wordpress
Module wordpress
API
Definitions
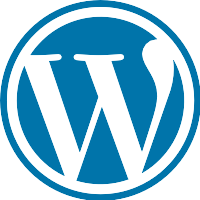
ballerinax/wordpress Ballerina library
Overview
This is a generated connector for WordPress API v1.0 OpenAPI specification.
The WordPress REST API provides an interface for applications to interact with your WordPress site. WordPress API provides a rich set of tools and interfaces for building sites, and you should not feel pressured to use the REST API if your site is already working the way you expect. You do not need to use the REST API to build a WordPress theme or plugin.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a WordPress account account
- Obtain tokens by following this guide.
Quickstart
To use the WordPress connector in your Ballerina application, update the .bal file as follows
Step 1: Import connector
First, import the ballerinax/wordpress
module into the Ballerina project.
import ballerinax/wordpress;
Step 2: Create a new connector instance
Create a wordpress:ClientConfig
with the auth key and initialize the connector with it.
wordpress:ClientConfig configuration = {auth : { token = "<Enter your token here>" } }; wordpress:Client wpClient = check new(configuration, serviceUrl);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to get all posts.
Get all posts
public function main() { wordpress:Post[]|error posts = check wpClient->getPosts(); if (posts is wordpress:Post[]) { foreach var post in posts { log:printInfo(post.toString()); } } else { log:printError(posts.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
wordpress: Client
This is a generated connector for WordPress API v1.0 OpenAPI specification. The WordPress REST API provides an interface for applications to interact with your WordPress site. WordPress API provides a rich set of tools and interfaces for building sites, and you should not feel pressured to use the REST API if your site is already working the way you expect. You do not need to use the REST API to build a WordPress theme or plugin.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a WordPress account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string - URL of the target service
getPosts
List Posts
Parameters
- context string? (default ()) - Scope under which the request is made; determines fields present in response.
createPosts
Create Post
Parameters
- payload PostsBody -
getSinglePost
Get Single Post
Parameters
- id string - Id of object
- context string? (default ()) - Scope under which the request is made; determines fields present in response.
updateSinglePost
function updateSinglePost(string id, PostsIdBody payload, string? context) returns Post|error
Update Single Post
Parameters
- id string - Id of object
- payload PostsIdBody -
- context string? (default ()) - Scope under which the request is made; determines fields present in response.
deleteSinglePost
Delete Single Post
Parameters
- id string - Id of object
- force boolean? (default ()) - Whether to bypass trash and force deletion.
getPostRevisions
Get post revisions
Parameters
- id string - Id of object
- context string? (default ()) - Scope under which the request is made; determines fields present in response.
getSinglePostRevisions
function getSinglePostRevisions(string id, string revisionid, string? context) returns Revision|error
Get single post revisions
Parameters
- id string - Id of object
- revisionid string - Id of revision
- context string? (default ()) - Scope under which the request is made; determines fields present in response.
deleteSinglePostRevisions
Delete single post revisions
listPages
List Pages
Parameters
- context string? (default ()) - Scope under which the request is made; determines fields present in response.
createPage
Create Page
Parameters
- payload PagesBody -
getSinglePage
Get Single Page
Parameters
- id string - Id of object
- context string? (default ()) - Scope under which the request is made; determines fields present in response.
updateSinglePage
function updateSinglePage(string id, PagesIdBody payload) returns Page|error
Update Single Page
deleteSinglePage
Delete Single Page
Parameters
- id string - Id of object
- force boolean? (default ()) - Whether to bypass trash and force deletion.
listMedia
List Media
Parameters
- context string? (default ()) - Scope under which the request is made; determines fields present in response.
createMedia
Create Media
Parameters
- payload MediaBody -
getSingleMedia
Get Single Media
Parameters
- id string - Id of object
- context string? (default ()) - Scope under which the request is made; determines fields present in response.
updateSingleMedia
function updateSingleMedia(string id, MediaIdBody payload) returns Media|error
Update Single Media
delteSingleMedia
Delete Single Media
Parameters
- id string - Id of object
- force boolean? (default ()) - Whether to bypass trash and force deletion.
listType
List Type
Parameters
- context string? (default ()) - Scope under which the request is made; determines fields present in response.
getSingleType
Get Single Type
Parameters
- id string - Id of object
- context string? (default ()) - Scope under which the request is made; determines fields present in response.
listStatus
List Status
Parameters
- context string? (default ()) - Scope under which the request is made; determines fields present in response.
getSingleStatus
Get Single Status
Parameters
- id string - Id of object
- context string? (default ()) - Scope under which the request is made; determines fields present in response.
listComments
List Comments
Parameters
- context string? (default ()) - Scope under which the request is made; determines fields present in response.
createComment
function createComment(CommentsBody payload) returns Comment|error
Create Comment
Parameters
- payload CommentsBody -
getSingleComment
Get Single Comment
Parameters
- id string - Id of object
- context string? (default ()) - Scope under which the request is made; determines fields present in response.
updateSingleComment
function updateSingleComment(string id, CommentsIdBody payload) returns Comment|error
Update Single Comment
deleteSingleComment
Delete Single Comment
Parameters
- id string - Id of object
- force boolean? (default ()) - Whether to bypass trash and force deletion.
listTaxonomy
List Taxonomy
Parameters
- context string? (default ()) - Scope under which the request is made; determines fields present in response.
getSingleTaxonomy
Get Single Taxonomy
Parameters
- id string - Id of object
- context string? (default ()) - Scope under which the request is made; determines fields present in response.
listCategories
List categories
Parameters
- context string? (default ()) - Scope under which the request is made; determines fields present in response.
createCategory
function createCategory(CategoriesBody payload) returns Category|error
Create Category
Parameters
- payload CategoriesBody -
getSingleCategory
Get Single Category
Parameters
- id string - Id of object
- context string? (default ()) - Scope under which the request is made; determines fields present in response.
updateSingleCategory
function updateSingleCategory(string id, CategoriesIdBody payload) returns Category|error
Update Single Category
deleteSingleCategory
Delete Single Category
Parameters
- id string - Id of object
- force boolean? (default ()) - Whether to bypass trash and force deletion.
gettagList
List Tags
Parameters
- context string? (default ()) - Scope under which the request is made; determines fields present in response.
createTag
Create Tag
Parameters
- payload TagsBody -
getSingleTag
Get Single Tag
Parameters
- id string - Id of object
- context string? (default ()) - Scope under which the request is made; determines fields present in response.
updateSingleTag
function updateSingleTag(string id, TagsIdBody payload) returns Tag|error
Update Single Tag
deleteSingleTag
Delete Single Tag
Parameters
- id string - Id of object
- force boolean? (default ()) - Whether to bypass trash and force deletion.
listUsers
List Users
Parameters
- context string? (default ()) - Scope under which the request is made; determines fields present in response.
createUser
Create User
Parameters
- payload UsersBody -
getSingleUser
Get Single User
Parameters
- id string - Id of object
- context string? (default ()) - Scope under which the request is made; determines fields present in response.
updateSingleUser
function updateSingleUser(string id, UsersIdBody payload) returns User|error
Update Single User
deleteSingleUser
Delete Single User
Records
wordpress: CategoriesBody
Fields
- description string? - The description for the resource
- name string - HTML title for the resource.
- slug string? - Limit result set to posts with a specific slug.
- parent int? - The id for the parent of the object.
wordpress: CategoriesIdBody
Fields
- description string? - The description for the resource
- name string - HTML title for the resource.
- slug string? - Limit result set to posts with a specific slug.
- parent int? - The id for the parent of the object.
wordpress: Category
Fields
- Fields Included from *Tag
- parent int? - The id for the parent of the object.
wordpress: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
wordpress: Comment
Fields
- id int? - Unique identifier for the object.
- author int? - The id for the author of the object.
- author_email string? - Email address for the object author.
- author_ip string? - IP address for the object author.
- author_name string? - Display name for the object author.
- author_url string? - URL for the object author.
- author_user_agent string? - User agent for the object author.
- content record {}? - The content for the object.
- date string? - The date the object was published, in the site's timezone.
- date_gmt string? - The date the object was published, as GMT.
- karma string? - Karma for the object
- link string? - URL to the object.
- parent int? - The id for the parent of the object.
- post int? - The id for the associated post of the resource.
- status string? - A named status for the object.
- 'type string? - Type of Post for the object.
- author_avatar_urls string? - Avatar URLs for the object author.
wordpress: CommentsBody
Fields
- date string? - The date the object was published, in the site's timezone.
- date_gmt string? - The date the object was published, as GMT.
- password string? - The A password to protect access to the post.
- slug string? - Limit result set to posts with a specific slug.
- status string? - Limit result set to posts assigned a specific status.Default publish
- title record {}? - The title for the object.
- author int? - Limit result set to posts assigned to specific authors.
- comment_status string? - Whether or not comments are open on the object
- ping_status string? - Whether or not the object can be pinged.
- alt_text string? - Alternative text to display when resource is not displayed.
- caption string? - The caption for the resource.
- description string? - The description for the resource
- post string? - The id for the associated post of the resource.
wordpress: CommentsIdBody
Fields
- date string? - The date the object was published, in the site's timezone.
- date_gmt string? - The date the object was published, as GMT.
- password string? - The A password to protect access to the post.
- slug string? - Limit result set to posts with a specific slug.
- status string? - Limit result set to posts assigned a specific status.Default publish
- title record {}? - The title for the object.
- author int? - Limit result set to posts assigned to specific authors.
- comment_status string? - Whether or not comments are open on the object
- ping_status string? - Whether or not the object can be pinged.
- alt_text string? - Alternative text to display when resource is not displayed.
- caption string? - The caption for the resource.
- description string? - The description for the resource
- post string? - The id for the associated post of the resource.
wordpress: CommonPostPage
Fields
- date string? - The date the object was published, in the site's timezone.
- date_gmt string? - The date the object was published, as GMT.
- guid record {}? - The globally unique identifier for the object.
- id int? - Unique identifier for the object.
- link string? - URL to the object.
- modified string? - The date the object was last modified, in the site's timezone.
- modified_gmt string? - The date the object was last modified, as GMT.
- password string? - The A password to protect access to the post.
- slug string? - An alphanumeric identifier for the object unique to its type.
- status string? - A named status for the object.
- 'type string? - Type of Post for the object.
- title record {}? - The title for the object.
- content record {}? - The content for the object.
- author int? - The id for the author of the object.
- excerpt record {}? - The excerpt for the object
- featured_media int? - The id of the featured media for the object.
- comment_status string? - Whether or not comments are open on the object
- ping_status string? - Whether or not the object can be pinged.
wordpress: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
wordpress: Media
Fields
- date string? - The date the object was published, in the site's timezone.
- date_gmt string? - The date the object was published, as GMT.
- guid record {}? - The globally unique identifier for the object.
- id int? - Unique identifier for the object.
- modified string? - The date the object was last modified, in the site's timezone.
- modified_gmt string? - The date the object was last modified, as GMT.
- password string? - The A password to protect access to the post.
- slug string? - An alphanumeric identifier for the object unique to its type.
- status string? - A named status for the object.
- 'type string? - Type of Post for the object.
- title string? - The title for the object.
- author int? - The id for the author of the object.
- comment_status string? - Whether or not comments are open on the object
- ping_status string? - Whether or not the object can be pinged.
- alt_text string? - Alternative text to display when resource is not displayed
- caption string? - The caption for the resource.
- description string? - The description for the resource.
- media_type string? - Type of resource.
- mime_type string? - Mime type of resource.
- media_details string? - Details about the resource file, specific to its type.
- post int? - The id for the associated post of the resource.
- source_url string? - URL to the original resource file.
wordpress: MediaBody
Fields
- date string? - The date the object was published, in the site's timezone.
- date_gmt string? - The date the object was published, as GMT.
- password string? - The A password to protect access to the post.
- slug string? - Limit result set to posts with a specific slug.
- status string? - Limit result set to posts assigned a specific status.Default publish
- title record {}? - The title for the object.
- author int? - Limit result set to posts assigned to specific authors.
- comment_status string? - Whether or not comments are open on the object
- ping_status string? - Whether or not the object can be pinged.
- alt_text string? - Alternative text to display when resource is not displayed.
- caption string? - The caption for the resource.
- description string? - The description for the resource
- post string? - The id for the associated post of the resource.
wordpress: MediaIdBody
Fields
- date string? - The date the object was published, in the site's timezone.
- date_gmt string? - The date the object was published, as GMT.
- password string? - The A password to protect access to the post.
- slug string? - Limit result set to posts with a specific slug.
- status string? - Limit result set to posts assigned a specific status.Default publish
- title record {}? - The title for the object.
- author int? - Limit result set to posts assigned to specific authors.
- comment_status string? - Whether or not comments are open on the object
- ping_status string? - Whether or not the object can be pinged.
- alt_text string? - Alternative text to display when resource is not displayed.
- caption string? - The caption for the resource.
- description string? - The description for the resource
- post string? - The id for the associated post of the resource.
wordpress: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "/oauth1/request") - Refresh URL
wordpress: Page
Fields
- Fields Included from *CommonPostPage
- date string
- date_gmt string
- guid record { anydata... }
- id int
- link string
- modified string
- modified_gmt string
- password string
- slug string
- status string
- type string
- title record { anydata... }
- content record { anydata... }
- author int
- excerpt record { anydata... }
- featured_media int
- comment_status string
- ping_status string
- anydata...
- parent int? - The id for the parent of the object.
- menu_order int? - The order of the object in relation to other object of its type.
- template string? - The theme file to use to display the object.
wordpress: PagesBody
Fields
- date string? - The date the object was published, in the site's timezone.
- date_gmt string? - The date the object was published, as GMT.
- password string? - The A password to protect access to the post.
- slug string? - Limit result set to posts with a specific slug.
- status string? - Limit result set to posts assigned a specific status.Default publish
- parent int? - The id for the parent of the object.
- title record {}? - The title for the object.
- content string? - The content for the object.
- author int? - Limit result set to posts assigned to specific authors.
- excerpt record {}? - The excerpt for the object
- featured_media string? - The id of the featured media for the object.
- comment_status string? - Whether or not comments are open on the object
- ping_status string? - Whether or not the object can be pinged.
- menu_order int? - The order of the object in relation to other object of its type.
- template int? - The theme file to use to display the object.
wordpress: PagesIdBody
Fields
- date string? - The date the object was published, in the site's timezone.
- date_gmt string? - The date the object was published, as GMT.
- password string? - The A password to protect access to the post.
- slug string? - Limit result set to posts with a specific slug.
- status string? - Limit result set to posts assigned a specific status.Default publish
- parent int? - The id for the parent of the object.
- title record {}? - The title for the object.
- content string? - The content for the object.
- author int? - Limit result set to posts assigned to specific authors.
- excerpt record {}? - The excerpt for the object
- featured_media string? - The id of the featured media for the object.
- comment_status string? - Whether or not comments are open on the object
- ping_status string? - Whether or not the object can be pinged.
- menu_order int? - The order of the object in relation to other object of its type.
- template int? - The theme file to use to display the object.
wordpress: Post
Fields
- Fields Included from *CommonPostPage
- date string
- date_gmt string
- guid record { anydata... }
- id int
- link string
- modified string
- modified_gmt string
- password string
- slug string
- status string
- type string
- title record { anydata... }
- content record { anydata... }
- author int
- excerpt record { anydata... }
- featured_media int
- comment_status string
- ping_status string
- anydata...
- format string? - The format for the object.
- sticky boolean? - Whether or not the object should be treated as sticky.
- categories int[]? - The terms assigned to the object in the category taxonomy.
- tags int[]? - he terms assigned to the object in the post_tag taxonomy.
wordpress: PostsBody
Fields
- date string? - The date the object was published, in the site's timezone.
- date_gmt string? - The date the object was published, as GMT.
- password string? - The A password to protect access to the post.
- slug string? - Limit result set to posts with a specific slug.
- status string? - Limit result set to posts assigned a specific status.Default publish
- title record {}? - The title for the object.
- content string? - The content for the object.
- author int? - Limit result set to posts assigned to specific authors.
- excerpt record {}? - The excerpt for the object
- featured_media string? - The id of the featured media for the object.
- comment_status string? - Whether or not comments are open on the object
- ping_status string? - Whether or not the object can be pinged.
- sticky boolean? - Whether or not the object should be treated as sticky.
- categories string[]? - Limit result set to all items that have the specified term assigned in the categories taxonomy.
- tags string[]? - Limit result set to all items that have the specified term assigned in the tags taxonomy.
wordpress: PostsIdBody
Fields
- page int? - Current page of the collection. Default 1
- per_page int? - Maximum number of items to be returned in result set. Default 10
- search string? - Limit results to those matching a string.
- after string? - Limit response to resources published after a given ISO8601 compliant date.
- author int? - Limit result set to posts assigned to specific authors.
- author_exclude string? - Ensure result set excludes posts assigned to specific authors.
- before string? - Limit response to resources published before a given ISO8601 compliant date.
- exclude string? - Ensure result set excludes specific ids.
- include string? - Ensure result set includes specific ids.
- offset string? - Offset the result set by a specific number of items.
- 'order string? - Order sort attribute ascending or descending. Default desc
- orderby string? - Sort collection by object attribute. Default date
- slug string? - Limit result set to posts with a specific slug.
- status string? - Limit result set to posts assigned a specific status.Default publish
- filter string? - Use WP Query arguments to modify the response; private query vars require appropriate authorization.
- categories string[]? - Limit result set to all items that have the specified term assigned in the categories taxonomy.
- tags string[]? - Limit result set to all items that have the specified term assigned in the tags taxonomy.
wordpress: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
wordpress: Revision
Fields
- date string? - The date the object was published, in the site's timezone.
- date_gmt string? - The date the object was published, as GMT.
- guid record {}? - The globally unique identifier for the object.
- id int? - Unique identifier for the object.
- modified string? - The date the object was last modified, in the site's timezone.
- modified_gmt string? - The date the object was last modified, as GMT.
- slug string? - An alphanumeric identifier for the object unique to its type.
- title string? - The title for the object.
- content record {}? - The content for the object.
- author int? - The id for the author of the object.
- excerpt record {}? - The excerpt for the object
- parent int? - The id for the parent of the object.
wordpress: Status
Fields
- 'private boolean? - Whether posts with this resource should be private.
- protected boolean? - Whether posts with this resource should be protected.
- 'public boolean? - Whether posts of this resource should be shown in the front end of the site.
- queryable boolean? - Whether posts with this resource should be publicly-queryable.
- show_in_list boolean? - Whether to include posts in the edit listing for their post type.
- name string? - The title for the resource.
- slug string? - An alphanumeric identifier for the object unique to its type.
wordpress: Tag
Fields
- id int? - Unique identifier for the object.
- count int? - Number of published posts for the resource.
- description string? - The description for the resource.
- link string? - URL to the object.
- name string? - The title for the resource.
- slug string? - An alphanumeric identifier for the object unique to its type.
- taxonomy string? - Type attribution for the resource.
wordpress: TagsBody
Fields
- description string? - The description for the resource
- name string - HTML title for the resource.
- slug string? - Limit result set to posts with a specific slug.
wordpress: TagsIdBody
Fields
- description string? - The description for the resource
- name string - HTML title for the resource.
- slug string? - Limit result set to posts with a specific slug.
wordpress: Taxonomy
Fields
- Fields Included from *Type
- show_cloud boolean? - Whether or not the term cloud should be displayed.
- types string[]? - Types associated with resource.
wordpress: Type
Fields
- capabilities string[]? - All capabilities used by the resource.
- description string? - A human-readable description of the resource.
- hierarchical boolean? - Whether or not the resource should have children.
- labels record {}? - Human-readable labels for the resource for various contexts.
- name string? - The title for the resource.
- slug string? - An alphanumeric identifier for the object unique to its type.
wordpress: User
Fields
- id int? - Unique identifier for the object.
- username string? - Login name for the resource.
- name string? - Display name for the resource.
- first_name string? - First name for the resource.
- last_name string? - Last name for the resource.
- email string? - The email address for the resource.
- url string? - URL of the resource.
- description string? - Description of the resource.
- link string? - Author URL to the resource.
- nickname string? - The nickname for the resource.
- slug string? - An alphanumeric identifier for the resource.
- registered_date string? - Registration date for the resource.
- roles string[]? - Roles assigned to the resource.
- password string? - Password for the resource (never included).
- capabilities string[]? - All capabilities assigned to the resource.
- extra_capabilities string[]? - Any extra capabilities assigned to the resource.
- avatar_urls string[]? - Avatar URLs for the resource.
wordpress: UsersBody
Fields
- username string? - The user name for the resource.
- name string - HTML title for the resource.
- first_name string? - The first name for the resource.
- last_name string? - The last name for the resource.
- email string? - Email of the resource.
- url string? - URL of the resource.
- description string? - The description for the resource
- nickname string? - The nickname for the resource.
- slug string? - Limit result set to posts with a specific slug.
- roles string[]? - Roles assigned to the resource.
- password string? - The A password to protect access to the post.
- capabilities string[]? - All capabilities used by the resource.
wordpress: UsersIdBody
Fields
- username string? - The user name for the resource.
- name string - HTML title for the resource.
- first_name string? - The first name for the resource.
- last_name string? - The last name for the resource.
- email string? - Email of the resource.
- url string? - URL of the resource.
- description string? - The description for the resource
- nickname string? - The nickname for the resource.
- slug string? - Limit result set to posts with a specific slug.
- roles string[]? - Roles assigned to the resource.
- password string? - The A password to protect access to the post.
- capabilities string[]? - All capabilities used by the resource.
String types
wordpress: FormdataAfter
FormdataAfter
Limit response to resources published after a given ISO8601 compliant date.
wordpress: FormdataAltText
FormdataAltText
Alternative text to display when resource is not displayed.
wordpress: FormdataAuthor
FormdataAuthor
Limit result set to posts assigned to specific authors.
wordpress: FormdataAuthorEmail
FormdataAuthorEmail
Limit result set to that from a specific author email. Requires authorization.
wordpress: FormdataAuthorExclude
FormdataAuthorExclude
Ensure result set excludes posts assigned to specific authors.
wordpress: FormdataBefore
FormdataBefore
Limit response to resources published before a given ISO8601 compliant date.
wordpress: FormdataCaption
FormdataCaption
The caption for the resource.
wordpress: FormdataCommentStatus
FormdataCommentStatus
Whether or not comments are open on the object
wordpress: FormdataContent
FormdataContent
The content for the object.
wordpress: FormdataDate
FormdataDate
The date the object was published, in the site's timezone.
wordpress: FormdataDateGmt
FormdataDateGmt
The date the object was published, as GMT.
wordpress: FormdataDescription
FormdataDescription
The description for the resource
wordpress: FormdataEmail
FormdataEmail
Email of the resource.
wordpress: FormdataExcerpt
FormdataExcerpt
The excerpt for the object
wordpress: FormdataExclude
FormdataExclude
Ensure result set excludes specific ids.
wordpress: FormdataFeaturedMedia
FormdataFeaturedMedia
The id of the featured media for the object.
wordpress: FormdataFilter
FormdataFilter
Use WP Query arguments to modify the response; private query vars require appropriate authorization.
wordpress: FormdataFirstName
FormdataFirstName
The first name for the resource.
wordpress: FormdataInclude
FormdataInclude
Ensure result set includes specific ids.
wordpress: FormdataKarma
FormdataKarma
Limit result set to that of a particular comment karma. Requires authorization
wordpress: FormdataLastName
FormdataLastName
The last name for the resource.
wordpress: FormdataMediaType
FormdataMediaType
Type of resource.
wordpress: FormdataMimeType
FormdataMimeType
Alternative text to display when resource is not displayed.
wordpress: FormdataName
FormdataName
HTML title for the resource.
wordpress: FormdataNickname
FormdataNickname
The nickname for the resource.
wordpress: FormdataOffset
FormdataOffset
Offset the result set by a specific number of items.
wordpress: FormdataOrder
FormdataOrder
Order sort attribute ascending or descending. Default desc
wordpress: FormdataOrderby
FormdataOrderby
Sort collection by object attribute. Default date
wordpress: FormdataParentExclude
FormdataParentExclude
Ensure result set excludes specific ids.
wordpress: FormdataPassword
FormdataPassword
The A password to protect access to the post.
wordpress: FormdataPingStatus
FormdataPingStatus
Whether or not the object can be pinged.
wordpress: FormdataPost
FormdataPost
The id for the associated post of the resource.
wordpress: FormdataReassign
FormdataReassign
wordpress: FormdataSearch
FormdataSearch
Limit results to those matching a string.
wordpress: FormdataSlug
FormdataSlug
Limit result set to posts with a specific slug.
wordpress: FormdataStatus
FormdataStatus
Limit result set to posts assigned a specific status.Default publish
wordpress: FormdataTitle
FormdataTitle
The title for the object.
wordpress: FormdataType
FormdataType
Limit result set to comments assigned a specific type. Requires authorization. Default comment
wordpress: FormdataUrl
FormdataUrl
URL of the resource.
wordpress: FormdataUsername
FormdataUsername
The user name for the resource.
Integer types
wordpress: FormdataMenuOrder
FormdataMenuOrder
The order of the object in relation to other object of its type.
wordpress: FormdataPage
FormdataPage
Current page of the collection. Default 1
wordpress: FormdataParent
FormdataParent
The id for the parent of the object.
wordpress: FormdataPerPage
FormdataPerPage
Maximum number of items to be returned in result set. Default 10
wordpress: FormdataTemplate
FormdataTemplate
The theme file to use to display the object.
Import
import ballerinax/wordpress;
Metadata
Released date: about 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 0
Current verison: 7
Weekly downloads
Keywords
Website & App Building/Website Builders
Cost/Freemium
Contributors