xero.finance
Module xero.finance
API
Definitions
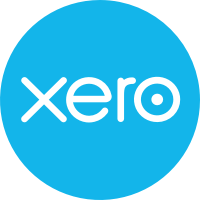
ballerinax/xero.finance Ballerina library
Overview
This is a generated connector for Xero Finance API v2.16.0 OpenAPI specification. The Finance API is a collection of endpoints which customers can use in the course of a loan application, which may assist lenders to gain the confidence they need to provide capital.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Xero account
- Obtain tokens by following this guide
Quickstart
To use the Xero Finance connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/xero.finance
module into the Ballerina project.
import ballerinax/xero.finance;
Step 2: Create a new connector instance
Create a finance:ClientConfig
with the access token
obtained, and initialize the connector with it.
finance:ClientConfig clientConfig = { auth: { token: <ACCESS_TOKEN> } }; finance:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to retrieve a list of all the users registered, and a history of their accounting transactions using the connector.
Get user activities
public function main() returns error? { finance:Statements response = check baseClient->getUserActivities(<Xero_Identifier_For_Tenant>); log:printInfo(response.toString()); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
xero.finance: Client
This is a generated connector for Xero Finance API v2.16.0 OpenAPI specification. The Finance API is a collection of endpoints which customers can use in the course of a loan application, which may assist lenders to gain the confidence they need to provide capital.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Xero account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.xero.com/finance.xro/1.0" - URL of the target service
getCashValidation
function getCashValidation(string xeroTenantId, string? balanceDate, string? asAtSystemDate, string? beginDate) returns CashValidationResponse[]|error
Get cash validation
Parameters
- xeroTenantId string - Xero identifier for Tenant
- balanceDate string? (default ()) - Date, yyyy-MM-dd. If no parameter is provided, the current date will be used. The ‘balance date’ will return transactions based on the accounting date entered by the user. Transactions before the balanceDate will be included. The user has discretion as to which accounting period the transaction relates to. The ‘balance date’ will control the latest maximum date of transactions included in the aggregate numbers. Balance date does not affect the CurrentStatement object, as this will always return the most recent statement before asAtSystemDate (if specified)
- asAtSystemDate string? (default ()) - Date, yyyy-MM-dd. If no parameter is provided, the current date will be used. The ‘as at’ date will return transactions based on the creation date. It reflects the date the transactions were entered into Xero, not the accounting date. The ‘as at’ date can not be overridden by the user. This can be used to estimate a ‘historical frequency of reconciliation’. The ‘as at’ date will affect the current statement in the response, as any candidate statements created after this date will be filtered out. Thus the current statement returned will be the most recent statement prior to the specified ‘as at’ date. Be aware that neither the begin date, nor the balance date, will affect the current statement. Note; information is only presented when system architecture allows, meaning historical cash validation information will be an estimate. In addition, delete events are not aware of the ‘as at’ functionality in this endpoint, meaning that transactions deleted at the time the API is accessed will be considered to always have been deleted.
- beginDate string? (default ()) - Date, yyyy-MM-dd. If no parameter is provided, the aggregate results will be drawn from the user’s total history. The ‘begin date’ will return transactions based on the accounting date entered by the user. Transactions after the beginDate will be included. The user has discretion as to which accounting period the transaction relates to.
Return Type
- CashValidationResponse[]|error - Success
getAccountUsage
function getAccountUsage(string xeroTenantId, string? startMonth, string? endMonth) returns AccountUsageResponse|error
Get account usage
Parameters
- xeroTenantId string - Xero identifier for Tenant
- startMonth string? (default ()) - Date, yyyy-MM. If no parameter is provided, the month 12 months prior to the end month will be used. Account usage for up to 12 months from this date will be returned.
- endMonth string? (default ()) - Date, yyyy-MM. If no parameter is provided, the current month will be used. Account usage for up to 12 months prior to this date will be returned.
Return Type
- AccountUsageResponse|error - Success
getLockHistory
function getLockHistory(string xeroTenantId, string? endDate) returns LockHistoryResponse|error
Get lock history
Parameters
- xeroTenantId string - Xero identifier for Tenant
- endDate string? (default ()) - Date, yyyy-MM-dd. If no parameter is provided, the current date will be used. Lock History for up to 12 months before this date will be returned.
Return Type
- LockHistoryResponse|error - Success
getReportHistory
function getReportHistory(string xeroTenantId, string? endDate) returns ReportHistoryResponse|error
Get report history
Parameters
- xeroTenantId string - Xero identifier for Tenant
- endDate string? (default ()) - Date, yyyy-MM-dd. If no parameter is provided, the current date will be used. Reports published up to 12 months before this date will be returned.
Return Type
- ReportHistoryResponse|error - Success
getUserActivities
function getUserActivities(string xeroTenantId, string? dataMonth) returns UserActivitiesResponse|error
Get user activities
Parameters
- xeroTenantId string - Xero identifier for Tenant
- dataMonth string? (default ()) - Date, yyyy-MM. If no parameter is provided, the month immediately previous to the current month will be used. The user activities for the specified month will be returned.
Return Type
- UserActivitiesResponse|error - Success
getFinancialStatementBalanceSheet
function getFinancialStatementBalanceSheet(string xeroTenantId, string? balanceDate) returns BalanceSheetResponse|error
Get Balance Sheet report
Parameters
- xeroTenantId string - Xero identifier for Tenant
- balanceDate string? (default ()) - Specifies the date for balance sheet report. Format yyyy-MM-dd. If no parameter is provided, the current date will be used.
Return Type
- BalanceSheetResponse|error - Success
getFinancialStatementCashflow
function getFinancialStatementCashflow(string xeroTenantId, string? startDate, string? endDate) returns CashflowResponse|error
Get Cash flow report
Parameters
- xeroTenantId string - Xero identifier for Tenant
- startDate string? (default ()) - Date e.g. yyyy-MM-dd. Specifies the start date for cash flow report. If no parameter is provided, the date of 12 months before the end date will be used.
- endDate string? (default ()) - Date e.g. yyyy-MM-dd. Specifies the end date for cash flow report. If no parameter is provided, the current date will be used.
Return Type
- CashflowResponse|error - Success
getFinancialStatementProfitAndLoss
function getFinancialStatementProfitAndLoss(string xeroTenantId, string? startDate, string? endDate) returns ProfitAndLossResponse|error
Get Profit & Loss report
Parameters
- xeroTenantId string - Xero identifier for Tenant
- startDate string? (default ()) - Date e.g. yyyy-MM-dd. Specifies the start date for profit and loss report. If no parameter is provided, the date of 12 months before the end date will be used.
- endDate string? (default ()) - Date e.g. yyyy-MM-dd. Specifies the end date for profit and loss report. If no parameter is provided, the current date will be used.
Return Type
- ProfitAndLossResponse|error - Success
getFinancialStatementTrialBalance
function getFinancialStatementTrialBalance(string xeroTenantId, string? endDate) returns TrialBalanceResponse|error
Get Trial Balance report
Parameters
- xeroTenantId string - Xero identifier for Tenant
- endDate string? (default ()) - Date e.g. yyyy-MM-dd. Specifies the end date for trial balance report. If no parameter is provided, the current date will be used.
Return Type
- TrialBalanceResponse|error - Success
Records
xero.finance: AccountUsage
Fields
- month string? - The month this usage item contains data for
- accountId string? - The account this usage item contains data for
- currencyCode string? - The currency code this usage item contains data for
- totalReceived decimal? - Total received
- countReceived int? - Count of received
- totalPaid decimal? - Total paid
- countPaid int? - Count of paid
- totalManualJournal decimal? - Total value of manual journals
- countManualJournal int? - Count of manual journals
- accountName string? - The name of the account
- reportingCode string? - Shown if set
- reportingCodeName string? - Shown if set
- reportCodeUpdatedDateUtc string? - Last modified date UTC format
xero.finance: AccountUsageResponse
Fields
- organisationId string? - The requested Organisation to which the data pertains
- startMonth string? - The start month of the report
- endMonth string? - The end month of the report
- accountUsage AccountUsage[]? -
xero.finance: BalanceSheetAccountDetail
Fields
- code string? - Accounting code
- accountID string? - ID of the account
- name string? - Account name
- reportingCode string? - Reporting code
- total decimal? - Total movement on this account
xero.finance: BalanceSheetAccountGroup
Fields
- accountTypes BalanceSheetAccountType[]? -
- total decimal? - Total value of all the accounts in this type
xero.finance: BalanceSheetAccountType
Fields
- accountType string? - The type of the account. See <a href='https://developer.xero.com/documentation/api/types#AccountTypes'>Account Types</a>
- accounts BalanceSheetAccountDetail[]? - A list of all accounts of this type. Refer to the Account section below for each account element detail.
- total decimal? - Total value of all the accounts in this type
xero.finance: BalanceSheetResponse
Fields
- balanceDate string? - Balance date of the report
- asset BalanceSheetAccountGroup? -
- liability BalanceSheetAccountGroup? -
- equity BalanceSheetAccountGroup? -
xero.finance: BankStatementResponse
Fields
- statementLines StatementLinesResponse? -
- currentStatement CurrentStatementResponse? -
xero.finance: CashAccountResponse
Fields
- unreconciledAmountPos decimal? - Total value of transactions in the journals which are not reconciled to bank statement lines, and have a positive (debit) value.
- unreconciledAmountNeg decimal? - Total value of transactions in the journals which are not reconciled to bank statement lines, and have a negative (credit) value.
- startingBalance decimal? - Starting (or historic) balance from the journals (manually keyed in by users on account creation - unverified).
- accountBalance decimal? - Current cash at bank accounting value from the journals.
- balanceCurrency string? - Currency which the cashAccount transactions relate to.
xero.finance: CashBalance
Fields
- openingCashBalance decimal? - Opening balance of cash and cash equivalents
- closingCashBalance decimal? - Closing balance of cash and cash equivalents
- netCashMovement decimal? - Net movement of cash and cash equivalents for the period
xero.finance: CashflowAccount
Fields
- accountId string? - ID of the account
- accountType string? - The type of the account. See <a href='https://developer.xero.com/documentation/api/types#AccountTypes'>Account Types</a>
- accountClass string? - The class of the account. See <a href='https://developer.xero.com/documentation/api/types#AccountClassTypes'>Account Class Types</a>
- code string? - Account code
- name string? - Account name
- reportingCode string? - Reporting code used for cash flow classification
- total decimal? - Total amount for the account
xero.finance: CashflowActivity
Fields
- name string? - Name of the cashflow activity type. It will be either Operating Activities, Investing Activities or Financing Activities
- total decimal? - Total value of the activity type
- cashflowTypes CashflowType[]? -
xero.finance: CashflowResponse
Fields
- startDate string? - Start date of the report
- endDate string? - End date of the report
- cashBalance CashBalance? -
- cashflowActivities CashflowActivity[]? - Break down of cash and cash equivalents for the period
xero.finance: CashflowType
Fields
- name string? - Name of the activity
- total decimal? - Total value of the activity
- accounts CashflowAccount[]? - List of the accounts in this activity
xero.finance: CashValidationResponse
Fields
- accountId string? - The Xero identifier for an account
- statementBalance StatementBalanceResponse? -
- statementBalanceDate string? - UTC Date when the last bank statement item was entered into Xero. This date is represented in ISO 8601 format.
- bankStatement BankStatementResponse? -
- cashAccount CashAccountResponse? -
xero.finance: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
xero.finance: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
xero.finance: CurrentStatementResponse
Fields
- startDate string? - Looking at the most recent bank statement, this field indicates the first date which transactions on this statement pertain to. This date is represented in ISO 8601 format.
- endDate string? - Looking at the most recent bank statement, this field indicates the last date which transactions on this statement pertain to. This date is represented in ISO 8601 format.
- startBalance decimal? - Looking at the most recent bank statement, this field indicates the balance before the transactions on the statement are applied (note, this is not always populated by the bank in every single instance (~10%)).
- endBalance decimal? - Looking at the most recent bank statement, this field indicates the balance after the transactions on the statement are applied (note, this is not always populated by the bank in every single instance (~10%)).
- importedDateTimeUtc string? - Looking at the most recent bank statement, this field indicates when the document was imported into Xero. This date is represented in ISO 8601 format.
- importSourceType string? - Looking at the most recent bank statement, this field indicates the source of the data (direct bank feed, indirect bank feed, file upload, or manual keying).
xero.finance: DataSourceResponse
Fields
- directBankFeed decimal? - Sum of the amounts of all statement lines where the source of the data was a direct bank feed in to Xero. This gives an indication on the certainty of correctness of the data.
- indirectBankFeed decimal? - Sum of the amounts of all statement lines where the source of the data was a indirect bank feed to Xero (usually via Yodlee). This gives an indication on the certainty of correctness of the data.
- fileUpload decimal? - Sum of the amounts of all statement lines where the source of the data was a CSV file upload in to Xero. This gives an indication on the certainty of correctness of the data.
- manual decimal? - Sum of the amounts of all statement lines where the source of the data was manually keyed in to Xero. This gives an indication on the certainty of correctness of the data.
- directBankFeedPos decimal? - Sum of the amounts of all statement lines where the source of the data was a direct bank feed in to Xero. This gives an indication on the certainty of correctness of the data. Only positive transactions are included.
- indirectBankFeedPos decimal? - Sum of the amounts of all statement lines where the source of the data was a indirect bank feed to Xero (usually via Yodlee). This gives an indication on the certainty of correctness of the data. Only positive transactions are included.
- fileUploadPos decimal? - Sum of the amounts of all statement lines where the source of the data was a CSV file upload in to Xero. This gives an indication on the certainty of correctness of the data. Only positive transactions are included.
- manualPos decimal? - Sum of the amounts of all statement lines where the source of the data was manually keyed in to Xero. This gives an indication on the certainty of correctness of the data. Only positive transactions are included.
- directBankFeedNeg decimal? - Sum of the amounts of all statement lines where the source of the data was a direct bank feed in to Xero. This gives an indication on the certainty of correctness of the data. Only negative transactions are included.
- indirectBankFeedNeg decimal? - Sum of the amounts of all statement lines where the source of the data was a indirect bank feed to Xero (usually via Yodlee). This gives an indication on the certainty of correctness of the data. Only negative transactions are included.
- fileUploadNeg decimal? - Sum of the amounts of all statement lines where the source of the data was a CSV file upload in to Xero. This gives an indication on the certainty of correctness of the data. Only negative transactions are included.
- manualNeg decimal? - Sum of the amounts of all statement lines where the source of the data was manually keyed in to Xero. This gives an indication on the certainty of correctness of the data. Only negative transactions are included.
- otherPos decimal? - Sum of the amounts of all statement lines where the source of the data was any other category. This gives an indication on the certainty of correctness of the data. Only positive transactions are included.
- otherNeg decimal? - Sum of the amounts of all statement lines where the source of the data was any other category. This gives an indication on the certainty of correctness of the data. Only negative transactions are included.
- other decimal? - Sum of the amounts of all statement lines where the source of the data was any other category. This gives an indication on the certainty of correctness of the data.
xero.finance: HistoryRecordResponse
Fields
- changes string? - The type of change recorded against the document
- dateUTCString string? - UTC date that the history record was created
- dateUTC string? - UTC date that the history record was created
- user string? - The users first and last name
- details string? - Description of the change event or transaction
xero.finance: LockHistoryModel
Fields
- hardLockDate string? - Date the account hard lock was set
- softLockDate string? - Date the account soft lock was set
- updatedDateUtc string? - The system date time that the lock was updated
xero.finance: LockHistoryResponse
Fields
- organisationId string? - The requested Organisation to which the data pertains
- endDate string? - The end date of the report
- lockDates LockHistoryModel[]? -
xero.finance: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://identity.xero.com/connect/token") - Refresh URL
xero.finance: PnlAccount
Fields
- accountID string? - ID of the account
- accountType string? - The type of the account. See <a href='https://developer.xero.com/documentation/api/types#AccountTypes'>Account Types</a>
- code string? - Account code
- name string? - Account name
- reportingCode string? - Reporting code (Shown if set)
- total decimal? - Total movement on this account
xero.finance: PnlAccountClass
Fields
- total decimal? - Total revenue/expense value
- accountTypes PnlAccountType[]? - Contains trading income and other income for revenue section / operating expenses and direct cost for expense section if the data is available for each section. Refer to the account type element below
xero.finance: PnlAccountType
Fields
- total decimal? - Total movement on this account type
- title string? - Name of this account type, it will be either Trading Income or Other Income for Revenue section / Direct Cost or Operating Expenses for Expense section
- accounts PnlAccount[]? - A list of the movement on each account detail during the query period. Refer to the account detail element below
xero.finance: PracticeResponse
Fields
- xeroPartnerSince int? - Year of becoming a partner.
- tier string? - Customer tier e.g. Silver
- location string? - Country of location.
- organisationCount int? - Organisation count.
- staffCertified boolean? - Staff certified (true/false).
xero.finance: Problem
Fields
- 'type ProblemType? -
- title string? -
- status int? -
- detail string? -
xero.finance: ProfitAndLossResponse
Fields
- startDate string? - Start date of the report
- endDate string? - End date of the report
- netProfitLoss decimal? - Net profit loss value
- revenue PnlAccountClass? -
- expense PnlAccountClass? -
xero.finance: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
xero.finance: ReportHistoryModel
Fields
- reportName string? - Report code or report title
- reportDateText string? - The date or date range of the report
- publishedDateUtc string? - The system date time that the report was published
xero.finance: ReportHistoryResponse
Fields
- organisationId string? - The requested Organisation to which the data pertains
- endDate string? - The end date of the report
- reports ReportHistoryModel[]? -
xero.finance: StatementBalanceResponse
Fields
- value decimal? - Total closing balance of the account. This includes both reconciled and unreconciled bank statement lines. The closing balance will always be represented as a positive number, with it’s debit/credit status defined in the statementBalanceDebitCredit field.
- 'type string? - The DEBIT or CREDIT status of the account. Cash accounts in credit have a negative balance.
xero.finance: StatementLinesResponse
Fields
- unreconciledAmountPos decimal? - Sum of the amounts of all statement lines where both the reconciled flag is set to FALSE, and the amount is positive.
- unreconciledAmountNeg decimal? - Sum of the amounts of all statement lines where both the reconciled flag is set to FALSE, and the amount is negative.
- unreconciledLines int? - Count of all statement lines where the reconciled flag is set to FALSE.
- avgDaysUnreconciledPos decimal? - Sum-product of age of statement line in days multiplied by transaction amount, divided by the sum of transaction amount - in for those statement lines in which the reconciled flag is set to FALSE, and the amount is positive. Provides an indication of the age of unreconciled transactions.
- avgDaysUnreconciledNeg decimal? - Sum-product of age of statement line in days multiplied by transaction amount, divided by the sum of transaction amount - in for those statement lines in which the reconciled flag is set to FALSE, and the amount is negative. Provides an indication of the age of unreconciled transactions.
- earliestUnreconciledTransaction string? - UTC Date which is the earliest transaction date of a statement line for which the reconciled flag is set to FALSE. This date is represented in ISO 8601 format.
- latestUnreconciledTransaction string? - UTC Date which is the latest transaction date of a statement line for which the reconciled flag is set to FALSE. This date is represented in ISO 8601 format.
- deletedAmount decimal? - Sum of the amounts of all deleted statement lines. Transactions may be deleted due to duplication or otherwise.
- totalAmount decimal? - Sum of the amounts of all statement lines. This is used as a metric of comparison to the unreconciled figures above.
- dataSource DataSourceResponse? -
- earliestReconciledTransaction string? - UTC Date which is the earliest transaction date of a statement line for which the reconciled flag is set to TRUE. This date is represented in ISO 8601 format.
- latestReconciledTransaction string? - UTC Date which is the latest transaction date of a statement line for which the reconciled flag is set to TRUE. This date is represented in ISO 8601 format.
- reconciledAmountPos decimal? - Sum of the amounts of all statement lines where both the reconciled flag is set to TRUE, and the amount is positive.
- reconciledAmountNeg decimal? - Sum of the amounts of all statement lines where both the reconciled flag is set to TRUE, and the amount is negative.
- reconciledLines int? - Count of all statement lines where the reconciled flag is set to TRUE
- totalAmountPos decimal? - Sum of the amounts of all statement lines where the amount is positive
- totalAmountNeg decimal? - Sum of the amounts of all statement lines where the amount is negative.
xero.finance: TrialBalanceAccount
Fields
- accountId string? - ID of the account
- accountType string? - The type of the account. See <a href='https://developer.xero.com/documentation/api/types#AccountTypes'>Account Types</a>
- accountCode string? - Customer defined alpha numeric account code e.g 200 or SALES
- accountClass string? - The class of the account. See <a href='https://developer.xero.com/documentation/api/types#AccountClassTypes'>Account Class Types</a>
- status string? - Accounts with a status of ACTIVE can be updated to ARCHIVED. See <a href='https://developer.xero.com/documentation/api/types#AccountStatusCodes'>Account Status Codes</a>
- reportingCode string? - Reporting code (Shown if set)
- accountName string? - Name of the account
- balance TrialBalanceEntry? -
- signedBalance decimal? - Value of balance. Expense and Asset accounts code debits as positive. Revenue, Liability, and Equity accounts code debits as negative
- accountMovement TrialBalanceMovement? -
xero.finance: TrialBalanceEntry
Fields
- value decimal? - Net movement or net balance in the account
- entryType string? - Sign (Debit/Credit) of the movement of balance in the account
xero.finance: TrialBalanceMovement
Fields
- debits decimal? - Debit amount
- credits decimal? - Credit amount
- movement TrialBalanceEntry? -
- signedMovement decimal? - Value of movement. Expense and Asset accounts code debits as positive. Revenue, Liability, and Equity accounts code debits as negative
xero.finance: TrialBalanceResponse
Fields
- startDate string? - Start date of the report
- endDate string? - End date of the report
- accounts TrialBalanceAccount[]? - Refer to the accounts section below
xero.finance: UserActivitiesResponse
Fields
- organisationId string? - The requested Organisation to which the data pertains
- dataMonth string? - The month of the report
- users UserResponse[]? -
xero.finance: UserResponse
Fields
- userId string? - The Xero identifier for the user
- userCreatedDateUtc string? - Timestamp of user creation.
- lastLoginDateUtc string? - Timestamp of user last login
- isExternalPartner boolean? - User is external partner.
- hasAccountantRole boolean? - User has Accountant role.
- monthPeriod string? - Month period in format yyyy-MM.
- numberOfLogins int? - Number of times the user has logged in.
- numberOfDocumentsCreated int? - Number of documents created.
- netValueDocumentsCreated decimal? - Net value of documents created.
- absoluteValueDocumentsCreated decimal? - Absolute value of documents created.
- attachedPractices PracticeResponse[]? -
- historyRecords HistoryRecordResponse[]? -
String types
xero.finance: ProblemType
ProblemType
Import
import ballerinax/xero.finance;
Metadata
Released date: over 2 years ago
Version: 1.4.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.2.1
GraalVM compatible: Yes
Pull count
Total: 5
Current verison: 0
Weekly downloads
Keywords
Finance/Accounting
Cost/Paid
Contributors
Dependencies