trello
Module trello
API
Definitions
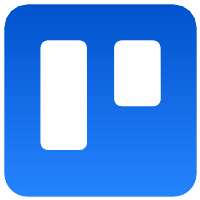
ballerinax/trello Ballerina library
Overview
This is a generated connector for Trello API v1 OpenAPI specification.
Client endpoint of Trello API provides capability to perform CRUD (Create, Read, Update, and Delete) operations on resources in a Trello account.
Prerequisites
- Create a Trello account
- Obtain tokens
- Use this guide to obtain the API key and generate a token related to your account.
Quickstart
To use the Trello connector in your Ballerina application, update the .bal file as follows:
Step 1 - Import connector
First, import the ballerinax/trello module into the Ballerina project.
import ballerinax/trello;
Step 2 - Create a new connector instance
You can now make the connection configuration using the API key and token.
trello:ApiKeysConfig configuration = { key: <TRELLO_API_KEY>, token: <TRELLO_API_TOKEN> }; trello:Client trelloClient = check new Client(configuration);
Step 3 - Invoke connector operation
- Add a new Board
public function main() { trello:Boards board = { idBoardSource: "<THE_ID_OF_PARENT_BOARD>" }; http:Response|error boardInfo = trelloClient->addBoards(board); }
- Get a Borad
public function main() { trello:Boards board = { idBoardSource: "<THE_ID_OF_PARENT_BOARD>" }; http:Response|error boardInfo = trelloClient->getBoardsByIdBoard(boardId); if (boardInfo is http:Response) { json|error info = boardInfo.getJsonPayload(); if (info is json) { log:printInfo("Board Info " + info.toString()); } } }
- Use
bal run
command to compile and run the Ballerina program
Clients
trello: Client
This is a generated connector for Trello API v1 OpenAPI specification. Client endpoint of Trello API provides capability to perform CRUD (Create, Read, Update, and Delete) operations on resources in a Trello account.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Trello account and obtain tokens following this guide.
init (ApiKeysConfig apiKeyConfig, ConnectionConfig config, string serviceUrl)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://trello.com/1" - URL of the target service
getBatch
Get batch
Parameters
- urls string - List of API v1 GET routes, not including the version prefix
addBoards
Add new board
Parameters
- payload Boards - Attributes of "Boards" to be added.
getBoardsByIdBoard
function getBoardsByIdBoard(string idBoard, string? actions, string? actionsEntities, string? actionsDisplay, string actionsFormat, string? actionsSince, string actionsLimit, string actionFields, string? actionMember, string actionMemberFields, string? actionMembercreator, string actionMembercreatorFields, string cards, string cardFields, string? cardAttachments, string cardAttachmentFields, string cardChecklists, string? cardStickers, string boardStars, string labels, string labelFields, string labelsLimit, string lists, string listFields, string memberships, string? membershipsMember, string membershipsMemberFields, string members, string memberFields, string membersInvited, string membersinvitedFields, string checklists, string checklistFields, string? organization, string organizationFields, string organizationMemberships, string? myPrefs, string fields) returns Response|error
Get board by ID
Parameters
- idBoard string - Board_id
- actions string? (default ()) - All or a comma-separated list of: addAttachmentToCard, addChecklistToCard, addMemberToBoard, addMemberToCard, addMemberToOrganization, addToOrganizationBoard, commentCard, convertToCardFromCheckItem, copyBoard, copyCard, copyCommentCard, createBoard, createCard, createList, createOrganization, deleteAttachmentFromCard, deleteBoardInvitation, deleteCard, deleteOrganizationInvitation, disablePowerUp, emailCard, enablePowerUp, makeAdminOfBoard, makeNormalMemberOfBoard, makeNormalMemberOfOrganization, makeObserverOfBoard, memberJoinedTrello, moveCardFromBoard, moveCardToBoard, moveListFromBoard, moveListToBoard, removeChecklistFromCard, removeFromOrganizationBoard, removeMemberFromCard, unconfirmedBoardInvitation, unconfirmedOrganizationInvitation, updateBoard, updateCard, updateCard:closed, updateCard:desc, updateCard:idList, updateCard:name, updateCheckItemStateOnCard, updateChecklist, updateList, updateList:closed, updateList:name, updateMember or updateOrganization
- actionsEntities string? (default ()) - True or false
- actionsDisplay string? (default ()) - True or false
- actionsFormat string (default "list") - One of: count, list or minimal
- actionsSince string? (default ()) - A date, null or lastView
- actionsLimit string (default "50") - A number from 0 to 1000
- actionFields string (default "all") - All or a comma-separated list of: data, date, idMemberCreator or type
- actionMember string? (default ()) - True or false
- actionMemberFields string (default "avatarHash, fullName, initials and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- actionMembercreator string? (default ()) - True or false
- actionMembercreatorFields string (default "avatarHash, fullName, initials and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- cards string (default "none") - One of: all, closed, none, open or visible
- cardFields string (default "all") - All or a comma-separated list of: badges, checkItemStates, closed, dateLastActivity, desc, descData, due, email, idAttachmentCover, idBoard, idChecklists, idLabels, idList, idMembers, idMembersVoted, idShort, labels, manualCoverAttachment, name, pos, shortLink, shortUrl, subscribed or url
- cardAttachments string? (default ()) - A boolean value or "cover" for only card cover attachments
- cardAttachmentFields string (default "all") - All or a comma-separated list of: bytes, date, edgeColor, idMember, isUpload, mimeType, name, previews or url
- cardChecklists string (default "none") - One of: all or none
- cardStickers string? (default ()) - True or false
- boardStars string (default "none") - One of: mine or none
- labels string (default "none") - One of: all or none
- labelFields string (default "all") - All or a comma-separated list of: color, idBoard, name or uses
- labelsLimit string (default "50") - A number from 0 to 1000
- lists string (default "none") - One of: all, closed, none or open
- listFields string (default "all") - All or a comma-separated list of: closed, idBoard, name, pos or subscribed
- memberships string (default "none") - All or a comma-separated list of: active, admin, deactivated, me or normal
- membershipsMember string? (default ()) - True or false
- membershipsMemberFields string (default "fullName and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- members string (default "none") - One of: admins, all, none, normal or owners
- memberFields string (default "avatarHash, initials, fullName, username and confirmed") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- membersInvited string (default "none") - One of: admins, all, none, normal or owners
- membersinvitedFields string (default "avatarHash, initials, fullName and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- checklists string (default "none") - One of: all or none
- checklistFields string (default "all") - All or a comma-separated list of: idBoard, idCard, name or pos
- organization string? (default ()) - True or false
- organizationFields string (default "name and displayName") - All or a comma-separated list of: billableMemberCount, desc, descData, displayName, idBoards, invitations, invited, logoHash, memberships, name, powerUps, prefs, premiumFeatures, products, url or website
- organizationMemberships string (default "none") - All or a comma-separated list of: active, admin, deactivated, me or normal
- myPrefs string? (default ()) - True or false
- fields string (default "name, desc, descData, closed, idOrganization, pinned, url, shortUrl, prefs and labelNames") - All or a comma-separated list of: closed, dateLastActivity, dateLastView, desc, descData, idOrganization, invitations, invited, labelNames, memberships, name, pinned, powerUps, prefs, shortLink, shortUrl, starred, subscribed or url
updateBoardsByIdBoard
Update boards by ID
getBoardsActionsByIdBoard
function getBoardsActionsByIdBoard(string idBoard, string? entities, string? display, string filter, string fields, string 'limit, string format, string? since, string? before, string page, string? idModels, string? member, string memberFields, string? memberCreator, string membercreatorFields) returns Response|error
Get boards actions by ID board
Parameters
- idBoard string - Board_id
- entities string? (default ()) - True or false
- display string? (default ()) - True or false
- filter string (default "all") - All or a comma-separated list of: addAttachmentToCard, addChecklistToCard, addMemberToBoard, addMemberToCard, addMemberToOrganization, addToOrganizationBoard, commentCard, convertToCardFromCheckItem, copyBoard, copyCard, copyCommentCard, createBoard, createCard, createList, createOrganization, deleteAttachmentFromCard, deleteBoardInvitation, deleteCard, deleteOrganizationInvitation, disablePowerUp, emailCard, enablePowerUp, makeAdminOfBoard, makeNormalMemberOfBoard, makeNormalMemberOfOrganization, makeObserverOfBoard, memberJoinedTrello, moveCardFromBoard, moveCardToBoard, moveListFromBoard, moveListToBoard, removeChecklistFromCard, removeFromOrganizationBoard, removeMemberFromCard, unconfirmedBoardInvitation, unconfirmedOrganizationInvitation, updateBoard, updateCard, updateCard:closed, updateCard:desc, updateCard:idList, updateCard:name, updateCheckItemStateOnCard, updateChecklist, updateList, updateList:closed, updateList:name, updateMember or updateOrganization
- fields string (default "all") - All or a comma-separated list of: data, date, idMemberCreator or type
- 'limit string (default "50") - A number from 0 to 1000
- format string (default "list") - One of: count, list or minimal
- since string? (default ()) - A date, null or lastView
- before string? (default ()) - A date, or null
- page string (default "0") - Page * limit must be less than 1000
- idModels string? (default ()) - Only return actions related to these model ids
- member string? (default ()) - True or false
- memberFields string (default "avatarHash, fullName, initials and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- memberCreator string? (default ()) - True or false
- membercreatorFields string (default "avatarHash, fullName, initials and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
getBoardsBoardStarsByIdBoard
Get boards board stars by ID
addBoardsCalendarKeyGenerateByIdBoard
Add boards calendar key generate by ID
Parameters
- idBoard string - Board_id
getBoardsCardsByIdBoard
function getBoardsCardsByIdBoard(string idBoard, string? actions, string? attachments, string attachmentFields, string? stickers, string? members, string memberFields, string? checkItemStates, string checklists, string? 'limit, string? since, string? before, string filter, string fields) returns Response|error
Get boards cards by ID board
Parameters
- idBoard string - Board_id
- actions string? (default ()) - All or a comma-separated list of: addAttachmentToCard, addChecklistToCard, addMemberToBoard, addMemberToCard, addMemberToOrganization, addToOrganizationBoard, commentCard, convertToCardFromCheckItem, copyBoard, copyCard, copyCommentCard, createBoard, createCard, createList, createOrganization, deleteAttachmentFromCard, deleteBoardInvitation, deleteCard, deleteOrganizationInvitation, disablePowerUp, emailCard, enablePowerUp, makeAdminOfBoard, makeNormalMemberOfBoard, makeNormalMemberOfOrganization, makeObserverOfBoard, memberJoinedTrello, moveCardFromBoard, moveCardToBoard, moveListFromBoard, moveListToBoard, removeChecklistFromCard, removeFromOrganizationBoard, removeMemberFromCard, unconfirmedBoardInvitation, unconfirmedOrganizationInvitation, updateBoard, updateCard, updateCard:closed, updateCard:desc, updateCard:idList, updateCard:name, updateCheckItemStateOnCard, updateChecklist, updateList, updateList:closed, updateList:name, updateMember or updateOrganization
- attachments string? (default ()) - A boolean value or "cover" for only card cover attachments
- attachmentFields string (default "all") - All or a comma-separated list of: bytes, date, edgeColor, idMember, isUpload, mimeType, name, previews or url
- stickers string? (default ()) - True or false
- members string? (default ()) - True or false
- memberFields string (default "avatarHash, fullName, initials and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- checkItemStates string? (default ()) - True or false
- checklists string (default "none") - One of: all or none
- 'limit string? (default ()) - A number from 1 to 1000
- since string? (default ()) - A date, or null
- before string? (default ()) - A date, or null
- filter string (default "visible") - One of: all, closed, none, open or visible
- fields string (default "all") - All or a comma-separated list of: badges, checkItemStates, closed, dateLastActivity, desc, descData, due, email, idAttachmentCover, idBoard, idChecklists, idLabels, idList, idMembers, idMembersVoted, idShort, labels, manualCoverAttachment, name, pos, shortLink, shortUrl, subscribed or url
getBoardsCardsByIdBoardByFilter
Get boards cards by ID board by filter
getBoardsCardsByIdBoardByIdCard
function getBoardsCardsByIdBoardByIdCard(string idBoard, string idCard, string? attachments, string attachmentFields, string? actions, string? actionsEntities, string? actionsDisplay, string actionsLimit, string actionFields, string actionMembercreatorFields, string? members, string memberFields, string? checkItemStates, string checkitemstateFields, string? labels, string checklists, string checklistFields, string fields) returns Response|error
Get boards cards by ID board by ID card
Parameters
- idBoard string - Board_id
- idCard string - IdCard
- attachments string? (default ()) - A boolean value or "cover" for only card cover attachments
- attachmentFields string (default "all") - All or a comma-separated list of: bytes, date, edgeColor, idMember, isUpload, mimeType, name, previews or url
- actions string? (default ()) - All or a comma-separated list of: addAttachmentToCard, addChecklistToCard, addMemberToBoard, addMemberToCard, addMemberToOrganization, addToOrganizationBoard, commentCard, convertToCardFromCheckItem, copyBoard, copyCard, copyCommentCard, createBoard, createCard, createList, createOrganization, deleteAttachmentFromCard, deleteBoardInvitation, deleteCard, deleteOrganizationInvitation, disablePowerUp, emailCard, enablePowerUp, makeAdminOfBoard, makeNormalMemberOfBoard, makeNormalMemberOfOrganization, makeObserverOfBoard, memberJoinedTrello, moveCardFromBoard, moveCardToBoard, moveListFromBoard, moveListToBoard, removeChecklistFromCard, removeFromOrganizationBoard, removeMemberFromCard, unconfirmedBoardInvitation, unconfirmedOrganizationInvitation, updateBoard, updateCard, updateCard:closed, updateCard:desc, updateCard:idList, updateCard:name, updateCheckItemStateOnCard, updateChecklist, updateList, updateList:closed, updateList:name, updateMember or updateOrganization
- actionsEntities string? (default ()) - True or false
- actionsDisplay string? (default ()) - True or false
- actionsLimit string (default "50") - A number from 0 to 1000
- actionFields string (default "all") - All or a comma-separated list of: data, date, idMemberCreator or type
- actionMembercreatorFields string (default "avatarHash, fullName, initials and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- members string? (default ()) - True or false
- memberFields string (default "avatarHash, initials, fullName and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- checkItemStates string? (default ()) - True or false
- checkitemstateFields string (default "all") - All or a comma-separated list of: idCheckItem or state
- labels string? (default ()) - True or false
- checklists string (default "none") - One of: all or none
- checklistFields string (default "all") - All or a comma-separated list of: idBoard, idCard, name or pos
- fields string (default "all") - All or a comma-separated list of: badges, checkItemStates, closed, dateLastActivity, desc, descData, due, email, idAttachmentCover, idBoard, idChecklists, idLabels, idList, idMembers, idMembersVoted, idShort, labels, manualCoverAttachment, name, pos, shortLink, shortUrl, subscribed or url
getBoardsChecklistsByIdBoard
function getBoardsChecklistsByIdBoard(string idBoard, string cards, string cardFields, string checkItems, string checkitemFields, string filter, string fields) returns Response|error
Get boards checklists by ID board
Parameters
- idBoard string - Board_id
- cards string (default "none") - One of: all, closed, none, open or visible
- cardFields string (default "all") - All or a comma-separated list of: badges, checkItemStates, closed, dateLastActivity, desc, descData, due, email, idAttachmentCover, idBoard, idChecklists, idLabels, idList, idMembers, idMembersVoted, idShort, labels, manualCoverAttachment, name, pos, shortLink, shortUrl, subscribed or url
- checkItems string (default "all") - One of: all or none
- checkitemFields string (default "name, nameData, pos and state") - All or a comma-separated list of: name, nameData, pos, state or type
- filter string (default "all") - One of: all or none
- fields string (default "all") - All or a comma-separated list of: idBoard, idCard, name or pos
addBoardsChecklistsByIdBoard
function addBoardsChecklistsByIdBoard(string idBoard, BoardsChecklists payload) returns Response|error
Add boards checklists by ID board
Parameters
- idBoard string - Board_id
- payload BoardsChecklists - Attributes of "Boards Checklists" to be added.
updateBoardsClosedByIdBoard
function updateBoardsClosedByIdBoard(string idBoard, BoardsClosed payload) returns Response|error
Update boards closed by ID board
Parameters
- idBoard string - Board_id
- payload BoardsClosed - Attributes of "Boards Closed" to be updated.
getBoardsDeltasByIdBoard
function getBoardsDeltasByIdBoard(string idBoard, string tags, string ixLastUpdate) returns Response|error
Get boards deltas by ID board
Parameters
- idBoard string - Board_id
- tags string - A valid tag for subscribing
- ixLastUpdate string - A number from -1 to Infinity
updateBoardsDescByIdBoard
function updateBoardsDescByIdBoard(string idBoard, BoardsDesc payload) returns Response|error
Update boards desc by ID board
addBoardsEmailKeyGenerateByIdBoard
Add boards email key generate by ID board
Parameters
- idBoard string - Board_id
updateBoardsIdOrganizationByIdBoard
function updateBoardsIdOrganizationByIdBoard(string idBoard, BoardsIdorganization payload) returns Response|error
Update boards ID organization by ID board
Parameters
- idBoard string - Board_id
- payload BoardsIdorganization - Attributes of "Boards ID Organization" to be updated.
updateBoardsLabelNamesBlueByIdBoard
function updateBoardsLabelNamesBlueByIdBoard(string idBoard, LabelnamesBlue payload) returns Response|error
Update boards label names blue by ID board
Parameters
- idBoard string - Board_id
- payload LabelnamesBlue - Attributes of "Label Names Blue" to be updated.
updateBoardsLabelNamesGreenByIdBoard
function updateBoardsLabelNamesGreenByIdBoard(string idBoard, LabelnamesGreen payload) returns Response|error
Update boards label names green by ID board
Parameters
- idBoard string - Board_id
- payload LabelnamesGreen - Attributes of "Label Names Green" to be updated.
updateBoardsLabelNamesOrangeByIdBoard
function updateBoardsLabelNamesOrangeByIdBoard(string idBoard, LabelnamesOrange payload) returns Response|error
Update boards label names orange by ID board
Parameters
- idBoard string - Board_id
- payload LabelnamesOrange - Attributes of "Label Names Orange" to be updated.
updateBoardsLabelNamesPurpleByIdBoard
function updateBoardsLabelNamesPurpleByIdBoard(string idBoard, LabelnamesPurple payload) returns Response|error
Update boards label names purple by ID board
Parameters
- idBoard string - Board_id
- payload LabelnamesPurple - Attributes of "Label Names Purple" to be updated.
updateBoardsLabelNamesRedByIdBoard
function updateBoardsLabelNamesRedByIdBoard(string idBoard, LabelnamesRed payload) returns Response|error
Update boards label names red by ID board
Parameters
- idBoard string - Board_id
- payload LabelnamesRed - Attributes of "Label Names Red" to be updated.
updateBoardsLabelNamesYellowByIdBoard
function updateBoardsLabelNamesYellowByIdBoard(string idBoard, LabelnamesYellow payload) returns Response|error
Update boards label names yellow by ID board
Parameters
- idBoard string - Board_id
- payload LabelnamesYellow - Attributes of "Label Names Yellow" to be updated.
getBoardsLabelsByIdBoard
function getBoardsLabelsByIdBoard(string idBoard, string fields, string 'limit) returns Response|error
Get boards labels by ID board
Parameters
- idBoard string - Board_id
- fields string (default "all") - All or a comma-separated list of: color, idBoard, name or uses
- 'limit string (default "50") - A number from 0 to 1000
addBoardsLabelsByIdBoard
function addBoardsLabelsByIdBoard(string idBoard, BoardsLabels payload) returns Response|error
Add boards labels by ID board
Parameters
- idBoard string - Board_id
- payload BoardsLabels - Attributes of "Boards Labels" to be added.
getBoardsLabelsByIdBoardByIdLabel
function getBoardsLabelsByIdBoardByIdLabel(string idBoard, string idLabel, string fields) returns Response|error
Get boards labels by ID board by ID label
Parameters
- idBoard string - Board_id
- idLabel string - IdLabel
- fields string (default "all") - All or a comma-separated list of: color, idBoard, name or uses
getBoardsListsByIdBoard
function getBoardsListsByIdBoard(string idBoard, string cards, string cardFields, string filter, string fields) returns Response|error
Get boards lists by ID board
Parameters
- idBoard string - Board_id
- cards string (default "none") - One of: all, closed, none, open or visible
- cardFields string (default "all") - All or a comma-separated list of: badges, checkItemStates, closed, dateLastActivity, desc, descData, due, email, idAttachmentCover, idBoard, idChecklists, idLabels, idList, idMembers, idMembersVoted, idShort, labels, manualCoverAttachment, name, pos, shortLink, shortUrl, subscribed or url
- filter string (default "open") - One of: all, closed, none or open
- fields string (default "all") - All or a comma-separated list of: closed, idBoard, name, pos or subscribed
addBoardsListsByIdBoard
function addBoardsListsByIdBoard(string idBoard, BoardsLists payload) returns Response|error
Add boards lists by ID board
getBoardsListsByIdBoardByFilter
Get boards lists by ID board by filter
addBoardsMarkAsViewedByIdBoard
Add boards mark as viewed by ID board
Parameters
- idBoard string - Board_id
getBoardsMembersByIdBoard
function getBoardsMembersByIdBoard(string idBoard, string filter, string fields, string? activity) returns Response|error
Get boards members by ID board
Parameters
- idBoard string - Board_id
- filter string (default "all") - One of: admins, all, none, normal or owners
- fields string (default "fullName and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- activity string? (default ()) - True or false ; works for premium organizations only.
updateBoardsMembersByIdBoard
function updateBoardsMembersByIdBoard(string idBoard, BoardsMembers payload) returns Response|error
Update boards members by ID board
Parameters
- idBoard string - Board_id
- payload BoardsMembers - Attributes of "Boards Members" to be updated.
getBoardsMembersByIdBoardByFilter
Get boards members by ID board by filter
updateBoardsMembersByIdBoardByIdMember
function updateBoardsMembersByIdBoardByIdMember(string idBoard, string idMember, BoardsMembers payload) returns Response|error
Update boards members by ID board by ID member
Parameters
- idBoard string - Board_id
- idMember string - IdMember
- payload BoardsMembers - Attributes of "Boards Members" to be updated.
deleteBoardsMembersByIdBoardByIdMember
function deleteBoardsMembersByIdBoardByIdMember(string idBoard, string idMember) returns Response|error
Delete boards members by ID board by ID member
getBoardsMembersCardsByIdBoardByIdMember
function getBoardsMembersCardsByIdBoardByIdMember(string idBoard, string idMember, string? actions, string? attachments, string attachmentFields, string? members, string memberFields, string? checkItemStates, string checklists, string? board, string boardFields, string? list, string listFields, string filter, string fields) returns Response|error
Get boards members cards by ID board by ID member
Parameters
- idBoard string - Board_id
- idMember string - IdMember
- actions string? (default ()) - All or a comma-separated list of: addAttachmentToCard, addChecklistToCard, addMemberToBoard, addMemberToCard, addMemberToOrganization, addToOrganizationBoard, commentCard, convertToCardFromCheckItem, copyBoard, copyCard, copyCommentCard, createBoard, createCard, createList, createOrganization, deleteAttachmentFromCard, deleteBoardInvitation, deleteCard, deleteOrganizationInvitation, disablePowerUp, emailCard, enablePowerUp, makeAdminOfBoard, makeNormalMemberOfBoard, makeNormalMemberOfOrganization, makeObserverOfBoard, memberJoinedTrello, moveCardFromBoard, moveCardToBoard, moveListFromBoard, moveListToBoard, removeChecklistFromCard, removeFromOrganizationBoard, removeMemberFromCard, unconfirmedBoardInvitation, unconfirmedOrganizationInvitation, updateBoard, updateCard, updateCard:closed, updateCard:desc, updateCard:idList, updateCard:name, updateCheckItemStateOnCard, updateChecklist, updateList, updateList:closed, updateList:name, updateMember or updateOrganization
- attachments string? (default ()) - A boolean value or "cover" for only card cover attachments
- attachmentFields string (default "all") - All or a comma-separated list of: bytes, date, edgeColor, idMember, isUpload, mimeType, name, previews or url
- members string? (default ()) - True or false
- memberFields string (default "avatarHash, fullName, initials and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- checkItemStates string? (default ()) - True or false
- checklists string (default "none") - One of: all or none
- board string? (default ()) - True or false
- boardFields string (default "name, desc, closed, idOrganization, pinned, url and prefs") - All or a comma-separated list of: closed, dateLastActivity, dateLastView, desc, descData, idOrganization, invitations, invited, labelNames, memberships, name, pinned, powerUps, prefs, shortLink, shortUrl, starred, subscribed or url
- list string? (default ()) - True or false
- listFields string (default "all") - All or a comma-separated list of: closed, idBoard, name, pos or subscribed
- filter string (default "visible") - One of: all, closed, none, open or visible
- fields string (default "all") - All or a comma-separated list of: badges, checkItemStates, closed, dateLastActivity, desc, descData, due, email, idAttachmentCover, idBoard, idChecklists, idLabels, idList, idMembers, idMembersVoted, idShort, labels, manualCoverAttachment, name, pos, shortLink, shortUrl, subscribed or url
getBoardsMembersInvitedByIdBoard
Get boards members invited by ID board
Parameters
- idBoard string - Board_id
- fields string (default "all") - All or a comma-separated list of: avatarHash, avatarSource, bio, bioData, confirmed, email, fullName, gravatarHash, idBoards, idBoardsPinned, idOrganizations, idPremOrgsAdmin, initials, loginTypes, memberType, oneTimeMessagesDismissed, prefs, premiumFeatures, products, status, status, trophies, uploadedAvatarHash, url or username
getBoardsMembersInvitedByIdBoardByField
function getBoardsMembersInvitedByIdBoardByField(string idBoard, string 'field) returns Response|error
Get boards members invited by ID board by field
getBoardsMembershipsByIdBoard
function getBoardsMembershipsByIdBoard(string idBoard, string filter, string? member, string memberFields) returns Response|error
Get boards memberships by ID board
Parameters
- idBoard string - Board_id
- filter string (default "all") - All or a comma-separated list of: active, admin, deactivated, me or normal
- member string? (default ()) - True or false
- memberFields string (default "fullName and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
getBoardsMembershipsByIdBoardByIdMembership
function getBoardsMembershipsByIdBoardByIdMembership(string idBoard, string idMembership, string? member, string memberFields) returns Response|error
Get boards memberships by ID board by ID membership
Parameters
- idBoard string - Board_id
- idMembership string - IdMembership
- member string? (default ()) - True or false
- memberFields string (default "fullName and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
updateBoardsMembershipsByIdBoardByIdMembership
function updateBoardsMembershipsByIdBoardByIdMembership(string idBoard, string idMembership, BoardsMemberships payload) returns Response|error
Update boards memberships by ID board by ID membership
Parameters
- idBoard string - Board_id
- idMembership string - IdMembership
- payload BoardsMemberships - Attributes of "Boards Memberships" to be updated.
getBoardsMyPrefsByIdBoard
Get boards my prefs by ID board
Parameters
- idBoard string - Board_id
updateBoardsMyPrefsEmailPositionByIdBoard
function updateBoardsMyPrefsEmailPositionByIdBoard(string idBoard, MyprefsEmailposition payload) returns Response|error
Update boards my prefs email position by ID board
Parameters
- idBoard string - Board_id
- payload MyprefsEmailposition - Attributes of "My Prefs Email Position" to be updated.
updateBoardsMyPrefsIdEmailListByIdBoard
function updateBoardsMyPrefsIdEmailListByIdBoard(string idBoard, MyprefsIdemaillist payload) returns Response|error
Update boards my prefs ID email list by ID board
Parameters
- idBoard string - Board_id
- payload MyprefsIdemaillist - Attributes of "My Prefs ID Email List" to be updated.
updateBoardsMyPrefsShowListGuideByIdBoard
function updateBoardsMyPrefsShowListGuideByIdBoard(string idBoard, MyprefsShowlistguide payload) returns Response|error
Update boards my prefs show list guide by ID board
Parameters
- idBoard string - Board_id
- payload MyprefsShowlistguide - Attributes of "My Prefs Show List Guide" to be updated.
updateBoardsMyPrefsShowSidebarByIdBoard
function updateBoardsMyPrefsShowSidebarByIdBoard(string idBoard, MyprefsShowsidebar payload) returns Response|error
Update boards my prefs show sidebar by ID board
Parameters
- idBoard string - Board_id
- payload MyprefsShowsidebar - Attributes of "My Prefs Show Sidebar" to be updated.
updateBoardsMyPrefsShowSidebarActivityByIdBoard
function updateBoardsMyPrefsShowSidebarActivityByIdBoard(string idBoard, MyprefsShowsidebaractivity payload) returns Response|error
Update boards my prefs show sidebar activity by ID board
Parameters
- idBoard string - Board_id
- payload MyprefsShowsidebaractivity - Attributes of "My Prefs Show Sidebar Activity" to be updated.
updateBoardsMyPrefsShowSidebarBoardActionsByIdBoard
function updateBoardsMyPrefsShowSidebarBoardActionsByIdBoard(string idBoard, MyprefsShowsidebarboardactions payload) returns Response|error
Update boards my prefs show sidebar board actions by ID board
Parameters
- idBoard string - Board_id
- payload MyprefsShowsidebarboardactions - Attributes of "My Prefs Show Sidebar Board Actions" to be updated.
updateBoardsMyPrefsShowSidebarMembersByIdBoard
function updateBoardsMyPrefsShowSidebarMembersByIdBoard(string idBoard, MyprefsShowsidebarmembers payload) returns Response|error
Update boards my prefs show sidebar members by ID board
Parameters
- idBoard string - Board_id
- payload MyprefsShowsidebarmembers - Attributes of "My Prefs Show Sidebar Members" to be updated.
updateBoardsNameByIdBoard
function updateBoardsNameByIdBoard(string idBoard, BoardsName payload) returns Response|error
Update boards name by ID board
getBoardsOrganizationByIdBoard
Get boards organization by ID board
Parameters
- idBoard string - Board_id
- fields string (default "all") - All or a comma-separated list of: billableMemberCount, desc, descData, displayName, idBoards, invitations, invited, logoHash, memberships, name, powerUps, prefs, premiumFeatures, products, url or website
getBoardsOrganizationByIdBoardByField
function getBoardsOrganizationByIdBoardByField(string idBoard, string 'field) returns Response|error
Get boards organization by ID board by field
addBoardsPowerUpsByIdBoard
function addBoardsPowerUpsByIdBoard(string idBoard, BoardsPowerups payload) returns Response|error
Add boards power ups by ID board
Parameters
- idBoard string - Board_id
- payload BoardsPowerups - Attributes of "Boards Power Ups" to be added.
deleteBoardsPowerUpsByIdBoardByPowerUp
function deleteBoardsPowerUpsByIdBoardByPowerUp(string idBoard, string powerUp) returns Response|error
Delete boards power ups by ID board by power up
updateBoardsPrefsBackgroundByIdBoard
function updateBoardsPrefsBackgroundByIdBoard(string idBoard, PrefsBackground payload) returns Response|error
Update boards prefs background by ID board
Parameters
- idBoard string - Board_id
- payload PrefsBackground - Attributes of "Prefs Background" to be updated.
updateBoardsPrefsCalendarFeedEnabledByIdBoard
function updateBoardsPrefsCalendarFeedEnabledByIdBoard(string idBoard, PrefsCalendarfeedenabled payload) returns Response|error
Update boards prefs calendar feed enabled by ID board
Parameters
- idBoard string - Board_id
- payload PrefsCalendarfeedenabled - Attributes of "Prefs Calendar Feed Enabled" to be updated.
updateBoardsPrefsCardAgingByIdBoard
function updateBoardsPrefsCardAgingByIdBoard(string idBoard, PrefsCardaging payload) returns Response|error
Update boards prefs card aging by ID board
Parameters
- idBoard string - Board_id
- payload PrefsCardaging - Attributes of "Prefs Card Aging" to be updated.
updateBoardsPrefsCardCoversByIdBoard
function updateBoardsPrefsCardCoversByIdBoard(string idBoard, PrefsCardcovers payload) returns Response|error
Update boards prefs card covers by ID board
Parameters
- idBoard string - Board_id
- payload PrefsCardcovers - Attributes of "Prefs Card Covers" to be updated.
updateBoardsPrefsCommentsByIdBoard
function updateBoardsPrefsCommentsByIdBoard(string idBoard, PrefsComments payload) returns Response|error
Update boards prefs comments by ID board
Parameters
- idBoard string - Board_id
- payload PrefsComments - Attributes of "Prefs Comments" to be updated.
updateBoardsPrefsInvitationsByIdBoard
function updateBoardsPrefsInvitationsByIdBoard(string idBoard, PrefsInvitations payload) returns Response|error
Update boards prefs invitations by ID board
Parameters
- idBoard string - Board_id
- payload PrefsInvitations - Attributes of "Prefs Invitations" to be updated.
updateBoardsPrefsPermissionLevelByIdBoard
function updateBoardsPrefsPermissionLevelByIdBoard(string idBoard, PrefsPermissionlevel payload) returns Response|error
Update boards prefs permission level by ID board
Parameters
- idBoard string - Board_id
- payload PrefsPermissionlevel - Attributes of "Prefs Permission Level" to be updated.
updateBoardsPrefsSelfJoinByIdBoard
function updateBoardsPrefsSelfJoinByIdBoard(string idBoard, PrefsSelfjoin payload) returns Response|error
Update boards prefs self join by ID board
Parameters
- idBoard string - Board_id
- payload PrefsSelfjoin - Attributes of "Prefs Self Join" to be updated.
updateBoardsPrefsVotingByIdBoard
function updateBoardsPrefsVotingByIdBoard(string idBoard, PrefsVoting payload) returns Response|error
Update boards prefs voting by ID board
Parameters
- idBoard string - Board_id
- payload PrefsVoting - Attributes of "Prefs Voting" to be updated.
updateBoardsSubscribedByIdBoard
function updateBoardsSubscribedByIdBoard(string idBoard, BoardsSubscribed payload) returns Response|error
Update boards subscribed by ID board
Parameters
- idBoard string - Board_id
- payload BoardsSubscribed - Attributes of "Boards Subscribed" to be updated.
getBoardsByIdBoardByField
Get boards by ID board by field
addCards
Add cards
Parameters
- payload Cards - Attributes of "Cards" to be added.
getCardsByIdCard
function getCardsByIdCard(string idCard, string? actions, string? actionsEntities, string? actionsDisplay, string actionsLimit, string actionFields, string actionMembercreatorFields, string? attachments, string attachmentFields, string? members, string memberFields, string? membersVoted, string membervotedFields, string? checkItemStates, string checkitemstateFields, string checklists, string checklistFields, string? board, string boardFields, string? list, string listFields, string? stickers, string stickerFields, string fields) returns Response|error
Get cards by ID card
Parameters
- idCard string - Card ID or shortlink
- actions string? (default ()) - All or a comma-separated list of: addAttachmentToCard, addChecklistToCard, addMemberToBoard, addMemberToCard, addMemberToOrganization, addToOrganizationBoard, commentCard, convertToCardFromCheckItem, copyBoard, copyCard, copyCommentCard, createBoard, createCard, createList, createOrganization, deleteAttachmentFromCard, deleteBoardInvitation, deleteCard, deleteOrganizationInvitation, disablePowerUp, emailCard, enablePowerUp, makeAdminOfBoard, makeNormalMemberOfBoard, makeNormalMemberOfOrganization, makeObserverOfBoard, memberJoinedTrello, moveCardFromBoard, moveCardToBoard, moveListFromBoard, moveListToBoard, removeChecklistFromCard, removeFromOrganizationBoard, removeMemberFromCard, unconfirmedBoardInvitation, unconfirmedOrganizationInvitation, updateBoard, updateCard, updateCard:closed, updateCard:desc, updateCard:idList, updateCard:name, updateCheckItemStateOnCard, updateChecklist, updateList, updateList:closed, updateList:name, updateMember or updateOrganization
- actionsEntities string? (default ()) - True or false
- actionsDisplay string? (default ()) - True or false
- actionsLimit string (default "50") - A number from 0 to 1000
- actionFields string (default "all") - All or a comma-separated list of: data, date, idMemberCreator or type
- actionMembercreatorFields string (default "avatarHash, fullName, initials and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- attachments string? (default ()) - A boolean value or "cover" for only card cover attachments
- attachmentFields string (default "all") - All or a comma-separated list of: bytes, date, edgeColor, idMember, isUpload, mimeType, name, previews or url
- members string? (default ()) - True or false
- memberFields string (default "avatarHash, fullName, initials and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- membersVoted string? (default ()) - True or false
- membervotedFields string (default "avatarHash, fullName, initials and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- checkItemStates string? (default ()) - True or false
- checkitemstateFields string (default "all") - All or a comma-separated list of: idCheckItem or state
- checklists string (default "none") - One of: all or none
- checklistFields string (default "all") - All or a comma-separated list of: idBoard, idCard, name or pos
- board string? (default ()) - True or false
- boardFields string (default "name, desc, descData, closed, idOrganization, pinned, url and prefs") - All or a comma-separated list of: closed, dateLastActivity, dateLastView, desc, descData, idOrganization, invitations, invited, labelNames, memberships, name, pinned, powerUps, prefs, shortLink, shortUrl, starred, subscribed or url
- list string? (default ()) - True or false
- listFields string (default "all") - All or a comma-separated list of: closed, idBoard, name, pos or subscribed
- stickers string? (default ()) - True or false
- stickerFields string (default "all") - All or a comma-separated list of: image, imageScaled, imageUrl, left, rotate, top or zIndex
- fields string (default "badges, checkItemStates, closed, dateLastActivity, desc, descData, due, email, idBoard, idChecklists, idLabels, idList, idMembers, idShort, idAttachmentCover, manualCoverAttachment, labels, name, pos, shortUrl and url") - All or a comma-separated list of: badges, checkItemStates, closed, dateLastActivity, desc, descData, due, email, idAttachmentCover, idBoard, idChecklists, idLabels, idList, idMembers, idMembersVoted, idShort, labels, manualCoverAttachment, name, pos, shortLink, shortUrl, subscribed or url
updateCardsByIdCard
Update cards by ID card
deleteCardsByIdCard
Delete cards by ID card
Parameters
- idCard string - Card ID or shortlink
getCardsActionsByIdCard
function getCardsActionsByIdCard(string idCard, string? entities, string? display, string filter, string fields, string 'limit, string format, string? since, string? before, string page, string? idModels, string? member, string memberFields, string? memberCreator, string membercreatorFields) returns Response|error
Get cards actions by ID card
Parameters
- idCard string - Card ID or shortlink
- entities string? (default ()) - True or false
- display string? (default ()) - True or false
- filter string (default "commentCard and updateCard:idList") - All or a comma-separated list of: addAttachmentToCard, addChecklistToCard, addMemberToBoard, addMemberToCard, addMemberToOrganization, addToOrganizationBoard, commentCard, convertToCardFromCheckItem, copyBoard, copyCard, copyCommentCard, createBoard, createCard, createList, createOrganization, deleteAttachmentFromCard, deleteBoardInvitation, deleteCard, deleteOrganizationInvitation, disablePowerUp, emailCard, enablePowerUp, makeAdminOfBoard, makeNormalMemberOfBoard, makeNormalMemberOfOrganization, makeObserverOfBoard, memberJoinedTrello, moveCardFromBoard, moveCardToBoard, moveListFromBoard, moveListToBoard, removeChecklistFromCard, removeFromOrganizationBoard, removeMemberFromCard, unconfirmedBoardInvitation, unconfirmedOrganizationInvitation, updateBoard, updateCard, updateCard:closed, updateCard:desc, updateCard:idList, updateCard:name, updateCheckItemStateOnCard, updateChecklist, updateList, updateList:closed, updateList:name, updateMember or updateOrganization
- fields string (default "all") - All or a comma-separated list of: data, date, idMemberCreator or type
- 'limit string (default "50") - A number from 0 to 1000
- format string (default "list") - One of: count, list or minimal
- since string? (default ()) - A date, null or lastView
- before string? (default ()) - A date, or null
- page string (default "0") - Page * limit must be less than 1000
- idModels string? (default ()) - Only return actions related to these model ids
- member string? (default ()) - True or false
- memberFields string (default "avatarHash, fullName, initials and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- memberCreator string? (default ()) - True or false
- membercreatorFields string (default "avatarHash, fullName, initials and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
addCardsActionsCommentsByIdCard
function addCardsActionsCommentsByIdCard(string idCard, ActionsComments payload) returns Response|error
Add cards actions comments by ID card
Parameters
- idCard string - Card ID or shortlink
- payload ActionsComments - Attributes of "Actions Comments" to be added.
updateCardsActionsCommentsByIdCardByIdAction
function updateCardsActionsCommentsByIdCardByIdAction(string idCard, string idAction, CardsActionsComments payload) returns Response|error
Update cards actions comments by ID card by ID action
Parameters
- idCard string - Card ID or shortlink
- idAction string - IdAction
- payload CardsActionsComments - Attributes of "Cards Actions Comments" to be updated.
deleteCardsActionsCommentsByIdCardByIdAction
function deleteCardsActionsCommentsByIdCardByIdAction(string idCard, string idAction) returns Response|error
Delete cards actions comments by ID card by ID action
getCardsAttachmentsByIdCard
function getCardsAttachmentsByIdCard(string idCard, string fields, string? filter) returns Response|error
Get cards attachments by ID card
Parameters
- idCard string - Card ID or shortlink
- fields string (default "all") - All or a comma-separated list of: bytes, date, edgeColor, idMember, isUpload, mimeType, name, previews or url
- filter string? (default ()) - A boolean value or "cover" for only card cover attachments
addCardsAttachmentsByIdCard
function addCardsAttachmentsByIdCard(string idCard, CardsAttachments payload) returns Response|error
Add cards attachments by ID card
Parameters
- idCard string - Card ID or shortlink
- payload CardsAttachments - Attributes of "Cards Attachments" to be added.
getCardsAttachmentsByIdCardByIdAttachment
function getCardsAttachmentsByIdCardByIdAttachment(string idCard, string idAttachment, string fields) returns Response|error
Get cards attachments by ID card by ID attachment
Parameters
- idCard string - Card ID or shortlink
- idAttachment string - IdAttachment
- fields string (default "all") - All or a comma-separated list of: bytes, date, edgeColor, idMember, isUpload, mimeType, name, previews or url
deleteCardsAttachmentsByIdCardByIdAttachment
function deleteCardsAttachmentsByIdCardByIdAttachment(string idCard, string idAttachment) returns Response|error
Delete cards attachments by ID card by ID attachment
getCardsBoardByIdCard
Get cards board by ID card
Parameters
- idCard string - Card ID or shortlink
- fields string (default "all") - All or a comma-separated list of: closed, dateLastActivity, dateLastView, desc, descData, idOrganization, invitations, invited, labelNames, memberships, name, pinned, powerUps, prefs, shortLink, shortUrl, starred, subscribed or url
getCardsBoardByIdCardByField
Get cards board by ID card by field
getCardsCheckItemStatesByIdCard
Get cards check item states by ID card
Parameters
- idCard string - Card ID or shortlink
- fields string (default "all") - All or a comma-separated list of: idCheckItem or state
updateCardsChecklistCheckItemByIdCardByIdChecklistCurrentByIdCheckItem
function updateCardsChecklistCheckItemByIdCardByIdChecklistCurrentByIdCheckItem(string idCard, string idChecklistCurrent, string idCheckItem, CardsChecklistIdchecklistcurrentCheckitem payload) returns Response|error
Update cards checklist check item by ID card by ID checklist current by ID check item
Parameters
- idCard string - Card ID or shortlink
- idChecklistCurrent string - IdChecklistCurrent
- idCheckItem string - IdCheckItem
- payload CardsChecklistIdchecklistcurrentCheckitem - Attributes of "Cards Checklist ID Checklist Current Check Item" to be updated.
addCardsChecklistCheckItemByIdCardByIdChecklist
function addCardsChecklistCheckItemByIdCardByIdChecklist(string idCard, string idChecklist, CardsChecklistCheckitem payload) returns Response|error
Add cards checklist check item by ID card by ID checklist
Parameters
- idCard string - Card ID or shortlink
- idChecklist string - IdChecklist
- payload CardsChecklistCheckitem - Attributes of "Cards Checklist Check Item" to be added.
deleteCardsChecklistCheckItemByIdCardByIdChecklistByIdCheckItem
function deleteCardsChecklistCheckItemByIdCardByIdChecklistByIdCheckItem(string idCard, string idChecklist, string idCheckItem) returns Response|error
Delete cards checklist check item by ID card by ID checklist by ID check item
Parameters
- idCard string - Card ID or shortlink
- idChecklist string - IdChecklist
- idCheckItem string - IdCheckItem
addCardsChecklistCheckItemConvertToCardByIdCardByIdChecklistByIdCheckItem
function addCardsChecklistCheckItemConvertToCardByIdCardByIdChecklistByIdCheckItem(string idCard, string idChecklist, string idCheckItem) returns Response|error
Add cards checklist check item convert to card by ID card by ID checklist by ID check item
Parameters
- idCard string - Card ID or shortlink
- idChecklist string - IdChecklist
- idCheckItem string - IdCheckItem
updateCardsChecklistCheckItemNameByIdCardByIdChecklistByIdCheckItem
function updateCardsChecklistCheckItemNameByIdCardByIdChecklistByIdCheckItem(string idCard, string idChecklist, string idCheckItem, CardsChecklistCheckitemName payload) returns Response|error
Update cards checklist check item name by ID card by ID checklist by ID check item
Parameters
- idCard string - Card ID or shortlink
- idChecklist string - IdChecklist
- idCheckItem string - IdCheckItem
- payload CardsChecklistCheckitemName - Attributes of "Cards Checklist Check Item Name" to be updated.
updateCardsChecklistCheckItemPosByIdCardByIdChecklistByIdCheckItem
function updateCardsChecklistCheckItemPosByIdCardByIdChecklistByIdCheckItem(string idCard, string idChecklist, string idCheckItem, CardsChecklistCheckitemPos payload) returns Response|error
Update cards checklist check item pos by ID card by ID checklist by ID check item
Parameters
- idCard string - Card ID or shortlink
- idChecklist string - IdChecklist
- idCheckItem string - IdCheckItem
- payload CardsChecklistCheckitemPos - Attributes of "Cards Checklist Check Item Pos" to be updated.
updateCardsChecklistCheckItemStateByIdCardByIdChecklistByIdCheckItem
function updateCardsChecklistCheckItemStateByIdCardByIdChecklistByIdCheckItem(string idCard, string idChecklist, string idCheckItem, CardsChecklistCheckitemState payload) returns Response|error
Update cards checklist check item state by ID card by ID checklist by ID check item
Parameters
- idCard string - Card ID or shortlink
- idChecklist string - IdChecklist
- idCheckItem string - IdCheckItem
- payload CardsChecklistCheckitemState - Attributes of "Cards Checklist Check Item State" to be updated.
getCardsChecklistsByIdCard
function getCardsChecklistsByIdCard(string idCard, string cards, string cardFields, string checkItems, string checkitemFields, string filter, string fields) returns Response|error
Get cards checklists by ID card
Parameters
- idCard string - Card ID or shortlink
- cards string (default "none") - One of: all, closed, none, open or visible
- cardFields string (default "all") - All or a comma-separated list of: badges, checkItemStates, closed, dateLastActivity, desc, descData, due, email, idAttachmentCover, idBoard, idChecklists, idLabels, idList, idMembers, idMembersVoted, idShort, labels, manualCoverAttachment, name, pos, shortLink, shortUrl, subscribed or url
- checkItems string (default "all") - One of: all or none
- checkitemFields string (default "name, nameData, pos and state") - All or a comma-separated list of: name, nameData, pos, state or type
- filter string (default "all") - One of: all or none
- fields string (default "all") - All or a comma-separated list of: idBoard, idCard, name or pos
addCardsChecklistsByIdCard
function addCardsChecklistsByIdCard(string idCard, CardsChecklists payload) returns Response|error
Add cards checklists by ID card
Parameters
- idCard string - Card ID or shortlink
- payload CardsChecklists - Attributes of "Cards Checklists" to be added.
deleteCardsChecklistsByIdCardByIdChecklist
function deleteCardsChecklistsByIdCardByIdChecklist(string idCard, string idChecklist) returns Response|error
Delete cards checklists by ID card by ID checklist
updateCardsClosedByIdCard
function updateCardsClosedByIdCard(string idCard, CardsClosed payload) returns Response|error
Update cards closed by ID card
Parameters
- idCard string - Card ID or shortlink
- payload CardsClosed - Attributes of "Cards Closed" to be updated.
updateCardsDescByIdCard
Update cards desc by ID card
Parameters
- idCard string - Card ID or shortlink
- payload CardsDesc - Attributes of "Cards Desc" to be updated.
updateCardsDueByIdCard
Update cards due by ID card
Parameters
- idCard string - Card ID or shortlink
- payload CardsDue - Attributes of "Cards Due" to be updated.
updateCardsIdAttachmentCoverByIdCard
function updateCardsIdAttachmentCoverByIdCard(string idCard, CardsIdattachmentcover payload) returns Response|error
Update cards ID attachment cover by ID card
Parameters
- idCard string - Card ID or shortlink
- payload CardsIdattachmentcover - Attributes of "Cards ID Attachment Cover" to be updated.
updateCardsIdBoardByIdCard
function updateCardsIdBoardByIdCard(string idCard, CardsIdboard payload) returns Response|error
Update cards ID board by ID card
Parameters
- idCard string - Card ID or shortlink
- payload CardsIdboard - Attributes of "Cards ID Board" to be updated.
addCardsIdLabelsByIdCard
function addCardsIdLabelsByIdCard(string idCard, CardsIdlabels payload) returns Response|error
Add cards ID labels by ID card
Parameters
- idCard string - Card ID or shortlink
- payload CardsIdlabels - Attributes of "Cards ID Labels" to be added.
deleteCardsIdLabelsByIdCardByIdLabel
Delete cards ID labels by ID card by ID label
updateCardsIdListByIdCard
function updateCardsIdListByIdCard(string idCard, CardsIdlist payload) returns Response|error
Update cards ID list by ID card
Parameters
- idCard string - Card ID or shortlink
- payload CardsIdlist - Attributes of "Cards ID List" to be updated.
updateCardsIdMembersByIdCard
function updateCardsIdMembersByIdCard(string idCard, CardsIdmembers payload) returns Response|error
Update cards ID members by ID card
Parameters
- idCard string - Card ID or shortlink
- payload CardsIdmembers - Attributes of "Cards ID Members" to be updated.
addCardsIdMembersByIdCard
function addCardsIdMembersByIdCard(string idCard, CardsIdmembers payload) returns Response|error
Add cards ID members by ID card
Parameters
- idCard string - Card ID or shortlink
- payload CardsIdmembers - Attributes of "Cards ID Members" to be added.
deleteCardsIdMembersByIdCardByIdMember
function deleteCardsIdMembersByIdCardByIdMember(string idCard, string idMember) returns Response|error
Delete cards ID members by ID card by ID member
updateCardsLabelsByIdCard
function updateCardsLabelsByIdCard(string idCard, CardsLabels payload) returns Response|error
Update cards labels by ID card
Parameters
- idCard string - Card ID or shortlink
- payload CardsLabels - Attributes of "Cards Labels" to be updated.
addCardsLabelsByIdCard
function addCardsLabelsByIdCard(string idCard, CardsLabels payload) returns Response|error
Add cards labels by ID card
Parameters
- idCard string - Card ID or shortlink
- payload CardsLabels - Attributes of "Cards Labels" to be added.
deleteCardsLabelsByIdCardByColor
Delete cards labels by ID card by color
getCardsListByIdCard
Get cards list by ID card
Parameters
- idCard string - Card ID or shortlink
- fields string (default "all") - All or a comma-separated list of: closed, idBoard, name, pos or subscribed
getCardsListByIdCardByField
Get cards list by ID card by field
addCardsMarkAssociatedNotificationsReadByIdCard
Add cards mark associated notifications read by ID card
Parameters
- idCard string - Card ID or shortlink
getCardsMembersByIdCard
Get cards members by ID card
Parameters
- idCard string - Card ID or shortlink
- fields string (default "avatarHash, fullName, initials and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
getCardsMembersVotedByIdCard
Get cards members voted by ID card
Parameters
- idCard string - Card ID or shortlink
- fields string (default "avatarHash, fullName, initials and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
addCardsMembersVotedByIdCard
function addCardsMembersVotedByIdCard(string idCard, CardsMembersvoted payload) returns Response|error
Add cards members voted by ID card
Parameters
- idCard string - Card ID or shortlink
- payload CardsMembersvoted - Attributes of "Cards Members Voted" to be added.
deleteCardsMembersVotedByIdCardByIdMember
function deleteCardsMembersVotedByIdCardByIdMember(string idCard, string idMember) returns Response|error
Delete cards members voted by ID card by ID member
updateCardsNameByIdCard
Update cards name by ID card
Parameters
- idCard string - Card ID or shortlink
- payload CardsName - Attributes of "Cards Name" to be updated.
updateCardsPosByIdCard
Update cards pos by ID card
Parameters
- idCard string - Card ID or shortlink
- payload CardsPos - Attributes of "Cards Pos" to be updated.
getCardsStickersByIdCard
Get cards stickers by ID card
Parameters
- idCard string - Card ID or shortlink
- fields string (default "all") - All or a comma-separated list of: image, imageScaled, imageUrl, left, rotate, top or zIndex
addCardsStickersByIdCard
function addCardsStickersByIdCard(string idCard, CardsStickers payload) returns Response|error
Add cards stickers by ID card
Parameters
- idCard string - Card ID or shortlink
- payload CardsStickers - Attributes of "Cards Stickers" to be added.
getCardsStickersByIdCardByIdSticker
function getCardsStickersByIdCardByIdSticker(string idCard, string idSticker, string fields) returns Response|error
Get cards stickers by ID card by ID sticker
Parameters
- idCard string - Card ID or shortlink
- idSticker string - IdSticker
- fields string (default "all") - All or a comma-separated list of: image, imageScaled, imageUrl, left, rotate, top or zIndex
updateCardsStickersByIdCardByIdSticker
function updateCardsStickersByIdCardByIdSticker(string idCard, string idSticker, CardsStickers payload) returns Response|error
Update cards stickers by ID card by ID sticker
Parameters
- idCard string - Card ID or shortlink
- idSticker string - IdSticker
- payload CardsStickers - Attributes of "Cards Stickers" to be updated.
deleteCardsStickersByIdCardByIdSticker
function deleteCardsStickersByIdCardByIdSticker(string idCard, string idSticker) returns Response|error
Delete cards stickers by ID card by ID sticker
updateCardsSubscribedByIdCard
function updateCardsSubscribedByIdCard(string idCard, CardsSubscribed payload) returns Response|error
Update cards subscribed by ID card
Parameters
- idCard string - Card ID or shortlink
- payload CardsSubscribed - Attributes of "Cards Subscribed" to be updated.
getCardsByIdCardByField
Get cards by ID card by field
addChecklists
function addChecklists(Checklists payload) returns Response|error
Add checklists
Parameters
- payload Checklists - Attributes of "Checklists" to be added.
getChecklistsByIdChecklist
function getChecklistsByIdChecklist(string idChecklist, string cards, string cardFields, string checkItems, string checkitemFields, string fields) returns Response|error
Get checklists by ID checklist
Parameters
- idChecklist string - IdChecklist
- cards string (default "none") - One of: all, closed, none, open or visible
- cardFields string (default "all") - All or a comma-separated list of: badges, checkItemStates, closed, dateLastActivity, desc, descData, due, email, idAttachmentCover, idBoard, idChecklists, idLabels, idList, idMembers, idMembersVoted, idShort, labels, manualCoverAttachment, name, pos, shortLink, shortUrl, subscribed or url
- checkItems string (default "all") - One of: all or none
- checkitemFields string (default "name, nameData, pos and state") - All or a comma-separated list of: name, nameData, pos, state or type
- fields string (default "all") - All or a comma-separated list of: idBoard, idCard, name or pos
updateChecklistsByIdChecklist
function updateChecklistsByIdChecklist(string idChecklist, Checklists payload) returns Response|error
Update checklists by ID checklist
Parameters
- idChecklist string - IdChecklist
- payload Checklists - Attributes of "Checklists" to be updated.
deleteChecklistsByIdChecklist
Delete checklists by ID checklist
Parameters
- idChecklist string - IdChecklist
getChecklistsBoardByIdChecklist
Get checklists board by ID checklist
Parameters
- idChecklist string - IdChecklist
- fields string (default "all") - All or a comma-separated list of: closed, dateLastActivity, dateLastView, desc, descData, idOrganization, invitations, invited, labelNames, memberships, name, pinned, powerUps, prefs, shortLink, shortUrl, starred, subscribed or url
getChecklistsBoardByIdChecklistByField
function getChecklistsBoardByIdChecklistByField(string idChecklist, string 'field) returns Response|error
Get checklists board by ID checklist by field
getChecklistsCardsByIdChecklist
function getChecklistsCardsByIdChecklist(string idChecklist, string? actions, string? attachments, string attachmentFields, string? stickers, string? members, string memberFields, string? checkItemStates, string checklists, string? 'limit, string? since, string? before, string filter, string fields) returns Response|error
Get checklists cards by ID checklist
Parameters
- idChecklist string - IdChecklist
- actions string? (default ()) - All or a comma-separated list of: addAttachmentToCard, addChecklistToCard, addMemberToBoard, addMemberToCard, addMemberToOrganization, addToOrganizationBoard, commentCard, convertToCardFromCheckItem, copyBoard, copyCard, copyCommentCard, createBoard, createCard, createList, createOrganization, deleteAttachmentFromCard, deleteBoardInvitation, deleteCard, deleteOrganizationInvitation, disablePowerUp, emailCard, enablePowerUp, makeAdminOfBoard, makeNormalMemberOfBoard, makeNormalMemberOfOrganization, makeObserverOfBoard, memberJoinedTrello, moveCardFromBoard, moveCardToBoard, moveListFromBoard, moveListToBoard, removeChecklistFromCard, removeFromOrganizationBoard, removeMemberFromCard, unconfirmedBoardInvitation, unconfirmedOrganizationInvitation, updateBoard, updateCard, updateCard:closed, updateCard:desc, updateCard:idList, updateCard:name, updateCheckItemStateOnCard, updateChecklist, updateList, updateList:closed, updateList:name, updateMember or updateOrganization
- attachments string? (default ()) - A boolean value or "cover" for only card cover attachments
- attachmentFields string (default "all") - All or a comma-separated list of: bytes, date, edgeColor, idMember, isUpload, mimeType, name, previews or url
- stickers string? (default ()) - True or false
- members string? (default ()) - True or false
- memberFields string (default "avatarHash, fullName, initials and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- checkItemStates string? (default ()) - True or false
- checklists string (default "none") - One of: all or none
- 'limit string? (default ()) - A number from 1 to 1000
- since string? (default ()) - A date, or null
- before string? (default ()) - A date, or null
- filter string (default "open") - One of: all, closed, none or open
- fields string (default "all") - All or a comma-separated list of: badges, checkItemStates, closed, dateLastActivity, desc, descData, due, email, idAttachmentCover, idBoard, idChecklists, idLabels, idList, idMembers, idMembersVoted, idShort, labels, manualCoverAttachment, name, pos, shortLink, shortUrl, subscribed or url
getChecklistsCardsByIdChecklistByFilter
function getChecklistsCardsByIdChecklistByFilter(string idChecklist, string filter) returns Response|error
Get checklists cards by ID checklist by filter
getChecklistsCheckItemsByIdChecklist
function getChecklistsCheckItemsByIdChecklist(string idChecklist, string filter, string fields) returns Response|error
Get checklists check items by ID checklist
Parameters
- idChecklist string - IdChecklist
- filter string (default "all") - One of: all or none
- fields string (default "name, nameData, pos and state") - All or a comma-separated list of: name, nameData, pos, state or type
addChecklistsCheckItemsByIdChecklist
function addChecklistsCheckItemsByIdChecklist(string idChecklist, ChecklistsCheckitems payload) returns Response|error
Add checklists check items by ID checklist
Parameters
- idChecklist string - IdChecklist
- payload ChecklistsCheckitems - Attributes of "Checklists Check Items" to be added.
getChecklistsCheckItemsByIdChecklistByIdCheckItem
function getChecklistsCheckItemsByIdChecklistByIdCheckItem(string idChecklist, string idCheckItem, string fields) returns Response|error
Get checklists check items by ID checklist by ID check item
Parameters
- idChecklist string - IdChecklist
- idCheckItem string - IdCheckItem
- fields string (default "name, nameData, pos and state") - All or a comma-separated list of: name, nameData, pos, state or type
deleteChecklistsCheckItemsByIdChecklistByIdCheckItem
function deleteChecklistsCheckItemsByIdChecklistByIdCheckItem(string idChecklist, string idCheckItem) returns Response|error
Delete checklists check items by ID checklist by ID check item
updateChecklistsIdCardByIdChecklist
function updateChecklistsIdCardByIdChecklist(string idChecklist, ChecklistsIdcard payload) returns Response|error
Update checklists ID card by ID checklist
Parameters
- idChecklist string - IdChecklist
- payload ChecklistsIdcard - Attributes of "Checklists ID Card" to be updated.
updateChecklistsNameByIdChecklist
function updateChecklistsNameByIdChecklist(string idChecklist, ChecklistsName payload) returns Response|error
Update checklists name by ID checklist
Parameters
- idChecklist string - IdChecklist
- payload ChecklistsName - Attributes of "Checklists Name" to be updated.
updateChecklistsPosByIdChecklist
function updateChecklistsPosByIdChecklist(string idChecklist, ChecklistsPos payload) returns Response|error
Update checklists pos by ID checklist
Parameters
- idChecklist string - IdChecklist
- payload ChecklistsPos - Attributes of "Checklists Pos" to be updated.
getChecklistsByIdChecklistByField
function getChecklistsByIdChecklistByField(string idChecklist, string 'field) returns Response|error
Get checklists by ID checklist by field
addLabels
Add labels
Parameters
- payload Labels - Attributes of "Labels" to be added.
getLabelsByIdLabel
Get labels by ID label
Parameters
- idLabel string - IdLabel
- fields string (default "all") - All or a comma-separated list of: color, idBoard, name or uses
updateLabelsByIdLabel
Update labels by ID label
deleteLabelsByIdLabel
Delete labels by ID label
Parameters
- idLabel string - IdLabel
getLabelsBoardByIdLabel
Get labels board by ID label
Parameters
- idLabel string - IdLabel
- fields string (default "all") - All or a comma-separated list of: closed, dateLastActivity, dateLastView, desc, descData, idOrganization, invitations, invited, labelNames, memberships, name, pinned, powerUps, prefs, shortLink, shortUrl, starred, subscribed or url
getLabelsBoardByIdLabelByField
Get labels board by ID label by field
updateLabelsColorByIdLabel
function updateLabelsColorByIdLabel(string idLabel, LabelsColor payload) returns Response|error
Update labels color by ID label
updateLabelsNameByIdLabel
function updateLabelsNameByIdLabel(string idLabel, LabelsName payload) returns Response|error
Update labels name by ID label
addLists
Add lists
Parameters
- payload Lists - Attributes of "Lists" to be added.
getListsByIdList
function getListsByIdList(string idList, string cards, string cardFields, string? board, string boardFields, string fields) returns Response|error
Get lists by ID list
Parameters
- idList string - IdList
- cards string (default "none") - One of: all, closed, none or open
- cardFields string (default "all") - All or a comma-separated list of: badges, checkItemStates, closed, dateLastActivity, desc, descData, due, email, idAttachmentCover, idBoard, idChecklists, idLabels, idList, idMembers, idMembersVoted, idShort, labels, manualCoverAttachment, name, pos, shortLink, shortUrl, subscribed or url
- board string? (default ()) - True or false
- boardFields string (default "name, desc, descData, closed, idOrganization, pinned, url and prefs") - All or a comma-separated list of: closed, dateLastActivity, dateLastView, desc, descData, idOrganization, invitations, invited, labelNames, memberships, name, pinned, powerUps, prefs, shortLink, shortUrl, starred, subscribed or url
- fields string (default "name, closed, idBoard and pos") - All or a comma-separated list of: closed, idBoard, name, pos or subscribed
updateListsByIdList
Update lists by ID list
getListsActionsByIdList
function getListsActionsByIdList(string idList, string? entities, string? display, string filter, string fields, string 'limit, string format, string? since, string? before, string page, string? idModels, string? member, string memberFields, string? memberCreator, string membercreatorFields) returns Response|error
Get lists actions by ID list
Parameters
- idList string - IdList
- entities string? (default ()) - True or false
- display string? (default ()) - True or false
- filter string (default "all") - All or a comma-separated list of: addAttachmentToCard, addChecklistToCard, addMemberToBoard, addMemberToCard, addMemberToOrganization, addToOrganizationBoard, commentCard, convertToCardFromCheckItem, copyBoard, copyCard, copyCommentCard, createBoard, createCard, createList, createOrganization, deleteAttachmentFromCard, deleteBoardInvitation, deleteCard, deleteOrganizationInvitation, disablePowerUp, emailCard, enablePowerUp, makeAdminOfBoard, makeNormalMemberOfBoard, makeNormalMemberOfOrganization, makeObserverOfBoard, memberJoinedTrello, moveCardFromBoard, moveCardToBoard, moveListFromBoard, moveListToBoard, removeChecklistFromCard, removeFromOrganizationBoard, removeMemberFromCard, unconfirmedBoardInvitation, unconfirmedOrganizationInvitation, updateBoard, updateCard, updateCard:closed, updateCard:desc, updateCard:idList, updateCard:name, updateCheckItemStateOnCard, updateChecklist, updateList, updateList:closed, updateList:name, updateMember or updateOrganization
- fields string (default "all") - All or a comma-separated list of: data, date, idMemberCreator or type
- 'limit string (default "50") - A number from 0 to 1000
- format string (default "list") - One of: count, list or minimal
- since string? (default ()) - A date, null or lastView
- before string? (default ()) - A date, or null
- page string (default "0") - Page * limit must be less than 1000
- idModels string? (default ()) - Only return actions related to these model ids
- member string? (default ()) - True or false
- memberFields string (default "avatarHash, fullName, initials and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- memberCreator string? (default ()) - True or false
- membercreatorFields string (default "avatarHash, fullName, initials and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
addListsArchiveAllCardsByIdList
Add lists archive all cards by ID list
Parameters
- idList string - IdList
getListsBoardByIdList
Get lists board by ID list
Parameters
- idList string - IdList
- fields string (default "all") - All or a comma-separated list of: closed, dateLastActivity, dateLastView, desc, descData, idOrganization, invitations, invited, labelNames, memberships, name, pinned, powerUps, prefs, shortLink, shortUrl, starred, subscribed or url
getListsBoardByIdListByField
Get lists board by ID list by field
getListsCardsByIdList
function getListsCardsByIdList(string idList, string? actions, string? attachments, string attachmentFields, string? stickers, string? members, string memberFields, string? checkItemStates, string checklists, string? 'limit, string? since, string? before, string filter, string fields) returns Response|error
Get lists cards by ID list
Parameters
- idList string - IdList
- actions string? (default ()) - All or a comma-separated list of: addAttachmentToCard, addChecklistToCard, addMemberToBoard, addMemberToCard, addMemberToOrganization, addToOrganizationBoard, commentCard, convertToCardFromCheckItem, copyBoard, copyCard, copyCommentCard, createBoard, createCard, createList, createOrganization, deleteAttachmentFromCard, deleteBoardInvitation, deleteCard, deleteOrganizationInvitation, disablePowerUp, emailCard, enablePowerUp, makeAdminOfBoard, makeNormalMemberOfBoard, makeNormalMemberOfOrganization, makeObserverOfBoard, memberJoinedTrello, moveCardFromBoard, moveCardToBoard, moveListFromBoard, moveListToBoard, removeChecklistFromCard, removeFromOrganizationBoard, removeMemberFromCard, unconfirmedBoardInvitation, unconfirmedOrganizationInvitation, updateBoard, updateCard, updateCard:closed, updateCard:desc, updateCard:idList, updateCard:name, updateCheckItemStateOnCard, updateChecklist, updateList, updateList:closed, updateList:name, updateMember or updateOrganization
- attachments string? (default ()) - A boolean value or "cover" for only card cover attachments
- attachmentFields string (default "all") - All or a comma-separated list of: bytes, date, edgeColor, idMember, isUpload, mimeType, name, previews or url
- stickers string? (default ()) - True or false
- members string? (default ()) - True or false
- memberFields string (default "avatarHash, fullName, initials and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- checkItemStates string? (default ()) - True or false
- checklists string (default "none") - One of: all or none
- 'limit string? (default ()) - A number from 1 to 1000
- since string? (default ()) - A date, or null
- before string? (default ()) - A date, or null
- filter string (default "open") - One of: all, closed, none or open
- fields string (default "all") - All or a comma-separated list of: badges, checkItemStates, closed, dateLastActivity, desc, descData, due, email, idAttachmentCover, idBoard, idChecklists, idLabels, idList, idMembers, idMembersVoted, idShort, labels, manualCoverAttachment, name, pos, shortLink, shortUrl, subscribed or url
addListsCardsByIdList
function addListsCardsByIdList(string idList, ListsCards payload) returns Response|error
Add lists cards by ID list
getListsCardsByIdListByFilter
Get lists cards by ID list by filter
updateListsClosedByIdList
function updateListsClosedByIdList(string idList, ListsClosed payload) returns Response|error
Update lists closed by ID list
updateListsIdBoardByIdList
function updateListsIdBoardByIdList(string idList, ListsIdboard payload) returns Response|error
Update lists ID board by ID list
Parameters
- idList string - IdList
- payload ListsIdboard - Attributes of "Lists ID Board" to be updated.
addListsMoveAllCardsByIdList
function addListsMoveAllCardsByIdList(string idList, ListsMoveallcards payload) returns Response|error
Add lists move all cards by ID list
Parameters
- idList string - IdList
- payload ListsMoveallcards - Attributes of "Lists Move All Cards" to be added.
updateListsNameByIdList
Update lists name by ID list
updateListsPosByIdList
Update lists pos by ID list
updateListsSubscribedByIdList
function updateListsSubscribedByIdList(string idList, ListsSubscribed payload) returns Response|error
Update lists subscribed by ID list
Parameters
- idList string - IdList
- payload ListsSubscribed - Attributes of "Lists Subscribed" to be updated.
getListsByIdListByField
Get lists by ID list by field
getMembersByIdMember
function getMembersByIdMember(string idMember, string? actions, string? actionsEntities, string? actionsDisplay, string actionsLimit, string actionFields, string? actionSince, string? actionBefore, string cards, string cardFields, string? cardMembers, string cardMemberFields, string? cardAttachments, string cardAttachmentFields, string? cardStickers, string? boards, string boardFields, string? boardActions, string? boardActionsEntities, string? boardActionsDisplay, string boardActionsFormat, string? boardActionsSince, string boardActionsLimit, string boardActionFields, string boardLists, string boardMemberships, string? boardOrganization, string boardOrganizationFields, string? boardsInvited, string boardsinvitedFields, string? boardStars, string? savedSearches, string organizations, string organizationFields, string? organizationPaidAccount, string organizationsInvited, string organizationsinvitedFields, string? notifications, string? notificationsEntities, string? notificationsDisplay, string notificationsLimit, string notificationFields, string? notificationMembercreator, string notificationMembercreatorFields, string? notificationBefore, string? notificationSince, string tokens, string? paidAccount, string boardBackgrounds, string customBoardBackgrounds, string customStickers, string customEmoji, string fields) returns Response|error
Get members by ID member
Parameters
- idMember string - IdMember or username
- actions string? (default ()) - All or a comma-separated list of: addAttachmentToCard, addChecklistToCard, addMemberToBoard, addMemberToCard, addMemberToOrganization, addToOrganizationBoard, commentCard, convertToCardFromCheckItem, copyBoard, copyCard, copyCommentCard, createBoard, createCard, createList, createOrganization, deleteAttachmentFromCard, deleteBoardInvitation, deleteCard, deleteOrganizationInvitation, disablePowerUp, emailCard, enablePowerUp, makeAdminOfBoard, makeNormalMemberOfBoard, makeNormalMemberOfOrganization, makeObserverOfBoard, memberJoinedTrello, moveCardFromBoard, moveCardToBoard, moveListFromBoard, moveListToBoard, removeChecklistFromCard, removeFromOrganizationBoard, removeMemberFromCard, unconfirmedBoardInvitation, unconfirmedOrganizationInvitation, updateBoard, updateCard, updateCard:closed, updateCard:desc, updateCard:idList, updateCard:name, updateCheckItemStateOnCard, updateChecklist, updateList, updateList:closed, updateList:name, updateMember or updateOrganization
- actionsEntities string? (default ()) - True or false
- actionsDisplay string? (default ()) - True or false
- actionsLimit string (default "50") - A number from 0 to 1000
- actionFields string (default "all") - All or a comma-separated list of: data, date, idMemberCreator or type
- actionSince string? (default ()) - A date, null or lastView
- actionBefore string? (default ()) - A date, or null
- cards string (default "none") - One of: all, closed, none, open or visible
- cardFields string (default "all") - All or a comma-separated list of: badges, checkItemStates, closed, dateLastActivity, desc, descData, due, email, idAttachmentCover, idBoard, idChecklists, idLabels, idList, idMembers, idMembersVoted, idShort, labels, manualCoverAttachment, name, pos, shortLink, shortUrl, subscribed or url
- cardMembers string? (default ()) - True or false
- cardMemberFields string (default "avatarHash, fullName, initials and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- cardAttachments string? (default ()) - A boolean value or "cover" for only card cover attachments
- cardAttachmentFields string (default "url and previews") - All or a comma-separated list of: bytes, date, edgeColor, idMember, isUpload, mimeType, name, previews or url
- cardStickers string? (default ()) - True or false
- boards string? (default ()) - All or a comma-separated list of: closed, members, open, organization, pinned, public, starred or unpinned
- boardFields string (default "name, closed, idOrganization and pinned") - All or a comma-separated list of: closed, dateLastActivity, dateLastView, desc, descData, idOrganization, invitations, invited, labelNames, memberships, name, pinned, powerUps, prefs, shortLink, shortUrl, starred, subscribed or url
- boardActions string? (default ()) - All or a comma-separated list of: addAttachmentToCard, addChecklistToCard, addMemberToBoard, addMemberToCard, addMemberToOrganization, addToOrganizationBoard, commentCard, convertToCardFromCheckItem, copyBoard, copyCard, copyCommentCard, createBoard, createCard, createList, createOrganization, deleteAttachmentFromCard, deleteBoardInvitation, deleteCard, deleteOrganizationInvitation, disablePowerUp, emailCard, enablePowerUp, makeAdminOfBoard, makeNormalMemberOfBoard, makeNormalMemberOfOrganization, makeObserverOfBoard, memberJoinedTrello, moveCardFromBoard, moveCardToBoard, moveListFromBoard, moveListToBoard, removeChecklistFromCard, removeFromOrganizationBoard, removeMemberFromCard, unconfirmedBoardInvitation, unconfirmedOrganizationInvitation, updateBoard, updateCard, updateCard:closed, updateCard:desc, updateCard:idList, updateCard:name, updateCheckItemStateOnCard, updateChecklist, updateList, updateList:closed, updateList:name, updateMember or updateOrganization
- boardActionsEntities string? (default ()) - True or false
- boardActionsDisplay string? (default ()) - True or false
- boardActionsFormat string (default "list") - One of: count, list or minimal
- boardActionsSince string? (default ()) - A date, null or lastView
- boardActionsLimit string (default "50") - A number from 0 to 1000
- boardActionFields string (default "all") - All or a comma-separated list of: data, date, idMemberCreator or type
- boardLists string (default "none") - One of: all, closed, none or open
- boardMemberships string (default "none") - All or a comma-separated list of: active, admin, deactivated, me or normal
- boardOrganization string? (default ()) - True or false
- boardOrganizationFields string (default "name and displayName") - All or a comma-separated list of: billableMemberCount, desc, descData, displayName, idBoards, invitations, invited, logoHash, memberships, name, powerUps, prefs, premiumFeatures, products, url or website
- boardsInvited string? (default ()) - All or a comma-separated list of: closed, members, open, organization, pinned, public, starred or unpinned
- boardsinvitedFields string (default "name, closed, idOrganization and pinned") - All or a comma-separated list of: closed, dateLastActivity, dateLastView, desc, descData, idOrganization, invitations, invited, labelNames, memberships, name, pinned, powerUps, prefs, shortLink, shortUrl, starred, subscribed or url
- boardStars string? (default ()) - True or false
- savedSearches string? (default ()) - True or false
- organizations string (default "none") - One of: all, members, none or public
- organizationFields string (default "all") - All or a comma-separated list of: billableMemberCount, desc, descData, displayName, idBoards, invitations, invited, logoHash, memberships, name, powerUps, prefs, premiumFeatures, products, url or website
- organizationPaidAccount string? (default ()) - True or false
- organizationsInvited string (default "none") - One of: all, members, none or public
- organizationsinvitedFields string (default "all") - All or a comma-separated list of: billableMemberCount, desc, descData, displayName, idBoards, invitations, invited, logoHash, memberships, name, powerUps, prefs, premiumFeatures, products, url or website
- notifications string? (default ()) - All or a comma-separated list of: addAdminToBoard, addAdminToOrganization, addedAttachmentToCard, addedMemberToCard, addedToBoard, addedToCard, addedToOrganization, cardDueSoon, changeCard, closeBoard, commentCard, createdCard, declinedInvitationToBoard, declinedInvitationToOrganization, invitedToBoard, invitedToOrganization, makeAdminOfBoard, makeAdminOfOrganization, memberJoinedTrello, mentionedOnCard, removedFromBoard, removedFromCard, removedFromOrganization, removedMemberFromCard, unconfirmedInvitedToBoard, unconfirmedInvitedToOrganization or updateCheckItemStateOnCard
- notificationsEntities string? (default ()) - True or false
- notificationsDisplay string? (default ()) - True or false
- notificationsLimit string (default "50") - A number from 1 to 1000
- notificationFields string (default "all") - All or a comma-separated list of: data, date, idMemberCreator, type or unread
- notificationMembercreator string? (default ()) - True or false
- notificationMembercreatorFields string (default "avatarHash, fullName, initials and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- notificationBefore string? (default ()) - An ID, or null
- notificationSince string? (default ()) - An ID, or null
- tokens string (default "none") - One of: all or none
- paidAccount string? (default ()) - True or false
- boardBackgrounds string (default "none") - One of: all, custom, default, none or premium
- customBoardBackgrounds string (default "none") - One of: all or none
- customStickers string (default "none") - One of: all or none
- customEmoji string (default "none") - One of: all or none
- fields string (default "all") - All or a comma-separated list of: avatarHash, avatarSource, bio, bioData, confirmed, email, fullName, gravatarHash, idBoards, idBoardsPinned, idOrganizations, idPremOrgsAdmin, initials, loginTypes, memberType, oneTimeMessagesDismissed, prefs, premiumFeatures, products, status, status, trophies, uploadedAvatarHash, url or username
updateMembersByIdMember
Update members by ID member
Parameters
- idMember string - IdMember or username
- payload Members - Attributes of "Members" to be updated.
getMembersActionsByIdMember
function getMembersActionsByIdMember(string idMember, string? entities, string? display, string filter, string fields, string 'limit, string format, string? since, string? before, string page, string? idModels, string? member, string memberFields, string? memberCreator, string membercreatorFields) returns Response|error
Get members actions by ID member
Parameters
- idMember string - IdMember or username
- entities string? (default ()) - True or false
- display string? (default ()) - True or false
- filter string (default "all") - All or a comma-separated list of: addAttachmentToCard, addChecklistToCard, addMemberToBoard, addMemberToCard, addMemberToOrganization, addToOrganizationBoard, commentCard, convertToCardFromCheckItem, copyBoard, copyCard, copyCommentCard, createBoard, createCard, createList, createOrganization, deleteAttachmentFromCard, deleteBoardInvitation, deleteCard, deleteOrganizationInvitation, disablePowerUp, emailCard, enablePowerUp, makeAdminOfBoard, makeNormalMemberOfBoard, makeNormalMemberOfOrganization, makeObserverOfBoard, memberJoinedTrello, moveCardFromBoard, moveCardToBoard, moveListFromBoard, moveListToBoard, removeChecklistFromCard, removeFromOrganizationBoard, removeMemberFromCard, unconfirmedBoardInvitation, unconfirmedOrganizationInvitation, updateBoard, updateCard, updateCard:closed, updateCard:desc, updateCard:idList, updateCard:name, updateCheckItemStateOnCard, updateChecklist, updateList, updateList:closed, updateList:name, updateMember or updateOrganization
- fields string (default "all") - All or a comma-separated list of: data, date, idMemberCreator or type
- 'limit string (default "50") - A number from 0 to 1000
- format string (default "list") - One of: count, list or minimal
- since string? (default ()) - A date, null or lastView
- before string? (default ()) - A date, or null
- page string (default "0") - Page * limit must be less than 1000
- idModels string? (default ()) - Only return actions related to these model ids
- member string? (default ()) - True or false
- memberFields string (default "avatarHash, fullName, initials and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- memberCreator string? (default ()) - True or false
- membercreatorFields string (default "avatarHash, fullName, initials and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
addMembersAvatarByIdMember
function addMembersAvatarByIdMember(string idMember, MembersAvatar payload) returns Response|error
Add members avatar by ID member
Parameters
- idMember string - IdMember or username
- payload MembersAvatar - Attributes of "Members Avatar" to be added.
updateMembersAvatarSourceByIdMember
function updateMembersAvatarSourceByIdMember(string idMember, MembersAvatarsource payload) returns Response|error
Update members avatar source by ID member
Parameters
- idMember string - IdMember or username
- payload MembersAvatarsource - Attributes of "Members Avatar Source" to be updated.
updateMembersBioByIdMember
function updateMembersBioByIdMember(string idMember, MembersBio payload) returns Response|error
Update members bio by ID member
Parameters
- idMember string - IdMember or username
- payload MembersBio - Attributes of "Members Bio" to be updated.
getMembersBoardBackgroundsByIdMember
function getMembersBoardBackgroundsByIdMember(string idMember, string filter) returns Response|error
Get members board backgrounds by ID member
Parameters
- idMember string - IdMember or username
- filter string (default "all") - One of: all, custom, default, none or premium
addMembersBoardBackgroundsByIdMember
function addMembersBoardBackgroundsByIdMember(string idMember, MembersBoardbackgrounds payload) returns Response|error
Add members board backgrounds by ID member
Parameters
- idMember string - IdMember or username
- payload MembersBoardbackgrounds - Attributes of "Members Board Backgrounds" to be added.
getMembersBoardBackgroundsByIdMemberByIdBoardBackground
function getMembersBoardBackgroundsByIdMemberByIdBoardBackground(string idMember, string idBoardBackground, string fields) returns Response|error
Get members board backgrounds by ID member by ID board background
Parameters
- idMember string - IdMember or username
- idBoardBackground string - IdBoardBackground
- fields string (default "all") - All or a comma-separated list of: brightness, fullSizeUrl, scaled or tile
updateMembersBoardBackgroundsByIdMemberByIdBoardBackground
function updateMembersBoardBackgroundsByIdMemberByIdBoardBackground(string idMember, string idBoardBackground, MembersBoardbackgrounds payload) returns Response|error
Update members board backgrounds by ID member by ID board background
Parameters
- idMember string - IdMember or username
- idBoardBackground string - IdBoardBackground
- payload MembersBoardbackgrounds - Attributes of "Members Board Backgrounds" to be updated.
deleteMembersBoardBackgroundsByIdMemberByIdBoardBackground
function deleteMembersBoardBackgroundsByIdMemberByIdBoardBackground(string idMember, string idBoardBackground) returns Response|error
Delete members board backgrounds by ID member by ID board background
getMembersBoardStarsByIdMember
Get members board stars by ID member
Parameters
- idMember string - IdMember or username
addMembersBoardStarsByIdMember
function addMembersBoardStarsByIdMember(string idMember, MembersBoardstars payload) returns Response|error
Add members board stars by ID member
Parameters
- idMember string - IdMember or username
- payload MembersBoardstars - Attributes of "Members Board Stars" to be added.
getMembersBoardStarsByIdMemberByIdBoardStar
function getMembersBoardStarsByIdMemberByIdBoardStar(string idMember, string idBoardStar) returns Response|error
Get members board stars by ID member by ID board star
updateMembersBoardStarsByIdMemberByIdBoardStar
function updateMembersBoardStarsByIdMemberByIdBoardStar(string idMember, string idBoardStar, MembersBoardstars payload) returns Response|error
Update members board stars by ID member by ID board star
Parameters
- idMember string - IdMember or username
- idBoardStar string - IdBoardStar
- payload MembersBoardstars - Attributes of "Members Board Stars" to be updated.
deleteMembersBoardStarsByIdMemberByIdBoardStar
function deleteMembersBoardStarsByIdMemberByIdBoardStar(string idMember, string idBoardStar) returns Response|error
Delete members board stars by ID member by ID board star
updateMembersBoardStarsIdBoardByIdMemberByIdBoardStar
function updateMembersBoardStarsIdBoardByIdMemberByIdBoardStar(string idMember, string idBoardStar, MembersBoardstarsIdboard payload) returns Response|error
Update members board stars ID board by ID member by ID board star
Parameters
- idMember string - IdMember or username
- idBoardStar string - IdBoardStar
- payload MembersBoardstarsIdboard - Attributes of "Members Board Stars ID Board" to be updated.
updateMembersBoardStarsPosByIdMemberByIdBoardStar
function updateMembersBoardStarsPosByIdMemberByIdBoardStar(string idMember, string idBoardStar, MembersBoardstarsPos payload) returns Response|error
Update members board stars pos by ID member by ID board star
Parameters
- idMember string - IdMember or username
- idBoardStar string - IdBoardStar
- payload MembersBoardstarsPos - Attributes of "Members Board Stars Pos" to be updated.
getMembersBoardsByIdMember
function getMembersBoardsByIdMember(string idMember, string filter, string fields, string? actions, string? actionsEntities, string actionsLimit, string actionsFormat, string? actionsSince, string actionFields, string memberships, string? organization, string organizationFields, string lists) returns Response|error
Get members boards by ID member
Parameters
- idMember string - IdMember or username
- filter string (default "all") - All or a comma-separated list of: closed, members, open, organization, pinned, public, starred or unpinned
- fields string (default "all") - All or a comma-separated list of: closed, dateLastActivity, dateLastView, desc, descData, idOrganization, invitations, invited, labelNames, memberships, name, pinned, powerUps, prefs, shortLink, shortUrl, starred, subscribed or url
- actions string? (default ()) - All or a comma-separated list of: addAttachmentToCard, addChecklistToCard, addMemberToBoard, addMemberToCard, addMemberToOrganization, addToOrganizationBoard, commentCard, convertToCardFromCheckItem, copyBoard, copyCard, copyCommentCard, createBoard, createCard, createList, createOrganization, deleteAttachmentFromCard, deleteBoardInvitation, deleteCard, deleteOrganizationInvitation, disablePowerUp, emailCard, enablePowerUp, makeAdminOfBoard, makeNormalMemberOfBoard, makeNormalMemberOfOrganization, makeObserverOfBoard, memberJoinedTrello, moveCardFromBoard, moveCardToBoard, moveListFromBoard, moveListToBoard, removeChecklistFromCard, removeFromOrganizationBoard, removeMemberFromCard, unconfirmedBoardInvitation, unconfirmedOrganizationInvitation, updateBoard, updateCard, updateCard:closed, updateCard:desc, updateCard:idList, updateCard:name, updateCheckItemStateOnCard, updateChecklist, updateList, updateList:closed, updateList:name, updateMember or updateOrganization
- actionsEntities string? (default ()) - True or false
- actionsLimit string (default "50") - A number from 0 to 1000
- actionsFormat string (default "list") - One of: count, list or minimal
- actionsSince string? (default ()) - A date, null or lastView
- actionFields string (default "all") - All or a comma-separated list of: data, date, idMemberCreator or type
- memberships string (default "none") - All or a comma-separated list of: active, admin, deactivated, me or normal
- organization string? (default ()) - True or false
- organizationFields string (default "name and displayName") - All or a comma-separated list of: billableMemberCount, desc, descData, displayName, idBoards, invitations, invited, logoHash, memberships, name, powerUps, prefs, premiumFeatures, products, url or website
- lists string (default "none") - One of: all, closed, none or open
getMembersBoardsByIdMemberByFilter
Get members boards by ID member by filter
getMembersBoardsInvitedByIdMember
Get members boards invited by ID member
Parameters
- idMember string - IdMember or username
- fields string (default "all") - All or a comma-separated list of: closed, dateLastActivity, dateLastView, desc, descData, idOrganization, invitations, invited, labelNames, memberships, name, pinned, powerUps, prefs, shortLink, shortUrl, starred, subscribed or url
getMembersBoardsInvitedByIdMemberByField
function getMembersBoardsInvitedByIdMemberByField(string idMember, string 'field) returns Response|error
Get members boards invited by ID member by field
getMembersCardsByIdMember
function getMembersCardsByIdMember(string idMember, string? actions, string? attachments, string attachmentFields, string? stickers, string? members, string memberFields, string? checkItemStates, string checklists, string? 'limit, string? since, string? before, string filter, string fields) returns Response|error
Get members cards by ID member
Parameters
- idMember string - IdMember or username
- actions string? (default ()) - All or a comma-separated list of: addAttachmentToCard, addChecklistToCard, addMemberToBoard, addMemberToCard, addMemberToOrganization, addToOrganizationBoard, commentCard, convertToCardFromCheckItem, copyBoard, copyCard, copyCommentCard, createBoard, createCard, createList, createOrganization, deleteAttachmentFromCard, deleteBoardInvitation, deleteCard, deleteOrganizationInvitation, disablePowerUp, emailCard, enablePowerUp, makeAdminOfBoard, makeNormalMemberOfBoard, makeNormalMemberOfOrganization, makeObserverOfBoard, memberJoinedTrello, moveCardFromBoard, moveCardToBoard, moveListFromBoard, moveListToBoard, removeChecklistFromCard, removeFromOrganizationBoard, removeMemberFromCard, unconfirmedBoardInvitation, unconfirmedOrganizationInvitation, updateBoard, updateCard, updateCard:closed, updateCard:desc, updateCard:idList, updateCard:name, updateCheckItemStateOnCard, updateChecklist, updateList, updateList:closed, updateList:name, updateMember or updateOrganization
- attachments string? (default ()) - A boolean value or "cover" for only card cover attachments
- attachmentFields string (default "all") - All or a comma-separated list of: bytes, date, edgeColor, idMember, isUpload, mimeType, name, previews or url
- stickers string? (default ()) - True or false
- members string? (default ()) - True or false
- memberFields string (default "avatarHash, fullName, initials and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- checkItemStates string? (default ()) - True or false
- checklists string (default "none") - One of: all or none
- 'limit string? (default ()) - A number from 1 to 1000
- since string? (default ()) - A date, or null
- before string? (default ()) - A date, or null
- filter string (default "visible") - One of: all, closed, none, open or visible
- fields string (default "all") - All or a comma-separated list of: badges, checkItemStates, closed, dateLastActivity, desc, descData, due, email, idAttachmentCover, idBoard, idChecklists, idLabels, idList, idMembers, idMembersVoted, idShort, labels, manualCoverAttachment, name, pos, shortLink, shortUrl, subscribed or url
getMembersCardsByIdMemberByFilter
Get members cards by ID member by filter
getMembersCustomBoardBackgroundsByIdMember
function getMembersCustomBoardBackgroundsByIdMember(string idMember, string filter) returns Response|error
Get members custom board backgrounds by ID member
addMembersCustomBoardBackgroundsByIdMember
function addMembersCustomBoardBackgroundsByIdMember(string idMember, MembersCustomboardbackgrounds payload) returns Response|error
Add members custom board backgrounds by ID member
Parameters
- idMember string - IdMember or username
- payload MembersCustomboardbackgrounds - Attributes of "Members Custom Board Backgrounds" to be added.
getMembersCustomBoardBackgroundsByIdMemberByIdBoardBackground
function getMembersCustomBoardBackgroundsByIdMemberByIdBoardBackground(string idMember, string idBoardBackground, string fields) returns Response|error
Get members custom board backgrounds by ID member by ID board background
Parameters
- idMember string - IdMember or username
- idBoardBackground string - IdBoardBackground
- fields string (default "all") - All or a comma-separated list of: brightness, fullSizeUrl, scaled or tile
updateMembersCustomBoardBackgroundsByIdMemberByIdBoardBackground
function updateMembersCustomBoardBackgroundsByIdMemberByIdBoardBackground(string idMember, string idBoardBackground, MembersCustomboardbackgrounds payload) returns Response|error
Update members custom board backgrounds by ID member by ID board background
Parameters
- idMember string - IdMember or username
- idBoardBackground string - IdBoardBackground
- payload MembersCustomboardbackgrounds - Attributes of "Members Custom Board Backgrounds" to be updated.
deleteMembersCustomBoardBackgroundsByIdMemberByIdBoardBackground
function deleteMembersCustomBoardBackgroundsByIdMemberByIdBoardBackground(string idMember, string idBoardBackground) returns Response|error
Delete members custom board backgrounds by ID member by ID board background
getMembersCustomEmojiByIdMember
Get members custom emoji by ID member
addMembersCustomEmojiByIdMember
function addMembersCustomEmojiByIdMember(string idMember, MembersCustomemoji payload) returns Response|error
Add members custom emoji by ID member
Parameters
- idMember string - IdMember or username
- payload MembersCustomemoji - Attributes of "Members Custom Emoji" to be added.
getMembersCustomEmojiByIdMemberByIdCustomEmoji
function getMembersCustomEmojiByIdMemberByIdCustomEmoji(string idMember, string idCustomEmoji, string fields) returns Response|error
Get members custom emoji by ID member by ID custom emoji
Parameters
- idMember string - IdMember or username
- idCustomEmoji string - IdCustomEmoji
- fields string (default "all") - All or a comma-separated list of: name or url
getMembersCustomStickersByIdMember
Get members custom stickers by ID member
addMembersCustomStickersByIdMember
function addMembersCustomStickersByIdMember(string idMember, MembersCustomstickers payload) returns Response|error
Add members custom stickers by ID member
Parameters
- idMember string - IdMember or username
- payload MembersCustomstickers - Attributes of "Members Custom Stickers" to be added.
getMembersCustomStickersByIdMemberByIdCustomSticker
function getMembersCustomStickersByIdMemberByIdCustomSticker(string idMember, string idCustomSticker, string fields) returns Response|error
Get members custom stickers by ID member by ID custom sticker
Parameters
- idMember string - IdMember or username
- idCustomSticker string - IdCustomSticker
- fields string (default "all") - All or a comma-separated list of: scaled or url
deleteMembersCustomStickersByIdMemberByIdCustomSticker
function deleteMembersCustomStickersByIdMemberByIdCustomSticker(string idMember, string idCustomSticker) returns Response|error
Delete members custom stickers by ID member by ID custom sticker
getMembersDeltasByIdMember
function getMembersDeltasByIdMember(string idMember, string tags, string ixLastUpdate) returns Response|error
Get members deltas by ID member
Parameters
- idMember string - IdMember or username
- tags string - A valid tag for subscribing
- ixLastUpdate string - A number from -1 to Infinity
updateMembersFullNameByIdMember
function updateMembersFullNameByIdMember(string idMember, MembersFullname payload) returns Response|error
Update members full name by ID member
Parameters
- idMember string - IdMember or username
- payload MembersFullname - Attributes of "Members Full Name" to be updated.
updateMembersInitialsByIdMember
function updateMembersInitialsByIdMember(string idMember, MembersInitials payload) returns Response|error
Update members initials by ID member
Parameters
- idMember string - IdMember or username
- payload MembersInitials - Attributes of "Members Initials" to be updated.
getMembersNotificationsByIdMember
function getMembersNotificationsByIdMember(string idMember, string? entities, string? display, string filter, string readFilter, string fields, string 'limit, string page, string? before, string? since, string? memberCreator, string membercreatorFields) returns Response|error
Get members notifications by ID member
Parameters
- idMember string - IdMember or username
- entities string? (default ()) - True or false
- display string? (default ()) - True or false
- filter string (default "all") - All or a comma-separated list of: addAdminToBoard, addAdminToOrganization, addedAttachmentToCard, addedMemberToCard, addedToBoard, addedToCard, addedToOrganization, cardDueSoon, changeCard, closeBoard, commentCard, createdCard, declinedInvitationToBoard, declinedInvitationToOrganization, invitedToBoard, invitedToOrganization, makeAdminOfBoard, makeAdminOfOrganization, memberJoinedTrello, mentionedOnCard, removedFromBoard, removedFromCard, removedFromOrganization, removedMemberFromCard, unconfirmedInvitedToBoard, unconfirmedInvitedToOrganization or updateCheckItemStateOnCard
- readFilter string (default "all") - One of: all, read or unread
- fields string (default "all") - All or a comma-separated list of: data, date, idMemberCreator, type or unread
- 'limit string (default "50") - A number from 1 to 1000
- page string (default "0") - A number from 0 to 100
- before string? (default ()) - An ID, or null
- since string? (default ()) - An ID, or null
- memberCreator string? (default ()) - True or false
- membercreatorFields string (default "avatarHash, fullName, initials and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
getMembersNotificationsByIdMemberByFilter
function getMembersNotificationsByIdMemberByFilter(string idMember, string filter) returns Response|error
Get members notifications by ID member by filter
addMembersOneTimeMessagesDismissedByIdMember
function addMembersOneTimeMessagesDismissedByIdMember(string idMember, MembersOnetimemessagesdismissed payload) returns Response|error
Add members one time messages dismissed by ID member
Parameters
- idMember string - IdMember or username
- payload MembersOnetimemessagesdismissed - Attributes of "Members One Time Messages Dismissed" to be added.
getMembersOrganizationsByIdMember
function getMembersOrganizationsByIdMember(string idMember, string filter, string fields, string? paidAccount) returns Response|error
Get members organizations by ID member
Parameters
- idMember string - IdMember or username
- filter string (default "all") - One of: all, members, none or public
- fields string (default "all") - All or a comma-separated list of: billableMemberCount, desc, descData, displayName, idBoards, invitations, invited, logoHash, memberships, name, powerUps, prefs, premiumFeatures, products, url or website
- paidAccount string? (default ()) - True or false
getMembersOrganizationsByIdMemberByFilter
function getMembersOrganizationsByIdMemberByFilter(string idMember, string filter) returns Response|error
Get members organizations by ID member by filter
getMembersOrganizationsInvitedByIdMember
function getMembersOrganizationsInvitedByIdMember(string idMember, string fields) returns Response|error
Get members organizations invited by ID member
Parameters
- idMember string - IdMember or username
- fields string (default "all") - All or a comma-separated list of: billableMemberCount, desc, descData, displayName, idBoards, invitations, invited, logoHash, memberships, name, powerUps, prefs, premiumFeatures, products, url or website
getMembersOrganizationsInvitedByIdMemberByField
function getMembersOrganizationsInvitedByIdMemberByField(string idMember, string 'field) returns Response|error
Get members organizations invited by ID member by field
updateMembersPrefsColorBlindByIdMember
function updateMembersPrefsColorBlindByIdMember(string idMember, PrefsColorblind payload) returns Response|error
Update members prefs color blind by ID member
Parameters
- idMember string - IdMember or username
- payload PrefsColorblind - Attributes of "Prefs Color Blind" to be updated.
updateMembersPrefsLocaleByIdMember
function updateMembersPrefsLocaleByIdMember(string idMember, PrefsLocale payload) returns Response|error
Update members prefs locale by ID member
Parameters
- idMember string - IdMember or username
- payload PrefsLocale - Attributes of "Prefs Locale" to be updated.
updateMembersPrefsMinutesBetweenSummariesByIdMember
function updateMembersPrefsMinutesBetweenSummariesByIdMember(string idMember, PrefsMinutesbetweensummaries payload) returns Response|error
Update members prefs minutes between summaries by ID member
Parameters
- idMember string - IdMember or username
- payload PrefsMinutesbetweensummaries - Attributes of "Prefs Minutes Between Summaries" to be updated.
getMembersSavedSearchesByIdMember
Get members saved searches by ID member
Parameters
- idMember string - IdMember or username
addMembersSavedSearchesByIdMember
function addMembersSavedSearchesByIdMember(string idMember, MembersSavedsearches payload) returns Response|error
Add members saved searches by ID member
Parameters
- idMember string - IdMember or username
- payload MembersSavedsearches - Attributes of "Members Saved Searches" to be added.
getMembersSavedSearchesByIdMemberByIdSavedSearch
function getMembersSavedSearchesByIdMemberByIdSavedSearch(string idMember, string idSavedSearch) returns Response|error
Get members saved searches by ID member by ID saved search
updateMembersSavedSearchesByIdMemberByIdSavedSearch
function updateMembersSavedSearchesByIdMemberByIdSavedSearch(string idMember, string idSavedSearch, MembersSavedsearches payload) returns Response|error
Update members saved searches by ID member by ID saved search
Parameters
- idMember string - IdMember or username
- idSavedSearch string - IdSavedSearch
- payload MembersSavedsearches - Attributes of "Members Saved Searches" to be updated.
deleteMembersSavedSearchesByIdMemberByIdSavedSearch
function deleteMembersSavedSearchesByIdMemberByIdSavedSearch(string idMember, string idSavedSearch) returns Response|error
Delete members saved searches by ID member by ID saved search
updateMembersSavedSearchesNameByIdMemberByIdSavedSearch
function updateMembersSavedSearchesNameByIdMemberByIdSavedSearch(string idMember, string idSavedSearch, MembersSavedsearchesName payload) returns Response|error
Update members saved searches name by ID member by ID saved search
Parameters
- idMember string - IdMember or username
- idSavedSearch string - IdSavedSearch
- payload MembersSavedsearchesName - Attributes of "Members Saved Searches Name" to be updated.
updateMembersSavedSearchesPosByIdMemberByIdSavedSearch
function updateMembersSavedSearchesPosByIdMemberByIdSavedSearch(string idMember, string idSavedSearch, MembersSavedsearchesPos payload) returns Response|error
Update members saved searches pos by ID member by ID saved search
Parameters
- idMember string - IdMember or username
- idSavedSearch string - IdSavedSearch
- payload MembersSavedsearchesPos - Attributes of "Members Saved Searches Pos" to be updated.
updateMembersSavedSearchesQueryByIdMemberByIdSavedSearch
function updateMembersSavedSearchesQueryByIdMemberByIdSavedSearch(string idMember, string idSavedSearch, MembersSavedsearchesQuery payload) returns Response|error
Update members saved searches query by ID member by ID saved search
Parameters
- idMember string - IdMember or username
- idSavedSearch string - IdSavedSearch
- payload MembersSavedsearchesQuery - Attributes of "Members Saved Searches Query" to be updated.
getMembersTokensByIdMember
Get members tokens by ID member
updateMembersUsernameByIdMember
function updateMembersUsernameByIdMember(string idMember, MembersUsername payload) returns Response|error
Update members username by ID member
Parameters
- idMember string - IdMember or username
- payload MembersUsername - Attributes of "Members Username" to be updated.
getMembersByIdMemberByField
Get members by ID member by field
addNotificationsAllRead
Add notifications all read
getNotificationsByIdNotification
function getNotificationsByIdNotification(string idNotification, string? display, string? entities, string fields, string? memberCreator, string membercreatorFields, string? board, string boardFields, string? list, string? card, string cardFields, string? organization, string organizationFields, string? member, string memberFields) returns Response|error
Get notifications by ID notification
Parameters
- idNotification string - IdNotification
- display string? (default ()) - True or false
- entities string? (default ()) - True or false
- fields string (default "all") - All or a comma-separated list of: data, date, idMemberCreator, type or unread
- memberCreator string? (default ()) - True or false
- membercreatorFields string (default "avatarHash, fullName, initials and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- board string? (default ()) - True or false
- boardFields string (default "name") - All or a comma-separated list of: closed, dateLastActivity, dateLastView, desc, descData, idOrganization, invitations, invited, labelNames, memberships, name, pinned, powerUps, prefs, shortLink, shortUrl, starred, subscribed or url
- list string? (default ()) - True or false
- card string? (default ()) - True or false
- cardFields string (default "name") - All or a comma-separated list of: badges, checkItemStates, closed, dateLastActivity, desc, descData, due, email, idAttachmentCover, idBoard, idChecklists, idLabels, idList, idMembers, idMembersVoted, idShort, labels, manualCoverAttachment, name, pos, shortLink, shortUrl, subscribed or url
- organization string? (default ()) - True or false
- organizationFields string (default "displayName") - All or a comma-separated list of: billableMemberCount, desc, descData, displayName, idBoards, invitations, invited, logoHash, memberships, name, powerUps, prefs, premiumFeatures, products, url or website
- member string? (default ()) - True or false
- memberFields string (default "avatarHash, fullName, initials and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
updateNotificationsByIdNotification
function updateNotificationsByIdNotification(string idNotification, Notifications payload) returns Response|error
Update notifications by ID notification
Parameters
- idNotification string - IdNotification
- payload Notifications - Attributes of "Notifications" to be updated.
getNotificationsBoardByIdNotification
function getNotificationsBoardByIdNotification(string idNotification, string fields) returns Response|error
Get notifications board by ID notification
Parameters
- idNotification string - IdNotification
- fields string (default "all") - All or a comma-separated list of: closed, dateLastActivity, dateLastView, desc, descData, idOrganization, invitations, invited, labelNames, memberships, name, pinned, powerUps, prefs, shortLink, shortUrl, starred, subscribed or url
getNotificationsBoardByIdNotificationByField
function getNotificationsBoardByIdNotificationByField(string idNotification, string 'field) returns Response|error
Get notifications board by ID notification by field
getNotificationsCardByIdNotification
function getNotificationsCardByIdNotification(string idNotification, string fields) returns Response|error
Get notifications card by ID notification
Parameters
- idNotification string - IdNotification
- fields string (default "all") - All or a comma-separated list of: badges, checkItemStates, closed, dateLastActivity, desc, descData, due, email, idAttachmentCover, idBoard, idChecklists, idLabels, idList, idMembers, idMembersVoted, idShort, labels, manualCoverAttachment, name, pos, shortLink, shortUrl, subscribed or url
getNotificationsCardByIdNotificationByField
function getNotificationsCardByIdNotificationByField(string idNotification, string 'field) returns Response|error
Get notifications card by ID notification by field
getNotificationsDisplayByIdNotification
Get notifications display by ID notification
Parameters
- idNotification string - IdNotification
getNotificationsEntitiesByIdNotification
Get notifications entities by ID notification
Parameters
- idNotification string - IdNotification
getNotificationsListByIdNotification
function getNotificationsListByIdNotification(string idNotification, string fields) returns Response|error
Get notifications list by ID notification
Parameters
- idNotification string - IdNotification
- fields string (default "all") - All or a comma-separated list of: closed, idBoard, name, pos or subscribed
getNotificationsListByIdNotificationByField
function getNotificationsListByIdNotificationByField(string idNotification, string 'field) returns Response|error
Get notifications list by ID notification by field
getNotificationsMemberByIdNotification
function getNotificationsMemberByIdNotification(string idNotification, string fields) returns Response|error
Get notifications member by ID notification
Parameters
- idNotification string - IdNotification
- fields string (default "all") - All or a comma-separated list of: avatarHash, avatarSource, bio, bioData, confirmed, email, fullName, gravatarHash, idBoards, idBoardsPinned, idOrganizations, idPremOrgsAdmin, initials, loginTypes, memberType, oneTimeMessagesDismissed, prefs, premiumFeatures, products, status, status, trophies, uploadedAvatarHash, url or username
getNotificationsMemberByIdNotificationByField
function getNotificationsMemberByIdNotificationByField(string idNotification, string 'field) returns Response|error
Get notifications member by ID notification by field
getNotificationsMemberCreatorByIdNotification
function getNotificationsMemberCreatorByIdNotification(string idNotification, string fields) returns Response|error
Get notifications member creator by ID notification
Parameters
- idNotification string - IdNotification
- fields string (default "all") - All or a comma-separated list of: avatarHash, avatarSource, bio, bioData, confirmed, email, fullName, gravatarHash, idBoards, idBoardsPinned, idOrganizations, idPremOrgsAdmin, initials, loginTypes, memberType, oneTimeMessagesDismissed, prefs, premiumFeatures, products, status, status, trophies, uploadedAvatarHash, url or username
getNotificationsMemberCreatorByIdNotificationByField
function getNotificationsMemberCreatorByIdNotificationByField(string idNotification, string 'field) returns Response|error
Get notifications member creator by ID notification by field
getNotificationsOrganizationByIdNotification
function getNotificationsOrganizationByIdNotification(string idNotification, string fields) returns Response|error
Get notifications organization by ID notification
Parameters
- idNotification string - IdNotification
- fields string (default "all") - All or a comma-separated list of: billableMemberCount, desc, descData, displayName, idBoards, invitations, invited, logoHash, memberships, name, powerUps, prefs, premiumFeatures, products, url or website
getNotificationsOrganizationByIdNotificationByField
function getNotificationsOrganizationByIdNotificationByField(string idNotification, string 'field) returns Response|error
Get notifications organization by ID notification by field
updateNotificationsUnreadByIdNotification
function updateNotificationsUnreadByIdNotification(string idNotification, NotificationsUnread payload) returns Response|error
Update notifications unread by ID notification
Parameters
- idNotification string - IdNotification
- payload NotificationsUnread - Attributes of "Notifications Unread" to be updated.
getNotificationsByIdNotificationByField
function getNotificationsByIdNotificationByField(string idNotification, string 'field) returns Response|error
Get notifications by ID notification by field
addOrganizations
function addOrganizations(Organizations payload) returns Response|error
Add organizations
Parameters
- payload Organizations - Attributes of "Organizations" to be added.
getOrganizationsByIdOrg
function getOrganizationsByIdOrg(string idOrg, string? actions, string? actionsEntities, string? actionsDisplay, string actionsLimit, string actionFields, string memberships, string? membershipsMember, string membershipsMemberFields, string members, string memberFields, string? memberActivity, string membersInvited, string membersinvitedFields, string boards, string boardFields, string? boardActions, string? boardActionsEntities, string? boardActionsDisplay, string boardActionsFormat, string? boardActionsSince, string boardActionsLimit, string boardActionFields, string boardLists, string? paidAccount, string fields) returns Response|error
Get organizations by ID org
Parameters
- idOrg string - IdOrg or name
- actions string? (default ()) - All or a comma-separated list of: addAttachmentToCard, addChecklistToCard, addMemberToBoard, addMemberToCard, addMemberToOrganization, addToOrganizationBoard, commentCard, convertToCardFromCheckItem, copyBoard, copyCard, copyCommentCard, createBoard, createCard, createList, createOrganization, deleteAttachmentFromCard, deleteBoardInvitation, deleteCard, deleteOrganizationInvitation, disablePowerUp, emailCard, enablePowerUp, makeAdminOfBoard, makeNormalMemberOfBoard, makeNormalMemberOfOrganization, makeObserverOfBoard, memberJoinedTrello, moveCardFromBoard, moveCardToBoard, moveListFromBoard, moveListToBoard, removeChecklistFromCard, removeFromOrganizationBoard, removeMemberFromCard, unconfirmedBoardInvitation, unconfirmedOrganizationInvitation, updateBoard, updateCard, updateCard:closed, updateCard:desc, updateCard:idList, updateCard:name, updateCheckItemStateOnCard, updateChecklist, updateList, updateList:closed, updateList:name, updateMember or updateOrganization
- actionsEntities string? (default ()) - True or false
- actionsDisplay string? (default ()) - True or false
- actionsLimit string (default "50") - A number from 0 to 1000
- actionFields string (default "all") - All or a comma-separated list of: data, date, idMemberCreator or type
- memberships string (default "none") - All or a comma-separated list of: active, admin, deactivated, me or normal
- membershipsMember string? (default ()) - True or false
- membershipsMemberFields string (default "fullName and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- members string (default "none") - One of: admins, all, none, normal or owners
- memberFields string (default "avatarHash, fullName, initials, username and confirmed") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- memberActivity string? (default ()) - True or false ; works for premium organizations only.
- membersInvited string (default "none") - One of: admins, all, none, normal or owners
- membersinvitedFields string (default "avatarHash, initials, fullName and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- boards string (default "none") - All or a comma-separated list of: closed, members, open, organization, pinned, public, starred or unpinned
- boardFields string (default "all") - All or a comma-separated list of: closed, dateLastActivity, dateLastView, desc, descData, idOrganization, invitations, invited, labelNames, memberships, name, pinned, powerUps, prefs, shortLink, shortUrl, starred, subscribed or url
- boardActions string? (default ()) - All or a comma-separated list of: addAttachmentToCard, addChecklistToCard, addMemberToBoard, addMemberToCard, addMemberToOrganization, addToOrganizationBoard, commentCard, convertToCardFromCheckItem, copyBoard, copyCard, copyCommentCard, createBoard, createCard, createList, createOrganization, deleteAttachmentFromCard, deleteBoardInvitation, deleteCard, deleteOrganizationInvitation, disablePowerUp, emailCard, enablePowerUp, makeAdminOfBoard, makeNormalMemberOfBoard, makeNormalMemberOfOrganization, makeObserverOfBoard, memberJoinedTrello, moveCardFromBoard, moveCardToBoard, moveListFromBoard, moveListToBoard, removeChecklistFromCard, removeFromOrganizationBoard, removeMemberFromCard, unconfirmedBoardInvitation, unconfirmedOrganizationInvitation, updateBoard, updateCard, updateCard:closed, updateCard:desc, updateCard:idList, updateCard:name, updateCheckItemStateOnCard, updateChecklist, updateList, updateList:closed, updateList:name, updateMember or updateOrganization
- boardActionsEntities string? (default ()) - True or false
- boardActionsDisplay string? (default ()) - True or false
- boardActionsFormat string (default "list") - One of: count, list or minimal
- boardActionsSince string? (default ()) - A date, null or lastView
- boardActionsLimit string (default "50") - A number from 0 to 1000
- boardActionFields string (default "all") - All or a comma-separated list of: data, date, idMemberCreator or type
- boardLists string (default "none") - One of: all, closed, none or open
- paidAccount string? (default ()) - True or false
- fields string (default "name, displayName, desc, descData, url, website, logoHash, products and powerUps") - All or a comma-separated list of: billableMemberCount, desc, descData, displayName, idBoards, invitations, invited, logoHash, memberships, name, powerUps, prefs, premiumFeatures, products, url or website
updateOrganizationsByIdOrg
function updateOrganizationsByIdOrg(string idOrg, Organizations payload) returns Response|error
Update organizations by ID org
Parameters
- idOrg string - IdOrg or name
- payload Organizations - Attributes of "Organizations" to be updated.
deleteOrganizationsByIdOrg
Delete organizations by ID org
Parameters
- idOrg string - IdOrg or name
getOrganizationsActionsByIdOrg
function getOrganizationsActionsByIdOrg(string idOrg, string? entities, string? display, string filter, string fields, string 'limit, string format, string? since, string? before, string page, string? idModels, string? member, string memberFields, string? memberCreator, string membercreatorFields) returns Response|error
Get organizations actions by ID org
Parameters
- idOrg string - IdOrg or name
- entities string? (default ()) - True or false
- display string? (default ()) - True or false
- filter string (default "all") - All or a comma-separated list of: addAttachmentToCard, addChecklistToCard, addMemberToBoard, addMemberToCard, addMemberToOrganization, addToOrganizationBoard, commentCard, convertToCardFromCheckItem, copyBoard, copyCard, copyCommentCard, createBoard, createCard, createList, createOrganization, deleteAttachmentFromCard, deleteBoardInvitation, deleteCard, deleteOrganizationInvitation, disablePowerUp, emailCard, enablePowerUp, makeAdminOfBoard, makeNormalMemberOfBoard, makeNormalMemberOfOrganization, makeObserverOfBoard, memberJoinedTrello, moveCardFromBoard, moveCardToBoard, moveListFromBoard, moveListToBoard, removeChecklistFromCard, removeFromOrganizationBoard, removeMemberFromCard, unconfirmedBoardInvitation, unconfirmedOrganizationInvitation, updateBoard, updateCard, updateCard:closed, updateCard:desc, updateCard:idList, updateCard:name, updateCheckItemStateOnCard, updateChecklist, updateList, updateList:closed, updateList:name, updateMember or updateOrganization
- fields string (default "all") - All or a comma-separated list of: data, date, idMemberCreator or type
- 'limit string (default "50") - A number from 0 to 1000
- format string (default "list") - One of: count, list or minimal
- since string? (default ()) - A date, null or lastView
- before string? (default ()) - A date, or null
- page string (default "0") - Page * limit must be less than 1000
- idModels string? (default ()) - Only return actions related to these model ids
- member string? (default ()) - True or false
- memberFields string (default "avatarHash, fullName, initials and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- memberCreator string? (default ()) - True or false
- membercreatorFields string (default "avatarHash, fullName, initials and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
getOrganizationsBoardsByIdOrg
function getOrganizationsBoardsByIdOrg(string idOrg, string filter, string fields, string? actions, string? actionsEntities, string actionsLimit, string actionsFormat, string? actionsSince, string actionFields, string memberships, string? organization, string organizationFields, string lists) returns Response|error
Get organizations boards by ID org
Parameters
- idOrg string - IdOrg or name
- filter string (default "all") - All or a comma-separated list of: closed, members, open, organization, pinned, public, starred or unpinned
- fields string (default "all") - All or a comma-separated list of: closed, dateLastActivity, dateLastView, desc, descData, idOrganization, invitations, invited, labelNames, memberships, name, pinned, powerUps, prefs, shortLink, shortUrl, starred, subscribed or url
- actions string? (default ()) - All or a comma-separated list of: addAttachmentToCard, addChecklistToCard, addMemberToBoard, addMemberToCard, addMemberToOrganization, addToOrganizationBoard, commentCard, convertToCardFromCheckItem, copyBoard, copyCard, copyCommentCard, createBoard, createCard, createList, createOrganization, deleteAttachmentFromCard, deleteBoardInvitation, deleteCard, deleteOrganizationInvitation, disablePowerUp, emailCard, enablePowerUp, makeAdminOfBoard, makeNormalMemberOfBoard, makeNormalMemberOfOrganization, makeObserverOfBoard, memberJoinedTrello, moveCardFromBoard, moveCardToBoard, moveListFromBoard, moveListToBoard, removeChecklistFromCard, removeFromOrganizationBoard, removeMemberFromCard, unconfirmedBoardInvitation, unconfirmedOrganizationInvitation, updateBoard, updateCard, updateCard:closed, updateCard:desc, updateCard:idList, updateCard:name, updateCheckItemStateOnCard, updateChecklist, updateList, updateList:closed, updateList:name, updateMember or updateOrganization
- actionsEntities string? (default ()) - True or false
- actionsLimit string (default "50") - A number from 0 to 1000
- actionsFormat string (default "list") - One of: count, list or minimal
- actionsSince string? (default ()) - A date, null or lastView
- actionFields string (default "all") - All or a comma-separated list of: data, date, idMemberCreator or type
- memberships string (default "none") - All or a comma-separated list of: active, admin, deactivated, me or normal
- organization string? (default ()) - True or false
- organizationFields string (default "name and displayName") - All or a comma-separated list of: billableMemberCount, desc, descData, displayName, idBoards, invitations, invited, logoHash, memberships, name, powerUps, prefs, premiumFeatures, products, url or website
- lists string (default "none") - One of: all, closed, none or open
getOrganizationsBoardsByIdOrgByFilter
Get organizations boards by ID org by filter
getOrganizationsDeltasByIdOrg
function getOrganizationsDeltasByIdOrg(string idOrg, string tags, string ixLastUpdate) returns Response|error
Get organizations deltas by ID org
Parameters
- idOrg string - IdOrg or name
- tags string - A valid tag for subscribing
- ixLastUpdate string - A number from -1 to Infinity
updateOrganizationsDescByIdOrg
function updateOrganizationsDescByIdOrg(string idOrg, OrganizationsDesc payload) returns Response|error
Update organizations desc by ID org
Parameters
- idOrg string - IdOrg or name
- payload OrganizationsDesc - Attributes of "Organizations Desc" to be updated.
updateOrganizationsDisplayNameByIdOrg
function updateOrganizationsDisplayNameByIdOrg(string idOrg, OrganizationsDisplayname payload) returns Response|error
Update organizations display name by ID org
Parameters
- idOrg string - IdOrg or name
- payload OrganizationsDisplayname - Attributes of "Organizations Display Name" to be updated.
addOrganizationsLogoByIdOrg
function addOrganizationsLogoByIdOrg(string idOrg, OrganizationsLogo payload) returns Response|error
Add organizations logo by ID org
Parameters
- idOrg string - IdOrg or name
- payload OrganizationsLogo - Attributes of "Organizations Logo" to be added.
deleteOrganizationsLogoByIdOrg
Delete organizations logo by ID org
Parameters
- idOrg string - IdOrg or name
getOrganizationsMembersByIdOrg
function getOrganizationsMembersByIdOrg(string idOrg, string filter, string fields, string? activity) returns Response|error
Get organizations members by ID org
Parameters
- idOrg string - IdOrg or name
- filter string (default "all") - One of: admins, all, none, normal or owners
- fields string (default "fullName and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- activity string? (default ()) - True or false ; works for premium organizations only.
updateOrganizationsMembersByIdOrg
function updateOrganizationsMembersByIdOrg(string idOrg, OrganizationsMembers payload) returns Response|error
Update organizations members by ID org
Parameters
- idOrg string - IdOrg or name
- payload OrganizationsMembers - Attributes of "Organizations Members" to be updated.
getOrganizationsMembersByIdOrgByFilter
Get organizations members by ID org by filter
updateOrganizationsMembersByIdOrgByIdMember
function updateOrganizationsMembersByIdOrgByIdMember(string idOrg, string idMember, OrganizationsMembers payload) returns Response|error
Update organizations members by ID org by ID member
Parameters
- idOrg string - IdOrg or name
- idMember string - IdMember
- payload OrganizationsMembers - Attributes of "Organizations Members" to be updated.
deleteOrganizationsMembersByIdOrgByIdMember
function deleteOrganizationsMembersByIdOrgByIdMember(string idOrg, string idMember) returns Response|error
Delete organizations members by ID org by ID member
deleteOrganizationsMembersAllByIdOrgByIdMember
function deleteOrganizationsMembersAllByIdOrgByIdMember(string idOrg, string idMember) returns Response|error
Delete organizations members all by ID org by ID member
getOrganizationsMembersCardsByIdOrgByIdMember
function getOrganizationsMembersCardsByIdOrgByIdMember(string idOrg, string idMember, string? actions, string? attachments, string attachmentFields, string? members, string memberFields, string? checkItemStates, string checklists, string? board, string boardFields, string? list, string listFields, string filter, string fields) returns Response|error
Get organizations members cards by ID org by ID member
Parameters
- idOrg string - IdOrg or name
- idMember string - IdMember
- actions string? (default ()) - All or a comma-separated list of: addAttachmentToCard, addChecklistToCard, addMemberToBoard, addMemberToCard, addMemberToOrganization, addToOrganizationBoard, commentCard, convertToCardFromCheckItem, copyBoard, copyCard, copyCommentCard, createBoard, createCard, createList, createOrganization, deleteAttachmentFromCard, deleteBoardInvitation, deleteCard, deleteOrganizationInvitation, disablePowerUp, emailCard, enablePowerUp, makeAdminOfBoard, makeNormalMemberOfBoard, makeNormalMemberOfOrganization, makeObserverOfBoard, memberJoinedTrello, moveCardFromBoard, moveCardToBoard, moveListFromBoard, moveListToBoard, removeChecklistFromCard, removeFromOrganizationBoard, removeMemberFromCard, unconfirmedBoardInvitation, unconfirmedOrganizationInvitation, updateBoard, updateCard, updateCard:closed, updateCard:desc, updateCard:idList, updateCard:name, updateCheckItemStateOnCard, updateChecklist, updateList, updateList:closed, updateList:name, updateMember or updateOrganization
- attachments string? (default ()) - A boolean value or "cover" for only card cover attachments
- attachmentFields string (default "all") - All or a comma-separated list of: bytes, date, edgeColor, idMember, isUpload, mimeType, name, previews or url
- members string? (default ()) - True or false
- memberFields string (default "avatarHash, fullName, initials and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- checkItemStates string? (default ()) - True or false
- checklists string (default "none") - One of: all or none
- board string? (default ()) - True or false
- boardFields string (default "name, desc, closed, idOrganization, pinned, url and prefs") - All or a comma-separated list of: closed, dateLastActivity, dateLastView, desc, descData, idOrganization, invitations, invited, labelNames, memberships, name, pinned, powerUps, prefs, shortLink, shortUrl, starred, subscribed or url
- list string? (default ()) - True or false
- listFields string (default "all") - All or a comma-separated list of: closed, idBoard, name, pos or subscribed
- filter string (default "visible") - One of: all, closed, none, open or visible
- fields string (default "all") - All or a comma-separated list of: badges, checkItemStates, closed, dateLastActivity, desc, descData, due, email, idAttachmentCover, idBoard, idChecklists, idLabels, idList, idMembers, idMembersVoted, idShort, labels, manualCoverAttachment, name, pos, shortLink, shortUrl, subscribed or url
updateOrganizationsMembersDeactivatedByIdOrgByIdMember
function updateOrganizationsMembersDeactivatedByIdOrgByIdMember(string idOrg, string idMember, OrganizationsMembersDeactivated payload) returns Response|error
Update organizations members deactivated by ID org by ID member
Parameters
- idOrg string - IdOrg or name
- idMember string - IdMember
- payload OrganizationsMembersDeactivated - Attributes of "Organizations Members Deactivated" to be updated.
getOrganizationsMembersInvitedByIdOrg
Get organizations members invited by ID org
Parameters
- idOrg string - IdOrg or name
- fields string (default "all") - All or a comma-separated list of: avatarHash, avatarSource, bio, bioData, confirmed, email, fullName, gravatarHash, idBoards, idBoardsPinned, idOrganizations, idPremOrgsAdmin, initials, loginTypes, memberType, oneTimeMessagesDismissed, prefs, premiumFeatures, products, status, status, trophies, uploadedAvatarHash, url or username
getOrganizationsMembersInvitedByIdOrgByField
function getOrganizationsMembersInvitedByIdOrgByField(string idOrg, string 'field) returns Response|error
Get organizations members invited by ID org by field
getOrganizationsMembershipsByIdOrg
function getOrganizationsMembershipsByIdOrg(string idOrg, string filter, string? member, string memberFields) returns Response|error
Get organizations memberships by ID org
Parameters
- idOrg string - IdOrg or name
- filter string (default "all") - All or a comma-separated list of: active, admin, deactivated, me or normal
- member string? (default ()) - True or false
- memberFields string (default "fullName and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
getOrganizationsMembershipsByIdOrgByIdMembership
function getOrganizationsMembershipsByIdOrgByIdMembership(string idOrg, string idMembership, string? member, string memberFields) returns Response|error
Get organizations memberships by ID org by ID membership
Parameters
- idOrg string - IdOrg or name
- idMembership string - IdMembership
- member string? (default ()) - True or false
- memberFields string (default "fullName and username") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
updateOrganizationsMembershipsByIdOrgByIdMembership
function updateOrganizationsMembershipsByIdOrgByIdMembership(string idOrg, string idMembership, OrganizationsMemberships payload) returns Response|error
Update organizations memberships by ID org by ID membership
Parameters
- idOrg string - IdOrg or name
- idMembership string - IdMembership
- payload OrganizationsMemberships - Attributes of "Organizations Memberships" to be updated.
updateOrganizationsNameByIdOrg
function updateOrganizationsNameByIdOrg(string idOrg, OrganizationsName payload) returns Response|error
Update organizations name by ID org
Parameters
- idOrg string - IdOrg or name
- payload OrganizationsName - Attributes of "Organizations Name" to be updated.
updateOrganizationsPrefsAssociatedDomainByIdOrg
function updateOrganizationsPrefsAssociatedDomainByIdOrg(string idOrg, PrefsAssociateddomain payload) returns Response|error
Update organizations prefs associated domain by ID org
Parameters
- idOrg string - IdOrg or name
- payload PrefsAssociateddomain - Attributes of "Prefs Associated Domain" to be updated.
deleteOrganizationsPrefsAssociatedDomainByIdOrg
Delete organizations prefs associated domain by ID org
Parameters
- idOrg string - IdOrg or name
updateOrganizationsPrefsBoardVisibilityRestrictOrgByIdOrg
function updateOrganizationsPrefsBoardVisibilityRestrictOrgByIdOrg(string idOrg, PrefsBoardvisibilityrestrict payload) returns Response|error
Update organizations prefs board visibility restrict org by ID org
Parameters
- idOrg string - IdOrg or name
- payload PrefsBoardvisibilityrestrict - Attributes of "Prefs Board Visibility Restrict" to be updated.
updateOrganizationsPrefsBoardVisibilityRestrictPrivateByIdOrg
function updateOrganizationsPrefsBoardVisibilityRestrictPrivateByIdOrg(string idOrg, PrefsBoardvisibilityrestrict payload) returns Response|error
Update organizations prefs board visibility restrict private by ID org
Parameters
- idOrg string - IdOrg or name
- payload PrefsBoardvisibilityrestrict - Attributes of "Prefs Board Visibility Restrict" to be updated.
updateOrganizationsPrefsBoardVisibilityRestrictPublicByIdOrg
function updateOrganizationsPrefsBoardVisibilityRestrictPublicByIdOrg(string idOrg, PrefsBoardvisibilityrestrict payload) returns Response|error
Update organizations prefs board visibility restrict public by ID org
Parameters
- idOrg string - IdOrg or name
- payload PrefsBoardvisibilityrestrict - Attributes of "Prefs Board Visibility Restrict" to be updated.
updateOrganizationsPrefsExternalMembersDisabledByIdOrg
function updateOrganizationsPrefsExternalMembersDisabledByIdOrg(string idOrg, PrefsExternalmembersdisabled payload) returns Response|error
Update organizations prefs external members disabled by ID org
Parameters
- idOrg string - IdOrg or name
- payload PrefsExternalmembersdisabled - Attributes of "Prefs External Members Disabled" to be updated.
updateOrganizationsPrefsGoogleAppsVersionByIdOrg
function updateOrganizationsPrefsGoogleAppsVersionByIdOrg(string idOrg, PrefsGoogleappsversion payload) returns Response|error
Update organizations prefs google apps version by ID org
Parameters
- idOrg string - IdOrg or name
- payload PrefsGoogleappsversion - Attributes of "Prefs Google Apps Version" to be updated.
updateOrganizationsPrefsOrgInviteRestrictByIdOrg
function updateOrganizationsPrefsOrgInviteRestrictByIdOrg(string idOrg, PrefsOrginviterestrict payload) returns Response|error
Update organizations prefs org invite restrict by ID org
Parameters
- idOrg string - IdOrg or name
- payload PrefsOrginviterestrict - Attributes of "Prefs Org Invite Restrict" to be updated.
deleteOrganizationsPrefsOrgInviteRestrictByIdOrg
function deleteOrganizationsPrefsOrgInviteRestrictByIdOrg(string idOrg, string value) returns Response|error
Delete organizations prefs org invite restrict by ID org
Parameters
- idOrg string - IdOrg or name
- value string - An email address with optional expansion tokens
updateOrganizationsPrefsPermissionLevelByIdOrg
function updateOrganizationsPrefsPermissionLevelByIdOrg(string idOrg, PrefsPermissionlevel payload) returns Response|error
Update organizations prefs permission level by ID org
Parameters
- idOrg string - IdOrg or name
- payload PrefsPermissionlevel - Attributes of "Prefs Permission Level" to be updated.
updateOrganizationsWebsiteByIdOrg
function updateOrganizationsWebsiteByIdOrg(string idOrg, OrganizationsWebsite payload) returns Response|error
Update organizations website by ID org
Parameters
- idOrg string - IdOrg or name
- payload OrganizationsWebsite - Attributes of "Organizations Website" to be updated.
getOrganizationsByIdOrgByField
Get organizations by ID org by field
getSearch
function getSearch(string query, string idOrganizations, string idBoards, string? idCards, string modelTypes, string boardFields, string boardsLimit, string cardFields, string cardsLimit, string cardsPage, string? cardBoard, string? cardList, string? cardMembers, string? cardStickers, string? cardAttachments, string organizationFields, string organizationsLimit, string memberFields, string membersLimit, string? partial) returns Response|error
Get search
Parameters
- query string - A string with a length from 1 to 16384
- idOrganizations string - A comma-separated list of objectIds, 24-character hex strings
- idBoards string (default "mine") - A comma-separated list of objectIds, 24-character hex strings
- idCards string? (default ()) - A comma-separated list of objectIds, 24-character hex strings
- modelTypes string (default "all") - All or a comma-separated list of: actions, boards, cards, members or organizations
- boardFields string (default "name and idOrganization") - All or a comma-separated list of: closed, dateLastActivity, dateLastView, desc, descData, idOrganization, invitations, invited, labelNames, memberships, name, pinned, powerUps, prefs, shortLink, shortUrl, starred, subscribed or url
- boardsLimit string (default "10") - A number from 1 to 1000
- cardFields string (default "all") - All or a comma-separated list of: badges, checkItemStates, closed, dateLastActivity, desc, descData, due, email, idAttachmentCover, idBoard, idChecklists, idLabels, idList, idMembers, idMembersVoted, idShort, labels, manualCoverAttachment, name, pos, shortLink, shortUrl, subscribed or url
- cardsLimit string (default "10") - A number from 1 to 1000
- cardsPage string (default "0") - A number from 0 to 100
- cardBoard string? (default ()) - True or false
- cardList string? (default ()) - True or false
- cardMembers string? (default ()) - True or false
- cardStickers string? (default ()) - True or false
- cardAttachments string? (default ()) - A boolean value or "cover" for only card cover attachments
- organizationFields string (default "name and displayName") - All or a comma-separated list of: billableMemberCount, desc, descData, displayName, idBoards, invitations, invited, logoHash, memberships, name, powerUps, prefs, premiumFeatures, products, url or website
- organizationsLimit string (default "10") - A number from 1 to 1000
- memberFields string (default "avatarHash, fullName, initials, username and confirmed") - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- membersLimit string (default "10") - A number from 1 to 1000
- partial string? (default ()) - True or false
getSearchMembers
function getSearchMembers(string query, string 'limit, string? idBoard, string? idOrganization, string? onlyOrgMembers) returns Response|error
Get search members
Parameters
- query string - A string with a length from 1 to 16384
- 'limit string (default "8") - A number from 1 to 20
- idBoard string? (default ()) - An ID, or null
- idOrganization string? (default ()) - An ID, or null
- onlyOrgMembers string? (default ()) - A boolean
addSessions
Add sessions
Parameters
- payload Sessions - Attributes of "Sessions" to be added.
getSessionsSocket
Get sessions socket
updateSessionsByIdSession
Update sessions by ID session
updateSessionsStatusByIdSession
function updateSessionsStatusByIdSession(string idSession, SessionsStatus payload) returns Response|error
Update sessions status by ID session
Parameters
- idSession string - IdSession
- payload SessionsStatus - Attributes of "Sessions Status" to be updated.
getTypesById
Get types by ID
Parameters
- iD string - ID
Records
trello: Actions
Fields
- text string? - A string with a length from 1 to 16384
trello: ActionsComments
Fields
- text string? - A string with a length from 1 to 16384
trello: ActionsText
Fields
- value string? - A string with a length from 1 to 16384
trello: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- 'key string - Represents API Key
key
- token string - Represents API Key
token
trello: Boards
Fields
- closed string? - True or false
- desc string? - A string with a length from 0 to 16384
- idBoardSource string? - The ID of the board to copy into the new board
- idOrganization string? - The ID or name of the organization to add the board to.
- keepFromSource string? - Components of the source board to copy.
- name string? - A string with a length from 1 to 16384
- powerUps string? - All or a comma-separated list of: calendar, cardAging, recap or voting
- prefs_background string? - A string with a length from 0 to 16384
- prefs_cardAging string? - One of: pirate or regular
- prefs_cardCovers string? - True or false
- prefs_comments string? - One of: disabled, members, observers, org or public
- prefs_invitations string? - One of: admins or members
- prefs_permissionLevel string? - One of: org, private or public
- prefs_selfJoin string? - True or false
- prefs_voting string? - One of: disabled, members, observers, org or public
- subscribed string? - True or false
trello: BoardsChecklists
Fields
- name string? - A string with a length from 1 to 16384
trello: BoardsClosed
Fields
- value string? - True or false
trello: BoardsDesc
Fields
- value string? - A string with a length from 0 to 16384
trello: BoardsIdorganization
Fields
- value string? - A string with a length from 0 to 16384
trello: BoardsLabels
Fields
- color string? - A valid label color or null
- name string? - A string with a length from 0 to 16384
trello: BoardsLists
Fields
- name string? - A string with a length from 1 to 16384
- pos string? - A position. top , bottom , or a positive number.
trello: BoardsMembers
Fields
- email string? - An email address
- fullName string? - A string with a length of at least 1. Cannot begin or end with a space.
- 'type string? - One of: admin, normal or observer
trello: BoardsMemberships
Fields
- member_fields string? - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- 'type string? - One of: admin, normal or observer
trello: BoardsName
Fields
- value string? - A string with a length from 1 to 16384
trello: BoardsPowerups
Fields
- value string? - One of: calendar, cardAging, recap or voting
trello: BoardsSubscribed
Fields
- value string? - True or false
trello: Cards
Fields
- closed string? - True or false
- desc string? - A string with a length from 0 to 16384
- due string? - A date, or null
- fileSource string? - A file
- idAttachmentCover string? - ID of the image attachment of this card to use as its cover, or null for no cover
- idBoard string? - ID of the board the card should be moved to
- idCardSource string? - The ID of the card to copy into a new card.
- idLabels string? - A comma-separated list of objectIds, 24-character hex strings
- idList string? - ID of the list that the card should be added to
- idMembers string? - A comma-separated list of objectIds, 24-character hex strings
- keepFromSource string? - Properties of the card to copy over from the source.
- labels string? - All or a comma-separated list of: blue, green, orange, purple, red or yellow
- name string? - The name of the new card. It isn't required if the name is being copied from provided by a URL, file or card that is being copied.
- pos string? - A position. top , bottom , or a positive number.
- subscribed string? - True or false
- urlSource string? - A URL starting with http:// or https:// or null
trello: CardsActionsComments
Fields
- text string? - A string with a length from 1 to 16384
trello: CardsAttachments
Fields
- file string? - A file
- mimeType string? - A string with a length from 0 to 256
- name string? - A string with a length from 0 to 256
- url string? - A URL starting with http:// or https:// or null
trello: CardsChecklistCheckitem
Fields
- name string? - A string with a length from 1 to 16384
- pos string? - A position. top , bottom , or a positive number.
trello: CardsChecklistCheckitemName
Fields
- value string? - A string with a length from 1 to 16384
trello: CardsChecklistCheckitemPos
Fields
- value string? - A position. top , bottom , or a positive number.
trello: CardsChecklistCheckitemState
Fields
- value string? - One of: complete, false, incomplete or true
trello: CardsChecklistIdchecklistcurrentCheckitem
Fields
- idChecklist string? - An ID, or null
- name string? - A string with a length from 1 to 16384
- pos string? - A position. top , bottom , or a positive number.
- state string? - One of: complete, false, incomplete or true
trello: CardsChecklists
Fields
- idChecklistSource string? - The ID of the source checklist to copy into a new checklist.
- name string? - A string with a length from 0 to 16384
- value string? - The ID of the checklist to add to the card, or null to create a new one.
trello: CardsClosed
Fields
- value string? - True or false
trello: CardsDesc
Fields
- value string? - A string with a length from 0 to 16384
trello: CardsDue
Fields
- value string? - A date, or null
trello: CardsIdattachmentcover
Fields
- value string? - ID of the image attachment of this card to use as its cover, or null for no cover
trello: CardsIdboard
Fields
- idList string? - ID of the list that the card should be moved to on the new board
- value string? - ID of the board the card should be moved to
trello: CardsIdlabels
Fields
- value string? - The ID of the label to add
trello: CardsIdlist
Fields
- value string? - ID of the list the card should be moved to
trello: CardsIdmembers
Fields
- value string? - The ID of the member to add to the card
trello: CardsLabels
Fields
- color string? - A valid label color or null
- name string? - A string with a length from 0 to 16384
- value string? - All or a comma-separated list of: blue, green, orange, purple, red or yellow
trello: CardsMembersvoted
Fields
- value string? - The ID of the member to vote 'yes' on the card
trello: CardsName
Fields
- value string? - A string with a length from 1 to 16384
trello: CardsPos
Fields
- value string? - A position. top , bottom , or a positive number.
trello: CardsStickers
Fields
- image string? - A string with a length from 0 to 16384
- left string? - Undefined
- rotate string? - Undefined
- top string? - Undefined
- zIndex string? - Valid Z values for stickers, must be an integer
trello: CardsSubscribed
Fields
- value string? - True or false
trello: Checklists
Fields
- idBoard string? - ID of the board that the checklist should be added to
- idCard string? - ID of the card that the checklist should be added to
- idChecklistSource string? - The ID of the source checklist to copy into a new checklist.
- name string? - A string with a length from 0 to 16384
- pos string? - A position. top , bottom , or a positive number.
trello: ChecklistsCheckitems
Fields
- checked string? - True or false
- name string? - A string with a length from 1 to 16384
- pos string? - A position. top , bottom , or a positive number.
trello: ChecklistsIdcard
Fields
- value string? - The ID of the card that the checklist is on
trello: ChecklistsName
Fields
- value string? - A string with a length from 1 to 16384
trello: ChecklistsPos
Fields
- value string? - A position. top , bottom , or a positive number.
trello: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
trello: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
trello: LabelnamesBlue
Fields
- value string? - A string with a length from 0 to 16384
trello: LabelnamesGreen
Fields
- value string? - A string with a length from 0 to 16384
trello: LabelnamesOrange
Fields
- value string? - A string with a length from 0 to 16384
trello: LabelnamesPurple
Fields
- value string? - A string with a length from 0 to 16384
trello: LabelnamesRed
Fields
- value string? - A string with a length from 0 to 16384
trello: LabelnamesYellow
Fields
- value string? - A string with a length from 0 to 16384
trello: Labels
Fields
- color string? - A valid label color or null
- idBoard string? - An ID
- name string? - A string with a length from 0 to 16384
trello: LabelsColor
Fields
- value string? - A valid label color or null
trello: LabelsName
Fields
- value string? - A string with a length from 0 to 16384
trello: Lists
Fields
- closed string? - True or false
- idBoard string? - ID of the board that the list should be added to
- idListSource string? - The ID of the list to copy into a new list.
- name string? - A string with a length from 1 to 16384
- pos string? - A position. top , bottom , or a positive number.
- subscribed string? - True or false
trello: ListsCards
Fields
- desc string? - A string with a length from 0 to 16384
- due string? - A date, or null
- idMembers string? - A comma-separated list of objectIds, 24-character hex strings
- labels string? - All or a comma-separated list of: blue, green, orange, purple, red or yellow
- name string? - A string with a length from 1 to 16384
trello: ListsClosed
Fields
- value string? - True or false
trello: ListsIdboard
Fields
- pos string? - Position of the list on the new board
- value string? - ID of the board the list should be moved to
trello: ListsMoveallcards
Fields
- idBoard string? - ID of the board that the cards should be moved to
trello: ListsName
Fields
- value string? - A string with a length from 1 to 16384
trello: ListsPos
Fields
- value string? - A position. top , bottom , or a positive number.
trello: ListsSubscribed
Fields
- value string? - True or false
trello: Members
Fields
- avatarSource string? - One of: gravatar, none or upload
- bio string? - A string with a length from 0 to 16384
- fullName string? - A string with a length of at least 1. Cannot begin or end with a space.
- initials string? - A string with a length from 1 to 4. Cannot begin or end with a space
- username string? - A string with a length of at least 3. Only lowercase letters, underscores, and numbers are allowed. Must be unique.
trello: MembersAvatar
Fields
- file string? - A file
trello: MembersAvatarsource
Fields
- value string? - One of: gravatar, none or upload
trello: MembersBio
Fields
- value string? - A string with a length from 0 to 16384
trello: MembersBoardbackgrounds
Fields
- brightness string? - One of: dark, light or unknown
- file string? - A file
- tile string? - True or false
trello: MembersBoardstars
Fields
- idBoard string? - The ID of the board to star
- pos string? - A position. top , bottom , or a positive number.
trello: MembersBoardstarsIdboard
Fields
- value string? - An ID
trello: MembersBoardstarsPos
Fields
- value string? - A position. top , bottom , or a positive number.
trello: MembersCustomboardbackgrounds
Fields
- brightness string? - One of: dark, light or unknown
- file string? - A file
- tile string? - True or false
trello: MembersCustomemoji
Fields
- file string? - A file
- name string? - A string with a length from 2 to 64
trello: MembersCustomstickers
Fields
- file string? - A file
trello: MembersFullname
Fields
- value string? - A string with a length of at least 1. Cannot begin or end with a space.
trello: MembersInitials
Fields
- value string? - A string with a length from 1 to 4. Cannot begin or end with a space
trello: MembersOnetimemessagesdismissed
Fields
- value string? - Type of message dismissed
trello: MembersSavedsearches
Fields
- name string? - A non-empty string with at least one non-space character
- pos string? - A position. top , bottom , or a positive number.
- query string? - A string with a length from 1 to 16384
trello: MembersSavedsearchesName
Fields
- value string? - A non-empty string with at least one non-space character
trello: MembersSavedsearchesPos
Fields
- value string? - A position. top , bottom , or a positive number.
trello: MembersSavedsearchesQuery
Fields
- value string? - A string with a length from 1 to 16384
trello: MembersUsername
Fields
- value string? - A string with a length of at least 3. Only lowercase letters, underscores, and numbers are allowed. Must be unique.
trello: MyprefsEmailposition
Fields
- value string? - One of: bottom or top
trello: MyprefsIdemaillist
Fields
- value string? - An ID
trello: MyprefsShowlistguide
Fields
- value string? - True or false
trello: MyprefsShowsidebar
Fields
- value string? - True or false
trello: MyprefsShowsidebaractivity
Fields
- value string? - True or false
trello: MyprefsShowsidebarboardactions
Fields
- value string? - True or false
trello: MyprefsShowsidebarmembers
Fields
- value string? - True or false
trello: Notifications
Fields
- unread string? - True or false
trello: NotificationsUnread
Fields
- value string? - True or false
trello: Organizations
Fields
- desc string? - A string with a length from 0 to 16384
- displayName string? - A string with a length of at least 1. Cannot begin or end with a space.
- name string? - A string with a length from 0 to 16384
- website string? - A URL starting with http:// or https:// or null
trello: OrganizationsDesc
Fields
- value string? - A string with a length from 0 to 16384
trello: OrganizationsDisplayname
Fields
- value string? - A string with a length of at least 1. Cannot begin or end with a space.
trello: OrganizationsLogo
Fields
- file string? - A file
trello: OrganizationsMembers
Fields
- email string? - An email address
- fullName string? - A string with a length of at least 1. Cannot begin or end with a space.
- 'type string? - One of: admin, normal or observer
trello: OrganizationsMembersDeactivated
Fields
- value string? - True or false
trello: OrganizationsMemberships
Fields
- member_fields string? - All or a comma-separated list of: avatarHash, bio, bioData, confirmed, fullName, idPremOrgsAdmin, initials, memberType, products, status, url or username
- 'type string? - One of: admin, normal or observer
trello: OrganizationsName
Fields
- value string? - A string with a length of at least 3. Only lowercase letters, underscores, and numbers are allowed. Must be unique.
trello: OrganizationsWebsite
Fields
- value string? - A URL starting with http:// or https:// or null
trello: PrefsAssociateddomain
Fields
- value string? - The google apps domain to link this org to.
trello: PrefsBackground
Fields
- value string? - A standard background name, or the ID of a custom background
trello: PrefsBoardvisibilityrestrict
Fields
- value string? - One of: admin, none or org
trello: PrefsCalendarfeedenabled
Fields
- value string? - True or false
trello: PrefsCardaging
Fields
- value string? - One of: pirate or regular
trello: PrefsCardcovers
Fields
- value string? - True or false
trello: PrefsColorblind
Fields
- value string? - True or false
trello: PrefsComments
Fields
- value string? - One of: disabled, members, observers, org or public
trello: PrefsExternalmembersdisabled
Fields
- value string? - True or false
trello: PrefsGoogleappsversion
Fields
- value string? - A number from 1 to 2
trello: PrefsInvitations
Fields
- value string? - One of: admins or members
trello: PrefsLocale
Fields
- value string? - A string with a length from 0 to 255
trello: PrefsMinutesbetweensummaries
Fields
- value string? - -1 (disabled), 1 or 60
trello: PrefsOrginviterestrict
Fields
- value string? - An email address with optional expansion tokens
trello: PrefsPermissionlevel
Fields
- value string? - One of: private or public
trello: PrefsSelfjoin
Fields
- value string? - True or false
trello: PrefsVoting
Fields
- value string? - One of: disabled, members, observers, org or public
trello: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
trello: Sessions
Fields
- idBoard string? - The ID of the board you're viewing. Boards with no viewers will not get updates about members' statuses.
- status string? - One of: active, disconnected or idle
trello: SessionsStatus
Fields
- value string? - One of: active, disconnected or idle
trello: TokensWebhooks
Fields
- callbackURL string? - A valid URL that is reachable with a HEAD request
- description string? - A string with a length from 0 to 16384
- idModel string? - ID of the model to be monitored
trello: Webhooks
Fields
- active string? - True or false
- callbackURL string? - A valid URL that is reachable with a HEAD request
- description string? - A string with a length from 0 to 16384
- idModel string? - ID of the model that should be hooked
trello: WebhooksActive
Fields
- value string? - True or false
trello: WebhooksCallbackurl
Fields
- value string? - A valid URL that is reachable with a HEAD request
trello: WebhooksDescription
Fields
- value string? - A string with a length from 0 to 16384
trello: WebhooksIdmodel
Fields
- value string? - ID of the model to be monitored
Import
import ballerinax/trello;
Metadata
Released date: almost 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 2069
Current verison: 305
Weekly downloads
Keywords
Productivity/Task Management
Cost/Freeemium
Contributors
Dependencies