Module zoom
API
Definitions
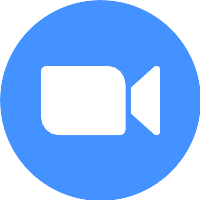
ballerinax/zoom Ballerina library
Overview
This is a generated connector for Zoom API v2.0.0 OpenAPI Specification.
The Zoom API allows developers to access information from Zoom. You can use this API to build private services or public applications on the Zoom App Marketplace. To learn how to get your credentials and create private/public applications, read our Authorization Guide. All endpoints are available via https
and are located at api.zoom.us/v2/
. For instance you can list all users on an account via https://api.zoom.us/v2/users/
.
Configuring connector
Prerequisites
- Create a Zoom account by clicking the
Sign Up
link here: https://marketplace.zoom.us/. Once you activate your account, you’ll be ready to join as a developer. - Obtain tokens
- Create an OAuth2.0 app by visiting Zoom App Marketplace. Then provide app related information and get client credentials. Please follow the steps in here to create and publish a zoom app successfully.
- Generate tokens for your Zoom app following the authorization code flow. Follow the detailed steps given here
- Please note that to set live stream details meeting host need to have a Pro license.
Quickstart
To use the Zoom connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
import ballerinax/zoom;
Step 2: Create a new connector instance
Configure the connection using http:OAuth2RefreshTokenGrantConfig, http:BearerTokenConfig or http:JwtIssuerConfig
zoom:ClientConfig configuration = { auth: { refreshUrl: "https://zoom.us/oauth/token", refreshToken : "<REFRESH_TOKEN>", clientId : "<CLIENT_ID>", clientSecret : "<CLIENT_SECRET>" } }; zoom:Client zoomClient = check new (configuration);
Step 3: Invoke connector operation
- Create Zoom meeting
public function main() returns error? { zoom:MeetingDetails meetingDetails = { topic: "My Test Meeting", start_time: "2021-07-15T13:00:00", duration: 60, timezone: "Asia/Calcutta" }; zoom:CompoundCreateMeetingResponse meeting = check zoomClient->createMeeting("me", meetingDetails); }
- Use
bal run
command to compile and run the Ballerina program.
Clients
zoom: Client
This is a generated connector for Zoom API Version 2.0.0 OpenAPI Specification.
The Zoom API allows developers to access information from Zoom. You can use this API to build private services or public applications on the Zoom App Marketplace. To learn how to get your credentials and create private/public applications, read our Authorization Guide.
All endpoints are available via https
and are located at api.zoom.us/v2/
. For instance you can list all users on an account via https://api.zoom.us/v2/users/
.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Please create a Zoom account and obtain tokens following this guide. Configure required scopes when obtaining the tokens.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.zoom.us/v2" - URL of the target service
listUsers
function listUsers(string status, int pageSize, string? roleId, string? pageNumber, string? includeFields, string? nextPageToken) returns ListUsersResponse|error
List users
Parameters
- status string (default "active") - The user's status. active, live or upcoming. Default is active.
- pageSize int (default 30) - The number of records returned within a single API call.
- roleId string? (default ()) - The role's unique ID. Use this parameter to filter the response by a specific role.
- pageNumber string? (default ()) - The page number of the current page in the returned records.
- includeFields string? (default ()) - Use this parameter to display one of the following attributes in the API call's response. custom_attributes or host_key.
- nextPageToken string? (default ()) - The next page token is used to paginate through large result sets. A next page token will be returned whenever the set of available results exceeds the current page size. The expiration period for this token is 15 minutes.
Return Type
- ListUsersResponse|error - HTTP Status Code:200. User list returned.
listDashboardMeetings
function listDashboardMeetings(string 'from, string to, string 'type, int pageSize, string? nextPageToken, string? groupId, string? includeFields) returns ListDashboardMeetingsResponse|error
List dashboard meetings
Parameters
- 'from string - Start date in 'yyyy-mm-dd' format. The date range defined by the 'from' and 'to' parameters should only be one month as the report includes only one month worth of data at once.
- to string - End date in 'yyyy-mm-dd' format.
- 'type string (default "live") - Specify a value to get the response for the corresponding meeting type. The value of this field can be one of the following:
past
- Meeting that already occurred in the specified date range.pastOne
- Past meetings that were attended by only one user.live
- Live meetings. If you do not provide this field, the default value will belive
and thus, the API will only query responses for live meetings.
- pageSize int (default 30) - The number of records returned within a single API call.
- nextPageToken string? (default ()) - The next page token is used to paginate through large result sets. A next page token will be returned whenever the set of available results exceeds the current page size. The expiration period for this token is 15 minutes.
- groupId string? (default ()) - The group ID. The API response will only contain meetings where the host is a member of the queried group ID.
- includeFields string? (default ()) - Set the value of this field to 'tracking_fields' if you would like to include tracking fields of each meeting in the response.
Return Type
- ListDashboardMeetingsResponse|error - HTTP Status Code:200. Dashboard meetings returned. Only available for paid accounts that have dashboard feature enabled.
listDashboardMeetingParticipants
function listDashboardMeetingParticipants(MeetingId meetingId, string 'type, int pageSize, string? nextPageToken, string? includeFields) returns ListDashboardMeetingParticipantsResponse|error
List dashboard meeting participants
Parameters
- meetingId MeetingId - The meeting's ID or universally unique ID (UUID). If you provide a meeting ID, the API will return a response for the latest meeting instance. If you provide a meeting UUID that begins with a
/
character or contains the//
characters, you must double-encode the meeting UUID before making an API request.
- 'type string (default "live") - The type of meeting to query: *
past
— All past meetings. *pastOne
— All past one-user meetings. *live
- All live meetings. This value defaults tolive
.
- pageSize int (default 30) - The number of records returned within a single API call.
- nextPageToken string? (default ()) - The next page token is used to paginate through large result sets. A next page token will be returned whenever the set of available results exceeds the current page size. The expiration period for this token is 15 minutes.
- includeFields string? (default ()) - Provide
registrant_id
as the value for this field if you would like to see the registrant ID attribute in the response of this API call. A registrant ID is a unique identifier of a meeting registrant. This is not supported forlive
meeting types.
Return Type
- ListDashboardMeetingParticipantsResponse|error - HTTP Status Code:200. Meeting participants returned. Only available for paid accounts that have enabled the dashboard feature.
listMeetings
function listMeetings(string userId, string 'type, int pageSize, string? nextPageToken, string? pageNumber) returns ListMeetingsResponse|error
List meetings
Parameters
- userId string - The user ID or email address of the user. For user-level apps, pass
me
as the value for userId.
- 'type string (default "live") - The meeting types. Scheduled, live or upcoming
- pageSize int (default 30) - The number of records returned within a single API call.
- nextPageToken string? (default ()) - The next page token is used to paginate through large result sets. A next page token will be returned whenever the set of available results exceeds the current page size. The expiration period for this token is 15 minutes.
- pageNumber string? (default ()) - The page number of the current page in the returned records.
Return Type
- ListMeetingsResponse|error - HTTP Status Code:200. List of meetings returned.
createMeeting
function createMeeting(string userId, MeetingDetails payload) returns CreateMeetingResponse|error
Create a meeting
Parameters
- userId string - The user ID or email address of the user. For user-level apps, pass me as the value for userId.
- payload MeetingDetails - Meeting detailed.
Return Type
- CreateMeetingResponse|error - HTTP Status Code:201 - Meeting created.
listMeetingRegistrants
function listMeetingRegistrants(int meetingId, string? occurrenceId, string status, int pageSize, int pageNumber, string? nextPageToken) returns ListMeetingRegistrantsResponse|error
List meeting registrants
Parameters
- meetingId int - MThe meeting ID in long format. The data type of this field is "long"(represented as int64 in JSON).
- occurrenceId string? (default ()) - The meeting occurrence ID.
- status string (default "approved") - The registrant status
- pageSize int (default 30) - The number of records returned within a single API call.
- pageNumber int (default 1) - Deprecated - The page number of the current page in the returned records.
- nextPageToken string? (default ()) - The next page token is used to paginate through large result sets. A next page token will be returned whenever the set of available results exceeds the current page size. The expiration period for this token is 15 minutes.
Return Type
- ListMeetingRegistrantsResponse|error - HTTP Status Code:200. Successfully listed meeting registrants.
addMeetingRegistrant
function addMeetingRegistrant(int meetingId, AddMeetingRegistrantRequest payload, string? occurrenceIds) returns AddMeetingRegistrantResponse|error
Add meeting registrant
Parameters
- meetingId int - The meeting ID in long format. The data type of this field is "long"(represented as int64 in JSON).
- payload AddMeetingRegistrantRequest - Meeting Registrant Details
- occurrenceIds string? (default ()) - Occurrence IDs. You can find these with the meeting get API. Multiple values separated by comma.
Return Type
- AddMeetingRegistrantResponse|error - Meeting registrant's details
getMeetingRegistrantsQuestions
function getMeetingRegistrantsQuestions(int meetingId) returns RegistrantQuestions|error
List registration questions
Parameters
- meetingId int - The meeting ID in long format. The data type of this field is "long"(represented as int64 in JSON). While storing it in your database, store it as a long data type and not as an integer, as the Meeting IDs can be longer than 10 digits.
Return Type
- RegistrantQuestions|error - HTTP Status Code:
200
. Meeting Registrant Question object returned
updateMeetingRegistrantQuestions
function updateMeetingRegistrantQuestions(int meetingId, RegistrantQuestions payload) returns Response|error
Update registration questions
Parameters
- meetingId int - The meeting ID in long format. The data type of this field is "long"(represented as int64 in JSON). While storing it in your database, store it as a long data type and not as an integer, as the Meeting IDs can be longer than 10 digits.
- payload RegistrantQuestions - Meeting Registrant Questions
updateMeetingRegistrantStatus
function updateMeetingRegistrantStatus(int meetingId, UpdateMeetingRegistrantstatusRequest payload, string? occurrenceId) returns Response|error
Update registrant's status
Parameters
- meetingId int - The meeting ID in long format. The data type of this field is "long"(represented as int64 in JSON). While storing it in your database, store it as a long data type and not as an integer, as the Meeting IDs can be longer than 10 digits.
- payload UpdateMeetingRegistrantstatusRequest - Request payload for meeting registrant status update
- occurrenceId string? (default ()) - The meeting occurrence ID.
deleteMeetingregistrant
function deleteMeetingregistrant(int meetingId, string registrantId, string? occurrenceId) returns Response|error
Delete a meeting registrant
Parameters
- meetingId int - The meeting ID.
- registrantId string - The meeting registrant ID.
- occurrenceId string? (default ()) - The meeting occurence ID.
getMeetingById
function getMeetingById(int meetingId, string? occurrenceId, boolean? showPreviousOccurrences) returns GetMeetingDetailsResponse|error
Get a meeting
Parameters
- meetingId int - The meeting ID in long format. The data type of this field is "long"(represented as int64 in JSON). While storing it in your database, store it as a long data type and not as an integer, as the Meeting IDs can be longer than 10 digits.
- occurrenceId string? (default ()) - Meeting Occurrence ID. Provide this field to view meeting details of a particular occurrence of the recurring meeting.
- showPreviousOccurrences boolean? (default ()) - Set the value of this field to
true
if you would like to view meeting details of all previous occurrences of a recurring meeting.
Return Type
- GetMeetingDetailsResponse|error - HTTP Status Code:
200
Meeting object returned.
deleteMeeting
function deleteMeeting(int meetingId, string? occurrenceId, boolean? scheduleForReminder, string? cancelMeetingReminder) returns Response|error
Delete a meeting
Parameters
- meetingId int - The meeting ID in long format. The data type of this field is "long"(represented as int64 in JSON). While storing it in your database, store it as a long data type and not as an integer, as the Meeting IDs can be longer than 10 digits.
- occurrenceId string? (default ()) - The meeting occurrence ID.
- scheduleForReminder boolean? (default ()) -
true
: Notify host and alternative host about the meeting cancellation via email.false
: Do not send any email notification.
- cancelMeetingReminder string? (default ()) -
true
: Notify registrants about the meeting cancellation via email.false
: Do not send any email notification to meeting registrants. The default value of this field isfalse
.
updateMeeting
function updateMeeting(int meetingId, UpdateMeetingRequest payload, string? occurrenceId) returns Response|error
Update a meeting
Parameters
- meetingId int - The meeting ID in long format. The data type of this field is "long"(represented as int64 in JSON). While storing it in your database, store it as a long data type and not as an integer, as the Meeting IDs can be longer than 10 digits.
- payload UpdateMeetingRequest - Meeting
- occurrenceId string? (default ()) - Meeting occurrence id. Support change of agenda, start_time, duration, settings: {host_video, participant_video, join_before_host, mute_upon_entry, waiting_room, watermark, auto_recording}
updateMeetingStatus
function updateMeetingStatus(int meetingId, UpdateMeetingstatusRequest payload) returns Response|error
Update meeting status
Parameters
- meetingId int - The meeting ID in long format. The data type of this field is "long"(represented as int64 in JSON). While storing it in your database, store it as a long data type and not as an integer, as the Meeting IDs can be longer than 10 digits.
- payload UpdateMeetingstatusRequest - Meeting status update details
listPastMeetings
function listPastMeetings(int meetingId) returns ListPastMeetingsResponse|error
List ended meeting instances
Parameters
- meetingId int - The meeting ID in long format. The data type of this field is "long"(represented as int64 in JSON). While storing it in your database, store it as a long data type and not as an integer, as the Meeting IDs can be longer than 10 digits.
Return Type
- ListPastMeetingsResponse|error - HTTP Status Code:
200
. List of ended meeting instances returned.
listPastMeetingPolls
function listPastMeetingPolls(string meetingId) returns ListPastMeetingPollsResponse|error
List past meeting's poll results
Parameters
- meetingId string - The meeting ID or the meeting UUID. If a meeting ID is provided in the request instead of a UUID, the response will be for the latest meeting instance. If a UUID starts with "/" or contains "//" (example: "/ajXp112QmuoKj4854875=="), you must double encode the UUID before making an API request.
Return Type
- ListPastMeetingPollsResponse|error - HTTP Status Code:
200
OK. Polls returned successfully.
getPastMeetingDetails
function getPastMeetingDetails(string meetingUUID) returns PastMeetingDetailsResponse|error
Get past meeting details
Parameters
- meetingUUID string - The meeting UUID. Each meeting instance will generate its own Meeting UUID (i.e., after a meeting ends, a new UUID will be generated for the next instance of the meeting). Please double encode your UUID when using it for other API calls if the UUID begins with a '/'or contains '//' in it.
Return Type
- PastMeetingDetailsResponse|error - HTTP Status Code:
200
. Meeting details returned.
listPastMeetingParticipants
function listPastMeetingParticipants(string meetingUUID, int pageSize, string? nextPageToken) returns ListPastMeetingParticipantsResponse|error
List past meeting participants
Parameters
- meetingUUID string - The meeting UUID. Each meeting instance will generate its own Meeting UUID (i.e., after a meeting ends, a new UUID will be generated for the next instance of the meeting). Please double encode your UUID when using it for other API calls if the UUID begins with a '/'or contains '//' in it.
- pageSize int (default 30) - The number of records returned within a single API call.
- nextPageToken string? (default ()) - The next page token is used to paginate through large result sets. A next page token will be returned whenever the set of available results exceeds the current page size. The expiration period for this token is 15 minutes.
Return Type
- ListPastMeetingParticipantsResponse|error - HTTP Status Code:
200
. Meeting participants' report returned.
getMeetingPolls
function getMeetingPolls(int meetingId) returns MeetingPollsResponse|error
List meeting polls
Parameters
- meetingId int - The meeting ID in long format. The data type of this field is "long"(represented as int64 in JSON). While storing it in your database, store it as a long data type and not as an integer, as the Meeting IDs can be longer than 10 digits.
Return Type
- MeetingPollsResponse|error - HTTP Status Code:. List polls of a Meeting returned
createMeetingPoll
function createMeetingPoll(int meetingId, CreateMeetingPollRequest payload) returns CreateMeetingPollResponse|error
Create a meeting poll
Parameters
- meetingId int - The meeting ID in long format. The data type of this field is "long"(represented as int64 in JSON). While storing it in your database, store it as a long data type and not as an integer, as the Meeting IDs can be longer than 10 digits.
- payload CreateMeetingPollRequest - Meeting poll object
Return Type
- CreateMeetingPollResponse|error - HTTP Status Code:
201
. Meeting Poll Created
getMeetingPoll
function getMeetingPoll(int meetingId, string pollId) returns GetMeetingPollResponse|error
Get a meeting poll
Parameters
- meetingId int - The meeting ID in long format. The data type of this field is "long"(represented as int64 in JSON). While storing it in your database, store it as a long data type and not as an integer, as the Meeting IDs can be longer than 10 digits.
- pollId string - The poll ID
Return Type
- GetMeetingPollResponse|error - HTTP Status Code:
200
. Meeting Poll object returned
updateMeetingPoll
function updateMeetingPoll(int meetingId, string pollId, UpdateMeetingPollRequest payload) returns Response|error
Update a meeting poll
Parameters
- meetingId int - The meeting ID in long format. The data type of this field is "long"(represented as int64 in JSON). While storing it in your database, store it as a long data type and not as an integer, as the Meeting IDs can be longer than 10 digits.
- pollId string - The poll ID
- payload UpdateMeetingPollRequest - Meeting Poll
deleteMeetingPoll
Delete a meeting poll
Parameters
- meetingId int - The meeting ID in long format. The data type of this field is "long"(represented as int64 in JSON). While storing it in your database, store it as a long data type and not as an integer, as the Meeting IDs can be longer than 10 digits.
- pollId string - The poll ID
createBatchPolls
function createBatchPolls(string meetingId, CreateBatchPollsRequest payload) returns CreateBatchPollsResponse|error
Perform batch poll creation
Return Type
- CreateBatchPollsResponse|error - HTTP Status Code:
201
. Meeting Poll Created
getMeetingInvitation
function getMeetingInvitation(int meetingId) returns GetMeetingInvitationResponse|error
Get meeting invitation
Parameters
- meetingId int - The meeting ID in long format. The data type of this field is "long"(represented as int64 in JSON). While storing it in your database, store it as a long data type and not as an integer, as the Meeting IDs can be longer than 10 digits.
Return Type
- GetMeetingInvitationResponse|error - HTTP Status Code:
200
<br> Meeting invitation returned.
getLiveStreamDetails
function getLiveStreamDetails(string meetingId) returns GetLiveStreamDetailsResponse|error
Get live stream details
Parameters
- meetingId string - Unique identifier of the meeting.
Return Type
- GetLiveStreamDetailsResponse|error - HTTP Status Code:
200
OK. Live Stream details returned.
updateMeetingLiveStream
function updateMeetingLiveStream(int meetingId, UpdateMeetingLiveStreamDetailsRequest payload) returns Response|error
Update a live stream
Parameters
- meetingId int - The meeting ID in long format. The data type of this field is "long"(represented as int64 in JSON). While storing it in your database, store it as a long data type and not as an integer, as the Meeting IDs can be longer than 10 digits.
- payload UpdateMeetingLiveStreamDetailsRequest - Meeting
listWebinarRegistrants
function listWebinarRegistrants(int webinarId, string? occurrenceId, string status, string? trackingSourceId, int pageSize, int pageNumber, string? nextPageToken) returns ListWebinarRegistrantsResponse|error
List webinar registrants
Parameters
- webinarId int - The webinar ID in "long" format(represented as int64 data type in JSON).
- occurrenceId string? (default ()) - The meeting occurrence ID.
- status string (default "approved") - The registrant status:
pending
- Registrant's status is pending.approved
- Registrant's status is approved.denied
- Registrant's status is denied.
- trackingSourceId string? (default ()) - The tracking source ID for the registrants. Useful if you share the webinar registration page in multiple locations. See Creating source tracking links for webinar registration for details.
- pageSize int (default 30) - The number of records returned within a single API call.
- pageNumber int (default 1) - The page number of the current page in the returned records.
- nextPageToken string? (default ()) - The next page token is used to paginate through large result sets. A next page token will be returned whenever the set of available results exceeds the current page size. The expiration period for this token is 15 minutes.
Return Type
- ListWebinarRegistrantsResponse|error - HTTP Status Code:
200
Webinar plan subscription is missing. Enable webinar for this user once the subscription is added.
listWebinarParticipants
function listWebinarParticipants(string webinarId, int pageSize, string? nextPageToken) returns ListWebinarParticipantsResponse|error
List webinar participants
Parameters
- webinarId string - Unique identifier of the webinar. You can retrieve the value of this field by calling the list webinars API.
- pageSize int (default 30) - The number of records returned within a single API call.
- nextPageToken string? (default ()) - The next page token is used to paginate through large result sets. A next page token will be returned whenever the set of available results exceeds the current page size. The expiration period for this token is 15 minutes.
Return Type
- ListWebinarParticipantsResponse|error - Webinar participants' details
listWebinarAbsentees
function listWebinarAbsentees(string webinarUUID, string? occurrenceId, int pageSize, string? nextPageToken) returns ListWebinarAbsentees|error
List webinar absentees
Parameters
- webinarUUID string - The Webinar UUID. Each Webinar instance will generate its own Webinar UUID (i.e., after a Webinar ends, a new UUID will be generated for the next instance of the Webinar). Please double encode your UUID when using it for API calls if the UUID begins with a '/' or contains '//' in it.
- occurrenceId string? (default ()) - The meeting occurrence ID.
- pageSize int (default 30) - The number of records returned within a single API call.
- nextPageToken string? (default ()) - The next page token is used to paginate through large result sets. A next page token will be returned whenever the set of available results exceeds the current page size. The expiration period for this token is 15 minutes.
Return Type
- ListWebinarAbsentees|error - HTTP Status Code:
200
Success. Error Code:200
Webinar plan subscription is missing. Enable webinar for this user once the subscription is added:{userId}.
listMeetingTemplates
function listMeetingTemplates(string userId) returns ListMeetingTemplatesResponse|error
List meeting templates
Parameters
- userId string - Unique identifier of the user. Retrieve the value of this field by calling the List users API.
Return Type
- ListMeetingTemplatesResponse|error - HTTP Status Code:
200
OK
Records
zoom: AddMeetingRegistrantRequest
Registrant base object
Fields
- language string? - Registrant's language preference for confirmation emails. The value can be one of the following:
en-US
,de-DE
,es-ES
,fr-FR
,jp-JP
,pt-PT
,ru-RU
,zh-CN
,zh-TW
,ko-KO
,it-IT
,vi-VN
.
- auto_approve boolean? - Enable auto approve
- address string? - Registrant's address.
- city string? - Registrant's city.
- comments string? - A field that allows registrants to provide any questions or comments that they might have.
- custom_questions RegistrantCustomQuestion[]? - Custom questions.
- email string - A valid email address of the registrant.
- first_name string - Registrant's first name.
- industry string? - Registrant's Industry.
- job_title string? - Registrant's job title.
- last_name string? - Registrant's last name.
- no_of_employees string? - Number of Employees:
1-20
,21-50
,51-100
,101-500
,500-1,000
,1,001-5,000
,5,001-10,000
,More than 10,000
- org string? - Registrant's Organization.
- phone string? - Registrant's Phone number.
- purchasing_time_frame string? - This field can be included to gauge interest of webinar attendees towards buying your product or service.
Purchasing Time Frame:
Within a month``1-3 months``4-6 months``More than 6 months``No timeframe
- role_in_purchase_process string? - Role in Purchase Process:
Decision Maker
,Evaluator/Recommender
,Influencer
,Not involved
- state string? - Registrant's State/Province.
- zip string? - Registrant's Zip/Postal Code.
zoom: AddMeetingRegistrantResponse
Fields
- id int? - Meeting ID: Unique identifier of the meeting in "long" format(represented as int64 data type in JSON), also known as the meeting number.
- registrant_id string? - Unique identifier of the registrant.
- start_time string? - The start time for the meeting.
- topic string? - Topic of the meeting.
zoom: AddPollQuestionsResponse
Response received from adding poll quetions
Fields
- id string? - Meeting Poll ID
- questions PollQuestions[]? - Poll questions
- status string? - Status of the Meeting Poll:<br>
notstart
- Poll not started<br>started
- Poll started<br>ended
- Poll ended<br>sharing
- Sharing poll results
- title string? - Title for the Poll
zoom: ApprovalAndDenialInfo
Approve or block users from specific regions/countries from joining this meeting.
Fields
- approved_list string[]? - List of countries/regions from where participants can join this meeting.
- denied_list string[]? - List of countries/regions from where participants can not join this meeting.
- enable boolean? -
true
: Setting enabled to either allow users or block users from specific regions to join your meetings.false
: Setting disabled.
- method string? - Specify whether to allow users from specific regions to join thismeeting; or block users from specific regions from oining this meeting. Values: approve or deny
zoom: ApprovedOrDeniedCountriesDetails
Approve or block users from specific regions/countries from joining this meeting.
Fields
- approved_list string[]? - List of countries/regions from where participants can join this meeting.
- denied_list string[]? - List of countries/regions from where participants can not join this meeting.
- enable boolean? -
true
: Setting enabled to either allow users or block users from specific regions to join your meetings.false
: Setting disabled.
- method string? - Specify whether to allow users from specific regions to join this meeting; or block users from specific regions from joining this meeting.
approve
: Allow users from specific regions/countries to join this meeting. If this setting is selected, the approved regions/countries must be included in theapproved_list
.deny
: Block users from specific regions/countries from joining this meeting. If this setting is selected, the approved regions/countries must be included in thedenied_list
zoom: BreakoutRoomsDetails
Fields
- name string? - Name of the breakout room.
- participants string[]? - Email addresses of the participants who are to be assigned to the breakout room.
zoom: BreakoutRoomSettings
Setting to pre-assign breakout rooms
Fields
- enable boolean? - Set the value of this field to
true
if you would like to enable the pre-assigned breakout rooms.
- rooms BreakoutRoomsDetails[]? - Create room(s).
zoom: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
zoom: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
zoom: CreateBatchPollsRequest
Fields
- questions PollQuestions[]? - Poll questions
- title string? - Meeting Poll Title.
zoom: CreateBatchPollsResponse
Fields
- polls AddPollQuestionsResponse[]? -
zoom: CreateMeetingPollRequest
Poll
Fields
- questions PollQuestions[]? - Array of Polls
- title string? - Title for the poll.
zoom: CreateMeetingPollResponse
Fields
- id string? - Meeting Poll ID
- status string? - Status of the Meeting Poll:<br>
notstart
- Poll not started<br>started
- Poll started<br>ended
- Poll ended<br>sharing
- Sharing poll results
- questions PollQuestions[]? - Array of Polls
- title string? - Title for the poll.
zoom: CreateMeetingResponse
Details of the created meeting.
Fields
- Fields Included from *MeetingMetadata
- Fields Included from *RequestedMeetingDetails
- agenda string
- created_at string
- duration int
- h323_password string
- join_url string
- occurrences MeetingOccurenceDetails[]
- password string
- pmi int
- recurrence ReccurenceDetails
- settings MeetingSettings
- start_time string
- start_url string
- timezone string
- topic string
- tracking_fields RequestedMeetingTrackingDetails[]
- type int
- anydata...
zoom: CustomAttributeDetails
User's Custom Attribute Details
Fields
- 'key string? - The custom attribute's unique ID.
- name string? - The custom attribute's name.
- value string? - The custom attribute's value.
zoom: CustomKeys
Fields
- 'key string? - Custom key associated with the user.
- value string? - Value of the custom key associated with the user.
zoom: CustomQuestions
Fields
- answers string[]? - Answer choices for the question. Can not be used for
short
question type as this type of question requires registrants to type out the answer.
- required boolean? - Indicates whether or not the custom question is required to be answered by participants or not.
- title string? - Title of the custom question.
- 'type string? - Type of the question being asked.
zoom: CustomRegistrantQuestions
Fields
- field_name string? - Field name of the question.
- required boolean? - Indicates whether or not the displayed fields are required to be filled out by registrants.
zoom: DashboardMeetingList
List of dashboard meetings
Fields
- meetings MeetingMetricDetails[]? - List of Meeting metric details.
zoom: DashboardMeetingParticipantsList
List of dashboard meeting participants
Fields
- participants MeetingParticipantsDetails[]? - List of Meeting participants details.
zoom: DurationObject
Duration Details
Fields
- 'from string? - Start date for this report in 'yyyy-mm-dd' format.
- to string? - End date for this report in 'yyyy-mm-dd' format.
zoom: EndedMeetingDetails
Ended meeting details
Fields
- start_time string? - Start time
- uuid string? - Meeting UUID. Unique meeting ID. Each meeting instance will generate its own Meeting UUID (i.e., after a meeting ends, a new UUID will be generated for the next instance of the meeting). Please double encode your UUID when using it for API calls if the UUID begins with a '/'or contains '//' in it.
zoom: GetLiveStreamDetailsResponse
Fields
- page_url string? - Live streaming page URL. This is the URL using which anyone can view the live stream of the meeting.
- stream_key string? - Stream Key.
- stream_url string? - Stream URL.
zoom: GetMeetingDetailsResponse
Details of the meeting.
Fields
- Fields Included from *MeetingFullMetadata
- Fields Included from *RequestedMeetingDetails
- agenda string
- created_at string
- duration int
- h323_password string
- join_url string
- occurrences MeetingOccurenceDetails[]
- password string
- pmi int
- recurrence ReccurenceDetails
- settings MeetingSettings
- start_time string
- start_url string
- timezone string
- topic string
- tracking_fields RequestedMeetingTrackingDetails[]
- type int
- anydata...
zoom: GetMeetingInvitationResponse
Meeting invitation details.
Fields
- invitation string? - Meeting invitation.
zoom: GetMeetingPollResponse
Fields
- id string? - Meeting Poll ID
- status string? - Status of the Meeting Poll:<br>
notstart
- Poll not started<br>started
- Poll started<br>ended
- Poll ended<br>sharing
- Sharing poll results
- questions PollQuestions[]? - Array of Polls
- title string? - Title for the poll.
zoom: GlobalDialInNumbersDetails
Fields
- city string? - City of the number, if any. For example, Chicago.
- country string? - Country code. For example, BR.
- country_name string? - Full name of country. For example, Brazil.
- number string? - Phone number. For example, +1 2332357613.
- 'type string? - Type of number.
zoom: InterpreterDetails
Fields
- email string? - Email address of the interpreter.
- languages string? - Languages for interpretation. The string must contain two separated by a comma. For example, if the language is to be interpreted from English to Chinese, the value of this field should be US,CN.
zoom: LanguageInterpretationDetails
Language interpretation for meetings.
Fields
- enable boolean? - Indicate whether or not you would like to enable language interpretation or this meeting.
- interpreters InterpreterDetails[]? - Information associated with the interpreter.
zoom: ListDashboardMeetingParticipantsResponse
List of dashboard meetings
Fields
- Fields Included from *PaginationObject
- Fields Included from *DashboardMeetingParticipantsList
- participants MeetingParticipantsDetails[]
- anydata...
zoom: ListDashboardMeetingsResponse
List of dashboard meetings
Fields
- Fields Included from *DurationObject
- Fields Included from *PaginationObject
- Fields Included from *DashboardMeetingList
- meetings MeetingMetricDetails[]
- anydata...
zoom: ListMeetingRegistrantsResponse
List of users.
Fields
- Fields Included from *PaginationObject
- Fields Included from *RegistrantsList
- registrants RegistrantDetails[]
- anydata...
zoom: ListMeetingsResponse
List of meetings
Fields
- Fields Included from *PaginationObject
- Fields Included from *MeetingList
- meetings MeetingObject[]
- anydata...
zoom: ListMeetingTemplatesResponse
Fields
- templates TemplateDetails[]? -
- total_records int? - Total records found for this request.
zoom: ListPastMeetingParticipantsResponse
List of participants
Fields
- Fields Included from *PaginationObject
- Fields Included from *MeetingPartcipantsList
- participants PartcipantDetails[]
- anydata...
zoom: ListPastMeetingPollsResponse
Fields
- id int? - Meeting ID: Unique identifier of the meeting in "long" format(represented as int64 data type in JSON), also known as the meeting number.
- questions UserSubmittedAnswers[]? -
- start_time string? - The start time of the meeting.
- uuid string? - Meeting UUID.
zoom: ListPastMeetingsResponse
List of Meetings
Fields
- meetings EndedMeetingDetails[]? - List of ended meeting instances.
zoom: ListUsersResponse
List of users
Fields
- Fields Included from *PaginationObject
- Fields Included from *UsersList
- users UserDetails[]
- anydata...
zoom: ListWebinarAbsentees
List of users.
Fields
- Fields Included from *PaginationObject
- Fields Included from *RegistrantsList
- registrants RegistrantDetails[]
- anydata...
zoom: ListWebinarParticipantsResponse
Fields
- next_page_token string? - The next page token is used to paginate through large result sets. A next page token will be returned whenever the set of available results exceeds the current page size. The expiration period for this token is 15 minutes.
- page_count int? - The number of pages returned for this request.
- page_size int? - The total number of records returned from a single API call.
- participants PartcipantDetails[]? - ParticipantsDetails
- total_records int? - The total number of records available across all pages.
zoom: ListWebinarRegistrantsResponse
List of users.
Fields
- Fields Included from *PaginationObject
- Fields Included from *RegistrantsList
- registrants RegistrantDetails[]
- anydata...
zoom: MeetingDetails
Base object for meeting
Fields
- agenda string? - Meeting description.
- duration int? - Meeting duration (minutes). Used for scheduled meetings only.
- password string? - Passcode to join the meeting.
- recurrence ReccurenceDetails? - Recurrence related meeting informations
- schedule_for string? - If you would like to schedule this meeting for someone else in your account, provide the Zoom user id or email address of the user here.
- settings MeetingSettingsInRequest? - Meeting settings
- start_time string? - Meeting start time. We support two formats for
start_time
- local time and GMT.To set time as GMT the format should beyyyy-MM-dd
THH:mm:ssZ
. To set time using a specific timezone, useyyyy-MM-dd
THH:mm:ss
format and specify the timezone id in thetimezone
field OR leave it blank and the timezone set on your Zoom account will be used. You can also set the time as UTC as the timezone field
- template_id string? - Unique identifier of the admin meeting template. To create admin meeting templates, contact the Zoom support team.
- topic string? - Meeting topic.
- tracking_fields MeetingTrackingDetails[]? - Tracking fields
- 'type int? - Meeting Type: 1 - Instant meeting. 2 - Scheduled meeting. 3 - Recurring meeting with no fixed time. 8 - Recurring meeting with fixed time.
zoom: MeetingFullMetadata
Detailed Meeting Metadata
Fields
- assistant_id string? - Unique identifier of the scheduler who scheduled this meeting on behalf of the host. This field is only returned if you used "schedule_for" option in the Create a Meeting API request.
- host_email string? - Email address of the meeting host.
- host_id string? - ID of the user who is set as host of meeting.
- id int? - Meeting ID: Unique identifier of the meeting in "long" format(represented as int64 data type in JSON), also known as the meeting number.
- uuid string? - Unique meeting ID. Each meeting instance will generate its own Meeting UUID (i.e., after a meeting ends, a new UUID will be generated for the next instance of the meeting). You can retrieve a list of UUIDs from past meeting instances using this API . Please double encode your UUID when using it for API calls if the UUID begins with a '/'or contains '//' in it.
zoom: MeetingList
List of meetings
Fields
- meetings MeetingObject[]? - List of Meeting objects.
zoom: MeetingMetadata
Meeting Metadata
Fields
- assistant_id string? - Unique identifier of the scheduler who scheduled this meeting on behalf of the host. This field is only returned if you used "schedule_for" option in the Create a Meeting API request.
- host_email string? - Email address of the meeting host.
- id int - Meeting ID: Unique identifier of the meeting in "long" format(represented as int64 data type in JSON), also known as the meeting number.
- registration_url string? - URL using which registrants can register for a meeting. This field is only returned for meetings that have enabled registration.
zoom: MeetingMetricDetails
Meeting metric details
Fields
- host string? - Host display name.
- custom_keys MeetingmetricdetailsCustomKeys[]? - Custom keys and values assigned to the meeting.
- dept string? - Department of the host.
- duration string? - Meeting duration. Formatted as hh:mm:ss, for example:
16:08
for 16 minutes and 8 seconds.
- email string? - Email address of the host.
- end_time string? - Meeting end time.
- has_3rd_party_audio boolean? - Indicates whether or not third party audio was used in the meeting.
- has_archiving boolean? - Whether the archiving feature was used in the meeting.
- has_pstn boolean? - Indicates whether or not the PSTN was used in the meeting.
- has_recording boolean? - Indicates whether or not the recording feature was used in the meeting.
- has_screen_share boolean? - Indicates whether or not screenshare feature was used in the meeting.
- has_sip boolean? - Indicates whether or not someone joined the meeting using SIP.
- has_video boolean? - Indicates whether or not video was used in the meeting.
- has_voip boolean? - Indicates whether or not VoIP was used in the meeting.
- id int? - Meeting ID: Unique identifier of the meeting in "long" format(represented as int64 data type in JSON), also known as the meeting number.
- in_room_participants int? - The number of Zoom Room participants in the meeting.
- participants int? - Meeting participant count.
- start_time string? - Meeting start time.
- topic string? - Meeting topic.
- tracking_fields MeetingmetricdetailsTrackingFields[]? - Tracking fields and values assigned to the meeting.
- user_type string? - License type of the user.
- uuid string? - Meeting UUID. Please double encode your UUID when using it for API calls if the UUID begins with a '/'or contains '//' in it.
- audio_quality string? - The meeting's audio quality score:
good
— The audio is almost flawless and the quality is excellent.fair
— The audio occasionally has distortion, noise, and other problems, but the content is basically continuous. Participants can communicate normally.poor
— The audio often has distortion, noise, and other problems, but the content is basically continuous. Participants can communicate normally.bad
— The sound quality is extremely poor and the audio content is almost inaudible.
- video_quality string? - The meeting's video quality score:
good
— The video is almost flawless and the quality is excellent.fair
— The video definition is high, occasionally gets stuck, fast or slow, or other problems, but the frequency is very low and the video quality is good.poor
— The video definition is not high, but not many problems exist. The video quality is mediocre.bad
— The picture is very blurred and often gets stuck.
- screen_share_quality string? - The meeting's screen share quality score:
good
— The video is almost flawless and the quality is excellent.fair
— The video definition is high, occasionally gets stuck, fast or slow, or other problems, but the frequency is very low and the video quality is good.poor
— The video definition is not high, but not many problems exist. The video quality is mediocre.bad
— The picture is very blurred and often gets stuck.
zoom: MeetingmetricdetailsCustomKeys
Fields
- 'key string? - Custom key associated with the meeting.
- value string? - Value of the custom key associated with the meeting.
zoom: MeetingmetricdetailsTrackingFields
Fields
- 'field string? - Label of the tracking field.
- value string? - Value of the tracking field.
zoom: MeetingObject
Fields
- created_at string? - Time of creation.
- duration int? - Meeting duration.
- host_id string? - ID of the user who is set as the host of the meeting.
- id int? - Meeting ID - also known as the meeting number in double (int64) format.
- join_url string? - Join URL.
- start_time string? - Meeting start time.
- timezone string? - Timezone to format the meeting start time.
- topic string? - Meeting topic.
- 'type int? - Meeting Type: 1 - Instant meeting. 2 - Scheduled meeting. 3 - Recurring meeting with no fixed time. 8 - Recurring meeting with fixed time.
- uuid string? - Unique Meeting ID. Each meeting instance will generate its own Meeting UUID.
zoom: MeetingOccurenceDetails
Occurence object. This object is only returned for Recurring Webinars.
Fields
- duration int? - Duration.
- occurrence_id string? - Occurrence ID: Unique Identifier that identifies an occurrence of a recurring webinar. Recurring webinars can have a maximum of 50 occurrences.
- start_time string? - Start time.
- status string? - Occurrence status.
zoom: MeetingPartcipantsList
List of meeting participants
Fields
- participants PartcipantDetails[]? - Array of meeting participant objects.
zoom: MeetingParticipantsDetails
Information about the meeting participants. If a participant left a meeting and rejoined the same meeting, their information will appear as many times as they joined the meeting.
Fields
- audio_quality string? - The participant's audio quality score. The API only returns this value when the Meeting quality scores and network alerts on Dashboard setting is enabled in the Zoom Web Portal and the Show meeting quality score and network alerts on Dashboard option is selected in Account Settings:
good
— The audio is almost flawless and the quality is excellent.fair
— The audio occasionally has distortion, noise, and other problems, but the content is basically continuous. Participants can communicate normally.poor
— The audio often has distortion, noise, and other problems, but the content is basically continuous. Participants can communicate normally.bad
— The sound quality is extremely poor and the audio content is almost inaudible.
- camera string? - The type of camera that the participant used during the meeting.
Note: This response returns an empty string (
““
) value for any users who are not a part of the host's account (external users).
- connection_type string? - The participant's connection type.
- customer_key string? - The participant's SDK identifier. This value can be alphanumeric, up to a maximum length of 15 characters.
- data_center string? - The data center where participant's meeting data is stored.
- device string? - The type of device the participant used to join the meeting:
Phone
— The participant joined via PSTN.H.323/SIP
— The participant joined via an H.323 or SIP device.Windows
— The participant joined via VoIP using a Windows device.Mac
— The participant joined via VoIP using a Mac device.iOS
— The participant joined via VoIP using an iOS device.Android
— The participant joined via VoIP using an Android device.
““
) value for any users who are not a part of the host's account (external users).
- domain string? - The participant's PC domain.
Note: This response returns an empty string (
““
) value for any users who are not a part of the host's account (external users).
- email string? - The participant's email address. If the participant is not part of the host's account, this returns an empty string value, with some exceptions. See Email address display rules for details.
- from_sip_uri string? - The meeting participant's SIP From header URI. The API only returns this response when the participant joins a meeting via SIP.
- full_data_center string? - The data center where participant's meeting data is stored. This field includes a semicolon-separated list of HTTP Tunnel (HT), Cloud Room Connector (CRC), and Real-Time Web Gateway (RWG) location information.
- harddisk_id string? - The participant's hard disk ID.
Note: This response returns an empty string (
““
) value for any users who are not a part of the host's account (external users).
- id string? - The participant's universally unique ID. This value is the same as the participant's user ID if the participant joins the meeting by logging into Zoom. If the participant joins the meeting without logging into Zoom, this returns an empty value.
- in_room_participants int? - The number of participants that joined via Zoom Room.
- ip_address string? - The participant's IP address.
- join_time string? - The time at which participant joined the meeting.
- leave_reason string? - The reason why the participant left the meeting, where
$name
is the participant's username:$name left the meeting.
$name got disconnected from the meeting.
Host ended the meeting.
Host closed the meeting.
Host started a new meeting.
Network connection error.
Host did not join.
Exceeded free meeting minutes limit.
Removed by host.
Unknown reason.
Leave waiting room.
Removed by host from waiting room.
- leave_time string? - The time at which a participant left the meeting. For live meetings, this field will only return if a participant has left the ongoing meeting.
- location string? - The participant's location.
- mac_addr string? - The participant's MAC address.
Note: This response returns an empty string (
““
) value for any users who are not a part of the host's account (external users).
- microphone string? - The type of microphone that the participant used during the meeting.
Note: This response returns an empty string (
““
) value for any users who are not a part of the host's account (external users).
- network_type string? - The participant's network type:
Wired
Wifi
PPP
— Point-to-Point.Cellular
— 3G, 4G, and 5G cellular.Others
— An unknown device.
- participant_user_id string? - The participant's universally unique ID (UUID):
- If the participant joins the meeting by logging into Zoom, this value is the
id
value in the Get a user API response. - If the participant joins the meeting without logging into Zoom, this returns an empty string value.
- If the participant joins the meeting by logging into Zoom, this value is the
- pc_name string? - The participant's PC name.
- recording boolean? - Whether the recording feature was used during the meeting.
- registrant_id string? - The participant's unique registrant ID. This field only returns if you pass the
registrant_id
value for theinclude_fields
query parameter. This field does not return if thetype
query parameter is thelive
value.
- role string? - The participant's role:
host
— Host.attendee
— Attendee.
- screen_share_quality string? - The participant's screen share quality score. The API only returns this value when the Meeting quality scores and network alerts on Dashboard setting is enabled in the Zoom Web Portal and the Show meeting quality score and network alerts on Dashboard option is selected in Account Settings:
good
— The video is almost flawless and the quality is excellent.fair
— The video definition is high, occasionally gets stuck, fast or slow, or other problems, but the frequency is very low and the video quality is good.poor
— The video definition is not high, but not many problems exist. The video quality is mediocre.bad
— The picture is very blurred and often gets stuck.
- share_application boolean? - Whether the participant chose to share an iPhone/iPad app during the screenshare.
- share_desktop boolean? - Whether the participant chose to share their desktop during the screenshare.
- share_whiteboard boolean? - Whether the participant chose to share their whiteboard during the screenshare.
- sip_uri string? - The meeting participant's SIP (Session Initiation Protocol) Contact header URI. The API only returns this response when the participant joins a meeting via SIP.
- speaker string? - The type of speaker that the participant used during the meeting.
Note: This response returns an empty string (
““
) value for any users who are not a part of the host's account (external users).
- status string? - The participant's status:
in_meeting
— In a meeting.in_waiting_room
— In a waiting room.
- user_id string? - The participant's ID. This value assigned to a participant upon joining a meeting and is only valid for the meeting's duration.
- user_name string? - The participant's display name.
- 'version string? - The participant's Zoom client version.
- video_quality string? - The participant's video quality score. The API only returns this value when the Meeting quality scores and network alerts on Dashboard setting is enabled in the Zoom Web Portal and the Show meeting quality score and network alerts on Dashboard option is selected in Account Settings:
good
— The video is almost flawless and the quality is excellent.fair
— The video definition is high, occasionally gets stuck, fast or slow, or other problems, but the frequency is very low and the video quality is good.poor
— The video definition is not high, but not many problems exist. The video quality is mediocre.bad
— The picture is very blurred and often gets stuck.
- bo_mtg_id string? - The breakout room ID. Each breakout room is assigned a unique ID.
zoom: MeetingPollsResponse
Poll List
Fields
- polls PollDetails[]? - Array of Polls
- total_records int? - The number of all records available across pages
zoom: MeetingSettings
Meeting settings
Fields
- allow_multiple_devices boolean? - Allow attendees to join the meeting from multiple devices. This setting only works for meetings that require registration.
- alternative_hosts string? - Alternative host's emails or IDs: multiple values are separated by a semicolon.
- alternative_hosts_email_notification boolean? - Flag to determine whether to send email notifications to alternative hosts, default value is true.
- approval_type int? - The default value is
2
. To enable registration required, set the approval type to0
or1
.0
- Automatically approve.1
- Manually approve.2
- No registration required.
- approved_or_denied_countries_or_regions ApprovedOrDeniedCountriesDetails? - Approve or block users from specific regions/countries from joining this meeting.
- audio string? - Determine how participants can join the audio portion of the meeting. both : Both Telephony and VoIP, telephony :Telephony only, voip:VoIP only.
- authentication_domains string? - If user has configured "Sign Into Zoom with Specified Domains" option, this will list the domains that are authenticated.
- authentication_exception ParticipantDetails[]? - The participants added here will receive unique meeting invite links and bypass authentication.
- authentication_name string? - Authentication name set in the authentication profile.
- authentication_option string? - Specify the authentication type for users to join a meeting with
meeting_authentication
setting set totrue
.
- auto_recording string? - Automatic recording:
local
- Record on local.cloud
- Record on cloud.none
- Disabled.
- breakout_room BreakoutRoomSettings? - Setting to pre-assign breakout rooms
- close_registration boolean? - Close registration after event date
- cn_meeting boolean? - Host meeting in China.
- contact_email string? - Contact email for registration
- contact_name string? - Contact name for registration
- custom_keys CustomKeys[]? - Custom keys and values assigned to the meeting.
- encryption_type string? - Choose between enhanced encryption and end-to-end encryption when starting or a meeting. When using end-to-end encryption, several features (e.g. cloud recording, phone/SIP/H.323 dial-in) will be automatically disabled. The value of this field can be one of the following:
enhanced_encryption
: Enhanced encryption. Encryption is stored in the cloud if you enable this option.e2ee
: End-to-end encryption. The encryption key is stored in your local device and can not be obtained by anyone else. Enabling this setting also disables the following features: join before host, cloud recording, streaming, live transcription, breakout rooms, polling, 1:1 private chat, and meeting reactions.
- enforce_login boolean? - Only signed in users can join this meeting. This field is deprecated and will not be supported in the future. As an alternative, use the "meeting_authentication", "authentication_option" and "authentication_domains" fields to understand the authentication configurations set for the meeting.
- enforce_login_domains string? - Only signed in users with specified domains can join meetings. This field is deprecated and will not be supported in the future. As an alternative, use the "meeting_authentication", "authentication_option" and "authentication_domains" fields to understand the authentication configurations set for the meeting.
- global_dial_in_countries string[]? - List of global dial-in countries
- global_dial_in_numbers GlobalDialInNumbersDetails[]? - Global Dial-in Countries/Regions
- host_video boolean? - Start video when the host joins the meeting.
- in_meeting boolean? - Host meeting in India.
- jbh_time int? - If the value of "join_before_host" field is set to true, this field can be used to indicate time limits within which a participant may join a meeting before a host. The value of this field can be one of the following:
0
: Allow participant to join anytime.5
: Allow participant to join 5 minutes before meeting start time.10
: Allow participant to join 10 minutes before meeting start time.
- join_before_host boolean? - Allow participants to join the meeting before the host starts the meeting. Only used for scheduled or recurring meetings.
- language_interpretation LanguageInterpretationDetails? - Language interpretation for meetings.
- meeting_authentication boolean? -
true
- Only authenticated users can join meetings.
- mute_upon_entry boolean? - Mute participants upon entry.
- participant_video boolean? - Start video when participants join the meeting.
- registrants_confirmation_email boolean? - Send confirmation email to registrants upon successful registration.
- registrants_email_notification boolean? - Send email notifications to registrants about approval, cancellation, denial of the registration. The value of this field must be set to true in order to use the
registrants_confirmation_email
field.
- registration_type int? - Registration type. Used for recurring meeting with fixed time only.
1
- Attendees register once and can attend any of the occurrences.2
- Attendees need to register for each occurrence to attend.3
- occurrences to attend.
- show_share_button boolean? - Show social share buttons on the meeting registration page. This setting only works for meetings that require registration.
- use_pmi boolean? - Use a personal meeting ID. Only used for scheduled meetings and recurring meetings with no fixed time.
- waiting_room boolean? - Enable waiting room
- watermark boolean? - Add watermark when viewing a shared screen.
zoom: MeetingSettingsInRequest
Meeting settings
Fields
- additional_data_center_regions string[]? - Enable additional data center regions for this meeting. Provide the value in the form of array of country code(s) for the countries which are available as data center regions
- allow_multiple_devices boolean? - If set to
true
, attendees will be allowed to join a meeting from multiple devices.
- alternative_hosts string? - Alternative host's emails or IDs: multiple values separated by a comma.
- alternative_hosts_email_notification boolean? - Flag to determine whether to send email notifications to alternative hosts, default value is true.
- approval_type int? - The default value is
2
. To enable registration required, set the approval type to0
or1
.0
- Automatically approve.1
- Manually approve.2
- No registration required.
- approved_or_denied_countries_or_regions ApprovalAndDenialInfo? - Approve or block users from specific regions/countries from joining this meeting.
- audio string? - Determine how participants can join the audio portion of the meeting. both : Both Telephony and VoIP, telephony :Telephony only, voip:VoIP only.
- authentication_domains string? - Meeting authentication domains. This option, allows you to specify the rule so that Zoom users, whose email address contains a certain domain, can join the meeting.
- authentication_option string? - Specify the authentication type for users to join a meeting with
meeting_authentication
setting set totrue
.
- auto_recording string? - Automatic recording: local - Record on local.cloud - Record on cloud.none - Disabled.
- breakout_room BreakoutRoomSettings? - Setting to pre-assign breakout rooms
- close_registration boolean? - Close registration after event date
- cn_meeting boolean? - Host meeting in China.
- contact_email string? - Contact email for registration
- contact_name string? - Contact name for registration
- encryption_type string? - Choose between enhanced encryption and end-to-end encryption. Values: enhanced_encryption, e2ee
- global_dial_in_countries string[]? - List of global dial-in countries
- host_video boolean? - Start video when the host joins the meeting.
- in_meeting boolean? - Host meeting in India.
- jbh_time int? - If the value of join_before_host field is set to true, this field can be used to indicate time limits within which a participant may join a meeting before a host. The value of this field can be one of the following: 0: Allow participant to join anytime. 5: Allow participant to join 5 minutes before meeting start time. 10: Allow participant to join 10 minutes before meeting start time.
- join_before_host boolean? - Allow participants to join the meeting before the host starts the meeting. This field can only used for scheduled or recurring meetings.
- language_interpretation LanguageInterpretationDetails? - Language interpretation for meetings.
- meeting_authentication boolean? - Only authenticated users can join meeting if the value of this field is set to
true
.
- mute_upon_entry boolean? - Mute participants upon entry.
- participant_video boolean? - Start video when participants join the meeting.
- registrants_email_notification boolean? - Send email notifications to registrants about approval, cancellation, denial of the registration. The value of this field must be set to true in order to use the
registrants_confirmation_email
field.
- registration_type int? - Registration type. Used for recurring meeting with fixed time only.
1
- Attendees register once and can attend any of the occurrences.2
- Attendees need to register for each occurrence to attend.3
- occurrences to attend.
- show_share_button boolean? - If set to
true
, the registration page for the meeting will include social share buttons.
- use_pmi boolean? - Use Personal Meeting ID instead of an automatically generated meeting ID. It can only be used for scheduled meetings, instant meetings and recurring meetings with no fixed time.
- waiting_room boolean? - Enable waiting room. Note that if the value of this field is set to
true
, it will override and disable thejoin_before_host
setting.
- watermark boolean? - Add watermark when viewing a shared screen.
zoom: MeetingTrackingDetails
Fields
- 'field string - Label of the tracking field.
- value string? - Tracking fields value
zoom: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://zoom.us/oauth/token") - Refresh URL
zoom: PaginationObject
Pagination Object
Fields
- next_page_token string? - The next page token is used to paginate through large result sets. A next page token will be returned whenever the set of available results exceeds the current page size. The expiration period for this token is 15 minutes.
- page_count int? - The number of pages returned for the request made.
- page_number int? - This field has been deprecated.
- page_size int? - The number of records returned with a single API call.
- total_records int? - The total number of all the records available across pages.
zoom: PartcipantDetails
Participant's details
Fields
- id string? - Universally unique identifier of the Participant. It is the same as the User ID of the participant if the participant joins the meeting by logging into Zoom. If the participant joins the meeting without logging in, the value of this field will be blank.
- name string? - Participant display name.
- user_email string? - Email address of the user. This field will be returned if the user logged into Zoom to join the meeting.
zoom: ParticipantDetails
Fields
- email string? - Email address of the participant.
- name string? - Name of the participant.
zoom: PastMeetingDetailsResponse
Fields
- duration int? - Meeting duration.
- end_time string? - Meeting end time (GMT).
- host_id string? - Host ID.
- id int? - Meeting ID: Unique identifier of the meeting in "long" format(represented as int64 data type in JSON), also known as the meeting number.
- participants_count int? - Number of meeting participants.
- start_time string? - Meeting start time (GMT).
- topic string? - Meeting topic.
- total_minutes int? - Sum of meeting minutes from all participants in the meeting.
- 'type int? - Meeting type.
- user_email string? - User email.
- user_name string? - User display name.
- uuid string? - Meeting UUID.
zoom: PollDetails
Fields
- id string? - ID of Poll
- status string? - Status of Poll:<br>
notstart
- Poll not started<br>started
- Poll started<br>ended
- Poll ended<br>sharing
- Sharing poll results
- questions PollQuestions[]? - Array of Polls
- title string? - Title for the poll.
zoom: PollQuestionAnswer
Answers submitted for poll questions
Fields
- answer string? - Answer submitted by the user.
- date_time string? - Date and time at which the answer to the poll was submitted.
- polling_id string? - Unique identifier of the poll.
- question string? - Question asked during the poll.
zoom: PollQuestions
Poll questions
Fields
- answers string[]? - Possible answers for the question.
- name string? - Name of the question.
- 'type string? - Question type:<br>
single
- Single choice<br>mutliple
- Multiple choice
zoom: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
zoom: ReccurenceDetails
Recurrence related meeting informations
Fields
- end_date_time string? - Select the final date on which the meeting will recur before it is canceled. Should be in UTC time, such as 2017-11-25T12:00:00Z. (Cannot be used with "end_times".)
- end_times int(default 1) - Select how many times the meeting should recur before it is canceled. (Cannot be used with "end_date_time".)
- monthly_day int(default 1) - Use this field only if you're scheduling a recurring meeting of type
3
to state which day in a month, the meeting should recur. The value range is from 1 to 31. For instance, if you would like the meeting to recur on 23rd of each month, provide23
as the value of this field and1
as the value of therepeat_interval
field. Instead, if you would like the meeting to recur every three months, on 23rd of the month, change the value of therepeat_interval
field to3
.
- monthly_week int? - Use this field only if you're scheduling a recurring meeting of type
3
to state the week of the month when the meeting should recur. If you use this field, you must also use themonthly_week_day
field to state the day of the week when the meeting should recur.-1
- Last week of the month.1
- First week of the month.2
- Second week of the month.3
- Third week of the month.4
- Fourth week of the month.
- monthly_week_day int? - Use this field only if you're scheduling a recurring meeting of type
3
to state a specific day in a week when the monthly meeting should recur. To use this field, you must also use themonthly_week
field.1
- Sunday.2
- Monday.3
- Tuesday.4
- Wednesday.5
- Thursday.6
- Friday.7
- Saturday.
- repeat_interval int? - Define the interval at which the meeting should recur. For instance, if you would like to schedule a meeting that recurs every two months, you must set the value of this field as
2
and the value of thetype
parameter as3
. For a daily meeting, the maximum interval you can set is90
days. For a weekly meeting the maximum interval that you can set is of12
weeks. For a monthly meeting, there is a maximum of3
months.
- 'type int - Recurrence meeting types:
1
- Daily.2
- Weekly.3
- Monthly.
- weekly_days string(default "1") - This field is required if you're scheduling a recurring meeting of type
2
to state which day(s) of the week the meeting should repeat. The value for this field could be a number between1
to7
in string format. For instance, if the meeting should recur on Sunday, provide"1"
as the value of this field. Note: If you would like the meeting to occur on multiple days of a week, you should provide comma separated values for this field. For instance, if the meeting should recur on Sundays and Tuesdays provide"1,3"
as the value of this field.1
- Sunday.2
- Monday.3
- Tuesday.4
- Wednesday.5
- Thursday.6
- Friday.7
- Saturday.
zoom: RegistrantCustomQuestion
Custom Question.
Fields
- title string? - Question title
- value string? - Question value
zoom: RegistrantDetails
Fields
- id string? - Registrant ID.
- address string? - Registrant's address.
- city string? - Registrant's city.
- comments string? - A field that allows registrants to provide any questions or comments that they might have.
- country string? - Registrant's country.
- custom_questions RegistrantCustomQuestion[]? - Custom questions.
- email string - A valid email address of the registrant.
- first_name string - Registrant's first name.
- industry string? - Registrant's Industry.
- job_title string? - Registrant's job title.
- last_name string? - Registrant's last name.
- no_of_employees string? - Number of Employees:
1-20
,21-50
,51-100
,101-500
,500-1,000
,1,001-5,000
,5,001-10,000
,More than 10,000
- org string? - Registrant's Organization.
- phone string? - Registrant's Phone number.
- purchasing_time_frame string? - This field can be included to gauge interest of webinar attendees towards buying your product or service.
Purchasing Time Frame:
Within a month``1-3 months``4-6 months``More than 6 months``No timeframe
- role_in_purchase_process string? - Role in Purchase Process:
Decision Maker
,Evaluator/Recommender
,Influencer
,Not involved
- state string? - Registrant's State/Province.
- zip string? - Registrant's Zip/Postal Code.
- create_time string? - The time at which the registrant registered.
- join_url string? - The URL using which an approved registrant can join the webinar.
- status string? - The status of the registrant's registration.
approved
: User has been successfully approved for the webinar.pending
: The registration is still pending.denied
: User has been denied from joining the webinar.
zoom: RegistrantQuestions
Meeting Registrant Questions
Fields
- custom_questions CustomQuestions[]? - Array of Registrant Custom Questions
- questions CustomRegistrantQuestions[]? - Array of Registrant Questions
zoom: RegistrantsList
Meeting Registrnats's Details
Fields
- registrants RegistrantDetails[]? - List of registrant objects.
zoom: RegistriantDetails
Registrant's details
Fields
- email string? - Registrant email
- id string? - Registrant Id
zoom: RequestedMeetingDetails
Meeting object
Fields
- agenda string? - Agenda
- created_at string? - The date and time at which this meeting was created.
- duration int? - Meeting duration.
- h323_password string? - H.323/SIP room system password
- join_url string? - URL for participants to join the meeting. This URL should only be shared with users that you would like to invite for the meeting.
- occurrences MeetingOccurenceDetails[]? - Array of occurrence objects.
- password string? - Meeting password. Password may only contain the following characters:
[a-z A-Z 0-9 @ - _ * !]
If "Require a password when scheduling new meetings" setting has been enabled and locked for the user, the password field will be autogenerated in the response even if it is not provided in the API request.
- pmi int? - Personal Meeting Id. Only used for scheduled meetings and recurring meetings with no fixed time.
- recurrence ReccurenceDetails? - Recurrence related meeting informations
- settings MeetingSettings? - Meeting settings
- start_time string? - Meeting start date-time in UTC/GMT. Example: "2020-03-31T12:02:00Z"
- start_url string? - URL to start the meeting. This URL should only be used by the host of the meeting and should not be shared with anyone other than the host of the meeting as anyone with this URL will be able to login to the Zoom Client as the host of the meeting.
- timezone string? - Timezone to format start_time
- topic string? - Meeting topic
- tracking_fields RequestedMeetingTrackingDetails[]? - Tracking fields
- 'type int? - Meeting Type
zoom: RequestedMeetingTrackingDetails
Fields
- 'field string? - Label of the tracking field.
- value string? - Value for the field.
- visible boolean? - Indicates whether the tracking field is visible in the meeting scheduling options in the Zoom Web Portal or not.
true
: Tracking field is visible.false
: Tracking field is not visible to the users in the meeting options in the Zoom Web Portal but the field was used while scheduling this meeting via API. An invisible tracking field can be used by users while scheduling meetings via API only.
zoom: TemplateDetails
Template details
Fields
- id string? - Unique identifier of the template.
- name string? - Name of the template.
- 'type int? - Type of the template. The value of this field can be one of the following:<br>
1
: meeting template <br>2
: admin meeting template
zoom: TrackingFields
Fields
- 'field string? - Tracking fields type
- value string? - Tracking fields value
zoom: UpdateMeetingLiveStreamDetailsRequest
Meeting live stream.
Fields
- page_url string? - The livestream page URL.
- stream_key string - Stream name and key.
- stream_url string - Streaming URL.
zoom: UpdateMeetingPollRequest
Poll
Fields
- questions PollQuestions[]? - Array of Polls
- title string? - Title for the poll.
zoom: UpdateMeetingRegistrantstatusRequest
Meeting registrant status update details
Fields
- action string - Registrant Status:<br>
approve
- Approve registrant.<br>cancel
- Cancel previously approved registrant's registration.<br>deny
- Deny registrant.
- registrants RegistriantDetails[]? - List of registrants.
zoom: UpdateMeetingRequest
Fields
- schedule_for string? - Email or userId if you want to schedule meeting for another user.
- agenda string? - Meeting description.
- duration int? - Meeting duration (minutes). Used for scheduled meetings only.
- password string? - Meeting passcode. Passcode may only contain the following characters: [a-z A-Z 0-9 @ - _ *] and can have a maximum of 10 characters. Note: If the account owner or the admin has configured minimum passcode requirement settings, the passcode value provided here must meet those requirements. <br><br>If the requirements are enabled, you can view those requirements by calling either the Get User Settings API or the Get Account Settings API.
- recurrence ReccurenceDetails? - Recurrence related meeting informations
- settings MeetingSettings? - Meeting settings
- start_time string? - Meeting start time. When using a format like "yyyy-MM-dd'T'HH:mm:ss'Z'", always use GMT time. When using a format like "yyyy-MM-dd'T'HH:mm:ss", you should use local time and specify the time zone. Only used for scheduled meetings and recurring meetings with a fixed time.
- template_id string? - Unique identifier of the meeting template. Use this field if you would like to schedule the meeting from a meeting template. You can retrieve the value of this field by calling the List meeting templates API.
- topic string? - Meeting topic.
- tracking_fields TrackingFields[]? - Tracking fields
- 'type int? - Meeting Types:<br>
1
- Instant meeting.<br>2
- Scheduled meeting.<br>3
- Recurring meeting with no fixed time.<br>8
- Recurring meeting with a fixed time.
zoom: UpdateMeetingstatusRequest
Fields
zoom: UserDetails
User Details
Fields
- created_at string? - The time at which the user's account was created.
- custom_attributes CustomAttributeDetails[]? - Information about the user's custom attributes. This field is only returned if users are assigned custom attributes and you provided the
custom_attributes
value for theinclude_fields
query parameter in the API request.
- dept string? - The user's department.
- email string - The user's email address.
- employee_unique_id string? - The employee's unique ID. The this field only returns when SAML single sign-on (SSO) is enabled or the
login_type
value is101
(SSO).
- first_name string? - The user's first name.
- group_ids string[]? - The IDs of groups where the user is a member.
- id string? - The user's ID. The API does not return this value for users with the
pending
status.
- im_group_ids string[]? - The IDs of IM directory groups where the user is a member.
- last_client_version string? - The last client version that user used to log in.
- last_login_time string? - The user's last login time. This field has a three-day buffer period. For example, if user first logged in on
2020-01-01
and then logged out and logged in on2020-01-02
, this value will still reflect the login time of2020-01-01
. However, if the user logs in on2020-01-04
, the value of this field will reflect the corresponding login time since it exceeds the three-day buffer period.
- last_name string? - The user's last name.
- plan_united_type string? - This field is returned if the user is enrolled in the Zoom United plan.
- pmi int? - The user's Personal Meeting ID (PMI).
- status string? - The user's status:
active
— An active user.inactive
— A deactivated user.pending
— A pending user.
- timezone string? - The user's timezone.
- 'type int - The user's assigned plan type:
1
— Basic.2
— Licensed.3
— On-prem.99
— None (this can only be set withssoCreate
).
- verified int? - Display whether the user's email address for the Zoom account is verified:
1
— A verified user email.0
— The user's email not verified.
zoom: UsersList
Users Details
Fields
- users UserDetails[]? - Information about the users.
zoom: UserSubmittedAnswers
Answers submitted by users
Fields
- email string? - Email address of the user who submitted answers to the poll.
- name string? - Name of the user who submitted answers to the poll. If "anonymous" option is enabled for a poll, the participant's polling information will be kept anonymous and the value of
name
field will be "Anonymous Attendee".
- question_details PollQuestionAnswer[]? - Poll question answers
Union types
Import
import ballerinax/zoom;
Metadata
Released date: over 1 year ago
Version: 1.7.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 2443
Current verison: 298
Weekly downloads
Keywords
Communication/Video Conferencing
Cost/Freemium
Contributors