aws.ses
Module aws.ses
API
Declarations
Definitions
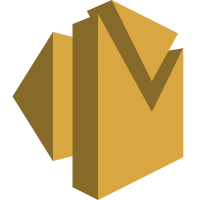
ballerinax/aws.ses Ballerina library
Overview
The Ballerina AWS SES connector provides the capability to programatically handle AWS SES related operations.
This module supports Amazon SES REST API v2.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create an AWS account
- Obtain tokens
Quickstart
To use the AWS SES connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
Import the ballerinax/aws.ses
module into the Ballerina project.
import ballerinax/aws.ses;
Step 2: Create a new connector instance
Create an ses:ConnectionConfig
with the tokens obtained, and initialize the connector with it.
ses:ConnectionConfig amazonSesConfig = { awsCredentials: { accessKeyId: "<ACCESS_KEY_ID>", secretAccessKey: "<SECRET_ACCESS_KEY>" } }; ses:Client amazonSesClient = check new(amazonSesConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to create an email identity using the connector.ublic function main() returns error? { string emailIdentity = "<Your_Email_Identity>"; // Email address or Domain can be used. EmailIdentityCreationRequest request = { EmailIdentity: emailIdentity }; EmailIdentity response = check amazonSesClient->createEmailIdentity(request);
-
Use
bal run
command to compile and run the Ballerina program.
Clients
aws.ses: Client
The Ballerina AWS SES connector provides the capability to access AWS Simple Email Service related operations. This connector lets you to to send email messages to your customers.
Constructor
Initializes the connector. During initialization you have to pass access key id and secret access key Create an AWS account and obtain tokens following [this guide] (https://docs.aws.amazon.com/IAM/latest/UserGuide/id_credentials_access-keys.html).
init (ConnectionConfig config)
- config ConnectionConfig - The configurations to be used when initializing the client
createContactList
function createContactList(ContactListCreationRequest contactListCreationRequest) returns error?
Creates a contact list.
Parameters
- contactListCreationRequest ContactListCreationRequest - The request payload to create contact list
Return Type
- error? - An error on failure or else
()
updateContactList
function updateContactList(string contactListName, ContactList updateContactListRequest) returns error?
Updates contact list metadata. This operation does a complete replacement.
Parameters
- contactListName string - The name of the contact list
- updateContactListRequest ContactList - The request payload to update the contact list
Return Type
- error? - An error on failure or else
()
getContactList
function getContactList(string contactListName) returns ContactList|error
Returns contact list metadata. It does not return any information about the contacts present in the list.
Parameters
- contactListName string - The name of the contact list
Return Type
- ContactList|error - An aws.ses:ContactList record on success else an error
listContactLists
function listContactLists() returns ContactLists|error
Lists all of the contact lists available.
Return Type
- ContactLists|error - An aws.ses:ContactLists record on success else an error
deleteContactList
Deletes a contact list and all of the contacts on that list.
Parameters
- contactListName string - The name of the contact list
Return Type
- error? - An error on failure or else
()
createContact
function createContact(string contactListName, ContactCreationRequest contactCreationRequest) returns error?
Creates a contact, which is an end-user who is receiving the email, and adds them to a contact list.
Parameters
- contactListName string - The name of the contact list
- contactCreationRequest ContactCreationRequest - The request payload to create contact
Return Type
- error? - An error on failure or else
()
updateContact
function updateContact(string contactListName, string emailAddress, ContactUpdateRequest contactUpdateRequest) returns error?
Updates a contact's preferences for a list. It is not necessary to specify all existing topic preferences in the TopicPreferences object, just the ones that need updating.
Parameters
- contactListName string - The name of the contact list
- emailAddress string - The contact's email addres
- contactUpdateRequest ContactUpdateRequest - The request payload to update the contact
Return Type
- error? - An error on failure or else
()
getContact
Returns a contact from a contact list.
Parameters
- contactListName string - The name of the contact list
- emailAddress string - The contact's email addres
listContacts
Lists the contacts present in a specific contact list.
Parameters
- contactListName string - The name of the contact list
deleteContact
Removes a contact from a contact list.
Parameters
- contactListName string - The name of the contact list
- emailAddress string - The contact's email addres
Return Type
- error? - An error on failure or else
()
createCustomVerificationEmailTemplate
function createCustomVerificationEmailTemplate(CustomVerificationEmailTemplate emailTemplate) returns error?
Creates a new custom verification email template.
Parameters
- emailTemplate CustomVerificationEmailTemplate - The request payload to create custom verification email template
Return Type
- error? - An error on failure or else
()
updateCustomVerificationEmailTemplate
function updateCustomVerificationEmailTemplate(string templateName, CustomVerificationEmailUpdate emailTemplateUpdateRequest) returns error?
Updates an existing custom verification email template.
Parameters
- templateName string - The name of the custom verification email template that you want to update
- emailTemplateUpdateRequest CustomVerificationEmailUpdate - The request payload to update custom verification email template
Return Type
- error? - An error on failure or else
()
getCustomVerificationEmailTemplate
function getCustomVerificationEmailTemplate(string templateName) returns CustomVerificationEmailTemplate|error
Returns the custom email verification template for the template name you specify.
Parameters
- templateName string - The name of the custom verification email template that you want to retrieve
Return Type
- CustomVerificationEmailTemplate|error - An aws.ses:CustomVerificationEmailTemplate record on success else an error
listCustomVerificationEmailTemplates
function listCustomVerificationEmailTemplates() returns CustomVerificationTempListPage|error
Lists the existing custom verification email templates for your account in the current AWS Region.
Return Type
- CustomVerificationTempListPage|error - An aws.ses:CustomVerificationTempListPage record on success else an error
deleteCustomVerificationEmailTemplate
Deletes an existing custom verification email template.
Parameters
- templateName string - The name of the custom verification email template that you want to delete
Return Type
- error? - An error on failure or else
()
createEmailTemplate
function createEmailTemplate(EmailTemplate emailTemplateCreationRequest) returns error?
Creates an email template. Email templates enable you to send personalized email to one or more destinations in a single API operation.
Parameters
- emailTemplateCreationRequest EmailTemplate - The request payload to create email template
Return Type
- error? - An error on failure or else
()
updateEmailTemplate
function updateEmailTemplate(string templateName, EmailTemplateUpdateRequest emailTemplateUpdateRequest) returns error?
Updates an email template. Email templates enable you to send personalized email to one or more destinations in a single API operation.
Parameters
- templateName string - The name of the template
- emailTemplateUpdateRequest EmailTemplateUpdateRequest - The request payload to update email template
Return Type
- error? - An error on failure or else
()
getEmailTemplate
function getEmailTemplate(string templateName) returns EmailTemplate|error
Displays the template object (which includes the subject line, HTML part and text part) for the template you specify.
Parameters
- templateName string - The name of the template
Return Type
- EmailTemplate|error - An aws.ses:EmailTemplate record on success else an error
listEmailTemplates
function listEmailTemplates() returns EmailTemplateListPage|error
Lists the email templates present in your Amazon SES account in the current AWS Region.
Return Type
- EmailTemplateListPage|error - An aws.ses:EmailTemplateListPage record on success else an error
deleteEmailTemplate
Deletes an email template.
Parameters
- templateName string - The name of the template to be deleted
Return Type
- error? - An error on failure or else
()
createEmailIdentity
function createEmailIdentity(EmailIdentityCreationRequest emailIdentityCreationRequest) returns EmailIdentity|error
Starts the process of verifying an email identity. An identity is an email address or domain that you use when you send email. Before you can use an identity to send email, you first have to verify it.
Parameters
- emailIdentityCreationRequest EmailIdentityCreationRequest - The request payload to create email identity
Return Type
- EmailIdentity|error - An aws.ses:EmailIdentity on success else an error
getEmailIdentity
function getEmailIdentity(string emailIdentity) returns EmailIdentityInfo|error
Provides information about a specific identity, including the identity's verification status, sending authorization policies, its DKIM authentication status, and its custom Mail-From settings.
Parameters
- emailIdentity string - The email identity
Return Type
- EmailIdentityInfo|error - An aws.ses:EmailIdentityInfo record on success else an error
listEmailIdentities
function listEmailIdentities() returns EmailIdentitiesListPage|error
Returns a list of all of the email identities that are associated with your AWS account. An identity can be either an email address or a domain. This operation returns identities that are verified as well as those that aren't. This operation returns identities that are associated with Amazon SES and Amazon Pinpoint.
Return Type
- EmailIdentitiesListPage|error - An aws.ses:EmailIdentitiesListPage record on success else an error
deleteEmailIdentity
Deletes an email identity. An identity can be either an email address or a domain name.
Parameters
- emailIdentity string - The identity (that is, the email address or domain) to delete
Return Type
- error? - An error on failure or else
()
sendEmail
function sendEmail(EmailRequest requestPayload) returns MessageSentResponse|error
Sends an email message. You can use the Amazon SES API v2 to send the following types of messages: • Simple – A standard email message. When you create this type of message, you specify the sender, the recipient, and the message body, and Amazon SES assembles the message for you. • Raw – A raw, MIME-formatted email message. When you send this type of email, you have to specify all of the message headers, as well as the message body. You can use this message type to send messages that contain attachments. The message that you specify has to be a valid MIME message. • Templated – A message that contains personalization tags. When you send this type of email, Amazon SES API v2 automatically replaces the tags with values that you specify.
Parameters
- requestPayload EmailRequest - The request payload to send email
Return Type
- MessageSentResponse|error - An aws.ses:MessageSentResponse record on success else an error
sendCustomVerificationEmail
function sendCustomVerificationEmail(CustomVerificationEmailRequest requestPayload) returns MessageSentResponse|error
Adds an email address to the list of identities for your Amazon SES account in the current AWS Region and attempts to verify it. As a result of executing this operation, a customized verification email is sent to the specified address. To use this operation, you must first create a custom verification email template.
Parameters
- requestPayload CustomVerificationEmailRequest - The request payload to send custom verification email
Return Type
- MessageSentResponse|error - An aws.ses:MessageSentResponse record on success else an error
sendBulkEmail
function sendBulkEmail(BulkEmailRequest requestPayload) returns BulkEmailResponse|error
Composes an email message to multiple destinations.
Parameters
- requestPayload BulkEmailRequest - The request payload to send bulk email
Return Type
- BulkEmailResponse|error - An aws.ses:BulkEmailResponse record on success else an error
Enums
aws.ses: BehaviorOnMxFailure
Members
aws.ses: DkimAttributesStatus
Members
aws.ses: IdentityType
Members
aws.ses: SigningAttributesOrigin
Members
aws.ses: SubscriptionStatus
Members
Records
aws.ses: AwsCredentials
Represents AWS credentials.
Fields
- accessKeyId string - AWS access key
- secretAccessKey string - AWS secret key
aws.ses: AwsTemporaryCredentials
Represents AWS temporary credentials.
Fields
- accessKeyId string - AWS access key
- secretAccessKey string - AWS secret key
- securityToken string - AWS secret token
aws.ses: Body
Represents the body of the email message.
Fields
- Text Subject - The text
aws.ses: BulkEmailContent
Represents an object that contains the body of the message. You can specify a template message.
Fields
- Template Template? - The template to use for the bulk email message
aws.ses: BulkEmailEntry
Represents the bulk email entry.
Fields
- Destination Destination - Represents the destination of the message, consisting of To:, CC:, and BCC: fields
- ReplacementEmailContent ReplacementEmailContent? - The ReplacementEmailContent associated with a BulkEmailEntry
- ReplacementTags MessageTag[]? - A list of tags, in the form of name/value pairs, to apply to an email that you send using the SendBulkTemplatedEmail operation
aws.ses: BulkEmailEntryResult
Represents the result of the SendBulkEmail operation of each specified BulkEmailEntry.
Fields
- Error string? - A description of an error that prevented a message being sent using the SendBulkTemplatedEmail operation
- MessageId string? - The unique message identifier returned from the SendBulkTemplatedEmail operation
- Status string? - The status of a message sent using the SendBulkTemplatedEmail operation
aws.ses: BulkEmailRequest
Represents the request payload to send bulk email
Fields
- BulkEmailEntries BulkEmailEntry[] - The list of bulk email entry objects
- ConfigurationSetName string? - The name of the configuration set to use when sending the email
- DefaultContent BulkEmailContent - An object that contains the body of the message. You can specify a template message.
- DefaultEmailTags MessageTag[]? - A list of tags, in the form of name/value pairs, to apply to an email that you send using the SendEmail operation
- FeedbackForwardingEmailAddress string? - The address that you want bounce and complaint notifications to be sent to
- FeedbackForwardingEmailAddressIdentityArn string? - This parameter is used only for sending authorization. It is the ARN of the identity that is associated with the sending authorization policy that permits you to use the email address specified in the FeedbackForwardingEmailAddress parameter.
- FromEmailAddress string? - The email address to use as the "From" address for the email. The address that you specify has to be verified.
- FromEmailAddressIdentityArn string? - This parameter is used only for sending authorization. It is the ARN of the identity that is associated with the sending authorization policy that permits you to use the email address specified in the FromEmailAddress parameter.
- ReplyToAddresses string[]? - The "Reply-to" email addresses for the message. When the recipient replies to the message, each Reply-to address receives the reply.
aws.ses: BulkEmailResponse
Represents the response after sent bulk email.
Fields
- BulkEmailEntryResults BulkEmailEntryResult[]? - The array of BulkEmailEntryResult objects
aws.ses: ConnectionConfig
Represents the AWS SES Connector configurations.
Fields
- Fields Included from *ConnectionConfig
- auth AuthConfig
- httpVersion HttpVersion
- http1Settings ClientHttp1Settings
- http2Settings ClientHttp2Settings
- timeout decimal
- forwarded string
- poolConfig PoolConfiguration
- cache CacheConfig
- compression Compression
- circuitBreaker CircuitBreakerConfig
- retryConfig RetryConfig
- responseLimits ResponseLimitConfigs
- secureSocket ClientSecureSocket
- proxy ProxyConfig
- validation boolean
- anydata...
- auth never? -
- awsCredentials AwsCredentials|AwsTemporaryCredentials - AWS credentials
- region string(default DEFAULT_REGION) - AWS Region
aws.ses: Contact
Represents the contact.
Fields
- ContactListName string? - The name of the contact list to which the contact belongs
- CreatedTimestamp decimal? - A timestamp noting when the contact was created
- EmailAddress string? - The contact's email addres
- LastUpdatedTimestamp decimal? - A timestamp noting the last time the contact's information was updated
- TopicDefaultPreferences TopicPreference[]? - The default topic preferences applied to the contact
- Fields Included from *ContactUpdateRequest
- AttributesData string|()
- TopicPreferences TopicPreference[]|()
- UnsubscribeAll boolean
- anydata...
aws.ses: ContactCreationRequest
Represents the request payload to create contact.
Fields
- EmailAddress string - The contact's email address
- Fields Included from *ContactUpdateRequest
- AttributesData string|()
- TopicPreferences TopicPreference[]|()
- UnsubscribeAll boolean
- anydata...
aws.ses: ContactList
Represents the contact list.
Fields
- ContactListName string? - The name of the contact list
- CreatedTimestamp decimal? - A timestamp noting when the contact list was created
- Description string? - A description of what the contact list is about
- LastUpdatedTimestamp decimal? - A timestamp noting the last time the contact list was updated
- Tags Tag[]? - The tags associated with a contact list
- Topics Topic[]? - An interest group, theme, or label within a list
aws.ses: ContactListCreationRequest
Represents the request to create contact list.
Fields
- ContactListName string - The name of the contact list
- Description string? - A description of what the contact list is about
- Tags Tag[]? - The tags associated with a contact list
- Topics Topic[]? - An interest group, theme, or label within a list
aws.ses: ContactLists
Represents the list of contact lists.
Fields
- ContactLists ContactList[] - The available contact lists
- NextToken string? - A string token indicating that there might be additional contact lists available to be listed
aws.ses: Contacts
Represents the list of contacts
Fields
- Contacts Contact[]? - The contacts present in a specific contact list
- NextToken string? - A string token indicating that there might be additional contacts available to be listed
aws.ses: ContactUpdateRequest
Represents the request payload to update contact.
Fields
- AttributesData string? - The attribute data attached to a contact
- TopicPreferences TopicPreference[]? - The contact's preferences for being opted-in to or opted-out of topics
- UnsubscribeAll boolean? - A boolean value status noting if the contact is unsubscribed from all contact list topics
aws.ses: Content
Represents the email message content.
Fields
- Simple Message - The simple message content
aws.ses: CustomVerificationTempListPage
Represents the list of custom verification email templates.
Fields
- CustomVerificationEmailTemplates CustomVerificationEmailTemplateElement[]? - A list of the custom verification email templates that exist in your account
- NextToken string? - A token indicating that there are additional custom verification email templates available to be listed
aws.ses: CustomVerificationEmailRequest
Represents the request payload to send custom verification email.
Fields
- EmailAddress string - The email address to verify
- TemplateName string - The name of the custom verification email template to use when sending the verification email
- ConfigurationSetName string? - Name of a configuration set to use when sending the verification email
aws.ses: CustomVerificationEmailTemplate
Represents the custom verification email template.
Fields
- Fields Included from *CustomVerificationEmailTemplateElement
- TemplateContent string - The content of the email, composed of a subject line, an HTML part, and a text-only part
aws.ses: CustomVerificationEmailTemplateElement
Represents the element of custom verification email template.
Fields
- FailureRedirectionURL string - The URL that the recipient of the verification email is sent to if his or her address is not successfully verified
- FromEmailAddress string - The email address that the custom verification email is sent from
- SuccessRedirectionURL string - The URL that the recipient of the verification email is sent to if his or her address is successfully verified
- TemplateName string - The name of the custom verification email template
- TemplateSubject string - The subject line of the custom verification email
aws.ses: CustomVerificationEmailUpdate
Represents the request payload to update custom verification email template.
Fields
- FailureRedirectionURL string - The URL that the recipient of the verification email is sent to if his or her address is not successfully verified
- FromEmailAddress string - The email address that the custom verification email is sent from
- SuccessRedirectionURL string - The URL that the recipient of the verification email is sent to if his or her address is successfully verified
- TemplateContent string - The content of the custom verification email
- TemplateSubject string - The subject line of the custom verification email
aws.ses: Destination
Represents an object that describes the recipients for an email.
Fields
- BccAddresses string[]? - An array that contains the email addresses of the "BCC" (blind carbon copy) recipients for the email
- CcAddresses string[]? - An array that contains the email addresses of the "CC" (carbon copy) recipients for the email
- ToAddresses string[]? - An array that contains the email addresses of the "To" recipients for the email
aws.ses: DkimAttributes
Represents an object that contains information about the DKIM authentication status for an email identity.
Fields
- SigningAttributesOrigin SigningAttributesOrigin? - A string that indicates how DKIM was configured for the identity
- SigningEnabled boolean? - If the value is true, then the messages that you send from the identity are signed using DKIM. If the value is false, then the messages that you send from the identity aren't DKIM-signed.
- SigningKeyLength int? - Field Description
- Status DkimAttributesStatus? - Describes whether or not Amazon SES has successfully located the DKIM records in the DNS records for the domain
- Tokens string[]? - The tokens which are used in DKIM authentication
aws.ses: DkimSigningAttributes
Represents an object that contains information about the tokens used for setting up Bring Your Own DKIM (BYODKIM).
Fields
- DomainSigningPrivateKey string - A private key that's used to generate a DKIM signature
- DomainSigningSelector string - A string that's used to identify a public key in the DNS configuration for a domain
aws.ses: EmailIdentitiesListPage
Represents the list of email identities.
Fields
- EmailIdentities IdentityInfo[]? - An array that includes all of the email identities associated with your AWS account
- NextToken string? - A token that indicates that there are additional configuration sets to list
aws.ses: EmailIdentity
Represents the email identity
Fields
- DkimAttributes DkimAttributes? - An object that contains information about the DKIM attributes for the identity
- IdentityType IdentityType? - The email identity type. Note: the MANAGED_DOMAIN identity type is not supported.
- VerifiedForSendingStatus boolean? - Specifies whether or not the identity is verified
aws.ses: EmailIdentityCreationRequest
Represents the request payload to create email identity.
Fields
- EmailIdentity string - The email address or domain to verify
- ConfigurationSetName string? - The configuration set to use by default when sending from this identity
- DkimSigningAttributes DkimSigningAttributes? - DKIM signing attributes
- Tags Tag[]? - An array of objects that define the tags (keys and values) to associate with the email identity
aws.ses: EmailIdentityInfo
Represents the information of email identity.
Fields
- ConfigurationSetName string? - The configuration set used by default when sending from this identity
- Fields Included from *EmailIdentity
- DkimAttributes DkimAttributes|()
- IdentityType "MANAGED_DOMAIN"|"DOMAIN"|"EMAIL_ADDRESS"|()
- VerifiedForSendingStatus boolean|()
- anydata...
- FeedbackForwardingStatus boolean - The feedback forwarding configuration for the identity
- MailFromAttributes MailFromAttributes - An object that contains information about the Mail-From attributes for the email identity
- Tags Tag[]? - An array of objects that define the tags (keys and values) that are associated with the email identity
aws.ses: EmailRequest
Represents the request payload to send email.
Fields
- Content Content - An object that contains the body of the message. You can send either a Simple message Raw message or a template Message.
- Destination Destination - An object that contains the recipients of the email message
- FromEmailAddress string - The email address to use as the "From" address for the email. The address that you specify has to be verified.
aws.ses: EmailTemplate
Represents the email template.
Fields
- TemplateContent EmailTemplateContent - The content of the email template, composed of a subject line, an HTML part, and a text-only part
- TemplateName string - The name of the template
aws.ses: EmailTemplateContent
Represents the content of the email, composed of a subject line, an HTML part, and a text-only part.
Fields
- Html string? - The HTML body of the email
- Subject string? - The subject line of the email
- Text string? - The email body that will be visible to recipients whose email clients do not display HTML
aws.ses: EmailTemplateListPage
Represents the list of email templates.
Fields
- TemplatesMetadata EmailTemplateMetadata[]? - An array the contains the name and creation time stamp for each template in your Amazon SES account
- NextToken string? - A token indicating that there are additional email templates available to be listed
aws.ses: EmailTemplateMetadata
Represents the email template metadata.
Fields
- CreatedTimestamp decimal? - A timestamp noting when the contact was created
- TemplateName string? - The name of the template
aws.ses: EmailTemplateUpdateRequest
Represents the request payload to update email template.
Fields
- TemplateContent EmailTemplateContent - The content of the email, composed of a subject line, an HTML part, and a text-only part
aws.ses: IdentityInfo
Represents the information of identity.
Fields
- IdentityName string? - The address or domain of the identity
- IdentityType IdentityType? - The email identity type. Note: the MANAGED_DOMAIN type is not supported for email identity types.
- SendingEnabled boolean? - Indicates whether or not you can send email from the identity
aws.ses: MailFromAttributes
Represents a list of attributes that are associated with a MAIL FROM domain.
Fields
- BehaviorOnMxFailure BehaviorOnMxFailure - The action to take if the required MX record can't be found when you send an email
- MailFromDomain string - The name of a domain that an email identity uses as a custom MAIL FROM domain
- MailFromDomainStatus string - The status of the MAIL FROM domain
aws.ses: Message
Represents the email message that you're sending.
Fields
- Body Body - The body of the message. You can specify an HTML version of the message, a text-only version of the message, or both.
- Subject Subject - The subject line of the email
aws.ses: MessageSentResponse
Represents the response after sent an email.
Fields
- MessageId string? - A unique identifier for the message that is generated when the message is accepted
aws.ses: MessageTag
Represents the name and value of a tag that you apply to an email.
Fields
- Name string - The name of the message tag
- Value string - The value of the message tag
aws.ses: ReplacementEmailContent
Represents the ReplaceEmailContent object to be used for a specific BulkEmailEntry.
Fields
- ReplacementTemplate ReplacementTemplate? - The ReplacementTemplate associated with ReplacementEmailContent
aws.ses: ReplacementTemplate
Represents an object which contains ReplacementTemplateData to be used for a specific BulkEmailEntry.
Fields
- ReplacementTemplateData string? - A list of replacement values to apply to the template. This parameter is a JSON object, typically consisting of key-value pairs in which the keys correspond to replacement tags in the email template.
aws.ses: Subject
Represents the subject of the email.
Fields
- Charset string - The character set for the content. Because of the constraints of the SMTP protocol, Amazon SES uses 7-bit ASCII by default. If the text includes characters outside of the ASCII range, you have to specify a character set. For example, you could specify UTF-8, ISO-8859-1, or Shift_JIS.
- Data string - The content of the message itself
aws.ses: Tag
Represents tag.
Fields
- Key string - One part of a key-value pair that defines a tag. The maximum length of a tag key is 128 characters. The minimum length is 1 character.
- Value string - The optional part of a key-value pair that defines a tag. The maximum length of a tag value is 256 characters. The minimum length is 0 characters.
aws.ses: Template
Represents an object that defines the email template to use for an email message, and the values to use for any message variables in that template.
Fields
- TemplateArn string? - The Amazon Resource Name (ARN) of the template
- TemplateData string? - An object that defines the values to use for message variables in the template
- TemplateName string? - The name of the template
aws.ses: Topic
Represents topic.
Fields
- DefaultSubscriptionStatus SubscriptionStatus - The default subscription status to be applied to a contact if the contact has not noted their preference for subscribing to a topic
- Description string? - A description of what the topic is about, which the contact will see
- DisplayName string - The name of the topic the contact will see
- TopicName string - The name of the topic
aws.ses: TopicPreference
Represents the contact's preference for being opted-in to or opted-out of a topic.
Fields
- SubscriptionStatus SubscriptionStatus - The contact's subscription status to a topic which is either OPT_IN or OPT_OUT
- TopicName string - The name of the topic
Import
import ballerinax/aws.ses;
Metadata
Released date: almost 2 years ago
Version: 2.1.0
License: Apache-2.0
Compatibility
Platform: java11
Ballerina version: 2201.4.1
Pull count
Total: 2246
Current verison: 245
Weekly downloads
Keywords
Communication/Email
Cost/Freemium
Vendor/Amazon
Contributors