zendesk.support
Module zendesk.support
API
Definitions
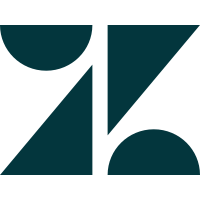
ballerinax/zendesk.support Ballerina library
Overview
This is a generated connector for Zendesk Support API v2 OpenAPI specification. Zendesk Support is a simple system for tracking, prioritizing and solving customer support tickets.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create an Zendesk account.
- Obtain tokens - Follow this guide.
Quickstart
To use the Zendesk Support connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/zendesk.support
module into the Ballerina project.
import ballerinax/zendesk.support as zensupport;
Step 2: Create a new connector instance
Create a zensupport:ClientConfig
with the username and password obtained, then initialize the connector with it and the service URL (Zendesk Support URL) according to the Zendesk Support documentation.
zensupport:ClientConfig clientConfig = { auth: { username: <ZENDESK_EMAIL>, password: <ZENDESK_PASSWORD> } }; zensupport:Client baseClient = check new Client(clientConfig, serviceUrl = "<https://{YOUR_SUBDOMAIN}.zendesk.comL>");
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to create a user using the connector.
public function main() returns error? { zensupport:CreateUserInfo user = { user:{ name: "Roger Wilco", email: "roger@gmail.com", organization: { name: "Rogers Organization" }, role: "agent" } }; zensupport:UserResponse userResult = check baseClient->createUser(user); log:printInfo(userResult.toString()); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
zendesk.support: Client
This is a generated connector for Zendesk Support API v2 OpenAPI specification. Zendesk Support is a simple system for tracking, prioritizing and solving customer support tickets.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create an Zendesk account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string - URL of the target service
listUsers
List Users.
createUser
function createUser(CreateUserInfo payload) returns UserResponse|error
Create User.
Parameters
- payload CreateUserInfo - The information for create user request
Return Type
- UserResponse|error - Returns detail of user created
searchUsers
Search Users.
Parameters
- query string? (default ()) - Query
Return Type
- json|error - Returns users matching the searchable string
getUserById
function getUserById(string user_id) returns UserResponse|error
Get User By Id.
Parameters
- user_id string - User Id
Return Type
- UserResponse|error - Returns user belong to the user id
deleteUserById
function deleteUserById(string user_id) returns UserResponse|error
Delete User By Id.
Parameters
- user_id string - User Id
Return Type
- UserResponse|error - Returns detail of user deleted
listTickets
List Tickets.
createTicket
function createTicket(CreateTicketInfo payload) returns TicketResponse|error
Create Ticket.
Parameters
- payload CreateTicketInfo - The information for create ticket request
Return Type
- TicketResponse|error - Returns detail of created ticket
updateTicket
function updateTicket(string ticket_id, UpdateTicketInfo payload) returns json|error
Update Ticket.
Parameters
- ticket_id string - Ticket Id
- payload UpdateTicketInfo - The information for update ticket request
Return Type
- json|error - Returns deatil of updated ticket by ticket id
createOrganization
function createOrganization(CreateOrganizationInfo payload) returns OrganizationResponse|error
Create Organization.
Parameters
- payload CreateOrganizationInfo - The information for create organization request
Return Type
- OrganizationResponse|error - Organization created
Records
zendesk.support: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
zendesk.support: Collaborator
Details of a collaborator
Fields
- name string? -
- email string? -
zendesk.support: Comment
Ticket comments represent the conversation between requesters, collaborators, and agents
Fields
- author_id int? - author_id
- body string? - body
- html_body string? - html_body
- 'public boolean? - public
zendesk.support: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth CredentialsConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
zendesk.support: CreateOrganizationInfo
The information for create organization request
Fields
- organization OrganizationInfo? - The organization information to create
zendesk.support: CreateTicketInfo
The information for create ticket request
Fields
- ticket TicketInfo? - The ticket information to create
zendesk.support: CreateUserInfo
The information for create user request
Fields
- user UserInfo? - The user information to create
zendesk.support: CustomField
Custom fields for the ticket
Fields
- id int? -
- value string? -
zendesk.support: EmailCC
Agent or end users email CCs to add or delete from the ticket
Fields
- user_id string? -
- action string? -
- user_email string? -
- user_name string? -
zendesk.support: Follower
Agent followers to add or delete from the ticket
Fields
- user_id string? -
- action string? -
- user_email string? -
- user_name string? -
zendesk.support: Organization
Fields
- url string? - The API url of this organization
- id int? - Automatically assigned id when the organization is created
- name string? - A unique name for the organization
- shared_tickets boolean? - End users in this organization are able to see each other's tickets
- shared_comments boolean? - End users in this organization are able to see each other's comments on tickets
- external_id string? - A unique external id to associate organizations to an external record
- created_at string? - The time the organization was created
- updated_at string? - The time the organization was updated
- domain_names string[]? - An array of domain names associated with this organization
- details string? - Any details obout the organization, such as the address
- notes string? - Any notes you have about the organization
- group_id int? - New tickets from users in this organization are automatically put in this group
- tags string[]? - The tags of the organization
- organization_fields record {}? - organization_fields
zendesk.support: OrganizationInfo
The organization information to create
Fields
- name string? - organization name
zendesk.support: OrganizationResponse
Fields
- organization Organization? -
zendesk.support: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
zendesk.support: Source
More information about the source of the ticket
Fields
- rel string? -
- 'from SourceDetail? -
- to SourceDetail? -
zendesk.support: SourceDetail
Fields
- id int? -
- title string? -
zendesk.support: Ticket
Fields
- allow_attachments boolean? - Permission for agents to add add attachments to a comment. Defaults to true
- allow_channelback boolean? - Is false if channelback is disabled, true otherwise. Only applicable for channels framework ticket
- assignee_id int? - The agent currently assigned to the ticket
- brand_id int? - Enterprise only. The id of the brand this ticket is associated with
- created_at string? - When this ticket record was created
- description string? - Read-only first comment on the ticket. When creating a ticket, use comment to set the description
- due_at string? - If this is a ticket of type "task" it has a due date. Due date format uses ISO 8601 format
- external_id string? - An id you can use to link Zendesk Support tickets to local records
- forum_topic_id int? - The topic in the Zendesk Web portal this ticket originated from, if any. The Web portal is deprecated
- group_id int? - The group this ticket is assigned to
- has_incidents boolean? - Is true if a ticket is a problem type and has one or more incidents linked to it. Otherwise, the value is false.
- id int? - Automatically assigned when the ticket is created
- is_public boolean? - Is true if any comments are public, false otherwise
- organization_id int? - The organization of the requester. You can only specify the ID of an organization associated with the requester
- priority string? - The urgency with which the ticket should be addressed. Allowed values are "urgent", "high", "normal", or "low"
- problem_id int? - For tickets of type "incident", the ID of the problem the incident is linked to
- raw_subject string? - The dynamic content placeholder, if present, or the "subject" value
- recipient string? - The original recipient e-mail address of the ticket
- requester_id int? - The user who requested this ticket
- status string? - The state of the ticket. Allowed values are "new", "open", "pending", "hold", "solved", or "closed"The state of the ticket. Allowed values are "new", "open", "pending", "hold", "solved", or "closed".
- subject string? - The value of the subject field for this ticket
- submitter_id int? - The user who submitted the ticket. The submitter always becomes the author of the first comment on the ticket
- ticket_form_id int? - Enterprise only. The id of the ticket form to render for the ticket
- 'type string? - The type of this ticket. Allowed values are "problem", "incident", "question", or "task" The type of this ticket. Allowed values are "problem", "incident", "question", or "task".
- updated_at string? - When this record last got updated
- url string? - The API url of this ticket
- via_followup_source_id int? - The id of a closed ticket when creating a follow-up ticket
zendesk.support: TicketInfo
The ticket information to create
Fields
- assignee_email string? - The email address of the agent to assign the ticket to
- assignee_id int? - The agent currently assigned to the ticket
- attribute_value_ids int[]? - An array of the IDs of attribute values to be associated with the ticket
- collaborator_ids int[]? - The ids of users currently CC'ed on the ticket
- custom_fields CustomField[]? - Custom fields for the ticket
- custom_status_id int? - The custom ticket status id of the ticket
- due_at string? - If this is a ticket of type "task" it has a due date. Due date format uses ISO 8601 format.
- email_ccs EmailCC[]? - An array of objects that represent agent or end users email CCs to add or delete from the ticket
- external_id string? - An id you can use to link Zendesk Support tickets to local records
- followers Follower[]? - An array of objects that represent agent followers to add or delete from the ticket
- group_id int? - The group this ticket is assigned to
- organization_id int? - The organization of the requester. You can only specify the ID of an organization associated with the requester.
- priority string? - Priority of ticket. Allowed values are "urgent", "high", "normal", or "low".
- problem_id int? - For tickets of type "incident", the ID of the problem the incident is linked to
- requester_id int? - The user who requested this ticket
- safe_update boolean? - Optional boolean. When true and an update_stamp date is included, protects against ticket update collisions and returns a message to let you know if one occurs.
- sharing_agreement_ids int[]? - The ids of the sharing agreements used for this ticket
- status string? - The state of the ticket. Allowed values are "new", "open", "pending", "hold", "solved", or "closed".
- subject string? - The value of the subject field for this ticket
- tags string[]? - The array of tags applied to this ticket
- 'type string? - The type of this ticket. Allowed values are "problem", "incident", "question", or "task".
- updated_stamp string? - Datetime of last update received from API. See the safe_update property
- brand_id int? - Enterprise only. The id of the brand this ticket is associated with
- collaborators (string|int|Collaborator)[]? - Users to add as cc's when creating a ticket.
- email_cc_ids int[]? - The ids of agents or end users currently CC'ed on the ticket.
- follower_ids int[]? - The ids of agents currently following the ticket.
- macro_ids int[]? - List of macro IDs to be recorded in the ticket audit
- raw_subject string? - The dynamic content placeholder, if present, or the "subject" value
- recipient string? - The original recipient e-mail address of the ticket
- submitter_id int? - The user who submitted the ticket
- ticket_form_id int? - Enterprise only. The id of the ticket form to render for the ticket
- via Via? - How or why an action or event was created
- via_followup_source_id int? - The id of a closed ticket when creating a follow-up ticket
- comment Comment? - Ticket comments represent the conversation between requesters, collaborators, and agents
zendesk.support: TicketInfoUpdate
The information for create ticket request
Fields
- ticket TicketInfoUpdate_ticket? -
zendesk.support: TicketInfoUpdate_ticket
Fields
- subject string? - subject
- priority string? - Priority of ticket. Allowed values are "urgent", "high", "normal", or "low"
- comment Comment? - Ticket comments represent the conversation between requesters, collaborators, and agents
zendesk.support: TicketResponse
Ticket comments represent the conversation between requesters, collaborators, and agents
Fields
- ticket Ticket? -
zendesk.support: Tickets
Fields
- tickets Ticket[]? -
- next_page int? - next_page
- previous_page int? - previous_page
- count int? - count
zendesk.support: UpdateTicketInfo
The information for create ticket request
Fields
- ticket TicketInfoUpdate? - The information for create ticket request
zendesk.support: User
Fields
- active boolean? - False if the user has been deleted
- alias string? - An alias displayed to end users
- chat_only boolean? - Whether or not the user is a chat-only agent
- created_at string? - The time the user was created
- custom_role_id int? - A custom role if the user is an agent on the Enterprise plan or above
- default_group_id int? - The id of the user's default group
- details string? - Any details you want to store about the user, such as an address
- email string? - The user's primary email address
- external_id string? - A unique identifier from another system. The API treats the id as case insensitive. Example - "ian1" and "Ian1" are the same user
- iana_time_zone string? - The time zone for the user
- id int? - Automatically assigned id when the user is created
- last_login_at string? - The last time the user signed in to Zendesk Support
- locale string? - The user's locale. A BCP-47 compliant tag for the locale. If both "locale" and "locale_id" are present on create or update, "locale_id" is ignored and only "locale" is used.
- locale_id int? - The user's language identifier
- moderator boolean? - Designates whether the user has forum moderation capabilities
- name string? - The user's name
- notes string? - Any notes you want to store about the user
- only_private_comments boolean? - true if the user can only create private comments
- organization_id int? - The id of the user's organization. If the user has more than one organization memberships, the id of the user's default organization
- phone string? - The user's primary phone number
- report_csv boolean? - Whether or not the user can access the CSV report on the Search tab of the Reporting page in the Support admin interface
- restricted_agent boolean? - If the agent has any restrictions; false for admins and unrestricted agents, true for other agents
- role string? - The user's role. Possible values are "end-user", "agent", or "admin"
- role_type int? - The user's role id. 0 for custom agents, 1 for light agent, 2 for chat agent, 3 for chat agent added to the Support account as a contributor (Chat Phase 4), and 5 for billing admins
- shared boolean? - If the user is shared from a different Zendesk Support instance. Ticket sharing accounts only
- shared_agent boolean? - If the user is a shared agent from a different Zendesk Support instance. Ticket sharing accounts only
- shared_phone_number boolean? - Whether the phone number is shared or not
- signature string? - The user's signature. Only agents and admins can have signatures
- suspended boolean? - If the agent is suspended. Tickets from suspended users are also suspended, and these users cannot sign in to the end user portal
- ticket_restriction string? - Specifies which tickets the user has access to. Possible values are - "organization", "groups", "assigned", "requested", null
- time_zone string? - The user's time zone
- two_factor_auth_enabled boolean? - If two factor authentication is enabled
- updated_at string? - The time the user was last updated
- url string? - The user's API url
- verified boolean? - Any of the user's identities is verified
zendesk.support: UserInfo
The user information to create
Fields
- custom_role_id string? - A custom role if the user is an agent on the Enterprise plan or above
- email string? - The user's primary email address
- name string? - The user's name
- organization OrganizationInfo? - The organization information to create
- role string? - The user's role. Possible values are "end-user", "agent", or "admin"
zendesk.support: UserResponse
Fields
- user User? -
zendesk.support: Users
Fields
- users Users_users[]? -
- next_page int? - next_page
- previous_page int? - previous_page
- count int? - count
zendesk.support: Users_users
Fields
- id int? - id
- url string? - url
- name string? - name
- email string? - email
zendesk.support: Via
How or why an action or event was created
Fields
- 'source Source? - More information about the source of the ticket
Import
import ballerinax/zendesk.support;
Metadata
Released date: almost 2 years ago
Version: 1.6.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
Pull count
Total: 25
Current verison: 10
Weekly downloads
Keywords
Support/Customer Support
Cost/Freemium
Contributors
Dependencies