formstack
Module formstack
API
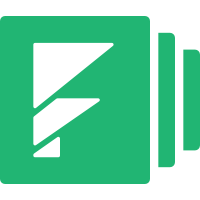
ballerinax/formstack Ballerina library
Overview
This is a generated connector for Formstack REST API v2.0 OpenAPI specification. Formstack is a workplace productivity platform that helps organizations streamline digital work through no-code online forms, documents, and signatures.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create an Formstack account.
- Obtain tokens - Follow this guide.
Quickstart
To use the Formstack connector in your Ballerina application, update the .bal file as follows
Step 1: Import connector
First, import the ballerinax/formstack
module into the Ballerina project.
import ballerinax/formstack;
Step 2: Create a new connector instance
Create a formstack:ClientConfig
with the access token, and initialize the connector with it.
configurable http:BearerTokenConfig & readonly authConfig = ?; formstack:ClientConfig clientConfig = {auth : authConfig}; formstack:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to get the details of the specified form.
public function main() returns error? { formstack:Form form = check baseClient->getFormById(4771452); log:printInfo(form.toString()); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
formstack: Client
This is a generated connector for Formstack REST API v2.0 OpenAPI specification. The Formstack REST API provides a way that helps organizations streamline digital work through no-code online forms, documents, and signatures.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create an Formstack account and obtain tokens following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://www.formstack.com/api/v2" - URL of the target service
getAllForms
Get all forms in your account
Parameters
- folders boolean? (default ()) - Flag to return forms in lists separated by folder
- page int? (default ()) - Page to use when paginating through forms. Starts at 1
- perPage int? (default ()) - Number of forms to return per page. Minimum page limit is 10
getFormById
Get the details of the specified form
Parameters
- id int - Form ID
updateFormById
function updateFormById(int id, FormRequest payload) returns SuccessOperation|error
Update the details of the specified form
Return Type
- SuccessOperation|error - Successful response
deleteFormById
function deleteFormById(int id) returns SuccessOperation|error
Delete the specified form
Parameters
- id int - Form ID
Return Type
- SuccessOperation|error - Successful response
copyFormById
Create a copy of the specified form
Parameters
- id int - Form ID
getBasicFormById
Get the basic details of the specified form
Parameters
- id int - Form ID
getHtmlFormById
Get the html of the specified form
Parameters
- id int - Form ID
getAllFolders
Get all folders on your account and their subfolders
Parameters
- page int? (default ()) - Page to use when paginating through forms. Starts at 1
- perPage int? (default ()) - Number of forms to return per page. Minimum page limit is 10
getFolderById
Get details for the specified folder or subfolder
Parameters
- id int - Folder ID
updateFolderById
function updateFolderById(int id, FolderRequest payload) returns SuccessOperation|error
Update the specified folder
Return Type
- SuccessOperation|error - Successful response
deleteFolderById
function deleteFolderById(int id) returns SuccessOperation|error
Delete the specified folder
Parameters
- id int - Folder ID
Return Type
- SuccessOperation|error - Successful response
getAllFields
Get all fields for the specified form
Parameters
- id int - Form ID
Return Type
- Fields|error - Successful response
getFieldById
Get the details of the specified field
Parameters
- id int - Field ID
updateFieldById
function updateFieldById(int id, FieldRequest payload) returns SuccessOperation|error
Update the specified field
Return Type
- SuccessOperation|error - Successful response
deleteFieldById
function deleteFieldById(int id) returns SuccessOperation|error
Delete the specified field
Parameters
- id int - Field ID
Return Type
- SuccessOperation|error - Successful response
Records
formstack: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
formstack: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
formstack: Field
Fields
- id string? -
- label string? -
- hide_label string? -
- description string? -
- name string? -
- 'type string? -
- required string? -
- options Option[]? -
formstack: FieldRequest
Fields
- field_type string? - Field type
- label string? - Field label
- hide_label boolean? - Flag to show or hide the label
- description string? - Field description (shown below field)
- description_callout boolean? - Flag to show the description in a callout box
- default_value string? - Initial value for the field
- options Option[]? - Array of option labels
formstack: Folder
Fields
- id string? - Form ID
- name string? -
- parent string? -
- permissions string? -
- subfolders Folder[]? -
formstack: FolderRequest
Fields
- name string? - Folder name
formstack: Folders
Fields
- folders Folder[]? - Folders details
- total int? - Total forms
formstack: Form
Fields
- id string? - Form ID
- created string? -
- db string? -
- deleted string? -
- folder string? -
- language string? -
- num_columns string? -
- progress_meter string? -
- timezone string? -
- rss_url string? -
- viewkey string? -
- thumbnail_url string? -
- can_access_1q_feature boolean? -
- is_workflow_form boolean? -
- inactive boolean? -
- submit_button_title string? -
- permissions int? -
- submissions_unread string? -
- should_display_one_question_at_a_time boolean? -
- views string? -
- has_approvers boolean? -
- can_edit boolean? -
- url string? -
- is_workflow_published boolean? -
- submissions_today int? -
- encrypted boolean? -
- submissions string? -
- name string? -
- edit_url string? -
- updated string? -
- data_url string? -
- summary_url string? -
- last_submission_id string? -
- last_submission_time string? -
formstack: FormRequest
Fields
- name string? - The form name
- db boolean? - Flag to disable or enable submissions to be saved in our database. Value 1 if true and 0 if false
- template string? - Template ID for the template you want to use
- num_columns int? - Number (1-4) of columns the form layout will have
- label_position string? - Sets the default field label position. Possible values are top and left
- submit_button_title string? - Sets the submit button title
- password string? - Sets a password for the form
- use_ssl boolean? - Flag to disable or enable SSL (only available on accounts that have security features)
- timezone string? - Sets the timezone for the form
- language string? - Sets the language for the form - use ISO 639-1 language codes
- active boolean? - Flag to make the form active/inactive
- disabled_message string? - The message to show when the form is inactive
formstack: Forms
Fields
- forms Form[]? - Forms details
- total int? - Total forms
formstack: HtmlForm
Fields
- id string? - Form ID
- html string? - HTML form representation
formstack: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "/oauth/token") - Refresh URL
formstack: Operation
Fields
- id string? - Form ID
formstack: Option
Fields
- label string? -
- value string? -
- imageUrl string? -
formstack: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
formstack: SuccessOperation
Fields
- success string? - Operation status
- id string? - Form ID
Import
import ballerinax/formstack;
Metadata
Released date: over 1 year ago
Version: 1.3.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 0
Current verison: 0
Weekly downloads
Keywords
Finance/Task Management
Cost/Freemium
Contributors