aws.dynamodb
Module aws.dynamodb
API
Declarations
Definitions
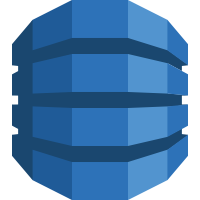
ballerinax/aws.dynamodb Ballerina library
Overview
The Ballerina AWS DynamoDB connector provides the capability to programatically handle AWS DynamoDB related operations.
This module supports Amazon DynamoDB REST API 20120810.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create an AWS account
- Obtain tokens
Quickstart
To use the AWS DynamoDB connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
Import the ballerinax/aws.dynamodb
module into the Ballerina project.
import ballerinax/aws.dynamodb;
Step 2: Create a new connector instance
Create an dynamodb:ConnectionConfig
with the tokens obtained, and initialize the connector with it.
dynamodb:ConnectionConfig amazonDynamodbConfig = { awsCredentials: { accessKeyId: "<ACCESS_KEY_ID>", secretAccessKey: "<SECRET_ACCESS_KEY>" }, region: "<REGION>" }; dynamodb:Client amazonDynamoDBClient = check new(amazonDynamodbConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to create table in DynamoDB using the connector.public function main() returns error? { dynamodb:TableCreateRequest payload = { AttributeDefinitions: [ { AttributeName: "ForumName", AttributeType: "S" }, { AttributeName: "Subject", AttributeType: "S" }, { AttributeName: "LastPostDateTime", AttributeType: "S" } ], TableName: mainTable, KeySchema: [ { AttributeName: "ForumName", KeyType: HASH }, { AttributeName: "Subject", KeyType: RANGE } ], LocalSecondaryIndexes: [ { IndexName: "LastPostIndex", KeySchema: [ { AttributeName: "ForumName", KeyType: HASH }, { AttributeName: "LastPostDateTime", KeyType: RANGE } ], Projection: { ProjectionType: KEYS_ONLY } } ], ProvisionedThroughput: { ReadCapacityUnits: 5, WriteCapacityUnits: 5 }, Tags: [ { Key: "Owner", Value: "BlueTeam" } ] }; dynamodb:TableCreateResponse createTablesResult = check dynamoDBClient->createTable(payload); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
aws.dynamodb: Client
The Ballerina AWS DynamoDB connector provides the capability to access AWS Simple Email Service related operations. This connector lets you to to send email messages to your customers.
Constructor
Initializes the connector. During initialization you have to pass access key id, secret access key, and region. Create an AWS account and obtain tokens following this guide.
init (ConnectionConfig config)
- config ConnectionConfig -
createTable
function createTable(TableCreateInput tableCreationInput) returns TableDescription|error
Creates a table. The CreateTable operation adds a new table to your account. In an AWS account, table names must be unique within each Region. That is, you can have two tables with same name if you create the tables in different Regions.
Parameters
- tableCreationInput TableCreateInput - The request payload to create a table
Return Type
- TableDescription|error - If success, dynamodb:TableDescription record, else an error
deleteTable
function deleteTable(string tableName) returns TableDescription|error
Deletes a table.
Parameters
- tableName string - The name of the table to delete
Return Type
- TableDescription|error - If success, dynamodb:TableDescription record, else an error
describeTable
function describeTable(string tableName) returns TableDescription|error
Describes a table.
Parameters
- tableName string - The name of the table to delete
Return Type
- TableDescription|error - If success, dynamodb:TableDescription record, else an error
listTables
Lists all tables.
updateTable
function updateTable(TableUpdateInput tableUpdateInput) returns TableDescription|error
Updates a table.
Parameters
- tableUpdateInput TableUpdateInput - The request payload to update a table
Return Type
- TableDescription|error - If success, dynamodb:TableDescription record, else an error
createItem
function createItem(ItemCreateInput itemCreateInput) returns ItemDescription|error
Creates a new item, or replaces an old item with a new item. If an item that has the same primary key as the new item already exists in the specified table, the new item completely replaces the existing item. You can perform a conditional put operation (add a new item if one with the specified primary key doesn't exist), or replace an existing item if it has certain attribute values.
Parameters
- itemCreateInput ItemCreateInput - The request payload to create an item
Return Type
- ItemDescription|error - If success, dynamodb:ItemDescription record, else an error
getItem
function getItem(ItemGetInput itemGetInput) returns ItemGetOutput|error
Gets an item.
Parameters
- itemGetInput ItemGetInput - The request payload to get an item
Return Type
- ItemGetOutput|error - If success, dynamodb:GetItemOutput record, else an error
deleteItem
function deleteItem(ItemDeleteInput itemDeleteInput) returns ItemDescription|error
Deletes an item.
Parameters
- itemDeleteInput ItemDeleteInput - The request payload to delete an item
Return Type
- ItemDescription|error - If success, dynamodb:ItemDescription record, else an error
updateItem
function updateItem(ItemUpdateInput itemUpdateInput) returns ItemDescription|error
Updates an item
Parameters
- itemUpdateInput ItemUpdateInput - The request payload to update an item
Return Type
- ItemDescription|error - If success, dynamodb:ItemDescription record, else an error
query
function query(QueryInput queryInput) returns stream<QueryOutput, error?>|error
Returns all items with a particular partition key value. You must provide the name of the partition key attribute and a single value for that attribute. Optionally, you can provide a sort key attribute and use a comparison operator to refine the search results.
Parameters
- queryInput QueryInput - The request payload to query
Return Type
- stream<QueryOutput, error?>|error - If success, streamdynamodb:QueryOutput,error?, else an error
scan
function scan(ScanInput scanInput) returns stream<ScanOutput, error?>|error
Returns one or more items and item attributes by accessing every item in a table or a secondary index.
Parameters
- scanInput ScanInput - The request payload to scan
Return Type
- stream<ScanOutput, error?>|error - If success, streamdynamodb:ScanOutput,error?, else an error
getBatchItems
function getBatchItems(BatchItemGetInput batchItemGetInput) returns stream<BatchItem, error?>|error
Returns the attributes of one or more items from one or more tables. You identify requested items by primary key.
Parameters
- batchItemGetInput BatchItemGetInput - The request payload to get items as batch
Return Type
writeBatchItems
function writeBatchItems(BatchItemInsertInput batchItemInsertInput) returns BatchItemInsertOutput|error
Puts or deletes multiple items in one or more tables.
Parameters
- batchItemInsertInput BatchItemInsertInput - The request payload to write items as batch
Return Type
- BatchItemInsertOutput|error - If success, dynamodb:BatchItemInsertOutput record, else an error
describeLimits
function describeLimits() returns LimitDescription|error
Returns the current provisioned-capacity quotas for your AWS account in a Region, both for the Region as a whole and for any one DynamoDB table that you create there.
Return Type
- LimitDescription|error - If success, dynamodb:LimitDescription record, else an error
createBackup
function createBackup(BackupCreateInput backupCreateInput) returns BackupDetails|error
Creates a back up from the given table
Parameters
- backupCreateInput BackupCreateInput - The request payload to backup the table
Return Type
- BackupDetails|error - If success, dynamodb:BackupDetails record, else an error
deleteBackup
function deleteBackup(string backupArn) returns BackupDescription|error
Deletes an existing backup of a table.
Parameters
- backupArn string - The backupArn of the table that needs to be deleted
Return Type
- BackupDescription|error - If success, dynamodb:BackupDescription record, else an error
getTTL
function getTTL(string tableName) returns TTLDescription|error
The description of the Time to Live (TTL) status on the specified table.
Parameters
- tableName string - Table name
Return Type
- TTLDescription|error - If success, dynamodb:TTLDescription record, else an error
Enums
aws.dynamodb: Action
Represents the actions that can be performed on a DynamoDB table.
Members
aws.dynamodb: AttributeType
Represents the attribute types supported by Amazon DynamoDB.
Members
aws.dynamodb: BillingMode
Represents the billing mode of a DynamoDB table.
Members
aws.dynamodb: ComparisonOperator
Represents the comparison operators used in DynamoDB queries.
Members
aws.dynamodb: ConditionalOperator
Represents the conditional operator used in DynamoDB operations.
Members
aws.dynamodb: eventName
Represents the event name.
Members
aws.dynamodb: IndexStatus
Represents the status of an index.
Members
aws.dynamodb: KeyType
Represents the key type of an attribute in a DynamoDB table.
This enum is used in the KeySchema
of a TableSchema
to specify the type of the key attribute.
Members
aws.dynamodb: ProjectionType
Represents the types of projections that can be used in a DynamoDB query.
Members
aws.dynamodb: ReplicaStatus
Represents the status of a replica in Amazon DynamoDB.
Members
aws.dynamodb: ReturnConsumedCapacity
Represents the return consumed capacity mode for DynamoDB operations.
Members
aws.dynamodb: ReturnItemCollectionMetrics
Represents the enum for the return item collection metrics in AWS DynamoDB.
Members
aws.dynamodb: ReturnValues
Represents the possible return values of a DynamoDB operation.
Members
aws.dynamodb: Select
Represents the SELECT operation in DynamoDB.
Members
aws.dynamodb: ShardIteratorType
Represents the type of shard iterator to be used when reading data from a DynamoDB stream.
Members
aws.dynamodb: SSEType
Represents the types of server-side encryption supported by DynamoDB.
Members
aws.dynamodb: Status
Represents the status of a DynamoDB operation.
Members
aws.dynamodb: StreamStatus
Represents the status of a stream.
Members
aws.dynamodb: StreamViewType
Represents the stream view type of a DynamoDB table.
Members
aws.dynamodb: TableStatus
Represents the status of an AWS DynamoDB table.
Members
aws.dynamodb: TimeToLiveStatus
Represents the status of the time to live (TTL) attribute of an item in Amazon DynamoDB.
Members
Records
aws.dynamodb: ArchivalSummary
Contains details of a table archival operation.
Fields
- ArchivalBackupArn? string? - The Amazon Resource Name (ARN) of the backup the table was archived to, when applicable in the archival reason. If you wish to restore this backup to the same table name, you will need to delete the original table
- ArchivalDateTime? int? - The date and time when table archival was initiated by DynamoDB, in UNIX epoch time format
- ArchivalReason? string? - The reason DynamoDB archived the table. Currently, the only possible value is: INACCESSIBLE_ENCRYPTION_CREDENTIALS - The table was archived due to the table's AWS KMS key being inaccessible for more than seven days. An On-Demand backup was created at the archival time
aws.dynamodb: AttributeDefinition
Represents an attribute for describing the key schema for the table and indexes.
Fields
- AttributeName string - A name for the attribute
- AttributeType AttributeType - The data type for the attribute, where: S - the attribute is of type String, N - the attribute is of type Number, B - the attribute is of type Binary
aws.dynamodb: AttributeValue
Represents the data for an attribute. Each attribute value is described as a name-value pair. The name is the data type, and the value is the data itself.
Fields
- B? string? - An attribute of type Binary
- BOOL? boolean? - An attribute of type Boolean
- BS? string[]? - An attribute of type Binary Set
- L? AttributeValue[]? - An attribute of type List
- M? map<AttributeValue>? - An attribute of type Map
- N? string? - An attribute of type Number
- NS? string[]? - An attribute of type Number Set
- NULL? boolean? - An attribute of type Null
- S? string? - An attribute of type String
- SS? string[]? - An attribute of type String Set
aws.dynamodb: AttributeValueUpdate
For the UpdateItem operation, represents the attributes to be modified, the action to perform on each, and the new value for each.
Fields
- Action? Action? - Specifies how to perform the update. Valid values are PUT (default), DELETE, and ADD. The behavior depends on whether the specified primary key already exists in the table
- Value? AttributeValue? - Represents the data for an attribute
aws.dynamodb: AwsCredentials
Represents AWS credentials.
Fields
- accessKeyId string - AWS access key
- secretAccessKey string - AWS secret key
aws.dynamodb: AwsTemporaryCredentials
Represents AWS temporary credentials.
Fields
- accessKeyId string - AWS access key
- secretAccessKey string - AWS secret key
- securityToken string - AWS secret token
aws.dynamodb: BackupCreateInput
Represents an request to perform a CreateBackup operation on a table.
Fields
- BackupName string - Name for the backup
- TableName string - Name of the table
aws.dynamodb: BackupDescription
Represents a response after performing a CreateBackup operation on a table.
Fields
- BackupDetails? BackupDetails - Contains the details of the backup created for the table
- SourceTableDetails? SourceTableDetails - Contains the details of the table when the backup was created
- SourceTableFeatureDetails? SourceTableFeatureDetails - Contains the details of the features enabled on the table when the backup was created
aws.dynamodb: BackupDetails
Contains the details of the backup created for the table.
Fields
- BackupArn string - ARN associated with the backup
- BackupCreationDateTime decimal - Time at which the backup was created. This is the request time of the backup
- BackupName string - Name of the requested backup
- BackupStatus string - Backup can be in one of the following states: CREATING, ACTIVE, DELETED
- BackupType string - BackupType. One of USER, SYSTEM, AWS_BACKUP
- BackupExpiryDateTime? decimal - Time at which the automatic on-demand backup created by DynamoDB will expire. This SYSTEM on-demand backup expires automatically 35 days after its creation
- BackupSizeBytes? decimal - Size of the backup in bytes. DynamoDB updates this value approximately every six hours. Recent changes might not be reflected in this value
aws.dynamodb: BatchItem
Represents ItemByBatchGet
Fields
- ConsumedCapacity? ConsumedCapacity? - The read capacity units consumed by the entire BatchGetItem operation
- TableName? string? - The name of the table containing the requested item
- Item? map<AttributeValue>? - The requested item
aws.dynamodb: BatchItemGetInput
Represents the request payload for a BatchGetItem
operation.
Fields
- RequestItems map<KeysAndAttributes> - A map of one or more table names and, for each table, a map that describes one or more items to retrieve from that table
- ReturnConsumedCapacity? ReturnConsumedCapacity - Determines the level of detail about provisioned throughput consumption that is returned in the response. Valid Values: INDEXES | TOTAL | NONE
aws.dynamodb: BatchItemInsertInput
Represents the request payload BatchWriteItem
operation.
Fields
- RequestItems map<WriteRequest[]> - A map of one or more table names and, for each table, a list of operations to be performed (DeleteRequest or PutRequest)
- ReturnConsumedCapacity? ReturnConsumedCapacity - Determines the level of detail about provisioned throughput consumption that is returned in the response. Valid Values: INDEXES | TOTAL | NONE
- ReturnItemCollectionMetrics? ReturnItemCollectionMetrics - Determines whether item collection metrics are returned. Valid Values: SIZE | NONE
aws.dynamodb: BatchItemInsertOutput
Represents the response after WriteBatchItem
operation.
Fields
- ConsumedCapacity? ConsumedCapacity[]? - The capacity units consumed by the entire
BatchWriteItem
operation
- ItemCollectionMetrics? map<ItemCollectionMetrics[]>? - A list of tables that were processed by
BatchWriteItem
and, for each table, information about any item collections that were affected by individualDeleteItem
orPutItem
operations.
- UnprocessedItems? map<WriteRequest[]>? - A map of tables and requests against those tables that were not processed. The
UnprocessedItems
value is in the same form asRequestItems
, so you can provide this value directly to a subsequentBatchGetItem
operation.
aws.dynamodb: BillingModeSummary
Contains the details for the read/write capacity mode.
Fields
- BillingMode? BillingMode? - Controls how you are charged for read and write throughput and how you manage capacity. This setting can be changed later. PROVISIONED - Sets the read/write capacity mode to PROVISIONED. We recommend using PROVISIONED for predictable workloads. PAY_PER_REQUEST - Sets the read/write capacity mode to PAY_PER_REQUEST. We recommend using PAY_PER_REQUEST for unpredictable workloads.
- LastUpdateToPayPerRequestDateTime? int? - Represents the time when PAY_PER_REQUEST was last set as the read/write capacity mode
aws.dynamodb: Capacity
Represents the amount of provisioned throughput capacity consumed on a table or an index.
Fields
- CapacityUnits? float? - The total number of capacity units consumed on a table or an index
- ReadCapacityUnits? float? - The total number of read capacity units consumed on a table or an index
- WriteCapacityUnits? float? - The total number of write capacity units consumed on a table or an index
aws.dynamodb: Condition
Represents the selection criteria for a Query or Scan operation. For a Query operation, Condition is used for specifying the KeyConditions to use when querying table or an index. For KeyConditions, only the following comparison operators are supported: EQ | LE | LT | GE | GT | BEGINS_WITH | BETWEEN. For a Scan operation, Condition is used in a ScanFilter, which evaluates the scan results and returns only the desired values
Fields
- ComparisonOperator ComparisonOperator - A comparator for evaluating attributes. Valid Values: EQ | NE | IN | LE | LT | GE | GT | BETWEEN | NOT_NULL | NULL | CONTAINS | NOT_CONTAINS | BEGINS_WITH
- AttributeValueList? AttributeValue[]? - One or more values to evaluate against the supplied attribute. The number of values in the list depends on the ComparisonOperator being used
aws.dynamodb: ConnectionConfig
Represents the AWS DynamoDB Connector configurations.
Fields
- Fields Included from *ConnectionConfig
- auth AuthConfig
- httpVersion HttpVersion
- http1Settings ClientHttp1Settings
- http2Settings ClientHttp2Settings
- timeout decimal
- forwarded string
- poolConfig PoolConfiguration
- cache CacheConfig
- compression Compression
- circuitBreaker CircuitBreakerConfig
- retryConfig RetryConfig
- responseLimits ResponseLimitConfigs
- secureSocket ClientSecureSocket
- proxy ProxyConfig
- validation boolean
- anydata...
- auth? never -
- awsCredentials AwsCredentials|AwsTemporaryCredentials - AWS credentials
- region string - AWS Region
aws.dynamodb: ConsumedCapacity
The capacity units consumed by an operation. The data returned includes the total provisioned throughput consumed, along with statistics for the table and any indexes involved in the operation.
Fields
- CapacityUnits? float? - The total number of capacity units consumed by the operation
- ReadCapacityUnits? float? - The total number of read capacity units consumed by the operation
- Table? Capacity? - The amount of throughput consumed on the table affected by the operation
- TableName? string? - The name of the table that was affected by the operation
- WriteCapacityUnits? float? - The total number of write capacity units consumed by the operation
aws.dynamodb: CreateGlobalSecondaryIndexAction
Represents a new global secondary index to be added to an existing table.
Fields
- IndexName string - The name of the global secondary index to be created
- KeySchema KeySchemaElement[] - The key schema for the global secondary index
- Projection Projection - Represents attributes that are copied (projected) from the table into an index
- ProvisionedThroughput? ProvisionedThroughput? - Represents the provisioned throughput settings for the specified global secondary index
aws.dynamodb: CreateReplicationGroupMemberAction
Represents a replica to be created.
Fields
- RegionName string - The Region where the new replica will be created
- GlobalSecondaryIndexes? ReplicaGlobalSecondaryIndex[]? - Replica-specific global secondary index settings
- KMSMasterKeyId? string? - The AWS KMS customer master key (CMK) that should be used for AWS KMS encryption in the new replica
- ProvisionedThroughputOverride? ProvisionedThroughputOverride? - Replica-specific provisioned throughput. If not specified, uses the source table's provisioned throughput settings
aws.dynamodb: DeleteGlobalSecondaryIndexAction
Represents a global secondary index to be deleted from an existing table.
Fields
- IndexName string - The name of the global secondary index to be deleted
aws.dynamodb: DeleteReplicationGroupMemberAction
Represents a replica to be deleted.
Fields
- RegionName string - The Region where the replica exists
aws.dynamodb: DeleteRequest
Represents a request to perform a DeleteItem operation on an item.
Fields
- Key map<AttributeValue> - A map of attribute name to attribute values, representing the primary key of the item to delete. All of the table's primary key attributes must be specified, and their data types must match those of the table's key schema
aws.dynamodb: ExpectedAttributeValue
Represents a condition to be compared with an attribute value
Fields
- AttributeValueList? AttributeValue[]? - One or more values to evaluate against the supplied attribute
- ComparisonOperator? ComparisonOperator? - A comparator for evaluating attributes in the AttributeValueList. Valid Values: EQ | NE | IN | LE | LT | GE | GT | BETWEEN | NOT_NULL | NULL | CONTAINS | NOT_CONTAINS | BEGINS_WITH
- Exists? boolean? - Causes DynamoDB to evaluate the value before attempting a conditional operation
- Value? AttributeValue? - Represents the data for the expected attribute
aws.dynamodb: GlobalSecondaryIndex
Represents the properties of a global secondary index.
Fields
- Fields Included from *LocalSecondaryIndex
- IndexName string
- KeySchema KeySchemaElement[]
- Projection Projection
- anydata...
- ProvisionedThroughput ProvisionedThroughput - Represents the provisioned throughput settings for the specified global secondary index
aws.dynamodb: GlobalSecondaryIndexDescription
Represents the properties of a global secondary index.
Fields
- Fields Included from *LocalSecondaryIndexDescription
- IndexArn string|()
- IndexName string|()
- IndexSizeBytes int|()
- ItemCount int|()
- KeySchema KeySchemaElement[]|()
- Projection Projection|()
- anydata...
- Backfilling? boolean? - Indicates whether the index is currently backfilling
- IndexStatus? IndexStatus? - The current state of the global secondary index: CREATING - The index is being created, UPDATING - The index is being updated, DELETING - The index is being deleted, ACTIVE - The index is ready for use
- ProvisionedThroughput? ProvisionedThroughput? - Represents the provisioned throughput settings for the specified global secondary index
aws.dynamodb: GlobalSecondaryIndexInfo
Represents the properties of a global secondary index for the table when the backup was created.
Fields
- IndexName? string - The name of the global secondary index
- KeySchema? KeySchemaElement[] - The complete key schema for a global secondary index, which consists of one or more pairs of attribute names and key types: HASH - partition key and RANGE - sort key
- Projection? Projection - Represents attributes that are copied (projected) from the table into the global secondary index
- ProvisionedThroughput? ProvisionedThroughput - Represents the provisioned throughput settings for the specified global secondary index
aws.dynamodb: GlobalSecondaryIndexUpdate
Represents one of the following: A new global secondary index to be added to an existing table, New provisioned throughput parameters for an existing global secondary index, An existing global secondary index to be removed from an existing table.
Fields
- Create? CreateGlobalSecondaryIndexAction? - The parameters required for creating a global secondary index on an existing table
- Delete? DeleteGlobalSecondaryIndexAction? - The name of an existing global secondary index to be removed
- Update? UpdateGlobalSecondaryIndexAction? - The name of an existing global secondary index, along with new provisioned throughput settings to be applied to that index
aws.dynamodb: ItemCollectionMetrics
Information about item collections, if any, that were affected by the operation.
Fields
- ItemCollectionKey? map<AttributeValue>? - The partition key value of the item collection. This value is the same as the partition key value of the item
- SizeEstimateRangeGB? float[]? - An estimate of item collection size, in gigabytes. This value is a two-element array containing a lower bound and an upper bound for the estimate. The estimate includes the size of all the items in the table, plus the size of all attributes projected into all of the local secondary indexes on that table. Use this estimate to measure whether a local secondary index is approaching its size limit
aws.dynamodb: ItemCreateInput
Represents the request payload to put item.
Fields
- Item map<AttributeValue> - A map of attribute name/value pairs, one for each attribute. Only the primary key attributes are required; you can optionally provide other attribute name-value pairs for the item
- TableName string - The name of the table to contain the item
- ConditionalOperator? ConditionalOperator? - This is a legacy parameter. Use ConditionExpression instead. Valid Values: AND | OR
- ConditionExpression? string? - A condition that must be satisfied in order for a conditional PutItem operation to succeed
- Expected? map<ExpectedAttributeValue>? - This is a legacy parameter. Use ConditionExpression instead
- ExpressionAttributeValues? map<AttributeValue>? - One or more values that can be substituted in an expression
- ReturnConsumedCapacity? ReturnConsumedCapacity? - Determines the level of detail about provisioned throughput consumption that is returned in the response. Valid Values: INDEXES | TOTAL | NONE
- ReturnItemCollectionMetrics? ReturnItemCollectionMetrics? - Determines whether item collection metrics are returned. Valid Values: SIZE | NONE
- ReturnValues? ReturnValues? - Use ReturnValues if you want to get the item attributes as they appeared before they were updated with the PutItem request. For PutItem, the valid values are: NONE | ALL_OLD
aws.dynamodb: ItemDeleteInput
Represents the request payload to delete an item.
Fields
- Key map<AttributeValue> - A map of attribute names to AttributeValue objects, representing the primary key of the item to delete
- TableName string - The name of the table from which to delete the item
- ConditionalOperator? ConditionalOperator - This is a legacy parameter. Use ConditionExpression instead. Valid Values: AND | OR
- ConditionExpression? string - A condition that must be satisfied in order for a conditional DeleteItem to succeed
- Expected? map<ExpectedAttributeValue> - This is a legacy parameter. Use ConditionExpression instead
- ExpressionAttributeValues? map<AttributeValue> - One or more values that can be substituted in an expression
- ReturnConsumedCapacity? ReturnConsumedCapacity - Determines the level of detail about provisioned throughput consumption that is returned in the response. Valid Values: INDEXES | TOTAL | NONE
- ReturnItemCollectionMetrics? ReturnItemCollectionMetrics - Determines whether item collection metrics are returned. Valid Values: SIZE | NONE
- ReturnValues? ReturnValues - Use ReturnValues if you want to get the item attributes as they appeared before they were deleted. For DeleteItem, the valid values are: NONE | ALL_OLD
aws.dynamodb: ItemDescription
Represents the response of PutItem operation.
Fields
- Attributes? map<AttributeValue>? - The attribute values as they appeared before the PutItem operation, but only if ReturnValues is specified as ALL_OLD in the request. Each element consists of an attribute name and an attribute value
- ConsumedCapacity? ConsumedCapacity? - The capacity units consumed by the PutItem operation
- ItemCollectionMetrics? ItemCollectionMetrics? - Information about item collections, if any, that were affected by the PutItem operation
aws.dynamodb: ItemGetInput
Represents the request payload to get an Item.
Fields
- Key map<AttributeValue> - A map of attribute names to AttributeValue objects, representing the primary key of the item to retrieve
- TableName string - The name of the table containing the requested item
- AttributesToGet? string[] - This is a legacy parameter. Use ProjectionExpression instead
- ConsistentRead? boolean - Determines the read consistency model: If set to true, then the operation uses strongly consistent reads; otherwise, the operation uses eventually consistent reads
- ProjectionExpression? string - A string that identifies one or more attributes to retrieve from the table
- ReturnConsumedCapacity? ReturnConsumedCapacity - Determines the level of detail about provisioned throughput consumption that is returned in the response. Valid Values: INDEXES | TOTAL | NONE
aws.dynamodb: ItemGetOutput
Represents the response of GetItem
operation.
Fields
- ConsumedCapacity? ConsumedCapacity? - The capacity units consumed by the GetItem operation
- Item? map<AttributeValue>? - A map of attribute names to AttributeValue objects, as specified by ProjectionExpression
aws.dynamodb: ItemUpdateInput
Represents the request payload to update an item.
Fields
- Key map<AttributeValue> - The primary key of the item to be updated. Each element consists of an attribute name and a value for that attribute
- TableName string - The name of the table containing the item to update
- AttributeUpdates? map<AttributeValueUpdate> - This is a legacy parameter. Use UpdateExpression instead
- ConditionalOperator? ConditionalOperator - This is a legacy parameter. Use ConditionExpression instead. Valid Values: AND | OR
- ConditionExpression? string - This is a legacy parameter. Use ConditionExpression instead
- Expected? map<ExpectedAttributeValue> - This is a legacy parameter. Use ConditionExpression instead
- ExpressionAttributeValues? map<AttributeValue> - One or more values that can be substituted in an expression
- ReturnConsumedCapacity? ReturnConsumedCapacity - Determines the level of detail about provisioned throughput consumption that is returned in the response. Valid Values: INDEXES | TOTAL | NONE
- ReturnItemCollectionMetrics? ReturnItemCollectionMetrics - Determines whether item collection metrics are returned. Valid Values: SIZE | NONE
- ReturnValues? ReturnValues - Use ReturnValues if you want to get the item attributes as they appear before or after they are updated. Valid Values: NONE | ALL_OLD | UPDATED_OLD | ALL_NEW | UPDATED_NEW
- UpdateExpression? string - An expression that defines one or more attributes to be updated, the action to be performed on them, and new values for them
aws.dynamodb: KeysAndAttributes
Represents a set of primary keys and, for each key, the attributes to retrieve from the table.
Fields
- Keys map<AttributeValue>[] - The primary key attribute values that define the items and the attributes associated with the items
- AttributesToGet? string[]? - This is a legacy parameter. Use ProjectionExpression instead
- ConsistentRead? boolean? - The consistency of a read operation. If set to true, then a strongly consistent read is used; otherwise, an eventually consistent read is used
- ProjectionExpression? string? - A string that identifies one or more attributes to retrieve from the table
aws.dynamodb: KeySchemaElement
Represents a single element of a key schema. A key schema specifies the attributes that make up the primary key of a table, or the key attributes of an index.
Fields
- AttributeName string - The name of a key attribute
- KeyType KeyType - The role that this key attribute will assume: HASH - partition key, RANGE - sort key
aws.dynamodb: LimitDescription
Describes the current provisioned-capacity quotas for your AWS account in a Region, both for the Region as a whole and for any one DynamoDB table that you create there.
Fields
- AccountMaxReadCapacityUnits? int? - The maximum total read capacity units that your account allows you to provision across all of your tables in this Region
- AccountMaxWriteCapacityUnits? int? - The maximum total write capacity units that your account allows you to provision across all of your tables in this Region
- TableMaxReadCapacityUnits? int? - The maximum read capacity units that your account allows you to provision for a new table that you are creating in this Region, including the read capacity units
- TableMaxWriteCapacityUnits? int? - The maximum write capacity units that your account allows you to provision for a new table that you are creating in this Region, including the write capacity units provisioned for its global secondary indexes (GSIs).
aws.dynamodb: LocalSecondaryIndex
Represents the properties of a local secondary index.
Fields
- IndexName string - The name of the local secondary index. The name must be unique among all other indexes on this table
- KeySchema KeySchemaElement[] - The complete key schema for the local secondary index, consisting of one or more pairs of attribute names and key types: HASH - partition key, RANGE - sort key
- Projection Projection - Represents attributes that are copied (projected) from the table into the local secondary index. These are in addition to the primary key attributes and index key attributes, which are automatically projected
aws.dynamodb: LocalSecondaryIndexDescription
Represents the properties of a local secondary index.
Fields
- IndexArn? string? - The Amazon Resource Name (ARN) that uniquely identifies the index
- IndexName? string? - Represents the name of the local secondary index
- IndexSizeBytes? int? - The total size of the specified index, in bytes. DynamoDB updates this value approximately every six hours. Recent changes might not be reflected in this value
- ItemCount? int? - The number of items in the specified index. DynamoDB updates this value approximately every six hours. Recent changes might not be reflected in this value
- KeySchema? KeySchemaElement[]? - The complete key schema for the local secondary index, consisting of one or more pairs of attribute names and key types: HASH - partition key, RANGE - sort key
- Projection? Projection? - Represents attributes that are copied (projected) from the table into the global secondary index. These are in addition to the primary key attributes and index key attributes, which are automatically projected
aws.dynamodb: LocalSecondaryIndexInfo
Represents the properties of a local secondary index for the table when the backup was created.
Fields
- IndexName? string - The name of the global secondary index
- KeySchema? KeySchemaElement[] - The complete key schema for a global secondary index, which consists of one or more pairs of attribute names and key types: HASH - partition key and RANGE - sort key
- Projection? Projection - Represents attributes that are copied (projected) from the table into the global secondary index
aws.dynamodb: Projection
Represents attributes that are copied (projected) from the table into an index. These are in addition to the primary key attributes and index key attributes, which are automatically projected.
Fields
- NonKeyAttributes? string[]? - Represents the non-key attribute names which will be projected into the index
- ProjectionType? ProjectionType? - The set of attributes that are projected into the index: KEYS_ONLY - Only the index and primary keys are projected into the index, INCLUDE - In addition to the attributes described in KEYS_ONLY, the secondary index will include other non-key attributes that you specify, ALL - All of the table attributes are projected into the index
aws.dynamodb: ProvisionedThroughput
Represents the provisioned throughput settings for a specified table or index. The settings can be modified using the UpdateTable operation.
Fields
- ReadCapacityUnits int - The maximum number of strongly consistent reads consumed per second before DynamoDB returns a
ThrottlingException
- WriteCapacityUnits int - The maximum number of writes consumed per second before DynamoDB returns a
ThrottlingException
aws.dynamodb: ProvisionedThroughputDescription
Represents the provisioned throughput settings for the table, consisting of read and write capacity units, along with data about increases and decreases.
Fields
- LastDecreaseDateTime? int? - The date and time of the last provisioned throughput decrease for this table
- LastIncreaseDateTime? int? - The date and time of the last provisioned throughput increase for this table
- NumberOfDecreasesToday? int? - The number of provisioned throughput decreases for this table during this UTC calendar day
- ReadCapacityUnits? int? - The maximum number of strongly consistent reads consumed per second before DynamoDB returns a
ThrottlingException
- WriteCapacityUnits? int? - The maximum number of writes consumed per second before DynamoDB returns a
ThrottlingException
aws.dynamodb: ProvisionedThroughputOverride
Replica-specific provisioned throughput settings. If not specified, uses the source table's provisioned throughput settings.
Fields
- ReadCapacityUnits? int? - Replica-specific read capacity units. If not specified, uses the source table's read capacity settings
aws.dynamodb: PutRequest
Represents a request to perform a PutItem operation on an item.
Fields
- Item map<AttributeValue> - A map of attribute name to attribute values, representing the primary key of an item to be processed by
PutItem
. All of the table's primary key attributes must be specified, and their data types must match those of the table's key schema. If any attributes are present in the item that are part of an index key schema for the table, their types must match the index key schema
aws.dynamodb: QueryInput
Represents the request payload for Query
Operation.
Fields
- TableName string - The name of the table containing the requested items
- AttributesToGet? string[] - This is a legacy parameter. Use ProjectionExpression instead
- ConditionalOperator? ConditionalOperator - This is a legacy parameter. Use FilterExpression instead. Valid Values: AND | OR
- ConsistentRead? boolean - Determines the read consistency model: If set to true, then the operation uses strongly consistent reads; otherwise, the operation uses eventually consistent reads
- ExclusiveStartKey? map<AttributeValue>? - The primary key of the first item that this operation will evaluate
- ExpressionAttributeValues? map<AttributeValue> - One or more values that can be substituted in an expression
- FilterExpression? string - A string that contains conditions that DynamoDB applies after the Query operation, but before the data is returned to you. Items that do not satisfy the FilterExpression criteria are not returned
- IndexName? string - The name of an index to query. This index can be any local secondary index or global secondary index on the table. Note that if you use the IndexName parameter, you must also provide TableName.
- KeyConditionExpression? string - The condition that specifies the key values for items to be retrieved by the Query action
- Limit? int - The maximum number of items to evaluate (not necessarily the number of matching items)
- ProjectionExpression? string - A string that identifies one or more attributes to retrieve from the table
- ReturnConsumedCapacity? ReturnConsumedCapacity - Determines the level of detail about provisioned throughput consumption that is returned in the response. Valid Values: INDEXES | TOTAL | NONE
- ScanIndexForward? boolean - Specifies the order for index traversal: If true (default), the traversal is performed in ascending order; if false, the traversal is performed in descending order
- Select? Select - The attributes to be returned in the result. Valid Values: ALL_ATTRIBUTES | ALL_PROJECTED_ATTRIBUTES | SPECIFIC_ATTRIBUTES | COUNT
aws.dynamodb: QueryOutput
Represents the response of Query operation.
Fields
- ConsumedCapacity? ConsumedCapacity? - The capacity units consumed by the Query operation
- Item? map<AttributeValue>? - An Item that match the query criteria. It consists of an attribute name and the value for that attribute
aws.dynamodb: ReplicaDescription
Contains the details of the replica.
Fields
- GlobalSecondaryIndexes? ReplicaGlobalSecondaryIndexDescription[]? - Replica-specific global secondary index settings
- KMSMasterKeyArn? string? - The AWS KMS customer master key (CMK) of the replica that will be used for AWS KMS encryption
- ProvisionedThroughputOverride? ProvisionedThroughputOverride? - Replica-specific provisioned throughput. If not described, uses the source table's provisioned throughput settings
- RegionName? string? - The name of the Region
- ReplicaInaccessibleDateTime? int? - The time at which the replica was first detected as inaccessible. To determine cause of inaccessibility check the ReplicaStatus property
- ReplicaStatus? ReplicaStatus? - The current state of the replica. Valid Values: CREATING | CREATION_FAILED | UPDATING | DELETING | ACTIVE | REGION_DISABLED | INACCESSIBLE_ENCRYPTION_CREDENTIALS
- ReplicaStatusDescription? string? - Detailed information about the replica status
- ReplicaStatusPercentProgress? string? - Specifies the progress of a Create, Update, or Delete action on the replica as a percentage
aws.dynamodb: ReplicaGlobalSecondaryIndex
Represents the properties of a replica global secondary index.
Fields
- IndexName string - The name of the global secondary index
- ProvisionedThroughputOverride? ProvisionedThroughputOverride? - Replica table GSI-specific provisioned throughput. If not specified, uses the source table GSI's read capacity settings
aws.dynamodb: ReplicaGlobalSecondaryIndexDescription
Represents the properties of a replica global secondary index.
Fields
- IndexName? string? - The name of the global secondary index
- ProvisionedThroughputOverride? ProvisionedThroughputOverride? - If not described, uses the source table GSI's read capacity settings
aws.dynamodb: ReplicationGroupUpdate
Represents one of the following: A new replica to be added to an existing regional table or global table. This request invokes the CreateTableReplica action in the destination Region, New parameters for an existing replica. This request invokes the UpdateTable action in the destination Region. An existing replica to be deleted. The request invokes the DeleteTableReplica action in the destination Region, deleting the replica and all if its items in the destination Region.
Fields
- Create? CreateReplicationGroupMemberAction? - The parameters required for creating a replica for the table
- Delete? DeleteReplicationGroupMemberAction? - The parameters required for deleting a replica for the table
- Update? UpdateReplicationGroupMemberAction? - The parameters required for updating a replica for the table
aws.dynamodb: RestoreSummary
Contains details for the restore.
Fields
- RestoreDateTime int - Point in time or source backup time
- RestoreInProgress boolean - Indicates if a restore is in progress or not
- SourceBackupArn? string? - The Amazon Resource Name (ARN) of the backup from which the table was restored
- SourceTableArn? string? - The ARN of the source table of the backup that is being restored
aws.dynamodb: ScanInput
Represents the request payload for Scan
Operation.
Fields
- TableName string - The name of the table containing the requested items; or, if you provide IndexName, the name of the table to which that index belongs
- AttributesToGet? string[] - This is a legacy parameter. Use ProjectionExpression instead
- ConditionalOperator? ConditionalOperator - This is a legacy parameter. Use FilterExpression instead. Valid Values: AND | OR
- ConsistentRead? boolean - A Boolean value that determines the read consistency model during the scan
- ExclusiveStartKey? map<AttributeValue>? - The primary key of the first item that this operation will evaluate. Use the value that was
returned for
LastEvaluatedKey
in the previous operation
- ExpressionAttributeValues? map<AttributeValue> - One or more values that can be substituted in an expression
- FilterExpression? string - A string that contains conditions that DynamoDB applies after the Scan operation, but before the data is returned to you. Items that do not satisfy the FilterExpression criteria are not returned
- IndexName? string - The name of a secondary index to scan. This index can be any local secondary index or global secondary index. Note that if you use the IndexName parameter, you must also provide TableName
- Limit? int - The maximum number of items to evaluate (not necessarily the number of matching items)
- ProjectionExpression? string - A string that identifies one or more attributes to retrieve from the specified table or index
- ReturnConsumedCapacity? ReturnConsumedCapacity - Determines the level of detail about provisioned throughput consumption that is returned in the response. Valid Values: INDEXES | TOTAL | NONE
- Segment? int - For a parallel Scan request, Segment identifies an individual segment to be scanned by an application worker
- Select? Select - The attributes to be returned in the result. Valid Values: ALL_ATTRIBUTES | ALL_PROJECTED_ATTRIBUTES | SPECIFIC_ATTRIBUTES |COUNT
- TotalSegments? int - For a parallel Scan request, TotalSegments represents the total number of segments into which the Scan operation will be divided
aws.dynamodb: ScanOutput
Reperesents the response of Scan operation.
Fields
- ConsumedCapacity? ConsumedCapacity? - The capacity units consumed by the Scan operation
- Item? map<AttributeValue>? - An Item that match the scan criteria. It consists of an attribute name and the value for that attribute
aws.dynamodb: SourceTableDetails
Contains the details of the table when the backup was created.
Fields
- KeySchema? KeySchemaElement[] - Schema of the table
- ProvisionedThroughput? ProvisionedThroughput - Read IOPs and Write IOPS on the table when the backup was created
- TableCreationDateTime? decimal - Time when the source table was created
- TableId? string - Unique identifier for the table for which the backup was created
- TableName? string - The name of the table for which the backup was created
- BillingMode? BillingMode - Controls how you are charged for read and write throughput and how you manage capacity
- ItemCount? float - Number of items in the table. Note that this is an approximate value
- TableArn? string - ARN of the table for which backup was created
- TableSizeBytes? float - Size of the table in bytes. Note that this is an approximate value
aws.dynamodb: SourceTableFeatureDetails
Contains the details of the features enabled on the table when the backup was created. For example, LSIs, GSIs, streams, TTL.
Fields
- GlobalSecondaryIndexInfo? GlobalSecondaryIndexInfo - Represents the GSI properties for the table when the backup was created. It includes the IndexName, KeySchema, Projection, and ProvisionedThroughput for the GSIs on the table at the time of backup
- LocalSecondaryIndexInfo? LocalSecondaryIndexInfo - Represents the LSI properties for the table when the backup was created. It includes the IndexName, KeySchema and Projection for the LSIs on the table at the time of backup
- SSEDescription? SSEDescription - The description of the server-side encryption status on the table when the backup was created
- StreamSpecification? StreamSpecification - Stream settings on the table when the backup was created
- TimeToLiveDescription? TTLDescription - Time to Live settings on the table when the backup was created
aws.dynamodb: SSEDescription
The description of the server-side encryption status on the specified table.
Fields
- InaccessibleEncryptionDateTime? int? - Indicates the time, in UNIX epoch date format, when DynamoDB detected that the table's AWS KMS key was inaccessible
- KMSMasterKeyArn? string? - The AWS KMS customer master key (CMK) ARN used for the AWS KMS encryption
- SSEType? SSEType? - Server-side encryption type. The only supported value is: KMS - Server-side encryption that uses AWS Key Management Service. The key is stored in your account and is managed by AWS KMS (AWS KMS charges apply)
- Status? Status? - Represents the current state of server-side encryption. The only supported values are: ENABLED - Server-side encryption is enabled, UPDATING - Server-side encryption is being updated
aws.dynamodb: SSESpecification
Represents the settings used to enable server-side encryption.
Fields
- Enabled? boolean? - Indicates whether server-side encryption is done using an AWS managed CMK or an AWS owned CMK. If enabled (true), server-side encryption type is set to KMS and an AWS managed CMK is used (AWS KMS charges apply). If disabled (false) or not specified, server-side encryption is set to AWS owned CMK
- KMSMasterKeyId? string? - The AWS KMS customer master key (CMK) that should be used for the AWS KMS encryption. To specify a
CMK, use its key ID, Amazon Resource Name (ARN), alias name, or alias ARN. Note that you should
only provide this parameter if the key is different from the default DynamoDB customer master key
alias/aws/dynamodb
- SSEType? SSEType? - Server-side encryption type. The only supported value is: KMS - Server-side encryption that uses AWS Key Management Service. The key is stored in your account and is managed by AWS KMS (AWS KMS charges apply)
aws.dynamodb: StreamSpecification
Represents the DynamoDB Streams configuration for a table in DynamoDB.
Fields
- StreamEnabled boolean - Indicates whether DynamoDB Streams is enabled (true) or disabled (false) on the table
- StreamViewType? StreamViewType? - When an item in the table is modified, StreamViewType determines what information is written to the stream for this table. Valid values for StreamViewType are: KEYS_ONLY - Only the key attributes of the modified item are written to the stream. NEW_IMAGE - The entire item, as it appears after it was modified, is written to the stream. OLD_IMAGE - The entire item, as it appeared before it was modified, is written to the stream. NEW_AND_OLD_IMAGES - Both the new and the old item images of the item are written to the stream
aws.dynamodb: TableCreateInput
Represents the request payload to create table.
Fields
- AttributeDefinitions AttributeDefinition[] - An array of attributes that describe the key schema for the table and indexes
- KeySchema KeySchemaElement[] - Specifies the attributes that make up the primary key for a table or an index. The attributes in KeySchema must also be defined in the AttributeDefinitions array
- TableName string - The name of the table to create
- BillingMode? BillingMode - Controls how you are charged for read and write throughput and how you manage capacity. Valid Values: PROVISIONED | PAY_PER_REQUEST
- GlobalSecondaryIndexes? GlobalSecondaryIndex[] - One or more global secondary indexes (the maximum is 20) to be created on the table
- LocalSecondaryIndexes? LocalSecondaryIndex[] - One or more local secondary indexes (the maximum is 5) to be created on the table
- ProvisionedThroughput? ProvisionedThroughput - Represents the provisioned throughput settings for a specified table or index
- SSESpecification? SSESpecification - Represents the settings used to enable server-side encryption
- StreamSpecification? StreamSpecification - The settings for DynamoDB Streams on the table
- Tags? Tag[] - A list of key-value pairs to label the table
aws.dynamodb: TableDescription
Represents the properties of a table.
Fields
- ArchivalSummary? ArchivalSummary? - Contains information about the table archive
- AttributeDefinitions? AttributeDefinition[]? - An array of AttributeDefinition objects. Each of these objects describes one attribute in the table and index key schema
- BillingModeSummary? BillingModeSummary? - Contains the details for the read/write capacity mode
- CreationDateTime? int? - The date and time when the table was created, in UNIX epoch time format
- GlobalSecondaryIndexes? GlobalSecondaryIndexDescription[]? - The global secondary indexes, if any, on the table. Each index is scoped to a given partition key value
- GlobalTableVersion? string? - Represents the version of global tables in use, if the table is replicated across AWS Regions
- ItemCount? int? - The number of items in the specified table
- KeySchema? KeySchemaElement[]? - The primary key structure for the table
- LastDecreaseDateTimeatestStreamArn? string? - The Amazon Resource Name (ARN) that uniquely identifies the latest stream for this table
- LatestStreamLabel? string? - A timestamp, in ISO 8601 format, for this stream
- LocalSecondaryIndexes? LocalSecondaryIndexDescription[]? - Represents one or more local secondary indexes on the table
- ProvisionedThroughput? ProvisionedThroughputDescription? - The provisioned throughput settings for the table, consisting of read and write capacity units, along with data about increases and decreases
- Replicas? ReplicaDescription[]? - Represents replicas of the table
- RestoreSummary? RestoreSummary? - Contains details for the restore
- SSEDescription? SSEDescription? - The description of the server-side encryption status on the specified table
- StreamSpecification? StreamSpecification? - The current DynamoDB Streams configuration for the table
- TableArn? string? - The Amazon Resource Name (ARN) that uniquely identifies the table
- TableId? string? - Unique identifier for the table for which the backup was created
- TableName? string? - The name of the table
- TableSizeBytes? int? - The total size of the specified table, in bytes
- TableStatus? TableStatus? - The current state of the table. Valid Values: CREATING | UPDATING | DELETING | ACTIVE | INACCESSIBLE_ENCRYPTION_CREDENTIALS | ARCHIVING | ARCHIVED
aws.dynamodb: TableList
List of Tables.
Fields
- LastEvaluatedTableName? string - The name of the last table in the current page of results. Use this value as the ExclusiveStartTableName in a new request to obtain the next page of results, until all the table names are returned
- TableNames? string[] - The names of the tables associated with the current account at the current endpoint. The maximum size of this array is 100
aws.dynamodb: TableUpdateInput
Represents the request payload to update table.
Fields
- TableName string - The name of the table to be updated
- AttributeDefinitions? AttributeDefinition[] - An array of attributes that describe the key schema for the table and indexes. If you are adding a new global secondary index to the table, AttributeDefinitions must include the key element(s) of the new index
- BillingMode? BillingMode - Controls how you are charged for read and write throughput and how you manage capacity
- GlobalSecondaryIndexUpdates? GlobalSecondaryIndexUpdate[] - An array of one or more global secondary indexes for the table
- ProvisionedThroughput? ProvisionedThroughput - The new provisioned throughput settings for the specified table or index
- ReplicaUpdates? ReplicationGroupUpdate[] - A list of replica update actions (create, delete, or update) for the table
- SSESpecification? SSESpecification - The new server-side encryption settings for the specified table
- StreamSpecification? StreamSpecification - Represents the DynamoDB Streams configuration for the table
aws.dynamodb: Tag
Describes a tag. A tag is a key-value pair. You can add up to 50 tags to a single DynamoDB table.
Fields
- Key string - The key of the tag. Tag keys are case sensitive. Each DynamoDB table can only have up to one tag with the same key. If you try to add an existing tag (same key), the existing tag value will be updated to the new value
- Value string? - The value of the tag. Tag values are case-sensitive and can be null
aws.dynamodb: TTLDescription
The description of the Time to Live (TTL) status on the specified table
Fields
- AttributeName? string - The name of the TTL attribute for items in the table
- TimeToLiveStatus? TimeToLiveStatus - The TTL status for the table
aws.dynamodb: UpdateGlobalSecondaryIndexAction
Represents the new provisioned throughput settings to be applied to a global secondary index.
Fields
- IndexName string - The name of the global secondary index to be updated
- ProvisionedThroughput ProvisionedThroughput - Represents the provisioned throughput settings for the specified global secondary index
aws.dynamodb: UpdateReplicationGroupMemberAction
Represents a replica to be modified.
Fields
- Fields Included from *CreateReplicationGroupMemberAction
- RegionName string
- GlobalSecondaryIndexes ReplicaGlobalSecondaryIndex[]|()
- KMSMasterKeyId string|()
- ProvisionedThroughputOverride ProvisionedThroughputOverride|()
- anydata...
aws.dynamodb: WriteRequest
Represents an operation to perform - either DeleteItem or PutItem. You can only request one of these operations, not both, in a single WriteRequest. If you do need to perform both of these operations, you need to provide two separate WriteRequest objects.
Fields
- DeleteRequest? DeleteRequest? - A request to perform a DeleteItem operation
- PutRequest? PutRequest? - A request to perform a PutItem operation
Errors
aws.dynamodb: Error
Represents any error related to the dynamodb module.
Import
import ballerinax/aws.dynamodb;
Metadata
Released date: about 1 year ago
Version: 2.3.0
License: Apache-2.0
Compatibility
Platform: java17
Ballerina version: 2201.8.0-20230830-220400-8a7556d8
GraalVM compatible: Yes
Pull count
Total: 3071
Current verison: 2085
Weekly downloads
Keywords
Communication/Email
Cost/Free
Vendor/Google
Contributors