xero.bankfeeds
Module xero.bankfeeds
API
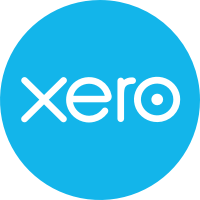
ballerinax/xero.bankfeeds Ballerina library
Overview
This is a generated connector for Xero Bank Feeds API v2.16.0 OpenAPI specification. The Bank Feeds API is a closed API that is only available to financial institutions that have an established financial services partnership with Xero. If you're an existing financial services partner that wants access, contact your local Partner Manager. If you're a financial institution who wants to provide bank feeds to your business customers, contact xero to become a financial services partner.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Xero account
- Obtain tokens by following this guide
Quickstart
To use the Xero Bank Feeds connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/xero.bankfeeds
module into the Ballerina project.
import ballerinax/xero.bankfeeds;
Step 2: Create a new connector instance
Create a bankfeeds:ClientConfig
with the access token
obtained, and initialize the connector with it.
bankfeeds:ClientConfig clientConfig = { auth: { token: <ACCESS_TOKEN> } }; bankfeeds:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to retrieve all statements using the connector.
Retrieve all statements
public function main() returns error? { bankfeeds:Statements response = check baseClient->getStatements(); log:printInfo(response.toString()); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
xero.bankfeeds: Client
This is a generated connector for Xero Bank Feeds API v2.16.0 OpenAPI specification. The Bank Feeds API is a closed API that is only available to financial institutions that have an established financial services partnership with Xero. If you're an existing financial services partner that wants access, contact your local Partner Manager. If you're a financial institution who wants to provide bank feeds to your business customers, contact xero to become a financial services partner.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Xero account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.xero.com/bankfeeds.xro/1.0" - URL of the target service
getFeedConnections
function getFeedConnections(string xeroTenantId, int? page, int? pageSize) returns FeedConnections|error
Searches for feed connections
Parameters
- xeroTenantId string - Xero identifier for Tenant
- page int? (default ()) - Page number which specifies the set of records to retrieve. By default the number of the records per set is 10. Example - https://api.xero.com/bankfeeds.xro/1.0/FeedConnections?page=1 to get the second set of the records. When page value is not a number or a negative number, by default, the first set of records is returned.
- pageSize int? (default ()) - Page size which specifies how many records per page will be returned (default 10). Example - https://api.xero.com/bankfeeds.xro/1.0/FeedConnections?pageSize=100 to specify page size of 100.
Return Type
- FeedConnections|error - search results matching criteria returned with pagination and items array
createFeedConnections
function createFeedConnections(string xeroTenantId, FeedConnections payload) returns FeedConnections|error
Create one or more new feed connection
Parameters
- xeroTenantId string - Xero identifier for Tenant
- payload FeedConnections - Feed Connection(s) array object in the body
Return Type
- FeedConnections|error - success new feed connection(s)response
getFeedConnection
function getFeedConnection(string xeroTenantId, string id) returns FeedConnection|error
Retrieve single feed connection based on a unique id provided
Parameters
- xeroTenantId string - Xero identifier for Tenant
- id string - Unique identifier for retrieving single object
Return Type
- FeedConnection|error - success returns a FeedConnection object matching the id in response
deleteFeedConnections
function deleteFeedConnections(string xeroTenantId, FeedConnections payload) returns FeedConnections|error
Delete an existing feed connection
Parameters
- xeroTenantId string - Xero identifier for Tenant
- payload FeedConnections - Feed Connections array object in the body
Return Type
- FeedConnections|error - Success response for deleted feed connection
getStatements
function getStatements(string xeroTenantId, int? page, int? pageSize, string xeroApplicationId, string xeroUserId) returns Statements|error
Retrieve all statements
Parameters
- xeroTenantId string - Xero identifier for Tenant
- page int? (default ()) - unique id for single object
- pageSize int? (default ()) - Page size which specifies how many records per page will be returned (default 10). Example - https://api.xero.com/bankfeeds.xro/1.0/Statements?pageSize=100 to specify page size of 100.
- xeroApplicationId string (default "00000000-0000-0000-0000-0000000010000") - Xero-Application-Id
- xeroUserId string (default "00000000-0000-0000-0000-0000030000000") - Xero-User-Id
Return Type
- Statements|error - success returns Statements array of objects response
createStatements
function createStatements(string xeroTenantId, Statements payload) returns Statements|error
Creates one or more new statements
Parameters
- xeroTenantId string - Xero identifier for Tenant
- payload Statements - Statements array of objects in the body
Return Type
- Statements|error - Success returns Statements array of objects in response
getStatement
Retrieve single statement based on unique id provided
Records
xero.bankfeeds: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
xero.bankfeeds: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
xero.bankfeeds: EndBalance
The StartBalance plus all the Statement Line Amounts should be equal to the EndBalance Amount.
Fields
- amount decimal? - Amount
- creditDebitIndicator CreditDebitIndicator? - If the statement balances are credit or debit, the CreditDebitIndicator should be specified from the perspective of the Customer.
xero.bankfeeds: Error
On error, the API consumer will receive an HTTP response with a HTTP Status Code of 4xx or 5xx and a Content-Type of application/problem+json.
Fields
- title string? - Human readable high level error description.
- status int? - The numeric HTTP Status Code, e.g. 404
- detail string? - Human readable detailed error description.
- 'type string? - Identifies the type of error.
xero.bankfeeds: FeedConnection
Fields
- id string? - GUID used to identify the Account.
- accountToken string? - This account identifier is generated by the financial institute (FI). This must be unique for your financial institute.
- accountNumber string? - String(40) when AccountType is BANK String(4) when AccountType is CREDITCARD The Account Number is used to match the feed to a Xero Bank Account. The API will create a new Xero Bank Account if a match to an existing Xero Bank Account is not found. Only the last 4 digits must be supplied for Credit Card numbers. Must be included if AccountId is not specified.
- accountName string? - The Account Name will be used for the creation of a new Xero Bank Account if a matching Xero Bank Account is not found.
- accountId string? - Xero identifier for a bank account in Xero. Must be included if AccountNumber is not specified.
- accountType string? - High level bank account type - BANK CREDITCARD BANK encompasses all bank account types other than credit cards.
- currency CurrencyCode? - 3 letter alpha code for the ISO-4217 currency code, e.g. USD, AUD.
- country CountryCode? - ISO-3166 alpha-2 country code, e.g. US, AU This element is required only when the Application supports multi-region. Talk to your Partner Manager to confirm if this is the case.
- status string? - the current status of the feed connection
- 'error Error? - On error, the API consumer will receive an HTTP response with a HTTP Status Code of 4xx or 5xx and a Content-Type of application/problem+json.
xero.bankfeeds: FeedConnections
Fields
- pagination Pagination? -
- items FeedConnection[]? -
xero.bankfeeds: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://identity.xero.com/connect/token") - Refresh URL
xero.bankfeeds: Pagination
Fields
- page int? - Page number which specifies the set of records to retrieve. Example - https://api.xero.com/bankfeeds.xro/1.0/Statements?page=2 to get the second set of the records. When page value is not a number or a negative number, by default, the first set of records is returned.
- pageSize int? - Page size which specifies how many records per page will be returned (default 50). Example - https://api.xero.com/bankfeeds.xro/1.0/Statements?pageSize=100 to specify page size of 100.
- pageCount int? - Number of pages available
- itemCount int? - Number of items returned
xero.bankfeeds: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
xero.bankfeeds: StartBalance
The starting balance of the statement
Fields
- amount decimal? - decimal(19,4) unsigned Opening/closing balance amount.
- creditDebitIndicator CreditDebitIndicator? - If the statement balances are credit or debit, the CreditDebitIndicator should be specified from the perspective of the Customer.
xero.bankfeeds: Statement
Fields
- id string? - GUID used to identify the Statement.
- feedConnectionId string? - The Xero generated feed connection Id that identifies the Xero Bank Account Container into which the statement should be delivered. This is obtained by calling GET FeedConnections.
- status string? - Current status of statements
- startDate string? - Opening balance date (can be no older than one year from the current date) ISO-8601 YYYY-MM-DD
- endDate string? - Closing balance date ISO-8601 YYYY-MM-DD
- startBalance StartBalance? - The starting balance of the statement
- endBalance EndBalance? - The StartBalance plus all the Statement Line Amounts should be equal to the EndBalance Amount.
- statementLines StatementLines? -
- errors Error[]? -
- statementLineCount int? -
xero.bankfeeds: StatementLine
the lines details for a statement
Fields
- postedDate string? - The date that the transaction was processed or cleared as seen in internet banking ISO-8601 YYYY-MM-DD
- description string? - Transaction description
- amount decimal? - Transaction amount
- creditDebitIndicator CreditDebitIndicator? - If the statement balances are credit or debit, the CreditDebitIndicator should be specified from the perspective of the Customer.
- transactionId string? - Financial institute's internal transaction identifier. If provided this field is factored into duplicate detection.
- payeeName string? - Typically the merchant or payee name
- reference string? - Optional field to enhance the Description
- chequeNumber string? - The cheque/check number
- transactionType string? - Descriptive transaction type
xero.bankfeeds: Statements
Fields
- pagination Pagination? -
- items Statement[]? -
String types
xero.bankfeeds: CountryCode
CountryCode
ISO-3166 alpha-2 country code, e.g. US, AU This element is required only when the Application supports multi-region. Talk to your Partner Manager to confirm if this is the case.
xero.bankfeeds: CreditDebitIndicator
CreditDebitIndicator
If the statement balances are credit or debit, the CreditDebitIndicator should be specified from the perspective of the Customer.
xero.bankfeeds: CurrencyCode
CurrencyCode
3 letter alpha code for the ISO-4217 currency code, e.g. USD, AUD.
Import
import ballerinax/xero.bankfeeds;
Metadata
Released date: over 2 years ago
Version: 1.4.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.2.1
GraalVM compatible: Yes
Pull count
Total: 3
Current verison: 1
Weekly downloads
Keywords
Finance/Accounting
Cost/Paid
Contributors
Dependencies