zoho.people
Module zoho.people
API
Definitions
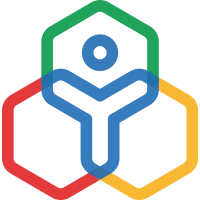
ballerinax/zoho.people Ballerina library
Overview
This is a generated connector for Zoho People OpenAPI specification.
Zoho People API provides the capability to integrate HR modules with third-party applications. With Zoho People API, you can extract employees' data and form data in XML or JSON format to develop new applications or integrate with your existing business applications.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Zoho People account.
- Obtain tokens by following this guide.
Quickstart
To use the Zoho People connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/zoho.people
module into the Ballerina project.
import ballerinax/zoho.people;
Step 2 - Create a new connector instance
You can now make the connection configuration using the <ACCESS_TOKEN>.
people:ClientConfig clientConfig = { auth : { token: `<ACCESS_TOKEN>` } }; people:Client zohoPeople = check new (clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example to get leave types of a specific employee.
public function main() { userId = <USER_ID> people:LeaveTypes|error leaveTypes = zohoPeople->getLeaveTypes(userId); if leaveTypes is error { log:printError(leaveTypes.toString()); } else { log:printInfo(leaveTypes.toString()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
zoho.people: Client
This is a generated connector for Zoho People OpenAPI specification. Zoho People API provides the capability to integrate HR modules with third-party applications. With Zoho People API, you can extract employees' data and form data in XML or JSON format to develop new applications or integrate with your existing business applications.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Zoho People account and obtain tokens by following this guide.
init (ClientConfig clientConfig, string serviceUrl)
- clientConfig ClientConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://people.zoho.com/people/api" - URL of the target service
insertRecord
function insertRecord(string inputType, string formLinkName, InputData payload) returns FormRecordResponse|error
Add a record to the form.
Parameters
- inputType string - Type of inputs(json or xml)
- formLinkName string - Form Lable Name
- payload InputData - Input data in stringify JSON format or XML format
Return Type
- FormRecordResponse|error - Success
updateRecord
function updateRecord(string inputType, string formLinkName, int recordId, InputData payload) returns FormRecordResponse|error
Modify the field values of the specified record.
Parameters
- inputType string - Type of inputs(json or xml)
- formLinkName string - Form Lable Name
- recordId int - Specifies the record Id of the record being fetched.
- payload InputData - Input data in stringify JSON format or XML format
Return Type
- FormRecordResponse|error - Success
addDepartment
function addDepartment(string xmlData) returns DepartmentResponse|error
Add various departments one by one
Parameters
- xmlData string - Input data in stringify XML format
Return Type
- DepartmentResponse|error - Success
getBulkRecords
function getBulkRecords(string formLinkName, int? sIndex, int? 'limit, string? searchColumn, string? searchValue, int? modifiedtime) returns Records|error
Fetch bulk records along with its tabular section details from particular forms. (maximum:200 records per api calls)
Parameters
- formLinkName string - Form Lable Name
- sIndex int? (default ()) - starting index of the records to fetch (record index starts from 1)
- 'limit int? (default ()) - number of records to be fetched in the current request (maximum 200 records)
- searchColumn string? (default ()) - Search EMPLOYEEID or EMPLOYEEMAILALIAS
- searchValue string? (default ()) - Search Employeeid of the employee or the Employee MailID
- modifiedtime int? (default ()) - To fetch records added & modified after the given time . value should be timestamp in milliseconds
getSingleRecord
Display the record of a specific form indexed using a Record ID
Parameters
- formLinkName string - Form Lable Name
- recordId int - Specifies the record Id of the record being fetched. The record Id can be identified using the getBulkRecords operation.
getRecordByID
Fetch all the record of a specific form indexed using a Record ID by section-wise
Parameters
- formLinkName string - Form Lable Name
- recordId int - Specifies the record Id of the record being fetched. The record Id can be identified using the getBulkRecords operation.
getForms
function getForms() returns FormDetailResponse|error
Retrieve the list of forms and its details available in your Zoho People account.
Return Type
- FormDetailResponse|error - Success
addLeave
function addLeave(InputData payload) returns FormRecordResponse|error
Add leave records using Add Leave API
Parameters
- payload InputData - Input data in stringify JSON format
Return Type
- FormRecordResponse|error - Success
addLeaveBalance
function addLeaveBalance(BalanceData payload, string? dateFormat) returns BalanceDataResponse|error
To modify an employee's leave balance
Parameters
- payload BalanceData - Balance data to insert in Json string format
- dateFormat string? (default ()) - Specify the date format (Org date format will be considered if nothing is specified)
Return Type
- BalanceDataResponse|error - Success
getSingleLeaveRecord
function getSingleLeaveRecord(int recordId) returns LeaveDetail|error
Fetch the record of leave from indexed using a Record ID
Parameters
- recordId int - Specifies the record Id of the leave record being fetched. The record Id can be identified using the fetch Records API.
Return Type
- LeaveDetail|error - Success
getLeaveTypes
function getLeaveTypes(string userId) returns LeaveTypes|error
Get leave types of a specific employee
Parameters
- userId string - Employee Id/Employee MailId/Record Id of the Employee
Return Type
- LeaveTypes|error - Success
getHolidays
Fetch specific holidays of any employees using their employee ID, email ID and record ID parameters.
Parameters
- userId string - Employee Id/Employee MailId/Record Id of the Employee
getAllHolidays
function getAllHolidays(string? location, string? shift, string? employee, boolean? upcoming, string? 'from, string? to, string? dateFormat) returns AllHolidays|error
Fetch the holidays of a location, shift or for an employee.
Parameters
- location string? (default ()) - Location name
- shift string? (default ()) - Shift name
- employee string? (default ()) - Emp ID or Email ID or Erecno of the employee
- upcoming boolean? (default ()) - To get the holidays for the next 365 days
- 'from string? (default ()) - From date
- to string? (default ()) - To date
- dateFormat string? (default ()) - Date format used to specify From and To as String
Return Type
- AllHolidays|error - Success
getLeaveReportOfUser
function getLeaveReportOfUser(int employee, string? to) returns LeaveReport|error
Get user report for current leave year.
Parameters
- employee int - Erecno of the employee
- to string? (default ()) - Report till date of the current year
Return Type
- LeaveReport|error - Success
addCase
function addCase(int categoryId, string subject, string? description) returns CreatedCase|error
Add case
Parameters
- categoryId int - Specify the category ID
- subject string - Specify the subject of the case
- description string? (default ()) - Specify the description of the case
Return Type
- CreatedCase|error - Success
viewCaseDetails
function viewCaseDetails(int recordId) returns CaseDetailsResponse|error
View case details
Parameters
- recordId int - Specify the record ID of the case
Return Type
- CaseDetailsResponse|error - Success
getAllCases
function getAllCases(int index, string? status, string? categoryId, string? query, string? requestorErecno, int? periodOfTime) returns CaseList|error
List all case details
Parameters
- index int - Specify the index of the listing
- status string? (default ()) - String of status IDs separated by (,) comma - (Open- "1", In Progress- "2", Awaiting action from Requestor- "3", On Hold- "4", Closed- "5") - empty for all status
- categoryId string? (default ()) - Category IDs separated by (,) comma
- query string? (default ()) - Specify the query string of the case
- requestorErecno string? (default ()) - Employee record IDs separated by (,) comma
- periodOfTime int? (default ()) - Send single time limit(Today - 0, Yesterday - 1, Past 7 days - 2, Past 30 days - 3)
viewListOfCategories
function viewListOfCategories() returns CaseCategory|error
List categories that a user case raise query to
Return Type
- CaseCategory|error - Success
triggerOnboarding
function triggerOnboarding(string onboardingType, int userId) returns OnboardingResponse|error
Trigger onboarding for an existing candidate or employee
Parameters
- onboardingType string - Select either one type for onboarding -
Employee
orCandidate
- userId int - Specify the record ID of the candidate employee
Return Type
- OnboardingResponse|error - Success
importBulkAttendance
function importBulkAttendance(AttendanceImportData payload, string? dateFormat) returns BulkAttendance|error
Import bulk check-in and check-out details of the employees
Parameters
- payload AttendanceImportData - Input data in stringify JSON array format
- dateFormat string? (default ()) - Format for date (Eg- yyyy-MM-dd HH:mm:ss)
Return Type
- BulkAttendance|error - Success
createAttendance
function createAttendance(string dateFormat, string? checkIn, string? checkOut, string? empId, string? emailId, string? mapId) returns AttendanceEntry[]|error
Capture the check-in and check-out entries of an individual employee
Parameters
- dateFormat string - Specify the correct date format(eg- dd/MM/yyyy HH:mm:ss )
- checkIn string? (default ()) - Specify the check-in time of an employee
- checkOut string? (default ()) - Specify the check-out time of an employee
- empId string? (default ()) - Specify the employee ID of an employee. Out of the 3 parameters - empId, emailId & mapId, at least one of them must be given as an input to map the entry of an employee.
- emailId string? (default ()) - Specify the email ID of an employee. Out of the 3 parameters - empId, emailId & mapId, at least one of them must be given as an input to map the entry of an employee.
- mapId string? (default ()) - Specify the mapper ID of an employee. Out of the 3 parameters - empId, emailId & mapId, at least one of them must be given as an input to map the entry of an employee.
Return Type
- AttendanceEntry[]|error - Success
getAttendanceEntries
function getAttendanceEntries(string? dateFormat, string? date, string? erecno, string? empId, string? emailId, string? mapId) returns AttendanceEntries|error
Get the employee attendance entries.
Parameters
- dateFormat string? (default ()) - Specify the response date format
- date string? (default ()) - Specify the date in organisation date format
- erecno string? (default ()) - Specify employee erecno
- empId string? (default ()) - Specify employee id. Out of the 3 parameters - empId, emailId & mapId, at least one of them must be given as an input to map the entry of an employee.
- emailId string? (default ()) - Specify employee email id. Out of the 3 parameters - empId, emailId & mapId, at least one of them must be given as an input to map the entry of an employee.
- mapId string? (default ()) - Specify mapper id. Out of the 3 parameters - empId, emailId & mapId, at least one of them must be given as an input to map the entry of an employee.
Return Type
- AttendanceEntries|error - Success
getLatestAttendance
function getLatestAttendance(int duration, string? dateTimeFormat) returns LatestAttendance|error
Get the latest attendance entries with Regularisation entries that has been added/updated within the latest minutes(given in param)
Parameters
- duration int - Duration in minutes within which the latest entries should be fetched
- dateTimeFormat string? (default ()) - Format of the date and time of Attendance entries in response is returned
Return Type
- LatestAttendance|error - Success
updateUserShift
function updateUserShift(string empId, string shiftName, string? fdate, string? tdate, string? dateFormat) returns ShiftUpdate|error
Map employees to respective shifts
Parameters
- empId string - Employee ID of an employee
- shiftName string - Shift to be mapped
- fdate string? (default ()) - From date
- tdate string? (default ()) - To date
- dateFormat string? (default ()) - Correct date format. Date Format of organisation or specify the format
Return Type
- ShiftUpdate|error - Success
getShiftDetails
function getShiftDetails(string sdate, string edate, string? empId, string? emailId, string? mapId) returns ShiftDetails|error
Fetch the shift configuration details of an employee
Parameters
- sdate string - Start date
- edate string - End date
- empId string? (default ()) - Employee ID of an employee. Out of the 3 parameters - empId, emailId & mapId, at least one of them must be given as an input to map the entry of an employee.
- emailId string? (default ()) - Mail ID of the employee. Out of the 3 parameters - empId, emailId & mapId, at least one of them must be given as an input to map the entry of an employee.
- mapId string? (default ()) - Mapper ID of the employee. Out of the 3 parameters - empId, emailId & mapId, at least one of them must be given as an input to map the entry of an employee.
Return Type
- ShiftDetails|error - Success
Records
zoho.people: Agent
Fields
- empid string? -
- allowPickup boolean? -
- valueId string? -
- erecno string? -
- employeePhoto string? -
- name string? -
- details string? -
- typeId string? -
- 'type string? -
- zuid string? -
- employeeEmailAlias string? -
zoho.people: AllHolidays
Fields
- data Holiday[]? -
- message string? -
- uri string? -
- status int? -
- error_msg string? -
- error_code int? -
zoho.people: AttendanceEntries
Fields
- firstIn string? -
- totalHrs string? -
- entries AttendanceEntryInfo[]? -
- lastOut_Location string? -
- lastOut string? -
- allowedToCheckIn boolean? -
- firstIn_Location string? -
- status string? -
zoho.people: AttendanceEntry
Fields
- inputType_in int? -
- inputType_out int? -
- tdate string? -
- fdate string? -
- punchIn string? -
- dateFormat string? -
- response string? -
- tsecs int? -
- msg string? -
zoho.people: AttendanceEntryInfo
Fields
- checkIn string? -
- checkOut_Location string? -
- sourceOfPunchOut string? -
- checkIn_Location string? -
- checkOut string? -
- sourceOfPunchIn string? -
zoho.people: AttendanceImportData
Fields
- data string -
zoho.people: BalanceData
Fields
- balanceData string -
zoho.people: BalanceDataResponse
Fields
- response BalancedataresponseResponse? -
zoho.people: BalancedataresponseResponse
Fields
- result record {}? -
- message string? -
- uri string? -
- status int? -
- errors record {}|record {}[]? -
zoho.people: BulkAttendance
Fields
- response string? -
zoho.people: CaseCategory
Fields
- response CasecategoryResponse? -
zoho.people: CasecategoryResponse
Fields
- result CaseCategoryResult[]? -
- message string? -
- uri string? -
- status int? -
zoho.people: CaseCategoryResult
Fields
- isAgent boolean? -
- categoryIcon string? -
- isEnabled boolean? -
- subCategoryList SubCategory[]? -
- categoryShortDescription string? -
- enable_moderation boolean? -
- serviceId string? -
- applicableLocations anydata[]? -
- categoryName string? -
- categoryId string? -
zoho.people: CaseDetail
Fields
- sourceId string? -
- agentId string? -
- agent Agent? -
- permittedCaseStatus PermittedCaseStatus[]? -
- permittedCasePriority PermittedCasePriority[]? -
- subject string? -
- description string? -
- 'source string? -
- categoryName string? -
- priorityId string? -
- isAgent boolean? -
- caseId string? -
- createdTime string? -
- permittedCaseSource PermittedCaseSource[]? -
- isEscalatedUser boolean? -
- agentValueId string? -
- agentTypeId string? -
- priority string? -
- requestor Requestor? -
- agentDetails string? -
- statusId string? -
- categoryIcon string? -
- categoryId string? -
- status string? -
zoho.people: CaseDetails
Fields
- assignedToList boolean? -
- filesList FileList[]? -
- caseDetails CaseDetail? -
- needCategoryChange boolean? -
- sla SLA? -
zoho.people: CaseDetailsResponse
Fields
- response CasedetailsresponseResponse? -
zoho.people: CasedetailsresponseResponse
Fields
- result CaseDetails? -
- message string? -
- uri string? -
- status int? -
- errors CaseErrors? -
zoho.people: CaseErrors
Fields
- desc string? -
- status string? -
zoho.people: CaseList
Fields
- response CaselistResponse? -
zoho.people: CaselistResponse
Fields
- result CaselistResponseResult? -
- message string? -
- uri string? -
- status int? -
zoho.people: CaselistResponseResult
Fields
- hrcaseList HrcaseList[]? -
- isNextAvailable boolean? -
zoho.people: ClientConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion string(default "1.1") - The HTTP version understood by the client
- http1Settings ClientHttp1Settings(default {}) - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings(default {}) - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- followRedirects FollowRedirects?(default ()) - Configurations associated with Redirection
- poolConfig PoolConfiguration?(default ()) - Configurations associated with request pooling
- cache CacheConfig(default {}) - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig?(default ()) - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig?(default ()) - Configurations associated with retrying
- cookieConfig CookieConfig?(default ()) - Configurations associated with cookies
- responseLimits ResponseLimitConfigs(default {}) - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket?(default ()) - SSL/TLS-related options
zoho.people: CreatedCase
Fields
- response CreatedcaseResponse? -
zoho.people: CreatedcaseResponse
Fields
- result CreatedcaseResponseResult? -
- message string? -
- uri string? -
- status int? -
- errors CaseErrors? -
zoho.people: CreatedcaseResponseResult
Fields
- zp_recordId string? -
- caseId string? -
- desc string? -
- status string? -
zoho.people: DepartmentResponse
Fields
- Response int? -
- pkId string? -
- code int? -
- message string|record {}|record {}[]? -
- errorcode int? -
- 'Response\ status int? -
zoho.people: Errors
Fields
- code int? -
- message string? -
zoho.people: Fields
Fields
- comptype string? -
- Options record {}? -
- description string? -
- labelname string? -
- ismandatory boolean? -
- displayType string? -
- ReferedFormId int? -
- isPrimary boolean? -
- displayname string? -
- autofillvalue string? -
- formcomponentid int? -
- ReferedFormName string? -
- ReferedFieldId int? -
- ReferedFieldName string? -
- maxLength int? -
zoho.people: FileList
Fields
- fileName string? -
- downloadUrl string? -
- filesize string? -
- fileUploadId string? -
zoho.people: FormDetail
Fields
- componentId int? -
- iscustom boolean? -
- displayName string? -
- formLinkName string? -
- PermissionDetails PermissionDetails? -
- isVisible boolean? -
- viewDetails ViewDetails? -
zoho.people: FormDetailResponse
Fields
- response FormdetailresponseResponse? -
zoho.people: FormdetailresponseResponse
Fields
- result FormDetail[]? -
- message string? -
- uri string? -
- status int? -
- errors record {}|record {}[]? -
zoho.people: FormFields
Fields
- response FormfieldsResponse? -
zoho.people: FormfieldsResponse
Fields
- result Fields[]? -
- message string? -
- uri string? -
- status int? -
- errors Errors? -
zoho.people: FormRecordResponse
Fields
- response FormrecordresponseResponse? -
zoho.people: FormrecordresponseResponse
Fields
- result FormrecordresponseResponseResult? -
- message string? -
- uri string? -
- status int? -
- errors record {}|record {}[]? -
zoho.people: FormrecordresponseResponseResult
Fields
- pkId string? -
- message string? -
zoho.people: Holiday
Fields
- isRestrictedHoliday boolean? -
- ShiftName string? -
- Remarks string? -
- LocationId string? -
- ShiftId string? -
- Date string? -
- isHalfday boolean? -
- Name string? -
- LocationName string? -
- Session int? -
- fromDate string? -
- toDate string? -
zoho.people: Holidays
Fields
- response HolidaysResponse? -
zoho.people: HolidaysResponse
Fields
- result Holiday[]? -
- message string? -
- uri string? -
- status int? -
- error_msg string? -
- error_code int? -
- errors Errors? -
zoho.people: HrcaseList
Fields
- agent Agent? -
- subject string? -
- SLA SLA? -
- priority string? -
- categoryName string? -
- requestor Requestor? -
- priorityId string? -
- recordId string? -
- statusId string? -
- caseId string? -
- createdTime string? -
- categoryId string? -
- status string? -
- hasAttachment boolean? -
zoho.people: InputData
Fields
- inputData string -
zoho.people: LatestAttendance
Fields
- response LatestattendanceResponse? -
zoho.people: LatestAttendanceEntries
Fields
- firstName string? -
- lastName string? -
- entries record {}[]? -
- erecNo int? -
- employeeId string? -
- emailId string? -
zoho.people: LatestattendanceResponse
Fields
- result LatestAttendanceEntries[]? -
- message string? -
- uri string? -
- status string? -
zoho.people: Leave
Fields
- Employee_ID string? -
- DayDetails record {}? -
- 'Leavetype\.ID string? -
- From string? -
- Unit string? -
- ApprovalStatus string? -
- Daystaken string? -
- Reasonforleave string? -
- TeamEmailID string? -
- Leavetype string? -
- To string? -
- 'Employee\_ID\.ID string? -
- DateOfRequest string? -
zoho.people: LeaveDetail
Fields
- response LeavedetailResponse? -
zoho.people: LeavedetailResponse
Fields
- result Leave[]? -
- message string? -
- uri string? -
- status int? -
- errors Errors? -
zoho.people: LeaveInfo
Fields
- taken int? -
- leavetypeName string? -
- available int? -
- 'type string? -
- leavetypeColor string? -
- leavetypeID int? -
zoho.people: LeaveReport
Fields
- employeeName string? -
- employeeId string? -
- leavetypes LeaveInfo[]? -
zoho.people: LeaveType
Fields
- Name string? -
- PermittedCount int? -
- AvailedCount int? -
- Unit string? -
zoho.people: LeaveTypes
Fields
- response LeavetypesResponse? -
zoho.people: LeavetypesResponse
Fields
- result LeaveType[]? -
- message string? -
- uri string? -
- status int? -
- error_msg string? -
- error_code int? -
- errors Errors? -
zoho.people: OnboardingResponse
Fields
- msg string? -
- Success boolean? -
zoho.people: PermissionDetails
Fields
- Add int? -
- Edit int? -
- View int? -
zoho.people: PermittedCasePriority
Fields
- sequence string? -
- optionValue string? -
- fcid string? -
- optionId string? -
- systemValue string? -
zoho.people: PermittedCaseSource
Fields
- sequence string? -
- isReopenDisabled boolean? -
- optionValue string? -
- fcid string? -
- optionId string? -
- systemValue string? -
zoho.people: PermittedCaseStatus
Fields
- sequence string? -
- optionValue string? -
- fcid string? -
- optionId string? -
- systemValue string? -
zoho.people: Records
Fields
- response RecordsResponse? -
zoho.people: RecordsResponse
Fields
- result record {}[]? -
- message string? -
- uri string? -
- status int? -
- errors record {}|record {}[]? -
zoho.people: Requestor
Fields
- empid string? -
- erecno string? -
- employeePhoto string? -
- name string? -
- zuid string? -
- employeeEmailAlias string? -
zoho.people: ShiftDetails
Fields
- userShiftDetails UserShiftDetails? -
zoho.people: ShiftList
Fields
- date string? -
- shiftId string? -
- shiftName string? -
- shiftColor string? -
- shiftStartTime string? -
- shiftEndTime string? -
- isWeekend boolean? -
zoho.people: ShiftUpdate
Fields
- result ShiftupdateResult? -
- msg string? -
zoho.people: ShiftupdateResult
Fields
- msg string? -
zoho.people: SLA
Fields
- nextViolation string? -
- currentStatus int? -
- isViolated boolean? -
zoho.people: SubCategory
Fields
- subcategoryname string? -
- subCategoryId string? -
zoho.people: UserShiftDetails
Fields
- employeeName string? -
- erecno string? -
- employeePhoto string? -
- locationId string? -
- emailId string? -
- employeeId string? -
- isShiftEditable boolean? -
- shiftList ShiftList[]? -
zoho.people: ViewDetails
Fields
- view_Id int? -
- view_Name string? -
String types
zoho.people: OnboardingType
OnboardingType
Import
import ballerinax/zoho.people;
Metadata
Released date: about 3 years ago
Version: 1.0.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.0.2
GraalVM compatible: Yes
Pull count
Total: 1346
Current verison: 801
Weekly downloads
Keywords
Human Resources/HRMS
Cost/Paid
Contributors