googleapis.gmail
Module googleapis.gmail
API
Declarations
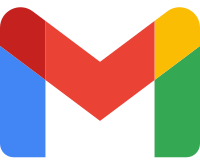
ballerinax/googleapis.gmail Ballerina library
Overview
Ballerina Gmail Connector provides the capability to send, read, and delete emails through the Gmail REST API. It also provides the ability to read, trash, untrash, and delete threads, as well as the ability to get the Gmail profile and mailbox history, etc. The connector supports OAuth 2.0 authentication.
This module supports Gmail API v1.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
-
Create a Google account. (If you already have one, you can use that.)
-
Obtain tokens
- Follow this guide
Quickstart
To use the Gmail connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
Import the ballerinax/googleapis.gmail
module into the Ballerina project.
import ballerinax/googleapis.gmail;
Step 2: Create a new connector instance
Create a gmail:ConnectionConfig
with the OAuth 2.0 tokens obtained, and initialize the connector with it.
gmail:ConnectionConfig gmailConfig = { auth: { refreshUrl: gmail:REFRESH_URL, refreshToken: <REFRESH_TOKEN>, clientId: <CLIENT_ID>, clientSecret: <CLIENT_SECRET> } }; gmail:Client gmailClient = check new (gmailConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to send an email using the connector.public function main() returns error? { string userId = "me"; gmail:MessageRequest messageRequest = { recipient : "aa@gmail.com", cc : "cc@gmail.com", subject : "Email-Subject", messageBody : "Email Message Body Text", // Set the content type of the mail as TEXT_PLAIN or TEXT_HTML. contentType : gmail:TEXT_PLAIN }; gmail:Message sendMessageResponse = check gmailClient->sendMessage(messageRequest, userId = userId); }
-
Use
bal run
command to compile and run the Ballerina program.
Functions
appendEncodedURIParameter
function appendEncodedURIParameter(string requestPath, string key, string value) returns string|error
Append given key and value as URI query parameter.
convertJSONToMessageType
function convertJSONToMessageType(json sourceMessageJsonObject) returns Message
Transforms JSON message object into Message Type Object.
Parameters
- sourceMessageJsonObject json -
json
message object
Return Type
- Message - Returns Message type object
convertJSONToThreadType
function convertJSONToThreadType(json sourceThreadJsonObject) returns MailThread
Transforms mail thread JSON object into MailThread type.
Parameters
- sourceThreadJsonObject json -
json
message thread object.
Return Type
- MailThread - Returns MailThread type
Classes
googleapis.gmail: MailboxHistoryStream
next
Clients
googleapis.gmail: Client
Ballerina Gmail connector provides the capability to access Gmail API. The connector let you to interact with users' Gmail inboxes through the Gmail REST API.
Constructor
Initializes the connector. During initialization you can pass either http:BearerTokenConfig if you have a bearer token or http:OAuth2RefreshTokenGrantConfig if you have Oauth tokens. Create a Google account and obtain tokens following this guide.
init (ConnectionConfig config)
- config ConnectionConfig - Configurations required to initialize the
Client
endpoint
listMessages
function listMessages(MsgSearchFilter? filter, string? userId) returns stream<Message, error?>|error
Lists the messages in user's mailbox.
Parameters
- filter MsgSearchFilter? (default ()) - Optional. MsgSearchFilter with optional query parameters to search messages.
- userId string? (default ()) - The user's email address. The special value me can be used to indicate the authenticated user.
Return Type
sendMessage
function sendMessage(MessageRequest message, string? threadId, string? userId) returns Message|error
Creates the raw base 64 encoded string of the whole message and send it as an email from the user's mailbox to its recipient.
Parameters
- message MessageRequest - MessageRequest to send. Note: Here if any attachments included in the message, those attachments need to be less than 25MB.
- threadId string? (default ()) - Optional. Required if message is expected to be send The ID of the thread the message belongs to. (The Subject headers must match)
- userId string? (default ()) - The user's email address. The special value me can be used to indicate the authenticated user.
Return Type
readMessage
function readMessage(string messageId, string? format, string[]? metadataHeaders, string? userId) returns Message|error
Reads the specified mail from users mailbox.
Parameters
- messageId string - The ID of the message to retrieve
- format string? (default ()) - Optional. The format to return the message in.
Acceptable values for format for a get message request are defined as following constants
in the module:
FORMAT_FULL
: Returns the full email message data with body content parsed in the payload field;the raw field is not used. (default)FORMAT_METADATA
: Returns only email message ID, labels, and email headers.FORMAT_MINIMAL
: Returns only email message ID and labels; does not return the email headers, body, or payload.FORMAT_RAW
: Returns the full email message data with body content in the raw field as a base64url encoded string. (the payload field is not included in the response)
- metadataHeaders string[]? (default ()) - Optional. The meta data headers array to include in the response when the format is given as FORMAT_METADATA.
- userId string? (default ()) - The user's email address. The special value me can be used to indicate the authenticated user.
Return Type
getAttachment
function getAttachment(string messageId, string attachmentId, string? userId) returns MessageBodyPart|error
Gets the specified message attachment from users mailbox.
Parameters
- messageId string - The ID of the message to retrieve
- attachmentId string - The ID of the attachment to retrieve
- userId string? (default ()) - The user's email address. The special value me can be used to indicate the authenticated user.
Return Type
- MessageBodyPart|error - If successful, returns MessageBodyPart type object of the specified attachment. Else returns error.
trashMessage
Moves the specified message to the trash.
Parameters
- messageId string - The ID of the message to trash
- userId string? (default ()) - The user's email address. The special value me can be used to indicate the authenticated user.
untrashMessage
Removes the specified message from the trash.
Parameters
- messageId string - The ID of the message to untrash
- userId string? (default ()) - The user's email address. The special value me can be used to indicate the authenticated user.
deleteMessage
Immediately and permanently deletes the specified message. This operation cannot be undone.
Parameters
- messageId string - The ID of the message to delete
- userId string? (default ()) - The user's email address. The special value me can be used to indicate the authenticated user.
Return Type
- error? - If successful, returns nothing. Else returns error.
modifyMessage
function modifyMessage(string messageId, string[] addLabelIds, string[] removeLabelIds, string? userId) returns Message|error
Modifies the labels on the specified message.
Parameters
- messageId string - The ID of the message to modify
- addLabelIds string[] - A list of Ids of labels to add to this message
- removeLabelIds string[] - A list Ids of labels to remove from this message
- userId string? (default ()) - The user's email address. The special value me can be used to indicate the authenticated user.
Return Type
listThreads
function listThreads(MsgSearchFilter? filter, string? userId) returns stream<MailThread, error?>|error
Lists the threads in user's mailbox.
Parameters
- filter MsgSearchFilter? (default ()) - Optional. The MsgSearchFilter with optional query parameters to search a thread.
- userId string? (default ()) - The user's email address. The special value me can be used to indicate the authenticated user.
Return Type
- stream<MailThread, error?>|error - If successful, returns stream<MailThread,error?>. Else returns error.
readThread
function readThread(string threadId, string? format, string[]? metadataHeaders, string? userId) returns MailThread|error
Reads the specified mail thread from users mailbox.
Parameters
- threadId string - The ID of the thread to retrieve
- format string? (default ()) - Optional. The format to return the messages in.
Acceptable values for format for a get thread request are defined as following constants
in the module:
FORMAT_FULL
: Returns the full email message data with body content parsed in the payload field;the raw field is not used. (default)FORMAT_METADATA
: Returns only email message ID, labels, and email headers.FORMAT_MINIMAL
: Returns only email message ID and labels; does not return the email headers, body, or payload.FORMAT_RAW
: Returns the full email message data with body content in the raw field as a base64url encoded string. (the payload field is not included in the response)
- metadataHeaders string[]? (default ()) - Optional. The meta data headers array to include in the reponse when the format is given
as
FORMAT_METADATA
.
- userId string? (default ()) - The user's email address. The special value me can be used to indicate the authenticated user.
Return Type
- MailThread|error - If successful, returns MailThread type of the specified mail thread. Else returns error.
trashThread
function trashThread(string threadId, string? userId) returns MailThread|error
Moves the specified mail thread to the trash.
Parameters
- threadId string - The ID of the thread to trash
- userId string? (default ()) - The user's email address. The special value me can be used to indicate the authenticated user.
Return Type
- MailThread|error - If successful, returns trashed MailThread record. Else returns error.
untrashThread
function untrashThread(string threadId, string? userId) returns MailThread|error
Removes the specified mail thread from the trash.
Parameters
- threadId string - The ID of the thread to untrash
- userId string? (default ()) - The user's email address. The special value me can be used to indicate the authenticated user.
Return Type
- MailThread|error - If successful, returns boolean status of untrashing. Else returns error.
deleteThread
Immediately and permanently deletes the specified mail thread. This operation cannot be undone.
Parameters
- threadId string - The ID of the thread to delete
- userId string? (default ()) - The user's email address. The special value me can be used to indicate the authenticated user.
Return Type
- error? - If successful, returns nothing. Else returns error.
modifyThread
function modifyThread(string threadId, string[] addLabelIds, string[] removeLabelIds, string? userId) returns MailThread|error
Modifies the labels on the specified thread.
Parameters
- threadId string - The ID of the thread to modify
- addLabelIds string[] - A list of IDs of labels to add to this thread
- removeLabelIds string[] - A list IDs of labels to remove from this thread
- userId string? (default ()) - The user's email address. The special value me can be used to indicate the authenticated user.
Return Type
- MailThread|error - If successful, returns modified MailThread type object. Else returns error.
getUserProfile
function getUserProfile(string? userId) returns UserProfile|error
Gets the current user's Gmail Profile.
Parameters
- userId string? (default ()) - The user's email address. The special value me can be used to indicate the authenticated user.
Return Type
- UserProfile|error - If successful, returns UserProfile type. Else returns error.
getLabel
Gets the label.
Parameters
- labelId string - The label ID
- userId string? (default ()) - The user's email address. The special value me can be used to indicate the authenticated user.
createLabel
function createLabel(string name, string labelListVisibility, string messageListVisibility, string? backgroundColor, string? textColor, string? userId) returns Label|error
Creates a new label.
Parameters
- name string - The display name of the label
- labelListVisibility string - The visibility of the label in the label list in the Gmail web interface.
Acceptable values are:
labelHide
: Do not show the label in the label list.labelShow
: Show the label in the label list.labelShowIfUnread
: Show the label if there are any unread messages with that label.
- messageListVisibility string - The visibility of messages with this label in the message list in the Gmail web
interface. Acceptable values are:
hide
: Do not show the label in the message list.show
: Show the label in the message list. (Default)
- backgroundColor string? (default ()) - Optional. The background color represented as hex string #RRGGBB (ex #000000). This field is required in order to set the color of a label.
- textColor string? (default ()) - Optional. The text color of the label, represented as hex string. This field is required in order to set the color of a label.
- userId string? (default ()) - The user's email address. The special value me can be used to indicate the authenticated user.
listLabels
Lists all labels in the user's mailbox.
Parameters
- userId string? (default ()) - The user's email address. The special value me can be used to indicate the authenticated user.
Return Type
deleteLabel
Deletes a label.
Parameters
- labelId string - The ID of the label to delete
- userId string? (default ()) - The user's email address. The special value me can be used to indicate the authenticated user.
Return Type
- error? - If successful, returns nothing. Else returns error.
updateLabel
function updateLabel(string labelId, string? name, string? messageListVisibility, string? labelListVisibility, string? backgroundColor, string? textColor, string? userId) returns Label|error
Updates a label.
Parameters
- labelId string - The ID of the label to update
- name string? (default ()) - Optional. The display name of the label
- messageListVisibility string? (default ()) - Optional. The visibility of messages with this label in the message list in the Gmail
web interface.
Acceptable values are:
hide
: Do not show the label in the message listshow
: Show the label in the message list
- labelListVisibility string? (default ()) - Optional. The visibility of the label in the label list in the Gmail web interface.
Acceptable values are:
labelHide
: Do not show the label in the label listlabelShow
: Show the label in the label listlabelShowIfUnread
: Show the label if there are any unread messages with that label
- backgroundColor string? (default ()) - Optional. The background color represented as hex string #RRGGBB (ex #000000).
- textColor string? (default ()) - Optional. The text color of the label, represented as hex string.
- userId string? (default ()) - The user's email address. The special value me can be used to indicate the authenticated user.
listHistory
function listHistory(string startHistoryId, string[]? historyTypes, string? labelId, string? maxResults, string? pageToken, string? userId) returns stream<History, error?>|error
Lists the history of all changes to the given mailbox. History results are returned in chronological order (increasing historyId).
Parameters
- startHistoryId string - Returns history records after the specified startHistoryId
- historyTypes string[]? (default ()) - Optional. Array of history types to be returned by the function.
Acceptable values are:
labelAdded
labelRemoved
messageAdded
messageDeleted
- labelId string? (default ()) - Optional. Only return messages with a label matching the ID
- maxResults string? (default ()) - Optional. The maximum number of history records to return
- pageToken string? (default ()) - Optional. Page token to retrieve a specific page of results in the list
- userId string? (default ()) - The user's email address. The special value me can be used to indicate the authenticated user.
Return Type
listDrafts
Lists the drafts in user's mailbox.
Parameters
- filter DraftSearchFilter? (default ()) - Optional. DraftSearchFilter with optional query parameters to search drafts.
- userId string? (default ()) - The user's email address. The special value me can be used to indicate the authenticated user.
Return Type
readDraft
Reads the specified draft from users mailbox.
Parameters
- draftId string - The ID of the draft to retrieve
- format string? (default ()) - Optional. The format to return the draft in.
Acceptable values for format for a get draft request are defined as following constants
in the module:
FORMAT_FULL
: Returns the full email message data with body content parsed in the payload field;the raw field is not used. (default)FORMAT_METADATA
: Returns only email message ID, labels, and email headers.FORMAT_MINIMAL
: Returns only email message ID and labels; does not return the email headers, body, or payload.FORMAT_RAW
: Returns the full email message data with body content in the raw field as a base64url encoded string. (the payload field is not included in the response)
- userId string? (default ()) - The user's email address. The special value me can be used to indicate the authenticated user.
Return Type
deleteDraft
Immediately and permanently deletes the specified draft.
Parameters
- draftId string - The ID of the draft to delete
- userId string? (default ()) - The user's email address. The special value me can be used to indicate the authenticated user.
Return Type
- error? - If successful, returns nothing. Else returns error.
createDraft
function createDraft(MessageRequest message, string? threadId, string? userId) returns string|error
Creates a new draft with the DRAFT label.
Parameters
- message MessageRequest - MessageRequest to create a draft
- threadId string? (default ()) - Optional. Thread ID of the draft to reply
- userId string? (default ()) - The user's email address. The special value me can be used to indicate the authenticated user.
Return Type
updateDraft
function updateDraft(string draftId, MessageRequest message, string? threadId, string? userId) returns string|error
Replaces a draft's content.
Parameters
- draftId string - The draft ID to update
- message MessageRequest - MessageRequest to update a draft
- threadId string? (default ()) - Optional. Thread ID of the draft to reply
- userId string? (default ()) - The user's email address. The special value me can be used to indicate the authenticated user.
Return Type
sendDraft
Sends the specified, existing draft to the recipients in the To, Cc, and Bcc headers.
Constants
googleapis.gmail: BASE_URL
Holds the value for URL of gmail.
googleapis.gmail: FORMAT
Holds the value for optional parameter name 'format'.
googleapis.gmail: FORMAT_FULL
Holds string for Gmail message/thread response format full.
googleapis.gmail: FORMAT_METADATA
Holds string for Gmail message/thread response format metadata.
googleapis.gmail: FORMAT_MINIMAL
Holds string for Gmail message/thread response format minimal.
googleapis.gmail: FORMAT_RAW
Holds string for Gmail message/thread response format raw.
googleapis.gmail: MESSAGE_RESOURCE
Holds the value for messages resource path.
googleapis.gmail: METADATA_HEADERS
Holds the value for optional parameter name 'metadataHeaders'.
googleapis.gmail: REFRESH_URL
Holds the value for URL of refresh token end point.
googleapis.gmail: TEXT_HTML
Holds value for message type text/html.
googleapis.gmail: TEXT_PLAIN
Holds value for message type text/plain.
googleapis.gmail: THREAD_RESOURCE
Holds the value for threads resoure path.
Records
googleapis.gmail: AttachmentPath
Represents an attachment file path and mime type of an attachment in a message request.
Fields
- attachmentPath string - The file path of the attachment
- mimeType string - The mime type of the attachment
For ex: If you are sending a pdf document, give the mime type as
application/pdf
. If you are sending a text file, give the mime type astext/plain
.
googleapis.gmail: Color
Represents the color of label.
Fields
- textColor string - The text color of the label, represented as hex string
- backgroundColor string - The background color represented as hex string
googleapis.gmail: ConnectionConfig
Client configuration details.
Fields
- Fields Included from *ConnectionConfig
- auth AuthConfig
- httpVersion HttpVersion
- http1Settings ClientHttp1Settings
- http2Settings ClientHttp2Settings
- timeout decimal
- forwarded string
- poolConfig PoolConfiguration
- cache CacheConfig
- compression Compression
- circuitBreaker CircuitBreakerConfig
- retryConfig RetryConfig
- responseLimits ResponseLimitConfigs
- secureSocket ClientSecureSocket
- proxy ProxyConfig
- validation boolean
- anydata...
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
googleapis.gmail: Draft
Represents a draft email in user's mailbox.
Fields
- id string - The immutable ID of the draft
- message Message? - The message content of the draft
googleapis.gmail: DraftListPage
Represents a page of the drafts list received as response for list drafts api call.
Fields
- drafts Draft[]? - Array of draft maps with draftId, messageId and threadId as keys
- resultSizeEstimate int - Estimated size of the whole list
- nextPageToken string? - Token for next page of mail drafts list
googleapis.gmail: DraftSearchFilter
Represents the optional search drafts filter fields.
Fields
- includeSpamTrash boolean? - Specifies whether to include drafts from SPAM and TRASH in the results
- maxResults int? - Maximum number of drafts to return in the page for a single request
- pageToken string? - Page token to retrieve a specific page of results in the list
- q string? - Query for searching . Only returns drafts matching the specified query. Supports the same query format as the Gmail search box.
googleapis.gmail: History
Represents a history reoced in MailboxHistoryPage.
Fields
- historyId string? - The ID of the mailbox's current history record
- id string? - The mailbox sequence ID
- messages Message[]? - List of messages changed in this history record
- messagesAdded HistoryEvent[]? - Messages added to the mailbox in this history record
- messagesDeleted HistoryEvent[]? - Messages deleted (not Trashed) from the mailbox in this history record
- labelsAdded HistoryEvent[]? - Array of maps of Labels added to messages in this history record
- labelsRemoved HistoryEvent[]? - Array of maps of Labels removed from messages in this history record
googleapis.gmail: HistoryEvent
Represents changes of messages in history record.
Fields
- message Message - The message changed
- labelIds string[]? - The label ids of the message
googleapis.gmail: InlineImagePath
Represents image file path and mime type of an inline image in a message request.
Fields
- imagePath string - The file path of the image
- mimeType string - The mime type of the image. The primary type should be
image
. For ex: If you are sending a jpg image, give the mime type asimage/jpeg
. If you are sending a png image, give the mime type asimage/png
.
googleapis.gmail: Label
Represents a Label which is used to categorize messaages and threads within the user's mailbox.
Fields
- id string - The immutable ID of the label
- name string - The display name of the label
- messageListVisibility string? - The visibility of messages with this label in the message list in the Gmail web interface. Acceptable values are: hide: Do not show the label in the message list. show: Show the label in the message list (Default)
- labelListVisibility string? - The visibility of the label in the label list in the Gmail web interface. Acceptable values are: labelHide: Do not show the label in the label list labelShow: Show the label in the label list (Default) labelShowIfUnread: Show the label if there are any unread messages with that label
- 'type string? -
- messagesTotal int? - The total number of messages with the label
- messagesUnread int? - The number of unread messages with the label
- threadsTotal int? - The total number of threads with the label
- threadsUnread int? - The number of unread threads with the label
- color Color? - Reperesents the color of label
googleapis.gmail: LabelList
Represents the list of labels.
Fields
- labels Label[] - The array of labels
googleapis.gmail: MailboxHistoryPage
Represents a page of the history list received as response for list history api call.
Fields
- history History[]? - List of history records. Any messages contained in the response will typically only have ID and threadId fields populated.
- nextPageToken string? - Page token to retrieve the next page of results in the list
- historyId string - The ID of the mailbox's current history record
googleapis.gmail: MailThread
Represents mail thread resource.
Fields
- id string - The unique ID of the thread
- historyId string? - The ID of the last history record that modified this thread
- snippet string? - A short part of the message text
- messages Message[]? - The list of messages in the thread
googleapis.gmail: Message
Represents message resource which will be received as a response from the Gmail API.
Fields
- threadId string - Thread ID which the message belongs to
- id string - Message ID
- labelIds string[]? - The label ids of the message
- raw string? - Represent the entire message in base64 encoded string
- snippet string? - Short part of the message text
- historyId string? - The ID of the last history record that modified the message
- internalDate string? - The internal message creation timestamp(epoch ms)
- sizeEstimate string? - Estimated size of the message in bytes
- headerTo string? - Email header To
- headerFrom string? - Email header From
- headerBcc string? - Email header Bcc
- headerCc string? - Email header Cc
- headerSubject string? - Email header Subject
- headerDate string? - Email header Date
- headerContentType string? - Email header ContentType
- mimeType string? - MIME type of the top level message part
- emailBodyInText MessageBodyPart? - MIME Message Part with text/plain content type
- emailBodyInHTML MessageBodyPart? - MIME Message Part with text/html content type
- emailInlineImages MessageBodyPart[]? - MIME Message Parts with inline images with the image/* content type. Note: Here attachments need to be less than 25MB.
- msgAttachments MessageBodyPart[]? - MIME Message Parts of the message consisting the attachments. Note: Here attachments need to be less than 25MB.
googleapis.gmail: MessageBodyPart
Represents the email message body part of a message resource response.
Fields
- data string? - The body data of the message part. This is a base64 encoded string
- mimeType string? - MIME type of the message part
- fileId string? - The file ID of the attachment/inline image in message part (This is empty unless the message part represent an inline image/attachment)
- fileName string? - The file name of the attachment/inline image in message part (This is empty unless the message part represent an inline image/attachment)
- partId string? - The part ID of the message part
- size int? - Number of bytes of message part data
googleapis.gmail: MessageListPage
Represents a page of the message list received as response for list messages api call.
Fields
- messages Message[]? - Array of message maps with messageId and threadId as keys
- resultSizeEstimate int - Estimated size of the whole list
- nextPageToken string? - Token for next page of message list
googleapis.gmail: MessageRequest
Represents message request to send a mail.
Fields
- recipient string - The recipient of the mail
- subject string - The subject of the mail
- messageBody string - The message body of the mail. Can be either plain text or html text.
- contentType string? - The content type of the mail, whether it is text/plain or text/html. Only pass one of the
constant values defined in the module;
TEXT_PLAIN
orTEXT_HTML
. If the message contains some rich content (Eg: inline image, table etc), then it should be text/html (TEXT_HTML
) other wise it will be text/plain (TEXT_PLAIN
).
- cc string? - The cc recipient of the mail. Optional.
- bcc string? - The bcc recipient of the mail. Optional.
- sender string? - The sender of the mail. Optional
- inlineImagePaths InlineImagePath[]? - The InlineImagePath array consisting the inline image file paths and mime types. Optional.
Note that inline images can only be send for
TEXT_HTML
messages.
- attachmentPaths AttachmentPath[]? - The AttachmentPath array consisting the attachment file paths and mime types. Optional.
googleapis.gmail: MsgSearchFilter
Represents the optional search message filter fields.
Fields
- includeSpamTrash boolean? - Specifies whether to include messages/threads from SPAM and TRASH in the results
- labelIds string[]? - Array of label ids. Only return messages/threads with labels that match all of the specified label Ids.
- maxResults int? - Maximum number of messages/threads to return in the page for a single request
- pageToken string? - Page token to retrieve a specific page of results in the list
- q string? - Query for searching messages/threads. Only returns messages/threads matching the specified query. Supports the same query format as the Gmail search box.
googleapis.gmail: ThreadListPage
Represents a page of the mail thread list received as response for list threads api call.
Fields
- threads MailThread[]? - Array of thread maps with threadId, snippet and historyId as keys
- resultSizeEstimate int - Estimated size of the whole list
- nextPageToken string? - Token for next page of mail thread list
googleapis.gmail: UserProfile
Represents Gmail UserProfile.
Fields
- emailAddress string - The user's email address
- messagesTotal int - The total number of messages in the mailbox
- threadsTotal int - The total number of threads in the mailbox
- historyId string - The ID of the mailbox's current history record
Union types
googleapis.gmail: Filter
Filter
Represents union of MsgSearchFilter and DraftSearchFilter.
Import
import ballerinax/googleapis.gmail;
Metadata
Released date: over 1 year ago
Version: 3.4.0
License: Apache-2.0
Compatibility
Platform: java11
Ballerina version: 2201.6.0
Pull count
Total: 11070
Current verison: 186
Weekly downloads
Keywords
Communication/Email
Cost/Free
Vendor/Google
Contributors