brex.team
Module brex.team
API
Definitions
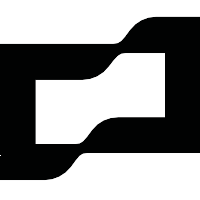
ballerinax/brex.team Ballerina library
Overview
This is a generated connector for Brex Team API v0.1 OpenAPI specification.
Team API lets you manage users, departments, locations and cards.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Brex account account
- Obtain tokens by following this guide
Quickstart
To use the Brex Onboarding connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/brex.team
module into the Ballerina project.
import ballerinax/brex.team;
Step 2: Create a new connector instance
Create a team:ClientConfig
with the <ACCESS_TOKEN>
obtained, and initialize the connector with it.
team:ClientConfig clientConfig = { authConfig : { token: <ACCESS_TOKEN> } }; team:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to list referrals created using the connector.
public function main() { team:ReferralPage|error response = baseClient->listReferralsGet(); if (response is team:ReferralPage) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
brex.team: Client
This is a generated connector for Brex Team API v0.1 OpenAPI specification. Team API lets you manage users, departments, locations and cards.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Brex account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://platform.staging.brexapps.com" - URL of the target service
listCardsByUserIdGet
List cards
Parameters
- userId string? (default ()) - User ID
- cursor string? (default ()) - Page cursor
- 'limit int? (default ()) - Object limit
createCardPost
function createCardPost(CreateCardRequest payload, string? idempotencyKey) returns Card|error
Create card
Parameters
- payload CreateCardRequest - Create card request
- idempotencyKey string? (default ()) - Idempotency key
getCardByIdGet
Get card
Parameters
- id string - Card ID
updateCardPut
function updateCardPut(string id, UpdateCardRequest payload, string? idempotencyKey) returns Card|error
Update card
Parameters
- id string - Card ID
- payload UpdateCardRequest - Update card request
- idempotencyKey string? (default ()) - Idenmpotency key
lockCardPost
function lockCardPost(string id, LockCardRequest payload, string? idempotencyKey) returns Card|error
Lock card
Parameters
- id string - Card ID
- payload LockCardRequest - Lock card request payload
- idempotencyKey string? (default ()) - Idempotency key
getCardNumberGet
function getCardNumberGet(string id) returns CardNumberResponse|error
Get card number
Parameters
- id string - Card ID
Return Type
- CardNumberResponse|error - getCardNumberGet 200 response
terminateCardPost
function terminateCardPost(string id, TerminateCardRequest payload, string? idempotencyKey) returns Card|error
Terminate card
Parameters
- id string - Card ID
- payload TerminateCardRequest - Terminate card request
- idempotencyKey string? (default ()) - Idempotency key
unlockCardPost
Unlock card
Parameters
- id string - Card ID
- payload json - Card unlock payload
- idempotencyKey string? (default ()) - Idempotency key
listDepartmentsGet
function listDepartmentsGet(string? cursor, int? 'limit) returns PageDepartmentresponse|error
List departments
Return Type
- PageDepartmentresponse|error - listDepartmentsGet 200 response
createDepartmentPost
function createDepartmentPost(CreateDepartmentRequest payload, string? idempotencyKey) returns DepartmentResponse|error
Create department
Parameters
- payload CreateDepartmentRequest - Create department request
- idempotencyKey string? (default ()) - Idempotency key
Return Type
- DepartmentResponse|error - createDepartmentPost 200 response
getDepartmentByIdGet
function getDepartmentByIdGet(string id) returns DepartmentResponse|error
Get department
Parameters
- id string - Department ID
Return Type
- DepartmentResponse|error - getDepartmentByIdGet 200 response
listLocationsGet
function listLocationsGet(string? cursor, int? 'limit) returns PageLocationresponse|error
List locations
Return Type
- PageLocationresponse|error - listLocationsGet 200 response
createLocationPost
function createLocationPost(CreateLocationRequest payload, string? idempotencyKey) returns LocationResponse|error
Create location
Parameters
- payload CreateLocationRequest - Create location request
- idempotencyKey string? (default ()) - Idempotency key
Return Type
- LocationResponse|error - createLocationPost 200 response
getLocationByIdGet
function getLocationByIdGet(string id) returns LocationResponse|error
Get location
Parameters
- id string - Location ID
Return Type
- LocationResponse|error - getLocationByIdGet 200 response
listUsersGet
function listUsersGet(string? cursor, int? 'limit) returns PageUserresponse|error
List users
Return Type
- PageUserresponse|error - listUsersGet 200 response
createUserPost
function createUserPost(CreateUserRequest payload, string? idempotencyKey) returns UserResponse|error
Invite user
Parameters
- payload CreateUserRequest - Create user request
- idempotencyKey string? (default ()) - Idempotency key
Return Type
- UserResponse|error - createUserPost 200 response
getMeGet
function getMeGet() returns UserResponse|error
Get current user
Return Type
- UserResponse|error - getMeGet 200 response
getUserByIdGet
function getUserByIdGet(string id) returns UserResponse|error
Get user
Parameters
- id string - User ID
Return Type
- UserResponse|error - getUserByIdGet 200 response
updateUserPut
function updateUserPut(string id, UpdateUserRequest payload, string? idempotencyKey) returns UserResponse|error
Update user
Parameters
- id string - User ID
- payload UpdateUserRequest - Update user request
- idempotencyKey string? (default ()) - Idempotency key
Return Type
- UserResponse|error - updateUserPut 200 response
getUserLimitGet
function getUserLimitGet(string id) returns UserLimitResponse|error
Get limit for the user
Parameters
- id string - User ID
Return Type
- UserLimitResponse|error - getUserLimitGet 200 response
setUserLimitPost
function setUserLimitPost(string id, SetUserLimitRequest payload, string? idempotencyKey) returns UserLimitResponse|error
Set limit for the user
Parameters
- id string - User ID
- payload SetUserLimitRequest - Set user limit request
- idempotencyKey string? (default ()) - Idempotency key
Return Type
- UserLimitResponse|error - setUserLimitPost 200 response
Records
brex.team: Address
Company business address (must be in the US; no PO box or virtual/forwarding addresses allowed).
Fields
- line1 string? - Address line 1, no PO Box.
- line2 string? - Address line 2 (e.g., apartment, suite, unit, or building).
- city string? - City, district, suburb, town, or village.
- state string? - For US-addressed the 2-letter State abbreviation. For international-addresses the County, Providence, or Region.
- country string? - Two-letter country code (ISO 3166-1 alpha-2).
- postal_code string? - ZIP or postal code.
- phone_number string? - Phone number.
brex.team: Card
Only cards with limit_type = CARD have spend_controls
Fields
- id string - Card ID
- owner CardOwner - Card owner information.
- status CardStatus? - Card status
- last_four string - Last four digits of card number
- card_name string - Card name
- card_type CardType? - Card type
- limit_type LimitType - If
card_type
=CARD
then the card is a vendor card and it doesn't rely on the user limit. A vendor has to be a virtual card. Ifcard_type
=USER
then the card is a corporate card. Check out this article about different card types.
- spend_controls SpendControl? - Spend control data
- billing_address Address - Company business address (must be in the US; no PO box or virtual/forwarding addresses allowed).
- mailing_address Address? - Company business address (must be in the US; no PO box or virtual/forwarding addresses allowed).
brex.team: CardExpiration
Month and year of expiration
Fields
- month int? - Month of expiration
- year int? - Year of expiration
brex.team: CardNumberResponse
Card number, CVV and expiration date of a card
Fields
- id string - Card number id
- number string - Card number
- cvv string - CVV
- expiration_date CardExpiration - Month and year of expiration
brex.team: CardOwner
Card owner information.
Fields
- 'type OwnerType? - Owner type
- user_id string? - User ID
brex.team: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
brex.team: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
brex.team: CreateCardRequest
The spend_controls
field is required when limit_type
= CARD
. The shipping_address
field is required for physical cards, and the first two lines of the address must be under 60 characters long.
Fields
- owner CardOwner - Card owner information.
- card_name string - Card name.
- card_type CardType - Card type
- limit_type LimitType - If
card_type
=CARD
then the card is a vendor card and it doesn't rely on the user limit. A vendor has to be a virtual card. Ifcard_type
=USER
then the card is a corporate card. Check out this article about different card types.
- spend_controls SpendControlRequest? - Spend control request
- mailing_address Address? - Company business address (must be in the US; no PO box or virtual/forwarding addresses allowed).
brex.team: CreateDepartmentRequest
Fields
- name string - Name of the department
- description string? - Description of the department
brex.team: CreateLocationRequest
Fields
- name string - Name of the location
- description string? - Description of the location
brex.team: CreateUserRequest
Fields
- first_name string - First name
- last_name string - Last name
- email string - Email address
- manager_id string? - The ID of the manager
- department_id string? - Department ID
- location_id string? - Location ID
brex.team: DepartmentResponse
Fields
- id string -
- name string - Name of the department
- description string? - Description of the department
brex.team: LocationResponse
Fields
- id string -
- name string - Name of the location
- description string? - Description of the location
brex.team: LockCardRequest
Parameters for locking a card.
Fields
- description string? - Description for locking a card
- reason ChangeCardReason - Reason for card termination.
brex.team: Money
Money fields can be signed or unsigned. Fields are signed (an unsigned value will be interpreted as positive).
Fields
- amount int? - The amount of money, in the smallest denomination of the currency indicated by currency. For example, when currency is USD, amount is in cents.
- currency string? - The type of currency, in ISO 4217 format. Default to USD if not specified
brex.team: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://accounts.brex.com/oauth2/v1/token") - Refresh URL
brex.team: PageCard
Fields
- next_cursor string? - Cursor for next page
- items Card[] - Array of Card ojects
brex.team: PageDepartmentresponse
Fields
- next_cursor string? - Cursor for next page
- items DepartmentResponse[] - Array of Department ojects
brex.team: PageLocationresponse
Fields
- next_cursor string? - Cursor for next page
- items LocationResponse[] - Array of Location response objects
brex.team: PageUserresponse
Fields
- next_cursor string? - Cursor for next page
- items UserResponse[] - Array of User response objects
brex.team: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
brex.team: SetUserLimitRequest
Request that sets the monthly user limit.
Fields
- monthly_limit Money? - Money fields can be signed or unsigned. Fields are signed (an unsigned value will be interpreted as positive).
brex.team: SpendControl
Spend control data
Fields
- spend_limit Money - Money fields can be signed or unsigned. Fields are signed (an unsigned value will be interpreted as positive).
- spend_available Money - Money fields can be signed or unsigned. Fields are signed (an unsigned value will be interpreted as positive).
- spend_duration SpendDuration? - Spend limit refresh frequency - MONTHLY: The spend limit refreshes every month - QUARTERLY: The spend limit refreshes every quarter - YEARLY: The spend limit refreshes every year - ONE_TIME: The limit does not refresh
- reason string? - Reason for spend control
- lock_after_date string? - Date to lock the spend
brex.team: SpendControlRequest
Spend control request
Fields
- spend_limit Money - Money fields can be signed or unsigned. Fields are signed (an unsigned value will be interpreted as positive).
- spend_duration SpendDuration - Spend limit refresh frequency - MONTHLY: The spend limit refreshes every month - QUARTERLY: The spend limit refreshes every quarter - YEARLY: The spend limit refreshes every year - ONE_TIME: The limit does not refresh
- reason string? - Reason for the spend control
- lock_after_date string? - Date for locking the spend control
brex.team: SpendControlUpdateRequest
Spend control request data
Fields
- spend_limit Money? - Money fields can be signed or unsigned. Fields are signed (an unsigned value will be interpreted as positive).
- spend_duration SpendDuration? - Spend limit refresh frequency - MONTHLY: The spend limit refreshes every month - QUARTERLY: The spend limit refreshes every quarter - YEARLY: The spend limit refreshes every year - ONE_TIME: The limit does not refresh
- lock_after_date string? - Date to lock the spend
brex.team: TerminateCardRequest
Parameters for terminating a card.
Fields
- description string? - Description for terminating a card
- reason ChangeCardReason - Reason for card termination.
brex.team: UpdateCardRequest
Fields
- spend_controls SpendControlUpdateRequest? - Spend control request data
brex.team: UpdateUserRequest
Fields
- status UpdateUserStatus? - Acceptable user status for update. To suspend a user, set status to 'disabled'. To unsuspend a user, set status to 'active'.
- manager_id string? - The user ID of the manager of this user
- department_id string? - Department ID
- location_id string? - Location ID
brex.team: UserLimitResponse
Fields
- monthly_limit Money? - Money fields can be signed or unsigned. Fields are signed (an unsigned value will be interpreted as positive).
brex.team: UserResponse
Fields
- id string - User ID
- first_name string - First name of the user
- last_name string - Last name of the user
- email string - Email address of the user
- status UserStatus? - Status of the user
- manager_id string? - The user id of the manager of this user
- department_id string? - Department ID
- location_id string? - Loaction ID
String types
brex.team: CardStatus
CardStatus
Card status
brex.team: CardType
CardType
Card type
brex.team: ChangeCardReason
ChangeCardReason
Reason for card termination.
brex.team: LimitType
LimitType
If card_type
= CARD
then the card is a vendor card and it doesn't rely on the user limit. A vendor has to be a virtual card. If card_type
= USER
then the card is a corporate card. Check out this article about different card types.
brex.team: OwnerType
OwnerType
Owner type
brex.team: SpendDuration
SpendDuration
Spend limit refresh frequency - MONTHLY: The spend limit refreshes every month - QUARTERLY: The spend limit refreshes every quarter - YEARLY: The spend limit refreshes every year - ONE_TIME: The limit does not refresh
brex.team: UpdateUserStatus
UpdateUserStatus
Acceptable user status for update. To suspend a user, set status to 'disabled'. To unsuspend a user, set status to 'active'.
brex.team: UserStatus
UserStatus
Status of the user
Import
import ballerinax/brex.team;
Metadata
Released date: over 1 year ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 2
Current verison: 1
Weekly downloads
Keywords
Human Resources/HRMS
Cost/Freemium
Contributors