googleapis.tasks
Module googleapis.tasks
API
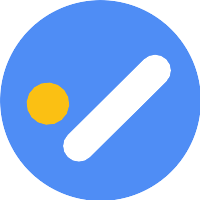
ballerinax/googleapis.tasks Ballerina library
Overview
This is a generated connector for Google Tasks API v1 OpenAPI specification. The Google Tasks API lets you manage your tasks and task lists.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Google account
- Obtain tokens - Follow this link
Quickstart
To use the Google Tasks connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/googleapis.tasks
module into the Ballerina project.
import ballerinax/googleapis.tasks;
Step 2: Create a new connector instance
Create a tasks:ClientConfig
with the OAuth2 tokens obtained, and initialize the connector with it.
tasks:ClientConfig clientConfig = { auth: { clientId: <CLIENT_ID>, clientSecret: <CLIENT_SECRET>, refreshUrl: <REFRESH_URL>, refreshToken: <REFRESH_TOKEN> } }; tasks:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to return all the authenticated user's task lists using the connector.
Return all the authenticated user's task lists
public function main() { tasks:TaskLists|error response = baseClient->listTasklists(); if (response is tasks:TaskLists) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
googleapis.tasks: Client
This is a generated connector for Google Tasks API v1 OpenAPI specification. The Google Tasks API lets you manage your tasks and task lists.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Google account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://tasks.googleapis.com/" - URL of the target service
clearTasks
function clearTasks(string tasklist, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Response|error
Clears all completed tasks from the specified task list. The affected tasks will be marked as 'hidden' and no longer be returned by default when retrieving all tasks for a task list.
Parameters
- tasklist string - Task list identifier.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
listTasks
function listTasks(string tasklist, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? completedMax, string? completedMin, string? dueMax, string? dueMin, int? maxResults, string? pageToken, boolean? showCompleted, boolean? showDeleted, boolean? showHidden, string? updatedMin) returns Tasks|error
Returns all tasks in the specified task list.
Parameters
- tasklist string - Task list identifier.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- completedMax string? (default ()) - Upper bound for a task's completion date (as a RFC 3339 timestamp) to filter by. Optional. The default is not to filter by completion date.
- completedMin string? (default ()) - Lower bound for a task's completion date (as a RFC 3339 timestamp) to filter by. Optional. The default is not to filter by completion date.
- dueMax string? (default ()) - Upper bound for a task's due date (as a RFC 3339 timestamp) to filter by. Optional. The default is not to filter by due date.
- dueMin string? (default ()) - Lower bound for a task's due date (as a RFC 3339 timestamp) to filter by. Optional. The default is not to filter by due date.
- maxResults int? (default ()) - Maximum number of task lists returned on one page. Optional. The default is 20 (max allowed: 100).
- pageToken string? (default ()) - Token specifying the result page to return. Optional.
- showCompleted boolean? (default ()) - Flag indicating whether completed tasks are returned in the result. Optional. The default is True. Note that showHidden must also be True to show tasks completed in first party clients, such as the web UI and Google's mobile apps.
- showDeleted boolean? (default ()) - Flag indicating whether deleted tasks are returned in the result. Optional. The default is False.
- showHidden boolean? (default ()) - Flag indicating whether hidden tasks are returned in the result. Optional. The default is False.
- updatedMin string? (default ()) - Lower bound for a task's last modification time (as a RFC 3339 timestamp) to filter by. Optional. The default is not to filter by last modification time.
insertTasks
function insertTasks(string tasklist, Task payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? parent, string? previous) returns Task|error
Creates a new task on the specified task list.
Parameters
- tasklist string - Task list identifier.
- payload Task - Task request
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- parent string? (default ()) - Parent task identifier. If the task is created at the top level, this parameter is omitted. Optional.
- previous string? (default ()) - Previous sibling task identifier. If the task is created at the first position among its siblings, this parameter is omitted. Optional.
getTasks
function getTasks(string tasklist, string task, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Task|error
Returns the specified task.
Parameters
- tasklist string - Task list identifier.
- task string - Task identifier.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
updateTasks
function updateTasks(string tasklist, string task, Task payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Task|error
Updates the specified task.
Parameters
- tasklist string - Task list identifier.
- task string - Task identifier.
- payload Task - Task request
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
deleteTasks
function deleteTasks(string tasklist, string task, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Response|error
Deletes the specified task from the task list.
Parameters
- tasklist string - Task list identifier.
- task string - Task identifier.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
patchTasks
function patchTasks(string tasklist, string task, Task payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Task|error
Updates the specified task. This method supports patch semantics.
Parameters
- tasklist string - Task list identifier.
- task string - Task identifier.
- payload Task - Task request
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
moveTasks
function moveTasks(string tasklist, string task, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, string? parent, string? previous) returns Task|error
Moves the specified task to another position in the task list. This can include putting it as a child task under a new parent and/or move it to a different position among its sibling tasks.
Parameters
- tasklist string - Task list identifier.
- task string - Task identifier.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- parent string? (default ()) - New parent task identifier. If the task is moved to the top level, this parameter is omitted. Optional.
- previous string? (default ()) - New previous sibling task identifier. If the task is moved to the first position among its siblings, this parameter is omitted. Optional.
listTasklists
function listTasklists(string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType, int? maxResults, string? pageToken) returns TaskLists|error
Returns all the authenticated user's task lists.
Parameters
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- maxResults int? (default ()) - Maximum number of task lists returned on one page. Optional. The default is 20 (max allowed: 100).
- pageToken string? (default ()) - Token specifying the result page to return. Optional.
insertTasklists
function insertTasklists(TaskList payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns TaskList|error
Creates a new task list and adds it to the authenticated user's task lists.
Parameters
- payload TaskList - TaskList request
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
getTasklists
function getTasklists(string tasklist, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns TaskList|error
Returns the authenticated user's specified task list.
Parameters
- tasklist string - Task list identifier.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
updateTasklists
function updateTasklists(string tasklist, TaskList payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns TaskList|error
Updates the authenticated user's specified task list.
Parameters
- tasklist string - Task list identifier.
- payload TaskList - TaskList request
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
deleteTasklists
function deleteTasklists(string tasklist, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns Response|error
Deletes the authenticated user's specified task list.
Parameters
- tasklist string - Task list identifier.
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
patchTasklists
function patchTasklists(string tasklist, TaskList payload, string? xgafv, string? alt, string? callback, string? fields, string? quotaUser, string? uploadProtocol, string? uploadType) returns TaskList|error
Updates the authenticated user's specified task list. This method supports patch semantics.
Parameters
- tasklist string - Task list identifier.
- payload TaskList - TaskList request
- xgafv string? (default ()) - V1 error format.
- alt string? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- uploadProtocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Records
googleapis.tasks: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
googleapis.tasks: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
googleapis.tasks: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://accounts.google.com/o/oauth2/token") - Refresh URL
googleapis.tasks: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
googleapis.tasks: Task
Fields
- completed string? - Completion date of the task (as a RFC 3339 timestamp). This field is omitted if the task has not been completed.
- deleted boolean? - Flag indicating whether the task has been deleted. The default is False.
- due string? - Due date of the task (as a RFC 3339 timestamp). Optional. The due date only records date information; the time portion of the timestamp is discarded when setting the due date. It isn't possible to read or write the time that a task is due via the API.
- etag string? - ETag of the resource.
- hidden boolean? - Flag indicating whether the task is hidden. This is the case if the task had been marked completed when the task list was last cleared. The default is False. This field is read-only.
- id string? - Task identifier.
- kind string? - Type of the resource. This is always "tasks#task".
- links TaskLinks[]? - Collection of links. This collection is read-only.
- notes string? - Notes describing the task. Optional.
- parent string? - Parent task identifier. This field is omitted if it is a top-level task. This field is read-only. Use the "move" method to move the task under a different parent or to the top level.
- position string? - String indicating the position of the task among its sibling tasks under the same parent task or at the top level. If this string is greater than another task's corresponding position string according to lexicographical ordering, the task is positioned after the other task under the same parent task (or at the top level). This field is read-only. Use the "move" method to move the task to another position.
- selfLink string? - URL pointing to this task. Used to retrieve, update, or delete this task.
- status string? - Status of the task. This is either "needsAction" or "completed".
- title string? - Title of the task.
- updated string? - Last modification time of the task (as a RFC 3339 timestamp).
googleapis.tasks: TaskLinks
Fields
- description string? - The description. In HTML speak: Everything between <a> and </a>.
- link string? - The URL.
- 'type string? - Type of the link, e.g. "email".
googleapis.tasks: TaskList
Fields
- etag string? - ETag of the resource.
- id string? - Task list identifier.
- kind string? - Type of the resource. This is always "tasks#taskList".
- selfLink string? - URL pointing to this task list. Used to retrieve, update, or delete this task list.
- title string? - Title of the task list.
- updated string? - Last modification time of the task list (as a RFC 3339 timestamp).
googleapis.tasks: TaskLists
Fields
- etag string? - ETag of the resource.
- items TaskList[]? - Collection of task lists.
- kind string? - Type of the resource. This is always "tasks#taskLists".
- nextPageToken string? - Token that can be used to request the next page of this result.
googleapis.tasks: Tasks
Fields
- etag string? - ETag of the resource.
- items Task[]? - Collection of tasks.
- kind string? - Type of the resource. This is always "tasks#tasks".
- nextPageToken string? - Token used to access the next page of this result.
Import
import ballerinax/googleapis.tasks;
Metadata
Released date: about 1 year ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 0
Current verison: 0
Weekly downloads
Keywords
Productivity/Task Management
Cost/Free
Vendor/Google
Contributors
Dependencies