spotify
Module spotify
API
Definitions
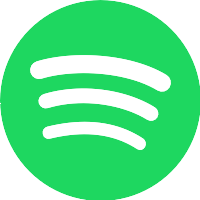
ballerinax/spotify Ballerina library
Overview
This is a generated connector from Spotify API v1 OpenAPI Specification.
Through the Spotify Web API, external applications retrieve Spotify content such as album data and playlists.
Configuring connector
Prerequisites
- Create Spotify user account by simply signing up at www.spotify.com.
- Obtain tokens
- Visit the Dashboard page at the Spotify Developer website and, if necessary, log in. Accept the latest Developer Terms of Service to complete your account set up.
- Follow the steps that are given in [here](Web API authentication visit here) to obtain tokens.
Quickstart
To use the Spotify connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
import ballerinax/spotify;
Step 2: Create a new connector instance
Configure the connection using OAuth2 refresh token grant config.
spotify:Configuration configuration = { auth: { refreshUrl: "https://accounts.spotify.com/api/token", refreshToken : "<REFRESH_TOKEN>", clientId : "<CLIENT_ID>", clientSecret : "<CLIENT_SECRET>" } }; spotify:Client spotifyClient = check new (configuration);
Step 3: Invoke connector operation
- Get list newly released albums in spotify.
public function main() returns error? { spotify:NewReleasesObject newReleases = check spotifyClient->getNewReleses(); }
- Use
bal run
command to compile and run the Ballerina program.
Clients
spotify: Client
This is a generated connector from Spotify API v1 OpenAPI Specification. Through the Spotify Web API, external applications retrieve Spotify content such as album data and playlists.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Please create a Spotify account and obtain tokens following this guide. Configure required scopes when obtaining the tokens.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.spotify.com/v1" - URL of the target service
getMyPlaylists
function getMyPlaylists(int? 'limit, int? offset) returns CurrentPlaylistDetails|error
Get a List of Current User's Playlists
Parameters
- 'limit int? (default ()) - 'The maximum number of playlists to return. Default: 20. Minimum: 1. Maximum: 50.'
- offset int? (default ()) - 'The index of the first playlist to return. Default: 0 (the first object). Maximum offset: 100.000. Use with
limit
to get the next set of playlists.'
Return Type
- CurrentPlaylistDetails|error - On success, the HTTP status code in the response header is
200
OK and the response body contains an array of simplified playlist objects (wrapped in a paging object) in JSON format. On error, the header status code is an error code and the response body contains an error object. Please note that the access token has to be tied to a user.
getPlaylistById
function getPlaylistById(string playlistId, string? market, string? fields, string? additionalTypes) returns PlaylistObject|error
Get a Playlist
Parameters
- playlistId string - The Spotify ID for the playlist.
- market string? (default ()) - An ISO 3166-1 alpha-2 country code or the string
from_token
. Provide this parameter if you want to apply Track Relinking. For episodes, if a valid user access token is specified in the request header, the country associated with the user account will take priority over this parameter. Note: If neither market or user country are provided, the episode is considered unavailable for the client.
- fields string? (default ()) - Filters for the query: a comma-separated list of the fields to return. If omitted, all fields are returned. For example, to get just the playlist''s description and URI:
fields=description,uri
. A dot separator can be used to specify non-reoccurring fields, while parentheses can be used to specify reoccurring fields within objects. For example, to get just the added date and user ID of the adder:fields=tracks.items(added_at,added_by.id)
. Use multiple parentheses to drill down into nested objects, for example:fields=tracks.items(track(name,href,album(name,href)))
. Fields can be excluded by prefixing them with an exclamation mark, for example:fields=tracks.items(track(name,href,album(!name,href)))
- additionalTypes string? (default ()) - A comma-separated list of item types that your client supports besides the default
track
type. Valid types are:track
andepisode
. Note : This parameter was introduced to allow existing clients to maintain their current behaviour and might be deprecated in the future. In addition to providing this parameter, make sure that your client properly handles cases of new types in the future by checking against thetype
field of each object.
Return Type
- PlaylistObject|error - On success, the response body contains a playlist object in JSON format and the HTTP status code in the response header is
200
OK. If an episode is unavailable in the givenmarket
, its information will not be included in the response. On error, the header status code is an error code and the response body contains an error object. Requesting playlists that you do not have the user's authorization to access returns error403
Forbidden.
updatePlaylist
function updatePlaylist(string contentType, string playlistId, ChangePlayListDetails payload) returns Response|error
Change a Playlist's Details
Parameters
- contentType string - The content type of the request body:
application/json
- playlistId string - The Spotify ID for the playlist.
- payload ChangePlayListDetails - Content to update the playlist
Return Type
- Response|error - On success the HTTP status code in the response header is
200
OK. On error, the header status code is an error code and the response body contains an error object. Trying to change a playlist when you do not have the user's authorization returns error403
Forbidden.
getPlaylistTracks
function getPlaylistTracks(string playlistId, string market, string? fields, int? 'limit, int? offset, string? additionalTypes) returns PlaylistTrackDetails|error
Get a Playlist's Items
Parameters
- playlistId string - The Spotify ID for the playlist.
- market string - An ISO 3166-1 alpha-2 country code or the string
from_token
. Provide this parameter if you want to apply Track Relinking. For episodes, if a valid user access token is specified in the request header, the country associated with the user account will take priority over this parameter. Note: If neither market or user country are provided, the episode is considered unavailable for the client.
- fields string? (default ()) - Filters for the query: a comma-separated list of the fields to return. If omitted, all fields are returned. For example, to get just the total number of items and the request limit:
fields=total,limit
A dot separator can be used to specify non-reoccurring fields, while parentheses can be used to specify reoccurring fields within objects. For example, to get just the added date and user ID of the adder:fields=items(added_at,added_by.id)
Use multiple parentheses to drill down into nested objects, for example:fields=items(track(name,href,album(name,href)))
Fields can be excluded by prefixing them with an exclamation mark, for example:fields=items.track.album(!external_urls,images)
- 'limit int? (default ()) - The maximum number of items to return. Default: 100. Minimum: 1. Maximum: 100.
- offset int? (default ()) - The index of the first item to return. Default: 0 (the first object).
- additionalTypes string? (default ()) - A comma-separated list of item types that your client supports besides the default
track
type. Valid types are:track
andepisode
. Note : This parameter was introduced to allow existing clients to maintain their current behaviour and might be deprecated in the future. In addition to providing this parameter, make sure that your client properly handles cases of new types in the future by checking against thetype
field of each object.
Return Type
- PlaylistTrackDetails|error - On success, the response body contains an array of track objects and episode objects (depends on the
additional_types
parameter), wrapped in a paging object in JSON format and the HTTP status code in the response header is200
OK. If an episode is unavailable in the givenmarket
, its information will not be included in the response. On error, the header status code is an error code and the response body contains an error object. Requesting playlists that you do not have the user's authorization to access returns error403
Forbidden.
reorderOrReplacePlaylistTracks
function reorderOrReplacePlaylistTracks(string playlistId, PlayListReorderDetails payload, string? uris) returns SnapshotIdObject|error?
Reorder or Replace a Playlist's Items
Parameters
- playlistId string - The Spotify ID for the playlist.
- payload PlayListReorderDetails - Information needed to reorder the playlist
- uris string? (default ()) - A comma-separated list of Spotify URIs to set, can be track or episode URIs. For example:
uris=spotify:track:4iV5W9uYEdYUVa79Axb7Rh,spotify:track:1301WleyT98MSxVHPZCA6M,spotify:episode:512ojhOuo1ktJprKbVcKyQ
A maximum of 100 items can be set in one request.
Return Type
- SnapshotIdObject|error? - On a successful reorder operation, the response body contains a
snapshot_id
in JSON format and the HTTP status code in the response header is200
OK. Thesnapshot_id
can be used to identify your playlist version in future requests. On a successful replace operation, the HTTP status code in the response header is201
Created. On error, the header status code is an error code, the response body contains an error object, and the existing playlist is unmodified. Trying to set an item when you do not have the user's authorization returns error403
Forbidden.
getPlayslistsByUserID
function getPlayslistsByUserID(string userId, int? 'limit, int? offset) returns UserPlayListDetails|error
Get a List of a User's Playlists
Parameters
- userId string - The user's Spotify user ID.
- 'limit int? (default ()) - The maximum number of playlists to return. Default: 20. Minimum: 1. Maximum: 50.
- offset int? (default ()) - The index of the first playlist to return. Default: 0 (the first object). Maximum offset: 100.000. Use with
limit
to get the next set of playlists.
Return Type
- UserPlayListDetails|error - On success, the HTTP status code in the response header is
200
OK and the response body contains an array of simplified playlist objects (wrapped in a paging object) in JSON format. On error, the header status code is an error code and the response body contains an error object.
createPlaylist
function createPlaylist(string userId, PlayListDetails payload) returns PlaylistObject|error
Create a Playlist
Parameters
- userId string - The user's Spotify user ID.
- payload PlayListDetails - Content to create playlist
Return Type
- PlaylistObject|error - On success, the response body contains the created playlist object in JSON format and the HTTP status code in the response header is
200
OK or201
Created. There is also aLocation
response header giving the Web API endpoint for the new playlist. On error, the header status code is an error code and the response body contains an error object. Trying to create a playlist when you do not have the user's authorization returns error403
Forbidden.
getNewReleses
function getNewReleses(string? country, int? 'limit, int? offset) returns NewReleasesObject|error
Get All New Releases
Parameters
- country string? (default ()) - A country: an ISO 3166-1 alpha-2 country code. Provide this parameter if you want the list of returned items to be relevant to a particular country. If omitted, the returned items will be relevant to all countries.
- 'limit int? (default ()) - The maximum number of items to return. Default: 20. Minimum: 1. Maximum: 50.
- offset int? (default ()) - The index of the first item to return. Default: 0 (the first object). Use with
limit
to get the next set of items.
Return Type
- NewReleasesObject|error - On success, the HTTP status code in the response header is
200
OK and the response body contains amessage
and analbums
object. Thealbums
object contains an array of simplified album objects (wrapped in a paging object) in JSON format. On error, the header status code is an error code and the response body contains an error object. Once you have retrieved the list, you can use Get an Album's Tracks to drill down further. The results are returned in an order reflected within the Spotify clients, and therefore may not be ordered by date.
getFeaturedPlaylists
function getFeaturedPlaylists(string? country, string? locale, string? timestamp, int? 'limit, int? offset) returns FeaturedPlaylistObject|error
Get All Featured Playlists
Parameters
- country string? (default ()) - A country: an ISO 3166-1 alpha-2 country code. Provide this parameter if you want the list of returned items to be relevant to a particular country. If omitted, the returned items will be relevant to all countries.
- locale string? (default ()) - The desired language, consisting of a lowercase ISO 639-1 language code and an uppercase ISO 3166-1 alpha-2 country code, joined by an underscore. For example:
es_MX
, meaning "Spanish (Mexico)". Provide this parameter if you want the results returned in a particular language (where available). Note that, iflocale
is not supplied, or if the specified language is not available, all strings will be returned in the Spotify default language (American English). Thelocale
parameter, combined with thecountry
parameter, may give odd results if not carefully matched. For examplecountry=SE&locale=de_DE
will return a list of categories relevant to Sweden but as German language strings.
- timestamp string? (default ()) - A timestamp in ISO 8601 format:
yyyy-MM-ddTHH:mm:ss
. Use this parameter to specify the user's local time to get results tailored for that specific date and time in the day. If not provided, the response defaults to the current UTC time. Example: "2014-10-23T09:00:00" for a user whose local time is 9AM. If there were no featured playlists (or there is no data) at the specified time, the response will revert to the current UTC time.
- 'limit int? (default ()) - The maximum number of items to return. Default: 20. Minimum: 1. Maximum: 50.
- offset int? (default ()) - The index of the first item to return. Default: 0 (the first object). Use with
limit
to get the next set of items.
Return Type
- FeaturedPlaylistObject|error - On success, the HTTP status code in the response header is
200
OK and the response body contains amessage
and aplaylists
object. Theplaylists
object contains an array of simplified playlist objects (wrapped in a paging object) in JSON format. On error, the header status code is an error code and the response body contains an error object. Once you have retrieved the list of playlist objects, you can use Get a Playlist and Get a Playlist's Tracks to drill down further.
Records
spotify: AlbumRestrictionObject
Album restriction object
Fields
- reason string? - The reason for the restriction. Supported values:
market
- The content item is not available in the given market.product
- The content item is not available for the user's subscription type.explicit
- The content item is explicit and the user's account is set to not play explicit content.
Additional reasons may be added in the future. Note: If you use this field, make sure that your application safely handles unknown values.
spotify: ArtistObject
Artist object
Fields
- external_urls ExternalUrlObject? - External URL object
- followers FollowersObject? - Followers object
- genres string[]? - A list of the genres the artist is associated with. For example:
"Prog Rock"
,"Post-Grunge"
. (If not yet classified, the array is empty.)
- href string? - A link to the Web API endpoint providing full details of the artist.
- id string - The Spotify ID for the artist.
- images ImageObject[]? - Images of the artist in various sizes, widest first.
- name string - The name of the artist.
- popularity int? - The popularity of the artist. The value will be between 0 and 100, with 100 being the most popular. The artist's popularity is calculated from the popularity of all the artist's tracks.
- 'type string? - The object type:
"artist"
- uri string? - The Spotify URI for the artist.
spotify: ChangePlayListDetails
Content to update the playlist
Fields
- collaborative boolean? - If
true
, the playlist will become collaborative and other users will be able to modify the playlist in their Spotify client. Note: You can only setcollaborative
totrue
on non-public playlists.
- description string? - Value for playlist description as displayed in Spotify Clients and in the Web API.
- name string? - The new name for the playlist, for example
"My New Playlist Title"
- 'public boolean? - If
true
the playlist will be public, iffalse
it will be private.
spotify: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
spotify: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
spotify: CopyrightObject
Copyright object
Fields
- text string? - The copyright text for this content.
- 'type string? - The type of copyright:
C
= the copyright,P
= the sound recording (performance) copyright.
spotify: CurrentPlaylistDetails
Current playlist details
Fields
- href string? - A link to the Web API endpoint returning the full result of the request
- items SimplifiedPlaylistObject[]? - The requested data.
- 'limit int? - The maximum number of items in the response (as set in the query or by default).
- next string? - URL to the next page of items. (
null
if none)
- offset int? - The offset of the items returned (as set in the query or by default)
- previous string? - URL to the previous page of items. (
null
if none)
- total int? - The total number of items available to return.
spotify: EpisodeObject
Episode object
Fields
- audio_preview_url string? - A URL to a 30 second preview (MP3 format) of the episode.
null
if not available.
- description string? - A description of the episode. HTML tags are stripped away from this field, use
html_description
field in case HTML tags are needed.
- duration_ms int? - The episode length in milliseconds.
- explicit boolean? - Whether or not the episode has explicit content (true = yes it does; false = no it does not OR unknown).
- external_urls ExternalUrlObject? - External URL object
- href string? - A link to the Web API endpoint providing full details of the episode.
- html_description string? - A description of the episode. This field may contain HTML tags.
- id string - The Spotify ID for the episode.
- images ImageObject[]? - The cover art for the episode in various sizes, widest first.
- is_externally_hosted boolean? - True if the episode is hosted outside of Spotify's CDN.
- is_playable boolean? - True if the episode is playable in the given market. Otherwise false.
- name string? - The name of the episode.
- release_date string? - The date the episode was first released, for example
"1981-12-15"
. Depending on the precision, it might be shown as"1981"
or"1981-12"
.
- release_date_precision string? - The precision with which
release_date
value is known:"year"
,"month"
, or"day"
.
- restrictions EpisodeRestrictionObject? - Episode restriction object
- resume_point ResumePointObject? - Resume point object
- show SimplifiedShowObject? - Simplified show object
- 'type string? - The object type: "episode".
- uri string? - The Spotify URI for the episode.
spotify: EpisodeRestrictionObject
Episode restriction object
Fields
- reason string? - The reason for the restriction. Supported values:
market
- The content item is not available in the given market.product
- The content item is not available for the user's subscription type.explicit
- The content item is explicit and the user's account is set to not play explicit content.
Additional reasons may be added in the future. Note: If you use this field, make sure that your application safely handles unknown values.
spotify: ErrorObject
Error object
Fields
- message string? - A short description of the cause of the error.
- status int? - The HTTP status code (also returned in the response header; see Response Status Codes for more information).
spotify: ErrorResponseObject
Error response object
Fields
- 'error ErrorObject? - Error object
spotify: ExternalIdObject
External Id object
Fields
- upc string? - Universal Product Code
spotify: ExternalUrlObject
External URL object
Fields
- spotify string? - The Spotify URL for the object.
spotify: FeaturedPlaylistObject
Featured playlist object
Fields
- message string? - Message
- playlists FeaturedplaylistobjectPlaylists? - Playlist details
spotify: FeaturedplaylistobjectPlaylists
Playlist details
Fields
- href string? - A link to the Web API endpoint returning the full result of the request
- items SimplifiedPlaylistObject[]? - The requested data.
- 'limit int? - The maximum number of items in the response (as set in the query or by default).
- next string? - URL to the next page of items. (
null
if none)
- offset int? - The offset of the items returned (as set in the query or by default)
- previous string? - URL to the previous page of items. (
null
if none)
- total int? - The total number of items available to return.
spotify: FollowersObject
Followers object
Fields
- href string? - A link to the Web API endpoint providing full details of the followers;
null
if not available. Please note that this will always be set to null, as the Web API does not support it at the moment.
- total int? - The total number of followers.
spotify: ImageObject
Image object
Fields
- height int? - The image height in pixels. If unknown:
null
or not returned.
- url string? - The source URL of the image.
- width int? - The image width in pixels. If unknown:
null
or not returned.
spotify: LinkedTrackObject
Linked track object
Fields
- external_urls ExternalUrlObject? - External URL object
- href string? - A link to the Web API endpoint providing full details of the track.
- id string - The Spotify ID for the track.
- 'type string? - The object type: "track".
- uri string? - The Spotify URI for the track.
spotify: NewReleasesObject
New release object
Fields
- albums NewreleasesobjectAlbums - Album details
spotify: NewreleasesobjectAlbums
Album details
Fields
- href string? - A link to the Web API endpoint returning the full result of the request
- items SimplifiedAlbumObject[]? - The requested data.
- 'limit int? - The maximum number of items in the response (as set in the query or by default).
- next string? - URL to the next page of items. (
null
if none)
- offset int? - The offset of the items returned (as set in the query or by default)
- previous string? - URL to the previous page of items. (
null
if none)
- total int? - The total number of items available to return.
spotify: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://accounts.spotify.com/api/token") - Refresh URL
spotify: PlayListDetails
Playlist details
Fields
- description string? - value for playlist description as displayed in Spotify Clients and in the Web API.
- name string - The name for the new playlist, for example
"Your Coolest Playlist"
. This name does not need to be unique; a user may have several playlists with the same name.
spotify: PlaylistObject
Playlist object
Fields
- collaborative boolean? -
true
if the owner allows other users to modify the playlist.
- description string? - The playlist description. Only returned for modified, verified playlists, otherwise
null
.
- external_urls ExternalUrlObject? - External URL object
- followers FollowersObject? - Followers object
- href string? - A link to the Web API endpoint providing full details of the playlist.
- id string - The Spotify ID for the playlist.
- images ImageObject[]? - Images for the playlist. The array may be empty or contain up to three images. The images are returned by size in descending order. See Working with Playlists. Note: If returned, the source URL for the image (
url
) is temporary and will expire in less than a day.
- name string? - The name of the playlist.
- owner PublicUserObject? - Public user object
- 'public boolean? - The playlist's public/private status:
true
the playlist is public,false
the playlist is private,null
the playlist status is not relevant. For more about public/private status, see Working with Playlists
- snapshot_id string? - The version identifier for the current playlist. Can be supplied in other requests to target a specific playlist version
- tracks PlaylistobjectTracks? - Information about the tracks of the playlist. Note, a track object may be
null
. This can happen if a track is no longer available.
- 'type string? - The object type: "playlist"
- uri string? - The Spotify URI for the playlist.
spotify: PlaylistobjectTracks
Information about the tracks of the playlist. Note, a track object may be null
. This can happen if a track is no longer available.
Fields
- href string? - A link to the Web API endpoint returning the full result of the request
- items PlaylistTrackObject[]? - The requested data.
- 'limit int? - The maximum number of items in the response (as set in the query or by default).
- next string? - URL to the next page of items. (
null
if none)
- offset int? - The offset of the items returned (as set in the query or by default)
- previous string? - URL to the previous page of items. (
null
if none)
- total int? - The total number of items available to return.
spotify: PlayListReorderDetails
Information needed to reorder the playlist
Fields
- insert_before int? - The position where the items should be inserted.
To reorder the items to the end of the playlist, simply set insert_before to the position after the last item.
Examples:
To reorder the first item to the last position in a playlist with 10 items, set range_start to 0, and insert_before to 10.
To reorder the last item in a playlist with 10 items to the start of the playlist, set range_start to 9, and insert_before to 0.
- range_length int? - The amount of items to be reordered. Defaults to 1 if not set.
The range of items to be reordered begins from the range_start position, and includes the range_length subsequent items.
Example:
To move the items at index 9-10 to the start of the playlist, range_start is set to 9, and range_length is set to 2.
- range_start int? - The position of the first item to be reordered.
- snapshot_id string? - The playlist's snapshot ID against which you want to make the changes.
- uris string[]? - A comma-separated list of Spotify URIs to set, can be track or episode URIs. For example:
uris=spotify:track:4iV5W9uYEdYUVa79Axb7Rh,spotify:track:1301WleyT98MSxVHPZCA6M,spotify:episode:512ojhOuo1ktJprKbVcKyQ
A maximum of 100 items can be set in one request.
spotify: PlaylistTrackDetails
Playlist track details
Fields
- href string? - A link to the Web API endpoint returning the full result of the request
- items PlaylistTrackObject[]? - The requested data.
- 'limit int? - The maximum number of items in the response (as set in the query or by default).
- next string? - URL to the next page of items. (
null
if none)
- offset int? - The offset of the items returned (as set in the query or by default)
- previous string? - URL to the previous page of items. (
null
if none)
- total int? - The total number of items available to return.
spotify: PlaylistTrackObject
Playlist track object
Fields
- added_at string? - The date and time the track or episode was added. Note that some very old playlists may return
null
in this field.
- added_by PublicUserObject? - Public user object
- is_local boolean? - Whether this track or episode is a local file or not.
- track TrackObject|EpisodeObject? - Information about the track or episode.
spotify: PlaylistTracksRefObject
Playlist track reference object
Fields
- href string? - A link to the Web API endpoint where full details of the playlist's tracks can be retrieved.
- total int? - Number of tracks in the playlist.
spotify: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
spotify: PublicUserObject
Public user object
Fields
- display_name string? - The name displayed on the user's profile.
null
if not available.
- external_urls ExternalUrlObject? - External URL object
- followers FollowersObject? - Followers object
- href string? - A link to the Web API endpoint for this user.
- id string - The Spotify user ID for this user.
- images ImageObject[]? - The user's profile image.
- 'type string? - The object type: "user"
- uri string? - The Spotify URI for this user.
spotify: ResumePointObject
Resume point object
Fields
- fully_played boolean? - Whether or not the episode has been fully played by the user.
- resume_position_ms int? - The user's most recent position in the episode in milliseconds.
spotify: SimplifiedAlbumObject
Simplified album object
Fields
- album_group string? - The field is present when getting an artist's albums. Possible values are "album", "single", "compilation", "appears_on". Compare to album_type this field represents relationship between the artist and the album.
- album_type string? - The type of the album: one of "album", "single", or "compilation".
- artists SimplifiedArtistObject[] - The artists of the album. Each artist object includes a link in
href
to more detailed information about the artist.
- available_markets string[]? - The markets in which the album is available: ISO 3166-1 alpha-2 country codes. Note that an album is considered available in a market when at least 1 of its tracks is available in that market.
- external_urls ExternalUrlObject? - External URL object
- href string? - A link to the Web API endpoint providing full details of the album.
- id string - The Spotify ID for the album.
- images ImageObject[]? - The cover art for the album in various sizes, widest first.
- name string? - The name of the album. In case of an album takedown, the value may be an empty string.
- release_date string? - The date the album was first released, for example
1981
. Depending on the precision, it might be shown as1981-12
or1981-12-15
.
- release_date_precision string? - The precision with which
release_date
value is known:year
,month
, orday
.
- restrictions AlbumRestrictionObject? - Album restriction object
- total_tracks int? - The total number of tracks in the album.
- 'type string? - The object type: "album"
- uri string? - The Spotify URI for the album.
spotify: SimplifiedArtistObject
Simplified artist object
Fields
- external_urls ExternalUrlObject? - External URL object
- href string? - A link to the Web API endpoint providing full details of the artist.
- id string - The Spotify ID for the artist.
- name string - The name of the artist.
- 'type string? - The object type:
"artist"
- uri string? - The Spotify URI for the artist.
spotify: SimplifiedPlaylistObject
Simplified playlist object
Fields
- collaborative boolean? -
true
if the owner allows other users to modify the playlist.
- description string? - The playlist description. Only returned for modified, verified playlists, otherwise
null
.
- external_urls ExternalUrlObject? - External URL object
- href string? - A link to the Web API endpoint providing full details of the playlist.
- id string - The Spotify ID for the playlist.
- images ImageObject[]? - Images for the playlist. The array may be empty or contain up to three images. The images are returned by size in descending order. See Working with Playlists. Note: If returned, the source URL for the image (
url
) is temporary and will expire in less than a day.
- name string - The name of the playlist.
- owner PublicUserObject? - Public user object
- 'public boolean? - The playlist's public/private status:
true
the playlist is public,false
the playlist is private,null
the playlist status is not relevant. For more about public/private status, see Working with Playlists
- snapshot_id string? - The version identifier for the current playlist. Can be supplied in other requests to target a specific playlist version
- tracks PlaylistTracksRefObject? - Playlist track reference object
- 'type string? - The object type: "playlist"
- uri string? - The Spotify URI for the playlist.
spotify: SimplifiedShowObject
Simplified show object
Fields
- available_markets string[]? - A list of the countries in which the show can be played, identified by their ISO 3166-1 alpha-2 code.
- copyrights CopyrightObject[]? - The copyright statements of the show.
- description string? - A description of the show. HTML tags are stripped away from this field, use
html_description
field in case HTML tags are needed.
- explicit boolean? - Whether or not the show has explicit content (true = yes it does; false = no it does not OR unknown).
- external_urls ExternalUrlObject? - External URL object
- href string? - A link to the Web API endpoint providing full details of the show.
- html_description string? - A description of the show. This field may contain HTML tags.
- id string - The Spotify ID for the show.
- images ImageObject[]? - The cover art for the show in various sizes, widest first.
- is_externally_hosted boolean? - True if all of the show's episodes are hosted outside of Spotify's CDN. This field might be
null
in some cases.
- media_type string? - The media type of the show.
- name string? - The name of the episode.
- publisher string? - The publisher of the show.
- 'type string? - The object type: "show".
- uri string? - The Spotify URI for the show.
spotify: SnapshotIdObject
Fields
- snapshot_id string? - The snapshot_id can be used to identify your playlist version in future requests.
spotify: TrackObject
Track object
Fields
- album SimplifiedAlbumObject? - Simplified album object
- artists ArtistObject[]? - The artists who performed the track. Each artist object includes a link in
href
to more detailed information about the artist.
- available_markets string[]? - A list of the countries in which the track can be played, identified by their ISO 3166-1 alpha-2 code.
- disc_number int? - The disc number (usually
1
unless the album consists of more than one disc).
- duration_ms int? - The track length in milliseconds.
- explicit boolean? - Whether or not the track has explicit lyrics (
true
= yes it does;false
= no it does not OR unknown).
- external_ids ExternalIdObject? - External Id object
- external_urls ExternalUrlObject? - External URL object
- href string? - A link to the Web API endpoint providing full details of the track.
- id string - The Spotify ID for the track.
- is_local boolean? - Whether or not the track is from a local file.
- is_playable boolean? - Part of the response when Track Relinking is applied. If
true
, the track is playable in the given market. Otherwisefalse
.
- linked_from LinkedTrackObject? - Linked track object
- name string? - The name of the track.
- popularity int? - The popularity of the track. The value will be between 0 and 100, with 100 being the most popular.
The popularity of a track is a value between 0 and 100, with 100 being the most popular. The popularity is calculated by algorithm and is based, in the most part, on the total number of plays the track has had and how recent those plays are.
Generally speaking, songs that are being played a lot now will have a higher popularity than songs that were played a lot in the past. Duplicate tracks (e.g. the same track from a single and an album) are rated independently. Artist and album popularity is derived mathematically from track popularity. Note that the popularity value may lag actual popularity by a few days: the value is not updated in real time.
- preview_url string? - A link to a 30 second preview (MP3 format) of the track. Can be
null
- restrictions TrackRestrictionObject? - Track restriction object
- track_number int? - The number of the track. If an album has several discs, the track number is the number on the specified disc.
- 'type string? - The object type: "track".
- uri string? - The Spotify URI for the track.
spotify: TrackRestrictionObject
Track restriction object
Fields
- reason string? - The reason for the restriction. Supported values:
market
- The content item is not available in the given market.product
- The content item is not available for the user's subscription type.explicit
- The content item is explicit and the user's account is set to not play explicit content.
Additional reasons may be added in the future. Note: If you use this field, make sure that your application safely handles unknown values.
spotify: UserPlayListDetails
User playlist Details
Fields
- href string? - A link to the Web API endpoint returning the full result of the request
- items SimplifiedPlaylistObject[]? - The requested data.
- 'limit int? - The maximum number of items in the response (as set in the query or by default).
- next string? - URL to the next page of items. (
null
if none)
- offset int? - The offset of the items returned (as set in the query or by default)
- previous string? - URL to the previous page of items. (
null
if none)
- total int? - The total number of items available to return.
Import
import ballerinax/spotify;
Metadata
Released date: almost 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 1983
Current verison: 249
Weekly downloads
Keywords
Content & Files/Video & Audio
Cost/Freemium
Contributors
Dependencies