pandadoc
Module pandadoc
API
Definitions
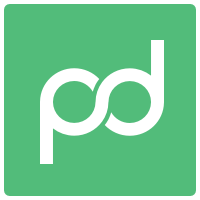
ballerinax/pandadoc Ballerina library
Overview
This is a generated connector from PandaDoc API version 4.3.0 OpenAPI Specification.
PandaDoc API spans a broad range of functionality to help you build incredible documents automation experiences inside your product. PandaDoc API is organized around REST. Our API has predictable resource-oriented URLs and uses standard HTTP response codes, authentication, and verbs.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a PandaDoc account.
- You can start using PandaDoc API with their free sandbox plan, which allows you to open all the available features.
- The sandbox API key you can generate on the Developer Dashboard with predefined rate limits.
Quickstart
To use the PandaDoc connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/pandadoc
module into the Ballerina project.
import ballerinax/pandadoc;
Step 2: Create a new connector instance
Create a pandadoc:AuthConfig
with the API-Key
obtained, and initialize the connector with it.
pandadoc:AuthConfig authConfig = { auth: { authorization: "API-Key <API Key>" } }; pandadoc:Client baseClient = check new Client(authConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to list opportunities, using the connector.
Gets a list of opportunities.
public function main() returns error? { pandadoc:DocumentListResponse response = check baseClient->listDocuments(); log:printInfo(response.toJsonString()); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
pandadoc: Client
This is a generated connector from PandaDoc API version 4.3.0 OpenAPI Specification. PandaDoc API spans a broad range of functionality to help you build incredible documents automation experiences inside your product. PandaDoc API is organized around REST. Our API has predictable resource-oriented URLs and uses standard HTTP response codes, authentication, and verbs.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
You can start using PandaDoc API with our free sandbox plan, which allows you to open all the available features.
The sandbox API key you can generate on the Developer Dashboard with predefined rate limits.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.pandadoc.com" - URL of the target service
accessToken
function accessToken(Oauth2AccessTokenBody payload) returns OAuth2AccessTokenResponse|error
Create/Refresh Access Token
Parameters
- payload Oauth2AccessTokenBody - Authentication request
Return Type
listDocuments
function listDocuments(string? completedFrom, string? completedTo, string? contactId, int? count, string? createdFrom, string? createdTo, boolean? deleted, string? id, string? folderUuid, string? formId, string? membershipId, string? metadata, string? modifiedFrom, string? modifiedTo, DocumentOrderingFieldsEnum? orderBy, int? page, string? q, DocumentStatusRequestEnum? status, DocumentStatusRequestEnum? statusNe, string? tag, string? templateId) returns DocumentListResponse|error
List documents
Parameters
- completedFrom string? (default ()) - Return results where the
date_completed
field (ISO 8601) is greater than or equal to this value.
- completedTo string? (default ()) - Return results where the
date_completed
field (ISO 8601) is less than or equal to this value.
- contactId string? (default ()) - Returns results where 'contact_id' is present in document as recipient or approver
- count int? (default ()) - Specify how many document results to return. Default is 50 documents, maximum is 100 documents.
- createdFrom string? (default ()) - Return results where the
date_created
field (ISO 8601) is greater than or equal to this value.
- createdTo string? (default ()) - Return results where the
date_created
field (ISO 8601) is less than this value.
- deleted boolean? (default ()) - Returns only the deleted documents.
- id string? (default ()) - Specify document's ID.
- folderUuid string? (default ()) - The UUID of the folder where the documents are stored.
- formId string? (default ()) - Specify the form used for documents creation. This parameter can't be used with template_id.
- membershipId string? (default ()) - Returns results where 'membership_id' is present in document as owner (should be member uuid)
- metadata string? (default ()) - Specify metadata to filter by in the format of
metadata_{metadata-key}={metadata-value}
such asmetadata_opportunity_id=2181432
. Themetadata_
prefix is always required.
- modifiedFrom string? (default ()) - Return results where the
date_modified
field (iso-8601) is greater than or equal to this value.
- modifiedTo string? (default ()) - Return results where the
date_modified
field (iso-8601) is less than this value.
- orderBy DocumentOrderingFieldsEnum? (default ()) - Specify the order of documents to return. Use
value
(for example,date_created
) for ASC and-value
(for example,-date_created
) for DESC.
- page int? (default ()) - Specify which page of the dataset to return.
- q string? (default ()) - Search query. Filter by document reference number (this token is stored on the template level) or name.
- status DocumentStatusRequestEnum? (default ()) - Specify the status of documents to return. * 0: document.draft * 1: document.sent * 2: document.completed * 3: document.uploaded * 4: document.error * 5: document.viewed * 6: document.waiting_approval * 7: document.approved * 8: document.rejected * 9: document.waiting_pay * 10: document.paid * 11: document.voided * 12: document.declined * 13: document.external_review
- statusNe DocumentStatusRequestEnum? (default ()) - Specify the status of documents to return (exclude). * 0: document.draft * 1: document.sent * 2: document.completed * 3: document.uploaded * 4: document.error * 5: document.viewed * 6: document.waiting_approval * 7: document.approved * 8: document.rejected * 9: document.waiting_pay * 10: document.paid * 11: document.voided * 12: document.declined * 13: document.external_review
- tag string? (default ()) - Search tag. Filter by document tag.
- templateId string? (default ()) - Specify the template used for documents creation. Parameter can't be used with form_id.
Return Type
createDocument
function createDocument(DocumentCreateRequest payload, string? editorVer) returns DocumentCreateResponse|error
Create document
Parameters
- payload DocumentCreateRequest - Use a PandaDoc template or an existing PDF to create a document.
- editorVer string? (default ()) - Set this parameter as
ev1
if you want to create a document from PDF with Classic Editor when both editors are enabled for the workspace.
Return Type
statusDocument
function statusDocument(string id) returns DocumentStatusResponse|error
Document status
Parameters
- id string - Specify document ID.
Return Type
deleteDocument
Delete document by id
Parameters
- id string - Document ID
changeDocumentStatus
function changeDocumentStatus(string id, DocumentStatusChangeRequest payload) returns Response|error
Document status change
Parameters
- id string - Specify document ID.
- payload DocumentStatusChangeRequest - Document status change request
detailsDocument
function detailsDocument(string id) returns DocumentDetailsResponse|error
Document details
Parameters
- id string - Document ID
Return Type
createDocumentLink
function createDocumentLink(string id, DocumentCreateLinkRequest payload) returns DocumentCreateLinkResponse|error
Create a Document Link
Parameters
- id string - Document ID
- payload DocumentCreateLinkRequest - Request to create document link
Return Type
sendDocument
function sendDocument(string id, DocumentSendRequest payload) returns DocumentSendResponse|error
Send Document
Return Type
downloadDocument
function downloadDocument(string id, string? watermarkColor, int? watermarkFontSize, float? watermarkOpacity, string? watermarkText) returns string|error
Document download
Parameters
- id string - Specify document ID.
- watermarkColor string? (default ()) - HEX code (for example
#FF5733
).
- watermarkFontSize int? (default ()) - Font size of the watermark.
- watermarkOpacity float? (default ()) - In range 0.0-1.0
- watermarkText string? (default ()) - Specify watermark text.
downloadProtectedDocument
Download document protected
Parameters
- id string - Specify document ID.
listLinkedObjects
function listLinkedObjects(string id) returns LinkedObjectListResponse|error
List Linked Objects
Parameters
- id string - Specify document ID.
Return Type
- LinkedObjectListResponse|error - Success response
createLinkedObject
function createLinkedObject(string id, LinkedObjectCreateRequest payload) returns LinkedObjectCreateResponse|error
Create Linked Object
Parameters
- id string - Specify document ID.
- payload LinkedObjectCreateRequest - Request to create linked object
Return Type
deleteLinkedObject
Delete Linked Object
listDocumentAttachments
function listDocumentAttachments(string id) returns DocumentAttachmentResponse[]|error
Document Attachment List
Parameters
- id string - Document UUID
Return Type
- DocumentAttachmentResponse[]|error - OK
createDocumentAttachment
function createDocumentAttachment(string id, DocumentAttachmentRequest payload) returns DocumentAttachmentResponse|error
Document Attachment Create
Parameters
- id string - Document UUID
- payload DocumentAttachmentRequest - Uploads attachment to document by using Multipart Form Data
Return Type
detailsDocumentAttachment
function detailsDocumentAttachment(string id, string attachmentId) returns DocumentAttachmentResponse|error
Document Attachment Details
Return Type
deleteDocumentAttachment
Document Attachment Delete
downloadDocumentAttachment
Document Attachment Download
transferDocumentOwnership
function transferDocumentOwnership(string id, DocumentTransferOwnershipRequest payload) returns Response|error
Update document ownership
Parameters
- id string - Specify document ID.
- payload DocumentTransferOwnershipRequest - Request to transfer document ownership
transferAllDocumentsOwnership
function transferAllDocumentsOwnership(DocumentTransferAllOwnershipRequest payload) returns Response|error
Transfer all documents ownership
Parameters
- payload DocumentTransferAllOwnershipRequest - Request to transfer all document ownerships
listContentLibraryItems
function listContentLibraryItems(string? q, string? id, boolean? deleted, string? folderUuid, int? count, int? page, string? tag) returns ContentLibraryItemListResponse|error
List Content Library Item
Parameters
- q string? (default ()) - Search query. Filter by content library item name.
- id string? (default ()) - Specify content library item ID.
- deleted boolean? (default ()) - Returns only the deleted content library items.
- folderUuid string? (default ()) - The UUID of the folder where the content library items are stored.
- count int? (default ()) - Specify how many content library items to return. Default is 50 content library items, maximum is 100 content library items.
- page int? (default ()) - Specify which page of the dataset to return.
- tag string? (default ()) - Search tag. Filter by content library item tag.
Return Type
detailsContentLibraryItem
function detailsContentLibraryItem(string id) returns ContentLibraryItemResponse|error
Details Content Library Item
Parameters
- id string - Content Library Item ID
Return Type
listTemplates
function listTemplates(string? q, boolean? shared, boolean? deleted, int? count, int? page, string? id, string? folderUuid, string[]? tag) returns TemplateListResponse|error
List Templates
Parameters
- q string? (default ()) - Optional search query. Filter by template name.
- shared boolean? (default ()) - Returns only the shared templates.
- deleted boolean? (default ()) - Optional. Returns only the deleted templates.
- count int? (default ()) - Optionally, specify how many templates to return. Default is 50 templates, maximum is 100 templates.
- page int? (default ()) - Optionally, specify which page of the dataset to return.
- id string? (default ()) - Optionally, specify template ID.
- folderUuid string? (default ()) - UUID of the folder where the templates are stored.
- tag string[]? (default ()) - Optional search tag. Filter by template tag.
Return Type
detailsTemplate
function detailsTemplate(string id) returns TemplateDetailsResponse|error
Details Template
Parameters
- id string - Template ID
Return Type
deleteTemplate
Delete Template
Parameters
- id string - Template ID
listForm
function listForm(int? count, int? page, string[]? status, string? orderBy, boolean? asc, string? name) returns FormListResponse|error
Forms
Parameters
- count int? (default ()) - Optionally, specify how many forms to return. Default is 50 forms, maximum is 100 forms.
- page int? (default ()) - Optionally, specify which page of the dataset to return.
- status string[]? (default ()) - Optionally, specify which status of the forms dataset to return.
- orderBy string? (default ()) - Optionally, specify the form dataset order to return.
- asc boolean? (default ()) - Optionally, specify sorting the result-set in ascending or descending order.
- name string? (default ()) - Specify the form name.
Return Type
- FormListResponse|error - List of forms
listDocumentFolders
function listDocumentFolders(string? parentUuid, int? count, int? page) returns DocumentsFolderListResponse|error
List Documents Folders
Parameters
- parentUuid string? (default ()) - The UUID of the folder containing folders. To list the folders located in the root folder, remove this parameter in the request.
- count int? (default ()) - Optionally, specify how many folders to return. Default is 50 folders, maximum is 100 folders.
- page int? (default ()) - Optionally, specify which page of the dataset to return.
Return Type
- DocumentsFolderListResponse|error - List of document folders
createDocumentFolder
function createDocumentFolder(DocumentsFolderCreateRequest payload) returns DocumentsFolderCreateResponse|error
Create Documents Folder
Parameters
- payload DocumentsFolderCreateRequest - Document folder details
Return Type
- DocumentsFolderCreateResponse|error - Success response
renameDocumentFolder
function renameDocumentFolder(string id, DocumentsFolderRenameRequest payload) returns DocumentsFolderRenameResponse|error
Rename Documents Folder
Parameters
- id string - The UUID of the folder that you are renaming.
- payload DocumentsFolderRenameRequest - Details to rename document folder
Return Type
listTemplateFolders
function listTemplateFolders(string? parentUuid, int? count, int? page) returns TemplatesFolderListResponse|error
List Templates Folders
Parameters
- parentUuid string? (default ()) - The UUID of the folder containing folders. To list the folders located in the root folder, remove this parameter in the request.
- count int? (default ()) - Optionally, specify how many folders to return. Default is 50 folders, maximum is 100 folders.
- page int? (default ()) - Optionally, specify which page of the dataset to return.
Return Type
createTemplateFolder
function createTemplateFolder(TemplatesFolderCreateRequest payload) returns TemplatesFolderCreateResponse|error
Create Templates Folder
Parameters
- payload TemplatesFolderCreateRequest - Details to create new folder for templates
Return Type
renameTemplateFolder
function renameTemplateFolder(string id, TemplatesFolderRenameRequest payload) returns TemplatesFolderRenameResponse|error
Rename Templates Folder
Parameters
- id string - The UUID of the folder which you are renaming.
- payload TemplatesFolderRenameRequest - Request to rename template folder
Return Type
listLogs
function listLogs(string? since, string? to, int? count, int? page, int[]? statuses, string[]? methods, string? search, string? environmentType) returns APILogListResponse|error
List API Log
Parameters
- since string? (default ()) - Determines a point in time from which logs should be fetched. Either a specific ISO 8601 datetime or a relative identifier such as "-90d" (for past 90 days).
- to string? (default ()) - Determines a point in time from which logs should be fetched. Either a specific ISO 8601 datetime or a relative identifier such as "-10d" (for past 10 days) or a special "now" value.
- count int? (default ()) - The amount of items on each page.
- page int? (default ()) - Page number of the results returned.
- statuses int[]? (default ()) - Returns only the predefined status codes. Allows 1xx, 2xx, 3xx, 4xx, and 5xx.
- methods string[]? (default ()) - Returns only the predefined HTTP methods. Allows GET, POST, PUT, PATCH, and DELETE.
- search string? (default ()) - Returns the results containing a string.
- environmentType string? (default ()) - Returns logs for production/sandbox.
Return Type
- APILogListResponse|error - OK
detailsLog
function detailsLog(string id) returns APILogDetailsResponse|error
Details API Log
Parameters
- id string - Log event id.
Return Type
listContacts
function listContacts() returns ContactListResponse|error
List contacts
Return Type
- ContactListResponse|error - OK
createContact
function createContact(ContactCreateRequest payload) returns ContactDetailsResponse|error
Create contact
Parameters
- payload ContactCreateRequest - Contact details
Return Type
detailsContact
function detailsContact(string id) returns ContactDetailsResponse|error
Get contact details by id
Parameters
- id string - Contact id.
Return Type
deleteContact
Delete contact by id
Parameters
- id string - Contact id.
updateContact
function updateContact(string id, ContactUpdateRequest payload) returns ContactDetailsResponse|error
Update contact by id
Return Type
listMembers
function listMembers() returns MemberListResponse|error
List members
Return Type
- MemberListResponse|error - OK
detailsCurrentMember
function detailsCurrentMember() returns MemberDetailsResponse|error
Current member details
Return Type
detailsMember
function detailsMember(string id) returns MemberDetailsResponse|error
Member details
Parameters
- id string - Membership id
Return Type
Records
pandadoc: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- authorization string - Represents API Key available in PandaDoc Developer Dashboard.
pandadoc: APILogDetailsResponse
Fields
- id string? -
- url string? -
- method string? -
- status int? -
- request_time string? -
- response_time string? -
- response_body record {}? -
- query_params_string string? -
- query_params_object record {}? -
- request_body record {}? -
- token_type string? -
- application string? -
- 'key string? -
- request_id string? -
- user_email string? -
- user_id string? -
pandadoc: APILogListResponse
Fields
- results ApiloglistresponseResults[]? -
pandadoc: ApiloglistresponseResults
Fields
- id string? -
- url string? -
- status int? -
- request_time string? -
- response_time string? -
pandadoc: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
pandadoc: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig|ApiKeysConfig - Provides Auth configurations needed when communicating with a remote HTTP endpoint.
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
pandadoc: ContactCreateRequest
Fields
- email string -
- first_name string? -
- last_name string? -
- company string? -
- job_title string? -
- phone string? -
- state string? -
- street_address string? -
- city string? -
- postal_code string? -
pandadoc: ContactDetailsResponse
Fields
- id string? -
- email string? -
- first_name string? -
- last_name string? -
- company string? -
- job_title string? -
- phone string? -
- state string? -
- street_address string? -
- city string? -
- postal_code string? -
pandadoc: ContactListResponse
Fields
- results ContactDetailsResponse[]? -
pandadoc: ContactUpdateRequest
Fields
- email string? -
- first_name string? -
- last_name string? -
- company string? -
- job_title string? -
- phone string? -
- state string? -
- street_address string? -
- city string? -
- postal_code string? -
pandadoc: ContentLibraryItemListResponse
Fields
- results ContentlibraryitemlistresponseResults[]? -
pandadoc: ContentlibraryitemlistresponseResults
Fields
- id string? -
- name string? -
- date_created string? -
- date_modified string? -
- 'version string? -
pandadoc: ContentLibraryItemResponse
Fields
- id string? -
- name string? -
- date_created string? -
- date_modified string? -
- created_by ContentlibraryitemresponseCreatedBy? -
- metadata record {}? -
- tokens record {}[]? -
- fields record {}[]? -
- pricing PricingTablesResponse? -
- tags string[]? -
- roles record {}[]? -
- 'version string? -
- content_placeholders record {}[]? -
- images record {}[]? -
pandadoc: ContentlibraryitemresponseCreatedBy
Fields
- id string? -
- email string? -
- first_name string? -
- last_name string? -
- avatar string? -
pandadoc: DocumentAttachmentRequest
Fields
- file string? - Binary file to be attached to a document
- 'source string? - URL link to the file to be attached to a document
- name string? - Optional name to set for uploaded file
pandadoc: DocumentAttachmentResponse
Fields
- uuid string? -
- date_created string? -
- created_by DocumentattachmentresponseCreatedBy? -
- name string? -
pandadoc: DocumentattachmentresponseCreatedBy
Fields
- id string? -
- email string? -
- first_name string? -
- last_name string? -
- avatar string? -
pandadoc: DocumentCreateByPdfRequest
Fields
- url string - Use a URL to specify the PDF. We support only URLs starting with https.
- recipients DocumentcreatebytemplaterequestRecipients[] - The list of recipients you're sending the document to. Every object must contain the email parameter. The
role
,first_name
andlast_name
parameters are optional. If therole
parameter passed, a person is assigned all fields matching their corresponding role. If not passed, a person will receive a read-only link to view the document. If thefirst_name
andlast_name
not passed the system 1. creates a new contact, if none exists with the givenemail
; or 2. gets the existing contact with the givenemail
that already exists.
- parse_form_fields boolean? - Set this parameter as
true
if you create a document from a PDF with form fields and asfalse
if you upload a PDF with field tags.
- name string? -
- tags string[]? - Mark your document with one or several tags.
- fields record {}? - If you are creating a document from a PDF with field tags, you can pass a list of the fields you'd like to pre-fill in the document. If you are creating a document from a PDF with form fields, list all the fields and provide the
role
parameter so that the fields are assigned to document recipients. You can provide empty value for the field so that it's not pre-filled: "value": "".
- metadata record {}? - You can pass arbitrary data in the key-value format to associate custom information with a document. This information is returned in any API requests for the document details by id.
pandadoc: DocumentCreateByTemplateRequest
Fields
- name string - Name the document you are creating.
- template_uuid string - The ID of a template you want to use. You can copy it from an in app template url such as
https://app.pandadoc.com/a/#/templates/{ID}/content
. A template ID is also obtained by listing templates.
- folder_uuid string? -
- recipients DocumentcreatebytemplaterequestRecipients[] - The list of recipients you're sending the document to. Every object must contain the email parameter. The
role
,first_name
andlast_name
parameters are optional. If therole
parameter passed, a person is assigned all fields matching their corresponding role. If not passed, a person will receive a read-only link to view the document. If thefirst_name
andlast_name
not passed the system 1. creates a new contact, if none exists with the givenemail
; or 2. gets the existing contact with the givenemail
that already exists.
- tokens DocumentcreatebytemplaterequestTokens[]? - You can pass a list of tokens/values to pre-fill tokens used in a template. Name is a token name in a template. Value is a real value you would like to replace a token with.
- fields record {}? - You can pass a list of fields/values to pre-fill fields used in a template. Note that the Signature field can't be pre-filled.
- metadata record {}? - You can pass arbitrary data in the key-value format to associate custom information with a document. This information is returned in any API requests for the document details by id.
- tags string[]? - Mark your document with one or several tags.
- images DocumentcreatebytemplaterequestImages[]? - You can pass a list of images to image blocks (one image in one block) for replacement.
- pricing_tables PricingTableRequest[]? - Information to construct or populate a pricing table can be passed when creating a document. All product information must be passed when creating a new document. Products stored in PandaDoc cannot be used to populate table rows at this time. Keep in mind that this is an array, so multiple table objects can be passed to a document. Make sure that "Automatically add products to this table" is enabled in the PandaDoc template pricing tables you wish to populate via API.
- content_placeholders DocumentcreatebytemplaterequestContentPlaceholders[]? - You may replace Content Library Item Placeholders with a few content library items each and pre-fill fields/variables values, pricing table items, and assign recipients to roles from there.
pandadoc: DocumentcreatebytemplaterequestContentLibraryItems
Fields
- id string -
- fields record {}? -
- pricing_tables PricingTableRequest[]? -
- recipients DocumentcreatebytemplaterequestRecipients[]? -
pandadoc: DocumentcreatebytemplaterequestContentPlaceholders
Fields
- content_library_items DocumentcreatebytemplaterequestContentLibraryItems[]? -
- block_id string -
pandadoc: DocumentcreatebytemplaterequestImages
Fields
- urls string[] -
- name string -
pandadoc: DocumentcreatebytemplaterequestRecipients
Fields
- email string -
- first_name string? -
- last_name string? -
- role string? -
- signing_order int? -
pandadoc: DocumentcreatebytemplaterequestTokens
Fields
- name string -
- value string -
pandadoc: DocumentCreateLinkRequest
Fields
- recipient string - The email address for the recipient you're creating a document link for.
- lifetime decimal? - Provide the number of seconds that a document link should be valid for. Default is 3600 seconds.
pandadoc: DocumentCreateLinkResponse
Fields
- id string? -
- expires_at string? -
pandadoc: DocumentCreateRequest
Fields
- name string? - Name the document you are creating.
- template_uuid string? - ID of the template you want to use. You can copy it from an in-app template URL such as
https://app.pandadoc.com/a/#/templates/{ID}/content
. A template ID is also obtained by listing templates.
- folder_uuid string? - ID of the folder where the created document should be stored.
- recipients DocumentcreaterequestRecipients[]? - The list of recipients you're sending the document to. Every object must contain the
email
parameter. Therole
,first_name
andlast_name
parameters are optional. If therole
parameter is passed, a person is assigned all fields matching their corresponding role. If a role was not passed, a person receives a read-only link to view the document. If thefirst_name
andlast_name
are not passed, the system does this 1. Creates a new contact, if none exists with the givenemail
; or 2. Gets the existing contact with the givenemail
that already exists.
- tokens DocumentcreatebytemplaterequestTokens[]? - You can pass a list of tokens/values to pre-fill tokens used in a template. Name is a token name in a template. Value is a real value you would like to replace a token with.
- fields record {}? - You can pass a list of fields/values to pre-fill fields used in a template. Please note Signature field can't be pre-filled.
- metadata record {}? - You can pass arbitrary data in the key-value format to associate custom information with a document. This information is returned in any API requests for the document details by id.
- tags string[]? - Mark your document with one or several tags.
- images DocumentcreaterequestImages[]? - You can pass a list of images to image blocks (one image in one block) for replacement.
- pricing_tables PricingTableRequest[]? - Information to construct or populate a pricing table can be passed when creating a document. All product information must be passed when creating a new document. Products stored in PandaDoc cannot be used to populate table rows at this time. Keep in mind that this is an array, so multiple table objects can be passed to a document.
- content_placeholders DocumentcreaterequestContentPlaceholders[]? - You may replace Content Library Item Placeholders with a few content library items each and pre-fill fields/variables values, pricing table items, and assign recipients to roles from there.
- url string? - Use a URL to specify the PDF. We support only URLs starting with https.
- parse_form_fields boolean? - Set this parameter as true if you create a document from a PDF with form fields and as false if you upload a PDF with field tags.
pandadoc: DocumentcreaterequestContentLibraryItems
Fields
- id string - Content library item id
- pricing_tables PricingTableRequest[]? -
- fields record {}? -
- recipients DocumentcreatebytemplaterequestRecipients[]? -
pandadoc: DocumentcreaterequestContentPlaceholders
Fields
- block_id string? - Content placeholder block id
- content_library_items DocumentcreaterequestContentLibraryItems[]? -
pandadoc: DocumentcreaterequestImages
Fields
- urls string[] -
- name string -
pandadoc: DocumentcreaterequestRecipients
Fields
- email string -
- first_name string? -
- last_name string? -
- role string? -
- signing_order int? -
pandadoc: DocumentCreateResponse
Fields
- id string? -
- name string? -
- status DocumentStatusEnum? -
- date_created string? -
- date_modified string? -
- expiration_date string? -
- uuid string? -
- links DocumentcreateresponseLinks[]? -
- info_message string? -
pandadoc: DocumentcreateresponseLinks
Fields
- rel string? -
- href string? -
- 'type string? -
pandadoc: DocumentDetailsResponse
Fields
- id string? -
- name string? -
- autonumbering_sequence_name_prefix anydata? -
- date_created string? -
- date_modified string? -
- date_completed string? -
- created_by DocumentdetailsresponseCreatedBy? -
- template DocumentdetailsresponseTemplate? -
- expiration_date anydata? -
- metadata record {}? -
- tokens record {}[]? -
- fields record {}[]? -
- pricing PricingTablesResponse? -
- 'version string? -
- tags string[]? -
- sent_by anydata? -
- recipients DocumentdetailsresponseRecipients[]? -
- grand_total DocumentdetailsresponseGrandTotal? -
- linked_objects DocumentdetailsresponseLinkedObjects[]? -
- status string? -
pandadoc: DocumentdetailsresponseCreatedBy
Fields
- id string? -
- membership_id string? -
- email string? -
- first_name string? -
- last_name string? -
- avatar string? -
pandadoc: DocumentdetailsresponseGrandTotal
Fields
- amount string? -
- currency string? -
pandadoc: DocumentdetailsresponseLinkedObjects
Fields
- provider string? -
- entity_type string? -
- entity_id string? -
pandadoc: DocumentdetailsresponseRecipients
Fields
- recipient_type string? -
- role string? -
- roles string[]? -
- last_name string? -
- signing_order anydata? -
- id string? -
- contact_id string? -
- first_name string? -
- email string? -
- has_completed boolean? -
- shared_link string? -
pandadoc: DocumentdetailsresponseTemplate
Fields
- id string? -
- name string? -
pandadoc: DocumentListResponse
Fields
- results DocumentlistresponseResults[]? -
pandadoc: DocumentlistresponseResults
Fields
- id string? -
- name string? -
- status string? -
- date_created string? -
- date_modified string? -
- expiration_date string? -
- 'version string? -
pandadoc: DocumentSendRequest
Fields
- message string? - A message that will be sent by email with a link to a document to sign.
- subject string? - Value that will be used as the email subject.
- silent boolean? - Disables sent, viewed, comment, and completed email notifications for document recipients and the document sender. By default, notifications emails are sent for specific actions. If set as true, it won't affect the "Approve document" email notification sent to the Approver.
pandadoc: DocumentSendResponse
Fields
- id string? -
- name string? -
- status string? -
- date_created string? -
- date_modified string? -
- expiration_date string? -
- 'version string? -
- uuid string? -
- shared_link string? -
pandadoc: DocumentsFolderCreateRequest
Fields
- name string - Name the folder for the Documents you are creating.
- parent_uuid string? - ID of the parent folder. To create a new folder in the root folder, remove this parameter in the request.
pandadoc: DocumentsFolderCreateResponse
Fields
- uuid string? -
- name string? -
- date_created string? -
pandadoc: DocumentsFolderListResponse
Fields
- results DocumentsfolderlistresponseResults[]? -
pandadoc: DocumentsfolderlistresponseResults
Fields
- uuid string? -
- name string? -
- date_created string? -
- has_folders boolean? -
- has_items boolean? -
pandadoc: DocumentsFolderRenameRequest
Fields
- name string - Provide a new name for the folder.
pandadoc: DocumentsFolderRenameResponse
Fields
- uuid string? -
- name string? -
- date_created string? -
pandadoc: DocumentStatusChangeRequest
Fields
- status DocumentStatusRequestEnum -
- note string? - Provide “private notes” regarding the manual status change.
- notify_recipients boolean? - Send a notification email about the status change to all recipients.
pandadoc: DocumentStatusResponse
Fields
- id string? -
- name string? -
- status string? -
- date_created string? -
- date_modified string? -
- date_completed string? -
- expiration_date string? -
- 'version string? -
- uuid string? -
pandadoc: DocumentTransferAllOwnershipRequest
Fields
- from_membership_id string? - A unique identifier of a workspace member from whom you want to transfer ownership.
- to_membership_id string? - A unique identifier of a workspace member to whom you want to transfer ownership.
pandadoc: DocumentTransferOwnershipRequest
Fields
- membership_id string? - A unique identifier of a workspace member.
pandadoc: FormListResponse
Fields
- results FormlistresponseResults[]? -
- has_next_page boolean? -
pandadoc: FormlistresponseResults
Fields
- id string? -
- name string? -
- date_created string? -
- date_modified string? -
- status string? -
pandadoc: IdStatusBody
Fields
- file string? - Binary attachment file
- data record {}? - JSON as a multipart/form-data string.
pandadoc: InlineResponse400
Fields
- 'error string? -
pandadoc: InlineResponse4001
Fields
- 'type string? -
- detail record {}? -
pandadoc: InlineResponse4002
Fields
- 'type string? -
- detail record {}? -
pandadoc: InlineResponse401
Fields
- 'type string? -
- detail string? -
pandadoc: InlineResponse403
Fields
- 'type string? -
- detail string? -
pandadoc: InlineResponse404
Fields
- 'type string? -
- detail string? -
pandadoc: InlineResponse409
Fields
- id string? -
- status string? -
- info_message string? -
pandadoc: InlineResponse429
Fields
- 'type string? -
- detail string? -
pandadoc: LinkedObjectCreateRequest
Fields
- provider string - CRM name (lowercase). See the list above.
- entity_type string - Entity type. The system validates if the type is supported. See the list for each CRM above.
- entity_id string - Entity unique identifier. The system validates if the entity exists.
pandadoc: LinkedObjectCreateResponse
Fields
- id string? -
- provider string? - CRM name (lowercase). See the list above.
- entity_type string? - Entity type. The system validates if the type is supported. See the list for each CRM above.
- entiry_id string? - Entity unique identifier. The system validates if the entity exists.
pandadoc: LinkedObjectListResponse
Fields
- linked_objects LinkedObjectCreateResponse[]? -
pandadoc: MemberDetailsResponse
Fields
- user_id string? - A unique identifier of the user in the organization.
- membership_id string? - A unique identifier of the user in the workspace.
- email string? - A user's email address.
- first_name string? - A user's first name.
- last_name string? - A user's last name.
- is_active boolean? - A boolean value that identifies if a member is active in the workspace.
- workspace string? - A name of the user's current active workspace.
- workspace_name string? - A unique identifier of the user's current active workspace.
- emails_verified boolean? - A boolean value that identifies if the email is verified.
- role string? - A member's role in the workspace.
- user_license string? - A user license in the organization.
- date_created string? - A date when a member was added to the workspace.
- date_modified string? - Last modified date of a member.
pandadoc: MemberListResponse
Fields
- results MemberDetailsResponse[]? -
pandadoc: OAuth2AccessTokenResponse
Fields
- access_token string? -
- token_type string? -
- expires_in decimal? -
- scope string? -
- refresh_token string? -
pandadoc: OAuth2CreateAccessTokenRequest
Fields
- grant_type string - This value must be set to
authorization_code
.
- client_id string - Client ID that is automatically generated after application creation in the Developer Dashboard.
- client_secret string - Client secret that is automatically generated after application creation in the Developer Dashboard.
- code string -
auth_code
from the server on the previous step (Authorize a PandaDoc User).
- scope string? - Requested permissions. Use
read+write
to create, send, delete, and download documents, andread
to view templates and document details.
pandadoc: OAuth2RefreshAccessTokenRequest
Fields
- grant_type string - This value must be set to
refresh_token
.
- client_id string - Client ID that is automatically generated after application creation in the Developer Dashboard.
- client_secret string - Client secret that is automatically generated after application creation in the Developer Dashboard.
- refresh_token string -
refresh_token
you received and stored from the server when initially creating anaccess_token
.
- scope string? - Requested permissions. Use
read+write
as our default value to send documents.
pandadoc: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://api.pandadoc.com/oauth2/access_token") - Refresh URL
pandadoc: PricingTableRequest
Fields
- name string -
- data_merge boolean? - When set to true all field names in data rows must be passed as external names defined in the template.
- options PricingtablerequestOptions? -
- sections PricingtablerequestSections[]? -
pandadoc: PricingtablerequestOptions
Fields
- currency string? -
- discount PricingtablerequestOptionsDiscount? -
- tax_first PricingtablerequestOptionsTaxFirst? -
- tax_second PricingtablerequestOptionsTaxSecond? -
pandadoc: PricingtablerequestOptionsDiscount
Fields
- 'type string -
- name string -
- value decimal -
pandadoc: PricingtablerequestOptionsTaxFirst
Fields
- 'type string -
- name string -
- value decimal -
pandadoc: PricingtablerequestOptionsTaxSecond
Fields
- 'type string -
- name string -
- value decimal -
pandadoc: PricingTableRequestRowData
Fields
- name string -
- description string? -
- price decimal -
- cost decimal? -
- qty int -
- sku string? -
- discount PricingTableRequestRowDataDiscount? -
- tax_first PricingTableRequestRowDataTaxFirst? -
- tax_second PricingTableRequestRowDataTaxSecond? -
pandadoc: PricingTableRequestRowDataDiscount
Fields
- value decimal? -
- 'type string? -
pandadoc: PricingTableRequestRowDataTaxFirst
Fields
- value decimal? -
- 'type string? -
pandadoc: PricingTableRequestRowDataTaxSecond
Fields
- value decimal? -
- 'type string? -
pandadoc: PricingTableRequestRowOptions
Fields
- qty_editable boolean? -
- optional_selected boolean? -
- optional boolean? -
pandadoc: PricingtablerequestRows
Fields
- options PricingTableRequestRowOptions? -
- data PricingTableRequestRowData? -
- custom_fields record {}? -
pandadoc: PricingtablerequestSections
Fields
- title string -
- default boolean? -
- multichoice_enabled boolean(default false) -
- rows PricingtablerequestRows[]? -
pandadoc: PricingTablesResponse
Fields
- tables PricingtablesresponseTables[]? -
- total string? -
pandadoc: PricingtablesresponseDiscount
Fields
- value string? -
- 'type string? -
pandadoc: PricingtablesresponseItems
Fields
- id string? -
- sku string? -
- qty string? -
- name string? -
- cost string? -
- price string? -
- description string? -
- custom_fields record {}? -
- custom_columns record {}? -
- discount PricingtablesresponseDiscount? -
- tax_first PricingtablesresponseDiscount? -
- tax_second PricingtablesresponseDiscount? -
- subtotal string? -
- options PricingtablesresponseOptions? -
- sale_price string? -
- taxes record {}? -
- discounts record {}? -
- fees record {}? -
- merged_data record {}? - Will contain all the fields in flat structure with external field names defined in the template.
pandadoc: PricingtablesresponseOptions
Fields
- optional boolean? -
- optional_selected boolean? -
- multichoice_enabled boolean? -
- multichoice_selected boolean? -
pandadoc: PricingtablesresponseSummary
Fields
- subtotal string? -
- total string? -
- discount string? -
- tax string? -
pandadoc: PricingtablesresponseTables
Fields
- name string? -
- id string? -
- total string? -
- is_included_in_total boolean? -
- summary PricingtablesresponseSummary? -
- items PricingtablesresponseItems[]? -
- currency string? -
pandadoc: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
pandadoc: TemplateDetailsResponse
Fields
- id string? -
- name string? -
- date_created string? -
- date_modified string? -
- created_by ContentlibraryitemresponseCreatedBy? -
- metadata record {}? -
- tokens TemplatedetailsresponseTokens[]? -
- fields record {}[]? -
- pricing PricingTablesResponse? -
- tags string[]? -
- roles TemplatedetailsresponseRoles[]? -
- 'version string? -
- content_placeholders TemplatedetailsresponseContentPlaceholders[]? -
- images TemplatedetailsresponseImages[]? -
pandadoc: TemplatedetailsresponseContentPlaceholders
Fields
- uuid string? -
- block_id string? -
- description string? -
pandadoc: TemplatedetailsresponseImages
Fields
- name string? -
- block_uuid string? -
- urls string[]? -
pandadoc: TemplatedetailsresponsePreassignedPerson
Fields
- email string? -
- 'type string? -
pandadoc: TemplatedetailsresponseRoles
Fields
- id string? -
- name string? -
- signing_order string? -
- preassigned_person TemplatedetailsresponsePreassignedPerson? -
pandadoc: TemplatedetailsresponseTokens
Fields
- name string? -
- value string? -
pandadoc: TemplateListResponse
Fields
- results TemplatelistresponseResults[]? -
pandadoc: TemplatelistresponseResults
Fields
- id string? -
- name string? -
- date_created string? -
- date_modified string? -
- 'version string? -
pandadoc: TemplatesFolderCreateRequest
Fields
- name string - Name the folder for Templates you are creating.
- parent_uuid string? - ID of the parent folder. To create a new folder in the root folder, remove this parameter in the request.
pandadoc: TemplatesFolderCreateResponse
Fields
- uuid string? -
- name string? -
- date_created string? -
pandadoc: TemplatesFolderListResponse
Fields
- results TemplatesfolderlistresponseResults[]? -
pandadoc: TemplatesfolderlistresponseResults
Fields
- uuid string? -
- name string? -
- date_created string? -
- has_folders boolean? -
- has_items boolean? -
pandadoc: TemplatesFolderRenameRequest
Fields
- name string - Provide a new name for the folder.
pandadoc: TemplatesFolderRenameResponse
Fields
- uuid string? -
- name string? -
- date_created string? -
pandadoc: V1DocumentsBody
Fields
- file string? - Binary PDF File
- data record {}? - JSON as a multipart/form-data string.
Union types
pandadoc: Oauth2AccessTokenBody
Oauth2AccessTokenBody
String types
Integer types
pandadoc: DocumentStatusRequestEnum
DocumentStatusRequestEnum
Import
import ballerinax/pandadoc;
Metadata
Released date: about 2 years ago
Version: 1.3.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 8
Current verison: 5
Weekly downloads
Keywords
Content & Files/Documents
Cost/Freemium
Contributors