salesforce
Modules
Module salesforce
API
Declarations
Definitions
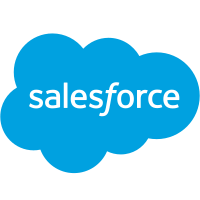
ballerinax/salesforce Ballerina library
Overview
Salesforce Sales Cloud is one of the leading Customer Relationship Management(CRM) software, provided by Salesforce.Inc. Salesforce enable users to efficiently manage sales and customer relationships through its APIs, robust and secure databases, and analytics services. Sales cloud provides serveral API packages to make operations on sObjects and metadata, execute queries and searches, and listen to change events through API calls using REST, SOAP, and CometD protocols.
Ballerina Salesforce connector supports Salesforce v59.0 REST API, Salesforce v59.0 SOAP API, Salesforce v59.0 APEX REST API, Salesforce v59.0 BULK API, and Salesforce v59.0 BULK V2 API.
Setup guide
-
Create a Salesforce account with the REST capability.
-
Go to Setup --> Apps --> App Manager
-
Create a New Connected App.
- Here we will be using https://test.salesforce.com as we are using sandbox environment. Users can use https://login.salesforce.com for normal usage.
-
After the creation user can get consumer key and secret through clicking on the
Manage Consumer Details
button. -
Next step would be to get the token.
- Log in to salesforce in your preferred browser and enter the following url.
https://<YOUR_INSTANCE>.salesforce.com/services/oauth2/authorize?response_type=code&client_id=<CONSUMER_KEY>&redirect_uri=<REDIRECT_URL>
-
Allow access if an alert pops up and the browser will be redirected to a Url like follows.
https://login.salesforce.com/?code=<ENCODED_CODE>
-
The code can be obtained after decoding the encoded code
- Get Access and Refresh tokens
-
Following request can be sent to obtain the tokens.
curl -X POST https://<YOUR_INSTANCE>.salesforce.com/services/oauth2/token?code=<CODE>&grant_type=authorization_code&client_id=<CONSUMER_KEY>&client_secret=<CONSUMER_SECRET>&redirect_uri=https://test.salesforce.com/
-
Tokens can be obtained from the response.
-
Quickstart
To use the Salesforce connector in your Ballerina application, modify the .bal file as follows:
Step 1: Import connector
Import the ballerinax/salesforce
package into the Ballerina project.
import ballerinax/salesforce;
Step 2: Create a new connector instance
Create a salesforce:ConnectionConfig
with the obtained OAuth2 tokens and initialize the connector with it.
salesforce:ConnectionConfig config = { baseUrl: baseUrl, auth: { clientId: clientId, clientSecret: clientSecret, refreshToken: refreshToken, refreshUrl: refreshUrl } }; salesforce:Client salesforce = check new (config);
Step 3: Invoke connector operation
- Now you can utilize the available operations. Note that they are in the form of remote operations.
Following is an example on how to create a record using the connector.
salesforce:CreationResponse response = check salesforce->create("Account", { "Name": "IT World", "BillingCity": "New York" });
- Use following command to compile and run the Ballerina program.
bal run
Examples
The salesforce
connector provides practical examples illustrating usage in various scenarios. Explore these examples below, covering use cases like creating sObjects, retrieving records, and executing bulk operations.
-
Salesforce REST API use cases - How to employ REST API of Salesforce to carryout various tasks.
-
Salesforce Bulk API use cases - How to employ Bulk API of Salesforce to execute Bulk jobs.
-
Salesforce Bulk v2 API use cases - How to employ Bulk v2 API to execute an ingest job.
-
Salesforce APEX REST API use cases - How to employ APEX REST API to create a case in Salesforce.
Clients
salesforce: Client
Ballerina Salesforce connector provides the capability to access Salesforce REST API. This connector lets you to perform operations for SObjects, query using SOQL, search using SOSL, and describe SObjects and organizational data.
Constructor
Initializes the connector. During initialization you can pass either http:BearerTokenConfig if you have a bearer token or http:OAuth2RefreshTokenGrantConfig if you have Oauth tokens. Create a Salesforce account and obtain tokens following this guide.
init (ConnectionConfig config)
- config ConnectionConfig -
getOrganizationMetaData
function getOrganizationMetaData() returns OrganizationMetadata|error
Gets metadata of your organization.
Return Type
- OrganizationMetadata|error -
OrganizationMetadata
record if successful or elseerror
getBasicInfo
function getBasicInfo(string sobjectName) returns SObjectBasicInfo|error
Gets basic data of the specified object.
Parameters
- sobjectName string - sObject name
Return Type
- SObjectBasicInfo|error -
SObjectBasicInfo
record if successful or elseerror
describe
function describe(string sObjectName) returns SObjectMetaData|error
Completely describes the individual metadata at all levels of the specified object. Can be used to retrieve the fields, URLs, and child relationships.
Parameters
- sObjectName string - sObject name value
Return Type
- SObjectMetaData|error -
SObjectMetaData
record if successful or elseerror
getPlatformAction
function getPlatformAction() returns SObjectBasicInfo|error
Query for actions displayed in the UI, given a user, a context, device format, and a record ID.
Return Type
- SObjectBasicInfo|error -
SObjectBasicInfo
record if successful or elseerror
getApiVersions
Lists summary details about each REST API version available.
getResources
Lists the resources available for the specified API version.
Parameters
- apiVersion string - API version (v37)
getLimits
Lists the Limits information for your organization.
Return Type
getById
function getById(string sobjectName, string id, typedesc<record {}> returnType) returns returnType|error
Gets an object record by ID.
Parameters
- sobjectName string - sObject name
- id string - sObject ID
- returnType typedesc<record {}> (default <>) - The payload, which is expected to be returned after data binding
Return Type
- returnType|error -
record
if successful or elseerror
getByExternalId
function getByExternalId(string sobjectName, string extIdField, string extId, typedesc<record {}> returnType) returns returnType|error
Gets an object record by external ID.
Parameters
- sobjectName string - sObject name
- extIdField string - External ID field name
- extId string - External ID value
- returnType typedesc<record {}> (default <>) - The payload, which is expected to be returned after data binding
Return Type
- returnType|error - Record if successful or else
error
create
function create(string sObjectName, record {} sObject) returns CreationResponse|error
Creates records based on relevant object type sent with json record.
Return Type
- CreationResponse|error -
CreationResponse
if successful or elseerror
update
Updates records based on relevant object ID.
Parameters
- sObjectName string - sObject name value
- id string - sObject ID
- sObject record {} - Record to be updated
Return Type
- error? -
Nil
if successful, else returns an error
upsert
function upsert(string sObjectName, string externalIdField, string externalId, record {} sObject) returns error?
Upsert a record based on the value of a specified external ID field.
Parameters
- sObjectName string - sObject name value
- externalIdField string - External ID field of an object
- externalId string - External ID
- sObject record {} - Record to be upserted
Return Type
- error? -
Nil
if successful or elseerror
delete
Delete existing records based on relevant object ID.
Return Type
- error? -
Nil
if successful or elseerror
listReports
Lists reports.
deleteReport
Deletes a report.
Parameters
- reportId string - Report Id
Return Type
- error? -
Nil
if the report deletion is successful or else an error
runReportSync
function runReportSync(string reportId) returns ReportInstanceResult|error
Runs an instance of a report synchronously.
Parameters
- reportId string - Report Id
Return Type
- ReportInstanceResult|error -
ReportInstanceResult
if successful or elseerror
runReportAsync
function runReportAsync(string reportId) returns ReportInstance|error
Runs an instance of a report asynchronously.
Parameters
- reportId string - Report Id
Return Type
- ReportInstance|error -
ReportInstance
if successful or elseerror
listAsyncRunsOfReport
function listAsyncRunsOfReport(string reportId) returns ReportInstance[]|error
Lists asynchronous runs of a Report.
Parameters
- reportId string - Report Id
Return Type
- ReportInstance[]|error - Array of
ReportInstance
if successful or elseerror
getReportInstanceResult
function getReportInstanceResult(string reportId, string instanceId) returns ReportInstanceResult|error
Get report instance result.
Return Type
- ReportInstanceResult|error -
ReportInstanceResult
if successful or elseerror
query
function query(string soql, typedesc<record {}> returnType) returns stream<returnType, error?>|error
Executes the specified SOQL query.
Parameters
- soql string - SOQL query
- returnType typedesc<record {}> (default <>) - The payload, which is expected to be returned after data binding
Return Type
- stream<returnType, error?>|error -
stream<{returnType}, error?>
if successful. Else, the occurrederror
search
function search(string sosl, typedesc<record {}> returnType) returns stream<returnType, error?>|error
Executes the specified SOSL search.
Parameters
- sosl string - SOSL search query
- returnType typedesc<record {}> (default <>) - The payload, which is expected to be returned after data binding
Return Type
- stream<returnType, error?>|error -
stream<{returnType}, error?>
record if successful. Else, the occurrederror
getDeletedRecords
function getDeletedRecords(string sObjectName, Civil startDate, Civil endDate) returns DeletedRecordsResult|error
Retrieves the list of individual records that have been deleted within the given timespan.
Parameters
- sObjectName string - SObject reference
- startDate Civil - Start date of the timespan
- endDate Civil - End date of the timespan
Return Type
- DeletedRecordsResult|error -
DeletedRecordsResult
record if successful or elseerror
getUpdatedRecords
function getUpdatedRecords(string sObjectName, Civil startDate, Civil endDate) returns UpdatedRecordsResults|error
Retrieves the list of individual records that have been updated within the given timespan.
Parameters
- sObjectName string - SObject reference
- startDate Civil - Start date of the timespan
- endDate Civil - End date of the timespan
Return Type
- UpdatedRecordsResults|error -
UpdatedRecordsResults
record if successful or elseerror
isPasswordExpired
Get the password information
Parameters
- userId string - User ID
resetPassword
Reset the user password.
Parameters
- userId string - User ID
Return Type
- byte[]|error -
byte[]
if successful or elseerror
changePassword
Change the user password
Return Type
- error? -
Nil
if successful or elseerror
getQuickActions
function getQuickActions(string sObjectName) returns QuickAction[]|error
Returns a list of actions and their details
Parameters
- sObjectName string - SObject reference
Return Type
- QuickAction[]|error -
QuickAction[]
if successful or elseerror
batch
function batch(Subrequest[] batchRequests, boolean haltOnError) returns BatchResult|error
Executes up to 25 sub-requests in a single request.
Parameters
- batchRequests Subrequest[] - A record containing all the requests
- haltOnError boolean (default false) - If true, the request halts when an error occurs on an individual sub-request
Return Type
- BatchResult|error -
BatchResult
if successful or elseerror
getNamedLayouts
function getNamedLayouts(string sObjectName, string layoutName, typedesc<record {}> returnType) returns returnType|error
Retrieves information about alternate named layouts for a given object.
Parameters
- sObjectName string - SObject reference
- layoutName string - Name of the layout
- returnType typedesc<record {}> (default <>) - The payload type, which is expected to be returned after data binding
Return Type
- returnType|error - Record of
returnType
if successful or elseerror
getInvocableActions
function getInvocableActions(string subContext, typedesc<record {}> returnType) returns returnType|error
Retrieve a list of general action types for the current organization.
Parameters
- subContext string - Sub context
- returnType typedesc<record {}> (default <>) - The payload type, which is expected to be returned after data binding
Return Type
- returnType|error - Record of
returnType
if successful or elseerror
invokeActions
function invokeActions(string subContext, record {} payload, typedesc<record {}> returnType) returns returnType|error
Invoke Actions.
Parameters
- subContext string - Sub context
- payload record {} - Payload for the action
- returnType typedesc<record {}> (default <>) - The type of the returned variable
Return Type
- returnType|error - Record of
returnType
if successful or elseerror
deleteRecordsUsingExtId
function deleteRecordsUsingExtId(string sObjectName, string externalId, string value) returns error?
Delete record using external Id.
Parameters
- sObjectName string - Name of the sObject
- externalId string - Name of the external id field
- value string - Value of the external id field
Return Type
- error? -
Nil
if successful or elseerror
apexRestExecute
function apexRestExecute(string urlPath, Method methodType, record {} payload, typedesc<record {}|string|int?> returnType) returns returnType|error
Access Salesforce APEX resource.
Parameters
- urlPath string - URI path
- methodType Method - HTTP method type
- payload record {} (default {}) - Payload
Return Type
- returnType|error -
string|int|record{}
type if successful or elseerror
createIngestJob
function createIngestJob(BulkCreatePayload payload) returns BulkJob|error
Creates a bulkv2 ingest job.
Parameters
- payload BulkCreatePayload - The payload for the bulk job
createQueryJob
function createQueryJob(BulkCreatePayload payload) returns BulkJob|error
Creates a bulkv2 query job.
Parameters
- payload BulkCreatePayload - The payload for the bulk job
createQueryJobAndWait
function createQueryJobAndWait(BulkCreatePayload payload) returns future<BulkJobInfo|error>|error
Creates a bulkv2 query job and provide future value.
Parameters
- payload BulkCreatePayload - The payload for the bulk job
Return Type
- future<BulkJobInfo|error>|error -
future<BulkJobInfo>
if successful elseerror
getJobInfo
function getJobInfo(string bulkJobId, BulkOperation bulkOperation) returns BulkJobInfo|error
Retrieves detailed information about a job.
Parameters
- bulkJobId string - Id of the bulk job
- bulkOperation BulkOperation - The processing operation for the job
Return Type
- BulkJobInfo|error -
BulkJobInfo
if successful or elseerror
addBatch
function addBatch(string bulkJobId, string|string[]|stream<string[], error?>|ReadableByteChannel content) returns error?
Uploads data for a job using CSV data.
Parameters
- bulkJobId string - Id of the bulk job
Return Type
- error? -
Nil
record if successful orerror
if unsuccessful
getAllJobs
Get details of all the jobs.
Parameters
- jobType JobType? (default ()) - Type of the job
getAllQueryJobs
Get details of all query jobs.
Parameters
- jobType JobType? (default ()) - Type of the job
getJobStatus
Get job status information.
getQueryResult
Get bulk query job results
Parameters
- bulkJobId string - Id of the bulk job
- maxRecords int? (default ()) - The maximum number of records to retrieve per set of results for the query
abortJob
function abortJob(string bulkJobId, BulkOperation bulkOperation) returns BulkJobInfo|error
Abort the bulkv2 job.
Parameters
- bulkJobId string - Id of the bulk job
- bulkOperation BulkOperation - The processing operation for the job
Return Type
- BulkJobInfo|error -
()
if successful elseerror
deleteJob
function deleteJob(string bulkJobId, BulkOperation bulkOperation) returns error?
Delete a bulkv2 job.
Parameters
- bulkJobId string - Id of the bulk job
- bulkOperation BulkOperation - The processing operation for the job
Return Type
- error? -
()
if successful elseerror
closeIngestJobAndWait
function closeIngestJobAndWait(string bulkJobId) returns error|future<BulkJobInfo|error>
Notifies Salesforce servers that the upload of job data is complete.
Parameters
- bulkJobId string - Id of the bulk job
Return Type
- error|future<BulkJobInfo|error> - future<BulkJobInfo> if successful else
error
closeIngestJob
function closeIngestJob(string bulkJobId) returns error|BulkJobCloseInfo
Notifies Salesforce servers that the upload of job data is complete.
Parameters
- bulkJobId string - Id of the bulk job
Return Type
- error|BulkJobCloseInfo - BulkJobInfo if successful else
error
Constants
salesforce: API_VERSION
Constant field API_VERSION
. Holds the value for the Salesforce API version.
salesforce: EMPTY_STRING
Constant field EMPTY_STRING
. Holds the value of "".
salesforce: ERR_EXTRACTING_ERROR_MSG
salesforce: HTTP_ERROR_MSG
salesforce: INVALID_CLIENT_CONFIG
Enums
salesforce: BulkOperation
Members
salesforce: ColumnDelimiterEnum
Members
salesforce: JobStateEnum
Members
salesforce: JobType
Members
salesforce: LineEndingEnum
Members
salesforce: Operation
Operation type of the bulk job.
Members
salesforce: Status
Represents status of the bulk jobs
Members
Records
salesforce: AllJobs
Represents output for get all jobs request
Fields
- done boolean - Indicates whether there are more records to retrieve.
- records BulkJobInfo[] - Array of job records.
- nextRecordsUrl string - URL to retrieve the next set of records.
salesforce: AsyncReportAttributes
Represents attributes of instance of an asynchronous report run.
Fields
- id string - Unique ID for an instance of a report that was run
- reportId string - Unique report ID
- reportName string - Display name of the report
- status string - Status of the report run
- ownerId string - API name of the user that created the instance
- requestDate string - Date and time when an instance of the report run was requested
- 'type string - Format of the resource
- completionDate string - Date, time when the instance of the report run finished
- errorMessage string - Error message if the instance run failed
- queryable boolean - Indicates if it is queryable
salesforce: Attribute
Defines the Attribute type. Contains the attribute information of the resultant record.
Fields
- 'type string - Type of the resultant record
- url string? - URL of the resultant record
salesforce: Attributes
Represent the Attributes at SObjectBasicInfo.
Fields
- 'type string - Type of the resultant record
- url string - URL of the resultant record
salesforce: BatchResult
Represent results of the batch request.
Fields
- hasErrors boolean - Indicates whether the batch request has errors
- results SubRequestResult[] - Results of the batch request
salesforce: BulkCreatePayload
Represents the bulk job creation request payload.
Fields
- 'object string? - the sObject type of the bulk job
- operation Operation - the operation type of the bulk job
- columnDelimiter ColumnDelimiterEnum? - the column delimiter of the payload
- contentType string? - the content type of the payload
- lineEnding LineEndingEnum? - the line ending of the payload
- externalIdFieldName string? - the external ID field name for upsert operations
- query string? - the SOQL query for query operations
salesforce: BulkJob
Represents the bulk job creation response.
Fields
- Fields Included from *BulkJobCloseInfo
- contentUrl string? - The URL to use for uploading the CSV data for the job.
- lineEnding string? - The line ending of the payload.
- columnDelimiter string? - The column delimiter of the payload.
salesforce: BulkJobCloseInfo
Represents bulk job related information when Closed.
Fields
- id string - The ID of the job.
- operation string - The operation type of the job.
- 'object string - The sObject type of the job.
- createdById string - The ID of the user who created the job.
- createdDate string - The date and time when the job was created.
- systemModstamp string - The date and time when the job was finished.
- state string - The state of the job.
- concurrencyMode string - The concurrency mode of the job.
- contentType string - The content type of the payload.
- apiVersion float - The API version.
salesforce: BulkJobInfo
Represents bulk job related information.
Fields
- Fields Included from *BulkJob
- retries int? - The number of times that Salesforce attempted to process the job.
- totalProcessingTime int? - The total time spent processing the job.
- apiActiveProcessingTime int? - The total time spent processing the job by API.
- apexProcessingTime int? - The total time spent to process triggers and other processes related to the job data;
- numberRecordsProcessed int? - The number of records already processed by the job.
salesforce: ConnectionConfig
Represents the Salesforce client configuration.
Fields
- Fields Included from *ConnectionConfig
- auth AuthConfig
- httpVersion HttpVersion
- http1Settings ClientHttp1Settings
- http2Settings ClientHttp2Settings
- timeout decimal
- forwarded string
- poolConfig PoolConfiguration
- cache CacheConfig
- compression Compression
- circuitBreaker CircuitBreakerConfig
- retryConfig RetryConfig
- responseLimits ResponseLimitConfigs
- secureSocket ClientSecureSocket
- proxy ProxyConfig
- validation boolean
- anydata...
- baseUrl string - The Salesforce endpoint URL
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig|OAuth2PasswordGrantConfig|OAuth2ClientCredentialsGrantConfig - Configurations related to client authentication
salesforce: CreationResponse
Response of object creation.
Fields
- id string - Created object ID
- errors anydata[] - Array of errors
- success boolean - Success flag
salesforce: DeletedRecordsResult
Represent the metadata of deleted records.
Fields
- earliestDateAvailable string - The earliest date covered by the results
- latestDateCovered string - The latest date covered by the results
salesforce: ErrorDetails
Additional details extracted from the Http error.
Fields
- errorCode string? - Error code from Salesforce
- message string? - Response body with extra information
salesforce: ErrorResponse
Represent the Error response for password access.
Fields
- message string - Error message
- errorCode string - Error code
salesforce: Limit
Defines the Limit type to list limits information for your org.
Fields
- Max int - The limit total for the org
- Remaining int - The total number of calls or events left for the org
- json... - Rest field
salesforce: OrganizationMetadata
Metadata for your organization and available to the logged-in user.
Fields
- encoding string - Encoding
- maxBatchSize int - Maximum batch size
- sobjects SObjectMetaData[] - Available SObjects
- json... - Rest field
salesforce: PasswordStatus
Represent the password status.
Fields
- isExpired boolean - Indicates whether the password is expired
salesforce: QuickAction
Represent a quick action.
Fields
- actionEnumOrId string - Action enum or ID
- label string - Action label
- name string - Action name
- 'type string - Action type
salesforce: Report
Represents a Report.
Fields
- id string - Unique report ID
- name string - Report display name
- url string - URL that returns report data
- describeUrl string - URL that retrieves report metadata
- instancesUrl string - Information for each instance of the report that was run asynchronously.
salesforce: ReportInstance
Represents an instance of a Report.
Fields
- id string - Unique ID for a report instance
- status string - Status of the report run
- requestDate string - Date and time when an instance of the report run was requested
- completionDate string - Date, time when the instance of the report run finished
- url string - URL where results of the report run for that instance are stored
- ownerId string - API name of the user that created the instance
- queryable boolean - Indicates if it is queryable
- hasDetailRows boolean - Indicates if it has detailed data
salesforce: ReportInstanceResult
Represents result of an asynchronous report run.
Fields
- attributes AsyncReportAttributes|SyncReportAttributes - Attributes for the instance of the report run
- allData boolean - Indicates if all report results are returned
- factMap map<json> - Collection of summary level data or both detailed and summary level data
- groupingsAcross map<json> - Collection of column groupings
- groupingsDown map<json> - Collection of row groupings
- reportMetadata map<json> - Information about the fields used to build the report
- hasDetailRows boolean - Indicates if it has detailed data
- reportExtendedMetadata map<json> - Information on report groupings, summary fields, and detailed data columns
salesforce: SObjectBasicInfo
Basic info of a SObject.
Fields
- objectDescribe SObjectMetaData - Metadata related to the SObject
- json... - Rest field
salesforce: SObjectMetaData
Metadata for an SObject, including information about each field, URLs, and child relationships.
Fields
- name string - SObject name
- createable boolean - Is createable
- deletable boolean - Is deletable
- updateable boolean - Is updateable
- queryable boolean - Is queryable
- label string - SObject label
- json... - Rest field
salesforce: Subrequest
Represent Subrequest of a batch.
Fields
- binaryPartName string? - Subrequest of a batch
- binaryPartNameAlias string? - Binary part name alias
- method string - Method of the subrequest
- richInput record {}? - Rich input of the subrequest
- url string - URL of the subrequest
salesforce: SubRequestResult
Represent a batch execution result.
Fields
- statusCode int - Status code of the batch execution
- result json - Result of the batch execution
salesforce: SyncReportAttributes
Represents attributes of instance of synchronous report run.
Fields
- reportId string - Unique report ID
- reportName string - Display name of the report
- 'type string - Format of the resource
- describeUrl string - Resource URL to get report metadata
- instancesUrl string - Resource URL to run a report asynchronously
salesforce: UpdatedRecordsResults
Represent the metadata of updated records.
Fields
- ids string[] - Array of updated record IDs
- latestDateCovered string - The latest date covered by the results
salesforce: Version
Defines the Salesforce version type.
Fields
- label string - Label of the Salesforce version
- url string - URL of the Salesforce version
- 'version string - Salesforce version number
Errors
salesforce: Error
Salesforce connector error.
Import
import ballerinax/salesforce;
Metadata
Released date: 5 months ago
Version: 8.0.2
License: Apache-2.0
Compatibility
Platform: java17
Ballerina version: 2201.8.4
GraalVM compatible: Yes
Pull count
Total: 95234
Current verison: 542
Weekly downloads
Keywords
Sales & CRM/Customer Relationship Management
Cost/Freemium
Contributors