Module ynab
API
Definitions
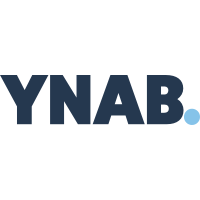
ballerinax/ynab Ballerina library
Overview
This is a generated connector for YNAB API v1.0.0 OpenAPI specification. YNAB API uses a REST based design, leverages the JSON data format, and relies upon HTTPS for transport. We respond with meaningful HTTP response codes and if an error occurs, we include error details in the response body. API Documentation is at https://api.youneedabudget.com
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create YNAB account
- Obtain tokens
- Log into YNAB account by visiting https://app.youneedabudget.com/users/authentication
- Obtain the
access token
following this guide
Quickstart
To use the YNAB connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/ynab
module into the Ballerina project.
import ballerinax/ynab;
Step 2: Create a new connector instance
Create a ynab:ClientConfig
with the access token
obtained, and initialize the connector with it.
ynab:ClientConfig clientConfig = { auth: { token: <ACCESS_TOKEN> } }; ynab:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to return a budgets list with summary information using the connector.
List budgets
public function main() { ynab:BudgetSummaryResponse|error response = baseClient->getBudgets(); if (response is ynab:BudgetSummaryResponse) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
ynab: Client
This is a generated connector for YNAB API v1.0.0 OpenAPI specification. YNAB API uses a REST based design, leverages the JSON data format, and relies upon HTTPS for transport. We respond with meaningful HTTP response codes and if an error occurs, we include error details in the response body. API Documentation is at https://api.youneedabudget.com
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a YNAB account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.youneedabudget.com/v1" - URL of the target service
getUser
function getUser() returns UserResponse|error
User info
Return Type
- UserResponse|error - The user info
getBudgets
function getBudgets(boolean? includeAccounts) returns BudgetSummaryResponse|error
List budgets
Parameters
- includeAccounts boolean? (default ()) - Whether to include the list of budget accounts
Return Type
- BudgetSummaryResponse|error - The list of budgets
getBudgetById
function getBudgetById(string budgetId, int? lastKnowledgeOfServer) returns BudgetDetailResponse|error
Single budget
Parameters
- budgetId string - The id of the budget. "last-used" can be used to specify the last used budget and "default" can be used if default budget selection is enabled (see: https://api.youneedabudget.com/#oauth-default-budget).
- lastKnowledgeOfServer int? (default ()) - The starting server knowledge. If provided, only entities that have changed since
last_knowledge_of_server
will be included.
Return Type
- BudgetDetailResponse|error - The requested budget
getBudgetSettingsById
function getBudgetSettingsById(string budgetId) returns BudgetSettingsResponse|error
Budget Settings
Parameters
- budgetId string - The id of the budget. "last-used" can be used to specify the last used budget and "default" can be used if default budget selection is enabled (see: https://api.youneedabudget.com/#oauth-default-budget).
Return Type
- BudgetSettingsResponse|error - The requested budget settings
getAccounts
function getAccounts(string budgetId, int? lastKnowledgeOfServer) returns AccountsResponse|error
Account list
Parameters
- budgetId string - The id of the budget. "last-used" can be used to specify the last used budget and "default" can be used if default budget selection is enabled (see: https://api.youneedabudget.com/#oauth-default-budget).
- lastKnowledgeOfServer int? (default ()) - The starting server knowledge. If provided, only entities that have changed since
last_knowledge_of_server
will be included.
Return Type
- AccountsResponse|error - The list of requested accounts
createAccount
function createAccount(string budgetId, byte[] payload) returns AccountResponse|error
Create a new account
Parameters
- budgetId string - The id of the budget ("last-used" can be used to specify the last used budget and "default" can be used if default budget selection is enabled (see: https://api.youneedabudget.com/#oauth-default-budget)
- payload byte[] - The account to create.
Return Type
- AccountResponse|error - The account was successfully created
getAccountById
function getAccountById(string budgetId, string accountId) returns AccountResponse|error
Single account
Parameters
- budgetId string - The id of the budget. "last-used" can be used to specify the last used budget and "default" can be used if default budget selection is enabled (see: https://api.youneedabudget.com/#oauth-default-budget).
- accountId string - The id of the account
Return Type
- AccountResponse|error - The requested account
getCategories
function getCategories(string budgetId, int? lastKnowledgeOfServer) returns CategoriesResponse|error
List categories
Parameters
- budgetId string - The id of the budget. "last-used" can be used to specify the last used budget and "default" can be used if default budget selection is enabled (see: https://api.youneedabudget.com/#oauth-default-budget).
- lastKnowledgeOfServer int? (default ()) - The starting server knowledge. If provided, only entities that have changed since
last_knowledge_of_server
will be included.
Return Type
- CategoriesResponse|error - The categories grouped by category group
getCategoryById
function getCategoryById(string budgetId, string categoryId) returns CategoryResponse|error
Single category
Parameters
- budgetId string - The id of the budget. "last-used" can be used to specify the last used budget and "default" can be used if default budget selection is enabled (see: https://api.youneedabudget.com/#oauth-default-budget).
- categoryId string - The id of the category
Return Type
- CategoryResponse|error - The requested category
getMonthCategoryById
function getMonthCategoryById(string budgetId, string month, string categoryId) returns CategoryResponse|error
Single category for a specific budget month
Parameters
- budgetId string - The id of the budget. "last-used" can be used to specify the last used budget and "default" can be used if default budget selection is enabled (see: https://api.youneedabudget.com/#oauth-default-budget).
- month string - The budget month in ISO format (e.g. 2016-12-01) ("current" can also be used to specify the current calendar month (UTC))
- categoryId string - The id of the category
Return Type
- CategoryResponse|error - The requested month category
updateMonthCategory
function updateMonthCategory(string budgetId, string month, string categoryId, byte[] payload) returns SaveCategoryResponse|error
Update a category for a specific month
Parameters
- budgetId string - The id of the budget. "last-used" can be used to specify the last used budget and "default" can be used if default budget selection is enabled (see: https://api.youneedabudget.com/#oauth-default-budget).
- month string - The budget month in ISO format (e.g. 2016-12-01) ("current" can also be used to specify the current calendar month (UTC))
- categoryId string - The id of the category
- payload byte[] - The category to update. Only
budgeted
amount can be updated and any other fields specified will be ignored.
Return Type
- SaveCategoryResponse|error - The month category was successfully updated
getPayees
function getPayees(string budgetId, int? lastKnowledgeOfServer) returns PayeesResponse|error
List payees
Parameters
- budgetId string - The id of the budget. "last-used" can be used to specify the last used budget and "default" can be used if default budget selection is enabled (see: https://api.youneedabudget.com/#oauth-default-budget).
- lastKnowledgeOfServer int? (default ()) - The starting server knowledge. If provided, only entities that have changed since
last_knowledge_of_server
will be included.
Return Type
- PayeesResponse|error - The requested list of payees
getPayeeById
function getPayeeById(string budgetId, string payeeId) returns PayeeResponse|error
Single payee
Parameters
- budgetId string - The id of the budget. "last-used" can be used to specify the last used budget and "default" can be used if default budget selection is enabled (see: https://api.youneedabudget.com/#oauth-default-budget).
- payeeId string - The id of the payee
Return Type
- PayeeResponse|error - The requested payee
getPayeeLocations
function getPayeeLocations(string budgetId) returns PayeeLocationsResponse|error
List payee locations
Parameters
- budgetId string - The id of the budget. "last-used" can be used to specify the last used budget and "default" can be used if default budget selection is enabled (see: https://api.youneedabudget.com/#oauth-default-budget).
Return Type
- PayeeLocationsResponse|error - The list of payee locations
getPayeeLocationById
function getPayeeLocationById(string budgetId, string payeeLocationId) returns PayeeLocationResponse|error
Single payee location
Parameters
- budgetId string - The id of the budget. "last-used" can be used to specify the last used budget and "default" can be used if default budget selection is enabled (see: https://api.youneedabudget.com/#oauth-default-budget).
- payeeLocationId string - id of payee location
Return Type
- PayeeLocationResponse|error - The payee location
getPayeeLocationsByPayee
function getPayeeLocationsByPayee(string budgetId, string payeeId) returns PayeeLocationsResponse|error
List locations for a payee
Parameters
- budgetId string - The id of the budget. "last-used" can be used to specify the last used budget and "default" can be used if default budget selection is enabled (see: https://api.youneedabudget.com/#oauth-default-budget).
- payeeId string - id of payee
Return Type
- PayeeLocationsResponse|error - The list of requested payee locations
getBudgetMonths
function getBudgetMonths(string budgetId, int? lastKnowledgeOfServer) returns MonthSummariesResponse|error
List budget months
Parameters
- budgetId string - The id of the budget. "last-used" can be used to specify the last used budget and "default" can be used if default budget selection is enabled (see: https://api.youneedabudget.com/#oauth-default-budget).
- lastKnowledgeOfServer int? (default ()) - The starting server knowledge. If provided, only entities that have changed since
last_knowledge_of_server
will be included.
Return Type
- MonthSummariesResponse|error - The list of budget months
getBudgetMonth
function getBudgetMonth(string budgetId, string month) returns MonthDetailResponse|error
Single budget month
Parameters
- budgetId string - The id of the budget. "last-used" can be used to specify the last used budget and "default" can be used if default budget selection is enabled (see: https://api.youneedabudget.com/#oauth-default-budget).
- month string - The budget month in ISO format (e.g. 2016-12-01) ("current" can also be used to specify the current calendar month (UTC))
Return Type
- MonthDetailResponse|error - The budget month detail
getTransactions
function getTransactions(string budgetId, string? sinceDate, string? 'type, int? lastKnowledgeOfServer) returns TransactionsResponse|error
List transactions
Parameters
- budgetId string - The id of the budget. "last-used" can be used to specify the last used budget and "default" can be used if default budget selection is enabled (see: https://api.youneedabudget.com/#oauth-default-budget).
- sinceDate string? (default ()) - If specified, only transactions on or after this date will be included. The date should be ISO formatted (e.g. 2016-12-30).
- 'type string? (default ()) - If specified, only transactions of the specified type will be included. "uncategorized" and "unapproved" are currently supported.
- lastKnowledgeOfServer int? (default ()) - The starting server knowledge. If provided, only entities that have changed since
last_knowledge_of_server
will be included.
Return Type
- TransactionsResponse|error - The list of requested transactions
createTransaction
function createTransaction(string budgetId, byte[] payload) returns SaveTransactionsResponse|error
Create a single transaction or multiple transactions
Parameters
- budgetId string - The id of the budget. "last-used" can be used to specify the last used budget and "default" can be used if default budget selection is enabled (see: https://api.youneedabudget.com/#oauth-default-budget).
- payload byte[] - The transaction or transactions to create. To create a single transaction you can specify a value for the
transaction
object and to create multiple transactions you can specify an array oftransactions
. It is expected that you will only provide a value for one of these objects.
Return Type
- SaveTransactionsResponse|error - The transaction or transactions were successfully created
updateTransactions
function updateTransactions(string budgetId, byte[] payload) returns SaveTransactionsResponse|error
Update multiple transactions
Parameters
- budgetId string - The id of the budget. "last-used" can be used to specify the last used budget and "default" can be used if default budget selection is enabled (see: https://api.youneedabudget.com/#oauth-default-budget).
- payload byte[] - The transactions to update. Each transaction must have either an
id
orimport_id
specified. Ifid
is specified as null animport_id
value can be provided which will allow transaction(s) to be updated by theirimport_id
. If anid
is specified, it will always be used for lookup.
Return Type
- SaveTransactionsResponse|error - The transactions were successfully updated
importTransactions
function importTransactions(string budgetId) returns TransactionsImportResponse|error
Import transactions
Parameters
- budgetId string - The id of the budget. "last-used" can be used to specify the last used budget and "default" can be used if default budget selection is enabled (see: https://api.youneedabudget.com/#oauth-default-budget).
Return Type
- TransactionsImportResponse|error - The request was successful but there were no transactions to import
getTransactionById
function getTransactionById(string budgetId, string transactionId) returns TransactionResponse|error
Single transaction
Parameters
- budgetId string - The id of the budget. "last-used" can be used to specify the last used budget and "default" can be used if default budget selection is enabled (see: https://api.youneedabudget.com/#oauth-default-budget).
- transactionId string - The id of the transaction
Return Type
- TransactionResponse|error - The requested transaction
updateTransaction
function updateTransaction(string budgetId, string transactionId, byte[] payload) returns TransactionResponse|error
Updates an existing transaction
Parameters
- budgetId string - The id of the budget. "last-used" can be used to specify the last used budget and "default" can be used if default budget selection is enabled (see: https://api.youneedabudget.com/#oauth-default-budget).
- transactionId string - The id of the transaction
- payload byte[] - The transaction to update
Return Type
- TransactionResponse|error - The transaction was successfully updated
bulkCreateTransactions
function bulkCreateTransactions(string budgetId, byte[] payload) returns BulkResponse|error
Bulk create transactions
Parameters
- budgetId string - The id of the budget. "last-used" can be used to specify the last used budget and "default" can be used if default budget selection is enabled (see: https://api.youneedabudget.com/#oauth-default-budget).
- payload byte[] - The list of transactions to create
Return Type
- BulkResponse|error - The bulk request was processed successfully
getTransactionsByAccount
function getTransactionsByAccount(string budgetId, string accountId, string? sinceDate, string? 'type, int? lastKnowledgeOfServer) returns TransactionsResponse|error
List account transactions
Parameters
- budgetId string - The id of the budget. "last-used" can be used to specify the last used budget and "default" can be used if default budget selection is enabled (see: https://api.youneedabudget.com/#oauth-default-budget).
- accountId string - The id of the account
- sinceDate string? (default ()) - If specified, only transactions on or after this date will be included. The date should be ISO formatted (e.g. 2016-12-30).
- 'type string? (default ()) - If specified, only transactions of the specified type will be included. "uncategorized" and "unapproved" are currently supported.
- lastKnowledgeOfServer int? (default ()) - The starting server knowledge. If provided, only entities that have changed since
last_knowledge_of_server
will be included.
Return Type
- TransactionsResponse|error - The list of requested transactions
getTransactionsByCategory
function getTransactionsByCategory(string budgetId, string categoryId, string? sinceDate, string? 'type, int? lastKnowledgeOfServer) returns HybridTransactionsResponse|error
List category transactions
Parameters
- budgetId string - The id of the budget. "last-used" can be used to specify the last used budget and "default" can be used if default budget selection is enabled (see: https://api.youneedabudget.com/#oauth-default-budget).
- categoryId string - The id of the category
- sinceDate string? (default ()) - If specified, only transactions on or after this date will be included. The date should be ISO formatted (e.g. 2016-12-30).
- 'type string? (default ()) - If specified, only transactions of the specified type will be included. "uncategorized" and "unapproved" are currently supported.
- lastKnowledgeOfServer int? (default ()) - The starting server knowledge. If provided, only entities that have changed since
last_knowledge_of_server
will be included.
Return Type
- HybridTransactionsResponse|error - The list of requested transactions
getTransactionsByPayee
function getTransactionsByPayee(string budgetId, string payeeId, string? sinceDate, string? 'type, int? lastKnowledgeOfServer) returns HybridTransactionsResponse|error
List payee transactions
Parameters
- budgetId string - The id of the budget. "last-used" can be used to specify the last used budget and "default" can be used if default budget selection is enabled (see: https://api.youneedabudget.com/#oauth-default-budget).
- payeeId string - The id of the payee
- sinceDate string? (default ()) - If specified, only transactions on or after this date will be included. The date should be ISO formatted (e.g. 2016-12-30).
- 'type string? (default ()) - If specified, only transactions of the specified type will be included. "uncategorized" and "unapproved" are currently supported.
- lastKnowledgeOfServer int? (default ()) - The starting server knowledge. If provided, only entities that have changed since
last_knowledge_of_server
will be included.
Return Type
- HybridTransactionsResponse|error - The list of requested transactions
getScheduledTransactions
function getScheduledTransactions(string budgetId, int? lastKnowledgeOfServer) returns ScheduledTransactionsResponse|error
List scheduled transactions
Parameters
- budgetId string - The id of the budget. "last-used" can be used to specify the last used budget and "default" can be used if default budget selection is enabled (see: https://api.youneedabudget.com/#oauth-default-budget).
- lastKnowledgeOfServer int? (default ()) - The starting server knowledge. If provided, only entities that have changed since
last_knowledge_of_server
will be included.
Return Type
- ScheduledTransactionsResponse|error - The list of requested scheduled transactions
getScheduledTransactionById
function getScheduledTransactionById(string budgetId, string scheduledTransactionId) returns ScheduledTransactionResponse|error
Single scheduled transaction
Parameters
- budgetId string - The id of the budget. "last-used" can be used to specify the last used budget and "default" can be used if default budget selection is enabled (see: https://api.youneedabudget.com/#oauth-default-budget).
- scheduledTransactionId string - The id of the scheduled transaction
Return Type
- ScheduledTransactionResponse|error - The requested Scheduled Transaction
Records
ynab: Account
Fields
- id string -
- name string -
- 'type string - The type of account. Note: payPal, merchantAccount, investmentAccount, and mortgage types have been deprecated and will be removed in the future.
- on_budget boolean - Whether this account is on budget or not
- closed boolean - Whether this account is closed or not
- note string? -
- balance int - The current balance of the account in milliunits format
- cleared_balance int - The current cleared balance of the account in milliunits format
- uncleared_balance int - The current uncleared balance of the account in milliunits format
- transfer_payee_id string - The payee id which should be used when transferring to this account
- direct_import_linked boolean? - Whether or not the account is linked to a financial institution for automatic transaction import.
- direct_import_in_error boolean? - If an account linked to a financial institution (direct_import_linked=true) and the linked connection is not in a healthy state, this will be true.
- deleted boolean - Whether or not the account has been deleted. Deleted accounts will only be included in delta requests.
ynab: AccountResponse
Fields
- data AccountresponseData -
ynab: AccountresponseData
Fields
- account Account -
ynab: AccountsResponse
Fields
- data AccountsresponseData -
ynab: AccountsresponseData
Fields
- accounts Account[] -
- server_knowledge int - The knowledge of the server
ynab: BudgetDetail
Fields
- Fields Included from *BudgetSummary
- id string
- name string
- last_modified_on string
- first_month string
- last_month string
- date_format DateFormat
- currency_format CurrencyFormat
- accounts Account[]
- anydata...
- accounts Account[]? -
- payees Payee[]? -
- payee_locations PayeeLocation[]? -
- category_groups CategoryGroup[]? -
- categories Category[]? -
- months MonthDetail[]? -
- transactions TransactionSummary[]? -
- subtransactions SubTransaction[]? -
- scheduled_transactions ScheduledTransactionSummary[]? -
- scheduled_subtransactions ScheduledSubTransaction[]? -
ynab: BudgetDetailResponse
Fields
- data BudgetdetailresponseData -
ynab: BudgetdetailresponseData
Fields
- budget BudgetDetail -
- server_knowledge int - The knowledge of the server
ynab: BudgetSettings
Fields
- date_format DateFormat - The date format setting for the budget. In some cases the format will not be available and will be specified as null.
- currency_format CurrencyFormat - The currency format setting for the budget. In some cases the format will not be available and will be specified as null.
ynab: BudgetSettingsResponse
Fields
- data BudgetsettingsresponseData -
ynab: BudgetsettingsresponseData
Fields
- settings BudgetSettings -
ynab: BudgetSummary
Fields
- id string -
- name string -
- last_modified_on string? - The last time any changes were made to the budget from either a web or mobile client
- first_month string? - The earliest budget month
- last_month string? - The latest budget month
- date_format DateFormat? - The date format setting for the budget. In some cases the format will not be available and will be specified as null.
- currency_format CurrencyFormat? - The currency format setting for the budget. In some cases the format will not be available and will be specified as null.
- accounts Account[]? - The budget accounts (only included if
include_accounts=true
specified as query parameter)
ynab: BudgetSummaryResponse
Fields
- data BudgetsummaryresponseData -
ynab: BudgetsummaryresponseData
Fields
- budgets BudgetSummary[] -
- default_budget BudgetSummary? -
ynab: BulkResponse
Fields
- data BulkresponseData -
ynab: BulkresponseData
Fields
- bulk BulkresponseDataBulk -
ynab: BulkresponseDataBulk
Fields
- transaction_ids string[] - The list of Transaction ids that were created.
- duplicate_import_ids string[] - If any Transactions were not created because they had an
import_id
matching a transaction already on the same account, the specified import_id(s) will be included in this list.
ynab: BulkTransactions
Fields
- transactions SaveTransaction[] -
ynab: CategoriesResponse
Fields
- data CategoriesresponseData -
ynab: CategoriesresponseData
Fields
- category_groups CategoryGroupWithCategories[] -
- server_knowledge int - The knowledge of the server
ynab: Category
Fields
- id string -
- category_group_id string -
- name string -
- hidden boolean - Whether or not the category is hidden
- original_category_group_id string? - If category is hidden this is the id of the category group it originally belonged to before it was hidden.
- note string? -
- budgeted int - Budgeted amount in milliunits format
- activity int - Activity amount in milliunits format
- balance int - Balance in milliunits format
- goal_type string? - The type of goal, if the category has a goal (TB='Target Category Balance', TBD='Target Category Balance by Date', MF='Monthly Funding', NEED='Plan Your Spending')
- goal_creation_month string? - The month a goal was created
- goal_target int? - The goal target amount in milliunits
- goal_target_month string? - The original target month for the goal to be completed. Only some goal types specify this date.
- goal_percentage_complete int? - The percentage completion of the goal
- goal_months_to_budget int? - The number of months, including the current month, left in the current goal period.
- goal_under_funded int? - The amount of funding still needed in the current month to stay on track towards completing the goal within the current goal period. This amount will generally correspond to the 'Underfunded' amount in the web and mobile clients except when viewing a category with a Needed for Spending Goal in a future month. The web and mobile clients will ignore any funding from a prior goal period when viewing category with a Needed for Spending Goal in a future month.
- goal_overall_funded int? - The total amount funded towards the goal within the current goal period.
- goal_overall_left int? - The amount of funding still needed to complete the goal within the current goal period.
- deleted boolean - Whether or not the category has been deleted. Deleted categories will only be included in delta requests.
ynab: CategoryGroup
Fields
- id string -
- name string -
- hidden boolean - Whether or not the category group is hidden
- deleted boolean - Whether or not the category group has been deleted. Deleted category groups will only be included in delta requests.
ynab: CategoryGroupWithCategories
Fields
- Fields Included from *CategoryGroup
- categories Category[] - Category group categories. Amounts (budgeted, activity, balance, etc.) are specific to the current budget month (UTC).
ynab: CategoryResponse
Fields
- data CategoryresponseData -
ynab: CategoryresponseData
Fields
- category Category -
ynab: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
ynab: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
ynab: CurrencyFormat
The currency format setting for the budget. In some cases the format will not be available and will be specified as null.
Fields
- iso_code string - ISO code
- example_format string - Example format
- decimal_digits int - Decimal digits
- decimal_separator string - Decimal separator
- symbol_first boolean - Symbol first
- group_separator string - Group separator
- currency_symbol string - Currency symbol
- display_symbol boolean - Display symbol
ynab: DateFormat
The date format setting for the budget. In some cases the format will not be available and will be specified as null.
Fields
- format string - Date format setting for the budget
ynab: ErrorDetail
Fields
- id string -
- name string -
- detail string -
ynab: ErrorResponse
Fields
- 'error ErrorDetail -
ynab: HybridTransaction
Fields
- Fields Included from *TransactionSummary
- 'type string - Whether the hybrid transaction represents a regular transaction or a subtransaction
- parent_transaction_id string? - For subtransaction types, this is the id of the parent transaction. For transaction types, this id will be always be null.
- account_name string -
- payee_name string? -
- category_name string? -
ynab: HybridTransactionsResponse
Fields
ynab: HybridtransactionsresponseData
Fields
- transactions HybridTransaction[] -
ynab: MonthDetail
Fields
- Fields Included from *MonthSummary
- categories Category[] - The budget month categories. Amounts (budgeted, activity, balance, etc.) are specific to the {month} parameter specified.
ynab: MonthDetailResponse
Fields
- data MonthdetailresponseData -
ynab: MonthdetailresponseData
Fields
- month MonthDetail -
ynab: MonthSummariesResponse
Fields
- data MonthsummariesresponseData -
ynab: MonthsummariesresponseData
Fields
- months MonthSummary[] -
- server_knowledge int - The knowledge of the server
ynab: MonthSummary
Fields
- month string -
- note string? -
- income int - The total amount of transactions categorized to 'Inflow: Ready to Assign' in the month
- budgeted int - The total amount budgeted in the month
- activity int - The total amount of transactions in the month, excluding those categorized to 'Inflow: Ready to Assign'
- to_be_budgeted int - The available amount for 'Ready to Assign'
- age_of_money int? - The Age of Money as of the month
- deleted boolean - Whether or not the month has been deleted. Deleted months will only be included in delta requests.
ynab: Payee
Fields
- id string -
- name string -
- transfer_account_id string? - If a transfer payee, the
account_id
to which this payee transfers to
- deleted boolean - Whether or not the payee has been deleted. Deleted payees will only be included in delta requests.
ynab: PayeeLocation
Fields
- id string -
- payee_id string -
- latitude string -
- longitude string -
- deleted boolean - Whether or not the payee location has been deleted. Deleted payee locations will only be included in delta requests.
ynab: PayeeLocationResponse
Fields
- data PayeelocationresponseData -
ynab: PayeelocationresponseData
Fields
- payee_location PayeeLocation -
ynab: PayeeLocationsResponse
Fields
- data PayeelocationsresponseData -
ynab: PayeelocationsresponseData
Fields
- payee_locations PayeeLocation[] -
ynab: PayeeResponse
Fields
- data PayeeresponseData -
ynab: PayeeresponseData
Fields
- payee Payee -
ynab: PayeesResponse
Fields
- data PayeesresponseData -
ynab: PayeesresponseData
Fields
- payees Payee[] -
- server_knowledge int - The knowledge of the server
ynab: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
ynab: SaveAccount
Fields
- name string - The name of the account
- 'type string - The account type
- balance int - The current balance of the account in milliunits format
ynab: SaveAccountWrapper
Fields
- account SaveAccount -
ynab: SaveCategoryResponse
Fields
- data SavecategoryresponseData -
ynab: SavecategoryresponseData
Fields
- category Category -
- server_knowledge int - The knowledge of the server
ynab: SaveMonthCategory
Fields
- budgeted int - Budgeted amount in milliunits format
ynab: SaveMonthCategoryWrapper
Fields
- category SaveMonthCategory -
ynab: SaveSubTransaction
Fields
- amount int - The subtransaction amount in milliunits format.
- payee_id string? - The payee for the subtransaction.
- payee_name string? - The payee name. If a
payee_name
value is provided andpayee_id
has a null value, thepayee_name
value will be used to resolve the payee by either (1) a matching payee rename rule (only if import_id is also specified on parent transaction) or (2) a payee with the same name or (3) creation of a new payee.
- category_id string? - The category for the subtransaction. Credit Card Payment categories are not permitted and will be ignored if supplied.
- memo string? -
ynab: SaveTransaction
Fields
- account_id string -
- date string - The transaction date in ISO format (e.g. 2016-12-01). Future dates (scheduled transactions) are not permitted. Split transaction dates cannot be changed and if a different date is supplied it will be ignored.
- amount int - The transaction amount in milliunits format. Split transaction amounts cannot be changed and if a different amount is supplied it will be ignored.
- payee_id string? - The payee for the transaction. To create a transfer between two accounts, use the account transfer payee pointing to the target account. Account transfer payees are specified as
tranfer_payee_id
on the account resource.
- payee_name string? - The payee name. If a
payee_name
value is provided andpayee_id
has a null value, thepayee_name
value will be used to resolve the payee by either (1) a matching payee rename rule (only ifimport_id
is also specified) or (2) a payee with the same name or (3) creation of a new payee.
- category_id string? - The category for the transaction. To configure a split transaction, you can specify null for
category_id
and provide asubtransactions
array as part of the transaction object. If an existing transaction is a split, thecategory_id
cannot be changed. Credit Card Payment categories are not permitted and will be ignored if supplied.
- memo string? -
- cleared string? - The cleared status of the transaction
- approved boolean? - Whether or not the transaction is approved. If not supplied, transaction will be unapproved by default.
- flag_color string? - The transaction flag
- import_id string? - If specified, the new transaction will be assigned this
import_id
and considered "imported". We will also attempt to match this imported transaction to an existing "user-entered" transation on the same account, with the same amount, and with a date +/-10 days from the imported transaction date.<br><br>Transactions imported through File Based Import or Direct Import (not through the API) are assigned an import_id in the format: 'YNAB:[milliunit_amount]:[iso_date]:[occurrence]'. For example, a transaction dated 2015-12-30 in the amount of -$294.23 USD would have an import_id of 'YNAB:-294230:2015-12-30:1'. If a second transaction on the same account was imported and had the same date and same amount, its import_id would be 'YNAB:-294230:2015-12-30:2'. Using a consistent format will prevent duplicates through Direct Import and File Based Import.<br><br>If import_id is omitted or specified as null, the transaction will be treated as a "user-entered" transaction. As such, it will be eligible to be matched against transactions later being imported (via DI, FBI, or API).
- subtransactions SaveSubTransaction[]? - An array of subtransactions to configure a transaction as a split. Updating
subtransactions
on an existing split transaction is not supported.
ynab: SaveTransactionsResponse
Fields
- data SavetransactionsresponseData -
ynab: SavetransactionsresponseData
Fields
- transaction_ids string[] - The transaction ids that were saved
- 'transaction TransactionDetail? -
- transactions TransactionDetail[]? - If multiple transactions were specified, the transactions that were saved
- duplicate_import_ids string[]? - If multiple transactions were specified, a list of import_ids that were not created because of an existing
import_id
found on the same account
- server_knowledge int - The knowledge of the server
ynab: SaveTransactionsWrapper
Fields
- 'transaction SaveTransaction? -
- transactions SaveTransaction[]? -
ynab: SaveTransactionWrapper
Fields
- 'transaction SaveTransaction -
ynab: ScheduledSubTransaction
Fields
- id string -
- scheduled_transaction_id string -
- amount int - The scheduled subtransaction amount in milliunits format
- memo string? -
- payee_id string? -
- category_id string? -
- transfer_account_id string? - If a transfer, the account_id which the scheduled subtransaction transfers to
- deleted boolean - Whether or not the scheduled subtransaction has been deleted. Deleted scheduled subtransactions will only be included in delta requests.
ynab: ScheduledTransactionDetail
Fields
- Fields Included from *ScheduledTransactionSummary
- account_name string -
- payee_name string? -
- category_name string? -
- subtransactions ScheduledSubTransaction[] - If a split scheduled transaction, the subtransactions.
ynab: ScheduledTransactionResponse
Fields
ynab: ScheduledtransactionresponseData
Fields
- scheduled_transaction ScheduledTransactionDetail -
ynab: ScheduledTransactionsResponse
Fields
ynab: ScheduledtransactionsresponseData
Fields
- scheduled_transactions ScheduledTransactionDetail[] -
- server_knowledge int - The knowledge of the server
ynab: ScheduledTransactionSummary
Fields
- id string -
- date_first string - The first date for which the Scheduled Transaction was scheduled.
- date_next string - The next date for which the Scheduled Transaction is scheduled.
- frequency string -
- amount int - The scheduled transaction amount in milliunits format
- memo string? -
- flag_color string? - The scheduled transaction flag
- account_id string -
- payee_id string? -
- category_id string? -
- transfer_account_id string? - If a transfer, the account_id which the scheduled transaction transfers to
- deleted boolean - Whether or not the scheduled transaction has been deleted. Deleted scheduled transactions will only be included in delta requests.
ynab: SubTransaction
Fields
- id string -
- transaction_id string -
- amount int - The subtransaction amount in milliunits format
- memo string? -
- payee_id string? -
- payee_name string? -
- category_id string? -
- category_name string? -
- transfer_account_id string? - If a transfer, the account_id which the subtransaction transfers to
- transfer_transaction_id string? - If a transfer, the id of transaction on the other side of the transfer
- deleted boolean - Whether or not the subtransaction has been deleted. Deleted subtransactions will only be included in delta requests.
ynab: TransactionDetail
Fields
- Fields Included from *TransactionSummary
- account_name string -
- payee_name string? -
- category_name string? -
- subtransactions SubTransaction[] - If a split transaction, the subtransactions.
ynab: TransactionResponse
Fields
- data TransactionresponseData -
ynab: TransactionresponseData
Fields
- 'transaction TransactionDetail -
ynab: TransactionsImportResponse
Fields
ynab: TransactionsimportresponseData
Fields
- transaction_ids string[] - The list of transaction ids that were imported.
ynab: TransactionsResponse
Fields
- data TransactionsresponseData -
ynab: TransactionsresponseData
Fields
- transactions TransactionDetail[] -
- server_knowledge int - The knowledge of the server
ynab: TransactionSummary
Fields
- id string -
- date string - The transaction date in ISO format (e.g. 2016-12-01)
- amount int - The transaction amount in milliunits format
- memo string? -
- cleared string - The cleared status of the transaction
- approved boolean - Whether or not the transaction is approved
- flag_color string? - The transaction flag
- account_id string -
- payee_id string? -
- category_id string? -
- transfer_account_id string? - If a transfer transaction, the account to which it transfers
- transfer_transaction_id string? - If a transfer transaction, the id of transaction on the other side of the transfer
- matched_transaction_id string? - If transaction is matched, the id of the matched transaction
- import_id string? - If the Transaction was imported, this field is a unique (by account) import identifier. If this transaction was imported through File Based Import or Direct Import and not through the API, the import_id will have the format: 'YNAB:[milliunit_amount]:[iso_date]:[occurrence]'. For example, a transaction dated 2015-12-30 in the amount of -$294.23 USD would have an import_id of 'YNAB:-294230:2015-12-30:1'. If a second transaction on the same account was imported and had the same date and same amount, its import_id would be 'YNAB:-294230:2015-12-30:2'.
- deleted boolean - Whether or not the transaction has been deleted. Deleted transactions will only be included in delta requests.
ynab: UpdateTransaction
Fields
- id string -
- Fields Included from *SaveTransaction
ynab: UpdateTransactionsWrapper
Fields
- transactions UpdateTransaction[] -
ynab: User
Fields
- id string -
ynab: UserResponse
Fields
- data UserresponseData -
ynab: UserresponseData
Fields
- user User -
Import
import ballerinax/ynab;
Metadata
Released date: about 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 6
Current verison: 5
Weekly downloads
Keywords
Finance/Asset Management
Cost/Paid
Contributors