microsoft.onedrive
Module microsoft.onedrive
API
Declarations
Definitions
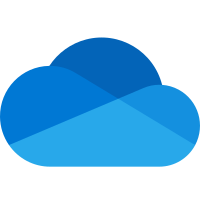
ballerinax/microsoft.onedrive Ballerina library
Overview
Ballerina connector for Microsoft OneDrive connects to the OneDrive file storage API in Microsoft Graph v1.0 via the Ballerina language. The connector allows you to programmatically perform basic drive functionalities such as file upload, download. It also allows you to share files and folders stored on Microsoft OneDrive.
This module supports Microsoft Graph API v1.0
and allows performing functions only on behalf of the currently signed-in user.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Microsoft 365 Personal account
- Create an Azure account to register an application in the Azure portal
- Obtain tokens
- Follow [Microsoft Documentation - Register an application with the Microsoft identity platform]](https://docs.microsoft.com/en-us/graph/auth-register-app-v2) to register an application with the Microsoft identity platform.
Quickstart
To use the OneDrive connector in your Ballerina application, update the .bal file as follows:
Step 1 - Import connector
Import the ballerinax/microsoft.onedrive
module into the Ballerina project.
import ballerinax/microsoft.onedrive;
Step 2 - Create a new connector instance
To make the connection, use the OAuth2 refresh token grant configuration.
onedrive:ConnectionConfig configuration = { auth: { refreshUrl: <REFRESH_URL>, refreshToken : <REFRESH_TOKEN>, clientId : <CLIENT_ID>, clientSecret : <CLIENT_SECRET>, scopes: [<NECESSARY_SCOPES>] } };
Step 3 - Invoke connector operation
- Create a folder
To create a folder, use the following code block:
string parentID = "<PARENT_FOLDER_ID>"; string newFolderName = "Samples_Test"; onedrive:FolderMetadata item = { name: newFolderName, conflictResolutionBehaviour : "rename" }; onedrive:DriveItemData|onedrive:Error driveItem = driveClient->createFolderById(parentID, item); if (driveItem is onedrive:DriveItemData) { log:printInfo("Folder Created " + driveItem.toString()); log:printInfo("Success!"); } else { log:printError(driveItem.message()); }
- Use
bal run
command to compile and run the Ballerina program.
Clients
microsoft.onedrive: Client
This is connecting to the Microsoft Graph RESTful web API that enables you to access Microsoft Cloud service resources.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Microsoft 365 Work and School account
and obtain tokens following this guide. Configure the Access token to
have the required permission.
init (ConnectionConfig config)
- config ConnectionConfig - Configurations required to initialize the
Client
endpoint
getRecentItems
function getRecentItems() returns DriveItemData[]|Error
Lists a set of items that have been recently used by the signed in user
.
This will include items that are in the user's drive as well as the items they have access to from other drives.
Return Type
- DriveItemData[]|Error - An array of
onedrive:DriveItemData
if sucess. Elseonedrive:Error
.
getItemsSharedWithMe
function getItemsSharedWithMe(string? queryParams) returns DriveItemData[]|error
Retrieves a collection of DriveItemData
resources that have been shared with the signed in user
of the OneDrive.
Parameters
Return Type
- DriveItemData[]|error - An array of
onedrive:DriveItemData
if sucess. Elseonedrive:Error
.
createFolderById
function createFolderById(string parentFolderId, FolderMetadata folderMetadata) returns DriveItemData|Error
Creates a new folder in a Drive with a specified parent item, referred with the parent folder's ID.
Parameters
- parentFolderId string - The folder ID of the parent folder where, the new folder will be created
- folderMetadata FolderMetadata - A record
onedrive:FolderMetadata
which contains the necessary data to create a folder
Return Type
- DriveItemData|Error - A record
onedrive:DriveItemData
if sucess. Elseonedrive:Error
.
createFolderByPath
function createFolderByPath(string parentFolderPath, FolderMetadata folderMetadata) returns DriveItemData|Error
Creates a new folder in a Drive with a specified parent item referred with the folder path.
Parameters
- parentFolderPath string - The folder path of the parent folder relative to the
root
of the respective Drive where, the new folder will be created- NOTE: When you want to create a folder on root itself, you must give the relative path of the new folder only.
- folderMetadata FolderMetadata - A record of type
FolderMetadata
which contains the necessary data to create a folder
Return Type
- DriveItemData|Error - A record
onedrive:DriveItemData
if sucess. Elseonedrive:Error
.
getItemMetadataById
function getItemMetadataById(string itemId, string? queryParams) returns DriveItemData|Error
Retrieves the metadata for a DriveItem in a Drive by item ID.
Parameters
- itemId string - The ID of the DriveItem
Return Type
- DriveItemData|Error - A record
onedrive:DriveItemData
if sucess. Elseonedrive:Error
.
getItemMetadataByPath
function getItemMetadataByPath(string itemPath, string? queryParams) returns DriveItemData|Error
Retrieves the metadata for a DriveItem in a Drive by file system path.
Parameters
- itemPath string - The file system path of the DriveItem. The hierarchy of the path allowed in this function is relative
to the
root
of the respective Drive. So, the relative path fromroot
must be provided.
Return Type
- DriveItemData|Error - A record
onedrive:DriveItemData
if sucess. Elseonedrive:Error
.
updateDriveItemById
function updateDriveItemById(string itemId, DriveItem replacementData) returns DriveItemData|Error
Updates the metadata for a DriveItem in a Drive referring by item ID.
Parameters
- itemId string - The ID of the DriveItem
- replacementData DriveItem - A record
onedrive:DriveItem
which contains the values for properties that should be updated
Return Type
- DriveItemData|Error - A record
onedrive:DriveItem
if sucess. Elseonedrive:Error
.
updateDriveItemByPath
function updateDriveItemByPath(string itemPath, DriveItem replacementData) returns DriveItemData|Error
Updates the metadata for a DriveItem in a Drive by file system path.
Parameters
- itemPath string - The file system path of the DriveItem
- The hierarchy of the path allowed in this function is relative to the
root
of the respective Drive. So, the relative path fromroot
must be provided.
- The hierarchy of the path allowed in this function is relative to the
- replacementData DriveItem - A record of type
DriveItem
which contains the values for properties that should be updated
Return Type
- DriveItemData|Error - A record
onedrive:DriveItem
if sucess. Elseonedrive:Error
.
deleteDriveItemById
Deletes a DriveItem in a Drive by using it's ID.
Parameters
- itemId string - The ID of the DriveItem
Return Type
- Error? -
onedrive:Error
if the operation fails or()
if nothing is to be returned
deleteDriveItemByPath
Deletes a DriveItem in a Drive by using it's file system path.
Parameters
- itemPath string - The file system path of the DriveItem
- The hierarchy of the path allowed in this function is relative to the
root
of the respective Drive. So, the relative path fromroot
must be provided.
- The hierarchy of the path allowed in this function is relative to the
Return Type
- Error? -
onedrive:Error
if the operation fails or()
if nothing is to be returned
restoreDriveItem
function restoreDriveItem(string itemId, string? parentFolderId, string? name) returns DriveItemData|Error
Restores a driveItem that has been deleted and is currently in the recycle bin.
NOTE: This functionality is
currently only available for OneDrive Personal.
Parameters
- itemId string - The ID of the DriveItem
- parentFolderId string? (default ()) - The ID of the parent item the deleted item will be restored to
- name string? (default ()) - The new name for the restored item
- If this isn't provided, the same name will be used as the original.
Return Type
- DriveItemData|Error - A record
onedrive:DriveItemData
if sucess. Elseonedrive:Error
.
copyDriveItemWithId
function copyDriveItemWithId(string itemId, string? name, ParentReference? parentReference) returns string|Error
Asynchronously creates a copy of a DriveItem (including any children), under a new parent item or at the same location with a new name.
Parameters
- itemId string - The ID of the DriveItem
- name string? (default ()) - The new name for the copy
- If this isn't provided, the same name will be used as the original.
- parentReference ParentReference? (default ()) - A record
onedrive:ParentReference
that represents reference to the parent item the copy will be created in
Return Type
copyDriveItemInPath
function copyDriveItemInPath(string itemPath, string? name, ParentReference? parentReference) returns string|Error
Asynchronously creates a copy of a DriveItem (including any children), under a new parent item or at the same location with a new name.
Parameters
- itemPath string - The file system path of the DriveItem
- The hierarchy of the path allowed in this function is relative to the
root
of the respective Drive. So, the relative path fromroot
must be provided.
- The hierarchy of the path allowed in this function is relative to the
- name string? (default ()) - The new name for the copy
- If this isn't provided, the same name will be used as the original.
- parentReference ParentReference? (default ()) - A record
onedrive:ParentReference
that represents reference to the parent item the copy will be created in.
Return Type
downloadFileById
function downloadFileById(string itemId, FileFormat? formatToConvert) returns File|Error
Downloads the contents of the primary stream (file) of a DriveItem using item ID.
NOTE: Only driveItems
with the file property can be downloaded.
Parameters
- itemId string - The ID of the DriveItem
- formatToConvert FileFormat? (default ()) - Specify the format the item's content should be downloaded as.
downloadFileByPath
function downloadFileByPath(string itemPath, FileFormat? formatToConvert) returns File|Error
Downloads the contents of the primary stream (file) of a DriveItem using item path.
NOTE: Only
driveItems with the file property can be downloaded.
Parameters
- itemPath string - The file system path of the File
- The hierarchy of the path allowed in this function is relative to the
root
of the respective Drive. So, the relative path fromroot
must be provided. - example: Use the itempath as
/Documents/MyFile.xlsx
if MyFile.xlsx is located inside a folder called Docuements.
- The hierarchy of the path allowed in this function is relative to the
- formatToConvert FileFormat? (default ()) - Specify the format the item's content should be downloaded as.
downloadFileByDownloadUrl
function downloadFileByDownloadUrl(string downloadUrl, ByteRange? partialContentOption) returns File|Error
Downloads the contents of the primary stream (file) of a DriveItem using download URL.
NOTE: Only driveItems
with the file property can be downloaded.
Parameters
- downloadUrl string - Download URL for a specific DriveItem
- partialContentOption ByteRange? (default ()) - The value for the
Range
header to download a partial range of bytes from the file
uploadFileToFolderByIdAsStream
function uploadFileToFolderByIdAsStream(string parentFolderId, string fileName, stream<byte[], Error?> binaryStream, string mimeType) returns DriveItemData|Error
Upload a new file to the Drive. This method only supports files up to 4MB in size.
Parameters
- parentFolderId string - The folder ID of the parent folder where, the new file will be uploaded
- fileName string - The name of the new file
- mimeType string - The media type of the uploading file
Return Type
- DriveItemData|Error - A record of type
DriveItemData
if success. ElseError
.
uploadFileToFolderByPathAsStream
function uploadFileToFolderByPathAsStream(string parentFolderPath, string fileName, stream<byte[], Error?> binaryStream, string mimeType) returns DriveItemData|Error
Upload a new file to the Drive. This method only supports files up to 4MB in size.
Parameters
- parentFolderPath string - The folder path of the parent folder relative to the
root
of the respective Drive where, the new folder will be created. NOTE: When you want to create a folder on root itself, you must give the relative path of the new folder only.
- fileName string - The name of the new file
- mimeType string - The media type of the uploading file
Return Type
- DriveItemData|Error - A record of type
DriveItemData
if success. ElseError
.
replaceFileUsingIdAsStream
function replaceFileUsingIdAsStream(string itemId, stream<byte[], Error?> binaryStream, string mimeType) returns DriveItemData|Error
Update the contents of an existing file in the Drive. This method only supports files up to 4MB in size. Here, the type of the file should be the same type as the file we replace with.
Parameters
- itemId string - The ID of the DriveItem
- mimeType string - The media type of the uploading file
Return Type
- DriveItemData|Error - A record of type
DriveItemData
if success. ElseError
.
replaceFileUsingPathAsStream
function replaceFileUsingPathAsStream(string itemPath, stream<byte[], Error?> binaryStream, string mimeType) returns DriveItemData|Error
Update the contents of an existing file in the Drive. This method only supports files up to 4MB in size. Here, the type of the file should be the same type as the file we replace with.
Parameters
- itemPath string - The file system path of the File. The hierarchy of the path allowed in this function is relative
to the
root
of the respective Drive. So, the relative path fromroot
must be provided.
- mimeType string - The media type of the uploading file
Return Type
- DriveItemData|Error - A record of type
DriveItemData
if success. ElseError
.
uploadFileToFolderById
function uploadFileToFolderById(string parentFolderId, string fileName, byte[] byteArray, string mimeType) returns DriveItemData|Error
Uploads a new file to the Drive.
This method only supports files up to 4MB in size.
Parameters
- parentFolderId string - The folder ID of the parent folder where, the new file will be uploaded
- fileName string - The name of the new file
- byteArray byte[] - An array of
byte
that represents a binary form of the file to be uploaded
- mimeType string - The mime type of the uploading file
Return Type
- DriveItemData|Error - A record
onedrive:DriveItemData
if sucess. Elseonedrive:Error
.
uploadFileToFolderByPath
function uploadFileToFolderByPath(string parentFolderPath, string fileName, byte[] byteArray, string mimeType) returns DriveItemData|Error
Uploads a new file to the Drive.
This method only supports files up to 4MB in size.
Parameters
- parentFolderPath string - The folder path of the parent folder relative to the
root
of the respective Drive where, the new folder will be created- NOTE: When you want to create a folder on root itself, you must give the relative path of the new folder only.
- fileName string - The name of the new file
- byteArray byte[] - An array of
byte
that represents a binary form of the file to be uploaded
- mimeType string - The mime type of the uploading file
Return Type
- DriveItemData|Error - A record
onedrive:DriveItemData
if sucess. Elseonedrive:Error
.
replaceFileUsingId
function replaceFileUsingId(string itemId, byte[] byteArray, string mimeType) returns DriveItemData|Error
Updates the contents of an existing file in the Drive.
This method only supports files up to 4MB in size.
Here, the type of the file should be the same type as the file we replace with.
Parameters
- itemId string - The ID of the DriveItem
- byteArray byte[] - An array of
byte
that represents a binary form of the file to be uploaded
- mimeType string - The mime type of the uploading file
Return Type
- DriveItemData|Error - A record
onedrive:DriveItemData
if sucess. Elseonedrive:Error
.
replaceFileUsingPath
function replaceFileUsingPath(string itemPath, byte[] byteArray, string mimeType) returns DriveItemData|Error
Updates the contents of an existing file in the Drive.
This method only supports files up to 4MB in size.
Here, the type of the file should be the same type as the file we replace with.
Parameters
- itemPath string - The file system path of the File
- The hierarchy of the path allowed in this function is relative to the
root
of the respective Drive. So, the relative path fromroot
must be provided. - example: Use the itempath as
/Documents/MyFile.xlsx
if MyFile.xlsx is located inside a folder called Docuements.
- The hierarchy of the path allowed in this function is relative to the
- byteArray byte[] - An array of
byte
that represents a binary form of the file to be uploaded
- mimeType string - The mime type of the uploading file
Return Type
- DriveItemData|Error - A record
onedrive:DriveItemData
if sucess. Elseonedrive:Error
.
resumableUploadDriveItem
function resumableUploadDriveItem(string itemPath, UploadMetadata itemInfo, stream<Block, Error?> binaryStream) returns DriveItemData|Error
Uploads files up to the maximum file size.
NOTE: Maximum bytes in any given request is less than 60 MiB.
Parameters
- itemPath string - The file system path of the file (with extention)
- The hierarchy of the path allowed in this function is relative to the
root
of the respective Drive. So, the relative path fromroot
must be provided.
- The hierarchy of the path allowed in this function is relative to the
- itemInfo UploadMetadata - Additional data about the file being uploaded
- binaryStream stream<Block, Error?> - Stream of content of file which we need to be uploaded
- The size of each byte range in the stream MUST be a multiple of 320 KiB (327,680 bytes).
- The recommended fragment size is between 5-10 MiB (5,242,880 bytes - 10,485,760 bytes)
- Note: For more information about upload large files, visit.
Return Type
- DriveItemData|Error - A record
onedrive:DriveItemData
if sucess. Elseonedrive:Error
.
searchDriveItems
function searchDriveItems(string searchText, string? queryParams) returns stream<DriveItemData, Error?>|Error
Searches the hierarchy of items for items matching a query.
Parameters
- searchText string - The query text used to search for items
- Values may be matched across several fields including filename, metadata, and file content.
Return Type
- stream<DriveItemData, Error?>|Error - A stream
onedrive:DriveItemData
if sucess. Elseonedrive:Error
.
getSharableLinkFromId
function getSharableLinkFromId(string itemId, PermissionOptions options) returns Permission|Error
Creates a new sharing link if the specified link type doesn't already exist for the
calling application.
If a sharing link of the specified type already exists for the app, the existing sharing
link will be returned.
Parameters
- itemId string - The ID of the DriveItem
- options PermissionOptions - A record
onedrive:PermissionOptions
that represents the properties of the sharing link your application is requesting
Return Type
- Permission|Error - A record
onedrive:Permission
if sucess. Elseonedrive:Error
.
getSharableLinkFromPath
function getSharableLinkFromPath(string itemPath, PermissionOptions options) returns Permission|Error
Creates a new sharing link if the specified link type doesn't already exist for the
calling application.
If a sharing link of the specified type already exists for the app, the existing sharing
link will be returned.
Parameters
- itemPath string - The file system path of the File
- The hierarchy of the path allowed in this function is relative to the
root
of the respective Drive. So, the relative path fromroot
must be provided.
- The hierarchy of the path allowed in this function is relative to the
- options PermissionOptions - A record
onedrive:PermissionOptions
that represents the properties of the sharing link your application is requesting
Return Type
- Permission|Error - A record
onedrive:Permission
if sucess. Elseonedrive:Error
.
getSharedDriveItem
function getSharedDriveItem(string sharingUrl) returns DriveItemData|Error
Accesses a shared DriveItem by using sharing URL.
Parameters
- sharingUrl string - The URL that represents a sharing link reated for a DriveItem
Return Type
- DriveItemData|Error - A record
onedrive:DriveItemData
if sucess. Elseonedrive:Error
.
sendSharingInvitationById
function sendSharingInvitationById(string itemId, ItemShareInvitation invitation) returns Permission|Error
Sends a sharing invitation for a DriveItem.
A sharing invitation provides permissions to the recipients and
optionally sends them an email with a sharing link.
Parameters
- itemId string - The ID of the DriveItem
- invitation ItemShareInvitation - A record
onedrive:ItemShareInvitation
that contain metadata for sharing
Return Type
- Permission|Error - A record
onedrive:Permission
if sucess. Elseonedrive:Error
.
sendSharingInvitationByPath
function sendSharingInvitationByPath(string itemPath, ItemShareInvitation invitation) returns Permission|Error
Sends a sharing invitation for a DriveItem.
A sharing invitation provides permissions to the recipients and
optionally sends them an email with a sharing link.
Parameters
- itemPath string - The file system path of the File
- The hierarchy of the path allowed in this function is relative to the
root
of the respective Drive. So, the relative path fromroot
must be provided.
- The hierarchy of the path allowed in this function is relative to the
- invitation ItemShareInvitation - A record
onedrive:ItemShareInvitation
that contain metadata for sharing
Return Type
- Permission|Error - A record
onedrive:Permission
if sucess. Elseonedrive:Error
.
Constants
microsoft.onedrive: DEFAULT_FRAGMENT_SIZE
The default fragment size for obtaining fragments of a file.(To upload as fragment size MUST be a multiple of 320 KiB (327,680 bytes)).
microsoft.onedrive: MAXIMUM_FRAGMENT_SIZE
The maximum fragment size for obtaining fragments of a file. (The maximum bytes in any given request muat be less than 60 MiB (62,914,560 bytes))
Enums
microsoft.onedrive: CheckInOption
The desired status of the document after the check-in operation is complete.
Members
microsoft.onedrive: ConflictResolutionBehaviour
Represents the conflict resolution behavior for actions in file operations. Default for PUT requests is REPLACE
Members
microsoft.onedrive: FileFormat
Represents the possible mimetypes of a file. Note: For more information about compatible formats, refer here: https://docs.microsoft.com/en-us/onedrive/developer/rest-api/api/driveitem_get_content_format?view=odsp-graph-online#query-string-parameters#
Members
microsoft.onedrive: LinkScope
The scope of the link represented by this permission.
Members
microsoft.onedrive: LinkType
The type of the link created.
Members
microsoft.onedrive: PermissionRole
Specify the roles that are to be granted to the recipients of the sharing invitation.
Members
microsoft.onedrive: PublicationLevel
The state of publication for a document.
Members
microsoft.onedrive: SortBy
The method by which the folder should be sorted.
Members
microsoft.onedrive: SortOrder
If value is descending
, indicates that items should be sorted in descending order. Otherwise, items should be
sorted ascending.
Members
microsoft.onedrive: ViewType
The type of view that should be used to represent the folder.
Members
Records
microsoft.onedrive: Audio
Resource that groups audio-related properties on an item into a single structure.
Fields
- album string? - The title of the album for this audio file
- albumArtist string? - The artist named on the album for the audio file
- artist string? - The performing artist for the audio file
- bitrate int? - Bitrate expressed in kbps
- composers string? - The name of the composer of the audio file
- copyright string? - Copyright information for the audio file
- disc Unsigned16? - The number of the disc this audio file came from
- discCount Unsigned16? - The total number of discs in this album
- duration int? - Duration of the audio file, expressed in milliseconds
- genre string? - The genre of this audio file
- hasDrm boolean? - Indicates if the file is protected with digital rights management
- isVariableBitrate boolean? - Indicates if the file is encoded with a variable bitrate
- title string? - The title of the audio file
- track Unsigned32? - The number of the track on the original disc for this audio file
- trackCount Unsigned32? - The total number of tracks on the original disc for this audio file
- year Unsigned32? - The year the audio file was recorded
microsoft.onedrive: ByteRange
Resource that contains the values to define a range of bytes.
Fields
- startByte int - The value of the starting index of bytes
- endByte int - The value of the ending index of bytes
microsoft.onedrive: CheckInOptions
Resource that contains options for Check-In a file.
Fields
- comment string - A check-in comment that is associated with the version
- checkInAs CheckInOption? - Optional. The status of the document after the check-in operation is complete.
Can be
published
orunspecified
.
microsoft.onedrive: ConnectionConfig
Client configuration details.
Fields
- Fields Included from *ConnectionConfig
- auth AuthConfig
- httpVersion HttpVersion
- http1Settings ClientHttp1Settings
- http2Settings ClientHttp2Settings
- timeout decimal
- forwarded string
- poolConfig PoolConfiguration
- cache CacheConfig
- compression Compression
- circuitBreaker CircuitBreakerConfig
- retryConfig RetryConfig
- responseLimits ResponseLimitConfigs
- secureSocket ClientSecureSocket
- proxy ProxyConfig
- validation boolean
- anydata...
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_1_1) - The HTTP version understood by the client
microsoft.onedrive: Deleted
Resource that indicates that the item has been deleted. The presence (non-null) of the resource value indicates that the file was deleted. A null (or missing) value indicates that the file is not deleted.
Fields
- state string? - Represents the state of the deleted item
microsoft.onedrive: DriveItem
Drive item data.
Fields
- name string? - Name of the item
- file File? - File metadata, if the item is a file
- folder Folder? - Folder metadata, if the item is a folder
- parentReference ParentReference? - Parent information, if the item has a parent
microsoft.onedrive: DriveItemData
Represents a file, folder, or other item stored in a drive.
Fields
- id string? - The unique identifier of the drive
- createdDateTime string? - Date and time of item creation
- cTag string? - An eTag for the content of the item. This eTag is not changed if only the metadata is changed. Note: This property is not returned if the item is a folder.
- eTag string? - ETag for the item
- lastModifiedDateTime string? - Date and time the item was last modified
- name string? - Name of the item
- size int? - Size of the item in bytes
- webUrl string? - URL that displays the resource in the browser
- description string? - Provides a user-visible description of the item
- webDavUrl string? - WebDAV compatible URL for the item
- root json? - If this property is non-null, it indicates that the driveItem is the top-most driveItem in the drive
- createdBy IdentitySet? - Identity of the user, device, or application which created the item
- lastModifiedBy IdentitySet? - Identity of the user, device, and application which last modified the item
- fileSystemInfo FileSystemInfo? - File system information on client
- parentReference ItemReference? - Parent information, if the item has a parent
- remoteItem DriveItemData? - Remote item data, if the item is shared from a drive other than the one being accessed
- downloadUrl string? - A URL that can be used to download this file's content. Authentication is not required with this URL.
- file File? - File metadata, if the item is a file
- folder Folder? - Folder metadata, if the item is a folder
- image Image? - Image metadata, if the item is an image
- photo Photo? - Photo metadata, if the item is a photo
- video Video? - Video metadata, if the item is a video
- audio Audio? - Audio metadata, if the item is an audio file
- location GeoCordinates? - Location metadata, if the item has location data
- package Package? - If present, indicates that this item is a package instead of a folder or file
- publication Publication? - Provides information about the published or checked-out state of an item, in locations that support such actions
- deleted Deleted? - Information about the deleted state of the item
- shared Shared? - Indicates that the item has been shared with others and provides information about the shared state of the
- searchResult SearchResult? - Search metadata, if the item is from a search result item
- sharepointIds SharePointId? - Returns identifiers useful for SharePoint REST compatibility
- specialFolder SpecialFolder? - If the current item is also available as a special folder, this facet is returned
- children DriveItemData[]? - Collection containing Item objects for the immediate children of item
- versions DriveItemVersion[]? - The list of previous versions of the item
- activities ItemActivity? - The list of recent activities that took place on this item
- permissions Permission[]? - The set of permissions for the item
- thumbnails ThumbnailSet[]? - Collection containing ThumbnailSet objects associated with the item
microsoft.onedrive: DriveItemVersion
Resource that represents a specific version of a DriveItem.
Fields
- id string? - The ID of the version
- lastModifiedBy IdentitySet? - Identity of the user which last modified the version
- lastModifiedDateTime string? - Date and time the version was last modified
- publication Publication? - Indicates the publication status of this particular version
- size int? - Indicates the size of the content stream for this version of the item
microsoft.onedrive: DriveRecipient
Resource that represents a person, group, or other recipient to share with using the invite action.
Fields
- email string - The email address for the recipient, if the recipient has an associated email address
- alias string? - The alias of the domain object, for cases where an email address is unavailable (e.g. security groups)
- objectId string? - The unique identifier for the recipient in the directory
microsoft.onedrive: EmbeddableData
Resource that represents the embeddable URLs for the preview.
Fields
- getUrl string? - URL suitable for embedding using HTTP GET (iframes, etc.)
- postUrl string? - URL suitable for embedding using HTTP POST (form post, JS, etc.)
- postParameters string? - POST parameters to include if using postUrl
microsoft.onedrive: File
Resource that groups file-related data items into a single structure.
Fields
- content byte[]? - A
byte[]
which represents the content of a file
- mimeType string? - The MIME type for the file
- hashes Hash? - Hashes of the file's binary content, if available
microsoft.onedrive: FileSystemInfo
Resource that contains properties that are reported by the device's local file system for the local version of an item.
Fields
- createdDateTime string? - The UTC date and time the file was created on a client
- lastAccessedDateTime string? - The UTC date and time the file was last accessed
- lastModifiedDateTime string? - The UTC date and time the file was last modified on a client
microsoft.onedrive: Folder
Resource that groups folder-related data on an item into a single structure.
Fields
- childCount Unsigned32? - Number of children contained immediately within this container
- view FolderView? - A collection of properties defining the recommended view for the folder
microsoft.onedrive: FolderMetadata
Represents necessary metadata in reference to a folder.
Fields
- name string - The name of the folder
- folder Folder(default { }) - Folder metadata, if the item is a folder
- parentReference ItemReference? - Parent information, if the item has a parent
- fileSystemInfo FileSystemInfo? - File system information on client
- conflictResolutionBehaviour ConflictResolutionBehaviour? - The conflict resolution behaviour
microsoft.onedrive: FolderView
Resource that provides or sets recommendations on the user-experience of a folder.
Fields
- sortBy SortBy? - The method by which the folder should be sorted
- sortOrder SortOrder? - Indicates that items should be sorted in descending order. Otherwise, items should be sorted ascending.
- viewType ViewType? - The type of view that should be used to represent the folder
microsoft.onedrive: GeoCordinates
Resource that provides geographic coordinates and elevation of a location based on metadata contained within the file.
Fields
- altitude float? - The altitude (height), in feet, above sea level for the item
- latitude float? - The latitude, in decimal, for the item
- longitude float? - he longitude, in decimal, for the item
microsoft.onedrive: Hash
Resource that groups available hashes into a single structure for an item.
Fields
- crc32Hash string? - The CRC32 value of the file in little endian (if available)
- sha1Hash string? - SHA1 hash for the contents of the file (if available)
- sha256Hash string? - SHA256 hash for the contents of the file (if available)
- quickXorHash string? - A proprietary hash of the file that can be used to determine if the contents of the file have changed
microsoft.onedrive: Identity
Represents an identity of a user, device, or application.
Fields
- displayName string? - The identity's display name
- id string? - Unique identifier for the identity
microsoft.onedrive: IdentitySet
represent a set of identities associated with various events for an item.
Fields
- application Identity? - The application associated with this action
- device Identity? - The device associated with this action
- user Identity? - The user associated with this action
microsoft.onedrive: Image
Resource that groups image-related properties into a single structure.
Fields
- width Unsigned32? - Width of the image, in pixels
- height Unsigned32? - Height of the image, in pixels
microsoft.onedrive: IncompleteData
Indicates that a resource was generated with incomplete data. The properties within might provide information about why the data is incomplete.
Fields
- missingDataBeforeDateTime string - The service does not have source data before the specified time
- wasThrottled boolean - Some data was not recorded due to excessive activity
microsoft.onedrive: ItemActionStat
Resource that provides aggregate details about an action over a period of time.
Fields
- actionCount Unsigned32 - The number of times the action took place
- actorCount Unsigned32 - The number of distinct actors that performed the action
microsoft.onedrive: ItemActivity
Resource that provides information about activities that took place on an item or within a container.
Fields
- id string? - The unique identifier of the activity
- actor IdentitySet? - Identity of who performed the action
- driveItem DriveItemData? - The driveItem that was the target of this activity
- activityDateTime string? - Details about when the activity took place
microsoft.onedrive: ItemActivityStat
Resource that provides information about activities that took place within an interval of time.
Fields
- activities ItemActivity[]? - Exposes the itemActivities represented in this itemActivityStat resource
- incompleteData IncompleteData? - Indicates that the statistics in this interval are based on incomplete data
- isTrending boolean? - Indicates whether the item is "trending"
- startDateTime string? - When the interval starts
- endDateTime string? - When the interval ends
- create ItemActionStat? - Statistics about the
create
actions in this interval
- delete ItemActionStat? - Statistics about the
edit
actions in this interval
- edit ItemActionStat? - Statistics about the
delete
actions in this interval
- move ItemActionStat? - Statistics about the
move
actions in this interval
- access ItemActionStat? - Statistics about the
access
actions in this interval
microsoft.onedrive: ItemAnalytics
Resource that provides analytics about activities that took place on an item. This resource is currently only available on SharePoint and OneDrive for Business.
Fields
- allTime ItemActivityStat? - Analytics over the item's lifespan
- lastSevenDays ItemActivityStat? - Analytics for the last seven days
microsoft.onedrive: ItemReference
Provides information necessary to address a DriveItem via the API.
Fields
- driveId string? - Unique identifier of the drive instance that contains the item
- driveType string? - Identifies the type of drive
- id string? - Unique identifier of the item in the drive
- name string? - The name of the item being referenced
- path string? - Path that can be used to navigate to the item
- shareId string? - A unique identifier for a shared resource that can be accessed via the Shares API
- sharepointIds SharePointId? - Returns identifiers useful for SharePoint REST compatibility
microsoft.onedrive: ItemShareInvitation
Resource that represents necessary information for a sharing invitation.
Fields
- requireSignIn boolean(default false) - Specifies whether the recipient of the invitation is required to sign-in to view the shared item.
- sendInvitation boolean(default false) - If true, a sharing link is sent to the recipient. Otherwise, a permission is granted directly without sending a notification.
- roles PermissionRole[] - Specify the roles that are to be granted to the recipients of the sharing invitation
- recipients DriveRecipient[] - A collection of recipients who will receive access and the sharing invitation
- message string? - A plain text formatted message that is included in the sharing invitation. Maximum length 2000 characters.
microsoft.onedrive: Package
Resource that indicates if a DriveItem is the top level item in a "package" or a collection of items that should be treated as a collection instead of individual items.
Fields
- 'type string? - A string indicating the type of package
microsoft.onedrive: ParentReference
The reference data for the destination folder where the item will be copied.
Fields
- id string - ID of the destination folder
- driveId string? - ID of the Drive the destination folder exist
microsoft.onedrive: Permission
Resource that provides information about a sharing permission granted for a DriveItem resource.
Fields
- id string? - The unique identifier of the permission among all permissions on the item
- grantedTo IdentitySet? - For user type permissions, the details of the users & applications for this permission
- grantedToIdentities IdentitySet[]? - For link type permissions, the details of the users to whom permission was granted
- inheritedFrom ItemReference? - Provides a reference to the ancestor of the current permission, if it is inherited from an ancestor
- invitation ShareInvitation? - Details of any associated sharing invitation for this permission
- link SharingLink? - Provides the link details of the current permission, if it is a link type permissions
- roles string[]? - The type of permission
- shareId string? - A unique token that can be used to access this shared item via the shares API
microsoft.onedrive: PermissionOptions
Resource that defines properties of the sharing link your application is requesting.
Fields
- 'type LinkType - The type of sharing link to create. Either
view
,edit
, orembed
- scope LinkScope? - The scope of link to create. Either
anonymous
ororganization
- password string? - The password of the sharing link that is set by the creator. Optional and OneDrive Personal only.
- expirationDateTime string? - A String with format of yyyy-MM-ddTHH:mm:ssZ of DateTime indicates the expiration time of the permission
microsoft.onedrive: Photo
Resource that provides photo and camera properties.
Fields
- cameraMake string? - Camera manufacturer
- cameraModel string? - Camera model
- exposureDenominator float? - The denominator for the exposure time fraction from the camera
- exposureNumerator float? - The numerator for the exposure time fraction from the camera
- fNumber float? - The F-stop value from the camera
- focalLength float? - The focal length from the camera
- iso Unsigned32? - The ISO value from the camera
- takenDateTime string? - Represents the date and time the photo was taken
microsoft.onedrive: PreviewOptions
Resource that contain defines properties of the embeddable URL your application is requesting.
Fields
- zoom int? - Optional. Zoom level to start at, if applicable.
microsoft.onedrive: Publication
Resource that provides details on the published status of a driveItemVersion or driveItem resource.
Fields
- level PublicationLevel? - The state of publication for this document. Either
published
orcheckout
.
- versionId string? - The unique identifier for the version that is visible to the current caller
microsoft.onedrive: SearchResult
Resource that indicates than an item is the response to a search query.
Fields
- onClickTelemetryUrl string? - A callback URL that can be used to record telemetry information.
microsoft.onedrive: Shared
Resource that indicates a DriveItem has been shared with others.
Fields
- owner IdentitySet? - The identity of the owner of the shared item
- scope string? - Indicates the scope of how the item is shared:
anonymous
,organization
, orusers
- sharedBy IdentitySet? - The identity of the user who shared the item
- sharedDateTime string? - he UTC date and time when the item was shared
microsoft.onedrive: ShareInvitation
Resource that groups invitation-related data items into a single structure.
Fields
- email string? - The email address provided for the recipient of the sharing invitation
- invitedBy IdentitySet? - Provides information about who sent the invitation that created this permission, if that information is available
- signInRequired boolean? - If true the recipient of the invitation needs to sign in in order to access the shared item
microsoft.onedrive: SharePointId
Resource that groups the various identifiers for an item stored in a SharePoint site or OneDrive for Business into a single structure.
Fields
- listId string? - The unique identifier (guid) for the item's list in SharePoint
- listItemId string? - An integer identifier for the item within the containing list
- listItemUniqueId string? - The unique identifier (guid) for the item within OneDrive for Business or a SharePoint site
- siteId string? - The unique identifier (guid) for the item's site collection (SPSite)
- siteUrl string? - The SharePoint URL for the site that contains the item
- tenantId string? - The unique identifier (guid) for the tenancy
- webId string? - The unique identifier (guid) for the item's site (SPWeb)
microsoft.onedrive: SharingLink
Resource that groups link-related data items into a single structure.
Fields
- application Identity? - The app the link is associated with
- 'type LinkType? - The type of the link created
- scope LinkScope? - The scope of the link represented by this permission. Value
anonymous
indicates the link is usable by anyone,organization
indicates the link is only usable for users signed into the same tenant.
- webHtml string? - For
embed
links, this property contains the HTML code for an <iframe> element that will embed the item in a webpage.
- webUrl string? - A URL that opens the item in the browser on the OneDrive website.
microsoft.onedrive: SpecialFolder
Resource that groups special folder-related data items into a single structure.
Fields
- name string? - The unique identifier for this item in the /drive/special collection
microsoft.onedrive: ThumbnailSet
Resource which is a keyed collection of thumbnail resources. It is used to represent a set of thumbnails associated with a DriveItem.
Fields
- id string? - The id within the item
- large Tumbnail? - A 1920x1920 scaled thumbnail
- medium Tumbnail? - A 176x176 scaled thumbnail
- small Tumbnail? - A 48x48 cropped thumbnail
- 'source Tumbnail? - A custom thumbnail image or the original image used to generate other thumbnails
microsoft.onedrive: Tumbnail
Resource that represents a thumbnail for an image, video, document, or any item that has a bitmap representation.
Fields
- height Unsigned32? - The height of the thumbnail, in pixels
- width Unsigned32? - The width of the thumbnail, in pixels
- sourceItemId string? - The unique identifier of the item that provided the thumbnail. This is only available when a folder thumbnail is requested.
- url string? - The URL used to fetch the thumbnail content
microsoft.onedrive: UploadMetadata
Resource that provide additional data about the file being uploaded and customizing the semantics of the upload operation.
Fields
- fileSize int - Provides an expected file size to perform a quota check prior to upload. Only on OneDrive Personal.
- name string? - The name of the item (filename and extension)
- description string? - Provides a user-visible description of the item. Only on OneDrive Personal.
- fileSystemInfo FileSystemInfo? - File system information on client
- conflictResolutionBehaviour ConflictResolutionBehaviour? - The conflict resolution behaviour
microsoft.onedrive: Video
Resource that groups video-related data items into a single structure.
Fields
- audioBitsPerSample Unsigned32? - Number of audio bits per sample
- audioChannels Unsigned32? - Number of audio channels
- audioFormat string? - Name of the audio format (AAC, MP3, etc.)
- audioSamplesPerSecond Unsigned32? - Number of audio samples per second
- bitrate Unsigned32? - Bit rate of the video in bits per second
- duration int? - Duration of the file in milliseconds
- fourCC string? - "Four character code" name of the video format
- frameRate float? - Frame rate of the video
- height Unsigned32? - Height of the video, in pixels
- width Unsigned32? - Width of the video, in pixels
Errors
microsoft.onedrive: Error
Union of all types of errors.
microsoft.onedrive: InputValidationError
An error which occur when providing an invalid value.
microsoft.onedrive: PayloadValidationError
An errror occur due to an invalid payload received.
microsoft.onedrive: QueryParameterValidationError
An error which occur when there is an invalid query parameter.
microsoft.onedrive: RequestFailedError
An error occur due to a failed failed request attempt.
Import
import ballerinax/microsoft.onedrive;
Metadata
Released date: about 2 years ago
Version: 2.3.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.2.1
GraalVM compatible: Yes
Pull count
Total: 2254
Current verison: 196
Weekly downloads
Keywords
Content & Files/File Management & Storage
Cost/Freemium
Vendor/Microsoft
Contributors