power.bi
Module power.bi
API
Definitions
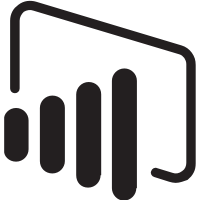
ballerinax/power.bi Ballerina library
Overview
This is a generated connector for Power BI API v1.0 OpenAPI specification.
Power BI REST API provides service endpoints for embedding, administration, and user resources.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create an Azure account.
- Obtain tokens by following this guide.
Quickstart
To use the Power BI connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/power.bi
module into the Ballerina project.
import ballerinax/power.bi;
Step 2: Create a new connector instance
Create a bi:ClientConfiguration
with the <ACCESS_TOKEN>
obtained, and initialize the connector with it.
bi:ClientConfiguration clientConfig = { auth : { token: `<ACCESS_TOKEN>` } }; bi:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example to list Attributes under a project using the connector.
public function main() { bi:Datasets|error response = baseClient->datasetsGetdatasets(); if (response is bi:Datasets) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
power.bi: Client
This is a generated connector for Power BI API v1.0 OpenAPI specification. Power BI REST API provides service endpoints for embedding, administration, and user resources.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create an Azure account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.powerbi.com/v1.0/myorg/" - URL of the target service
datasetsGetdatasets
Returns a list of datasets from "My Workspace".
datasetsPostdataset
function datasetsPostdataset(CreateDatasetRequest payload, string? defaultRetentionPolicy) returns Dataset|error
Creates a new dataset on "My Workspace".
Parameters
- payload CreateDatasetRequest - Dataset definition to create
- defaultRetentionPolicy string? (default ()) - The default retention policy
datasetsGetdataset
Returns the specified dataset from "My Workspace".
Parameters
- datasetId string - The dataset id
datasetsDeletedataset
Deletes the specified dataset from "My Workspace".
Parameters
- datasetId string - The dataset id
datasetsGettables
Returns a list of tables tables within the specified dataset from "My Workspace".
Parameters
- datasetId string - The dataset id
datasetsPuttable
Updates the metadata and schema for the specified table within the specified dataset from "My Workspace".
Parameters
- datasetId string - The dataset id
- tableName string - The table name
- payload Table - Table name and columns to update existing table
datasetsPostrows
function datasetsPostrows(string datasetId, string tableName, PostRowsRequest payload) returns Response|error
Adds new data rows to the specified table within the specified dataset from "My Workspace".
Parameters
- datasetId string - The dataset id
- tableName string - The table name
- payload PostRowsRequest - The request message
datasetsDeleterows
Deletes all rows from the specified table within the specified dataset from "My Workspace".
datasetsGetrefreshhistory
Returns the refresh history of the specified dataset from "My Workspace".
Parameters
- datasetId string - The dataset id
- top int? (default ()) - The requested number of entries in the refresh history. If not provided, the default is all available entries.
datasetsRefreshdataset
function datasetsRefreshdataset(string datasetId, RefreshRequest payload) returns Response|error
Triggers a refresh for the specified dataset from "My Workspace".
datasetsGetrefreshschedule
function datasetsGetrefreshschedule(string datasetId) returns RefreshSchedule|error
Returns the refresh schedule of the specified dataset from "My Workspace".
Parameters
- datasetId string - The dataset id
Return Type
- RefreshSchedule|error - OK
datasetsUpdaterefreshschedule
function datasetsUpdaterefreshschedule(string datasetId, RefreshScheduleRequest payload) returns Response|error
Updates the refresh schedule for the specified dataset from "My Workspace".
Parameters
- datasetId string - The dataset id
- payload RefreshScheduleRequest - Update Refresh Schedule parameters, by specifying all or some of the parameters
datasetsGetdirectqueryrefreshschedule
function datasetsGetdirectqueryrefreshschedule(string datasetId) returns DirectQueryRefreshSchedule|error
Returns the refresh schedule of a specified DirectQuery or LiveConnection dataset from "My Workspace".
Parameters
- datasetId string - The dataset id
Return Type
datasetsUpdatedirectqueryrefreshschedule
function datasetsUpdatedirectqueryrefreshschedule(string datasetId, DirectQueryRefreshScheduleRequest payload) returns Response|error
Updates the refresh schedule for the specified DirectQuery or LiveConnection dataset from "My Workspace".
Parameters
- datasetId string - The dataset id
- payload DirectQueryRefreshScheduleRequest - Patch DirectQuery or LiveConnection Refresh Schedule parameters, by specifying all or some of the parameters
datasetsGetparameters
function datasetsGetparameters(string datasetId) returns MashupParameters|error
Returns a list of parameters for the specified dataset from "My Workspace".
Parameters
- datasetId string - The dataset id
Return Type
- MashupParameters|error - OK
datasetsUpdateparameters
function datasetsUpdateparameters(string datasetId, UpdateMashupParametersRequest payload) returns Response|error
Updates the parameters values for the specified dataset from "My Workspace".
Parameters
- datasetId string - The dataset id
- payload UpdateMashupParametersRequest - Update mashup parameter request
datasetsGetdatasources
function datasetsGetdatasources(string datasetId) returns Datasources|error
Returns a list of datasources for the specified dataset from "My Workspace".
Parameters
- datasetId string - The dataset id
Return Type
- Datasources|error - OK
datasetsUpdatedatasources
function datasetsUpdatedatasources(string datasetId, UpdateDatasourcesRequest payload) returns Response|error
Updates the datasources of the specified dataset from "My Workspace".
Parameters
- datasetId string - The dataset id
- payload UpdateDatasourcesRequest - Update datasource request
datasetsSetalldatasetconnections
function datasetsSetalldatasetconnections(string datasetId, ConnectionDetails payload) returns Response|error
Updates all connections for the specified dataset from "My Workspace". This API only supports SQL DirectQuery datasets.
Note: This API is deprecated and no longer supported
datasetsBindtogateway
function datasetsBindtogateway(string datasetId, BindToGatewayRequest payload) returns Response|error
Binds the specified dataset from "My Workspace" to the specified gateway with (optional) given set of datasource Ids. This only supports the On-Premises Data Gateway.
Parameters
- datasetId string - The dataset id
- payload BindToGatewayRequest - The bind to gateway request
datasetsGetgatewaydatasources
function datasetsGetgatewaydatasources(string datasetId) returns GatewayDatasources|error
Returns a list of gateway datasources for the specified dataset from "My Workspace".
Parameters
- datasetId string - The dataset id
Return Type
- GatewayDatasources|error - OK
datasetsDiscovergateways
Returns a list of gateways which the specified dataset from "My Workspace" can be bound to.
Parameters
- datasetId string - The dataset id
usersRefreshuserpermissions
Refreshes user permissions in Power BI.
importsGetimports
Returns a list of imports from "My Workspace".
importsPostimport
function importsPostimport(string datasetDisplayName, ImportInfo payload, string? nameConflict, boolean? skipReport) returns Import|error
Creates new content on "My Workspace" from PBIX (Power BI Desktop), JSON, XLSX (Excel), RDL or file path in OneDrive for Business.
Parameters
- datasetDisplayName string - The display name of the dataset, should include file extension. Not supported when importing from OneDrive for Business.
- payload ImportInfo - The import to post
- nameConflict string? (default ()) - Determines what to do if a dataset with the same name already exists. Default value is 'Ignore'.<br/>Only Abort and Overwrite are supported with Rdl files.
- skipReport boolean? (default ()) - Determines whether to skip report import, if specified value must be 'true'. Only supported for PBIX files.
importsGetimport
Returns the specified import from "My Workspace".
Parameters
- importId string - The import id
importsCreatetemporaryuploadlocation
function importsCreatetemporaryuploadlocation() returns TemporaryUploadLocation|error
Creates a temporary blob storage to be used to import large .pbix files larger than 1 GB and up to 10 GB.
Return Type
reportsGetreports
Returns a list of reports from "My Workspace".
reportsGetreport
Returns the specified report from "My Workspace".
Parameters
- reportId string - The report id
reportsDeletereport
Deletes the specified report from "My Workspace".
Parameters
- reportId string - The report id
reportsClonereport
function reportsClonereport(string reportId, CloneReportRequest payload) returns Report|error
Clones the specified report from "My Workspace".
reportsExportreport
Exports the specified report from "My Workspace" to a .pbix file.
Parameters
- reportId string - The report id
reportsUpdatereportcontent
function reportsUpdatereportcontent(string reportId, UpdateReportContentRequest payload) returns Report|error
Updates the specified report from "My Workspace" to have the same content as the report in the request body.
Parameters
- reportId string - The report id
- payload UpdateReportContentRequest - UpdateReportContent parameters
reportsRebindreport
function reportsRebindreport(string reportId, RebindReportRequest payload) returns Response|error
Rebinds the specified report from "My Workspace" to the requested dataset.
reportsGetpages
Returns a list of pages within the specified report from "My Workspace".
Parameters
- reportId string - The report id
reportsGetpage
Returns the specified page within the specified report from "My Workspace".
reportsGetdatasources
function reportsGetdatasources(string reportId) returns Datasources|error
Returns a list of datasources for the specified RDL report from "My Workspace".
Parameters
- reportId string - The report id
Return Type
- Datasources|error - OK
reportsUpdatedatasources
function reportsUpdatedatasources(string reportId, UpdateRdlDatasourcesRequest payload) returns Response|error
Updates the datasources of the specified paginated report from "My Workspace". (Preview)
Parameters
- reportId string - The report id
- payload UpdateRdlDatasourcesRequest - Update RDL datasources request
reportsExporttofile
function reportsExporttofile(string reportId, ExportReportRequest payload) returns Export|error
Exports the specified report from "My Workspace" to the requested format.
Parameters
- reportId string - The report id
- payload ExportReportRequest - Export to file request parameters
reportsGetexporttofilestatus
Returns the status of the Export to file job from "My Workspace".
reportsGetfileofexporttofile
Returns the file of the Export to file job of the specified report from "My Workspace".
dashboardsGetdashboards
function dashboardsGetdashboards() returns Dashboards|error
Returns a list of dashboards from "My Workspace".
Return Type
- Dashboards|error - OK
dashboardsAdddashboard
function dashboardsAdddashboard(AddDashboardRequest payload) returns Dashboard|error
Creates a new empty dashboard on "My Workspace".
Parameters
- payload AddDashboardRequest - Add dashboard parameters
dashboardsGetdashboard
Returns the specified dashboard from "My Workspace".
Parameters
- dashboardId string - The dashboard id
dashboardsGettiles
Returns a list of tiles within the specified dashboard from "My Workspace".
Parameters
- dashboardId string - The dashboard id
dashboardsGettile
Returns the specified tile within the specified dashboard from "My Workspace".
dashboardsClonetile
function dashboardsClonetile(string dashboardId, string tileId, CloneTileRequest payload) returns Tile|error
Clones the specified tile from "My Workspace".
Parameters
- dashboardId string - The dashboard id
- tileId string - The tile id
- payload CloneTileRequest - Clone tile parameters
datasetsGetdatasetsingroup
Returns a list of datasets from the specified workspace.
Parameters
- groupId string - The workspace id
datasetsPostdatasetingroup
function datasetsPostdatasetingroup(string groupId, CreateDatasetRequest payload, string? defaultRetentionPolicy) returns Dataset|error
Creates a new dataset in the specified workspace.
Parameters
- groupId string - The workspace id
- payload CreateDatasetRequest - Create dataset parameters
- defaultRetentionPolicy string? (default ()) - The default retention policy
datasetsGetdatasettodataflowslinksingroup
function datasetsGetdatasettodataflowslinksingroup(string groupId) returns DatasetToDataflowLinksResponse|error
Returns a list of upstream dataflows for datasets from the specified workspace.
Parameters
- groupId string - The workspace id
Return Type
datasetsGetdatasetingroup
Returns the specified dataset from the specified workspace.
datasetsDeletedatasetingroup
Deletes the specified dataset from the specified workspace.
datasetsGettablesingroup
Returns a list of tables within the specified dataset from the specified workspace.
datasetsPuttableingroup
function datasetsPuttableingroup(string groupId, string datasetId, string tableName, Table payload) returns Table|error
Updates the metadata and schema for the specified table, within the specified dataset, from the specified workspace.
Parameters
- groupId string - The workspace id
- datasetId string - The dataset id
- tableName string - The table name
- payload Table - The request message
datasetsPostrowsingroup
function datasetsPostrowsingroup(string groupId, string datasetId, string tableName, PostRowsRequest payload) returns Response|error
Adds new data rows to the specified table, within the specified dataset, from the specified workspace.
Parameters
- groupId string - The workspace id
- datasetId string - The dataset id
- tableName string - The table name
- payload PostRowsRequest - The request message
datasetsDeleterowsingroup
function datasetsDeleterowsingroup(string groupId, string datasetId, string tableName) returns Response|error
Deletes all rows from the specified table, within the specified dataset, from the specified workspace.
Parameters
- groupId string - The workspace id
- datasetId string - The dataset id
- tableName string - The table name
datasetsGetrefreshhistoryingroup
function datasetsGetrefreshhistoryingroup(string groupId, string datasetId, int? top) returns Refreshes|error
Returns the refresh history of the specified dataset from the specified workspace.
Parameters
- groupId string - The workspace id
- datasetId string - The dataset id
- top int? (default ()) - The requested number of entries in the refresh history. If not provided, the default is all available entries.
datasetsRefreshdatasetingroup
function datasetsRefreshdatasetingroup(string groupId, string datasetId, RefreshRequest payload) returns Response|error
Triggers a refresh for the specified dataset from the specified workspace.
Parameters
- groupId string - The workspace id
- datasetId string - The dataset id
- payload RefreshRequest - Refresh request
datasetsGetrefreshscheduleingroup
function datasetsGetrefreshscheduleingroup(string groupId, string datasetId) returns RefreshSchedule|error
Returns the refresh schedule of the specified dataset from the specified workspace.
Return Type
- RefreshSchedule|error - OK
datasetsUpdaterefreshscheduleingroup
function datasetsUpdaterefreshscheduleingroup(string groupId, string datasetId, RefreshScheduleRequest payload) returns Response|error
Updates the refresh schedule for the specified dataset from the specified workspace.
Parameters
- groupId string - The workspace id
- datasetId string - The dataset id
- payload RefreshScheduleRequest - Update Refresh Schedule parameters, by specifying all or some of the parameters
datasetsGetdirectqueryrefreshscheduleingroup
function datasetsGetdirectqueryrefreshscheduleingroup(string groupId, string datasetId) returns DirectQueryRefreshSchedule|error
Returns the refresh schedule of a specified DirectQuery or LiveConnection dataset from the specified workspace.
Return Type
datasetsUpdatedirectqueryrefreshscheduleingroup
function datasetsUpdatedirectqueryrefreshscheduleingroup(string groupId, string datasetId, DirectQueryRefreshScheduleRequest payload) returns Response|error
Updates the refresh schedule for the specified DirectQuery or LiveConnection dataset from the specified workspace.
Parameters
- groupId string - The workspace id
- datasetId string - The dataset id
- payload DirectQueryRefreshScheduleRequest - Patch DirectQuery or LiveConnection Refresh Schedule parameters, by specifying all or some of the parameters
datasetsGetparametersingroup
function datasetsGetparametersingroup(string groupId, string datasetId) returns MashupParameters|error
Returns a list of parameters for the specified dataset from the specified workspace.
Return Type
- MashupParameters|error - OK
datasetsUpdateparametersingroup
function datasetsUpdateparametersingroup(string groupId, string datasetId, UpdateMashupParametersRequest payload) returns Response|error
Updates the parameters values for the specified dataset from the specified workspace.
Parameters
- groupId string - The workspace id
- datasetId string - The dataset id
- payload UpdateMashupParametersRequest - Update mashup parameter request
datasetsGetdatasourcesingroup
function datasetsGetdatasourcesingroup(string groupId, string datasetId) returns Datasources|error
Returns a list of datasources for the specified dataset from the specified workspace.
Return Type
- Datasources|error - OK
datasetsUpdatedatasourcesingroup
function datasetsUpdatedatasourcesingroup(string groupId, string datasetId, UpdateDatasourcesRequest payload) returns Response|error
Updates the datasources of the specified dataset from the specified workspace.
Parameters
- groupId string - The workspace id
- datasetId string - The dataset id
- payload UpdateDatasourcesRequest - Update datasource request
datasetsSetalldatasetconnectionsingroup
function datasetsSetalldatasetconnectionsingroup(string groupId, string datasetId, ConnectionDetails payload) returns Response|error
Updates all connections for the specified dataset from the specified workspace. This API only supports SQL DirectQuery datasets.
Note: This API is deprecated and no longer supported
Parameters
- groupId string - The workspace id
- datasetId string - The dataset id
- payload ConnectionDetails - The body
datasetsBindtogatewayingroup
function datasetsBindtogatewayingroup(string groupId, string datasetId, BindToGatewayRequest payload) returns Response|error
Binds the specified dataset from the specified workspace to the specified gateway with (optional) given set of datasource Ids. This only supports the On-Premises Data Gateway.
Parameters
- groupId string - The workspace id
- datasetId string - The dataset id
- payload BindToGatewayRequest - The bind to gateway request
datasetsGetgatewaydatasourcesingroup
function datasetsGetgatewaydatasourcesingroup(string groupId, string datasetId) returns GatewayDatasources|error
Returns a list of gateway datasources for the specified dataset from the specified workspace.
Return Type
- GatewayDatasources|error - OK
datasetsDiscovergatewaysingroup
Returns a list of gateways that the specified dataset from the specified workspace can be bound to.
datasetsTakeoveringroup
Transfers ownership over the specified dataset to the current authorized user.
importsGetimportsingroup
Returns a list of imports from the specified workspace.
Parameters
- groupId string - The workspace id
importsPostimportingroup
function importsPostimportingroup(string groupId, string datasetDisplayName, ImportInfo payload, string? nameConflict, boolean? skipReport) returns Import|error
Creates new content on the specified workspace from PBIX (Power BI Desktop), JSON, XLSX (Excel), RDL, or file path in OneDrive for Business.
Parameters
- groupId string - The workspace id
- datasetDisplayName string - The display name of the dataset should include file extension. Not supported when importing from OneDrive for Business. For importing or creating dataflows, this parameter should be hardcoded to model.json.
- payload ImportInfo - The import to post
- nameConflict string? (default ()) - Determines what to do if a dataset with the same name already exists. Default value is 'Ignore'.<br/>Only Abort and Overwrite are supported with Rdl files.<br/>Only Abort and GenerateUniqueName are supported with dataflow model.json files.
- skipReport boolean? (default ()) - Determines whether to skip report import, if specified value must be 'true'. Only supported for PBIX files.
importsGetimportingroup
Returns the specified import from the specified workspace.
importsCreatetemporaryuploadlocationingroup
function importsCreatetemporaryuploadlocationingroup(string groupId) returns TemporaryUploadLocation|error
Creates a temporary blob storage to be used to import large .pbix files larger than 1 GB and up to 10 GB.
Parameters
- groupId string - The workspace id
Return Type
reportsGetreportsingroup
Returns a list of reports from the specified workspace.
Parameters
- groupId string - The workspace id
reportsGetreportingroup
Returns the specified report from the specified workspace.
reportsDeletereportingroup
Deletes the specified report from the specified workspace.
reportsClonereportingroup
function reportsClonereportingroup(string groupId, string reportId, CloneReportRequest payload) returns Report|error
Clones the specified report from the specified workspace.
Parameters
- groupId string - The workspace id
- reportId string - The report id
- payload CloneReportRequest - Clone report parameters
reportsExportreportingroup
Exports the specified report from the specified workspace to a .pbix file.
reportsUpdatereportcontentingroup
function reportsUpdatereportcontentingroup(string groupId, string reportId, UpdateReportContentRequest payload) returns Report|error
Updates the specified report from the specified workspace to have the same content as the specified report in the request body.
Parameters
- groupId string - The workspace id
- reportId string - The report id
- payload UpdateReportContentRequest - UpdateReportContent parameters
reportsRebindreportingroup
function reportsRebindreportingroup(string groupId, string reportId, RebindReportRequest payload) returns Response|error
Rebinds the specified report from the specified workspace to the requested dataset.
Parameters
- groupId string - The workspace id
- reportId string - The report id
- payload RebindReportRequest - Rebind report parameters
reportsGetpagesingroup
Returns a list of pages within the specified report from the specified workspace.
reportsGetpageingroup
Returns the specified page within the specified report from the specified workspace.
Parameters
- groupId string - The workspace id
- reportId string - The report id
- pageName string - The page name
reportsGetdatasourcesingroup
function reportsGetdatasourcesingroup(string groupId, string reportId) returns Datasources|error
Returns a list of datasources for the specified RDL report from the specified workspace.
Return Type
- Datasources|error - OK
reportsUpdatedatasourcesingroup
function reportsUpdatedatasourcesingroup(string groupId, string reportId, UpdateRdlDatasourcesRequest payload) returns Response|error
Updates the datasources of the specified paginated report from the specified workspace. (Preview)
Parameters
- groupId string - The workspace id
- reportId string - The report id
- payload UpdateRdlDatasourcesRequest - Update RDL datasources request
reportsExporttofileingroup
function reportsExporttofileingroup(string groupId, string reportId, ExportReportRequest payload) returns Export|error
Exports the specified report from the specified workspace to the requested format.
Parameters
- groupId string - The workspace id
- reportId string - The report id
- payload ExportReportRequest - Export to file request parameters
reportsGetexporttofilestatusingroup
function reportsGetexporttofilestatusingroup(string groupId, string reportId, string exportId) returns Export|error
Returns the status of the Export to file job from the specified workspace.
Parameters
- groupId string - The workspace id
- reportId string - The report id
- exportId string - The export id
reportsGetfileofexporttofileingroup
function reportsGetfileofexporttofileingroup(string groupId, string reportId, string exportId) returns string|error
Returns the file of the Export to file job of the specified report from the specified group.
Parameters
- groupId string - The workspace id
- reportId string - The report id
- exportId string - The export id
dashboardsGetdashboardsingroup
function dashboardsGetdashboardsingroup(string groupId) returns Dashboards|error
Returns a list of dashboards from the specified workspace.
Parameters
- groupId string - The workspace id
Return Type
- Dashboards|error - OK
dashboardsAdddashboardingroup
function dashboardsAdddashboardingroup(string groupId, AddDashboardRequest payload) returns Dashboard|error
Creates a new empty dashboard on the specified workspace.
dashboardsGetdashboardingroup
Returns the specified dashboard from the specified workspace.
dashboardsGettilesingroup
Returns a list of tiles within the specified dashboard from the specified workspace.
dashboardsGettileingroup
function dashboardsGettileingroup(string groupId, string dashboardId, string tileId) returns Tile|error
Returns the specified tile within the specified dashboard from the specified workspace.
Parameters
- groupId string - The workspace id
- dashboardId string - The dashboard id
- tileId string - The tile id
dashboardsClonetileingroup
function dashboardsClonetileingroup(string groupId, string dashboardId, string tileId, CloneTileRequest payload) returns Tile|error
Clones the specified tile from the specified workspace.
Parameters
- groupId string - The workspace id
- dashboardId string - The dashboard id
- tileId string - The tile id
- payload CloneTileRequest - Clone tile parameters
reportsGeneratetokenforcreateingroup
function reportsGeneratetokenforcreateingroup(string groupId, GenerateTokenRequest payload) returns EmbedToken|error
Generates an embed token to allow report creation on the specified workspace based on the specified dataset.
This API is relevant only to 'App owns data' embed scenario. For more information about using this API, see Considerations when generating an embed token.
Return Type
- EmbedToken|error - OK
reportsGeneratetokeningroup
function reportsGeneratetokeningroup(string groupId, string reportId, GenerateTokenRequest payload) returns EmbedToken|error
Generates an embed token to view or edit the specified report from the specified workspace.
This API is relevant only to 'App owns data' embed scenario. For more information about using this API, see Considerations when generating an embed token.
Parameters
- groupId string - The workspace id
- reportId string - The report id
- payload GenerateTokenRequest - Generate token parameters
Return Type
- EmbedToken|error - OK
datasetsGeneratetokeningroup
function datasetsGeneratetokeningroup(string groupId, string datasetId, GenerateTokenRequest payload) returns EmbedToken|error
Generates an embed token to Embed Q&A based on the specified dataset from the specified workspace.
This API is relevant only to 'App owns data' embed scenario. For more information about using this API, see Considerations when generating an embed token.
Parameters
- groupId string - The workspace id
- datasetId string - The dataset id
- payload GenerateTokenRequest - Generate token parameters
Return Type
- EmbedToken|error - OK
dashboardsGeneratetokeningroup
function dashboardsGeneratetokeningroup(string groupId, string dashboardId, GenerateTokenRequest payload) returns EmbedToken|error
Generates an embed token to view the specified dashboard from the specified workspace.
This API is relevant only to the 'App owns data' embed scenario. For more information about using this API, see Considerations when generating an embed token.
Parameters
- groupId string - The workspace id
- dashboardId string - The dashboard id
- payload GenerateTokenRequest - Generate token parameters
Return Type
- EmbedToken|error - OK
tilesGeneratetokeningroup
function tilesGeneratetokeningroup(string groupId, string dashboardId, string tileId, GenerateTokenRequest payload) returns EmbedToken|error
Generates an embed token to view the specified tile from the specified workspace.
This API is relevant only to 'App owns data' embed scenario. Generates an embed token to view the specified tile from the specified workspace.
Parameters
- groupId string - The workspace id
- dashboardId string - The dashboard id
- tileId string - The tile id
- payload GenerateTokenRequest - Generate token parameters
Return Type
- EmbedToken|error - OK
appsGetapps
Returns a list of installed apps.
appsGetapp
Returns the specified installed app.
Parameters
- appId string - The app id
appsGetreports
Returns a list of reports from the specified app.
Parameters
- appId string - The app id
appsGetreport
Returns the specified report from the specified app.
appsGetdashboards
function appsGetdashboards(string appId) returns Dashboards|error
Returns a list of dashboards from the specified app.
Parameters
- appId string - The app id
Return Type
- Dashboards|error - OK
appsGetdashboard
Returns the specified dashboard from the specified app.
appsGettiles
Returns a list of tiles within the specified dashboard from the specified app.
appsGettile
Returns the specified tile within the specified dashboard from the specified app.
dataflowsGetdataflow
Exports the specified dataflow definition to a .json file.
dataflowsDeletedataflow
Deletes a dataflow from the CDS for Analytics storage, including its definition file and actual model.
dataflowsUpdatedataflow
function dataflowsUpdatedataflow(string groupId, string dataflowId, DataflowUpdateRequestMessage payload) returns Response|error
Update dataflow properties, capabilities and settings.
Parameters
- groupId string - The workspace id
- dataflowId string - The dataflow id
- payload DataflowUpdateRequestMessage - Patch dataflow properties, capabilities and settings
dataflowsRefreshdataflow
function dataflowsRefreshdataflow(string groupId, string dataflowId, RefreshRequest payload, string? processType) returns Response|error
Triggers a refresh for the specified dataflow. The only supported mail notification options are either in case of failure, or none. MailOnCompletion is not supported.
Parameters
- groupId string - The workspace id
- dataflowId string - The dataflow id
- payload RefreshRequest - Refresh request
- processType string? (default ()) - Type of refresh process to use.
dataflowsGetdataflowdatasources
function dataflowsGetdataflowdatasources(string groupId, string dataflowId) returns Datasources|error
Returns a list of datasources for the specified dataflow.
Return Type
- Datasources|error - OK
dataflowsGetdataflows
Returns a list of all dataflows from the specified workspace.
Parameters
- groupId string - The workspace id
dataflowsGetupstreamdataflowsingroup
function dataflowsGetupstreamdataflowsingroup(string groupId, string dataflowId) returns DependentDataflows|error
Returns a list of upstream dataflows for the specified dataflow.
Return Type
- DependentDataflows|error - OK
dataflowsUpdaterefreshschedule
function dataflowsUpdaterefreshschedule(string groupId, string dataflowId, RefreshScheduleRequest payload) returns Response|error
Creates or updates the specified dataflow refresh schedule configuration.
Parameters
- groupId string - The workspace id
- dataflowId string - The dataflow id
- payload RefreshScheduleRequest - The dataflow refresh schedule to create or update
dataflowsGetdataflowtransactions
function dataflowsGetdataflowtransactions(string groupId, string dataflowId) returns DataflowTransactions|error
Returns a list of transactions for the specified dataflow.
Return Type
dataflowsCanceldataflowtransaction
function dataflowsCanceldataflowtransaction(string groupId, string transactionId) returns DataflowTransactionStatus|error
Attempts to Cancel the specified transactions.
Return Type
gatewaysGetgateways
Returns a list of gateways for which the user is an admin.
gatewaysGetgateway
Returns the specified gateway.
Parameters
- gatewayId string - The gateway id
gatewaysGetdatasources
function gatewaysGetdatasources(string gatewayId) returns GatewayDatasources|error
Returns a list of datasources from the specified gateway.
Parameters
- gatewayId string - The gateway id
Return Type
- GatewayDatasources|error - OK
gatewaysCreatedatasource
function gatewaysCreatedatasource(string gatewayId, PublishDatasourceToGatewayRequest payload) returns GatewayDatasource|error
Creates a new datasource on the specified gateway.
Parameters
- gatewayId string - The gateway id
- payload PublishDatasourceToGatewayRequest - The datasource requested to create
Return Type
- GatewayDatasource|error - Created
gatewaysGetdatasource
function gatewaysGetdatasource(string gatewayId, string datasourceId) returns GatewayDatasource|error
Returns the specified datasource from the specified gateway.
Return Type
- GatewayDatasource|error - OK
gatewaysDeletedatasource
Deletes the specified datasource from the specified gateway.
gatewaysUpdatedatasource
function gatewaysUpdatedatasource(string gatewayId, string datasourceId, UpdateDatasourceRequest payload) returns Response|error
Updates the credentials of the specified datasource from the specified gateway.
Parameters
- gatewayId string - The gateway id
- datasourceId string - The datasource id
- payload UpdateDatasourceRequest - The update datasource request
gatewaysGetdatasourcestatus
Checks the connectivity status of the specified datasource from the specified gateway.
gatewaysGetdatasourceusers
function gatewaysGetdatasourceusers(string gatewayId, string datasourceId) returns DatasourceUsers|error
Returns a list of users who have access to the specified datasource.
Return Type
- DatasourceUsers|error - OK
gatewaysAdddatasourceuser
function gatewaysAdddatasourceuser(string gatewayId, string datasourceId, DatasourceUser payload) returns Response|error
Grants or updates the permissions required to use the specified datasource for the specified user.
Parameters
- gatewayId string - The gateway id
- datasourceId string - The datasource id
- payload DatasourceUser - The add user to datasource request
gatewaysDeletedatasourceuser
function gatewaysDeletedatasourceuser(string gatewayId, string datasourceId, string emailAdress) returns Response|error
Removes the specified user from the specified datasource.
Parameters
- gatewayId string - The gateway id
- datasourceId string - The datasource id
- emailAdress string - The user's email address or the service principal object id
groupsGetgroups
Returns a list of workspaces the user has access to.
Parameters
- filter string? (default ()) - Filters the results, based on a boolean condition
- top int? (default ()) - Returns only the first n results
- skip int? (default ()) - Skips the first n results
groupsCreategroup
function groupsCreategroup(GroupCreationRequest payload, boolean? workspaceV2) returns Group|error
Creates new workspace.
Parameters
- payload GroupCreationRequest - Create group request parameters
- workspaceV2 boolean? (default ()) - Preview feature: Create a workspace V2. The only supported value is true.
groupsDeletegroup
Deletes the specified workspace.
Parameters
- groupId string - The workspace id to delete
groupsGetgroupusers
function groupsGetgroupusers(string groupId) returns GroupUsers|error
Returns a list of users that have access to the specified workspace.
Parameters
- groupId string - The workspace id
Return Type
- GroupUsers|error - OK
groupsUpdategroupuser
Update the specified user permissions to the specified workspace.
groupsAddgroupuser
Grants the specified user permissions to the specified workspace.
groupsDeleteuseringroup
Deletes the specified user permissions from the specified workspace.
Parameters
- groupId string - The workspace id
- user string - The email address of the user or the service principal object id to delete
capacitiesGetcapacities
function capacitiesGetcapacities() returns Capacities|error
Returns a list of capacities the user has access to.
Return Type
- Capacities|error - OK
capacitiesGetworkloads
Returns the current state of the specified capacity workloads, if a workload is enabled also returns the maximum memory percentage that the workload can consume.
Parameters
- capacityId string - The capacity Id
capacitiesGetworkload
Returns the current state of a workload and if the workload is enabled also returns the maximum memory percentage that the workload can consume.
capacitiesPatchworkload
function capacitiesPatchworkload(string capacityId, string workloadName, PatchWorkloadRequest payload) returns Response|error
Changes the state of a specific workload to Enabled or Disabled. When enabling a workload the maximum memory percentage that the workload can consume must be set.
Parameters
- capacityId string - The capacity Id
- workloadName string - The name of the workload
- payload PatchWorkloadRequest - Patch workload parameters
capacitiesGetrefreshables
function capacitiesGetrefreshables(int top, string? expand, string? filter, int? skip) returns Refreshables|error
Returns a list of refreshables for all capacities of which the user has access to.
Parameters
- top int - Returns only the first n results.
- expand string? (default ()) - Expands related entities inline, receives a comma-separated list of data types. Supported: capacities and groups
- filter string? (default ()) - Filters the results based on a boolean condition
- skip int? (default ()) - Skips the first n results. Use with top to fetch results beyond the first 1000.
Return Type
- Refreshables|error - OK
capacitiesGetrefreshablesforcapacity
function capacitiesGetrefreshablesforcapacity(string capacityId, int top, string? expand, string? filter, int? skip) returns Refreshables|error
Returns a list of refreshables for the specified capacity the user has access to
Parameters
- capacityId string - The capacity id
- top int - Returns only the first n results.
- expand string? (default ()) - Expands related entities inline, receives a comma-separated list of data types. Supported: capacities and groups
- filter string? (default ()) - Filters the results based on a boolean condition
- skip int? (default ()) - Skips the first n results. Use with top to fetch results beyond the first 1000.
Return Type
- Refreshables|error - OK
capacitiesGetrefreshableforcapacity
function capacitiesGetrefreshableforcapacity(string capacityId, string refreshableId, string? expand) returns Refreshables|error
Returns the specified refreshable for the specified capacity the user has access to
Parameters
- capacityId string - The capacity id
- refreshableId string - The refreshable id
- expand string? (default ()) - Expands related entities inline, receives a comma-separated list of data types. Supported: capacities and groups
Return Type
- Refreshables|error - OK
groupsAssignmyworkspacetocapacity
function groupsAssignmyworkspacetocapacity(AssignToCapacityRequest payload) returns Response|error
Assigns "My Workspace" to the specified capacity.
Parameters
- payload AssignToCapacityRequest - Assign to capacity parameters
groupsAssigntocapacity
function groupsAssigntocapacity(string groupId, AssignToCapacityRequest payload) returns Response|error
Assigns the specified workspace to the specified capacity.
Parameters
- groupId string - The workspace id
- payload AssignToCapacityRequest - Assign to capacity parameters
groupsCapacityassignmentstatusmyworkspace
function groupsCapacityassignmentstatusmyworkspace() returns WorkspaceCapacityAssignmentStatus|error
Gets the status of "My Workspace" assignment to capacity operation.
Return Type
groupsCapacityassignmentstatus
function groupsCapacityassignmentstatus(string groupId) returns WorkspaceCapacityAssignmentStatus|error
Gets the status of the assignment to capacity operation of the specified workspace.
Parameters
- groupId string - The workspace id
Return Type
availablefeaturesGetavailablefeatures
function availablefeaturesGetavailablefeatures() returns AvailableFeatures|error
Returns a list of available features for the user
Return Type
- AvailableFeatures|error - OK
availablefeaturesGetavailablefeaturebyname
function availablefeaturesGetavailablefeaturebyname(string featureName) returns AvailableFeature|error
Returns the specified available feature for user by name.
Parameters
- featureName string - The feature name
Return Type
- AvailableFeature|error - OK
dataflowstorageaccountsGetdataflowstorageaccounts
function dataflowstorageaccountsGetdataflowstorageaccounts() returns DataflowStorageAccounts|error
Returns a list of dataflow storage accounts the user has access to.
Return Type
groupsAssigntodataflowstorage
function groupsAssigntodataflowstorage(string groupId, AssignToDataflowStorageRequest payload) returns Response|error
Assigns the specified workspace to the specified dataflow storage account.
Parameters
- groupId string - The workspace id
- payload AssignToDataflowStorageRequest - Assign to Power BI dataflow storage account parameters
workspaceinfoPostworkspaceinfo
function workspaceinfoPostworkspaceinfo(RequiredWorkspaces payload, boolean? lineage, boolean? datasourceDetails) returns ScanRequest|error
Initiate a call to receive metadata for the requested list of workspaces. (Preview)
Parameters
- payload RequiredWorkspaces - Required workspace IDs to get info for
- lineage boolean? (default ()) - Whether to return lineage info (upstream dataflows, tiles, datasource IDs)
- datasourceDetails boolean? (default ()) - Whether to return datasource details
Return Type
- ScanRequest|error - Accepted
workspaceinfoGetscanstatus
function workspaceinfoGetscanstatus(string scanId) returns ScanRequest|error
Gets scan status for the specified scan. (Preview)
Parameters
- scanId string - The scan id
Return Type
- ScanRequest|error - OK
workspaceinfoGetscanresult
function workspaceinfoGetscanresult(string scanId) returns WorkspaceInfoResponse|error
Gets scan result for the specified scan (should be called only after getting status Succeeded in the scan status API). Scan result will be available for up to 24 hours. (Preview)
Parameters
- scanId string - The scan id
Return Type
workspaceinfoGetmodifiedworkspaces
function workspaceinfoGetmodifiedworkspaces(string? modifiedSince) returns ModifiedWorkspaces|error
Gets a list of workspace IDs in the organization. When using modifiedSince, returns only the IDs of workspaces that had changed since the time specified in the modifiedSince parameter. If not provided, returns a list of all workspace IDs in the organization. modifiedSince parameter should range from 30 minutes to 30 days ago. Notice changes can take up to 30 minutes to take effect. (Preview)
Parameters
- modifiedSince string? (default ()) - Last modified date (must be in ISO 8601 compliant UTC format)
Return Type
- ModifiedWorkspaces|error - OK
capacitiesAssignworkspacestocapacity
function capacitiesAssignworkspacestocapacity(AssignWorkspacesToCapacityRequest payload) returns Response|error
Assigns the provided workspaces to the specified capacity.
Parameters
- payload AssignWorkspacesToCapacityRequest - Admin assign workspaces capacity parameters
capacitiesUnassignworkspacesfromcapacity
function capacitiesUnassignworkspacesfromcapacity(UnassignWorkspacesCapacityRequest payload) returns Response|error
Unassigns the provided workspaces from capacity.
Parameters
- payload UnassignWorkspacesCapacityRequest - Admin assign workspaces to shared capacity parameters
datasetsGetdatasetsasadmin
Returns a list of datasets for the organization.
Parameters
- filter string? (default ()) - Filters the results, based on a boolean condition
- top int? (default ()) - Returns only the first n results
- skip int? (default ()) - Skips the first n results
datasetsGetdatasourcesasadmin
function datasetsGetdatasourcesasadmin(string datasetId) returns Datasources|error
Returns a list of datasources for the specified dataset.
Parameters
- datasetId string - The dataset id
Return Type
- Datasources|error - OK
groupsGetgroupsasadmin
function groupsGetgroupsasadmin(int top, string? expand, string? filter, int? skip) returns Groups|error
Returns a list of workspaces for the organization.
Parameters
- top int - Returns only the first n results. This parameter is mandatory and must be in the range of 1-5000.
- expand string? (default ()) - Expands related entities inline, receives a comma-separated list of data types. Supported: users, reports, dashboards, datasets, dataflows, workbooks
- filter string? (default ()) - Filters the results based on a boolean condition
- skip int? (default ()) - Skips the first n results. Use with top to fetch results beyond the first 5000.
groupsUpdategroupasadmin
Updates the specified workspace properties.
groupsAdduserasadmin
Grants user permissions to the specified workspace.
groupsDeleteuserasadmin
Removes user permissions to the specified workspace.
Parameters
- groupId string - The workspace id
- user string - The user principal name (UPN) of the user to remove (usually the user's email).
groupsRestoredeletedgroupasadmin
function groupsRestoredeletedgroupasadmin(string groupId, GroupRestoreRequest payload) returns Response|error
Restores a deleted workspace.
Parameters
- groupId string - The workspace id
- payload GroupRestoreRequest - Details of the group restore request
dataflowsGetupstreamdataflowsingroupasadmin
function dataflowsGetupstreamdataflowsingroupasadmin(string groupId, string dataflowId) returns DependentDataflows|error
Returns a list of upstream dataflows for the specified dataflow.
Return Type
- DependentDataflows|error - OK
dashboardsGetdashboardsingroupasadmin
function dashboardsGetdashboardsingroupasadmin(string groupId, string? filter, int? top, int? skip) returns Dashboards|error
Returns a list of dashboards from the specified workspace.
Parameters
- groupId string - The workspace id
- filter string? (default ()) - Filters the results, based on a boolean condition
- top int? (default ()) - Returns only the first n results
- skip int? (default ()) - Skips the first n results
Return Type
- Dashboards|error - OK
reportsGetreportsingroupasadmin
function reportsGetreportsingroupasadmin(string groupId, string? filter, int? top, int? skip) returns Reports|error
Returns a list of reports from the specified workspace.
Parameters
- groupId string - The workspace id
- filter string? (default ()) - Filters the results, based on a boolean condition
- top int? (default ()) - Returns only the first n results
- skip int? (default ()) - Skips the first n results
datasetsGetdatasetsingroupasadmin
function datasetsGetdatasetsingroupasadmin(string groupId, string? filter, int? top, int? skip, string? expand) returns Datasets|error
Returns a list of datasets from the specified workspace.
Parameters
- groupId string - The workspace id
- filter string? (default ()) - Filters the results, based on a boolean condition
- top int? (default ()) - Returns only the first n results
- skip int? (default ()) - Skips the first n results
- expand string? (default ()) - Expands related entities inline
datasetsGetdatasettodataflowslinksingroupasadmin
function datasetsGetdatasettodataflowslinksingroupasadmin(string groupId) returns DatasetToDataflowLinksResponse|error
Returns a list of upstream dataflows for datasets from the specified workspace.
Parameters
- groupId string - The workspace id
Return Type
dataflowsGetdataflowsingroupasadmin
function dataflowsGetdataflowsingroupasadmin(string groupId, string? filter, int? top, int? skip) returns Dataflows|error
Returns a list of dataflows from the specified workspace.
Parameters
- groupId string - The workspace id
- filter string? (default ()) - Filters the results, based on a boolean condition
- top int? (default ()) - Returns only the first n results
- skip int? (default ()) - Skips the first n results
reportsGetreportsasadmin
Returns a list of reports for the organization.
Parameters
- filter string? (default ()) - Filters the results, based on a boolean condition
- top int? (default ()) - Returns only the first n results
- skip int? (default ()) - Skips the first n results
dashboardsGetdashboardsasadmin
function dashboardsGetdashboardsasadmin(string? expand, string? filter, int? top, int? skip) returns Dashboards|error
Returns a list of dashboards for the organization.
Parameters
- expand string? (default ()) - Expands related entities inline, receives a comma-separated list of data types. Supported: tiles
- filter string? (default ()) - Filters the results, based on a boolean condition
- top int? (default ()) - Returns only the first n results
- skip int? (default ()) - Skips the first n results
Return Type
- Dashboards|error - OK
dashboardsGettilesasadmin
Returns a list of tiles within the specified dashboard.
Parameters
- dashboardId string - The dashboard id
importsGetimportsasadmin
function importsGetimportsasadmin(string? expand, string? filter, int? top, int? skip) returns Imports|error
Returns a list of imports for the organization.
Parameters
- expand string? (default ()) - Expands related entities inline
- filter string? (default ()) - Filters the results, based on a boolean condition
- top int? (default ()) - Returns only the first n results
- skip int? (default ()) - Skips the first n results
adminGetpowerbiencryptionkeys
function adminGetpowerbiencryptionkeys() returns TenantKeys|error
Returns the encryption keys for the tenant.
Return Type
- TenantKeys|error - OK
adminAddpowerbiencryptionkey
function adminAddpowerbiencryptionkey(TenantKeyCreationRequest payload) returns TenantKey|error
Adds an encryption key for Power BI workspaces assigned to a capacity.
Parameters
- payload TenantKeyCreationRequest - Tenant key information
adminRotatepowerbiencryptionkey
function adminRotatepowerbiencryptionkey(string tenantKeyId, TenantKeyRotationRequest payload) returns TenantKey|error
Rotate the encryption key for Power BI workspaces assigned to a capacity.
Parameters
- tenantKeyId string - Tenant key id
- payload TenantKeyRotationRequest - Tenant key information
adminGetcapacitiesasadmin
function adminGetcapacitiesasadmin(string? expand) returns Capacities|error
Returns a list of capacities for the organization.
Parameters
- expand string? (default ()) - Expands related entities inline
Return Type
- Capacities|error - OK
adminPatchcapacityasadmin
function adminPatchcapacityasadmin(string capacityId, CapacityPatchRequest payload) returns Response|error
Changes the specific capacity information. Currently, only supports changing the capacity encryption key
Parameters
- capacityId string - The capacity Id
- payload CapacityPatchRequest - Patch capacity information
adminGetrefreshables
function adminGetrefreshables(int top, string? expand, string? filter, int? skip) returns Refreshables|error
Returns a list of refreshables for the organization within a capacity
Parameters
- top int - Returns only the first n results.
- expand string? (default ()) - Expands related entities inline, receives a comma-separated list of data types. Supported: capacities and groups
- filter string? (default ()) - Filters the results based on a boolean condition
- skip int? (default ()) - Skips the first n results. Use with top to fetch results beyond the first 1000.
Return Type
- Refreshables|error - OK
adminGetrefreshablesforcapacity
function adminGetrefreshablesforcapacity(string capacityId, int top, string? expand, string? filter, int? skip) returns Refreshables|error
Returns a list of refreshables for the specified capacity the user has access to
Parameters
- capacityId string - The capacity id
- top int - Returns only the first n results.
- expand string? (default ()) - Expands related entities inline, receives a comma-separated list of data types. Supported: capacities and groups
- filter string? (default ()) - Filters the results based on a boolean condition
- skip int? (default ()) - Skips the first n results. Use with top to fetch results beyond the first 1000.
Return Type
- Refreshables|error - OK
adminGetrefreshableforcapacity
function adminGetrefreshableforcapacity(string capacityId, string refreshableId, string? expand) returns Refreshables|error
Returns the specified refreshable for the specified capacity the user has access to
Parameters
- capacityId string - The capacity id
- refreshableId string - The refreshable id
- expand string? (default ()) - Expands related entities inline, receives a comma-separated list of data types. Supported: capacities and groups
Return Type
- Refreshables|error - OK
dataflowsGetdataflowsasadmin
Returns a list of dataflows for the organization.
Parameters
- filter string? (default ()) - Filters the results, based on a boolean condition
- top int? (default ()) - Returns only the first n results
- skip int? (default ()) - Skips the first n results
dataflowsExportdataflowasadmin
Exports the specified dataflow definition to a .json file.
Parameters
- dataflowId string - The dataflow id
dataflowsGetdataflowdatasourcesasadmin
function dataflowsGetdataflowdatasourcesasadmin(string dataflowId) returns Datasources|error
Returns a list of datasources for the specified dataflow.
Parameters
- dataflowId string - The dataflow id
Return Type
- Datasources|error - OK
embedtokenGeneratetoken
function embedtokenGeneratetoken(GenerateTokenRequestV2 payload) returns EmbedToken|error
Generates an embed token for multiple reports, datasets and target workspaces. Reports and datasets do not have to be related. The binding of a report to a dataset can be done during embedding. Creating a report can only be done in workspaces specified in targetWrokspaces.
This API is relevant only to 'App owns data' embed scenario. For more information about using this API, see Considerations when generating an embed token.
Parameters
- payload GenerateTokenRequestV2 - Generate token parameters
Return Type
- EmbedToken|error - OK
adminGetactivityevents
function adminGetactivityevents(string? startDateTime, string? endDateTime, string? continuationToken, string? filter) returns ActivityEventResponse|error
Returns a list of audit activity events for a tenant.
Parameters
- startDateTime string? (default ()) - Start date and time of the window for audit event results. Must be in ISO 8601 compliant UTC format.
- endDateTime string? (default ()) - End date and time of the window for audit event results. Must be in ISO 8601 compliant UTC format.
- continuationToken string? (default ()) - Token required to get the next chunk of the result set
- filter string? (default ()) - Filters the results based on a boolean condition, using 'Activity', 'UserId', or both properties. Supports only 'eq' and 'and' operators.
Return Type
informationprotectionRemovelabelsasadmin
function informationprotectionRemovelabelsasadmin(InformationProtectionArtifactsChangeLabel payload) returns InformationProtectionChangeLabelResponse|error
Remove sensitivity labels from artifacts by artifact ID.
Parameters
- payload InformationProtectionArtifactsChangeLabel - Composite of artifact Id lists per Type.
Return Type
informationprotectionSetlabelsasadmin
function informationprotectionSetlabelsasadmin(InformationProtectionChangeLabelDetails payload) returns InformationProtectionChangeLabelResponse|error
Set sensitivity labels on content in Power BI by artifact ID.
Parameters
- payload InformationProtectionChangeLabelDetails - Set label details.
Return Type
templateappsCreateinstallticket
function templateappsCreateinstallticket(CreateInstallTicketRequest payload) returns InstallTicket|error
Generates an installation ticket for Template Apps automated install flow.
Parameters
- payload CreateInstallTicketRequest - Create Install Ticket parameters
Return Type
- InstallTicket|error - OK
reportsTakeoveringroup
Transfers ownership over the specified paginated report datasources to the current authorized user.
Records
power.bi: ActivityEventResponse
Odata response wrapper for audit activity events list
Fields
- activityEventEntities Object[]? - The activity event entities
- continuationUri string? - Uri to get the next chunk of the result set
- continuationToken string? - Token to get the next chunk of the result set
power.bi: AddDashboardRequest
Power BI add dashboard request
Fields
- name string - The name of the new dashboard
power.bi: AdditionalFeatureInfo
Additional feature information
Fields
- Usage int? - The token generation usage (in %) from the limitation on shared capacity
power.bi: App
A Power BI Installed App
Fields
- id string - The app id
- name string? - The app name
- description string? - The app description
- lastUpdate string? - The last time the app was updated
- publishedBy string? - The app publisher
power.bi: Apps
Odata response wrapper for a Power BI installed App list
Fields
- value App[]? - The installed apps
power.bi: ArtifactId
Unique artifact ID: uuid format for dashboards/reports/dataflows.
Fields
- id string - ID
power.bi: ArtifactStringId
Unique artifact ID: string format (can be uuid) for datasets.
Fields
- id string - ID
power.bi: AssignToCapacityRequest
Power BI assign to capacity request
Fields
- capacityId string - The capacity id. To unassign from capacity, use Empty Guid (00000000-0000-0000-0000-000000000000).
power.bi: AssignToDataflowStorageRequest
Power BI assign to Power BI dataflow storage account request
Fields
- dataflowStorageId string - The Power BI dataflow storage account id. To unassign the specified workspace from a Power BI dataflow storage account, an empty GUID (00000000-0000-0000-0000-000000000000) should be provided as dataflowStorageId.
power.bi: AssignWorkspacesToCapacityRequest
Request body for assigning workspaces to a premium capacity as tenant admin
Fields
- capacityMigrationAssignments CapacityMigrationAssignment[] - Assignment contract for migrating workspaces to premium capacity as tenant admin
power.bi: AvailableFeature
Power BI available feature
Fields
- name string - The feature name
- state string - The feature state
- extendedState string - The feature extended state
- additionalInfo AdditionalFeatureInfo? - Additional feature information
power.bi: AvailableFeatures
Odata response wrapper for a Power BI available features list
Fields
- features AvailableFeature[]? - The available features list
power.bi: BindToGatewayRequest
The bind dataset to gateway request
Fields
- gatewayObjectId string - The gateway id
- datasourceObjectIds string[]? - datasourceObjectIds belonging to the gateway
power.bi: Capacities
Odata response wrapper for a Power BI capacity list
Fields
- value Capacity[]? - The Capacity List
power.bi: Capacity
A Power BI capacity
Fields
- id string - The capacity id
- displayName string? - The capacity display name
- admins string[]? - An array of capacity admins.
- sku string? - The capacity SKU.
- state string - The capacity state
- capacityUserAccessRight string - Access right user has on the capacity
- region string? - The Azure region where the capacity is provisioned
- tenantKeyId string? - The id of the encryption key (Only applicable for admin route)
- tenantKey TenantKey? - Encryption key information
power.bi: CapacityMigrationAssignment
Assignment contract for migrating workspaces to shared capacity as tenant admin
Fields
- workspacesToAssign string[] - Workspaces to be migrated to shared capacity
- targetCapacityObjectId string - Capacity id
power.bi: CapacityPatchRequest
Patch capacity request
Fields
- tenantKeyId string? - The id of the encryption key
power.bi: ChangeLabelStatus
Artifact ID with information protection label status
Fields
- id string - Unique artifact Id, uuid format for dashboard/report/dataflow, and string format for dataset.
- status string - Indicates the result of the label change operation
power.bi: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
power.bi: CloneReportRequest
Power BI clone report request
Fields
- name string - The new report name
- targetWorkspaceId string? - Optional parameter for specifying the target workspace id. Empty Guid (00000000-0000-0000-0000-000000000000) indicates 'My Workspace'. <br/>If not provided, the new report will be cloned within the same workspace as the source report.
- targetModelId string? - Optional parameter for specifying the target associated dataset id. <br/>If not provided, the new report will be associated with the same dataset as the source report
power.bi: CloneTileRequest
Power BI clone tile request
Fields
- targetDashboardId string - The target dashboard id
- targetWorkspaceId string? - Optional parameter for specifying the target workspace id. Empty Guid (00000000-0000-0000-0000-000000000000) indicates 'My Workspace'. <br/>If not provided, tile will be cloned within the same workspace as the source tile.
- targetReportId string? - Optional parameter <br/>When cloning a tile linked to a report, pass the target report id to rebind the new tile to a different report.
- targetModelId string? - Optional parameter <br/>When cloning a tile linked to a dataset, pass the target model id to rebind the new tile to a different dataset.
- positionConflictAction string? - Optional parameter for specifying the action in case of position conflict. <br/>If not provided, 'Tail' is used. <br/>If there is no conflict, clone tile to same position as in source.
power.bi: Column
A dataset column
Fields
- name string - The column name
- dataType string - The column data type
- formatString string? - (Optional) The format of the column as specified in FORMAT_STRING
- sortByColumn string? - (Optional) String name of a column in the same table to be used to order the current column
- dataCategory string? - (Optional) String value to be used for the data category which describes the data within this column
- isHidden boolean? - (Optional) Property indicating if the column is hidden from view. Default is false.
- summarizeBy string? - (Optional) Aggregate Function to use for summarizing this column
power.bi: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
power.bi: ConnectionDetails
Connection string wrapper.
Fields
- connectionString string - A dataset connection string.
power.bi: CreateDatasetRequest
A Power BI dataset
Fields
- name string - The dataset name
- tables Table[] - The dataset tables.
- relationships Relationship[]? - The dataset relationships.
- datasources Datasource[]? - The datasources associated with this dataset.
- defaultMode string? - The dataset mode or type.
power.bi: CreateInstallTicketRequest
Power BI Create Install Ticket Request
Fields
- installDetails TemplateAppInstallDetails[]? - List of install details
power.bi: CredentialDetails
The credential details
Fields
- credentials string - The credentials. Depends on the 'credentialType' value. See Update Datasource Examples.
- credentialType string - The credential type
- encryptedConnection string - User input for this attribute is currently ignored. Today, regardless of the provided value, we always try to establish an encrypted connection first but fall back to an unencrypted option in case of a failure. <br/>This will be fixed for the following data source types with the June 2019 gateway release causing a value of Encrypted to try only an encrypted connection and NotEncrypted with an unencrypted connection and there would be no fallback behavior <ul><li>Impala</li><li>MySql</li><li>DB2</li><li>Netezza</li><li>PostgreSQL</li><li>Sybase</li><li>Teradata</li><li>GoogleBigQuery</li><li>Amazon Redshift</li></ul>
- encryptionAlgorithm string - The encryption algorithm. For cloud datasource, use 'None'. For on-premises datasource, use gateway public key with 'RSA-OAEP' algorithm.
- privacyLevel string - The privacy level. Relevant when combining data from multiple sources.
- useCallerAADIdentity boolean? - Should the caller's AAD identity be used for OAuth2 credentials configuration
- useEndUserOAuth2Credentials boolean? - Should the end-user OAuth2 credentials be used for connecting to the datasource in DirectQuery mode. Only supported for Direct Query to SQL Azure.
power.bi: Dashboard
A Power BI dashboard. Below is a list of properties that may be returned for a dashboard. Only a subset of the properties will be returned depending on the API called, the caller permissions and the availability of the data in the Power BI database.
Fields
- id string - The dashboard id
- displayName string? - The dashboard display name
- isReadOnly boolean? - Is ReadOnly dashboard
- embedUrl string? - The dashboard embed url
- tiles Tile[]? - The tiles that belong to the dashboard.
- dataClassification string? - The data classification tag of the dashboard
- sensitivityLabel SensitivityLabel? - Artifact sensitivity label info
power.bi: Dashboards
Odata response wrapper for a Power BI dashboard collection
Fields
- value Dashboard[]? - The dashboard collection
power.bi: Dataflow
The metadata of a dataflow. Below is a list of properties that may be returned for a dataflow. Only a subset of the properties will be returned depending on the API called, the caller permissions and the availability of the data in the Power BI database.
Fields
- objectId string - The dataflow id
- name string? - The dataflow name
- description string? - The dataflow description
- modelUrl string? - A URL to the dataflow definition file (model.json)
- configuredBy string? - The dataflow owner
- modifiedBy string? - The user that modified this dataflow
- endorsementDetails EndorsementDetails? - Power BI endorsement details
- modifiedDateTime string? - modification date time
- datasourceUsages DatasourceUsage[]? - Datasource usages
- upstreamDataflows DependentDataflow[]? - Upstream Dataflows
- sensitivityLabel SensitivityLabel? - Artifact sensitivity label info
power.bi: Dataflows
Odata response wrapper for a dataflow metadata list
Fields
- value Dataflow[]? - The dataflow metadata List
power.bi: DataflowStorageAccount
A Power BI dataflow storage account
Fields
- id string - The Power BI dataflow storage account id
- name string? - The Power BI dataflow storage account name
- isEnabled boolean - Indicates if workspaces can be assigned to this storage account
power.bi: DataflowStorageAccounts
Odata response wrapper for Power BI dataflow storage account list
Fields
- value DataflowStorageAccount[]? - The Power BI dataflow storage account list
power.bi: DataflowTransaction
A Power BI dataflow transaction
Fields
- id string - The transaction id
- refreshType string? - The type of refresh transaction
- startTime string? - Start time of the transaction
- endTime string? - End time of the transaction
- status string? - Status of the transaction
power.bi: DataflowTransactions
A dataflow transaction odata list wrapper
Fields
- value DataflowTransaction[]? - The dataflow transactions
power.bi: DataflowTransactionStatus
Status of dataflow refresh transaction
Fields
- transactionId string? - Transaction id
- status string? - Status of transaction
power.bi: DataflowUpdateRequestMessage
Request payload for updating dataflow information
Fields
- name string? - New name of the dataflow
- description string? - New description for the dataflow
- allowNativeQueries boolean? - Allow native queries
- computeEngineBehavior string? - Compute Engine Behavior
power.bi: Dataset
A Power BI dataset. Below is a list of properties that may be returned for a dataset. Only a subset of the properties will be returned depending on the API called, the caller permissions and the availability of the data in the Power BI database.
Fields
- id string - The dataset id
- name string? - The dataset name
- configuredBy string? - The dataset owner
- addRowsAPIEnabled boolean? - Whether the dataset allows adding new rows
- webUrl string? - The dataset web url
- IsRefreshable boolean? - Can this dataset be refreshed
- IsEffectiveIdentityRequired boolean? - Whether the dataset requires an effective identity. This indicates that you must send an effective identity using the GenerateToken API.
- IsEffectiveIdentityRolesRequired boolean? - Whether RLS is defined inside the PBIX file. This indicates that you must specify a role.
- IsOnPremGatewayRequired boolean? - Dataset requires an On-premises Data Gateway
- Encryption Encryption? - Encryption information for a dataset
- CreatedDate string? - DateTime of creation of this dataset
- ContentProviderType string? - The content provider type for the dataset
- CreateReportEmbedURL string? - The dataset create report embed url
- QnaEmbedURL string? - The dataset qna embed url
- description string? - The dataset description
- endorsementDetails EndorsementDetails? - Power BI endorsement details
- datasourceUsages DatasourceUsage[]? - Datasource usages
- upstreamDataflows DependentDataflow[]? - Upstream Dataflows
- sensitivityLabel SensitivityLabel? - Artifact sensitivity label info
power.bi: Datasets
A dataset odata list wrapper
Fields
- value Dataset[]? - The datasets
power.bi: DatasetToDataflowLinkResponse
A Power BI dataset to dataflow link
Fields
- datasetObjectId string? - The dataset object id
- dataflowObjectId string? - The dataflow object id
- workspaceObjectId string? - The workspace object id
power.bi: DatasetToDataflowLinksResponse
Odata response wrapper for a Power BI dataset to dataflow links list
Fields
- value DatasetToDataflowLinkResponse[]? - The dataset to dataflow links
power.bi: Datasource
A Power BI datasource
Fields
- name string? - (Deprecated) The datasource name. Available only for DirectQuery.
- connectionString string? - (Deprecated) The datasource connection string. Available only for DirectQuery.
- datasourceType string? - The datasource type
- connectionDetails DatasourceConnectionDetails? - A Power BI datasource connection details
- gatewayId string? - The bound gateway id. Empty when not bound to a gateway.
- datasourceId string? - The bound datasource id. Empty when not bound to a gateway.
power.bi: DatasourceConnectionDetails
A Power BI datasource connection details
Fields
- server string? - The connection server.
- database string? - The connection database.
- url string? - The connection url
power.bi: Datasources
Odata response wrapper for a Power BI datasource collection
Fields
- value Datasource[]? - The datasource collection
power.bi: DatasourceUsage
Datasource usage
Fields
- datasourceInstanceId string - The datasource instance ID
power.bi: DatasourceUser
A Power BI user access right entry for datasource
Fields
- datasourceAccessRight string - The user access rights for the datasource.
- emailAddress string? - Email address of the user
- displayName string? - Display name of the principal
- principalType string? - The principal type
power.bi: DatasourceUsers
Odata response wrapper for a Power BI user access right for datasource List
Fields
- value DatasourceUser[]? - The user access right for datasource List
power.bi: DelegatedUser
Delegated user details. The user must be an existing user in Power BI and Azure AAD, who has signed in to Power BI during the last 3 months.
Fields
- emailAddress string - Delegated user email address.
power.bi: DependentDataflow
A Power BI Dependent dataflow
Fields
- targetDataflowId string? - The target dataflow id
- groupId string? - The target group id
power.bi: DependentDataflows
Odata response wrapper for a Power BI dependent dataflows list
Fields
- value DependentDataflow[]? - The dependent dataflows
power.bi: DirectQueryRefreshSchedule
A Power BI refresh schedule for DirectQuery or LiveConnection, specified by setting either the frequency or a combination of days and times.
Fields
- frequency int? - the refresh frequency in minutes, supported values are 15, 30, 60, 120, and 180.
- days string[]? - Days to execute the refresh
- times string[]? - Times to execute the refresh within each day
- localTimeZoneId string? - The Id of the Time zone to use. See Time Zone Info.
power.bi: DirectQueryRefreshScheduleRequest
Power BI refresh schedule request for DirectQuery or LiveConnection
Fields
- value DirectQueryRefreshSchedule - A Power BI refresh schedule for DirectQuery or LiveConnection, specified by setting either the frequency or a combination of days and times.
power.bi: EffectiveIdentity
Defines the user identity and roles, for more details see this article
Fields
- username string - The effective username reflected by a token for applying RLS rules (For OnPrem model, username can be composed of alpha-numerical characters or any of the following characters '.', '-', '_', '!', '#', '^', '~', '\', '@', also username cannot contain spaces. For Cloud model, username can be composed of all ASCII characters. username must be up to 256 characters)
- datasets string[]? - An array of datasets for which this identity applies
- roles string[]? - An array of RLS roles reflected by a token when applying RLS rules (identity can contain up to 50 roles, role can be composed of any character besides ',' and must be up to 50 characters)
- customData string? - The value of customdata to be used for applying RLS rules. Only supported for live connections to Azure Analysis Services.
- identityBlob IdentityBlob? - A blob for specifying the identity. Only supported for datasets with Direct Query connection to SQL Azure
- reports string[]? - An array of reports for which this identity applies, Only supported for paginated reports
power.bi: EmbedToken
Power BI embed token
Fields
- token string - Embed token
- tokenId string - Unique token Id. Can be used to correlate operations that use this token with the generate operation through audit logs.
- expiration string - Expiration time of token. In UTC.
power.bi: Encryption
Encryption information for a dataset
Fields
- EncryptionStatus string? - Dataset encryption status
power.bi: EndorsementDetails
Power BI endorsement details
Fields
- endorsement string? - The endorsement status
- certifiedBy string? - The user that certified the artifact
power.bi: Export
An object describing Export to file details and current state
Fields
- id string? - The Export to file job ID
- createdDateTime string? - The start time of the Export to file job
- lastActionDateTime string? - The time of last change in the Export to file job
- reportId string? - The ID of the exported report
- reportName string? - The name of the exported report
- status string? - The current state of the Export to file job
- percentComplete int? - Indicate job progress as percentage
- resourceLocation string? - The URL for retrieving the exported file
- ResourceFileExtension string? - The extension of the exported file
- expirationTime string? - The expiration time of the URL
power.bi: ExportFilter
A filter to be applied during the export operation
Fields
power.bi: ExportReportPage
A single page configuration for the export request
Fields
- pageName string - The page name
- visualName string? - Visual name to be exported. Should be provided in case only a single visual from this page is exported
- bookmark PageBookmark? - The bookmark to apply on a single page. Provide name or state, but not both.
power.bi: ExportReportRequest
The Export to file request
Fields
- format string - Export to file format
- powerBIReportConfiguration PowerBIReportExportConfiguration? - Power BI report Export to file configuration
- paginatedReportConfiguration PaginatedReportExportConfiguration? - Paginated report Export to file configuration
power.bi: ExportReportSettings
Export to file request settings
Fields
- locale string? - The locale to apply
- includeHiddenPages boolean? - A flag indicating whether to include hidden pages when exporting the entire report (when passing specific pages this property will be ignored). If not provided, the default behavior is to exclude hidden pages
power.bi: Gateway
A Power BI gateway
Fields
- id string - The gateway id
- name string? - The gateway name
- 'type string? - The gateway type
- gatewayAnnotation string? - Gateway metadata in json format
- publicKey GatewayPublicKey? - A Power BI gateway public key
- gatewayStatus string? - The gateway connectivity status
power.bi: GatewayDatasource
A Power BI gateway datasource
Fields
- id string - The unique id for this datasource
- gatewayId string - The associated gateway id
- datasourceName string? - The name of the datasource
- datasourceType string? - The type of the datasource
- connectionDetails string? - Connection details in json format
- credentialType string - Type of the datasource credentials
power.bi: GatewayDatasources
Odata response wrapper for a Power BI gateway datasource collection
Fields
- value GatewayDatasource[]? - List of gateway datasources
power.bi: GatewayPublicKey
A Power BI gateway public key
Fields
- exponent string? - The public key exponent
- modulus string? - The public key modulus
power.bi: Gateways
Odata response wrapper for a Power BI gateways list
Fields
- value Gateway[]? - The gateways
power.bi: GenerateTokenRequest
Power BI Generate Token Request
Fields
- accessLevel string? - Required access level for EmbedToken generation
- datasetId string? - Dataset id for report creation. Only applies when generating EmbedToken for report creation.
- allowSaveAs boolean? - Indicates an embedded report can be saved as a new report. Default value is 'false'. Only applies when generating EmbedToken for report embedding.
- identities EffectiveIdentity[]? - List of identities to use for RLS rules.
power.bi: GenerateTokenRequestV2
Power BI Generate Token Request V2
Fields
- datasets GenerateTokenRequestV2Dataset[]? - List of datasets
- reports GenerateTokenRequestV2Report[]? - List of reports
- targetWorkspaces GenerateTokenRequestV2TargetWorkspace[]? - List of workspaces Embed Token allows saving to
- identities EffectiveIdentity[]? - List of identities to use for RLS rules.
power.bi: GenerateTokenRequestV2Dataset
The dataset object for Generate Token Request V2.
Fields
- id string - Dataset Id
power.bi: GenerateTokenRequestV2Report
The report object for Generate Token Request V2.
Fields
- allowEdit boolean? - Indicates that the generated EmbedToken grand editing for this report
- id string - Report Id
power.bi: GenerateTokenRequestV2TargetWorkspace
The workspace object for Generate Token Request V2.
Fields
- id string - Workspace Id
power.bi: Group
A Power BI group
Fields
- id string - The workspace id
- name string? - The group name
- isReadOnly boolean? - Is the group read only
- isOnDedicatedCapacity boolean? - Is the group on dedicated capacity
- capacityId string? - The capacity id
- description string? - The group description. Available only for admin API calls.
- 'type string? - The type of group. Available only for admin API calls.
- state string? - The group state. Available only for admin API calls.
- users GroupUser[]? - The users that belong to the group, and their access rights. Available only for admin API calls.
- reports Report[]? - The reports that belong to the group. Available only for admin API calls.
- dashboards Dashboard[]? - The dashboards that belong to the group. Available only for admin API calls.
- datasets Dataset[]? - The datasets that belong to the group. Available only for admin API calls.
- dataflows Dataflow[]? - The dataflows that belong to the group. Available only for admin API calls.
- dataflowStorageId string? - The Power BI dataflow storage account id
- workbooks Workbook[]? - The workbooks that belong to the group. Available only for admin API calls.
power.bi: GroupCreationRequest
Power BI create a new group request
Fields
- name string - The name of the newly created group
power.bi: GroupRestoreRequest
Power BI request to restore a deleted group
Fields
- name string? - The name of the group to be restored.
- emailAddress string - The email address of the owner of the group to be restored.
power.bi: Groups
Odata response wrapper for a Power BI group list
Fields
- value Group[]? - The groups
power.bi: GroupUser
A Power BI user access right entry for workspace
Fields
- groupUserAccessRight string - Access rights user has for the workspace
- emailAddress string? - Email address of the user
- displayName string? - Display name of the principal
- principalType string? - The principal type
power.bi: GroupUsers
Odata response wrapper for a Power BI user access right for group List
Fields
- value GroupUser[]? - The user access right for group List
power.bi: IdentityBlob
A blob for specifying the identity. Only supported for datasets with Direct Query connection to SQL Azure
Fields
- value string - OAuth2 access token for SQL Azure
power.bi: Import
The import object
Fields
- id string - The import id
- name string? - The import name
- importState string? - The import upload state
- reports Report[]? - The reports associated with this import
- datasets Dataset[]? - The datasets associated with this import
- createdDateTime string? - Import creation time
- updatedDateTime string? - Import last update time
power.bi: ImportInfo
The information about the import
Fields
- filePath string? - The OneDrive for Business .xlsx file path to import, can be absolute or relative. .pbix files are not supported.
- connectionType string? - The import connection type for OneDrive for Business file
- fileUrl string? - The shared access signature (SAS) url of the temporary blob storage, used to import large .pbix files between 1 GB and 10 GB
power.bi: Imports
Odata response wrapper for a Power BI import collection
Fields
- value Import[]? - The imports collection
power.bi: InformationProtectionArtifactsChangeLabel
Composite of artifact IDs that will be used to update the information protection labels of those artifacts.
Fields
- dashboards ArtifactId[]? - List of unique dashboard IDs.
- reports ArtifactId[]? - List of unique report IDs.
- datasets ArtifactStringId[]? - List of unique dataset IDs.
- dataflows ArtifactId[]? - List of unique dataflow IDs.
power.bi: InformationProtectionChangeLabelDetails
Set label details.
Fields
- artifacts InformationProtectionArtifactsChangeLabel - Composite of artifact IDs that will be used to update the information protection labels of those artifacts.
- labelId string - Label ID (must be in the user’s policy).
- delegatedUser DelegatedUser? - Delegated user details. The user must be an existing user in Power BI and Azure AAD, who has signed in to Power BI during the last 3 months.
- assignmentMethod string? - Specifies whether the assigned label is to be regarded as having been set manually or as the result of automatic labeling. (default value is Standard).
power.bi: InformationProtectionChangeLabelResponse
Composite of artifact IDs and label change status.
Fields
- dashboards ChangeLabelStatus[]? - List of unique dashboard IDs with label change status.
- reports ChangeLabelStatus[]? - List of unique report IDs with label change status.
- dataflows ChangeLabelStatus[]? - List of unique dataflow IDs with label change status.
- datasets ChangeLabelStatus[]? - List of unique dataset IDs with label change status.
power.bi: InstallTicket
Power BI Template Apps automated install token
Fields
- ticket string - Install ticket
- ticketId string - Unique ticket Id. Can be used to correlate operations that use this ticket with the generate operation through audit logs.
- expiration string - Expiration time of token. In UTC.
power.bi: MashupParameter
Power BI dataset parameter
Fields
- name string - The parameter name
- 'type string - The parameter type
- currentValue string? - The parameter current value
- isRequired boolean - Is dataset parameter required
- suggestedValues string[]? - List of the parameter suggested values
power.bi: MashupParameters
Odata response wrapper for a Power BI dataset parameter list
Fields
- value MashupParameter[]? - The dataset parameter List
power.bi: Measure
A Power BI measure
Fields
- name string - The measure name
- expression string - A valid DAX expression
- formatString string? - (Optional) A string describing how the value should be formatted when it is displayed as specified in FORMAT_STRING
- description string? - (Optional) Measure description
- isHidden boolean? - (Optional) Is measure hidden
power.bi: ModifiedWorkspace
A modified workspace
Fields
- Id string - The workspace object ID.
power.bi: ModifiedWorkspaces
Odata response wrapper for modified workspaces list
Fields
- value ModifiedWorkspace[]? - The modified workspaces list
power.bi: Object
power.bi: Page
A Power BI report page
Fields
- name string? - The page name
- displayName string? - The page display name
- 'order int? - The page order
power.bi: PageBookmark
The bookmark to apply on a single page. Provide name or state, but not both.
Fields
- name string? - The bookmark name
- state string? - The bookmark state
power.bi: Pages
Odata response wrapper for a Power BI page collection
Fields
- value Page[]? - The page collection
power.bi: PaginatedReportExportConfiguration
Paginated report Export to file configuration
Fields
- identities EffectiveIdentity[]? - A single identity to use when exporting a report. Required when a report uses a Power BI dataset or an Azure AS datasource.
- formatSettings record {}? - Dictionary of format settings. The keys are the device info property names for the requested file format.
- parameterValues ParameterValue[]? - List of report parameters
power.bi: ParameterValue
Data contract for paginated report parameters
Fields
- name string? - The parameter name
- value string? - The parameter value
power.bi: PatchWorkloadRequest
Patch workload setting request
Fields
- state WorkloadState - The capacity workload state
- maxMemoryPercentageSetByUser int? - The memory percentage maximum Limit set by the user
power.bi: PostRowsRequest
Fields
- rows Object[]? - An array of data rows pushed to a dataset table
power.bi: PowerBIApiErrorResponseDetail
A Power BI error response details
Fields
- code string? - The error code.
- message string? - The error message.
- target string? - The error target.
power.bi: PowerBIReportExportConfiguration
Power BI report Export to file configuration
Fields
- settings ExportReportSettings? - Export to file request settings
- defaultBookmark PageBookmark? - The bookmark to apply on a single page. Provide name or state, but not both.
- reportLevelFilters ExportFilter[]? - List of report level filters to apply. Currently only one filter can be provided
- pages ExportReportPage[]? - List of pages to export and their properties. The same page may appear more than once with different visuals
- identities EffectiveIdentity[]? - List of identities to use for RLS rules
power.bi: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
power.bi: PublishDatasourceToGatewayRequest
A publish datasource to gateway request
Fields
- dataSourceType string - The datasource type
- connectionDetails string - The connection details
- credentialDetails CredentialDetails - The credential details
- dataSourceName string - The datasource name
power.bi: RdlDatasourceConnectionDetails
A paginated report datasource connection details.
Fields
- server string? - The connection server.
- database string? - The connection database.
power.bi: RebindReportRequest
Power BI rebind report request
Fields
- datasetId string - The new dataset for the rebound report. If the dataset resides in a different workspace than the report, a shared dataset will be created in the report's workspace
power.bi: Refresh
A Power BI refresh history entry
Fields
- refreshType string? - Type of refresh request
- startTime string? - DateTime of start
- endTime string? - DateTime of termination (may be empty if refresh is progress)
- serviceExceptionJson string? - Failure error code in json format (not empty only on error).
- status string? - 'Unknown' - Unknown completion state or refresh is in progress. endTime will be empty with this status. <br/>'Completed' - refresh completed successfully <br/> 'Failed' - Refresh failed. serviceExceptionJson will contain the error. <br/>'Disabled' - Refresh disabled by Selective Refresh.
- requestId string? - The identifier of the Refresh request. <br/>Please provide this identifier in all service requests
power.bi: Refreshable
A Power BI refreshable
Fields
- id string? - Object id of refreshable
- name string? - Display name of refreshable
- kind string? - The refreshable kind
- startTime string? - The start time of the window for which summary data exists
- endTime string? - The end time of the window for which summary data exists
- refreshCount int? - The number of refreshes within the summary time window
- refreshFailures int? - The number of refresh failures within the summary time window
- averageDuration decimal? - The average duration in seconds of a refresh within the summary time window
- medianDuration decimal? - The median duration in seconds of a refresh within the summary time window
- refreshesPerDay int? - The number of refreshes per day within the summary time window
- lastRefresh Refresh? - A Power BI refresh history entry
- refreshSchedule RefreshSchedule? - A Power BI refresh schedule for cached model
- configuredBy string[]? - Refreshable owners
- capacity Capacity? - A Power BI capacity
- group Group? - A Power BI group
power.bi: Refreshables
Power BI refreshable list
Fields
- value Refreshable[]? - The refreshables
power.bi: Refreshes
Odata response wrapper for a Power BI refresh history
Fields
- value Refresh[]? - The Refresh history list
power.bi: RefreshRequest
Power BI refresh request
Fields
- notifyOption string - Mail notification options (success and/or failure, or none)
power.bi: RefreshSchedule
A Power BI refresh schedule for cached model
Fields
- days string[]? - Days to execute the refresh
- times string[]? - Times to execute the refresh within each day
- enabled boolean? - Is the refresh enabled
- localTimeZoneId string? - The Id of the Time zone to use. See Time Zone Info.
- NotifyOption string? - Notification option at scheduled refresh termination
power.bi: RefreshScheduleRequest
Power BI refresh schedule request
Fields
- value RefreshSchedule - A Power BI refresh schedule for cached model
power.bi: Relationship
A relationship between tables in a dataset
Fields
- name string - The relationship name and identifier
- crossFilteringBehavior string(default "OneDirection") - The filter direction of the relationship
- fromTable string - The name of the foreign key table
- fromColumn string - The name of the foreign key column
- toTable string - The name of the primary key table
- toColumn string - The name of the primary key column
power.bi: Report
A Power BI report. Below is a list of properties that may be returned for a report. Only a subset of the properties will be returned depending on the API called, the caller permissions and the availability of the data in the Power BI database.
Fields
- id string - The report id
- name string? - The report name
- webUrl string? - The report web url
- embedUrl string? - The report embed url
- datasetId string? - The dataset id
- description string? - The report description
- createdBy string? - The report owner
- modifiedBy string? - The user that modified this report
- createdDateTime string? - The report created date time.
- modifiedDateTime string? - The report modified date time.
- endorsementDetails EndorsementDetails? - Power BI endorsement details
- sensitivityLabel SensitivityLabel? - Artifact sensitivity label info
- reportType string? - The report type
power.bi: Reports
Odata response wrapper for a Power BI report collection
Fields
- value Report[]? - The report collection
power.bi: RequiredWorkspaces
A required workspaces request
Fields
- workspaces string[]? - Required workspace IDs to be scaned. It should contain 1-100 workspaces.
power.bi: Row
A data row in a dataset
Fields
- id string? - The unique row id
power.bi: ScanRequest
A scan request
Fields
- id string? - The scan ID.
- createdDateTime string? - The scan created date time.
- status string? - The scan state.
- 'error PowerBIApiErrorResponseDetail? - A Power BI error response details
power.bi: SensitivityLabel
Artifact sensitivity label info
Fields
- labelId string - The sensitivity label ID
power.bi: SourceReport
Source from existing report
Fields
- sourceReportId string - source report id
- sourceWorkspaceId string? - source worksapce id
power.bi: Table
A dataset table
Fields
- name string - The table name
- columns Column[] - The column schema for this table
- rows Row[]? - The data rows within this table
- measures Measure[]? - The measures within this table
power.bi: Tables
Odata response wrapper for a Power BI table collection
Fields
- value Table[]? - The Power BI tables
power.bi: TemplateAppConfigurationRequest
Power BI Template App Automated install configuration. Dictionary of name-value pairs
Fields
- configuration record {}? - Configuration
power.bi: TemplateAppInstallDetails
Power BI Template App Install Details
Fields
- appId string - Unique application Id.
- packageKey string - Application version secure key
- ownerTenantId string - Application owner's tenant object Id
- config TemplateAppConfigurationRequest? - Power BI Template App Automated install configuration. Dictionary of name-value pairs
power.bi: TemporaryUploadLocation
Power BI update report content request
Fields
- Url string - The shared access signature (SAS) url for the temporary blob storage
- ExpirationTime string - The expiration time of the shared access signature (SAS) url
power.bi: TenantKey
Encryption key information
Fields
- id string? - The id of the encryption key
- name string? - The name of the encryption key
- keyVaultKeyIdentifier string? - Uri to the version of the Azure Key Vault key
- isDefault boolean? - Indicates that this key is set as default for the entire tenant. Any new capacity creation will inherit this key upon creation
- createdAt string? - Encryption key creation time
- updatedAt string? - Encryption key last update time
power.bi: TenantKeyCreationRequest
Add encryption key request
Fields
- name string? - The name of the encryption key
- keyVaultKeyIdentifier string? - Uri to the version of the Azure Key Vault key to be used
- isDefault boolean? - Indicates that this key is set as default for the entire tenant. Any new capacity creation will inherit this key upon creation
- activate boolean? - Indicates to activate any inactivated capacities to use this key for its encryption
power.bi: TenantKeyRotationRequest
Rotate encryption key request
Fields
- keyVaultKeyIdentifier string? - Uri to the version of the Azure Key Vault key to be used
power.bi: TenantKeys
Encryption keys information
Fields
- value TenantKey[]? - Encryption keys
power.bi: Tile
A Power BI tile
Fields
- id string - The tile id
- title string? - The dashboard display name
- rowSpan int? - number of rows a tile should span
- colSpan int? - number of columns a tile should span
- embedUrl string? - The tile embed url
- embedData string? - The tile embed data
- reportId string? - The report id. Available only for tiles created from a report.
- datasetId string? - The dataset id. Available only for tiles created from a report or using a dataset; for example, Q&A tiles.
power.bi: Tiles
Odata response wrapper for a Power BI tile collection
Fields
- value Tile[]? - The tile collection
power.bi: UnassignWorkspacesCapacityRequest
Request body for migrating workspaces to shared capacity as tenant admin
Fields
- workspacesToUnassign string[] - Workspaces to be migrated to shared capacity
power.bi: UpdateDatasourceConnectionRequest
Fields
- connectionDetails DatasourceConnectionDetails - A Power BI datasource connection details
- datasourceSelector Datasource? - A Power BI datasource
power.bi: UpdateDatasourceRequest
An update datasource to gateway request
Fields
- credentialDetails CredentialDetails - The credential details
power.bi: UpdateDatasourcesRequest
Fields
- updateDetails UpdateDatasourceConnectionRequest[] - The connection server
power.bi: UpdateMashupParameterDetails
Power BI dataset parameter update details
Fields
- name string - The parameter name
- newValue string? - The parameter new value
power.bi: UpdateMashupParametersRequest
Power BI dataset parameter update request
Fields
- updateDetails UpdateMashupParameterDetails[] - The dataset parameter list to update
power.bi: UpdateRdlDatasourceDetails
The new connection details and the target datasource name to be updated.
Fields
- connectionDetails RdlDatasourceConnectionDetails - A paginated report datasource connection details.
- datasourceName string - The target datasource name to be updated.
power.bi: UpdateRdlDatasourcesRequest
A paginated report datasources update request.
Fields
- updateDetails UpdateRdlDatasourceDetails[] - The paginated report datasources update details.
power.bi: UpdateReportContentRequest
Power BI update report content request
Fields
- sourceType string - The source type for the content update.
- sourceReport SourceReport - Source from existing report
power.bi: Workbook
A Power BI workbook
Fields
- name string? - The workbook name
- datasetId string? - DatasetId for workbooks. Only applies for workbooks that has an associated dataset.
power.bi: Workbooks
Power BI workbook list
Fields
- value Workbook[]? - The workbooks
power.bi: Workload
Capacity workload setting
Fields
- name string? - The workload name
- state WorkloadState - The capacity workload state
- maxMemoryPercentageSetByUser int? - The memory percentage maximum Limit set by the user
power.bi: Workloads
Odata response wrapper for capacity workload settings list
Fields
- value Workload[]? - The capacity workload settings list
power.bi: WorkspaceCapacityAssignmentStatus
Power BI workspace assignment status to capacity response
Fields
- status string - Workspace assignment status
- startTime string? - Start time of workspace assignment operation
- endTime string? - End time of workspace assignment operation
- capacityId string? - The capacity id
- activityId string? - The activity id of the acctual assignment operation, can be provided in case of assignment failures
power.bi: WorkspaceInfo
Workspace info details
Fields
- id string - The workspace object ID
- name string? - The workspace name
- description string? - The workspace description
- 'type string? - The workspace type
- state string? - The workspace state
- dataRetrievalState string? - The workspace data retrieval state
- isOnDedicatedCapacity boolean? - Whether the workspace is on dedicated capacity
- capacityId string? - The workspace capacity ID
- reports Report[]? - The reports associated with this workspace. The list of properties returned varies between APIs, thus you may not see them all as part of the API response.
- dashboards Dashboard[]? - The dashboards associated with this workspace. The list of properties returned varies between APIs, thus you may not see them all as part of the API response.
- datasets Dataset[]? - The datasets associated with this workspace. The list of properties returned varies between APIs, thus you may not see them all as part of the API response.
- dataflows Dataflow[]? - The dataflows associated with this workspace. The list of properties returned varies between APIs, thus you may not see them all as part of the API response.
power.bi: WorkspaceInfoResponse
Workspace info response
Fields
- workspaces WorkspaceInfo[]? - The workspace info associated with this scan
- datasourceInstances Datasource[]? - The datasources' instances associated with this scan
String types
power.bi: WorkloadState
WorkloadState
The capacity workload state
Import
import ballerinax/power.bi;
Metadata
Released date: about 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 7
Current verison: 6
Weekly downloads
Keywords
Business Intelligence/Analytics
Cost/Paid
Vendor/Microsoft
Contributors