receive calls. Can be
true
orfalse
.true
allows SIP Endpoints to register with the domain to receive calls,false
does not.
- EmergencyCallingEnabled boolean? - Whether emergency calling is enabled for the domain. If enabled, allows emergency calls on the domain from phone numbers with validated addresses.
- Secure boolean? - Whether secure SIP is enabled for the domain. If enabled, TLS will be enforced and SRTP will be negotiated on all incoming calls to this sip domain.
- ByocTrunkSid string? - The SID of the BYOC Trunk(Bring Your Own Carrier) resource that the Sip Domain will be associated with.
- EmergencyCallerSid string? - Whether an emergency caller sid is configured for the domain. If present, this phone number will be used as the callback for the emergency call.
twilio: CreateSipIpAccessControlListMappingRequest
Fields
- IpAccessControlListSid string - The unique id of the IP access control list to map to the SIP domain.
twilio: CreateSipIpAccessControlListRequest
Fields
- FriendlyName string - A human readable descriptive text that describes the IpAccessControlList, up to 255 characters long.
twilio: CreateSipIpAddressRequest
Fields
- FriendlyName string - A human readable descriptive text for this resource, up to 255 characters long.
- IpAddress string - An IP address in dotted decimal notation from which you want to accept traffic. Any SIP requests from this IP address will be allowed by Twilio. IPv4 only supported today.
- CidrPrefixLength int? - An integer representing the length of the CIDR prefix to use with this IP address when accepting traffic. By default the entire IP address is used.
twilio: CreateSiprecRequest
Fields
- Name string? - The user-specified name of this Siprec, if one was given when the Siprec was created. This may be used to stop the Siprec.
- ConnectorName string? - Unique name used when configuring the connector via Marketplace Add-on.
- Track Siprec_enum_track? -
- StatusCallback string? - Absolute URL of the status callback.
- StatusCallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The http method for the status_callback (one of GET, POST).
- Parameter1\.Name string? - Parameter name
- Parameter1\.Value string? - Parameter value
- Parameter2\.Name string? - Parameter name
- Parameter2\.Value string? - Parameter value
- Parameter3\.Name string? - Parameter name
- Parameter3\.Value string? - Parameter value
- Parameter4\.Name string? - Parameter name
- Parameter4\.Value string? - Parameter value
- Parameter5\.Name string? - Parameter name
- Parameter5\.Value string? - Parameter value
- Parameter6\.Name string? - Parameter name
- Parameter6\.Value string? - Parameter value
- Parameter7\.Name string? - Parameter name
- Parameter7\.Value string? - Parameter value
- Parameter8\.Name string? - Parameter name
- Parameter8\.Value string? - Parameter value
- Parameter9\.Name string? - Parameter name
- Parameter9\.Value string? - Parameter value
- Parameter10\.Name string? - Parameter name
- Parameter10\.Value string? - Parameter value
- Parameter11\.Name string? - Parameter name
- Parameter11\.Value string? - Parameter value
- Parameter12\.Name string? - Parameter name
- Parameter12\.Value string? - Parameter value
- Parameter13\.Name string? - Parameter name
- Parameter13\.Value string? - Parameter value
- Parameter14\.Name string? - Parameter name
- Parameter14\.Value string? - Parameter value
- Parameter15\.Name string? - Parameter name
- Parameter15\.Value string? - Parameter value
- Parameter16\.Name string? - Parameter name
- Parameter16\.Value string? - Parameter value
- Parameter17\.Name string? - Parameter name
- Parameter17\.Value string? - Parameter value
- Parameter18\.Name string? - Parameter name
- Parameter18\.Value string? - Parameter value
- Parameter19\.Name string? - Parameter name
- Parameter19\.Value string? - Parameter value
- Parameter20\.Name string? - Parameter name
- Parameter20\.Value string? - Parameter value
- Parameter21\.Name string? - Parameter name
- Parameter21\.Value string? - Parameter value
- Parameter22\.Name string? - Parameter name
- Parameter22\.Value string? - Parameter value
- Parameter23\.Name string? - Parameter name
- Parameter23\.Value string? - Parameter value
- Parameter24\.Name string? - Parameter name
- Parameter24\.Value string? - Parameter value
- Parameter25\.Name string? - Parameter name
- Parameter25\.Value string? - Parameter value
- Parameter26\.Name string? - Parameter name
- Parameter26\.Value string? - Parameter value
- Parameter27\.Name string? - Parameter name
- Parameter27\.Value string? - Parameter value
- Parameter28\.Name string? - Parameter name
- Parameter28\.Value string? - Parameter value
- Parameter29\.Name string? - Parameter name
- Parameter29\.Value string? - Parameter value
- Parameter30\.Name string? - Parameter name
- Parameter30\.Value string? - Parameter value
- Parameter31\.Name string? - Parameter name
- Parameter31\.Value string? - Parameter value
- Parameter32\.Name string? - Parameter name
- Parameter32\.Value string? - Parameter value
- Parameter33\.Name string? - Parameter name
- Parameter33\.Value string? - Parameter value
- Parameter34\.Name string? - Parameter name
- Parameter34\.Value string? - Parameter value
- Parameter35\.Name string? - Parameter name
- Parameter35\.Value string? - Parameter value
- Parameter36\.Name string? - Parameter name
- Parameter36\.Value string? - Parameter value
- Parameter37\.Name string? - Parameter name
- Parameter37\.Value string? - Parameter value
- Parameter38\.Name string? - Parameter name
- Parameter38\.Value string? - Parameter value
- Parameter39\.Name string? - Parameter name
- Parameter39\.Value string? - Parameter value
- Parameter40\.Name string? - Parameter name
- Parameter40\.Value string? - Parameter value
- Parameter41\.Name string? - Parameter name
- Parameter41\.Value string? - Parameter value
- Parameter42\.Name string? - Parameter name
- Parameter42\.Value string? - Parameter value
- Parameter43\.Name string? - Parameter name
- Parameter43\.Value string? - Parameter value
- Parameter44\.Name string? - Parameter name
- Parameter44\.Value string? - Parameter value
- Parameter45\.Name string? - Parameter name
- Parameter45\.Value string? - Parameter value
- Parameter46\.Name string? - Parameter name
- Parameter46\.Value string? - Parameter value
- Parameter47\.Name string? - Parameter name
- Parameter47\.Value string? - Parameter value
- Parameter48\.Name string? - Parameter name
- Parameter48\.Value string? - Parameter value
- Parameter49\.Name string? - Parameter name
- Parameter49\.Value string? - Parameter value
- Parameter50\.Name string? - Parameter name
- Parameter50\.Value string? - Parameter value
- Parameter51\.Name string? - Parameter name
- Parameter51\.Value string? - Parameter value
- Parameter52\.Name string? - Parameter name
- Parameter52\.Value string? - Parameter value
- Parameter53\.Name string? - Parameter name
- Parameter53\.Value string? - Parameter value
- Parameter54\.Name string? - Parameter name
- Parameter54\.Value string? - Parameter value
- Parameter55\.Name string? - Parameter name
- Parameter55\.Value string? - Parameter value
- Parameter56\.Name string? - Parameter name
- Parameter56\.Value string? - Parameter value
- Parameter57\.Name string? - Parameter name
- Parameter57\.Value string? - Parameter value
- Parameter58\.Name string? - Parameter name
- Parameter58\.Value string? - Parameter value
- Parameter59\.Name string? - Parameter name
- Parameter59\.Value string? - Parameter value
- Parameter60\.Name string? - Parameter name
- Parameter60\.Value string? - Parameter value
- Parameter61\.Name string? - Parameter name
- Parameter61\.Value string? - Parameter value
- Parameter62\.Name string? - Parameter name
- Parameter62\.Value string? - Parameter value
- Parameter63\.Name string? - Parameter name
- Parameter63\.Value string? - Parameter value
- Parameter64\.Name string? - Parameter name
- Parameter64\.Value string? - Parameter value
- Parameter65\.Name string? - Parameter name
- Parameter65\.Value string? - Parameter value
- Parameter66\.Name string? - Parameter name
- Parameter66\.Value string? - Parameter value
- Parameter67\.Name string? - Parameter name
- Parameter67\.Value string? - Parameter value
- Parameter68\.Name string? - Parameter name
- Parameter68\.Value string? - Parameter value
- Parameter69\.Name string? - Parameter name
- Parameter69\.Value string? - Parameter value
- Parameter70\.Name string? - Parameter name
- Parameter70\.Value string? - Parameter value
- Parameter71\.Name string? - Parameter name
- Parameter71\.Value string? - Parameter value
- Parameter72\.Name string? - Parameter name
- Parameter72\.Value string? - Parameter value
- Parameter73\.Name string? - Parameter name
- Parameter73\.Value string? - Parameter value
- Parameter74\.Name string? - Parameter name
- Parameter74\.Value string? - Parameter value
- Parameter75\.Name string? - Parameter name
- Parameter75\.Value string? - Parameter value
- Parameter76\.Name string? - Parameter name
- Parameter76\.Value string? - Parameter value
- Parameter77\.Name string? - Parameter name
- Parameter77\.Value string? - Parameter value
- Parameter78\.Name string? - Parameter name
- Parameter78\.Value string? - Parameter value
- Parameter79\.Name string? - Parameter name
- Parameter79\.Value string? - Parameter value
- Parameter80\.Name string? - Parameter name
- Parameter80\.Value string? - Parameter value
- Parameter81\.Name string? - Parameter name
- Parameter81\.Value string? - Parameter value
- Parameter82\.Name string? - Parameter name
- Parameter82\.Value string? - Parameter value
- Parameter83\.Name string? - Parameter name
- Parameter83\.Value string? - Parameter value
- Parameter84\.Name string? - Parameter name
- Parameter84\.Value string? - Parameter value
- Parameter85\.Name string? - Parameter name
- Parameter85\.Value string? - Parameter value
- Parameter86\.Name string? - Parameter name
- Parameter86\.Value string? - Parameter value
- Parameter87\.Name string? - Parameter name
- Parameter87\.Value string? - Parameter value
- Parameter88\.Name string? - Parameter name
- Parameter88\.Value string? - Parameter value
- Parameter89\.Name string? - Parameter name
- Parameter89\.Value string? - Parameter value
- Parameter90\.Name string? - Parameter name
- Parameter90\.Value string? - Parameter value
- Parameter91\.Name string? - Parameter name
- Parameter91\.Value string? - Parameter value
- Parameter92\.Name string? - Parameter name
- Parameter92\.Value string? - Parameter value
- Parameter93\.Name string? - Parameter name
- Parameter93\.Value string? - Parameter value
- Parameter94\.Name string? - Parameter name
- Parameter94\.Value string? - Parameter value
- Parameter95\.Name string? - Parameter name
- Parameter95\.Value string? - Parameter value
- Parameter96\.Name string? - Parameter name
- Parameter96\.Value string? - Parameter value
- Parameter97\.Name string? - Parameter name
- Parameter97\.Value string? - Parameter value
- Parameter98\.Name string? - Parameter name
- Parameter98\.Value string? - Parameter value
- Parameter99\.Name string? - Parameter name
- Parameter99\.Value string? - Parameter value
twilio: CreateStreamRequest
Fields
- Url string - Relative or absolute url where WebSocket connection will be established.
- Name string? - The user-specified name of this Stream, if one was given when the Stream was created. This may be used to stop the Stream.
- Track Stream_enum_track? -
- StatusCallback string? - Absolute URL of the status callback.
- StatusCallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The http method for the status_callback (one of GET, POST).
- Parameter1\.Name string? - Parameter name
- Parameter1\.Value string? - Parameter value
- Parameter2\.Name string? - Parameter name
- Parameter2\.Value string? - Parameter value
- Parameter3\.Name string? - Parameter name
- Parameter3\.Value string? - Parameter value
- Parameter4\.Name string? - Parameter name
- Parameter4\.Value string? - Parameter value
- Parameter5\.Name string? - Parameter name
- Parameter5\.Value string? - Parameter value
- Parameter6\.Name string? - Parameter name
- Parameter6\.Value string? - Parameter value
- Parameter7\.Name string? - Parameter name
- Parameter7\.Value string? - Parameter value
- Parameter8\.Name string? - Parameter name
- Parameter8\.Value string? - Parameter value
- Parameter9\.Name string? - Parameter name
- Parameter9\.Value string? - Parameter value
- Parameter10\.Name string? - Parameter name
- Parameter10\.Value string? - Parameter value
- Parameter11\.Name string? - Parameter name
- Parameter11\.Value string? - Parameter value
- Parameter12\.Name string? - Parameter name
- Parameter12\.Value string? - Parameter value
- Parameter13\.Name string? - Parameter name
- Parameter13\.Value string? - Parameter value
- Parameter14\.Name string? - Parameter name
- Parameter14\.Value string? - Parameter value
- Parameter15\.Name string? - Parameter name
- Parameter15\.Value string? - Parameter value
- Parameter16\.Name string? - Parameter name
- Parameter16\.Value string? - Parameter value
- Parameter17\.Name string? - Parameter name
- Parameter17\.Value string? - Parameter value
- Parameter18\.Name string? - Parameter name
- Parameter18\.Value string? - Parameter value
- Parameter19\.Name string? - Parameter name
- Parameter19\.Value string? - Parameter value
- Parameter20\.Name string? - Parameter name
- Parameter20\.Value string? - Parameter value
- Parameter21\.Name string? - Parameter name
- Parameter21\.Value string? - Parameter value
- Parameter22\.Name string? - Parameter name
- Parameter22\.Value string? - Parameter value
- Parameter23\.Name string? - Parameter name
- Parameter23\.Value string? - Parameter value
- Parameter24\.Name string? - Parameter name
- Parameter24\.Value string? - Parameter value
- Parameter25\.Name string? - Parameter name
- Parameter25\.Value string? - Parameter value
- Parameter26\.Name string? - Parameter name
- Parameter26\.Value string? - Parameter value
- Parameter27\.Name string? - Parameter name
- Parameter27\.Value string? - Parameter value
- Parameter28\.Name string? - Parameter name
- Parameter28\.Value string? - Parameter value
- Parameter29\.Name string? - Parameter name
- Parameter29\.Value string? - Parameter value
- Parameter30\.Name string? - Parameter name
- Parameter30\.Value string? - Parameter value
- Parameter31\.Name string? - Parameter name
- Parameter31\.Value string? - Parameter value
- Parameter32\.Name string? - Parameter name
- Parameter32\.Value string? - Parameter value
- Parameter33\.Name string? - Parameter name
- Parameter33\.Value string? - Parameter value
- Parameter34\.Name string? - Parameter name
- Parameter34\.Value string? - Parameter value
- Parameter35\.Name string? - Parameter name
- Parameter35\.Value string? - Parameter value
- Parameter36\.Name string? - Parameter name
- Parameter36\.Value string? - Parameter value
- Parameter37\.Name string? - Parameter name
- Parameter37\.Value string? - Parameter value
- Parameter38\.Name string? - Parameter name
- Parameter38\.Value string? - Parameter value
- Parameter39\.Name string? - Parameter name
- Parameter39\.Value string? - Parameter value
- Parameter40\.Name string? - Parameter name
- Parameter40\.Value string? - Parameter value
- Parameter41\.Name string? - Parameter name
- Parameter41\.Value string? - Parameter value
- Parameter42\.Name string? - Parameter name
- Parameter42\.Value string? - Parameter value
- Parameter43\.Name string? - Parameter name
- Parameter43\.Value string? - Parameter value
- Parameter44\.Name string? - Parameter name
- Parameter44\.Value string? - Parameter value
- Parameter45\.Name string? - Parameter name
- Parameter45\.Value string? - Parameter value
- Parameter46\.Name string? - Parameter name
- Parameter46\.Value string? - Parameter value
- Parameter47\.Name string? - Parameter name
- Parameter47\.Value string? - Parameter value
- Parameter48\.Name string? - Parameter name
- Parameter48\.Value string? - Parameter value
- Parameter49\.Name string? - Parameter name
- Parameter49\.Value string? - Parameter value
- Parameter50\.Name string? - Parameter name
- Parameter50\.Value string? - Parameter value
- Parameter51\.Name string? - Parameter name
- Parameter51\.Value string? - Parameter value
- Parameter52\.Name string? - Parameter name
- Parameter52\.Value string? - Parameter value
- Parameter53\.Name string? - Parameter name
- Parameter53\.Value string? - Parameter value
- Parameter54\.Name string? - Parameter name
- Parameter54\.Value string? - Parameter value
- Parameter55\.Name string? - Parameter name
- Parameter55\.Value string? - Parameter value
- Parameter56\.Name string? - Parameter name
- Parameter56\.Value string? - Parameter value
- Parameter57\.Name string? - Parameter name
- Parameter57\.Value string? - Parameter value
- Parameter58\.Name string? - Parameter name
- Parameter58\.Value string? - Parameter value
- Parameter59\.Name string? - Parameter name
- Parameter59\.Value string? - Parameter value
- Parameter60\.Name string? - Parameter name
- Parameter60\.Value string? - Parameter value
- Parameter61\.Name string? - Parameter name
- Parameter61\.Value string? - Parameter value
- Parameter62\.Name string? - Parameter name
- Parameter62\.Value string? - Parameter value
- Parameter63\.Name string? - Parameter name
- Parameter63\.Value string? - Parameter value
- Parameter64\.Name string? - Parameter name
- Parameter64\.Value string? - Parameter value
- Parameter65\.Name string? - Parameter name
- Parameter65\.Value string? - Parameter value
- Parameter66\.Name string? - Parameter name
- Parameter66\.Value string? - Parameter value
- Parameter67\.Name string? - Parameter name
- Parameter67\.Value string? - Parameter value
- Parameter68\.Name string? - Parameter name
- Parameter68\.Value string? - Parameter value
- Parameter69\.Name string? - Parameter name
- Parameter69\.Value string? - Parameter value
- Parameter70\.Name string? - Parameter name
- Parameter70\.Value string? - Parameter value
- Parameter71\.Name string? - Parameter name
- Parameter71\.Value string? - Parameter value
- Parameter72\.Name string? - Parameter name
- Parameter72\.Value string? - Parameter value
- Parameter73\.Name string? - Parameter name
- Parameter73\.Value string? - Parameter value
- Parameter74\.Name string? - Parameter name
- Parameter74\.Value string? - Parameter value
- Parameter75\.Name string? - Parameter name
- Parameter75\.Value string? - Parameter value
- Parameter76\.Name string? - Parameter name
- Parameter76\.Value string? - Parameter value
- Parameter77\.Name string? - Parameter name
- Parameter77\.Value string? - Parameter value
- Parameter78\.Name string? - Parameter name
- Parameter78\.Value string? - Parameter value
- Parameter79\.Name string? - Parameter name
- Parameter79\.Value string? - Parameter value
- Parameter80\.Name string? - Parameter name
- Parameter80\.Value string? - Parameter value
- Parameter81\.Name string? - Parameter name
- Parameter81\.Value string? - Parameter value
- Parameter82\.Name string? - Parameter name
- Parameter82\.Value string? - Parameter value
- Parameter83\.Name string? - Parameter name
- Parameter83\.Value string? - Parameter value
- Parameter84\.Name string? - Parameter name
- Parameter84\.Value string? - Parameter value
- Parameter85\.Name string? - Parameter name
- Parameter85\.Value string? - Parameter value
- Parameter86\.Name string? - Parameter name
- Parameter86\.Value string? - Parameter value
- Parameter87\.Name string? - Parameter name
- Parameter87\.Value string? - Parameter value
- Parameter88\.Name string? - Parameter name
- Parameter88\.Value string? - Parameter value
- Parameter89\.Name string? - Parameter name
- Parameter89\.Value string? - Parameter value
- Parameter90\.Name string? - Parameter name
- Parameter90\.Value string? - Parameter value
- Parameter91\.Name string? - Parameter name
- Parameter91\.Value string? - Parameter value
- Parameter92\.Name string? - Parameter name
- Parameter92\.Value string? - Parameter value
- Parameter93\.Name string? - Parameter name
- Parameter93\.Value string? - Parameter value
- Parameter94\.Name string? - Parameter name
- Parameter94\.Value string? - Parameter value
- Parameter95\.Name string? - Parameter name
- Parameter95\.Value string? - Parameter value
- Parameter96\.Name string? - Parameter name
- Parameter96\.Value string? - Parameter value
- Parameter97\.Name string? - Parameter name
- Parameter97\.Value string? - Parameter value
- Parameter98\.Name string? - Parameter name
- Parameter98\.Value string? - Parameter value
- Parameter99\.Name string? - Parameter name
- Parameter99\.Value string? - Parameter value
twilio: CreateTokenRequest
Fields
- Ttl int? - The duration in seconds for which the generated credentials are valid. The default value is 86400 (24 hours).
twilio: CreateUsageTriggerRequest
Fields
- CallbackUrl string - The URL we should call using
callback_method
when the trigger fires.
- TriggerValue string - The usage value at which the trigger should fire. For convenience, you can use an offset value such as
+30
to specify a trigger_value that is 30 units more than the current usage value. Be sure to urlencode a+
as%2B
.
- UsageCategory Usage_trigger_enum_usage_category -
- CallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
callback_url
. Can be:GET
orPOST
and the default isPOST
.
- FriendlyName string? - A descriptive string that you create to describe the resource. It can be up to 64 characters long.
- Recurring Usage_trigger_enum_recurring? -
- TriggerBy Usage_trigger_enum_trigger_field? -
twilio: CreateUserDefinedMessageRequest
Fields
- Content string - The User Defined Message in the form of URL-encoded JSON string.
- IdempotencyKey string? - A unique string value to identify API call. This should be a unique string value per API call and can be a randomly generated.
twilio: CreateUserDefinedMessageSubscriptionRequest
Fields
- Callback string - The URL we should call using the
method
to send user defined events to your application. URLs must contain a valid hostname (underscores are not permitted).
- IdempotencyKey string? - A unique string value to identify API call. This should be a unique string value per API call and can be a randomly generated.
- Method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method Twilio will use when requesting the above
Url
. EitherGET
orPOST
. Default isPOST
.
twilio: CreateValidationRequest
Fields
- FriendlyName string? - A descriptive string that you create to describe the new caller ID resource. It can be up to 64 characters long. The default value is a formatted version of the phone number.
- CallDelay int? - The number of seconds to delay before initiating the verification call. Can be an integer between
0
and60
, inclusive. The default is0
.
- Extension string? - The digits to dial after connecting the verification call.
- StatusCallback string? - The URL we should call using the
status_callback_method
to send status information about the verification process to your application.
- StatusCallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
status_callback
. Can be:GET
orPOST
, and the default isPOST
.
twilio: Incoming_phone_number
Fields
- address_sid string? - The SID of the Address resource associated with the phone number.
- address_requirements Incoming_phone_number_enum_address_requirement? -
- api_version string? - The API version used to start a new TwiML session.
- beta boolean? - Whether the phone number is new to the Twilio platform. Can be:
true
orfalse
.
- capabilities Incoming_phone_number_capabilities? - The set of Boolean properties that indicate whether a phone number can receive calls or messages. Capabilities are
Voice
,SMS
, andMMS
and each capability can be:true
orfalse
.
- friendly_name string? - The string that you assigned to describe the resource.
- identity_sid string? - The SID of the Identity resource that we associate with the phone number. Some regions require an Identity to meet local regulations.
- origin string? - The phone number's origin.
twilio
identifies Twilio-owned phone numbers andhosted
identifies hosted phone numbers.
- sid string? - The unique string that that we created to identify this IncomingPhoneNumber resource.
- sms_application_sid string? - The SID of the application that handles SMS messages sent to the phone number. If an
sms_application_sid
is present, we ignore allsms_*_url
values and use those of the application.
- sms_fallback_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
sms_fallback_url
. Can be:GET
orPOST
.
- sms_fallback_url string? - The URL that we call when an error occurs while retrieving or executing the TwiML from
sms_url
.
- sms_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
sms_url
. Can be:GET
orPOST
.
- sms_url string? - The URL we call when the phone number receives an incoming SMS message.
- status_callback string? - The URL we call using the
status_callback_method
to send status information to your application.
- status_callback_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
status_callback
. Can be:GET
orPOST
.
- trunk_sid string? - The SID of the Trunk that handles calls to the phone number. If a
trunk_sid
is present, we ignore all of the voice urls and voice applications and use those set on the Trunk. Setting atrunk_sid
will automatically delete yourvoice_application_sid
and vice versa.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
- voice_receive_mode Incoming_phone_number_enum_voice_receive_mode? -
- voice_application_sid string? - The SID of the application that handles calls to the phone number. If a
voice_application_sid
is present, we ignore all of the voice urls and use those set on the application. Setting avoice_application_sid
will automatically delete yourtrunk_sid
and vice versa.
- voice_caller_id_lookup boolean? - Whether we look up the caller's caller-ID name from the CNAM database ($0.01 per look up). Can be:
true
orfalse
.
- voice_fallback_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
voice_fallback_url
. Can be:GET
orPOST
.
- voice_fallback_url string? - The URL that we call when an error occurs retrieving or executing the TwiML requested by
url
.
- voice_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
voice_url
. Can be:GET
orPOST
.
- voice_url string? - The URL we call when the phone number receives a call. The
voice_url
will not be used if avoice_application_sid
or atrunk_sid
is set.
- emergency_status Incoming_phone_number_enum_emergency_status? -
- emergency_address_sid string? - The SID of the emergency address configuration that we use for emergency calling from this phone number.
- emergency_address_status Incoming_phone_number_enum_emergency_address_status? -
- bundle_sid string? - The SID of the Bundle resource that you associate with the phone number. Some regions require a Bundle to meet local Regulations.
- status string? -
twilio: Incoming_phone_number_capabilities
The set of Boolean properties that indicate whether a phone number can receive calls or messages. Capabilities are Voice
, SMS
, and MMS
and each capability can be: true
or false
.
Fields
- mms boolean? - Whether the phone number can send and receive MMS messages. Can be:
true
orfalse
.
- sms boolean? - Whether the phone number can send and receive SMS messages. Can be:
true
orfalse
.
- voice boolean? - Whether the phone number can receive voice calls. Can be:
true
orfalse
.
- fax boolean? - Whether the phone number can receive faxes. Can be:
true
orfalse
.
twilio: Incoming_phone_numberIncoming_phone_number_assigned_add_on
Fields
- sid string? - The unique string that that we created to identify the resource.
- resource_sid string? - The SID of the Phone Number to which the Add-on is assigned.
- friendly_name string? - The string that you assigned to describe the resource.
- description string? - A short description of the functionality that the Add-on provides.
- configuration anydata? - A JSON string that represents the current configuration of this Add-on installation.
- unique_name string? - An application-defined string that uniquely identifies the resource. It can be used in place of the resource's
sid
in the URL to address the resource.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
- subresource_uris record {}? - A list of related resources identified by their relative URIs.
twilio: Incoming_phone_numberIncoming_phone_number_assigned_add_onIncoming_phone_number_assigned_add_on_extension
Fields
- sid string? - The unique string that that we created to identify the resource.
- resource_sid string? - The SID of the Phone Number to which the Add-on is assigned.
- assigned_add_on_sid string? - The SID that uniquely identifies the assigned Add-on installation.
- friendly_name string? - The string that you assigned to describe the resource.
- product_name string? - A string that you assigned to describe the Product this Extension is used within.
- unique_name string? - An application-defined string that uniquely identifies the resource. It can be used in place of the resource's
sid
in the URL to address the resource.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
- enabled boolean? - Whether the Extension will be invoked.
twilio: Incoming_phone_numberIncoming_phone_number_local
Fields
- address_sid string? - The SID of the Address resource associated with the phone number.
- address_requirements Incoming_phone_number_local_enum_address_requirement? -
- api_version string? - The API version used to start a new TwiML session.
- beta boolean? - Whether the phone number is new to the Twilio platform. Can be:
true
orfalse
.
- capabilities Incoming_phone_number_capabilities? - The set of Boolean properties that indicate whether a phone number can receive calls or messages. Capabilities are
Voice
,SMS
, andMMS
and each capability can be:true
orfalse
.
- friendly_name string? - The string that you assigned to describe the resource.
- identity_sid string? - The SID of the Identity resource that we associate with the phone number. Some regions require an Identity to meet local regulations.
- origin string? - The phone number's origin.
twilio
identifies Twilio-owned phone numbers andhosted
identifies hosted phone numbers.
- sid string? - The unique string that that we created to identify the resource.
- sms_application_sid string? - The SID of the application that handles SMS messages sent to the phone number. If an
sms_application_sid
is present, we ignore allsms_*_url
values and use those of the application.
- sms_fallback_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
sms_fallback_url
. Can be:GET
orPOST
.
- sms_fallback_url string? - The URL that we call when an error occurs while retrieving or executing the TwiML from
sms_url
.
- sms_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
sms_url
. Can be:GET
orPOST
.
- sms_url string? - The URL we call when the phone number receives an incoming SMS message.
- status_callback string? - The URL we call using the
status_callback_method
to send status information to your application.
- status_callback_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
status_callback
. Can be:GET
orPOST
.
- trunk_sid string? - The SID of the Trunk that handles calls to the phone number. If a
trunk_sid
is present, we ignore all of the voice urls and voice applications and use those set on the Trunk. Setting atrunk_sid
will automatically delete yourvoice_application_sid
and vice versa.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
- voice_receive_mode Incoming_phone_number_local_enum_voice_receive_mode? -
- voice_application_sid string? - The SID of the application that handles calls to the phone number. If a
voice_application_sid
is present, we ignore all of the voice urls and use those set on the application. Setting avoice_application_sid
will automatically delete yourtrunk_sid
and vice versa.
- voice_caller_id_lookup boolean? - Whether we look up the caller's caller-ID name from the CNAM database ($0.01 per look up). Can be:
true
orfalse
.
- voice_fallback_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
voice_fallback_url
. Can be:GET
orPOST
.
- voice_fallback_url string? - The URL that we call when an error occurs retrieving or executing the TwiML requested by
url
.
- voice_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
voice_url
. Can be:GET
orPOST
.
- voice_url string? - The URL we call when this phone number receives a call. The
voice_url
will not be used if avoice_application_sid
or atrunk_sid
is set.
- emergency_status Incoming_phone_number_local_enum_emergency_status? -
- emergency_address_sid string? - The SID of the emergency address configuration that we use for emergency calling from this phone number.
- emergency_address_status Incoming_phone_number_local_enum_emergency_address_status? -
- bundle_sid string? - The SID of the Bundle resource that you associate with the phone number. Some regions require a Bundle to meet local Regulations.
- status string? -
twilio: Incoming_phone_numberIncoming_phone_number_mobile
Fields
- address_sid string? - The SID of the Address resource associated with the phone number.
- address_requirements Incoming_phone_number_mobile_enum_address_requirement? -
- api_version string? - The API version used to start a new TwiML session.
- beta boolean? - Whether the phone number is new to the Twilio platform. Can be:
true
orfalse
.
- capabilities Incoming_phone_number_capabilities? - The set of Boolean properties that indicate whether a phone number can receive calls or messages. Capabilities are
Voice
,SMS
, andMMS
and each capability can be:true
orfalse
.
- friendly_name string? - The string that you assigned to describe the resource.
- identity_sid string? - The SID of the Identity resource that we associate with the phone number. Some regions require an Identity to meet local regulations.
- origin string? - The phone number's origin.
twilio
identifies Twilio-owned phone numbers andhosted
identifies hosted phone numbers.
- sid string? - The unique string that that we created to identify the resource.
- sms_application_sid string? - The SID of the application that handles SMS messages sent to the phone number. If an
sms_application_sid
is present, we ignore allsms_*_url
values and use those of the application.
- sms_fallback_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
sms_fallback_url
. Can be:GET
orPOST
.
- sms_fallback_url string? - The URL that we call when an error occurs while retrieving or executing the TwiML from
sms_url
.
- sms_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
sms_url
. Can be:GET
orPOST
.
- sms_url string? - The URL we call when the phone number receives an incoming SMS message.
- status_callback string? - The URL we call using the
status_callback_method
to send status information to your application.
- status_callback_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
status_callback
. Can be:GET
orPOST
.
- trunk_sid string? - The SID of the Trunk that handles calls to the phone number. If a
trunk_sid
is present, we ignore all of the voice urls and voice applications and use those set on the Trunk. Setting atrunk_sid
will automatically delete yourvoice_application_sid
and vice versa.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
- voice_receive_mode Incoming_phone_number_mobile_enum_voice_receive_mode? -
- voice_application_sid string? - The SID of the application that handles calls to the phone number. If a
voice_application_sid
is present, we ignore all of the voice urls and use those set on the application. Setting avoice_application_sid
will automatically delete yourtrunk_sid
and vice versa.
- voice_caller_id_lookup boolean? - Whether we look up the caller's caller-ID name from the CNAM database ($0.01 per look up). Can be:
true
orfalse
.
- voice_fallback_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
voice_fallback_url
. Can be:GET
orPOST
.
- voice_fallback_url string? - The URL that we call when an error occurs retrieving or executing the TwiML requested by
url
.
- voice_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
voice_url
. Can be:GET
orPOST
.
- voice_url string? - The URL we call when the phone number receives a call. The
voice_url
will not be used if avoice_application_sid
or atrunk_sid
is set.
- emergency_status Incoming_phone_number_mobile_enum_emergency_status? -
- emergency_address_sid string? - The SID of the emergency address configuration that we use for emergency calling from this phone number.
- emergency_address_status Incoming_phone_number_mobile_enum_emergency_address_status? -
- bundle_sid string? - The SID of the Bundle resource that you associate with the phone number. Some regions require a Bundle to meet local Regulations.
- status string? -
twilio: Incoming_phone_numberIncoming_phone_number_toll_free
Fields
- address_sid string? - The SID of the Address resource associated with the phone number.
- address_requirements Incoming_phone_number_toll_free_enum_address_requirement? -
- api_version string? - The API version used to start a new TwiML session.
- beta boolean? - Whether the phone number is new to the Twilio platform. Can be:
true
orfalse
.
- capabilities Incoming_phone_number_capabilities? - The set of Boolean properties that indicate whether a phone number can receive calls or messages. Capabilities are
Voice
,SMS
, andMMS
and each capability can be:true
orfalse
.
- friendly_name string? - The string that you assigned to describe the resource.
- identity_sid string? - The SID of the Identity resource that we associate with the phone number. Some regions require an Identity to meet local regulations.
- origin string? - The phone number's origin.
twilio
identifies Twilio-owned phone numbers andhosted
identifies hosted phone numbers.
- sid string? - The unique string that that we created to identify the resource.
- sms_application_sid string? - The SID of the application that handles SMS messages sent to the phone number. If an
sms_application_sid
is present, we ignore allsms_*_url
values and use those of the application.
- sms_fallback_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
sms_fallback_url
. Can be:GET
orPOST
.
- sms_fallback_url string? - The URL that we call when an error occurs while retrieving or executing the TwiML from
sms_url
.
- sms_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
sms_url
. Can be:GET
orPOST
.
- sms_url string? - The URL we call when the phone number receives an incoming SMS message.
- status_callback string? - The URL we call using the
status_callback_method
to send status information to your application.
- status_callback_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
status_callback
. Can be:GET
orPOST
.
- trunk_sid string? - The SID of the Trunk that handles calls to the phone number. If a
trunk_sid
is present, we ignore all of the voice urls and voice applications and use those set on the Trunk. Setting atrunk_sid
will automatically delete yourvoice_application_sid
and vice versa.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
- voice_receive_mode Incoming_phone_number_toll_free_enum_voice_receive_mode? -
- voice_application_sid string? - The SID of the application that handles calls to the phone number. If a
voice_application_sid
is present, we ignore all of the voice urls and use those set on the application. Setting avoice_application_sid
will automatically delete yourtrunk_sid
and vice versa.
- voice_caller_id_lookup boolean? - Whether we look up the caller's caller-ID name from the CNAM database ($0.01 per look up). Can be:
true
orfalse
.
- voice_fallback_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
voice_fallback_url
. Can be:GET
orPOST
.
- voice_fallback_url string? - The URL that we call when an error occurs retrieving or executing the TwiML requested by
url
.
- voice_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
voice_url
. Can be:GET
orPOST
.
- voice_url string? - The URL we call when the phone number receives a call. The
voice_url
will not be used if avoice_application_sid
or atrunk_sid
is set.
- emergency_status Incoming_phone_number_toll_free_enum_emergency_status? -
- emergency_address_sid string? - The SID of the emergency address configuration that we use for emergency calling from this phone number.
- emergency_address_status Incoming_phone_number_toll_free_enum_emergency_address_status? -
- bundle_sid string? - The SID of the Bundle resource that you associate with the phone number. Some regions require a Bundle to meet local Regulations.
- status string? -
twilio: Key
Fields
- sid string? - The unique string that that we created to identify the Key resource.
- friendly_name string? - The string that you assigned to describe the resource.
twilio: ListAccountResponse
Fields
- accounts Account[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListAddressResponse
Fields
- addresses Address[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListApplicationResponse
Fields
- applications Application[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListAuthorizedConnectAppResponse
Fields
- authorized_connect_apps Authorized_connect_app[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListAvailablePhoneNumberCountryResponse
Fields
- countries Available_phone_number_country[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListAvailablePhoneNumberLocalResponse
Fields
- available_phone_numbers Available_phone_number_local[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListAvailablePhoneNumberMachineToMachineResponse
Fields
- available_phone_numbers Available_phone_number_machine_to_machine[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListAvailablePhoneNumberMobileResponse
Fields
- available_phone_numbers Available_phone_number_mobile[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListAvailablePhoneNumberNationalResponse
Fields
- available_phone_numbers Available_phone_number_national[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListAvailablePhoneNumberSharedCostResponse
Fields
- available_phone_numbers Available_phone_number_shared_cost[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListAvailablePhoneNumberTollFreeResponse
Fields
- available_phone_numbers Available_phone_number_toll_free[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListAvailablePhoneNumberVoipResponse
Fields
- available_phone_numbers Available_phone_number_voip[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListCallEventResponse
Fields
- events CallCall_event[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListCallNotificationResponse
Fields
- notifications CallCall_notification[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListCallRecordingResponse
Fields
- recordings CallCall_recording[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListCallResponse
Fields
- calls Call[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListConferenceRecordingResponse
Fields
- recordings ConferenceConference_recording[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListConferenceResponse
Fields
- conferences Conference[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListConnectAppResponse
Fields
- connect_apps Connect_app[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListDependentPhoneNumberResponse
Fields
- dependent_phone_numbers AddressDependent_phone_number[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListIncomingPhoneNumberAssignedAddOnExtensionResponse
Fields
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListIncomingPhoneNumberAssignedAddOnResponse
Fields
- assigned_add_ons Incoming_phone_numberIncoming_phone_number_assigned_add_on[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListIncomingPhoneNumberLocalResponse
Fields
- incoming_phone_numbers Incoming_phone_numberIncoming_phone_number_local[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListIncomingPhoneNumberMobileResponse
Fields
- incoming_phone_numbers Incoming_phone_numberIncoming_phone_number_mobile[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListIncomingPhoneNumberResponse
Fields
- incoming_phone_numbers Incoming_phone_number[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListIncomingPhoneNumberTollFreeResponse
Fields
- incoming_phone_numbers Incoming_phone_numberIncoming_phone_number_toll_free[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListKeyResponse
Fields
- keys Key[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListMediaResponse
Fields
- media_list MessageMedia[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListMemberResponse
Fields
- queue_members QueueMember[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListMessageResponse
Fields
- messages Message[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListNotificationResponse
Fields
- notifications Notification[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListOutgoingCallerIdResponse
Fields
- outgoing_caller_ids Outgoing_caller_id[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListParticipantResponse
Fields
- participants ConferenceParticipant[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListQueueResponse
Fields
- queues Queue[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListRecordingAddOnResultPayloadResponse
Fields
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListRecordingAddOnResultResponse
Fields
- add_on_results RecordingRecording_add_on_result[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListRecordingResponse
Fields
- recordings Recording[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListRecordingTranscriptionResponse
Fields
- transcriptions RecordingRecording_transcription[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListShortCodeResponse
Fields
- short_codes Short_code[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListSigningKeyResponse
Fields
- signing_keys Signing_key[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListSipAuthCallsCredentialListMappingResponse
Fields
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListSipAuthCallsIpAccessControlListMappingResponse
Fields
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListSipAuthRegistrationsCredentialListMappingResponse
Fields
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListSipCredentialListMappingResponse
Fields
- credential_list_mappings SipSip_domainSip_credential_list_mapping[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListSipCredentialListResponse
Fields
- credential_lists SipSip_credential_list[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListSipCredentialResponse
Fields
- credentials SipSip_credential_listSip_credential[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListSipDomainResponse
Fields
- domains SipSip_domain[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListSipIpAccessControlListMappingResponse
Fields
- ip_access_control_list_mappings SipSip_domainSip_ip_access_control_list_mapping[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListSipIpAccessControlListResponse
Fields
- ip_access_control_lists SipSip_ip_access_control_list[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListSipIpAddressResponse
Fields
- ip_addresses SipSip_ip_access_control_listSip_ip_address[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListTranscriptionResponse
Fields
- transcriptions Transcription[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListUsageRecordAllTimeResponse
Fields
- usage_records UsageUsage_recordUsage_record_all_time[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListUsageRecordDailyResponse
Fields
- usage_records UsageUsage_recordUsage_record_daily[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListUsageRecordLastMonthResponse
Fields
- usage_records UsageUsage_recordUsage_record_last_month[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListUsageRecordMonthlyResponse
Fields
- usage_records UsageUsage_recordUsage_record_monthly[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListUsageRecordResponse
Fields
- usage_records UsageUsage_record[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListUsageRecordThisMonthResponse
Fields
- usage_records UsageUsage_recordUsage_record_this_month[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListUsageRecordTodayResponse
Fields
- usage_records UsageUsage_recordUsage_record_today[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListUsageRecordYearlyResponse
Fields
- usage_records UsageUsage_recordUsage_record_yearly[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListUsageRecordYesterdayResponse
Fields
- usage_records UsageUsage_recordUsage_record_yesterday[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: ListUsageTriggerResponse
Fields
- usage_triggers UsageUsage_trigger[]? -
- end int? -
- first_page_uri string? -
- next_page_uri string? -
- page int? -
- page_size int? -
- previous_page_uri string? -
- 'start int? -
- uri string? -
twilio: Message
Fields
- body string? - The text content of the message
- num_segments string? - The number of segments that make up the complete message. SMS message bodies that exceed the character limit are segmented and charged as multiple messages. Note: For messages sent via a Messaging Service,
num_segments
is initially0
, since a sender hasn't yet been assigned.
- direction Message_enum_direction? -
- 'from string? - The sender's phone number (in E.164 format), alphanumeric sender ID, Wireless SIM, short code, or channel address (e.g.,
whatsapp:+15554449999
). For incoming messages, this is the number or channel address of the sender. For outgoing messages, this value is a Twilio phone number, alphanumeric sender ID, short code, or channel address from which the message is sent.
- to string? - The recipient's phone number (in E.164 format) or channel address (e.g.
whatsapp:+15552229999
)
- price string? - The amount billed for the message in the currency specified by
price_unit
. Theprice
is populated after the message has been sent/received, and may not be immediately availalble. View the Pricing page for more details.
- error_message string? - The description of the
error_code
if the Messagestatus
isfailed
orundelivered
. If no error was encountered, the value isnull
.
- uri string? - The URI of the Message resource, relative to
https://api.twilio.com
.
- num_media string? - The number of media files associated with the Message resource.
- status Message_enum_status? -
- messaging_service_sid string? - The SID of the Messaging Service associated with the Message resource. The value is
null
if a Messaging Service was not used.
- sid string? - The unique, Twilio-provided string that identifies the Message resource.
- error_code int? - The error code returned if the Message
status
isfailed
orundelivered
. If no error was encountered, the value isnull
.
- api_version string? - The API version used to process the Message
- subresource_uris record {}? - A list of related resources identified by their URIs relative to
https://api.twilio.com
twilio: MessageMedia
Fields
- parent_sid string? - The SID of the Message resource that is associated with this Media resource.
- sid string? - The unique string that identifies this Media resource.
- uri string? - The URI of this Media resource, relative to
https://api.twilio.com
.
twilio: MessageMessage_feedback
Fields
- message_sid string? - The SID of the Message resource associated with this MessageFeedback resource.
- outcome Message_feedback_enum_outcome? -
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
twilio: New_key
Fields
- sid string? - The unique string that that we created to identify the NewKey resource. You will use this as the basic-auth
user
when authenticating to the API.
- friendly_name string? - The string that you assigned to describe the resource.
- secret string? - The secret your application uses to sign Access Tokens and to authenticate to the REST API (you will use this as the basic-auth
password
). Note that for security reasons, this field is ONLY returned when the API Key is first created.
twilio: New_signing_key
Fields
- sid string? - The unique string that that we created to identify the NewSigningKey resource.
- friendly_name string? - The string that you assigned to describe the resource.
- secret string? - The secret your application uses to sign Access Tokens and to authenticate to the REST API (you will use this as the basic-auth
password
). Note that for security reasons, this field is ONLY returned when the API Key is first created.
twilio: Notification
Fields
- api_version string? - The API version used to generate the notification. Can be empty for events that don't have a specific API version, such as incoming phone calls.
- error_code string? - A unique error code for the error condition that is described in our Error Dictionary.
- log string? - An integer log level that corresponds to the type of notification:
0
is ERROR,1
is WARNING.
- message_text string? - The text of the notification.
- more_info string? - The URL for more information about the error condition. This value is a page in our Error Dictionary.
- request_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method used to generate the notification. If the notification was generated during a phone call, this is the HTTP Method used to request the resource on your server. If the notification was generated by your use of our REST API, this is the HTTP method used to call the resource on our servers.
- request_url string? - The URL of the resource that generated the notification. If the notification was generated during a phone call, this is the URL of the resource on your server that caused the notification. If the notification was generated by your use of our REST API, this is the URL of the resource you called.
- sid string? - The unique string that that we created to identify the Notification resource.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
twilio: NotificationInstance
Fields
- api_version string? - The API version used to generate the notification. Can be empty for events that don't have a specific API version, such as incoming phone calls.
- error_code string? - A unique error code for the error condition that is described in our Error Dictionary.
- log string? - An integer log level that corresponds to the type of notification:
0
is ERROR,1
is WARNING.
- message_text string? - The text of the notification.
- more_info string? - The URL for more information about the error condition. This value is a page in our Error Dictionary.
- request_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method used to generate the notification. If the notification was generated during a phone call, this is the HTTP Method used to request the resource on your server. If the notification was generated by your use of our REST API, this is the HTTP method used to call the resource on our servers.
- request_url string? - The URL of the resource that generated the notification. If the notification was generated during a phone call, this is the URL of the resource on your server that caused the notification. If the notification was generated by your use of our REST API, this is the URL of the resource you called.
- request_variables string? - The HTTP GET or POST variables we sent to your server. However, if the notification was generated by our REST API, this contains the HTTP POST or PUT variables you sent to our API.
- response_body string? - The HTTP body returned by your server.
- response_headers string? - The HTTP headers returned by your server.
- sid string? - The unique string that that we created to identify the Notification resource.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
twilio: Outgoing_caller_id
Fields
- sid string? - The unique string that that we created to identify the OutgoingCallerId resource.
- friendly_name string? - The string that you assigned to describe the resource.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
twilio: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
twilio: Queue
Fields
- current_size int? - The number of calls currently in the queue.
- friendly_name string? - A string that you assigned to describe this resource.
- uri string? - The URI of this resource, relative to
https://api.twilio.com
.
- average_wait_time int? - The average wait time in seconds of the members in this queue. This is calculated at the time of the request.
- sid string? - The unique string that that we created to identify this Queue resource.
- max_size int? - The maximum number of calls that can be in the queue. The default is 1000 and the maximum is 5000.
twilio: QueueMember
Fields
- date_enqueued string? - The date that the member was enqueued, given in RFC 2822 format.
- position int? - This member's current position in the queue.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
- wait_time int? - The number of seconds the member has been in the queue.
- queue_sid string? - The SID of the Queue the member is in.
twilio: Recording
Fields
- api_version string? - The API version used during the recording.
- conference_sid string? - The Conference SID that identifies the conference associated with the recording, if a conference recording.
- duration string? - The length of the recording in seconds.
- sid string? - The unique string that that we created to identify the Recording resource.
- price string? - The one-time cost of creating the recording in the
price_unit
currency.
- price_unit string? - The currency used in the
price
property. Example:USD
.
- status Recording_enum_status? -
- channels int? - The number of channels in the final recording file. Can be:
1
or2
. You can split a call with two legs into two separate recording channels if you record using TwiML Dial or the Outbound Rest API.
- 'source Recording_enum_source? -
- error_code int? - The error code that describes why the recording is
absent
. The error code is described in our Error Dictionary. This value is null if the recordingstatus
is notabsent
.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
- encryption_details anydata? - How to decrypt the recording if it was encrypted using Call Recording Encryption feature.
- subresource_uris record {}? - A list of related resources identified by their relative URIs.
- media_url string? - The URL of the media file associated with this recording resource. When stored externally, this is the full URL location of the media file.
twilio: RecordingRecording_add_on_result
Fields
- sid string? - The unique string that that we created to identify the Recording AddOnResult resource.
- status Recording_add_on_result_enum_status? -
- add_on_sid string? - The SID of the Add-on to which the result belongs.
- add_on_configuration_sid string? - The SID of the Add-on configuration.
- reference_sid string? - The SID of the recording to which the AddOnResult resource belongs.
- subresource_uris record {}? - A list of related resources identified by their relative URIs.
twilio: RecordingRecording_add_on_resultRecording_add_on_result_payload
Fields
- sid string? - The unique string that that we created to identify the Recording AddOnResult Payload resource.
- add_on_result_sid string? - The SID of the AddOnResult to which the payload belongs.
- label string? - The string provided by the vendor that describes the payload.
- add_on_sid string? - The SID of the Add-on to which the result belongs.
- add_on_configuration_sid string? - The SID of the Add-on configuration.
- content_type string? - The MIME type of the payload.
- reference_sid string? - The SID of the recording to which the AddOnResult resource that contains the payload belongs.
- subresource_uris record {}? - A list of related resources identified by their relative URIs.
twilio: RecordingRecording_transcription
Fields
- api_version string? - The API version used to create the transcription.
- duration string? - The duration of the transcribed audio in seconds.
- price decimal? - The charge for the transcript in the currency associated with the account. This value is populated after the transcript is complete so it may not be available immediately.
- sid string? - The unique string that that we created to identify the Transcription resource.
- status Recording_transcription_enum_status? -
- transcription_text string? - The text content of the transcription.
- 'type string? - The transcription type.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
twilio: Short_code
Fields
- api_version string? - The API version used to start a new TwiML session when an SMS message is sent to this short code.
- friendly_name string? - A string that you assigned to describe this resource. By default, the
FriendlyName
is the short code.
- short_code string? - The short code. e.g., 894546.
- sid string? - The unique string that that we created to identify this ShortCode resource.
- sms_fallback_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call the
sms_fallback_url
. Can be:GET
orPOST
.
- sms_fallback_url string? - The URL that we call if an error occurs while retrieving or executing the TwiML from
sms_url
.
- sms_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call the
sms_url
. Can be:GET
orPOST
.
- sms_url string? - The URL we call when receiving an incoming SMS message to this short code.
- uri string? - The URI of this resource, relative to
https://api.twilio.com
.
twilio: Signing_key
Fields
- sid string? -
- friendly_name string? -
- date_created string? -
- date_updated string? -
twilio: Sip
twilio: SipSip_credential_list
Fields
- friendly_name string? - A human readable descriptive text that describes the CredentialList, up to 64 characters long.
- sid string? - A 34 character string that uniquely identifies this resource.
- subresource_uris record {}? - A list of credentials associated with this credential list.
- uri string? - The URI for this resource, relative to
https://api.twilio.com
.
twilio: SipSip_credential_listSip_credential
Fields
- sid string? - A 34 character string that uniquely identifies this resource.
- account_sid string? - The unique id of the Account that is responsible for this resource.
- credential_list_sid string? - The unique id that identifies the credential list that includes this credential.
- username string? - The username for this credential.
- uri string? - The URI for this resource, relative to
https://api.twilio.com
twilio: SipSip_domain
Fields
- api_version string? - The API version used to process the call.
- auth_type string? - The types of authentication you have mapped to your domain. Can be:
IP_ACL
andCREDENTIAL_LIST
. If you have both defined for your domain, both will be returned in a comma delimited string. Ifauth_type
is not defined, the domain will not be able to receive any traffic.
- domain_name string? - The unique address you reserve on Twilio to which you route your SIP traffic. Domain names can contain letters, digits, and "-" and must end with
sip.twilio.com
.
- friendly_name string? - The string that you assigned to describe the resource.
- sid string? - The unique string that that we created to identify the SipDomain resource.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
- voice_fallback_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
voice_fallback_url
. Can be:GET
orPOST
.
- voice_fallback_url string? - The URL that we call when an error occurs while retrieving or executing the TwiML requested from
voice_url
.
- voice_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
voice_url
. Can be:GET
orPOST
.
- voice_status_callback_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
voice_status_callback_url
. EitherGET
orPOST
.
- voice_status_callback_url string? - The URL that we call to pass status parameters (such as call ended) to your application.
- voice_url string? - The URL we call using the
voice_method
when the domain receives a call.
- subresource_uris record {}? - A list of mapping resources associated with the SIP Domain resource identified by their relative URIs.
- sip_registration boolean? - Whether to allow SIP Endpoints to register with the domain to receive calls.
- emergency_calling_enabled boolean? - Whether emergency calling is enabled for the domain. If enabled, allows emergency calls on the domain from phone numbers with validated addresses.
- secure boolean? - Whether secure SIP is enabled for the domain. If enabled, TLS will be enforced and SRTP will be negotiated on all incoming calls to this sip domain.
- byoc_trunk_sid string? - The SID of the BYOC Trunk(Bring Your Own Carrier) resource that the Sip Domain will be associated with.
- emergency_caller_sid string? - Whether an emergency caller sid is configured for the domain. If present, this phone number will be used as the callback for the emergency call.
twilio: SipSip_domainSip_auth
twilio: SipSip_domainSip_authSip_auth_calls
twilio: SipSip_domainSip_authSip_auth_callsSip_auth_calls_credential_list_mapping
Fields
- friendly_name string? - The string that you assigned to describe the resource.
- sid string? - The unique string that that we created to identify the CredentialListMapping resource.
twilio: SipSip_domainSip_authSip_auth_callsSip_auth_calls_ip_access_control_list_mapping
Fields
- friendly_name string? - The string that you assigned to describe the resource.
- sid string? - The unique string that that we created to identify the IpAccessControlListMapping resource.
twilio: SipSip_domainSip_authSip_auth_registrations
twilio: SipSip_domainSip_authSip_auth_registrationsSip_auth_registrations_credential_list_mapping
Fields
- friendly_name string? - The string that you assigned to describe the resource.
- sid string? - The unique string that that we created to identify the CredentialListMapping resource.
twilio: SipSip_domainSip_credential_list_mapping
Fields
- account_sid string? - The unique id of the Account that is responsible for this resource.
- domain_sid string? - The unique string that is created to identify the SipDomain resource.
- friendly_name string? - A human readable descriptive text for this resource, up to 64 characters long.
- sid string? - A 34 character string that uniquely identifies this resource.
- uri string? - The URI for this resource, relative to
https://api.twilio.com
twilio: SipSip_domainSip_ip_access_control_list_mapping
Fields
- account_sid string? - The unique id of the Account that is responsible for this resource.
- domain_sid string? - The unique string that is created to identify the SipDomain resource.
- friendly_name string? - A human readable descriptive text for this resource, up to 64 characters long.
- sid string? - A 34 character string that uniquely identifies this resource.
- uri string? - The URI for this resource, relative to
https://api.twilio.com
twilio: SipSip_ip_access_control_list
Fields
- sid string? - A 34 character string that uniquely identifies this resource.
- friendly_name string? - A human readable descriptive text, up to 255 characters long.
- subresource_uris record {}? - A list of the IpAddress resources associated with this IP access control list resource.
- uri string? - The URI for this resource, relative to
https://api.twilio.com
twilio: SipSip_ip_access_control_listSip_ip_address
Fields
- sid string? - A 34 character string that uniquely identifies this resource.
- account_sid string? - The unique id of the Account that is responsible for this resource.
- friendly_name string? - A human readable descriptive text for this resource, up to 255 characters long.
- ip_address string? - An IP address in dotted decimal notation from which you want to accept traffic. Any SIP requests from this IP address will be allowed by Twilio. IPv4 only supported today.
- cidr_prefix_length int? - An integer representing the length of the CIDR prefix to use with this IP address when accepting traffic. By default the entire IP address is used.
- ip_access_control_list_sid string? - The unique id of the IpAccessControlList resource that includes this resource.
- uri string? - The URI for this resource, relative to
https://api.twilio.com
twilio: Token
Fields
- ice_servers Token_ice_servers[]? - An array representing the ephemeral credentials and the STUN and TURN server URIs.
- password string? - The temporary password that the username will use when authenticating with Twilio.
- ttl string? - The duration in seconds for which the username and password are valid.
- username string? - The temporary username that uniquely identifies a Token.
twilio: Token_ice_servers
Fields
- credential string? -
- username string? -
- url string? -
- urls string? -
twilio: Transcription
Fields
- api_version string? - The API version used to create the transcription.
- duration string? - The duration of the transcribed audio in seconds.
- price decimal? - The charge for the transcript in the currency associated with the account. This value is populated after the transcript is complete so it may not be available immediately.
- sid string? - The unique string that that we created to identify the Transcription resource.
- status Transcription_enum_status? -
- transcription_text string? - The text content of the transcription.
- 'type string? - The transcription type. Can only be:
fast
.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
twilio: UpdateAccountRequest
Fields
- FriendlyName string? - Update the human-readable description of this Account
- Status Account_enum_status? -
twilio: UpdateAddressRequest
Fields
- FriendlyName string? - A descriptive string that you create to describe the address. It can be up to 64 characters long.
- CustomerName string? - The name to associate with the address.
- Street string? - The number and street address of the address.
- City string? - The city of the address.
- Region string? - The state or region of the address.
- PostalCode string? - The postal code of the address.
- EmergencyEnabled boolean? - Whether to enable emergency calling on the address. Can be:
true
orfalse
.
- AutoCorrectAddress boolean? - Whether we should automatically correct the address. Can be:
true
orfalse
and the default istrue
. If empty ortrue
, we will correct the address you provide if necessary. Iffalse
, we won't alter the address you provide.
- StreetSecondary string? - The additional number and street address of the address.
twilio: UpdateApplicationRequest
Fields
- FriendlyName string? - A descriptive string that you create to describe the resource. It can be up to 64 characters long.
- ApiVersion string? - The API version to use to start a new TwiML session. Can be:
2010-04-01
or2008-08-01
. The default value is your account's default API version.
- VoiceUrl string? - The URL we should call when the phone number assigned to this application receives a call.
- VoiceMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
voice_url
. Can be:GET
orPOST
.
- VoiceFallbackUrl string? - The URL that we should call when an error occurs retrieving or executing the TwiML requested by
url
.
- VoiceFallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
voice_fallback_url
. Can be:GET
orPOST
.
- StatusCallback string? - The URL we should call using the
status_callback_method
to send status information to your application.
- StatusCallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
status_callback
. Can be:GET
orPOST
.
- VoiceCallerIdLookup boolean? - Whether we should look up the caller's caller-ID name from the CNAM database (additional charges apply). Can be:
true
orfalse
.
- SmsUrl string? - The URL we should call when the phone number receives an incoming SMS message.
- SmsMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
sms_url
. Can be:GET
orPOST
.
- SmsFallbackUrl string? - The URL that we should call when an error occurs while retrieving or executing the TwiML from
sms_url
.
- SmsFallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
sms_fallback_url
. Can be:GET
orPOST
.
- SmsStatusCallback string? - Same as message_status_callback: The URL we should call using a POST method to send status information about SMS messages sent by the application. Deprecated, included for backwards compatibility.
- MessageStatusCallback string? - The URL we should call using a POST method to send message status information to your application.
- PublicApplicationConnectEnabled boolean? - Whether to allow other Twilio accounts to dial this applicaton using Dial verb. Can be:
true
orfalse
.
twilio: UpdateCallFeedbackRequest
Fields
- QualityScore int? - The call quality expressed as an integer from
1
to5
where1
represents very poor call quality and5
represents a perfect call.
- Issue Call_feedback_enum_issues[]? - One or more issues experienced during the call. The issues can be:
imperfect-audio
,dropped-call
,incorrect-caller-id
,post-dial-delay
,digits-not-captured
,audio-latency
,unsolicited-call
, orone-way-audio
.
twilio: UpdateCallRecordingRequest
Fields
- Status Call_recording_enum_status -
- PauseBehavior string? - Whether to record during a pause. Can be:
skip
orsilence
and the default issilence
.skip
does not record during the pause period, whilesilence
will replace the actual audio of the call with silence during the pause period. This parameter only applies when settingstatus
is set topaused
.
twilio: UpdateCallRequest
Fields
- Url string? - The absolute URL that returns the TwiML instructions for the call. We will call this URL using the
method
when the call connects. For more information, see the Url Parameter section in Making Calls.
- Method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use when calling the
url
. Can be:GET
orPOST
and the default isPOST
. If anapplication_sid
parameter is present, this parameter is ignored.
- Status Call_enum_update_status? -
- FallbackUrl string? - The URL that we call using the
fallback_method
if an error occurs when requesting or executing the TwiML aturl
. If anapplication_sid
parameter is present, this parameter is ignored.
- FallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method that we should use to request the
fallback_url
. Can be:GET
orPOST
and the default isPOST
. If anapplication_sid
parameter is present, this parameter is ignored.
- StatusCallback string? - The URL we should call using the
status_callback_method
to send status information to your application. If nostatus_callback_event
is specified, we will send thecompleted
status. If anapplication_sid
parameter is present, this parameter is ignored. URLs must contain a valid hostname (underscores are not permitted).
- StatusCallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use when requesting the
status_callback
URL. Can be:GET
orPOST
and the default isPOST
. If anapplication_sid
parameter is present, this parameter is ignored.
- Twiml string? - TwiML instructions for the call Twilio will use without fetching Twiml from url. Twiml and url parameters are mutually exclusive
- TimeLimit int? - The maximum duration of the call in seconds. Constraints depend on account and configuration.
twilio: UpdateConferenceRecordingRequest
Fields
- Status Conference_recording_enum_status -
- PauseBehavior string? - Whether to record during a pause. Can be:
skip
orsilence
and the default issilence
.skip
does not record during the pause period, whilesilence
will replace the actual audio of the call with silence during the pause period. This parameter only applies when settingstatus
is set topaused
.
twilio: UpdateConferenceRequest
Fields
- Status Conference_enum_update_status? -
- AnnounceUrl string? - The URL we should call to announce something into the conference. The URL may return an MP3 file, a WAV file, or a TwiML document that contains
<Play>
,<Say>
,<Pause>
, or<Redirect>
verbs.
- AnnounceMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method used to call
announce_url
. Can be:GET
orPOST
and the default isPOST
twilio: UpdateConnectAppRequest
Fields
- AuthorizeRedirectUrl string? - The URL to redirect the user to after we authenticate the user and obtain authorization to access the Connect App.
- CompanyName string? - The company name to set for the Connect App.
- DeauthorizeCallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method to use when calling
deauthorize_callback_url
.
- DeauthorizeCallbackUrl string? - The URL to call using the
deauthorize_callback_method
to de-authorize the Connect App.
- Description string? - A description of the Connect App.
- FriendlyName string? - A descriptive string that you create to describe the resource. It can be up to 64 characters long.
- HomepageUrl string? - A public URL where users can obtain more information about this Connect App.
- Permissions Connect_app_enum_permission[]? - A comma-separated list of the permissions you will request from the users of this ConnectApp. Can include:
get-all
andpost-all
.
twilio: UpdateIncomingPhoneNumberRequest
Fields
- AccountSid string? - The SID of the Account that created the IncomingPhoneNumber resource to update. For more information, see Exchanging Numbers Between Subaccounts.
- ApiVersion string? - The API version to use for incoming calls made to the phone number. The default is
2010-04-01
.
- FriendlyName string? - A descriptive string that you created to describe this phone number. It can be up to 64 characters long. By default, this is a formatted version of the phone number.
- SmsApplicationSid string? - The SID of the application that should handle SMS messages sent to the number. If an
sms_application_sid
is present, we ignore all of thesms_*_url
urls and use those set on the application.
- SmsFallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method that we should use to call
sms_fallback_url
. Can be:GET
orPOST
and defaults toPOST
.
- SmsFallbackUrl string? - The URL that we should call when an error occurs while requesting or executing the TwiML defined by
sms_url
.
- SmsMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method that we should use to call
sms_url
. Can be:GET
orPOST
and defaults toPOST
.
- SmsUrl string? - The URL we should call when the phone number receives an incoming SMS message.
- StatusCallback string? - The URL we should call using the
status_callback_method
to send status information to your application.
- StatusCallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
status_callback
. Can be:GET
orPOST
and defaults toPOST
.
- VoiceApplicationSid string? - The SID of the application we should use to handle phone calls to the phone number. If a
voice_application_sid
is present, we ignore all of the voice urls and use only those set on the application. Setting avoice_application_sid
will automatically delete yourtrunk_sid
and vice versa.
- VoiceCallerIdLookup boolean? - Whether to lookup the caller's name from the CNAM database and post it to your app. Can be:
true
orfalse
and defaults tofalse
.
- VoiceFallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method that we should use to call
voice_fallback_url
. Can be:GET
orPOST
and defaults toPOST
.
- VoiceFallbackUrl string? - The URL that we should call when an error occurs retrieving or executing the TwiML requested by
url
.
- VoiceMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method that we should use to call
voice_url
. Can be:GET
orPOST
and defaults toPOST
.
- VoiceUrl string? - The URL that we should call to answer a call to the phone number. The
voice_url
will not be called if avoice_application_sid
or atrunk_sid
is set.
- EmergencyStatus Incoming_phone_number_enum_emergency_status? -
- EmergencyAddressSid string? - The SID of the emergency address configuration to use for emergency calling from this phone number.
- TrunkSid string? - The SID of the Trunk we should use to handle phone calls to the phone number. If a
trunk_sid
is present, we ignore all of the voice urls and voice applications and use only those set on the Trunk. Setting atrunk_sid
will automatically delete yourvoice_application_sid
and vice versa.
- VoiceReceiveMode Incoming_phone_number_enum_voice_receive_mode? -
- IdentitySid string? - The SID of the Identity resource that we should associate with the phone number. Some regions require an identity to meet local regulations.
- AddressSid string? - The SID of the Address resource we should associate with the phone number. Some regions require addresses to meet local regulations.
- BundleSid string? - The SID of the Bundle resource that you associate with the phone number. Some regions require a Bundle to meet local Regulations.
twilio: UpdateKeyRequest
Fields
- FriendlyName string? - A descriptive string that you create to describe the resource. It can be up to 64 characters long.
twilio: UpdateMemberRequest
Fields
- Url string - The absolute URL of the Queue resource.
- Method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - How to pass the update request data. Can be
GET
orPOST
and the default isPOST
.POST
sends the data as encoded form data andGET
sends the data as query parameters.
twilio: UpdateMessageRequest
Fields
- Body string? - The new
body
of the Message resource. To redact the text content of a Message, this parameter's value must be an empty string
- Status Message_enum_update_status? -
twilio: UpdateOutgoingCallerIdRequest
Fields
- FriendlyName string? - A descriptive string that you create to describe the resource. It can be up to 64 characters long.
twilio: UpdateParticipantRequest
Fields
- Muted boolean? - Whether the participant should be muted. Can be
true
orfalse
.true
will mute the participant, andfalse
will un-mute them. Anything value other thantrue
orfalse
is interpreted asfalse
.
- Hold boolean? - Whether the participant should be on hold. Can be:
true
orfalse
.true
puts the participant on hold, andfalse
lets them rejoin the conference.
- HoldUrl string? - The URL we call using the
hold_method
for music that plays when the participant is on hold. The URL may return an MP3 file, a WAV file, or a TwiML document that contains<Play>
,<Say>
,<Pause>
, or<Redirect>
verbs.
- HoldMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
hold_url
. Can be:GET
orPOST
and the default isGET
.
- AnnounceUrl string? - The URL we call using the
announce_method
for an announcement to the participant. The URL may return an MP3 file, a WAV file, or a TwiML document that contains<Play>
,<Say>
,<Pause>
, or<Redirect>
verbs.
- AnnounceMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
announce_url
. Can be:GET
orPOST
and defaults toPOST
.
- WaitUrl string? - The URL we call using the
wait_method
for the music to play while participants are waiting for the conference to start. The URL may return an MP3 file, a WAV file, or a TwiML document that contains<Play>
,<Say>
,<Pause>
, or<Redirect>
verbs. The default value is the URL of our standard hold music. Learn more about hold music.
- WaitMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
wait_url
. Can beGET
orPOST
and the default isPOST
. When using a static audio file, this should beGET
so that we can cache the file.
- BeepOnExit boolean? - Whether to play a notification beep to the conference when the participant exits. Can be:
true
orfalse
.
- EndConferenceOnExit boolean? - Whether to end the conference when the participant leaves. Can be:
true
orfalse
and defaults tofalse
.
- Coaching boolean? - Whether the participant is coaching another call. Can be:
true
orfalse
. If not present, defaults tofalse
unlesscall_sid_to_coach
is defined. Iftrue
,call_sid_to_coach
must be defined.
- CallSidToCoach string? - The SID of the participant who is being
coached
. The participant being coached is the only participant who can hear the participant who iscoaching
.
twilio: UpdatePaymentsRequest
Fields
- IdempotencyKey string - A unique token that will be used to ensure that multiple API calls with the same information do not result in multiple transactions. This should be a unique string value per API call and can be a randomly generated.
- StatusCallback string - Provide an absolute or relative URL to receive status updates regarding your Pay session. Read more about the Update and Complete/Cancel POST requests.
- Capture Payments_enum_capture? -
- Status Payments_enum_status? -
twilio: UpdateQueueRequest
Fields
- FriendlyName string? - A descriptive string that you created to describe this resource. It can be up to 64 characters long.
- MaxSize int? - The maximum number of calls allowed to be in the queue. The default is 1000. The maximum is 5000.
twilio: UpdateShortCodeRequest
Fields
- FriendlyName string? - A descriptive string that you created to describe this resource. It can be up to 64 characters long. By default, the
FriendlyName
is the short code.
- ApiVersion string? - The API version to use to start a new TwiML session. Can be:
2010-04-01
or2008-08-01
.
- SmsUrl string? - The URL we should call when receiving an incoming SMS message to this short code.
- SmsMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use when calling the
sms_url
. Can be:GET
orPOST
.
- SmsFallbackUrl string? - The URL that we should call if an error occurs while retrieving or executing the TwiML from
sms_url
.
- SmsFallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method that we should use to call the
sms_fallback_url
. Can be:GET
orPOST
.
twilio: UpdateSigningKeyRequest
Fields
- FriendlyName string? - A descriptive string that you created to describe this resource. It can be up to 64 characters long. By default, the
FriendlyName
is the same as theSid
.
twilio: UpdateSipCredentialListRequest
Fields
- FriendlyName string - A human readable descriptive text for a CredentialList, up to 64 characters long.
twilio: UpdateSipCredentialRequest
Fields
- Password string? - The password that the username will use when authenticating SIP requests. The password must be a minimum of 12 characters, contain at least 1 digit, and have mixed case. (eg
IWasAtSignal2018
)
twilio: UpdateSipDomainRequest
Fields
- FriendlyName string? - A descriptive string that you created to describe the resource. It can be up to 64 characters long.
- VoiceFallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
voice_fallback_url
. Can be:GET
orPOST
.
- VoiceFallbackUrl string? - The URL that we should call when an error occurs while retrieving or executing the TwiML requested by
voice_url
.
- VoiceMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
voice_url
- VoiceStatusCallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
voice_status_callback_url
. Can be:GET
orPOST
.
- VoiceStatusCallbackUrl string? - The URL that we should call to pass status parameters (such as call ended) to your application.
- VoiceUrl string? - The URL we should call when the domain receives a call.
- SipRegistration boolean? - Whether to allow SIP Endpoints to register with the domain to receive calls. Can be
true
orfalse
.true
allows SIP Endpoints to register with the domain to receive calls,false
does not.
- DomainName string? - The unique address you reserve on Twilio to which you route your SIP traffic. Domain names can contain letters, digits, and "-" and must end with
sip.twilio.com
.
- EmergencyCallingEnabled boolean? - Whether emergency calling is enabled for the domain. If enabled, allows emergency calls on the domain from phone numbers with validated addresses.
- Secure boolean? - Whether secure SIP is enabled for the domain. If enabled, TLS will be enforced and SRTP will be negotiated on all incoming calls to this sip domain.
- ByocTrunkSid string? - The SID of the BYOC Trunk(Bring Your Own Carrier) resource that the Sip Domain will be associated with.
- EmergencyCallerSid string? - Whether an emergency caller sid is configured for the domain. If present, this phone number will be used as the callback for the emergency call.
twilio: UpdateSipIpAccessControlListRequest
Fields
- FriendlyName string - A human readable descriptive text, up to 255 characters long.
twilio: UpdateSipIpAddressRequest
Fields
- IpAddress string? - An IP address in dotted decimal notation from which you want to accept traffic. Any SIP requests from this IP address will be allowed by Twilio. IPv4 only supported today.
- FriendlyName string? - A human readable descriptive text for this resource, up to 255 characters long.
- CidrPrefixLength int? - An integer representing the length of the CIDR prefix to use with this IP address when accepting traffic. By default the entire IP address is used.
twilio: UpdateSiprecRequest
Fields
- Status Siprec_enum_update_status -
twilio: UpdateStreamRequest
Fields
- Status Stream_enum_update_status -
twilio: UpdateUsageTriggerRequest
Fields
- CallbackMethod "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we should use to call
callback_url
. Can be:GET
orPOST
and the default isPOST
.
- CallbackUrl string? - The URL we should call using
callback_method
when the trigger fires.
- FriendlyName string? - A descriptive string that you create to describe the resource. It can be up to 64 characters long.
twilio: Usage
twilio: UsageUsage_record
Fields
- api_version string? - The API version used to create the resource.
- as_of string? - Usage records up to date as of this timestamp, formatted as YYYY-MM-DDTHH:MM:SS+00:00. All timestamps are in GMT
- category Usage_record_enum_category? -
- count string? - The number of usage events, such as the number of calls.
- count_unit string? - The units in which
count
is measured, such ascalls
for calls ormessages
for SMS.
- description string? - A plain-language description of the usage category.
- end_date string? - The last date for which usage is included in the UsageRecord. The date is specified in GMT and formatted as
YYYY-MM-DD
.
- price decimal? - The total price of the usage in the currency specified in
price_unit
and associated with the account.
- start_date string? - The first date for which usage is included in this UsageRecord. The date is specified in GMT and formatted as
YYYY-MM-DD
.
- subresource_uris record {}? - A list of related resources identified by their URIs. For more information, see List Subresources.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
- usage string? - The amount used to bill usage and measured in units described in
usage_unit
.
- usage_unit string? - The units in which
usage
is measured, such asminutes
for calls ormessages
for SMS.
twilio: UsageUsage_recordUsage_record_all_time
Fields
- api_version string? - The API version used to create the resource.
- as_of string? - Usage records up to date as of this timestamp, formatted as YYYY-MM-DDTHH:MM:SS+00:00. All timestamps are in GMT
- category Usage_record_all_time_enum_category? -
- count string? - The number of usage events, such as the number of calls.
- count_unit string? - The units in which
count
is measured, such ascalls
for calls ormessages
for SMS.
- description string? - A plain-language description of the usage category.
- end_date string? - The last date for which usage is included in the UsageRecord. The date is specified in GMT and formatted as
YYYY-MM-DD
.
- price decimal? - The total price of the usage in the currency specified in
price_unit
and associated with the account.
- start_date string? - The first date for which usage is included in this UsageRecord. The date is specified in GMT and formatted as
YYYY-MM-DD
.
- subresource_uris record {}? - A list of related resources identified by their URIs. For more information, see List Subresources.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
- usage string? - The amount used to bill usage and measured in units described in
usage_unit
.
- usage_unit string? - The units in which
usage
is measured, such asminutes
for calls ormessages
for SMS.
twilio: UsageUsage_recordUsage_record_daily
Fields
- api_version string? - The API version used to create the resource.
- as_of string? - Usage records up to date as of this timestamp, formatted as YYYY-MM-DDTHH:MM:SS+00:00. All timestamps are in GMT
- category Usage_record_daily_enum_category? -
- count string? - The number of usage events, such as the number of calls.
- count_unit string? - The units in which
count
is measured, such ascalls
for calls ormessages
for SMS.
- description string? - A plain-language description of the usage category.
- end_date string? - The last date for which usage is included in the UsageRecord. The date is specified in GMT and formatted as
YYYY-MM-DD
.
- price decimal? - The total price of the usage in the currency specified in
price_unit
and associated with the account.
- start_date string? - The first date for which usage is included in this UsageRecord. The date is specified in GMT and formatted as
YYYY-MM-DD
.
- subresource_uris record {}? - A list of related resources identified by their URIs. For more information, see List Subresources.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
- usage string? - The amount used to bill usage and measured in units described in
usage_unit
.
- usage_unit string? - The units in which
usage
is measured, such asminutes
for calls ormessages
for SMS.
twilio: UsageUsage_recordUsage_record_last_month
Fields
- api_version string? - The API version used to create the resource.
- as_of string? - Usage records up to date as of this timestamp, formatted as YYYY-MM-DDTHH:MM:SS+00:00. All timestamps are in GMT
- category Usage_record_last_month_enum_category? -
- count string? - The number of usage events, such as the number of calls.
- count_unit string? - The units in which
count
is measured, such ascalls
for calls ormessages
for SMS.
- description string? - A plain-language description of the usage category.
- end_date string? - The last date for which usage is included in the UsageRecord. The date is specified in GMT and formatted as
YYYY-MM-DD
.
- price decimal? - The total price of the usage in the currency specified in
price_unit
and associated with the account.
- start_date string? - The first date for which usage is included in this UsageRecord. The date is specified in GMT and formatted as
YYYY-MM-DD
.
- subresource_uris record {}? - A list of related resources identified by their URIs. For more information, see List Subresources.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
- usage string? - The amount used to bill usage and measured in units described in
usage_unit
.
- usage_unit string? - The units in which
usage
is measured, such asminutes
for calls ormessages
for SMS.
twilio: UsageUsage_recordUsage_record_monthly
Fields
- api_version string? - The API version used to create the resource.
- as_of string? - Usage records up to date as of this timestamp, formatted as YYYY-MM-DDTHH:MM:SS+00:00. All timestamps are in GMT
- category Usage_record_monthly_enum_category? -
- count string? - The number of usage events, such as the number of calls.
- count_unit string? - The units in which
count
is measured, such ascalls
for calls ormessages
for SMS.
- description string? - A plain-language description of the usage category.
- end_date string? - The last date for which usage is included in the UsageRecord. The date is specified in GMT and formatted as
YYYY-MM-DD
.
- price decimal? - The total price of the usage in the currency specified in
price_unit
and associated with the account.
- start_date string? - The first date for which usage is included in this UsageRecord. The date is specified in GMT and formatted as
YYYY-MM-DD
.
- subresource_uris record {}? - A list of related resources identified by their URIs. For more information, see List Subresources.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
- usage string? - The amount used to bill usage and measured in units described in
usage_unit
.
- usage_unit string? - The units in which
usage
is measured, such asminutes
for calls ormessages
for SMS.
twilio: UsageUsage_recordUsage_record_this_month
Fields
- api_version string? - The API version used to create the resource.
- as_of string? - Usage records up to date as of this timestamp, formatted as YYYY-MM-DDTHH:MM:SS+00:00. All timestamps are in GMT
- category Usage_record_this_month_enum_category? -
- count string? - The number of usage events, such as the number of calls.
- count_unit string? - The units in which
count
is measured, such ascalls
for calls ormessages
for SMS.
- description string? - A plain-language description of the usage category.
- end_date string? - The last date for which usage is included in the UsageRecord. The date is specified in GMT and formatted as
YYYY-MM-DD
.
- price decimal? - The total price of the usage in the currency specified in
price_unit
and associated with the account.
- start_date string? - The first date for which usage is included in this UsageRecord. The date is specified in GMT and formatted as
YYYY-MM-DD
.
- subresource_uris record {}? - A list of related resources identified by their URIs. For more information, see List Subresources.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
- usage string? - The amount used to bill usage and measured in units described in
usage_unit
.
- usage_unit string? - The units in which
usage
is measured, such asminutes
for calls ormessages
for SMS.
twilio: UsageUsage_recordUsage_record_today
Fields
- api_version string? - The API version used to create the resource.
- as_of string? - Usage records up to date as of this timestamp, formatted as YYYY-MM-DDTHH:MM:SS+00:00. All timestamps are in GMT
- category Usage_record_today_enum_category? -
- count string? - The number of usage events, such as the number of calls.
- count_unit string? - The units in which
count
is measured, such ascalls
for calls ormessages
for SMS.
- description string? - A plain-language description of the usage category.
- end_date string? - The last date for which usage is included in the UsageRecord. The date is specified in GMT and formatted as
YYYY-MM-DD
.
- price decimal? - The total price of the usage in the currency specified in
price_unit
and associated with the account.
- start_date string? - The first date for which usage is included in this UsageRecord. The date is specified in GMT and formatted as
YYYY-MM-DD
.
- subresource_uris record {}? - A list of related resources identified by their URIs. For more information, see List Subresources.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
- usage string? - The amount used to bill usage and measured in units described in
usage_unit
.
- usage_unit string? - The units in which
usage
is measured, such asminutes
for calls ormessages
for SMS.
twilio: UsageUsage_recordUsage_record_yearly
Fields
- api_version string? - The API version used to create the resource.
- as_of string? - Usage records up to date as of this timestamp, formatted as YYYY-MM-DDTHH:MM:SS+00:00. All timestamps are in GMT
- category Usage_record_yearly_enum_category? -
- count string? - The number of usage events, such as the number of calls.
- count_unit string? - The units in which
count
is measured, such ascalls
for calls ormessages
for SMS.
- description string? - A plain-language description of the usage category.
- end_date string? - The last date for which usage is included in the UsageRecord. The date is specified in GMT and formatted as
YYYY-MM-DD
.
- price decimal? - The total price of the usage in the currency specified in
price_unit
and associated with the account.
- start_date string? - The first date for which usage is included in this UsageRecord. The date is specified in GMT and formatted as
YYYY-MM-DD
.
- subresource_uris record {}? - A list of related resources identified by their URIs. For more information, see List Subresources.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
- usage string? - The amount used to bill usage and measured in units described in
usage_unit
.
- usage_unit string? - The units in which
usage
is measured, such asminutes
for calls ormessages
for SMS.
twilio: UsageUsage_recordUsage_record_yesterday
Fields
- api_version string? - The API version used to create the resource.
- as_of string? - Usage records up to date as of this timestamp, formatted as YYYY-MM-DDTHH:MM:SS+00:00. All timestamps are in GMT
- category Usage_record_yesterday_enum_category? -
- count string? - The number of usage events, such as the number of calls.
- count_unit string? - The units in which
count
is measured, such ascalls
for calls ormessages
for SMS.
- description string? - A plain-language description of the usage category.
- end_date string? - The last date for which usage is included in the UsageRecord. The date is specified in GMT and formatted as
YYYY-MM-DD
.
- price decimal? - The total price of the usage in the currency specified in
price_unit
and associated with the account.
- start_date string? - The first date for which usage is included in this UsageRecord. The date is specified in GMT and formatted as
YYYY-MM-DD
.
- subresource_uris record {}? - A list of related resources identified by their URIs. For more information, see List Subresources.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
- usage string? - The amount used to bill usage and measured in units described in
usage_unit
.
- usage_unit string? - The units in which
usage
is measured, such asminutes
for calls ormessages
for SMS.
twilio: UsageUsage_trigger
Fields
- api_version string? - The API version used to create the resource.
- callback_method "HEAD"|"GET"|"POST"|"PATCH"|"PUT"|"DELETE" ? - The HTTP method we use to call
callback_url
. Can be:GET
orPOST
.
- callback_url string? - The URL we call using the
callback_method
when the trigger fires.
- current_value string? - The current value of the field the trigger is watching.
- friendly_name string? - The string that you assigned to describe the trigger.
- recurring Usage_trigger_enum_recurring? -
- sid string? - The unique string that that we created to identify the UsageTrigger resource.
- trigger_by Usage_trigger_enum_trigger_field? -
- trigger_value string? - The value at which the trigger will fire. Must be a positive, numeric value.
- uri string? - The URI of the resource, relative to
https://api.twilio.com
.
- usage_category Usage_trigger_enum_usage_category? -
- usage_record_uri string? - The URI of the UsageRecord resource this trigger watches, relative to
https://api.twilio.com
.
twilio: Validation_request
Fields
- friendly_name string? - The string that you assigned to describe the resource.
- validation_code string? - The 6 digit validation code that someone must enter to validate the Caller ID when
phone_number
is called.
Union types
twilio: Conference_recording_enum_source
Conference_recording_enum_source
twilio: Usage_record_monthly_enum_category
Usage_record_monthly_enum_category
twilio: Payments_enum_capture
Payments_enum_capture
twilio: Account_enum_status
Account_enum_status
twilio: Payments_enum_payment_method
Payments_enum_payment_method
twilio: Incoming_phone_number_local_enum_emergency_status
Incoming_phone_number_local_enum_emergency_status
twilio: Call_recording_enum_source
Call_recording_enum_source
twilio: Conference_enum_status
Conference_enum_status
twilio: Recording_add_on_result_enum_status
Recording_add_on_result_enum_status
twilio: Payments_enum_status
Payments_enum_status
twilio: Incoming_phone_number_mobile_enum_emergency_status
Incoming_phone_number_mobile_enum_emergency_status
twilio: Transcription_enum_status
Transcription_enum_status
twilio: Incoming_phone_number_local_enum_address_requirement
Incoming_phone_number_local_enum_address_requirement
twilio: Call_feedback_enum_issues
Call_feedback_enum_issues
twilio: Stream_enum_status
Stream_enum_status
twilio: Message_enum_address_retention
Message_enum_address_retention
twilio: Usage_record_enum_category
Usage_record_enum_category
twilio: Connect_app_enum_permission
Connect_app_enum_permission
twilio: Usage_record_all_time_enum_category
Usage_record_all_time_enum_category
twilio: Usage_record_yesterday_enum_category
Usage_record_yesterday_enum_category
twilio: Message_enum_risk_check
Message_enum_risk_check
twilio: Call_enum_update_status
Call_enum_update_status
twilio: Call_feedback_summary_enum_status
Call_feedback_summary_enum_status
twilio: Recording_transcription_enum_status
Recording_transcription_enum_status
twilio: Incoming_phone_number_toll_free_enum_emergency_status
Incoming_phone_number_toll_free_enum_emergency_status
twilio: Usage_record_today_enum_category
Usage_record_today_enum_category
twilio: Conference_enum_reason_conference_ended
Conference_enum_reason_conference_ended
twilio: Incoming_phone_number_mobile_enum_address_requirement
Incoming_phone_number_mobile_enum_address_requirement
twilio: Message_feedback_enum_outcome
Message_feedback_enum_outcome
twilio: Call_enum_event
Call_enum_event
twilio: Incoming_phone_number_mobile_enum_voice_receive_mode
Incoming_phone_number_mobile_enum_voice_receive_mode
twilio: Usage_trigger_enum_usage_category
Usage_trigger_enum_usage_category
twilio: Stream_enum_track
Stream_enum_track
twilio: Usage_record_this_month_enum_category
Usage_record_this_month_enum_category
twilio: Siprec_enum_status
Siprec_enum_status
twilio: Usage_trigger_enum_trigger_field
Usage_trigger_enum_trigger_field
twilio: Incoming_phone_number_toll_free_enum_emergency_address_status
Incoming_phone_number_toll_free_enum_emergency_address_status
twilio: Account_enum_type
Account_enum_type
twilio: Dependent_phone_number_enum_address_requirement
Dependent_phone_number_enum_address_requirement
twilio: Siprec_enum_track
Siprec_enum_track
twilio: Incoming_phone_number_enum_emergency_address_status
Incoming_phone_number_enum_emergency_address_status
twilio: Incoming_phone_number_mobile_enum_emergency_address_status
Incoming_phone_number_mobile_enum_emergency_address_status
twilio: Usage_record_daily_enum_category
Usage_record_daily_enum_category
twilio: Usage_record_yearly_enum_category
Usage_record_yearly_enum_category
twilio: Recording_enum_status
Recording_enum_status
twilio: Incoming_phone_number_enum_voice_receive_mode
Incoming_phone_number_enum_voice_receive_mode
twilio: Payments_enum_token_type
Payments_enum_token_type
twilio: Dependent_phone_number_enum_emergency_status
Dependent_phone_number_enum_emergency_status
twilio: Incoming_phone_number_local_enum_voice_receive_mode
Incoming_phone_number_local_enum_voice_receive_mode
twilio: Message_enum_status
Message_enum_status
twilio: Usage_trigger_enum_recurring
Usage_trigger_enum_recurring
twilio: Participant_enum_status
Participant_enum_status
twilio: Conference_recording_enum_status
Conference_recording_enum_status
twilio: Call_recording_enum_status
Call_recording_enum_status
twilio: Incoming_phone_number_local_enum_emergency_address_status
Incoming_phone_number_local_enum_emergency_address_status
twilio: Incoming_phone_number_enum_address_requirement
Incoming_phone_number_enum_address_requirement
twilio: Incoming_phone_number_toll_free_enum_voice_receive_mode
Incoming_phone_number_toll_free_enum_voice_receive_mode
twilio: Incoming_phone_number_toll_free_enum_address_requirement
Incoming_phone_number_toll_free_enum_address_requirement
twilio: Message_enum_direction
Message_enum_direction
twilio: Incoming_phone_number_enum_emergency_status
Incoming_phone_number_enum_emergency_status
twilio: Payments_enum_bank_account_type
Payments_enum_bank_account_type
twilio: Recording_enum_source
Recording_enum_source
twilio: Message_enum_content_retention
Message_enum_content_retention
twilio: Authorized_connect_app_enum_permission
Authorized_connect_app_enum_permission
twilio: Call_enum_status
Call_enum_status
twilio: Usage_record_last_month_enum_category
Usage_record_last_month_enum_category
Import
import ballerinax/twilio;
Metadata
Released date: over 1 year ago
Version: 4.0.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.8.4
GraalVM compatible: Yes
Pull count
Total: 2258887
Current verison: 130
Weekly downloads
Keywords
Communication/Call & SMS
Cost/Paid
Contributors