opendesign
Module opendesign
API
Definitions
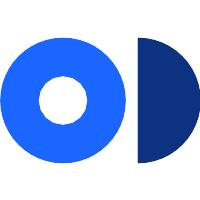
ballerinax/opendesign Ballerina library
Overview
This is a generated connector for Open Design REST API v0.3.4 OpenAPI specification.
Open Design read and display data from designs using code. Unlock the power of programmatic design.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Open Design account account
- Obtain tokens by following this guide.
Quickstart
To use the Brex Onboarding connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/opendesign
module into the Ballerina project.
import ballerinax/opendesign;
Step 2: Create a new connector instance
Create a opendesign:ClientConfig
with the <JWT_TOKEN>
obtained, and initialize the connector with it.
opendesign:ClientConfig clientConfig = { authConfig : { token: <JWT_TOKEN> } }; opendesign:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on test if your token is valid using the connector.
public function main() { opendesign:InlineResponse200|error response = baseClient->checkToken(); if (response is opendesign:InlineResponse200) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
opendesign: Client
This is a generated connector for Open Design REST API v0.3.4 OpenAPI specification. Open Design read and display data from designs using code. Unlock the power of programmatic design.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Open Design account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.opendesign.dev" - URL of the target service
checkToken
function checkToken() returns InlineResponse200|error
Auth Token Check
Return Type
- InlineResponse200|error - Returns info about the token.
postDesignUpload
function postDesignUpload(DesignsUploadBody payload) returns InlineResponse201|error
Design File Upload
Parameters
- payload DesignsUploadBody - Upload information
Return Type
- InlineResponse201|error - Returns the created design entity.
postDesignLink
function postDesignLink(DesignsLinkBody payload) returns InlineResponse201|error
URL-Based Design File Import
Parameters
- payload DesignsLinkBody - Design link information
Return Type
- InlineResponse201|error - Returns the created design entity.
postDesignFigmaLink
function postDesignFigmaLink(DesignsFigmalinkBody payload) returns InlineResponse2011|error
Figma Design File Import
Parameters
- payload DesignsFigmalinkBody - Figma design information
Return Type
- InlineResponse2011|error - Returns the created design entity or its processing status (in case a export was requested).
getDesignList
function getDesignList() returns InlineResponse2001|error
Design List
Return Type
- InlineResponse2001|error - Returns a list of design entities
getDesign
function getDesign(string designId) returns DesignProcessing|InlineResponse2002|error
Design Info
Parameters
- designId string - A unique identifier (UUID) of an imported design file.
Return Type
- DesignProcessing|InlineResponse2002|error - Returns the design entity or its processing status.
postDesignExport
function postDesignExport(string designId, DesignIdExportsBody payload) returns DesignExport|error
Start Design Export
Parameters
- designId string - A unique identifier (UUID) of an imported design file.
- payload DesignIdExportsBody - Design export target
Return Type
- DesignExport|error - Returns the started design export task entity.
getDesignExport
function getDesignExport(string designId, string exportId) returns DesignExport|error
Design Export Info
Parameters
- designId string - A unique identifier (UUID) of an imported design file.
- exportId string - An identifier of a export task of an imported design file.
Return Type
- DesignExport|error - Returns the design export task entity.
getDesignSummary
function getDesignSummary(string designId) returns DesignProcessing|InlineResponse2003|error
Design Summary
Parameters
- designId string - A unique identifier (UUID) of an imported design file.
Return Type
- DesignProcessing|InlineResponse2003|error - Returns an extended design entity with the complete page and artboard entity lists or the processing status of the design.
getDesignPageList
function getDesignPageList(string designId) returns InlineResponse2004|error
Page List
Parameters
- designId string - A unique identifier (UUID) of an imported design file.
Return Type
- InlineResponse2004|error - Returns a page entity list.
getDesignArtboardList
function getDesignArtboardList(string designId) returns InlineResponse2005|error
Artboard List
Parameters
- designId string - A unique identifier (UUID) of an imported design file.
Return Type
- InlineResponse2005|error - Returns a artboard entity list.
Records
opendesign: Artboard
Fields
- id ArtboardId - An identifier of an artboard within a design file.
- page_id string? - The design file page containing the artboard in case of paged (
has_pages=true
) designs.
- name string - The name of the design file artboard.
- status ArtboardStatusEnum - The processing status of an artboard within a design file.
- has_content boolean - Flag denoting there is content in the design file artboard and the client can obtain it via a GET
…/content
request.
opendesign: ArtboardContentNotFoundError
The specified artboard does not have any content.
Fields
- code string - Error code
- message string - Error message
- docs_url ErrorDocsUrl? - The URL of a documentation web page with more info about the error.
opendesign: ArtboardNotFoundError
The specified artboard is not present in the design file.
Fields
- code string - Error code
- message string - Error message
- docs_url ErrorDocsUrl? - The URL of a documentation web page with more info about the error.
opendesign: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
opendesign: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
opendesign: Design
A design file descriptor
Fields
- id string - A unique identifier (UUID) of an imported design file.
- name string - The name of the design.
- format DesignFormatEnum - The design file format.
- created_at int - UTC timestamp (in milliseconds) when the design file was imported.
- completed_at int - UTC timestamp (in milliseconds) when the processing of the design file successfully finished.
- status DesignStatusEnum - The processing status of the design file.
- has_pages boolean - Flag denoting there are pages in the design file and the client can obtain the page list via a GET
…/pages
request.
opendesign: DesignArtboardProcessing
A descriptor of a design artboard which is being processed.
Fields
- id ArtboardId - An identifier of an artboard within a design file.
- design_id DesignId - A unique identifier (UUID) of an imported design file.
- status ArtboardStatusEnum - The processing status of an artboard within a design file.
opendesign: DesignExport
Fields
- id string - An identifier of a export task of an imported design file.
- created_at int - UTC timestamp (in milliseconds) when the design export started.
- completed_at int - UTC timestamp (in milliseconds) when the design export successfully finished.
- status DesignExportStatusEnum - The processing status of the design file export task.
- result_format DesignExportTargetFormatEnum - The target design file format of a export. (Only Sketch is supported currently.)
- result_url string - The URL of the produced design file in the
result_format
format when the export is successfully finished.
opendesign: DesignExportNotFoundError
The specified design file export task is not found.
Fields
- code string - Error code
- message string - Error message
- docs_url ErrorDocsUrl? - The URL of a documentation web page with more info about the error.
opendesign: DesignIdExportsBody
Fields
- format DesignExportTargetFormatEnum - The target design file format of a export. (Only Sketch is supported currently.)
opendesign: DesignNotFoundError
The specified design file is not found.
Fields
- code string - Error code
- message string - Error message
- docs_url ErrorDocsUrl? - The URL of a documentation web page with more info about the error.
opendesign: DesignNotPagedError
The specified design file is not paged (has_pages=false
).
Fields
- code string - Error code
- message string - Error message
- docs_url ErrorDocsUrl? - The URL of a documentation web page with more info about the error.
opendesign: DesignNotProcessedError
The provided file format is not valid or supported.
Fields
- code string - Error code
- message string - Error message
- docs_url ErrorDocsUrl? - The URL of a documentation web page with more info about the error.
opendesign: DesignProcessing
A descriptor of a design file which is being processed.
Fields
- id string - A unique identifier (UUID) of an imported design file.
- status DesignStatusEnum - The processing status of the design file.
opendesign: DesignsFigmalinkBody
Fields
- design_name string? - Name of the design.
- figma_token string - An access token from Figma.
- figma_filekey string - ID of the file in Figma.
- figma_ids FigmaFrameId[]? - Frame IDs to sync. When not specified, all frames are included.
- exports DesignsfigmalinkExports[]? - Design file exports to intiate automatically with the import. (Only Sketch is supported currently as the target format.)
opendesign: DesignsfigmalinkExports
Fields
- format DesignExportTargetFormatEnum - The target design file format of a export. (Only Sketch is supported currently.)
opendesign: DesignsLinkBody
Fields
- url string - A publicly accessible URL of the design file to import.
- format DesignImportFormatEnum? - The design file format. (This is not needed when the format can be inferred from the file extension.)
- design_name string? - Name of the design. (When no name is provided, the server infers the name from the URL.)
opendesign: DesignSummary
Fields
- pages Page[]? - The list of pages within the paged (
has_pages=true
) design file.
- artboards Artboard[] - The list of artboards within the design file.
- id string - A unique identifier (UUID) of an imported design file.
- name string - The name of the design.
- format DesignFormatEnum - The design file format.
- created_at int - UTC timestamp (in milliseconds) when the design file was imported.
- completed_at int - UTC timestamp (in milliseconds) when the processing of the design file successfully finished.
- status DesignStatusEnum - The processing status of the design file.
- has_pages boolean - Flag denoting there are pages in the design file and the client can obtain the page list via a GET
…/pages
request.
opendesign: DesignsUploadBody
Fields
- file MultipartFilePart - A multipart file upload field.
- format DesignImportFormatEnum? - The design file format. (This is not needed when the format can be inferred from the file extension.)
opendesign: FigmaDesign
A descriptor of a figma design file
Fields
- format string - Design format
- id string - A unique identifier (UUID) of an imported design file.
- name string - The name of the design.
- created_at int - UTC timestamp (in milliseconds) when the design file was imported.
- completed_at int - UTC timestamp (in milliseconds) when the processing of the design file successfully finished.
- status DesignStatusEnum - The processing status of the design file.
- has_pages boolean - Flag denoting there are pages in the design file and the client can obtain the page list via a GET
…/pages
request.
opendesign: InactiveAccountError
The auth token provided via the Authorization
HTTP header is associated with an account which is either expired or blocked.
Fields
- code string - Error code
- message string - Error message
- docs_url ErrorDocsUrl? - The URL of a documentation web page with more info about the error.
opendesign: InlineResponse200
Fields
- expires_at int - UTC timestamp (in milliseconds) when the provided token expires.
opendesign: InlineResponse2001
Fields
- designs Design[] - List of design entities
opendesign: InlineResponse2004
Fields
- pages Page[] - The list of pages within the paged (
has_pages=true
) design file.
opendesign: InlineResponse2005
Fields
- artboards Artboard[] - The list of artboards within the design file.
opendesign: InlineResponse201
A design file descriptor
Fields
- design Design - A design file descriptor
opendesign: InlineResponse2011
Fields
- design FigmaDesign|DesignProcessing -
- exports DesignExport[] - Inititated design file exports for the import.
opendesign: InlineResponse400
Fields
- 'error InvalidInputError - Some of the input parameters are invalid or missing.
opendesign: InlineResponse4001
Fields
opendesign: InlineResponse4002
Fields
opendesign: InlineResponse4003
Fields
opendesign: InlineResponse4004
Fields
opendesign: InlineResponse401
Fields
- 'error AuthTokenError -
opendesign: InlineResponse404
Fields
- 'error DesignNotFoundError - The specified design file is not found.
opendesign: InlineResponse4041
Fields
opendesign: InlineResponse4042
Fields
- 'error DesignNotFoundError|ArtboardNotFoundError -
opendesign: InlineResponse406
Fields
- 'error DesignNotPagedError - The specified design file is not paged (
has_pages=false
).
opendesign: InlineResponse429
Fields
- 'error RateLimitReachedError - The rate limit for the operation has been reached.
opendesign: InlineResponse500
Fields
- 'error ServerError -
opendesign: InvalidAuthTokenError
The auth token provided via the Authorization
HTTP header is not valid.
Fields
- code string - Error code
- message string - Error message
- docs_url ErrorDocsUrl? - The URL of a documentation web page with more info about the error.
opendesign: InvalidFormatError
The provided file format is not valid or supported.
Fields
- code string - Error code
- message string - Error message
- docs_url ErrorDocsUrl? - The URL of a documentation web page with more info about the error.
opendesign: InvalidHeaderError
A required HTTP header is either not provided of invalid.
Fields
- code string - Error code
- message string - Error message
- docs_url ErrorDocsUrl? - The URL of a documentation web page with more info about the error.
opendesign: InvalidInputError
Some of the input parameters are invalid or missing.
Fields
- code string - Error code
- message string - Error message
- docs_url ErrorDocsUrl? - The URL of a documentation web page with more info about the error.
opendesign: InvalidUploadFileFieldError
The multipart request contain the file data under the wrong field name.
Fields
- code string - Error code
- message string - Error message
- docs_url ErrorDocsUrl? - The URL of a documentation web page with more info about the error.
opendesign: InvalidUploadLinkError
The provided file URL is not valid.
Fields
- code string - Error code
- message string - Error message
- docs_url ErrorDocsUrl? - The URL of a documentation web page with more info about the error.
opendesign: MissingUploadFileError
The multipart request does not contain file data.
Fields
- code string - Error code
- message string - Error message
- docs_url ErrorDocsUrl? - The URL of a documentation web page with more info about the error.
opendesign: MultipleDesignExportsError
The provided file format is not valid or supported.
Fields
- code string - Error code
- message string - Error message
- docs_url ErrorDocsUrl? - The URL of a documentation web page with more info about the error.
opendesign: NoAuthTokenError
The endpoint requires an auth token provided via the Authorization
HTTP header.
Fields
- code string - Error code
- message string - Error message
- docs_url ErrorDocsUrl? - The URL of a documentation web page with more info about the error.
opendesign: Page
Fields
- id PageId - An identifier of an page within a design file.
- name string -
- status PageStatusEnum - The processing status of a page within a design file.
opendesign: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
opendesign: RateLimitReachedError
The rate limit for the operation has been reached.
Fields
- code string - Error code
- message string - Error message
- docs_url ErrorDocsUrl? - The URL of a documentation web page with more info about the error.
opendesign: ServerError
Fields
- code string - Error code
- message string? - A human-readable technical message of the error.
- docs_url ErrorDocsUrl? - The URL of a documentation web page with more info about the error.
Union types
opendesign: AuthTokenError
AuthTokenError
opendesign: InlineResponse2002
InlineResponse2002
opendesign: InlineResponse2003
InlineResponse2003
Descriptor for a design
String types
opendesign: ArtboardId
ArtboardId
An identifier of an artboard within a design file.
opendesign: ArtboardStatusEnum
ArtboardStatusEnum
The processing status of an artboard within a design file.
opendesign: DesignExportStatusEnum
DesignExportStatusEnum
The processing status of the design file export task.
opendesign: DesignExportTargetFormatEnum
DesignExportTargetFormatEnum
The target design file format of a export. (Only Sketch is supported currently.)
opendesign: DesignFormatEnum
DesignFormatEnum
The design file format.
opendesign: DesignId
DesignId
A unique identifier (UUID) of an imported design file.
opendesign: DesignImportFormatEnum
DesignImportFormatEnum
The design file format. (This is not needed when the format can be inferred from the file extension.)
opendesign: DesignStatusEnum
DesignStatusEnum
The processing status of the design file.
opendesign: ErrorDocsUrl
ErrorDocsUrl
The URL of a documentation web page with more info about the error.
opendesign: FigmaFrameId
FigmaFrameId
An identifier of a "frame" component within a Figma file.
opendesign: MultipartFilePart
MultipartFilePart
A multipart file upload field.
opendesign: PageId
PageId
An identifier of an page within a design file.
opendesign: PageStatusEnum
PageStatusEnum
The processing status of a page within a design file.
Import
import ballerinax/opendesign;
Metadata
Released date: about 2 years ago
Version: 1.5.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 5
Current verison: 0
Weekly downloads
Keywords
Content & Files/Images & Design
Cost/Freemium
Contributors