salesforce
Modules
Module salesforce
API
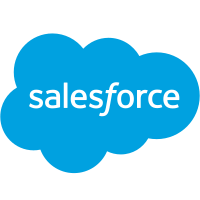
ballerinax/salesforce Ballerina library
Overview
Salesforce REST API provides CRUD operations for SObjects, query using SOQL, search using SOSL, and describe SObjects and organizational data.
This module supports Salesforce v48.0 REST API.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create Salesforce account
- Obtain tokens
- Client tokens - Follow the steps listed under OAuth 2.0 Web Server Flow for Web App Integration.
- Listener tokens - Follow the steps listed under Reset Your Security Token generate secret key and follow the steps listed under Subscription Channels to subscribe channels.
Quickstart
To use the Salesforce connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
Import the ballerinax/salesforce
module into the Ballerina project.
import ballerinax/salesforce;
Step 2: Create a new connector instance
Create a salesforce:ConnectionConfig
with the OAuth2 tokens obtained, and initialize the connector with it.
configurable string clientId = ?; configurable string clientSecret = ?; configurable string refreshToken = ?; configurable string refreshUrl = ?; configurable string baseUrl = ?; salesforce:ConnectionConfig sfConfig = { baseUrl: baseUrl, auth: { clientId: clientId, clientSecret: clientSecret, refreshToken: refreshToken, refreshUrl: refreshUrl } }; salesforce:Client baseClient = new(sfConfig);
Step 3: Invoke connector operation
- Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to create a record using the connector.
record{} accountRecord = { "Name": "IT World", "BillingCity": "Colombo 1" }; public function main() returns error? { salesforce:CreationResponse res = check baseClient->create("Account", accountRecord); }
- Use
bal run
command to compile and run the Ballerina program.
Clients
salesforce: Client
Ballerina Salesforce connector provides the capability to access Salesforce REST API. This connector lets you to perform operations for SObjects, query using SOQL, search using SOSL, and describe SObjects and organizational data.
Constructor
Initializes the connector. During initialization you can pass either http:BearerTokenConfig if you have a bearer token or http:OAuth2RefreshTokenGrantConfig if you have Oauth tokens. Create a Salesforce account and obtain tokens following this guide.
init (ConnectionConfig config)
- config ConnectionConfig -
getOrganizationMetaData
function getOrganizationMetaData() returns OrganizationMetadata|error
Gets metadata of your organization.
Return Type
- OrganizationMetadata|error -
OrganizationMetadata
record if successful or elseerror
getBasicInfo
function getBasicInfo(string sobjectName) returns SObjectBasicInfo|error
Gets basic data of the specified object.
Parameters
- sobjectName string - sObject name
Return Type
- SObjectBasicInfo|error -
SObjectBasicInfo
record if successful or elseerror
describe
function describe(string sObjectName) returns SObjectMetaData|error
Completely describes the individual metadata at all levels of the specified object. Can be used to retrieve the fields, URLs, and child relationships.
Parameters
- sObjectName string - sObject name value
Return Type
- SObjectMetaData|error -
SObjectMetaData
record if successful or elseerror
getPlatformAction
function getPlatformAction() returns SObjectBasicInfo|error
Query for actions displayed in the UI, given a user, a context, device format, and a record ID.
Return Type
- SObjectBasicInfo|error -
SObjectBasicInfo
record if successful or elseerror
getApiVersions
Lists summary details about each REST API version available.
getResources
Lists the resources available for the specified API version.
Parameters
- apiVersion string - API version (v37)
getLimits
Lists the Limits information for your organization.
Return Type
getById
function getById(string sobject, string id, string[] fields, typedesc<record {}> returnType) returns returnType|error
Gets an object record by ID.
Parameters
- sobject string - sObject name
- id string - sObject ID
- fields string[] (default []) - Fields to retrieve
- returnType typedesc<record {}> (default <>) - The payload, which is expected to be returned after data binding.
Return Type
- returnType|error - Record if successful or else
error
getByExternalId
function getByExternalId(string sobject, string extIdField, string extId, string[] fields, typedesc<record {}> returnType) returns returnType|error
Gets an object record by external ID.
Parameters
- sobject string - sObject name
- extIdField string - External ID field name
- extId string - External ID value
- fields string[] (default []) - Fields to retrieve
- returnType typedesc<record {}> (default <>) - The payload, which is expected to be returned after data binding.
Return Type
- returnType|error - Record if successful or else
error
create
function create(string sObjectName, record {} sObjectRecord) returns CreationResponse|error
Creates records based on relevant object type sent with json record.
Return Type
- CreationResponse|error - Creation response if successful or else
error
update
Updates records based on relevant object ID.
Parameters
- sObjectName string - sObject name value
- id string - sObject ID
- sObjectRecord record {} - Record to be updated
Return Type
- error? - Empty response if successful
error
upsert
function upsert(string sObjectName, string externalIdField, string externalId, record {} sObjectRecord) returns error?
Upsert a record based on the value of a specified external ID field.
Parameters
- sObjectName string - sObject name value
- externalIdField string - External ID field of an object
- externalId string - External ID
- sObjectRecord record {} - Record to be upserted
Return Type
- error? - Empty response if successful or else
error
delete
Delete existing records based on relevant object ID.
Return Type
- error? - Empty response if successful or else
error
listReports
Lists reports.
deleteReport
Deletes a report.
Parameters
- reportId string - Report Id
Return Type
- error? -
()
if the report deletion is successful or else an error
runReportSync
function runReportSync(string reportId) returns ReportInstanceResult|error
Runs an instance of a report synchronously.
Parameters
- reportId string - Report Id
Return Type
- ReportInstanceResult|error - ReportInstanceResult if successful or else
error
runReportAsync
function runReportAsync(string reportId) returns ReportInstance|error
Runs an instance of a report asynchronously.
Parameters
- reportId string - Report Id
Return Type
- ReportInstance|error - ReportInstance if successful or else
error
listAsyncRunsOfReport
function listAsyncRunsOfReport(string reportId) returns ReportInstance[]|error
Lists asynchronous runs of a Report.
Parameters
- reportId string - Report Id
Return Type
- ReportInstance[]|error - Array of ReportInstance if successful or else
error
getReportInstanceResult
function getReportInstanceResult(string reportId, string instanceId) returns ReportInstanceResult|error
Get report instance result.
Return Type
- ReportInstanceResult|error - ReportInstanceResult if successful or else
error
query
function query(string soql, typedesc<record {}> returnType) returns stream<returnType, error?>|error
Executes the specified SOQL query.
Parameters
- soql string - SOQL query
- returnType typedesc<record {}> (default <>) - The payload, which is expected to be returned after data binding.
Return Type
- stream<returnType, error?>|error -
stream<{returnType}, error?>
if successful. Else, the occurrederror
.
search
function search(string sosl, typedesc<record {}> returnType) returns stream<returnType, error?>|error
Executes the specified SOSL search.
Parameters
- sosl string - SOSL search query
- returnType typedesc<record {}> (default <>) - The payload, which is expected to be returned after data binding.
Return Type
- stream<returnType, error?>|error -
stream<{returnType}, error?>
record if successful. Else, the occurrederror
.
describeAvailableObjects
function describeAvailableObjects() returns OrgMetadata|Error
Lists the available objects and their metadata for your organization and available to the logged-in user.
Return Type
- OrgMetadata|Error -
OrgMetadata
record if successful or elsesfdc:Error
Deprecated
This function is deprecated as the method signature is altered. Use the new and improvedgetOrganizationMetaData()
function instead.
getSObjectBasicInfo
function getSObjectBasicInfo(string sobjectName) returns SObjectBasicInfo|Error
Describes the individual metadata for the specified object.
Parameters
- sobjectName string - SObject name
Return Type
- SObjectBasicInfo|Error -
SObjectBasicInfo
record if successful or elsesfdc:Error
Deprecated
This function is deprecated as the method signature is altered. Use the new and improvedgetBasicInfo(string sobjectName)
function instead.
describeSObject
function describeSObject(string sObjectName) returns SObjectMetaData|Error
Completely describes the individual metadata at all levels for the specified object. Can be used to retrieve the fields, URLs, and child relationships.
Parameters
- sObjectName string - SObject name value
Return Type
- SObjectMetaData|Error -
SObjectMetaData
record if successful or elsesfdc:Error
Deprecated
This function is deprecated as the method signature is altered. Use the new and improveddescribe(string sObjectName)
function instead.
sObjectPlatformAction
function sObjectPlatformAction() returns SObjectBasicInfo|Error
Query for actions displayed in the UI, given a user, a context, device format, and a record ID.
Return Type
- SObjectBasicInfo|Error -
SObjectBasicInfo
record if successful or elsesfdc:Error
Deprecated
This function is deprecated as the method signature is altered. Use the new and improvedgetPlatformAction()
function instead.
getAvailableApiVersions
Lists summary details about each REST API version available.
Return Type
getResourcesByApiVersion
Lists the resources available for the specified API version.
Parameters
- apiVersion string - API version (v37)
Return Type
getOrganizationLimits
Lists the Limits information for your organization.
Return Type
getRecord
Accesses records based on the specified object ID, can be used with external objects.
Parameters
- path string - Resource path
Return Type
- json|Error - JSON result if successful else or else
sfdc:Error
Deprecated
This function is deprecated as it returns a json response. Use the new and improvedgetById(string sObject, string id)
function instead.
getRecordById
Gets an object record by ID.
Return Type
- json|Error - JSON result if successful or else
sfdc:Error
Deprecated
This function is deprecated as it returns a json response. Use the new and improvedgetById(string sObject, string id)
function instead.
getRecordByExtId
function getRecordByExtId(string sobject, string extIdField, string extId, string... fields) returns json|Error
Gets an object record by external ID.
Parameters
- sobject string - SObject name
- extIdField string - External ID field name
- extId string - External ID value
- fields string... - Fields to retrieve
Return Type
- json|Error - JSON result if successful or else
sfdc:Error
Deprecated
This function is deprecated as it returns a json response. Use the new and improvedgetByExternalId(string sObject, string extIdField, string extId)
function instead.
createRecord
Creates records based on relevant object type sent with json record.
Return Type
deleteRecord
Delete existing records based on relevant object ID.
Return Type
- Error? - true if successful else false or else
sfdc:Error
Deprecated
This function is deprecated to rename it. Use the new and improveddelete(string sObjectName, string id)
function instead.
updateRecord
Updates records based on relevant object ID.
Parameters
- sObjectName string - SObject name value
- id string - SObject ID
- recordPayload json - JSON record to be updated
Return Type
- Error? - true if successful else false or else
sfdc:Error
Deprecated
This function is deprecated as it expects a json payload. Use the new and improvedupdate(string sObjectName, string id, record{} recordPayload)
function instead.
getAccountById
Accesses Account SObject records based on the Account object ID.
Return Type
- json|Error - JSON response if successful or else an sfdc:Error
Deprecated
This function is deprecated as it returns a json response. Use the new and improvedgetById(string sObject, string id)
function instead.
createAccount
Creates new Account object record.
Parameters
- accountRecord json - Account JSON record to be inserted
Return Type
deleteAccount
Deletes existing Account's records.
Parameters
- accountId string - Account ID
Return Type
- Error? -
true
if successfulfalse
otherwise, or an sfdc:Error in case of an errorDeprecated
This function is deprecated to rename it. Use the new and improveddelete(string sObjectName, string id)
function instead.
updateAccount
Updates existing Account object record.
Return Type
- Error? -
true
if successful,false
otherwise or an sfdc:Error in case of an errorDeprecated
This function is deprecated as it expects a json payload. Use the new and improvedupdate(string sObjectName, string id, record{} recordPayload)
function instead.
getLeadById
Accesses Lead SObject records based on the Lead object ID.
Return Type
- json|Error - JSON response if successful or else an sfdc:Error
Deprecated
This function is deprecated as it returns a json response. Use the new and improvedgetById(string sObject, string id)
function instead.
createLead
Creates new Lead object record.
Parameters
- leadRecord json - Lead JSON record to be inserted
Return Type
deleteLead
Deletes existing Lead's records.
Parameters
- leadId string - Lead ID
Return Type
- Error? -
true
if successful,false
otherwise or an sfdc:Error incase of an errorDeprecated
This function is deprecated to rename it. Use the new and improveddelete(string sObjectName, string id)
function instead.
updateLead
Updates existing Lead object record.
Return Type
- Error? -
true
if successful,false
otherwise or an sfdc:Error in case of an errorDeprecated
This function is deprecated as it expects a json payload. Use the new and improvedupdate(string sObjectName, string id, record{} recordPayload)
function instead.
getContactById
Accesses Contacts SObject records based on the Contact object ID.
Return Type
- json|Error - JSON result if successful or else an sfdc:Error
Deprecated
This function is deprecated as it returns a json response. Use the new and improvedgetById(string sObject, string id)
function instead.
createContact
Creates new Contact object record.
Parameters
- contactRecord json - JSON contact record
Return Type
deleteContact
Deletes existing Contact's records.
Parameters
- contactId string - Contact ID
Return Type
- Error? -
true
if successful,false
otherwise or an sfdc:Error in case of an errorDeprecated
This function is deprecated to rename it. Use the new and improveddelete(string sObjectName, string id)
function instead.
updateContact
Updates existing Contact object record.
Return Type
- Error? -
true
if successful,false
otherwise or an sfdc:Error in case of an errorDeprecated
This function is deprecated as it expects a json payload. Use the new and improvedupdate(string sObjectName, string id, record{} recordPayload)
function instead.
getOpportunityById
Accesses Opportunities SObject records based on the Opportunity object ID.
Return Type
- json|Error - JSON response if successful or else an sfdc:Error
Deprecated
This function is deprecated as it returns a json response. Use the new and improvedgetById(string sObject, string id)
function instead.
createOpportunity
Creates new Opportunity object record.
Parameters
- opportunityRecord json - JSON opportunity record
Return Type
deleteOpportunity
Deletes existing Opportunity's records.
Parameters
- opportunityId string - Opportunity ID
Return Type
- Error? -
true
if successful,false
otherwise or an sfdc:Error in case of an errorDeprecated
This function is deprecated to rename it. Use the new and improveddelete(string sObjectName, string id)
function instead.
updateOpportunity
Updates existing Opportunity object record.
Return Type
- Error? -
true
if successful,false
otherwise or an sfdc:Error in case of an errorDeprecated
This function is deprecated as it expects a json payload. Use the new and improvedupdate(string sObjectName, string id, record{} recordPayload)
function instead.
getProductById
Accesses Products SObject records based on the Product object ID.
Return Type
- json|Error - JSON result if successful or else an sfdc:Error
Deprecated
This function is deprecated as it returns a json response. Use the new and improvedgetById(string sObject, string id)
function instead.
createProduct
Creates new Product object record.
Parameters
- productRecord json - JSON product record
Return Type
deleteProduct
Deletes existing product's records.
Parameters
- productId string - Product ID
Return Type
- Error? -
true
if successful,false
otherwise or an sfdc:Error in case of an errorDeprecated
This function is deprecated to rename it. Use the new and improveddelete(string sObjectName, string id)
function instead.
updateProduct
Updates existing Product object record.
Return Type
- Error? -
true
if successful,false
otherwise or an sfdc:Error in case of an errorDeprecated
This function is deprecated as it expects a json payload. Use the new and improvedupdate(string sObjectName, string id, record{} recordPayload)
function instead.
getQueryResult
function getQueryResult(string receivedQuery) returns SoqlResult|Error
Executes the specified SOQL query.
Parameters
- receivedQuery string - Sent SOQL query
Return Type
- SoqlResult|Error -
SoqlResult
record if successful. Else, the occurredError
.Deprecated
This function is deprecated as it does not handle the pagination of records Use the new and improvedquery(string soql)
function instead.
getNextQueryResult
function getNextQueryResult(string nextRecordsUrl) returns SoqlResult|Error
If the query results are too large, retrieve the next batch of results using the nextRecordUrl.
Parameters
- nextRecordsUrl string - URL to get the next query results
Return Type
- SoqlResult|Error -
SoqlResult
record if successful. Else, the occurredError
.Deprecated
This function is deprecated as it is related to pagination of results inquery(string soql)
Use the new and improvedquery(string soql)
function instead.
getQueryResultStream
Executes the specified SOQL query.
Parameters
- receivedQuery string - Sent SOQL query
Return Type
searchSOSLString
function searchSOSLString(string searchString) returns SoslResult|Error
Executes the specified SOSL search.
Parameters
- searchString string - Sent SOSL search query
Return Type
- SoslResult|Error -
SoslResult
record if successful. Else, the occurredError
.Deprecated
This function is deprecated as it does not handle the pagination of returned data Use the new and improvedsearch(string sosl)
function instead.
searchSOSLStringStream
Executes the specified SOSL search.
Parameters
- searchString string - Sent SOSL search query
Constants
salesforce: API_VERSION
Constant field API_VERSION
. Holds the value for the Salesforce API version.
salesforce: EMPTY_STRING
Constant field EMPTY_STRING
. Holds the value of "".
salesforce: ERR_EXTRACTING_ERROR_MSG
salesforce: HTTP_ERROR_MSG
salesforce: INVALID_CLIENT_CONFIG
Records
salesforce: AsyncReportAttributes
Represents attributes of instance of an asynchronous report run
Fields
- id string - Unique ID for an instance of a report that was run
- reportId string - Unique report ID
- reportName string - Display name of the report
- status string - Status of the report run
- ownerId string - API name of the user that created the instance
- requestDate string - Date and time when an instance of the report run was requested
- 'type string - Format of the resource
- completionDate string - Date, time when the instance of the report run finished
- errorMessage string - Error message if the instance run failed
- queryable boolean - Indicates if it is queryable
salesforce: Attribute
Defines the Attribute type. Contains the attribute information of the resultant record.
Fields
- 'type string -
- url string? - URL of the resultant record
salesforce: ConnectionConfig
Represents the Salesforce client configuration.
Fields
- Fields Included from *ConnectionConfig
- auth AuthConfig
- httpVersion HttpVersion
- http1Settings ClientHttp1Settings
- http2Settings ClientHttp2Settings
- timeout decimal
- forwarded string
- poolConfig PoolConfiguration
- cache CacheConfig
- compression Compression
- circuitBreaker CircuitBreakerConfig
- retryConfig RetryConfig
- responseLimits ResponseLimitConfigs
- secureSocket ClientSecureSocket
- proxy ProxyConfig
- validation boolean
- anydata...
- baseUrl string - The Salesforce endpoint URL
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
salesforce: CreationResponse
Response of object creation.
Fields
- id string - Created object ID
- errors anydata[] - Array of errors
- success boolean - Success flag
salesforce: ErrorDetails
Additional details extracted from the Http error.
Fields
- statusCode int? - Http status code of the error
- body anydata? - Response body with extra information
salesforce: Limit
Defines the Limit type to list limits information for your org.
Fields
- Max int - The limit total for the org
- Remaining int - The total number of calls or events left for the org
- json... - Rest field
salesforce: OrganizationMetadata
Metadata for your organization and available to the logged-in user.
Fields
- encoding string - Encoding
- maxBatchSize int - Maximum batch size
- sobjects SObjectMetaData[] - Available SObjects
- json... - Rest field
salesforce: OrgMetadata
Metadata for your organization and available to the logged-in user.
Fields
- encoding string - Encoding
- maxBatchSize int - Maximum batch size
- sobjects SObjectMetaData[] - Available SObjects
Deprecated
This record is deprecated as the name is changed.
- json... - Rest field
salesforce: Report
Represents a Report
Fields
- id string - Unique report ID
- name string - Report display name
- url string - URL that returns report data
- describeUrl string - URL that retrieves report metadata
- instancesUrl string - Information for each instance of the report that was run asynchronously.
salesforce: ReportInstance
Represents an instance of a Report
Fields
- id string - Unique ID for a report instance
- status string - Status of the report run
- requestDate string - Date and time when an instance of the report run was requested
- completionDate string - Date, time when the instance of the report run finished
- url string - URL where results of the report run for that instance are stored
- ownerId string - API name of the user that created the instance
- queryable boolean - Indicates if it is queryable
- hasDetailRows boolean - Indicates if it has detailed data
salesforce: ReportInstanceResult
Represents result of an asynchronous report run
Fields
- attributes AsyncReportAttributes|SyncReportAttributes - Attributes for the instance of the report run
- allData boolean - Indicates if all report results are returned
- factMap map<json> - Collection of summary level data or both detailed and summary level data
- groupingsAcross map<json> - Collection of column groupings
- groupingsDown map<json> - Collection of row groupings
- reportMetadata map<json> - Information about the fields used to build the report
- hasDetailRows boolean - Indicates if it has detailed data
- reportExtendedMetadata map<json> - Information on report groupings, summary fields, and detailed data columns
salesforce: SObjectBasicInfo
Basic info of a SObject.
Fields
- objectDescribe SObjectMetaData - Metadata related to the SObject
- json... - Rest field
salesforce: SObjectMetaData
Metadata for an SObject, including information about each field, URLs, and child relationships.
Fields
- name string - SObject name
- createable boolean - Is createable
- deletable boolean - Is deletable
- updateable boolean - Is updateable
- queryable boolean - Is queryable
- label string - SObject label
- json... - Rest field
salesforce: SoqlRecord
Defines the SOQL query result record type.
Fields
- attributes Attribute - Attribute record
- json... - Rest field
salesforce: SoqlResult
Define the SOQL result type.
Fields
- done boolean - Query is completed or not
- totalSize int - The total number result records
- records SoqlRecord[] - Result records
- json... - Rest field
salesforce: SoslRecord
Defines SOSL query result.
Fields
- attributes Attribute - Attribute record
- Id string - ID of the matching object
- json... - Rest field
salesforce: SoslResult
Defines SOSL query result.
Fields
- searchRecords SoslRecord[] - Matching records for the given search string
- json... - Rest field
salesforce: SyncReportAttributes
Represents attributes of instance of synchronous report run
Fields
- reportId string - Unique report ID
- reportName string - Display name of the report
- 'type string - API resource format
- describeUrl string - Resource URL to get report metadata
- instancesUrl string - Resource URL to run a report asynchronously
salesforce: Version
Defines the Salesforce version type.
Fields
- label string - Label of the Salesforce version
- url string - URL of the Salesforce version
- 'version string -
Errors
salesforce: Error
Salesforce connector error.
Import
import ballerinax/salesforce;
Metadata
Released date: almost 2 years ago
Version: 7.6.0
License: Apache-2.0
Compatibility
Platform: java17
Ballerina version: 2201.8.0
GraalVM compatible: Yes
Pull count
Total: 96172
Current verison: 110
Weekly downloads
Keywords
Sales & CRM/Customer Relationship Management
Cost/Freemium
Contributors