zoho.crm.rest
Module zoho.crm.rest
API
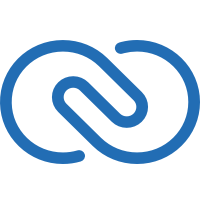
ballerinax/zoho.crm.rest Ballerina library
Overview
This is a generated connector for Zoho CRM REST OpenAPI specification.
Zoho CRM REST API provides the capability to unify customer data from across different applications and databases.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Zoho CRM account.
- Obtain tokens by following this guide.
Quickstart
To use the Zoho CRM REST connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/zoho.crm.rest
module into the Ballerina project.
import ballerinax/zoho.crm.rest;
Step 2 - Create a new connector instance
You can now make the connection configuration using the <ACCESS_TOKEN>.
rest:ClientConfig clientConfig = { auth : { token: `<ACCESS_TOKEN>` } }; rest:Client zohoClient = check new (clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example to add a record in Zoho.
public function main() returns error? { Response response = check zohoClient->addRecord("contacts", {data:[{Last_Name: "NC"}]}); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
zoho.crm.rest: Client
This is a generated connector for Zoho CRM OpenAPI specification. Zoho CRM REST API provides the capability to unify customer data from across different applications and databases.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Zoho account and obtain tokens.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://www.zohoapis.com/crm/v2" - URL of the target service
listRecords
function listRecords(string moduleApiName) returns RecordsData|error
Get the list of available records from a module.
Parameters
- moduleApiName string - Module name. Leads, Accounts, Contacts, Deals, Campaigns, Tasks, Cases, Events, Calls, Solutions, Products, Vendors, Price Books, Quotes, Sales Orders, Purchase Orders, Invoices, Activities, and custom modules.
Return Type
- RecordsData|error - Records
updateRecord
Update existing record in the module.
Parameters
- moduleApiName string - Module name. Leads, Accounts, Contacts, Deals, Campaigns, Tasks, Cases, Events, Calls, Solutions, Products, Vendors, Price Books, Quotes, Sales Orders, Purchase Orders, Invoices, Activities, and custom modules.
- payload json - Record
addRecord
Add new records to a module.
Parameters
- moduleApiName string - Module name. Leads, Accounts, Contacts, Deals, Campaigns, Tasks, Cases, Events, Calls, Solutions, Products, Vendors, Price Books, Quotes, Sales Orders, Purchase Orders, Invoices, Activities, and custom modules.
- payload json - Record
getRecord
function getRecord(string moduleApiName, string recordId) returns RecordsData|error?
Get a record from a module.
Parameters
- moduleApiName string - Module name. Leads, Accounts, Contacts, Deals, Campaigns, Tasks, Cases, Events, Calls, Solutions, Products, Vendors, Price Books, Quotes, Sales Orders, Purchase Orders, Invoices, Activities, and custom modules.
- recordId string - Record ID
Return Type
- RecordsData|error? - Record
deleteRecord
Delete existing record in the module.
Parameters
- moduleApiName string - Module name. Leads, Accounts, Contacts, Deals, Campaigns, Tasks, Cases, Events, Calls, Solutions, Products, Vendors, Price Books, Quotes, Sales Orders, Purchase Orders, Invoices, Activities, and custom modules.
- recordId string - Record ID
searchRecords
function searchRecords(string moduleApiName, string? criteria, string? email, string? phone, string? word, string? converted, string? approved, int? page, int? perPage) returns RecordsData|error?
Retrieve the records that match your search criteria.
Parameters
- moduleApiName string - Module name. Leads, Accounts, Contacts, Deals, Campaigns, Tasks, Cases, Events, Calls, Solutions, Products, Vendors, Price Books, Quotes, Sales Orders, Purchase Orders, Invoices, Activities, and custom modules.
- criteria string? (default ()) - Performs search by following the shown criteria
- email string? (default ()) - Performs module search by email. All the email fields of a particular module will be searched and listed.
- phone string? (default ()) - Performs module search by phone number. All the phone fields of a particular module will be searched and listed.
- word string? (default ()) - Performs global search by word.
- converted string? (default ()) - To get the list of converted records. Default value is false.
- approved string? (default ()) - To get the list of approved records. Default value is true.
- page int? (default ()) - To get the list of records from the respective pages. Default value for page is 1.
- perPage int? (default ()) - To get the list of records available per page. The default and the maximum possible value is 200.
Return Type
- RecordsData|error? - Records
convertLead
function convertLead(string recordId, LeadData payload) returns ConvertLeadResponse|error
Convert a lead into a contact or an account.
Return Type
- ConvertLeadResponse|error - Connections
Records
zoho.crm.rest: By
Fields
- name string? -
- id string? -
zoho.crm.rest: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
zoho.crm.rest: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth OAuth2ClientCredentialsGrantConfig|BearerTokenConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
zoho.crm.rest: ConvertLeadResponse
Fields
- data LeadResponse[]? -
zoho.crm.rest: Lead
Fields
- overwrite boolean? - Specify if the Lead details must be overwritten in the Contact/Account/Deal based on lead conversion mapping configuration.
- notify_lead_owner boolean? - Specify whether the lead owner must get notified about the lead conversion via email.
- notify_new_entity_owner boolean? - Specify whether the user to whom the contact/account is assigned to must get notified about the lead conversion via email.
- Accounts string? - Use this key to associate an account with the lead being converted. Pass the unique and valid account ID.
- Contacts string? - Use this key to associate a contact with the lead being converted. Pass the unique and valid contact ID.
- assign_to string? - Use this key to assign record owner for the new contact and account. Pass the unique and valid user ID.
- Deals LeadDeals? -
- carry_over_tags record {}? -
zoho.crm.rest: LeadData
Fields
- data Lead[]? -
zoho.crm.rest: LeadDeals
Fields
- Deal_Name string -
- Closing_Date string -
- Stage string -
zoho.crm.rest: LeadResponse
Fields
- Contacts string? -
- Deals string? -
- Account string? -
zoho.crm.rest: OAuth2ClientCredentialsGrantConfig
OAuth2 Client Credentials Grant Configs
Fields
- Fields Included from *OAuth2ClientCredentialsGrantConfig
- tokenUrl string(default "https://accounts.zoho.com/oauth/v2/token") - Token URL
zoho.crm.rest: Owner
Represents the name, ID, and email ID of the record owner.
Fields
- name string? - Name
- id string? - Owner ID
- email string? - Email
zoho.crm.rest: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
zoho.crm.rest: Record
Fields
- Owner Owner? - Represents the name, ID, and email ID of the record owner.
- '\$currency\_symbol string? - Represents the symbol of the currency of the organization. For instance, '₹'.
- '\$process\_flow boolean? - Represents if the record is a part of review process.
- Exchange_Rate int? - Represents the exchange rate set for the organization.
- Currency string? - Represents the currency of the organization.
- '\$approved boolean? - Represents if the current record is approved.
- '\$approval record {}? - Represents the details of the approval process.
- Created_Time string? - Represents the date and time at which the record was created.
- Modified_Time string? - Represents the date and time at which the record was last modified.
- '\$editable boolean? - Represents if the user can edit records in the current module.
- Created_By record {}? - Represents the name, ID, and email of the user who created the current record.
- Modified_By record {}? - Represents the name, ID, and email of the user who last modified the record.
- '\$orchestration boolean? - Represents if the current record is a part of orchestration.
zoho.crm.rest: RecordsData
Fields
- data Record[]? -
- info RecordsdataInfo? -
zoho.crm.rest: RecordsdataInfo
Fields
- per_page int? -
- count int? -
- page int? -
- more_records boolean? -
zoho.crm.rest: Response
Fields
- data ResponseData[]? -
zoho.crm.rest: ResponseData
Fields
- code string? -
- details ResponseDetails? -
- message string? -
- status string? -
zoho.crm.rest: ResponseDetails
Fields
- Modified_Time string? -
- Modified_By By? -
- Created_Time string? -
- id string? -
- Created_By By? -
Import
import ballerinax/zoho.crm.rest;
Metadata
Released date: almost 2 years ago
Version: 1.3.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 7
Current verison: 7
Weekly downloads
Keywords
Sales & CRM/Customer Relationship Management
Cost/Paid
Contributors
Dependencies