zendesk.voice
Module zendesk.voice
API
Definitions
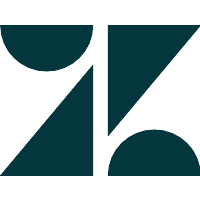
ballerinax/zendesk.voice Ballerina library
Overview
This is a generated connector for Zendesk Talk API v2 OpenAPI specification. Zendesk Voice provides capability to work with talk agents, groups, and tickets.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create an Zendesk account.
- Obtain tokens - Follow this guide.
Quickstart
To use the Zendesk Voice connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/zendesk.voice
module into the Ballerina project.
import ballerinax/zendesk.voice as zenvoice;
Step 2: Create a new connector instance
Create a zenvoice:ClientConfig
with the username and password obtained, then initialize the connector with it and the service URL (Zendesk Support URL) according to the Zendesk Support documentation.
zenvoice:ClientConfig clientConfig = { auth: { username: <ZENDESK_EMAIL>, password: <ZENDESK_PASSWORD> } }; zenvoice:Client baseClient = check new Client(clientConfig, serviceUrl = "<https://{YOUR_SUBDOMAIN}.zendesk.comL>");
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to list the phone numbers.
public function main() returns error? { zenvoice:PhoneNumbers phoneNumbers = check baseClient->listPhoneNumbers(); log:printInfo(phoneNumbers.toString()); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
zendesk.voice: Client
This is a generated connector for Zendesk Talk API v2 OpenAPI specification. Zendesk Talk API in conjunction with the Support API to work with Talk agents, groups, and tickets.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create an Zendesk account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string - URL of the target service
listPhoneNumbers
function listPhoneNumbers() returns PhoneNumbers|error
List phone numbers.
Return Type
- PhoneNumbers|error - Returns list of phone numbers
createPhoneNumber
function createPhoneNumber(PhoneNumberInfo payload) returns PhoneNumber|error
Create phone number (This endpoint is not available for trial accounts).
Parameters
- payload PhoneNumberInfo - The information for create phone number request
Return Type
- PhoneNumber|error - Returns detail of phone number created
searchAvailablePhoneNumbers
function searchAvailablePhoneNumbers(string country, string? areaCode, string? contains, boolean? tollFree) returns PhoneNumber|error
Search for available phone numbers (This endpoint is not available for trial accounts).
Parameters
- country string - The ISO country code
- areaCode string? (default ()) - Find phone numbers in the specified area code. (US and Canada only
- contains string? (default ()) - The regular expression used to search for phone numbers. Valid characters are '' and [0-9a-zA-Z]
- tollFree boolean? (default ()) - Whether the number should be toll-free or local
Return Type
- PhoneNumber|error - Returns available phone numbers
showPhoneNumber
function showPhoneNumber(string phoneNumberId) returns PhoneNumber|error
Show phone number.
Parameters
- phoneNumberId string - ID of a phone number
Return Type
- PhoneNumber|error - Returns phone number by id
deletePhoneNumberById
function deletePhoneNumberById(string phoneNumberId) returns PhoneNumber|error
Delete phone number by id.
Parameters
- phoneNumberId string - ID of a phone number
Return Type
- PhoneNumber|error - Returns detail of phone number deleted
listGreetingCategories
function listGreetingCategories() returns GreetingCategories|error
List greeting categories.
Return Type
- GreetingCategories|error - Returns list of greeting categories
showGreetingCategory
function showGreetingCategory(string greetingCategoriesId) returns GreetingCategory|error
Show greeting category.
Parameters
- greetingCategoriesId string - ID of the greeting category
Return Type
- GreetingCategory|error - Returns greeting category by id
listGreetings
List greetings.
createGreeting
function createGreeting(GreetingInfo payload) returns Greeting|error
Create greeting.
Parameters
- payload GreetingInfo - The information for create greeting request
showGreeting
Show greeting.
Parameters
- greetingsId string - ID of a greeting
deleteGreetingById
Delete greeting by id.
Parameters
- greetingsId string - ID of a greeting
Records
zendesk.voice: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
zendesk.voice: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth CredentialsConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
zendesk.voice: Greeting
Fields
- greeting GreetingGreeting? -
zendesk.voice: GreetingCategories
Fields
- greeting_categories GreetingcategoriesGreetingCategories[]? -
zendesk.voice: GreetingcategoriesGreetingCategories
Fields
- id int? - The greeting category ID
- name string? - The name of the greeting category
zendesk.voice: GreetingCategory
Fields
- greeting_category GreetingcategoryGreetingCategory? -
zendesk.voice: GreetingcategoryGreetingCategory
Fields
- id int? - The greeting category ID
- name string? - The name of the greeting category
zendesk.voice: GreetingGreeting
Fields
- category_id int? - The greeting category ID
- id int? - The greeting ID
- name string? - The name of the greeting category
zendesk.voice: GreetingInfo
The greeting information to create
Fields
- category_id string? - The greeting category ID
- name string? - The name of greeting
zendesk.voice: Greetings
Fields
- greetings GreetingsGreetings[]? -
- next_page int? - Next page
- previous_page int? - Previous page
- count int? - Count of records exists
zendesk.voice: GreetingsGreetings
Fields
- category_id int? - The greeting category ID
- id int? - The greeting ID
- name string? - The name of the greeting category
zendesk.voice: PhoneNumber
Fields
- phone_number PhonenumberPhoneNumber? -
zendesk.voice: PhoneNumberInfo
The phone number information to create
Fields
- token string? - Token returned by a search for available numbers.
zendesk.voice: PhonenumberPhoneNumber
Fields
- number string? - The phone number digits
- display_number string? - The formatted phone number
- toll_free boolean? - Whether the number is toll-free or local
- location string? - The number's geographical location. For example, "CA" or "Leeds"
- country_code string? - The ISO code of the country for the phone number
- token string? - A generated token unique for each phone number and used when provisioning the number
- price string? - The monthly cost of the phone number
- address_requirements string? - The type of address that must be supplied when purchasing the phone number. Possible values - "none", "local", "any", or "foreign"
zendesk.voice: PhoneNumbers
Fields
- phone_numbers PhonenumbersPhoneNumbers[]? -
- next_page int? - Next page
- previous_page int? - Previous page
- count int? - Count of records exists
zendesk.voice: PhonenumbersCapabilities
Whether a phone number has mms, sms, or voice capability
Fields
- sms boolean? - sms
- mms boolean? - mms
- voice boolean? - voice
zendesk.voice: PhonenumbersPhoneNumbers
Fields
- call_recording_consent string? - What call recording consent is set to
- capabilities PhonenumbersCapabilities? - Whether a phone number has mms, sms, or voice capability
- country_code string? - The ISO code of the country for this number
- created_at string? - The date and time the phone number was created
- default_greeting_ids string[]? - The names of default system greetings associated with the phone number
- default_group_id int? - Default group id
- display_number string? - The formatted phone number
- 'external boolean? - The external caller id number
- failover_number string? - Failover number associated with the phone number
- greeting_ids int[]? - Custom greetings associated with the phone number
- group_ids int[]? - An ids of associated groups
- id int? - Automatically assigned id upon creation
- ivr_id int? - ID of IVR associated with the phone number
- line_type string? - The type of line associated with the phone number
- location string? - The number's geographical location. For example, "CA" or "Leeds"
- name string? - The nickname if one is set. Otherwise the display_number
- nickname string? - The nickname of the number if one is set
- number string? - The phone number digits
- outbound_enabled boolean? - Whether or not the phone number has outbound enabled
- priority int? - Level of priority associated with the phone number
- recorded boolean? - Whether calls for the number are recorded or not
- schedule_id int? - ID of schedule associated with the phone number
- sms_enabled boolean? - Whether or not the phone number has sms enabled
- sms_group_id int? - The group associated with this phone number
- token string? - A generated token, unique for each phone number and used when provisioning the number
- toll_free boolean? - Whether the number is toll-free or local
- transcription boolean? - Whether calls for the number are transcribed or not
- voice_enabled boolean? - Whether or not the phone number has voice enabled
zendesk.voice: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
Import
import ballerinax/zendesk.voice;
Metadata
Released date: about 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 5
Current verison: 5
Weekly downloads
Keywords
Support/Customer Support
Cost/Freemium
Contributors
Dependencies