xero.files
Module xero.files
API
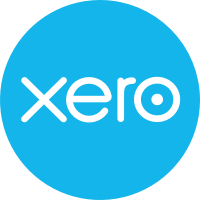
ballerinax/xero.files Ballerina library
Overview
This is a generated connector for Xero Files API v2.16.1 OpenAPI specification. The Files API provides access to the files, folders, and the association of files within a Xero organisation. It can be used to upload/download files, manage folders and associate files to invoices, contacts, payments etc.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Xero account
- Obtain tokens by following this guide
Quickstart
To use the Xero Files connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/xero.files
module into the Ballerina project.
import ballerinax/xero.files;
Step 2: Create a new connector instance
Create a files:ClientConfig
with the access token
obtained, and initialize the connector with it.
files:ClientConfig clientConfig = { auth: { token: <ACCESS_TOKEN> } }; files:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
You can get all the files by providing Xero tenant identifier as parameter.
files:Files response = check baseClient->getFiles(<Xero_Identifier_For_Tenant>);
-
Use
bal run
command to compile and run the Ballerina program.
Clients
xero.files: Client
This is a generated connector for Xero Files API v2.16.1 OpenAPI specification. The Files API provides access to the files, folders, and the association of files within a Xero organisation. It can be used to upload/download files, manage folders and associate files to invoices, contacts, payments etc.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Xero account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.xero.com/files.xro/1.0/" - URL of the target service
getFiles
Retrieves files
Parameters
- xeroTenantId string - Xero identifier for Tenant
- pagesize int? (default ()) - pass an optional page size value
- page int? (default ()) - number of records to skip for pagination
- sort string? (default ()) - values to sort by
uploadFile
function uploadFile(string xeroTenantId, UploadObject payload) returns FileObject|error
Uploads a File to the inbox
Parameters
- xeroTenantId string - Xero identifier for Tenant
- payload UploadObject - A record of type
UploadObject
which contains details to upload a file to inbox
Return Type
- FileObject|error - A successful request
getFile
function getFile(string xeroTenantId, string fileId) returns FileObject|error
Retrieves a file by a unique file ID
Return Type
- FileObject|error - search results matching criteria
updateFile
function updateFile(string xeroTenantId, string fileId, FileObject payload) returns FileObject|error
Update a file
Parameters
- xeroTenantId string - Xero identifier for Tenant
- fileId string - File id for single object
- payload FileObject - A record of type
FileObject
which contains details to update a file
Return Type
- FileObject|error - A successful request
deleteFile
Deletes a specific file
uploadFileToFolder
function uploadFileToFolder(string xeroTenantId, string folderId, UploadObject payload) returns FileObject|error
Uploads a File to a specific folder
Parameters
- xeroTenantId string - Xero identifier for Tenant
- folderId string - pass required folder id to save file to specific folder
- payload UploadObject - A record of type
UploadObject
which contains details to upload a file to specific folder
Return Type
- FileObject|error - A successful request
getFileContent
Retrieves the content of a specific file
getFileAssociations
function getFileAssociations(string xeroTenantId, string fileId) returns Association[]|error
Retrieves a specific file associations
Return Type
- Association[]|error - search results matching criteria
createFileAssociation
function createFileAssociation(string xeroTenantId, string fileId, Association payload) returns Association|error
Creates a new file association
Parameters
- xeroTenantId string - Xero identifier for Tenant
- fileId string - File id for single object
- payload Association - A record of type
Association
which contains details to create a new file association
Return Type
- Association|error - A successful request
deleteFileAssociation
function deleteFileAssociation(string xeroTenantId, string fileId, string objectId) returns Response|error
Deletes an existing file association
Parameters
- xeroTenantId string - Xero identifier for Tenant
- fileId string - File id for single object
- objectId string - Object id for single object
getAssociationsByObject
function getAssociationsByObject(string xeroTenantId, string objectId) returns Association[]|error
Retrieves an association object using a unique object ID
Parameters
- xeroTenantId string - Xero identifier for Tenant
- objectId string - Object id for single object
Return Type
- Association[]|error - search results matching criteria
getFolders
Retrieves folders
Parameters
- xeroTenantId string - Xero identifier for Tenant
- sort string? (default ()) - values to sort by
createFolder
Creates a new folder
Parameters
- xeroTenantId string - Xero identifier for Tenant
- payload Folder - A record of type
Folder
which contains details to create a new folder
getFolder
Retrieves specific folder by using a unique folder ID
Parameters
- xeroTenantId string - Xero identifier for Tenant
- folderId string - Folder id for single object
updateFolder
Updates an existing folder
Parameters
- xeroTenantId string - Xero identifier for Tenant
- folderId string - Folder id for single object
- payload Folder - A record of type
Folder
which contains details to update a existing folder
deleteFolder
Deletes a folder
Parameters
- xeroTenantId string - Xero identifier for Tenant
- folderId string - Folder id for single object
getInbox
Retrieves inbox folder
Parameters
- xeroTenantId string - Xero identifier for Tenant
Records
xero.files: Association
Fields
- FileId string? - The unique identifier of the file
- ObjectId string? - The identifier of the object that the file is being associated with (e.g. InvoiceID, BankTransactionID, ContactID)
- ObjectGroup ObjectGroup? - The Object Group that the object is in. These roughly correlate to the endpoints that can be used to retrieve the object via the core accounting API.
- ObjectType ObjectType? - The Object Type
xero.files: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
xero.files: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
xero.files: FileObject
Fields
- Name string? - File Name
- MimeType string? - MimeType of the file (image/png, image/jpeg, application/pdf, etc..)
- Size int? - Numeric value in bytes
- CreatedDateUtc string? - Created date in UTC
- UpdatedDateUtc string? - Updated date in UTC
- User User? -
- Id string? - File object's UUID
- FolderId string? - Folder relation object's UUID
xero.files: Files
Fields
- TotalCount int? -
- Page int? -
- PerPage int? -
- Items FileObject[]? -
xero.files: Folder
Fields
- Name string? - The name of the folder
- FileCount int? - The number of files in the folder
- Email string? - The email address used to email files to the inbox. Only the inbox will have this element.
- IsInbox boolean? - to indicate if the folder is the Inbox. The Inbox cannot be renamed or deleted.
- Id string? - Xero unique identifier for a folder Files
xero.files: Folders
Fields
- Folders Folder[]? -
xero.files: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://identity.xero.com/connect/token") - Refresh URL
xero.files: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
xero.files: UploadObject
Fields
- body string -
- name string - exact name of the file you are uploading
- filename string -
- mimeType string? -
xero.files: User
Fields
- Id string - Xero identifier
- Name string? - Key is Name, but returns Email address of user who created the file
- FirstName string? - First name of user
- LastName string? - Last name of user
- FullName string? - Last name of user
String types
Import
import ballerinax/xero.files;
Metadata
Released date: over 1 year ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 2
Current verison: 1
Weekly downloads
Keywords
Content & Files/Documents
Cost/Paid
Contributors