weaviate
Module weaviate
API
Definitions
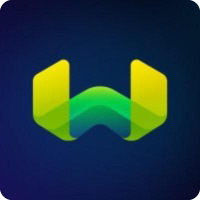
ballerinax/weaviate Ballerina library
Overview
This is a generated connector for the Weaviate Vector Search Engine API OpenAPI specification. Weaviate is an open-source vector search engine, which allows storing data objects and vector embeddings from the ML models, including the LLMs offered by OpenAI, Hugging Face, and Cohere. Weaviate provides a powerful GraphQL API for querying the embeddings while looking at the similarity and can scale seamlessly into billions of data objects.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Weaviate Cluster using the Weaviate Cloud Service or deploy using Docker/Kubernetes.
- Obtain the OIDC Authentication key.
Quick start
To use the Weaviate connector in your Ballerina application, update the .bal
file as follows:
Step 1: Import the connector
First, import the ballerinax/weaviate
module into the Ballerina project.
import ballerinax/weaviate;
Step 2: Create a new connector instance
Create and initialize a weaviate:Client
with your Service URL
and the obtained Authentication key
.
weaviate:Client weaviateClient = check new ({ auth: { token: "sk-XXXXXXXXX" } }, serviceURL: "https://weaviate-server:port/v1");
Step 3: Invoke the connector operation
-
Now, you can use the operations available within the connector. Following is an example of inserting new objects to the Weaviate vector storage as a batch operation.
string className = "DocClass"; // weaviate class name string[] text; // list of text float[][] embeddings; // list of embbedings for the texts int len = text.length(); // Creates the batch of Weaviate objects. weaviate:Object[] objArr = []; foreach int i in 0...len { objArr.push( { 'class: className, vector: embeddings[i], properties: { "docs": text[i] } }); } weaviate:ObjectsGetResponse[] responseArray = check weaviateClient->/batch/objects.post({ objects: objArr });
Once the new records are inserted, you can query the Weaviate vector storage using the Weaviate GraphQL API, similar to the example below.
float[] embeddings; // This is the embedding for the text being searched for similar content. string graphQLQuery = string`{ Get { DocStore ( nearVector: { vector: ${embeddings.toString()} } limit: 5 ){ docs _additional { certainty, id } } } }`; weaviate:GraphQLResponse|error results = check weaviateClient->/graphql.post({ query: graphQLQuery });
-
Use the
bal run
command to compile and run the Ballerina program.
Clients
weaviate: Client
This is a generated connector for Weaviate Vector Search Engine API OpenAPI specification. Weaviate API provide access to the manipulations of weaviate schema, objects and search vectors based on various criterias.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Cluster using Weaviate Cloud Service or deploy using Docker/Kubernetes and obtain the OIDC Authentication key.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "/v1" - URL of the target service
get objects
function get objects(string? after, int offset, int? 'limit, string? include, string? sort, string? 'order, string? 'class) returns ObjectsListResponse|error
Get a list of Objects.
Parameters
- after string? (default ()) - The starting ID of the result window.
- offset int (default 0) - The starting index of the result window. Default value is 0.
- 'limit int? (default ()) - The maximum number of items to be returned per page. Default value is set in Weaviate config.
- include string? (default ()) - Include additional information, such as classification infos. Allowed values include: classification, vector, interpretation
- sort string? (default ()) - Sort parameter to pass an information about the names of the sort fields
- 'order string? (default ()) - Order parameter to tell how to order (asc or desc) data within given field
- 'class string? (default ()) - Class parameter specifies the class from which to query objects
Return Type
- ObjectsListResponse|error - Successful response.
post objects
Create Objects between two Objects (object and subject).
Parameters
- payload Object -
- consistency_level string? (default ()) - Determines how many replicas must acknowledge a request before it is considered successful
get objects/[string className]/[string id]
function get objects/[string className]/[string id](string? include, string? consistency_level, string? node_name) returns Object|error
Get a specific Object based on its class and UUID. Also available as Websocket bus.
Parameters
- include string? (default ()) - Include additional information, such as classification infos. Allowed values include: classification, vector, interpretation
- consistency_level string? (default ()) - Determines how many replicas must acknowledge a request before it is considered successful
- node_name string? (default ()) - The target node which should fulfill the request
put objects/[string className]/[string id]
function put objects/[string className]/[string id](Object payload, string? consistency_level) returns Object|error
Update a class object based on its uuid
Parameters
- payload Object -
- consistency_level string? (default ()) - Determines how many replicas must acknowledge a request before it is considered successful
delete objects/[string className]/[string id]
function delete objects/[string className]/[string id](string? consistency_level) returns Response|error
Delete object based on its class and UUID.
Parameters
- consistency_level string? (default ()) - Determines how many replicas must acknowledge a request before it is considered successful
patch objects/[string className]/[string id]
function patch objects/[string className]/[string id](Object payload, string? consistency_level) returns Response|error
Update an Object based on its UUID (using patch semantics).
Parameters
- payload Object - RFC 7396-style patch, the body contains the object to merge into the existing object.
- consistency_level string? (default ()) - Determines how many replicas must acknowledge a request before it is considered successful
head objects/[string className]/[string id]
function head objects/[string className]/[string id](string? consistency_level) returns Response|error
Checks object's existence based on its class and uuid.
Parameters
- consistency_level string? (default ()) - Determines how many replicas must acknowledge a request before it is considered successful
post objects/validate
Validate an Object based on a schema.
Parameters
- payload Object -
post batch/objects
function post batch/objects(Batch_objects_body payload, string? consistency_level) returns ObjectsGetResponse[]|error
Creates new Objects based on a Object template as a batch.
Parameters
- payload Batch_objects_body -
- consistency_level string? (default ()) - Determines how many replicas must acknowledge a request before it is considered successful
Return Type
- ObjectsGetResponse[]|error - Request succeeded, see response body to get detailed information about each batched item.
post graphql
function post graphql(GraphQLQuery payload) returns GraphQLResponse|error
Get a response based on GraphQL
Parameters
- payload GraphQLQuery - The GraphQL query request parameters.
Return Type
- GraphQLResponse|error - Successful query (with select).
post graphql/batch
function post graphql/batch(GraphQLQueries payload) returns GraphQLResponses|error
Get a response based on GraphQL.
Parameters
- payload GraphQLQueries - The GraphQL queries.
Return Type
- GraphQLResponses|error - Successful query (with select).
get schema
Dump the current the database schema.
post schema
Create a new Object class in the schema.
Parameters
- payload Class -
get schema/[string className]
Get a single class from the schema
put schema/[string className]
Update settings of an existing schema class
Parameters
- payload Class -
delete schema/[string className]
Remove an Object class (and all data in the instances) from the schema.
Parameters
- force boolean? (default ()) -
post schema/[string className]/properties
Add a property to an Object class.
Parameters
- payload Property -
Records
weaviate: AdditionalProperties
Additional Meta information about a single object object.
Fields
- record {}... - Rest field
weaviate: Batch_objects_body
Fields
- fields string[]? - Define which fields need to be returned. Default value is ALL
- objects Object[]? -
weaviate: BatchDelete
Fields
- 'match BatchDelete_match? - Outlines how to find the objects to be deleted.
- output string? - Controls the verbosity of the output, possible values are: "minimal", "verbose". Defaults to "minimal".
- dryRun boolean? - If true, objects will not be deleted yet, but merely listed. Defaults to false.
weaviate: BatchDelete_match
Outlines how to find the objects to be deleted.
Fields
- 'class string? - Class (name) which objects will be deleted.
- 'where WhereFilter? - Filter search results using a where filter
weaviate: BatchDeleteResponse
Delete Objects response.
Fields
- 'match BatchDelete_match? - Outlines how to find the objects to be deleted.
- output string? - Controls the verbosity of the output, possible values are: "minimal", "verbose". Defaults to "minimal".
- dryRun boolean? - If true, objects will not be deleted yet, but merely listed. Defaults to false.
- results BatchDeleteResponse_results? - Results due to the deletion operation
weaviate: BatchDeleteResponse_results
Results due to the deletion operation
Fields
- matches int? - How many objects were matched by the filter.
- 'limit int? - The most amount of objects that can be deleted in a single query, equals QUERY_MAXIMUM_RESULTS.
- successful int? - How many objects were successfully deleted in this round.
- failed int? - How many objects should have been deleted but could not be deleted.
- objects BatchDeleteResponse_results_objects[]? - With output set to "minimal" only objects with error occurred will the be described. Successfully deleted objects would be omitted. Output set to "verbose" will list all of the objets with their respective statuses.
weaviate: BatchDeleteResponse_results_objects
Results for this specific Object.
Fields
- id string? - ID of the Object.
- status string? - Object processing status
- errors ErrorResponse? - An error response given by Weaviate end-points.
weaviate: BM25Config
tuning parameters for the BM25 algorithm
Fields
- k1 float? - calibrates term-weight scaling based on the term frequency within a document
- b float? - calibrates term-weight scaling based on the document length
weaviate: Class
Fields
- 'class string? - Name of the class as URI relative to the schema URL.
- vectorIndexType string? - Name of the vector index to use, eg. (HNSW)
- vectorIndexConfig record {}? - Vector-index config, that is specific to the type of index selected in vectorIndexType
- shardingConfig record {}? - Manage how the index should be sharded and distributed in the cluster
- replicationConfig ReplicationConfig? - Configure how replication is executed in a cluster
- invertedIndexConfig InvertedIndexConfig? - Configure the inverted index built into Weaviate
- vectorizer string? - Specify how the vectors for this class should be determined. The options are either 'none' - this means you have to import a vector with each object yourself - or the name of a module that provides vectorization capabilities, such as 'text2vec-contextionary'. If left empty, it will use the globally configured default which can itself either be 'none' or a specific module.
- moduleConfig record {}? - Configuration specific to modules this Weaviate instance has installed
- description string? - Description of the class.
- properties Property[]? - The properties of the class.
weaviate: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
weaviate: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
weaviate: Deprecation
Fields
- id string? - The id that uniquely identifies this particular deprecations (mostly used internally)
- status string? - Whether the problematic API functionality is deprecated (planned to be removed) or already removed
- apiType string? - Describes which API is effected, usually one of: REST, GraphQL
- msg string? - What this deprecation is about
- mitigation string? - User-required object to not be affected by the (planned) removal
- sinceVersion string? - The deprecation was introduced in this version
- plannedRemovalVersion string? - A best-effort guess of which upcoming version will remove the feature entirely
- removedIn string? - If the feature has already been removed, it was removed in this version
- removedTime string? - If the feature has already been removed, it was removed at this timestamp
- sinceTime string? - The deprecation was introduced in this version
- locations string[]? - The locations within the specified API affected by this deprecation
weaviate: ErrorResponse
An error response given by Weaviate end-points.
Fields
- 'error ErrorResponse_error[]? - List of errors
weaviate: ErrorResponse_error
Fields
- message string? -
weaviate: GeoCoordinates
GeoCoodinates based on longitute and latitude
Fields
- latitude float? - The latitude of the point on earth in decimal form
- longitude float? - The longitude of the point on earth in decimal form
weaviate: GraphQLError
An error response caused by a GraphQL query.
Fields
- locations GraphQLError_locations[]? - Location of the graphQL query
- message string? - error message give by the graphQL query
- path string[]? - paths in the schema
weaviate: GraphQLError_locations
Fields
- column int? -
- line int? -
weaviate: GraphQLQuery
GraphQL query based on: http://facebook.github.io/graphql/.
Fields
- operationName string? - The name of the operation if multiple exist in the query.
- query string? - Query based on GraphQL syntax.
- variables record {}? - Additional variables for the query.
weaviate: GraphQLResponse
GraphQL based response: http://facebook.github.io/graphql/.
Fields
- data record { JsonObject... }? - GraphQL data object.
- errors GraphQLError[]? - Array with errors.
weaviate: InvertedIndexConfig
Configure the inverted index built into Weaviate
Fields
- cleanupIntervalSeconds int? - Asynchronous index clean up happens every n seconds
- bm25 BM25Config? - tuning parameters for the BM25 algorithm
- stopwords StopwordConfig? - fine-grained control over stopword list usage
- indexTimestamps boolean? - Index each object by its internal timestamps
- indexNullState boolean? - Index each object with the null state
- indexPropertyLength boolean? - Index length of properties
weaviate: JsonObject
JSON object value.
weaviate: Object
Fields
- 'class string? - Class of the Object, defined in the schema.
- vectorWeights VectorWeights? - Allow custom overrides of vector weights as math expressions. E.g. "pancake": "7" will set the weight for the word pancake to 7 in the vectorization, whereas "w * 3" would triple the originally calculated word. This is an open object, with OpenAPI Specification 3.0 this will be more detailed. See Weaviate docs for more info. In the future this will become a key/value (string/string) object.
- properties PropertySchema? - This is an open object, with OpenAPI Specification 3.0 this will be more detailed. See Weaviate docs for more info. In the future this will become a key/value OR a SingleRef definition.
- id string? - ID of the Object.
- creationTimeUnix int? - Timestamp of creation of this Object in milliseconds since epoch UTC.
- lastUpdateTimeUnix int? - Timestamp of the last Object update in milliseconds since epoch UTC.
- vector C11yVector? - A Vector in the Contextionary
- additional AdditionalProperties? - Additional Meta information about a single object object.
weaviate: ObjectsGetResponse
Fields
- Fields Included from *Object
- class string
- vectorWeights VectorWeights|()
- properties PropertySchema
- id string
- creationTimeUnix int
- lastUpdateTimeUnix int
- vector C11yVector
- additional AdditionalProperties
- anydata...
- deprecations Deprecation[]? -
- result ObjectsGetResponse_result? - Results for this specific Object.
weaviate: ObjectsGetResponse_result
Results for this specific Object.
Fields
- status string? - Object processing status
- errors ErrorResponse? - An error response given by Weaviate end-points.
weaviate: ObjectsListResponse
List of Objects.
Fields
- objects Object[]? - The actual list of Objects.
- deprecations Deprecation[]? - deprecations
- totalResults int? - The total number of Objects for the query. The number of items in a response may be smaller due to paging.
weaviate: Property
Fields
- dataType string[]? - Can be a reference to another type when it starts with a capital (for example Person), otherwise "string" or "int".
- description string? - Description of the property.
- moduleConfig record {}? - Configuration specific to modules this Weaviate instance has installed
- name string? - Name of the property as URI relative to the schema URL.
- indexInverted boolean? - Optional. Should this property be indexed in the inverted index. Defaults to true. If you choose false, you will not be able to use this property in where filters. This property has no affect on vectorization decisions done by modules
- tokenization string? - Determines tokenization of the property as separate words or whole field. Optional. Applies to string, string[], text and text[] data types. Allowed values are
word
(default) andfield
for string and string[],word
(default) for text and text[]. Not supported for remaining data types
weaviate: PropertySchema
This is an open object, with OpenAPI Specification 3.0 this will be more detailed. See Weaviate docs for more info. In the future this will become a key/value OR a SingleRef definition.
weaviate: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
weaviate: ReferenceMetaClassification
This meta field contains additional info about the classified reference property
Fields
- overallCount int? - overall neighbors checked as part of the classification. In most cases this will equal k, but could be lower than k - for example if not enough data was present
- winningCount int? - size of the winning group, a number between 1..k
- losingCount int? - size of the losing group, can be 0 if the winning group size euqals k
- closestOverallDistance float? - The lowest distance of any neighbor, regardless of whether they were in the winning or losing group
- winningDistance float? - deprecated - do not use, to be removed in 0.23.0
- meanWinningDistance float? - Mean distance of all neighbors from the winning group
- closestWinningDistance float? - Closest distance of a neighbor from the winning group
- closestLosingDistance float? - The lowest distance of a neighbor in the losing group. Optional. If k equals the size of the winning group, there is no losing group
- losingDistance float? - deprecated - do not use, to be removed in 0.23.0
- meanLosingDistance float? - Mean distance of all neighbors from the losing group. Optional. If k equals the size of the winning group, there is no losing group.
weaviate: ReplicationConfig
Configure how replication is executed in a cluster
Fields
- factor int? - Number of times a class is replicated
weaviate: Schema
Definitions of semantic schemas (also see: https://github.com/weaviate/weaviate-semantic-schemas).
Fields
- classes Class[]? - Semantic classes that are available.
- maintainer string? - Email of the maintainer.
- name string? - Name of the schema.
weaviate: SingleRef
Either set beacon (direct reference) or set class and schema (concept reference)
Fields
- 'class string? - If using a concept reference (rather than a direct reference), specify the desired class name here
- schema PropertySchema? - This is an open object, with OpenAPI Specification 3.0 this will be more detailed. See Weaviate docs for more info. In the future this will become a key/value OR a SingleRef definition.
- beacon string? - If using a direct reference, specify the URI to point to the cross-ref here. Should be in the form of weaviate://localhost/<uuid> for the example of a local cross-ref to an object
- href string? - If using a direct reference, this read-only fields provides a link to the referenced resource. If 'origin' is globally configured, an absolute URI is shown - a relative URI otherwise.
- classification ReferenceMetaClassification? - This meta field contains additional info about the classified reference property
weaviate: StopwordConfig
fine-grained control over stopword list usage
Fields
- preset string? - pre-existing list of common words by language
- additions string[]? - stopwords to be considered additionally
- removals string[]? - stopwords to be removed from consideration
weaviate: VectorWeights
Allow custom overrides of vector weights as math expressions. E.g. "pancake": "7" will set the weight for the word pancake to 7 in the vectorization, whereas "w * 3" would triple the originally calculated word. This is an open object, with OpenAPI Specification 3.0 this will be more detailed. See Weaviate docs for more info. In the future this will become a key/value (string/string) object.
weaviate: WhereFilter
Filter search results using a where filter
Fields
- operands WhereFilter[]? - combine multiple where filters, requires 'And' or 'Or' operator
- operator string? - operator to use
- path string[]? - path to the property currently being filtered
- valueInt int? - value as integer
- valueNumber float? - value as number/float
- valueBoolean boolean? - value as boolean
- valueString string? - value as string
- valueText string? - value as text (on text props)
- valueDate string? - value as date (as string)
- valueGeoRange WhereFilterGeoRange? - filter within a distance of a georange
weaviate: WhereFilterGeoRange
filter within a distance of a georange
Fields
- geoCoordinates GeoCoordinates? - GeoCoodinates based on longitute and latitude
- distance WhereFilterGeoRange_distance? - distace based on geoCoordinates
weaviate: WhereFilterGeoRange_distance
distace based on geoCoordinates
Fields
- max float? - max distance
Import
import ballerinax/weaviate;
Metadata
Released date: about 2 years ago
Version: 1.0.2
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 22
Current verison: 13
Weekly downloads
Keywords
AI/Vector Databases
Cost/Freemium
Vendor/Weaviate
Embedding Search
Contributors
Dependencies