sinch.verification
Module sinch.verification
API
Definitions
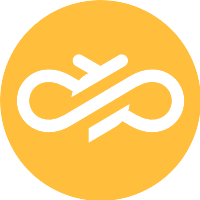
ballerinax/sinch.verification Ballerina library
Overview
This is a generated connector for Sinch Verification API v2.0 OpenAPI specification.
Verify users with SMS, flash calls (missed calls), a regular call, or data verification.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Sinch account account
- Obtain tokens by following this guide.
Quickstart
To use the Sinch Verification connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/sinch.verification
module into the Ballerina project.
import ballerinax/sinch.verification;
Step 2: Create a new connector instance
Create a verification:ClientConfig
with the <USERNAME>
and <PASSWORD>
obtained, and initialize the connector with it.
verification:ClientConfig clientConfig = { auth: { username: <USERNAME>, password: <PASSWORD> } }; verification:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example to start verification using the connector.
public function main() { verification:InitiateVerificationResource resources = { identity: { 'type: "number", endpoint: "+46700000000" }, method: "sms" }; verification:InitiateVerificationResponse|error response = baseClient->startVerification(resources); if (response is verification:InitiateVerificationResponse) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
sinch.verification: Client
This is a generated connector for Sinch Verification API v2.0 OpenAPI specification. Verify users with SMS, flash calls (missed calls), a regular call, or data verification.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Sinch account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://verificationapi-v1.sinch.com" - URL of the target service
startVerification
function startVerification(InitiateVerificationResource payload) returns InitiateVerificationResponse|error
Start verification
Parameters
- payload InitiateVerificationResource - Start verification request payload.
Return Type
- InitiateVerificationResponse|error - A successful response.
reportVerificationByIdentity
function reportVerificationByIdentity(string 'type, string endpoint, VerificationReportRequestResource payload) returns VerificationResponse|error
Report back on a started verification
Parameters
- 'type string - Currently only
number
type is supported.
- payload VerificationReportRequestResource - Verification report request payload.
Return Type
- VerificationResponse|error - A successful response.
verificationStatusById
function verificationStatusById(string id) returns VerificationResponse|error
Get verification by ID
Parameters
- id string - The ID of the verification.
Return Type
- VerificationResponse|error - A sucessful response.
reportVerificationById
function reportVerificationById(string id, VerificationReportRequestResource payload) returns VerificationResponse|error
Report back on a started verification
Parameters
- id string - The ID of the verification.
- payload VerificationReportRequestResource - Verification report request resource payload.
Return Type
- VerificationResponse|error - A sucessful response.
verificationStatusByIdentity
function verificationStatusByIdentity(string 'type, string endpoint, string method) returns VerificationResponse|error
Get verification by Identity
Parameters
- 'type string - Currently only
number
type is supported.
- method string - The method of the verification.
Return Type
- VerificationResponse|error - A sucessful response.
verificationStatusByReference
function verificationStatusByReference(string reference) returns VerificationResponse|error
Get verification by Reference
Parameters
- reference string - The custom reference of the verification.
Return Type
- VerificationResponse|error - A sucessful response.
Records
sinch.verification: CalloutInitiateVerificationResponse
Fields
- id string - Verification identifier used to query for status.
- callout CalloutinitiateverificationresponseCallout - The response contains information for the client to poll for the verification result.
sinch.verification: CalloutinitiateverificationresponseCallout
The response contains information for the client to poll for the verification result.
Fields
- startPollingAfter int? - The amount of time in seconds after the request is sent that the polling should start.
- stopPollingAfter int? - The amount of time in seconds after the request is sent that polling should stop.
- pollingInterval int? - The amount of time in seconds between each poll.
sinch.verification: CalloutRequestEventResponse
Fields
- action string? - Determines whether the verification can be executed.
- callout CalloutrequesteventresponseCallout? -
sinch.verification: CalloutrequesteventresponseCallout
Fields
- locale string? - Indicates the language that should be used for the text-to-speech message. Currently, only
en-US
is supported.
- ttsMenu string? - The message that can be played to the user when the phone call is picked up. The default value is: "To verify your phone number, please press {pin}. If you didn’t request this call, please hang up."
- wavFile string? - The
.wav
file that can be played to the user when the phone call is picked up.
- pin string? - The digit that the user should press to verify the phone number. The default value is
1
.
sinch.verification: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
sinch.verification: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth CredentialsConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
sinch.verification: DataInitiateVerificationResponse
Fields
- id string - Verification identifier used to query for status.
- seamless DatainitiateverificationresponseSeamless? - The response contains the target URI.
sinch.verification: DatainitiateverificationresponseSeamless
The response contains the target URI.
Fields
- targetUri string? - The target URI.
sinch.verification: FlashCallInitiateVerificationResponse
Fields
- id string - Verification identifier used to query for status.
- flashCall FlashcallinitiateverificationresponseFlashcall - The response contains the
cliFilter
andinterceptionTimeout
properties.
sinch.verification: FlashcallinitiateverificationresponseFlashcall
The response contains the cliFilter
and interceptionTimeout
properties.
Fields
- cliFilter string - Filter that should be applied for incoming calls to intercept the Flashcall.
- interceptionTimeout int - Amount of seconds client should wait for the Flashcall.
sinch.verification: FlashCallRequestEventResponse
Fields
- action string? - Determines whether the verification can be executed.
- flashCall FlashcallrequesteventresponseFlashcall? -
sinch.verification: FlashcallrequesteventresponseFlashcall
Fields
- cli string? - The phone number that will be displayed to the user when the flashcall is received on the user's phone. By default, the Sinch dashboard will randomly select the CLI that will be displayed during a flashcall from a pool of numbers. If you want to set your own CLI, you can specify it in the response to the Verification Request Event.
- dialTimeout int? - The maximum time that a flashcall verification will be active and can be completed. If the phone number hasn't been verified successfully during this time, then the verification request will fail. By default, the Sinch dashboard will automatically optimize dial time out during a flashcall. If you want to set your own dial time out for the flashcall, you can specify it in the response to the Verification Request Event.
sinch.verification: FlashcallVerificationReportRequest
Fields
- method string - The type of verification.
- flashCall FlashcallverificationreportrequestFlashcall - Contains information about the FlashCall verification.
sinch.verification: FlashcallverificationreportrequestFlashcall
Contains information about the FlashCall verification.
Fields
- cli string - The caller ID of the FlashCall.
sinch.verification: Identity
Specifies the type of endpoint that will be verified and the particular endpoint. number
is currently the only supported endpoint type.
Fields
- 'type string - Currently only
number
type is supported.
sinch.verification: InitiateVerificationResource
Fields
- identity Identity - Specifies the type of endpoint that will be verified and the particular endpoint.
number
is currently the only supported endpoint type.
- method VerificationMethod - The type of the verification request.
- reference string? - Used to pass your own reference in the request for tracking purposes.
- custom string? - Can be used to pass custom data in the request.
- flashCallOptions InitiateverificationresourceFlashcalloptions? - An optional object for flashCall verifications. It allows you to specify dial time out parameter for flashCall. FlashCallOptions object can be specified optionally, and only if the verification request was triggered from your backend (no SDK client) through an Application signed request.
sinch.verification: InitiateverificationresourceFlashcalloptions
An optional object for flashCall verifications. It allows you to specify dial time out parameter for flashCall. FlashCallOptions object can be specified optionally, and only if the verification request was triggered from your backend (no SDK client) through an Application signed request.
Fields
- dialTimeout int? - The dial timeout in seconds.
sinch.verification: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
sinch.verification: SMSInitiateVerificationResponse
Fields
- id string - Verification identifier used to query for status.
- sms SmsinitiateverificationresponseSms - The response contains the
template
of the SMS to be expected and intercepted.
sinch.verification: SmsinitiateverificationresponseSms
The response contains the template
of the SMS to be expected and intercepted.
Fields
- template string? - The expected SMS response.
sinch.verification: SMSRequestEventResponse
Fields
- action string? - Determines whether the verification can be executed.
- sms SmsrequesteventresponseSms? -
sinch.verification: SmsrequesteventresponseSms
Fields
- code string? - The SMS PIN that should be used. By default, the Sinch dashboard will automatically generate PIN codes for SMS verification. If you want to set your own PIN, you can specify it in the response to the Verification Request Event.
- acceptLanguage string[]? - The SMS verification content language. Set in the verification request.
sinch.verification: SmsVerificationReportRequest
Fields
- method string - The type of verification.
- sms SmsverificationreportrequestSms - Contains information about the SMS verification.
sinch.verification: SmsverificationreportrequestSms
Contains information about the SMS verification.
Fields
- code string - The code which was input by the user submitting the SMS verification.
- cli string? - The sender ID of the SMS.
sinch.verification: VerificationRequestEvent
Fields
- id string - The ID of the verification request.
- event string - The type of the event.
- method string - The verification method.
- identity Identity - Specifies the type of endpoint that will be verified and the particular endpoint.
number
is currently the only supported endpoint type.
- price VerificationrequesteventPrice? - The amount of money and currency of the verification request.
- reference string? - Used to pass your own reference in the request for tracking purposes.
- custom string? - Can be used to pass custom data in the request.
- acceptLanguage string[]? - Allows you to set or override if provided in the API request, the SMS verification content language. Only used with the SMS verification method. The content language specified in the API request or in the callback can be overridden by carrier provider specific templates, due to compliance and legal requirements, such as US shortcode requirements (pdf).
sinch.verification: VerificationrequesteventPrice
The amount of money and currency of the verification request.
Fields
- amount int? - The amount of money of the verification request.
- currencyId string? - The currency of the price.
sinch.verification: VerificationResponse
Fields
- id string? - Verification identifier used to query for status.
- method VerificationMethod? - The type of the verification request.
- status string? - The status of the verification request.
- reason string? - Displays the reason why a verification has
FAILED
, wasDENIED
, or wasABORTED
.
- reference string? - The reference ID that was optionally passed together with the verification request.
- 'source string? - Free text that the client is sending, used to show if the call/SMS was intercepted or not.
sinch.verification: VerificationResultEvent
Fields
- id string - The ID of the verification request.
- event string - The type of the event.
- method string - The verification method.
- identity Identity - Specifies the type of endpoint that will be verified and the particular endpoint.
number
is currently the only supported endpoint type.
- status string - The status of the verification request.
- reason string? - Displays the reason why a verification has
FAILED
, wasDENIED
, or wasABORTED
.
- reference string? - The reference ID that was optionally passed together with the verification request.
- 'source string? - Free text that the client is sending, used to show if the call/SMS was intercepted or not.
- custom string? - A custom string that can be provided during a verification request.
Union types
sinch.verification: InitiateVerificationResponse
InitiateVerificationResponse
sinch.verification: VerificationReportRequestResource
VerificationReportRequestResource
sinch.verification: VerificationRequestEventResponse
VerificationRequestEventResponse
sinch.verification: VerificationResultEventResponse
VerificationResultEventResponse
String types
sinch.verification: VerificationMethod
VerificationMethod
The type of the verification request.
Import
import ballerinax/sinch.verification;
Metadata
Released date: over 1 year ago
Version: 1.5.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 0
Current verison: 0
Weekly downloads
Keywords
IT Operations/Security & Identity Tools
Cost/Paid
Contributors
Dependencies