hubspot.marketing
Module hubspot.marketing
API
Definitions
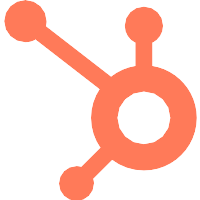
ballerinax/hubspot.marketing Ballerina library
Overview
This is a generated connector from HubSpot OpenAPI specification.
These APIs allow you to interact with HubSpot's Marketing Events Extension.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a HubSpot developer account
- Obtain tokens
- Use this guide to obtain the API keys related to your account.
Quickstart
To use the HubSpot Marketing connector in your Ballerina application, update the .bal file as follows:
Step 1 - Import connector
First, import the ballerinax/hubspot.marketing module into the Ballerina project.
import ballerinax/hubspot.marketing;
Step 2 - Create a new connector instance
You can now make the connection configuration using the access token.
marketing:ApiKeysConfig config = { hapikey : "<your apiKey>" }; marketing:Client baseClient = check new Client(clientConfig);
Step 3 - Invoke connector operation
- Create a marketing event
marketing:MarketingEventCreateRequestParams event = { eventName: "<EVENT_NAME>", eventOrganizer: "<EVENT_ORGANIZER>", customProperties: [], externalAccountId: "<EXTERNAL_ACCOUNT_ID>", externalEventId: "<EXTERNAL_EVENT_ID>" }; marketing:MarketingEventDefaultResponse|error bEvent = baseClient->createMarketingEvent(event); if (bEvent is marketing:MarketingEventDefaultResponse) { log:printInfo("Created behavoural event"); } else { log:printInfo(msg = bEvent.message()); }
- Use
bal run
command to compile and run the Ballerina program
Clients
hubspot.marketing: Client
This is a generated connector from HubSpot OpenAPI specification. These APIs allow you to interact with HubSpot's Marketing Events Extension.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a HubSpot account and obtain the API Key following this guide.
init (ApiKeysConfig apiKeyConfig, ConnectionConfig config, string serviceUrl)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.hubapi.com" - URL of the target service
createMarketingEvent
function createMarketingEvent(MarketingEventCreateRequest payload) returns MarketingEventDefaultResponse|error
Create a marketing event
Parameters
- payload MarketingEventCreateRequest - The details of the marketing event to create
Return Type
- MarketingEventDefaultResponse|error - successful operation
posteventsDelete
function posteventsDelete(MarketingEventExternalUniqueIdentifierBatch payload) returns Response|error
Delete multiple marketing events
Parameters
- payload MarketingEventExternalUniqueIdentifierBatch - The details of the marketing events to delete
eventsSearch
function eventsSearch(string q) returns MarketingEventSearchResponse|error
Search for marketing events
Parameters
- q string - The partial event id to search for
Return Type
- MarketingEventSearchResponse|error - successful operation
eventsCreateOrUpsert
function eventsCreateOrUpsert(MarketingEventBatchCreateRequest payload) returns MarketingEventCollectionResponse|error
Create or update multiple marketing events
Parameters
- payload MarketingEventBatchCreateRequest - The details of the marketing events to upsert
Return Type
- MarketingEventCollectionResponse|error - successful operation
getEventsExternaleventid
function getEventsExternaleventid(string externalEventId, string externalAccountId) returns PublicMarketingEventReadResponse|error
Get a marketing event
Parameters
- externalEventId string - The id of the marketing event to return
- externalAccountId string - The account id associated with the marketing event
Return Type
- PublicMarketingEventReadResponse|error - successful operation
updateEventsExternaleventid
function updateEventsExternaleventid(string externalEventId, MarketingEventCreateRequest payload) returns PublicMarketingEventResponse|error
Create or update a marketing event
Parameters
- externalEventId string - The id of the marketing event to upsert
- payload MarketingEventCreateRequest - The details of the marketing event to upsert
Return Type
- PublicMarketingEventResponse|error - successful operation
deleteEventsExternaleventid
function deleteEventsExternaleventid(string externalEventId, string externalAccountId) returns Response|error
Delete a marketing event
Parameters
- externalEventId string - The id of the marketing event to delete
- externalAccountId string - The account id associated with the marketing event
updateEvents
function updateEvents(string externalEventId, string externalAccountId, MarketingEventUpdateRequest payload) returns PublicMarketingEventResponse|error
Update a marketing event
Parameters
- externalEventId string - The id of the marketing event to update
- externalAccountId string - The account id associated with the marketing event
- payload MarketingEventUpdateRequest - The details of the marketing event to update
Return Type
- PublicMarketingEventResponse|error - successful operation
eventsExternaleventidCancel
function eventsExternaleventidCancel(string externalEventId, string externalAccountId) returns MarketingEventDefaultResponse|error
Mark a marketing event as cancelled
Parameters
- externalEventId string - The id of the marketing event to mark as cancelled
- externalAccountId string - The account id associated with the marketing event
Return Type
- MarketingEventDefaultResponse|error - successful operation
externaleventidSubscriberstateEmailUpsert
function externaleventidSubscriberstateEmailUpsert(string externalEventId, string subscriberState, string externalAccountId, MarketingEventEmailSubscriberBatch payload) returns Response|error
Record
Parameters
- externalEventId string - The id of the marketing event
- subscriberState string - The new subscriber state for the HubSpot contacts and the specified marketing event
- externalAccountId string - The account id associated with the marketing event
- payload MarketingEventEmailSubscriberBatch - The details of the contacts to subscribe to the event
eventsExternaleventidSubscriberstateUpsert
function eventsExternaleventidSubscriberstateUpsert(string externalEventId, string subscriberState, string externalAccountId, MarketingEventSubscriberBatch payload) returns Response|error
Record
Parameters
- externalEventId string - The id of the marketing event
- subscriberState string - The new subscriber state for the HubSpot contacts and the specified marketing event
- externalAccountId string - The account id associated with the marketing event
- payload MarketingEventSubscriberBatch - The details of the contacts to subscribe to the event
getAppidSettings
function getAppidSettings(int appId) returns EventDetailSettings|error
Retrieve the application settings
Parameters
- appId int - The id of the application to retrieve the settings for.
Return Type
- EventDetailSettings|error - successful operation
postAppidSettings
function postAppidSettings(int appId, EventDetailSettingsUrl payload) returns EventDetailSettings|error
Update the application settings
Parameters
- appId int - The id of the application to update the settings for.
- payload EventDetailSettingsUrl - The new application settings
Return Type
- EventDetailSettings|error - successful operation
Records
hubspot.marketing: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- hapikey string - Represents API Key
hapikey
hubspot.marketing: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
hubspot.marketing: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
hubspot.marketing: Error
Fields
- message string - A human readable message describing the error along with remediation steps where appropriate
- correlationId string - A unique identifier for the request. Include this value with any error reports or support tickets
- category string - The error category
- subCategory string? - A specific category that contains more specific detail about the error
- errors ErrorDetail[]? - further information about the error
- context record {}? - Context about the error condition
- links record {}? - A map of link names to associated URIs containing documentation about the error or recommended remediation steps
hubspot.marketing: ErrorCategory
Fields
- httpStatus string -
- name string -
hubspot.marketing: ErrorDetail
Fields
- message string - A human readable message describing the error along with remediation steps where appropriate
- 'in string? - The name of the field or parameter in which the error was found.
- code string? - The status code associated with the error detail
- subCategory string? - A specific category that contains more specific detail about the error
- context record {}? - Context about the error condition
hubspot.marketing: EventDetailSettings
Event detail settings
Fields
- appId int - The id of the application the settings are for
- eventDetailsUrl string - The url that will be used to fetch marketing event details by id
hubspot.marketing: EventDetailSettingsUrl
Event detail URL
Fields
- eventDetailsUrl string - The url that will be used to fetch marketing event details by id. Must contain a
%s
character sequence that will be substituted with the event id. For example:https://my.event.app/events/%s
hubspot.marketing: MarketingEventBatchCreateRequest
Fields
- inputs MarketingEventCreateRequest[] -
hubspot.marketing: MarketingEventCollectionResponse
Batch marketing event info
Fields
- status string - Status of the event
- results PublicMarketingEventResponse[] - Marketing event info
- numErrors int? - Error count
- errors StandardError[]? - Errors
- requestedAt string? - Requested at
- startedAt string - Started at
- completedAt string - Completed at
- links record {}? - Links
hubspot.marketing: MarketingEventCreateRequest
Marketing event
Fields
- eventName string - The name of the marketing event.
- eventType string? - Describes what type of event this is. For example:
WEBINAR
,CONFERENCE
,WORKSHOP
- startDateTime string? - The start date and time of the marketing event.
- endDateTime string? - The end date and time of the marketing event.
- eventOrganizer string - The name of the organizer of the marketing event.
- eventDescription string? - The description of the marketing event.
- eventUrl string? - A URL in the external event application where the marketing event can be managed.
- eventCancelled boolean? - Indicates if the marketing event has been cancelled. Defaults to
false
- customProperties PropertyValue[] - A list of PropertyValues. These can be whatever kind of property names and values you want. However, they must already exist on the HubSpot account's definition of the MarketingEvent Object. If they don't they will be filtered out and not set. In order to do this you'll need to create a new PropertyGroup on the HubSpot account's MarketingEvent object for your specific app and create the Custom Property you want to track on that HubSpot account. Do not create any new default properties on the MarketingEvent object as that will apply to all HubSpot accounts.
- externalAccountId string - The accountId that is associated with this marketing event in the external event application.
- externalEventId string - The id of the marketing event in the external event application.
hubspot.marketing: MarketingEventDefaultResponse
Marketing event
Fields
- eventName string - The name of the marketing event.
- eventType string? - The type of the marketing event.
- startDateTime string? - The start date and time of the marketing event.
- endDateTime string? - The end date and time of the marketing event.
- eventOrganizer string - The name of the organizer of the marketing event.
- eventDescription string? - The description of the marketing event.
- eventUrl string? - The URL in the external event application where the marketing event can be managed.
- eventCancelled boolean? - Indicates if the marketing event has been cancelled.
- customProperties PropertyValue[] - A list of PropertyValues. These can be whatever kind of property names and values you want. However, they must already exist on the HubSpot account's definition of the MarketingEvent Object. If they don't they will be filtered out and not set. In order to do this you'll need to create a new PropertyGroup on the HubSpot account's MarketingEvent object for your specific app and create the Custom Property you want to track on that HubSpot account. Do not create any new default properties on the MarketingEvent object as that will apply to all HubSpot accounts.
hubspot.marketing: MarketingEventEmailSubscriber
Marketing event email subscriber
Fields
- interactionDateTime int - The date and time at which the contact subscribed to the event.
- email string - The email address of the contact in HubSpot to associate with the event. Note that the contact must already exist in HubSpot; a contact will not be created.
hubspot.marketing: MarketingEventEmailSubscriberBatch
List of marketing event details to create or update
Fields
- inputs MarketingEventEmailSubscriber[] - List of marketing event details to create or update
hubspot.marketing: MarketingEventExternalUniqueIdentifier
External unique identifier of marketing event
Fields
- appId int - The id of the application that created the marketing event in HubSpot.
- externalAccountId string - The accountId that is associated with this marketing event in the external event application.
- externalEventId string - The id of the marketing event in the external event application.
hubspot.marketing: MarketingEventExternalUniqueIdentifierBatch
Fields
- inputs MarketingEventExternalUniqueIdentifier[] -
hubspot.marketing: MarketingEventSearchResponse
Fields
- results MarketingEventExternalUniqueIdentifier[] -
hubspot.marketing: MarketingEventSubscriber
Marketing event subscriber
Fields
- interactionDateTime int - The date and time at which the contact subscribed to the event.
- vid int - VID
hubspot.marketing: MarketingEventSubscriberBatch
List of HubSpot contacts to subscribe to the marketing event
Fields
- inputs MarketingEventSubscriber[] - List of HubSpot contacts to subscribe to the marketing event
hubspot.marketing: MarketingEventUpdateRequest
Marketing event update parameters
Fields
- eventName string? - The name of the marketing event.
- eventType string? - Describes what type of event this is. For example:
WEBINAR
,CONFERENCE
,WORKSHOP
- startDateTime string? - The start date and time of the marketing event.
- endDateTime string? - The end date and time of the marketing event.
- eventOrganizer string? - The name of the organizer of the marketing event.
- eventDescription string? - The description of the marketing event.
- eventUrl string? - A URL in the external event application where the marketing event can be managed.
- eventCancelled boolean? - Indicates if the marketing event has been cancelled. Defaults to
false
- customProperties PropertyValue[] - A list of PropertyValues. These can be whatever kind of property names and values you want. However, they must already exist on the HubSpot account's definition of the MarketingEvent Object. If they don't they will be filtered out and not set. In order to do this you'll need to create a new PropertyGroup on the HubSpot account's MarketingEvent object for your specific app and create the Custom Property you want to track on that HubSpot account. Do not create any new default properties on the MarketingEvent object as that will apply to all HubSpot accounts.
hubspot.marketing: PropertyValue
Fields
- name string -
- value string -
- timestamp int -
- sourceId string -
- sourceLabel string -
- 'source string -
- selectedByUser boolean -
- selectedByUserTimestamp int -
- sourceVid int[] -
- sourceMetadata string - Source metadata encoded as a base64 string. For example:
ZXhhbXBsZSBzdHJpbmc=
- requestId string -
- updatedByUserId int -
hubspot.marketing: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
hubspot.marketing: PublicMarketingEventReadResponse
Maketing event read response
Fields
- eventName string - The name of the marketing event.
- eventType string? - The type of the marketing event.
- startDateTime string? - The start date and time of the marketing event.
- endDateTime string? - The end date and time of the marketing event.
- eventOrganizer string - The name of the organizer of the marketing event.
- eventDescription string? - The description of the marketing event.
- eventUrl string? - A URL in the external event application where the marketing event can be managed.
- eventCancelled boolean? - Indicates if the marketing event has been cancelled.
- customProperties PropertyValue[] - A list of PropertyValues. These can be whatever kind of property names and values you want. However, they must already exist on the HubSpot account's definition of the MarketingEvent Object. If they don't they will be filtered out and not set. In order to do this you'll need to create a new PropertyGroup on the HubSpot account's MarketingEvent object for your specific app and create the Custom Property you want to track on that HubSpot account. Do not create any new default properties on the MarketingEvent object as that will apply to all HubSpot accounts.
- externalEventId string - The id of the marketing event in the external event application.
- registrants int - The number of HubSpot contacts that registered for this marketing event.
- attendees int - The number of HubSpot contacts that attended this marketing event.
- cancellations int - The number of HubSpot contacts that registered for this marketing event, but later cancelled their registration.
- noShows int - The number of HubSpot contacts that registered for this marketing event, but did not attend. This field only had a value when the event is over.
- createdAt string - Created date/time
- updatedAt string - Updated date/time
- id string - ID
hubspot.marketing: PublicMarketingEventResponse
Public marketing event info
Fields
- eventName string - The name of the marketing event.
- eventType string? - The type of the marketing event.
- startDateTime string? - The start date and time of the marketing event.
- endDateTime string? - The end date and time of the marketing event.
- eventOrganizer string - The name of the organizer of the marketing event.
- eventDescription string? - The description of the marketing event.
- eventUrl string? - A URL in the external event application where the marketing event can be managed.
- eventCancelled boolean? - Indicates if the marketing event has been cancelled.
- customProperties PropertyValue[] - A list of PropertyValues. These can be whatever kind of property names and values you want. However, they must already exist on the HubSpot account's definition of the MarketingEvent Object. If they don't they will be filtered out and not set. In order to do this you'll need to create a new PropertyGroup on the HubSpot account's MarketingEvent object for your specific app and create the Custom Property you want to track on that HubSpot account. Do not create any new default properties on the MarketingEvent object as that will apply to all HubSpot accounts.
- id string - ID
- createdAt string - Created date/time
- updatedAt string - Updated date/time
hubspot.marketing: StandardError
Fields
- status string -
- id string? -
- category ErrorCategory -
- subCategory record {}? -
- message string -
- errors ErrorDetail[] -
- context record {} -
- links record {} -
Import
import ballerinax/hubspot.marketing;
Metadata
Released date: over 1 year ago
Version: 2.3.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 2
Current verison: 0
Weekly downloads
Keywords
Marketing/Marketing Automation
Cost/Freemium
Contributors
Dependencies