googleapis.gmail
Module googleapis.gmail
Declarations
Definitions
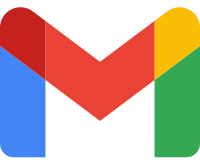
ballerinax/googleapis.gmail Ballerina library
Package overview
Gmail is a widely-used email service provided by Google LLC, enabling users to send and receive emails over the internet.
The ballerinax/googleapis.gmail
package offers APIs to connect and interact with Gmail API endpoints, specifically based on Gmail API v1.
Setup guide
To use the Gmail connector, you must have access to the Gmail REST API through a Google Cloud Platform (GCP) account and a project under it. If you do not have a GCP account, you can sign up for one here.
Step 1: Create a Google Cloud Platform Project
-
Open the Google Cloud Platform Console.
-
Click on the project drop-down menu and select an existing project or create a new one for which you want to add an API key.
Step 2: Enable Gmail API
-
Navigate to the Library tab and enable the Gmail API.
Step 3: Configure OAuth consent
-
Click on the OAuth consent screen tab in the Google Cloud Platform console.
-
Provide a name for the consent application and save your changes.
Step 4: Create OAuth client
-
Navigate to the Credentials tab in your Google Cloud Platform console.
-
Click on Create credentials and select OAuth client ID from the dropdown menu.
-
You will be directed to the Create OAuth client ID screen, where you need to fill in the necessary information as follows:
Field Value Application type Web Application Name GmailConnector Authorized Redirect URIs https://developers.google.com/oauthplayground -
After filling in these details, click on Create.
-
Make sure to save the provided Client ID and Client secret.
Step 5: Get the Access and Refresh token
Note: It is recommended to use the OAuth 2.0 playground to obtain the tokens.
-
Configure the OAuth playground with the OAuth client ID and client secret.
-
Authorize the Gmail APIs (Select all except the metadata scope).
-
Exchange the authorization code for tokens.
Quickstart
To use the gmail
connector in your Ballerina application, modify the .bal
file as follows:
Step 1: Import the module
Import the gmail
module.
import ballerinax/googleapis.gmail;
Step 2: Instantiate a new connector
Create a gmail:ConnectionConfig
with the obtained OAuth2.0 tokens and initialize the connector with it.
configurable string refreshToken = ?; configurable string clientId = ?; configurable string clientSecret = ?; gmail:Client gmail = check new gmail:Client( config = { auth: { refreshToken, clientId, clientSecret } } );
Step 3: Invoke the connector operation
Now, utilize the available connector operations.
Get unread emails in INBOX
gmail:MessageListPage messageList = check gmail->/users/me/messages(q = "label:INBOX is:unread");
Send email
gmail:MessageRequest message = { to: ["<recipient>"], subject: "Scheduled Maintenance Break Notification", bodyInHtml: string `<html> <head> <title>Scheduled Maintenance</title> </head> </html>`; }; gmail:Message sendResult = check gmail->/users/me/messages/send.post(message);
Step 4: Run the Ballerina application
bal run
Examples
The gmail
connector provides practical examples illustrating usage in various scenarios. Explore these examples, covering use cases like sending emails, retrieving messages, and managing labels.
-
Process customer feedback emails - Manage customer feedback emails by processing unread emails in the inbox, extracting details, and marking them as read.
-
Send maintenance break emails - Send emails for scheduled maintenance breaks
-
Automated Email Responses - Retrieve unread emails from the Inbox and subsequently send personalized responses.
-
Email Thread Search Search for email threads based on a specified query.
Report Issues
To report bugs, request new features, start new discussions, view project boards, etc., go to the Ballerina library parent repository.
Useful Links
- Chat live with us via our Discord server.
- Post all technical questions on Stack Overflow with the #ballerina tag.
Clients
googleapis.gmail: Client
The Gmail API lets you view and manage Gmail mailbox data like threads, messages, and labels.
Constructor
Gets invoked to initialize the connector
.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://gmail.googleapis.com/gmail/v1" - URL of the target service
get users/[string userId]/drafts
function get users/[string userId]/drafts(Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType, boolean? includeSpamTrash, int? maxResults, string? pageToken, string? q) returns ListDraftsResponse|error
Lists the drafts in the user's mailbox.
Parameters
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- includeSpamTrash boolean? (default ()) - Include drafts from
SPAM
andTRASH
in the results.
- maxResults int? (default ()) - Maximum number of drafts to return. This field defaults to 100. The maximum allowed value for this field is 500.
- pageToken string? (default ()) - Page token to retrieve a specific page of results in the list.
- q string? (default ()) - Only return draft messages matching the specified query. Supports the same query format as the Gmail search box. For example,
"from:someuser@example.com rfc822msgid: is:unread"
.
Return Type
- ListDraftsResponse|error - Successful response
post users/[string userId]/drafts
function post users/[string userId]/drafts(DraftRequest payload, Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType) returns Draft|error
Creates a new draft with the DRAFT
label.
Parameters
- payload DraftRequest - The draft to create.
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
post users/[string userId]/drafts/send
function post users/[string userId]/drafts/send(DraftRequest payload, Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType) returns Message|error
Sends the specified, existing draft to the recipients in the To
, Cc
, and Bcc
headers.
Parameters
- payload DraftRequest - The ID of the existing draft to send. (Optional) Updated draft if necessary.
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
get users/[string userId]/drafts/[string id]
function get users/[string userId]/drafts/[string id](Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType, "minimal"|"full"|"raw"|"metadata"? format) returns Draft|error
Gets the specified draft.
Parameters
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- format "minimal"|"full"|"raw"|"metadata"? (default ()) - The format to return the draft in.
put users/[string userId]/drafts/[string id]
function put users/[string userId]/drafts/[string id](DraftRequest payload, Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType) returns Draft|error
Replaces a draft's content.
Parameters
- payload DraftRequest - The updated draft to update.
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
delete users/[string userId]/drafts/[string id]
function delete users/[string userId]/drafts/[string id](Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType) returns error?
Immediately and permanently deletes the specified draft. Does not simply trash it.
Parameters
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- error? - Successful response
get users/[string userId]/history
function get users/[string userId]/history(Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType, ("messageAdded"|"messageDeleted"|"labelAdded"|"labelRemoved")[]? historyTypes, string? labelId, int? maxResults, string? pageToken, string? startHistoryId) returns ListHistoryResponse|error
Lists the history of all changes to the given mailbox. History results are returned in chronological order (increasing historyId
).
Parameters
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- historyTypes ("messageAdded"|"messageDeleted"|"labelAdded"|"labelRemoved")[]? (default ()) - History types to be returned by the function
- labelId string? (default ()) - Only return messages with a label matching the ID.
- maxResults int? (default ()) - Maximum number of history records to return. This field defaults to 100. The maximum allowed value for this field is 500.
- pageToken string? (default ()) - Page token to retrieve a specific page of results in the list.
- startHistoryId string? (default ()) - Required. Returns history records after the specified
startHistoryId
. The suppliedstartHistoryId
should be obtained from thehistoryId
of a message, thread, or previouslist
response. History IDs increase chronologically but are not contiguous with random gaps in between valid IDs. Supplying an invalid or out of datestartHistoryId
typically returns anHTTP 404
error code. AhistoryId
is typically valid for at least a week, but in some rare circumstances may be valid for only a few hours. If you receive anHTTP 404
error response, your application should perform a full sync. If you receive nonextPageToken
in the response, there are no updates to retrieve and you can store the returnedhistoryId
for a future request.
Return Type
- ListHistoryResponse|error - Successful response
get users/[string userId]/labels
function get users/[string userId]/labels(Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType) returns ListLabelsResponse|error
Lists all labels in the user's mailbox.
Parameters
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- ListLabelsResponse|error - Successful response
post users/[string userId]/labels
function post users/[string userId]/labels(Label payload, Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType) returns Label|error
Creates a new label.
Parameters
- payload Label - The label to create.
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
get users/[string userId]/labels/[string id]
function get users/[string userId]/labels/[string id](Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType) returns Label|error
Gets the specified label.
Parameters
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
put users/[string userId]/labels/[string id]
function put users/[string userId]/labels/[string id](Label payload, Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType) returns Label|error
Updates the specified label.
Parameters
- payload Label - The updated label to update.
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
delete users/[string userId]/labels/[string id]
function delete users/[string userId]/labels/[string id](Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType) returns error?
Immediately and permanently deletes the specified label and removes it from any messages and threads that it is applied to.
Parameters
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- error? - Successful response
patch users/[string userId]/labels/[string id]
function patch users/[string userId]/labels/[string id](Label payload, Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType) returns Label|error
Patch the specified label.
Parameters
- payload Label - The updated label to update.
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
get users/[string userId]/messages
function get users/[string userId]/messages(Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType, boolean? includeSpamTrash, string[]? labelIds, int? maxResults, string? pageToken, string? q) returns ListMessagesResponse|error
Lists the messages in the user's mailbox.
Parameters
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- includeSpamTrash boolean? (default ()) - Include messages from
SPAM
andTRASH
in the results.
- labelIds string[]? (default ()) - Only return messages with labels that match all of the specified label IDs. Messages in a thread might have labels that other messages in the same thread don't have. To learn more, see Manage labels on messages and threads.
- maxResults int? (default ()) - Maximum number of messages to return. This field defaults to 100. The maximum allowed value for this field is 500.
- pageToken string? (default ()) - Page token to retrieve a specific page of results in the list.
- q string? (default ()) - Only return messages matching the specified query. Supports the same query format as the Gmail search box. For example,
"from:someuser@example.com rfc822msgid: is:unread"
. Parameter cannot be used when accessing the api using the gmail.metadata scope.
Return Type
- ListMessagesResponse|error - Successful response
post users/[string userId]/messages
function post users/[string userId]/messages(MessageRequest payload, Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType, boolean? deleted, "receivedTime"|"dateHeader"? internalDateSource) returns Message|error
Directly inserts a message into only this user's mailbox similar to IMAP APPEND
, bypassing most scanning and classification. Does not send a message.
Parameters
- payload MessageRequest - The message to be inserted.
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- deleted boolean? (default ()) - Mark the email as permanently deleted (not TRASH) and only visible in Google Vault to a Vault administrator. Only used for Google Workspace accounts.
- internalDateSource "receivedTime"|"dateHeader"? (default ()) - Source for Gmail's internal date of the message.
post users/[string userId]/messages/batchDelete
function post users/[string userId]/messages/batchDelete(BatchDeleteMessagesRequest payload, Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType) returns error?
Deletes many messages by message ID. Provides no guarantees that messages were not already deleted or even existed at all.
Parameters
- payload BatchDeleteMessagesRequest - The IDs of the messages to delete.
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- error? - Successful response
post users/[string userId]/messages/batchModify
function post users/[string userId]/messages/batchModify(BatchModifyMessagesRequest payload, Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType) returns error?
Modifies the labels on the specified messages.
Parameters
- payload BatchModifyMessagesRequest - A list of labels to add/remove in messages.
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- error? - Successful response
post users/[string userId]/messages/'import
function post users/[string userId]/messages/'import(MessageRequest payload, Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType, boolean? deleted, "receivedTime"|"dateHeader"? internalDateSource, boolean? neverMarkSpam, boolean? processForCalendar) returns Message|error
Imports a message into only this user's mailbox, with standard email delivery scanning and classification similar to receiving via SMTP. This method doesn't perform SPF checks, so it might not work for some spam messages, such as those attempting to perform domain spoofing. This method does not send a message.
Parameters
- payload MessageRequest - The message to be imported.
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- deleted boolean? (default ()) - Mark the email as permanently deleted (not TRASH) and only visible in Google Vault to a Vault administrator. Only used for Google Workspace accounts.
- internalDateSource "receivedTime"|"dateHeader"? (default ()) - Source for Gmail's internal date of the message.
- neverMarkSpam boolean? (default ()) - Ignore the Gmail spam classifier decision and never mark this email as SPAM in the mailbox.
- processForCalendar boolean? (default ()) - Process calendar invites in the email and add any extracted meetings to the Google Calendar for this user.
post users/[string userId]/messages/send
function post users/[string userId]/messages/send(MessageRequest payload, Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType) returns Message|error
Sends the specified message to the recipients in the To
, Cc
, and Bcc
headers. For example usage, see Sending email.
Parameters
- payload MessageRequest - The message to be sent.
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
get users/[string userId]/messages/[string id]
function get users/[string userId]/messages/[string id](Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType, "minimal"|"full"|"raw"|"metadata"? format, string[]? metadataHeaders) returns Message|error
Gets the specified message.
Parameters
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- format "minimal"|"full"|"raw"|"metadata"? (default ()) - The format to return the message in.
- metadataHeaders string[]? (default ()) - When given and format is
METADATA
, only include headers specified.
delete users/[string userId]/messages/[string id]
function delete users/[string userId]/messages/[string id](Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType) returns error?
Immediately and permanently deletes the specified message. This operation cannot be undone. Prefer messages.trash
instead.
Parameters
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- error? - Successful response
post users/[string userId]/messages/[string id]/modify
function post users/[string userId]/messages/[string id]/modify(ModifyMessageRequest payload, Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType) returns Message|error
Modifies the labels on the specified message.
Parameters
- payload ModifyMessageRequest - A list of labels to add/remove on the message.
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
post users/[string userId]/messages/[string id]/trash
function post users/[string userId]/messages/[string id]/trash(Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType) returns Message|error
Moves the specified message to the trash.
Parameters
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
post users/[string userId]/messages/[string id]/untrash
function post users/[string userId]/messages/[string id]/untrash(Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType) returns Message|error
Removes the specified message from the trash.
Parameters
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
get users/[string userId]/messages/[string messageId]/attachments/[string id]
function get users/[string userId]/messages/[string messageId]/attachments/[string id](Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType) returns Attachment|error
Gets the specified message attachment.
Parameters
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- Attachment|error - Successful response
get users/[string userId]/profile
function get users/[string userId]/profile(Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType) returns Profile|error
Gets the current user's Gmail profile.
Parameters
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
get users/[string userId]/threads
function get users/[string userId]/threads(Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType, boolean? includeSpamTrash, string[]? labelIds, int? maxResults, string? pageToken, string? q) returns ListThreadsResponse|error
Lists the threads in the user's mailbox.
Parameters
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- includeSpamTrash boolean? (default ()) - Include threads from
SPAM
andTRASH
in the results.
- labelIds string[]? (default ()) - Only return threads with labels that match all of the specified label IDs.
- maxResults int? (default ()) - Maximum number of threads to return. This field defaults to 100. The maximum allowed value for this field is 500.
- pageToken string? (default ()) - Page token to retrieve a specific page of results in the list.
- q string? (default ()) - Only return threads matching the specified query. Supports the same query format as the Gmail search box. For example,
"from:someuser@example.com rfc822msgid: is:unread"
. Parameter cannot be used when accessing the api using the gmail.metadata scope.
Return Type
- ListThreadsResponse|error - Successful response
get users/[string userId]/threads/[string id]
function get users/[string userId]/threads/[string id](Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType, "full"|"metadata"|"minimal"? format, string[]? metadataHeaders) returns MailThread|error
Gets the specified thread.
Parameters
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
- format "full"|"metadata"|"minimal"? (default ()) - The format to return the messages in.
- metadataHeaders string[]? (default ()) - When given and format is METADATA, only include headers specified.
Return Type
- MailThread|error - Successful response
delete users/[string userId]/threads/[string id]
function delete users/[string userId]/threads/[string id](Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType) returns error?
Immediately and permanently deletes the specified thread. Any messages that belong to the thread are also deleted. This operation cannot be undone. Prefer threads.trash
instead.
Parameters
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- error? - Successful response
post users/[string userId]/threads/[string id]/modify
function post users/[string userId]/threads/[string id]/modify(ModifyThreadRequest payload, Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType) returns MailThread|error
Modifies the labels applied to the thread. This applies to all messages in the thread.
Parameters
- payload ModifyThreadRequest - A list labels to add/remove on the thread.
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- MailThread|error - Successful response
post users/[string userId]/threads/[string id]/trash
function post users/[string userId]/threads/[string id]/trash(Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType) returns MailThread|error
Moves the specified thread to the trash. Any messages that belong to the thread are also moved to the trash.
Parameters
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- MailThread|error - Successful response
post users/[string userId]/threads/[string id]/untrash
function post users/[string userId]/threads/[string id]/untrash(Xgafv? xgafv, string? access_token, Alt? alt, string? callback, string? fields, string? 'key, string? oauth_token, boolean? prettyPrint, string? quotaUser, string? upload_protocol, string? uploadType) returns MailThread|error
Removes the specified thread from the trash. Any messages that belong to the thread are also removed from the trash.
Parameters
- xgafv Xgafv? (default ()) - V1 error format.
- access_token string? (default ()) - OAuth access token.
- alt Alt? (default ()) - Data format for response.
- callback string? (default ()) - JSONP
- fields string? (default ()) - Selector specifying which fields to include in a partial response.
- 'key string? (default ()) - API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.
- oauth_token string? (default ()) - OAuth 2.0 token for the current user.
- prettyPrint boolean? (default ()) - Returns response with indentations and line breaks.
- quotaUser string? (default ()) - Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.
- upload_protocol string? (default ()) - Upload protocol for media (e.g. "raw", "multipart").
- uploadType string? (default ()) - Legacy upload protocol for media (e.g. "media", "multipart").
Return Type
- MailThread|error - Successful response
Constants
Records
googleapis.gmail: Attachment
An Attachment.
Fields
- attachmentId? string - Id of the attachment.
- data? string - The body data of a MIME message part.
- size? Signed32 - Number of bytes for the message part data (encoding notwithstanding).
googleapis.gmail: AttachmentFile
A file record to indicate attachment
Fields
- mimeType string - The mime type of the file (ex. application/pdf, text/plain)
- name string - The file name with extension. This will be used name the attachment/image in the mail.
- path string - The file path
googleapis.gmail: BatchDeleteMessagesRequest
Fields
- ids? string[] - The IDs of the messages to delete.
googleapis.gmail: BatchModifyMessagesRequest
Fields
- addLabelIds? string[] - A list of label IDs to add to messages.
- ids? string[] - The IDs of the messages to modify. There is a limit of 1000 ids per request.
- removeLabelIds? string[] - A list of label IDs to remove from messages.
googleapis.gmail: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy? ProxyConfig - Proxy server related options
googleapis.gmail: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings? ClientHttp1Settings - Configurations related to HTTP/1.x protocol
- http2Settings? ClientHttp2Settings - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig? PoolConfiguration - Configurations associated with request pooling
- cache? CacheConfig - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker? CircuitBreakerConfig - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig? RetryConfig - Configurations associated with retrying
- responseLimits? ResponseLimitConfigs - Configurations associated with inbound response size limits
- secureSocket? ClientSecureSocket - SSL/TLS-related options
- proxy? ProxyConfig - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
googleapis.gmail: Draft
A draft email in the user's mailbox.
Fields
- id? string - The immutable ID of the draft.
- message? Message - An email message.
googleapis.gmail: DraftRequest
Request payload to create a draft.
Fields
- id? string - The immutable ID of the draft.
- message? MessageRequest - An email message.
googleapis.gmail: History
A record of a change to the user's mailbox. Each history change may affect multiple messages in multiple ways.
Fields
- id? string - The mailbox sequence ID.
- labelsAdded? HistoryLabelAdded[] - Labels added to messages in this history record.
- labelsRemoved? HistoryLabelRemoved[] - Labels removed from messages in this history record.
- messages? Message[] - List of messages changed in this history record. The fields for specific change types, such as
messagesAdded
may duplicate messages in this field. We recommend using the specific change-type fields instead of this.
- messagesAdded? HistoryMessageAdded[] - Messages added to the mailbox in this history record.
- messagesDeleted? HistoryMessageDeleted[] - Messages deleted (not Trashed) from the mailbox in this history record.
googleapis.gmail: HistoryLabelAdded
Fields
- labelIds? string[] - Label IDs added to the message.
- message? Message - An email message.
googleapis.gmail: HistoryLabelRemoved
Fields
- labelIds? string[] - Label IDs removed from the message.
- message? Message - An email message.
googleapis.gmail: HistoryMessageAdded
Fields
- message? Message - An email message.
googleapis.gmail: HistoryMessageDeleted
Fields
- message? Message - An email message.
googleapis.gmail: ImageFile
A file record to indicate inline image
Fields
- Fields Included from *AttachmentFile
- contentId string - The content id of the image. This will be used to refer the image in the mail body.
googleapis.gmail: Label
Labels are used to categorize messages and threads within the user's mailbox. The maximum number of labels supported for a user's mailbox is 10,000.
Fields
- color? LabelColor - The color to assign to the label. Color is only available for labels that have their
type
set touser
.
- id? string - The immutable ID of the label.
- labelListVisibility? "labelShow"|"labelShowIfUnread"|"labelHide" - The visibility of the label in the label list in the Gmail web interface.
- messageListVisibility? "show"|"hide" - The visibility of messages with this label in the message list in the Gmail web interface.
- messagesTotal? Signed32 - The total number of messages with the label.
- messagesUnread? Signed32 - The number of unread messages with the label.
- name? string - The display name of the label.
- threadsTotal? Signed32 - The total number of threads with the label.
- threadsUnread? Signed32 - The number of unread threads with the label.
- 'type? "system"|"user" - The owner type for the label. User labels are created by the user and can be modified and deleted by the user and can be applied to any message or thread. System labels are internally created and cannot be added, modified, or deleted. System labels may be able to be applied to or removed from messages and threads under some circumstances but this is not guaranteed. For example, users can apply and remove the
INBOX
andUNREAD
labels from messages and threads, but cannot apply or remove theDRAFTS
orSENT
labels from messages or threads.
googleapis.gmail: LabelColor
The color to assign to the label. Color is only available for labels that have their type
set to user
.
Fields
- backgroundColor? string - The background color represented as hex string #RRGGBB (ex #000000). This field is required in order to set the color of a label. Only the following predefined set of color values are allowed: #000000, #434343, #666666, #999999, #cccccc, #efefef, #f3f3f3, #ffffff, #fb4c2f, #ffad47, #fad165, #16a766, #43d692, #4a86e8, #a479e2, #f691b3, #f6c5be, #ffe6c7, #fef1d1, #b9e4d0, #c6f3de, #c9daf8, #e4d7f5, #fcdee8, #efa093, #ffd6a2, #fce8b3, #89d3b2, #a0eac9, #a4c2f4, #d0bcf1, #fbc8d9, #e66550, #ffbc6b, #fcda83, #44b984, #68dfa9, #6d9eeb, #b694e8, #f7a7c0, #cc3a21, #eaa041, #f2c960, #149e60, #3dc789, #3c78d8, #8e63ce, #e07798, #ac2b16, #cf8933, #d5ae49, #0b804b, #2a9c68, #285bac, #653e9b, #b65775, #822111, #a46a21, #aa8831, #076239, #1a764d, #1c4587, #41236d, #83334c #464646, #e7e7e7, #0d3472, #b6cff5, #0d3b44, #98d7e4, #3d188e, #e3d7ff, #711a36, #fbd3e0, #8a1c0a, #f2b2a8, #7a2e0b, #ffc8af, #7a4706, #ffdeb5, #594c05, #fbe983, #684e07, #fdedc1, #0b4f30, #b3efd3, #04502e, #a2dcc1, #c2c2c2, #4986e7, #2da2bb, #b99aff, #994a64, #f691b2, #ff7537, #ffad46, #662e37, #ebdbde, #cca6ac, #094228, #42d692, #16a765
- textColor? string - The text color of the label, represented as hex string. This field is required in order to set the color of a label. Only the following predefined set of color values are allowed: #000000, #434343, #666666, #999999, #cccccc, #efefef, #f3f3f3, #ffffff, #fb4c2f, #ffad47, #fad165, #16a766, #43d692, #4a86e8, #a479e2, #f691b3, #f6c5be, #ffe6c7, #fef1d1, #b9e4d0, #c6f3de, #c9daf8, #e4d7f5, #fcdee8, #efa093, #ffd6a2, #fce8b3, #89d3b2, #a0eac9, #a4c2f4, #d0bcf1, #fbc8d9, #e66550, #ffbc6b, #fcda83, #44b984, #68dfa9, #6d9eeb, #b694e8, #f7a7c0, #cc3a21, #eaa041, #f2c960, #149e60, #3dc789, #3c78d8, #8e63ce, #e07798, #ac2b16, #cf8933, #d5ae49, #0b804b, #2a9c68, #285bac, #653e9b, #b65775, #822111, #a46a21, #aa8831, #076239, #1a764d, #1c4587, #41236d, #83334c #464646, #e7e7e7, #0d3472, #b6cff5, #0d3b44, #98d7e4, #3d188e, #e3d7ff, #711a36, #fbd3e0, #8a1c0a, #f2b2a8, #7a2e0b, #ffc8af, #7a4706, #ffdeb5, #594c05, #fbe983, #684e07, #fdedc1, #0b4f30, #b3efd3, #04502e, #a2dcc1, #c2c2c2, #4986e7, #2da2bb, #b99aff, #994a64, #f691b2, #ff7537, #ffad46, #662e37, #ebdbde, #cca6ac, #094228, #42d692, #16a765
googleapis.gmail: ListDraftsResponse
Fields
- drafts? Draft[] - List of drafts. Note that the
Message
property in eachDraft
resource only contains anid
and athreadId
. The messages.get method can fetch additional message details.
- nextPageToken? string - Token to retrieve the next page of results in the list.
- resultSizeEstimate? int - Estimated total number of results.
googleapis.gmail: ListHistoryResponse
Fields
- history? History[] - List of history records. Any
messages
contained in the response will typically only haveid
andthreadId
fields populated.
- historyId? string - The ID of the mailbox's current history record.
- nextPageToken? string - Page token to retrieve the next page of results in the list.
googleapis.gmail: ListLabelsResponse
Fields
- labels? Label[] - List of labels. Note that each label resource only contains an
id
,name
,messageListVisibility
,labelListVisibility
, andtype
. The labels.get method can fetch additional label details.
googleapis.gmail: ListMessagesResponse
List of messages.
Fields
- messages? Message[] - List of messages. Note that each message resource contains only an
id
and athreadId
. Additional message details can be fetched using the messages.get method.
- nextPageToken? string - Token to retrieve the next page of results in the list.
- resultSizeEstimate? int - Estimated total number of results.
googleapis.gmail: ListThreadsResponse
Fields
- nextPageToken? string - Page token to retrieve the next page of results in the list.
- resultSizeEstimate? int - Estimated total number of results.
- threads? MailThread[] - List of threads. Note that each thread resource does not contain a list of
messages
. The list ofmessages
for a given thread can be fetched using the threads.get method.
googleapis.gmail: MailThread
A collection of messages representing a conversation.
Fields
- historyId? string - The ID of the last history record that modified this thread.
- id? string - The unique ID of the thread.
- messages? Message[] - The list of messages in the thread.
- snippet? string - A short part of the message text.
googleapis.gmail: MailThreadRequest
Request payload used to create a collection of messages representing a conversation.
Fields
- id? string - The unique ID of the thread.
- messages? Message[] - The list of messages in the thread.
googleapis.gmail: Message
An email message.
Fields
- threadId string - The ID of the thread the message belongs to.
- id string - The immutable ID of the message.
- labelIds? string[] - List of IDs of labels applied to this message.
- raw? string - The entire email message in an RFC 2822 formatted. Returned in
messages.get
anddrafts.get
responses when theformat=RAW
parameter is supplied.
- snippet? string - A short part of the message text.
- historyId? string - The ID of the last history record that modified this message.
- internalDate? string - The internal message creation timestamp (epoch ms), which determines ordering in the inbox. For normal SMTP-received email, this represents the time the message was originally accepted by Google, which is more reliable than the
Date
header. However, for API-migrated mail, it can be configured by client to be based on theDate
header.
- sizeEstimate? Signed32 - Estimated size in bytes of the message.
- to? string[] - Email header To
- 'from? string - Email header From
- bcc? string[] - Email header Bcc
- cc? string[] - Email header Cc
- subject? string - Email header Subject
- date? string - Email header Date
- messageId? string - Email header Message-ID
- contentType? string - Email header ContentType
- mimeType? string - MIME type of the top level message part. Values in
multipart/alternative
such astext/plain
andtext/html
and inmultipart/*
includingmultipart/mixed
andmultipart/related
indicate that the message contains a structured body with MIME parts. Values inmessage/rfc822
indicate that the message is a container for the message parts that follow after the header.
- payload? MessagePart - Body of the message.
googleapis.gmail: MessagePart
A single MIME message part.
Fields
- filename? string - The filename of the attachment. Only present if this message part represents an attachment.
- mimeType? string - The MIME type of the message part.
- partId string - The immutable ID of the message part.
- attachmentId? string - When present, contains the ID of an external attachment that can be retrieved in a separate
messages.attachments.get
request. When not present, the entire content of the message part body is contained in the data field.
- data? string - The body data of a MIME message part. May be empty for MIME container types that have no message body or when the body data is sent as a separate attachment. An attachment ID is present if the body data is contained in a separate attachment.
- size? Signed32 - Number of bytes for the message part data.
- parts? MessagePart[] - The child MIME message parts of this part. This only applies to container MIME message parts, for example
multipart/*
. For non- container MIME message part types, such astext/plain
, this field is empty. For more information, see RFC 1521.
googleapis.gmail: MessageRequest
Message Send Request-Payload (Charset UTF-8 will be used to encode the message body).
Fields
- to? string[] - The recipients of the mail
- 'from? string - The sender of the mail
- subject? string - The subject of the mail
- cc? string[] - The cc recipients of the mail.
- bcc? string[] - The bcc recipients of the mail.
- bodyInText? string - Message body of content type
text/plain
.
- bodyInHtml? string - Message body of content type
text/html
.
- inlineImages? ImageFile[] - The file array consisting the inline images.
- attachments? AttachmentFile[] - The file array consisting the attachments.
- threadId? string - ID of the thread the message must be replied to.
- initialMessageId? string - Message-ID header of the message being replied to.
- references? string[] - List of Message-ID headers identifying ancestors of the message being replied to.
googleapis.gmail: ModifyMessageRequest
Fields
- addLabelIds? string[] - A list of IDs of labels to add to this message. You can add up to 100 labels with each update.
- removeLabelIds? string[] - A list IDs of labels to remove from this message. You can remove up to 100 labels with each update.
googleapis.gmail: ModifyThreadRequest
Fields
- addLabelIds? string[] - A list of IDs of labels to add to this thread. You can add up to 100 labels with each update.
- removeLabelIds? string[] - A list of IDs of labels to remove from this thread. You can remove up to 100 labels with each update.
googleapis.gmail: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://accounts.google.com/o/oauth2/token") - Refresh URL
googleapis.gmail: Profile
Profile for a Gmail user.
Fields
- emailAddress? string - The user's email address.
- historyId? string - The ID of the mailbox's current history record.
- messagesTotal? Signed32 - The total number of messages in the mailbox.
- threadsTotal? Signed32 - The total number of threads in the mailbox.
googleapis.gmail: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
Errors
googleapis.gmail: Error
Defines the generic error type for the gmail
module.
googleapis.gmail: FileGenericError
Error that occurs when there is an issue with inline images or attachments. This could be due to issues like file not found, unsupported file type, etc.
googleapis.gmail: ValueEncodeError
Error that occurs when an invalid encoded value is provided for the data
fields.
Union types
googleapis.gmail: Alt
Alt
Data format for response.
googleapis.gmail: Xgafv
Xgafv
V1 error format.
Import
import ballerinax/googleapis.gmail;
Metadata
Released date: 2 months ago
Version: 4.1.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.11.0
GraalVM compatible: Yes
Pull count
Total: 11874
Current verison: 199
Weekly downloads
Keywords
Communication/Email
Cost/Free
Vendor/Google
Contributors