worldbank
Module worldbank
API
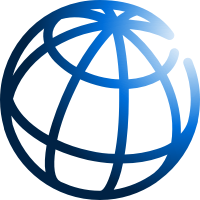
ballerinax/worldbank Ballerina library
Overview
This is a generated connector from World Bank API v2 OpenAPI Specification.
The World Bank Indicators API provides access to nearly 16,000 time series indicators. Most of these indicators are available online through tools such as Databank
and the Open Data
website. The API provides programmatic access to this same data. Many data series date back over 50 years, and can be used to create interesting applications.
Quickstart
To use the WorldBank connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
import ballerinax/worldbank
Step 2: Create a new connector instance
worldbank:Client worldbankClient = check new ();
Step 3: Invoke connector operation
- Get current population data by country.
public function main() { worldbank:CountryPopulation[] populationDetails = check worldbankClient->getPopulationByCountry(country_code="LKA" ,date="2000"); int population = <int>populationDetails[0]?.value; }
- Use
bal run
command to compile and run the Ballerina program.
Clients
worldbank: Client
This is a generated connector from World Bank API v2 OpenAPI Specification.
The World Bank Indicators API provides access to nearly 16,000 time series indicators. Most of these indicators are available online through tools such as Databank
and the Open Data
website. The API provides programmatic access to this same data. Many data series date back over 50 years, and can be used to create interesting applications.
Constructor
Gets invoked to initialize the connector
.
The connector initialization doesn't require setting the API credentials.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "http://api.worldbank.org/v2/" - URL of the target service
getPopulation
function getPopulation(string? date, int? page, int? perPage) returns IndicatorInformation[]|error
Get population of each country
Parameters
- date string? (default "2010") - Date by year (2010), Date-range by year(2005:2010), month(2010M02:2010M08) or quarter(2012Q1:2012Q3) that scopes the result-set.
- page int? (default ()) - Page number
- perPage int? (default ()) - Per page record count
Return Type
- IndicatorInformation[]|error - Population of each countries
getPopulationByCountry
function getPopulationByCountry(string countryCode, string? date, int? page, int? perPage) returns IndicatorInformation[]|error
Get population of a country
Parameters
- countryCode string - Country code (Example- AFG, ALB, LKA)
- date string? (default "2010") - Date by year (2010), Date-range by year(2005:2010), month(2010M02:2010M08) or quarter(2012Q1:2012Q3) that scopes the result-set.
- page int? (default ()) - Page number
- perPage int? (default ()) - Per page record count
Return Type
- IndicatorInformation[]|error - Yearly population of the given country
getGDP
function getGDP(string? date, int? page, int? perPage) returns IndicatorInformation[]|error
Get GDP of each country.
Parameters
- date string? (default "2010") - Date by year (2010), Date-range by year(2005:2010), month(2010M02:2010M08) or quarter(2012Q1:2012Q3) that scopes the result-set.
- page int? (default ()) - Page number
- perPage int? (default ()) - Per page record count
Return Type
- IndicatorInformation[]|error - GDP of each country
getGDPByCountry
function getGDPByCountry(string countryCode, string? date, int? page, int? perPage) returns IndicatorInformation[]|error
Get GDP of a country.
Parameters
- countryCode string - Country code (Example- AFG, ALB, LKA)
- date string? (default "2010") - Date by year (2010), Date-range by year(2005:2010), month(2010M02:2010M08) or quarter(2012Q1:2012Q3) that scopes the result-set.
- page int? (default ()) - Page number
- perPage int? (default ()) - Per page record count
Return Type
- IndicatorInformation[]|error - Yearly GDP of the given country
getAccessToElectricityPercentage
function getAccessToElectricityPercentage(string? date, int? page, int? perPage) returns IndicatorInformation[]|error
Get percentage of population with access to electricity of countries in the world.
Parameters
- date string? (default "2010") - Date by year (2010), Date-range by year(2005:2010), month(2010M02:2010M08) or quarter(2012Q1:2012Q3) that scopes the result-set.
- page int? (default ()) - Page number
- perPage int? (default ()) - Per page record count
Return Type
- IndicatorInformation[]|error - Population percentage having electricity of each country.
getAccessToElectricityPercentageByCountry
function getAccessToElectricityPercentageByCountry(string countryCode, string? date, int? page, int? perPage) returns IndicatorInformation[]|error
Get percentage of population with access to electricity of a given country.
Parameters
- countryCode string - Country code (Example- AFG, ALB, LKA)
- date string? (default "2010") - Date by year (2010), Date-range by year(2005:2010), month(2010M02:2010M08) or quarter(2012Q1:2012Q3) that scopes the result-set.
- page int? (default ()) - Page number
- perPage int? (default ()) - Per page record count
Return Type
- IndicatorInformation[]|error - Yearly population percentage having electricity of the given country.
getYouthLiteracyRate
function getYouthLiteracyRate(string? date, int? page, int? perPage) returns IndicatorInformation[]|error
Get literacy rate of youth (% of people ages 15-24) of countries in the world.
Parameters
- date string? (default "2010") - Date by year (2010), Date-range by year(2005:2010), month(2010M02:2010M08) or quarter(2012Q1:2012Q3) that scopes the result-set.
- page int? (default ()) - Page number
- perPage int? (default ()) - Per page record count
Return Type
- IndicatorInformation[]|error - Youth literacy rate of each country.
getYouthLiteracyRateByCountry
function getYouthLiteracyRateByCountry(string countryCode, string? date, int? page, int? perPage) returns IndicatorInformation[]|error
Get literacy rate of youth (% of people ages 15-24) of a country.
Parameters
- countryCode string - Country code (Example- AFG, ALB, LKA)
- date string? (default "2010") - Date by year (2010), Date-range by year(2005:2010), month(2010M02:2010M08) or quarter(2012Q1:2012Q3) that scopes the result-set.
- page int? (default ()) - Page number
- perPage int? (default ()) - Per page record count
Return Type
- IndicatorInformation[]|error - Youth literacy rate of the given country.
getGovernmentExpenditureOnPrimaryEducation
function getGovernmentExpenditureOnPrimaryEducation(string? date, int? page, int? perPage) returns IndicatorInformation[]|error
Get government expenditure on primary education of each country
Parameters
- date string? (default "2010") - Date by year (2010), Date-range by year(2005:2010), month(2010M02:2010M08) or quarter(2012Q1:2012Q3) that scopes the result-set.
- page int? (default ()) - Page number
- perPage int? (default ()) - Per page record count
Return Type
- IndicatorInformation[]|error - Government expenditure on primary education of each country.
getGovernmentExpenditureOnPrimaryEducationByCountry
function getGovernmentExpenditureOnPrimaryEducationByCountry(string countryCode, string? date, int? page, int? perPage) returns IndicatorInformation[]|error
Get government expenditure on primary education of a country.
Parameters
- countryCode string - Country code (Example- AFG, ALB, LKA)
- date string? (default "2010") - Date by year (2010), Date-range by year(2005:2010), month(2010M02:2010M08) or quarter(2012Q1:2012Q3) that scopes the result-set.
- page int? (default ()) - Page number
- perPage int? (default ()) - Per page record count
Return Type
- IndicatorInformation[]|error - Government expenditure on primary education of a country.
Records
worldbank: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy? ProxyConfig - Proxy server related options
worldbank: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_1_1) - The HTTP version understood by the client
- http1Settings? ClientHttp1Settings - Configurations related to HTTP/1.x protocol
- http2Settings? ClientHttp2Settings - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig? PoolConfiguration - Configurations associated with request pooling
- cache? CacheConfig - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker? CircuitBreakerConfig - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig? RetryConfig - Configurations associated with retrying
- responseLimits? ResponseLimitConfigs - Configurations associated with inbound response size limits
- secureSocket? ClientSecureSocket - SSL/TLS-related options
- proxy? ProxyConfig - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
worldbank: Country
Represent a Country
Fields
- id string - Country code
- value string - Country name
worldbank: Indicator
Data indicator
Fields
- id string - Id of the indicator
- value string - Value represent by the indicator
worldbank: IndicatorInformation
Represent country population.
Fields
- indicator Indicator - Data indicator
- country Country - Represent a Country
- date string - Date-range by year, month or quarter that scopes the result-set.
- value int? - Country population
- countryiso3code string - Country ISO3 Code
- unit string - Unit of measure
- 'decimal decimal - Decimal
- obs_status? string - OBS Status
worldbank: PaginationData
Fields
- page int -
- pages int -
- per_page int -
- total int -
- sourceid string? -
- sourcename string? -
- lastupdated string? -
worldbank: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
Array types
worldbank: WorldBankResponse
WorldBankResponse
Import
import ballerinax/worldbank;
Metadata
Released date: about 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 57388
Current verison: 24623
Weekly downloads
Keywords
Business Intelligence/Analytics
Cost/Free
Contributors
Dependencies