vonage.voice
Module vonage.voice
API
Definitions
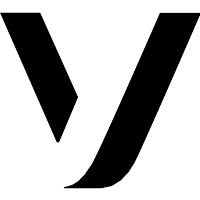
ballerinax/vonage.voice Ballerina library
Overview
This is a generated connector for Vonage Voice API v1.3.6 OpenAPI specification. The Voice API lets you create outbound calls, control in-progress calls and get information about historical calls. More information about the Voice API can be found at https://developer.nexmo.com/voice/voice-api/overview.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create Vonage account
- Obtain tokens
- Log into Vonage API Dashboard by visiting https://dashboard.nexmo.com/
- Go to https://dashboard.nexmo.com/getting-started/voice and follow the guidelines to create an application.
- Use the
Application ID
and thePrivate key
of the created application to generate aJWT token
. You can generate a JWT using the online tool. - Learn more about how Vonage APIs use JWTs.
Quickstart
To use the Vonage Voice connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/vonage.voice
module into the Ballerina project.
import ballerinax/vonage.voice as vv;
Step 2: Create a new connector instance
You can initialize the client as follows. You can now provide the JWT obtained above in the configuration.
Create a voice:ClientConfig
with the JWT token obtained, and initialize the connector with it.
vv:ClientConfig config = { auth: { token: "JWT" } } vv:Client baseClient = check new Client(config);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to create an outbound call using the connector. You can now make a call from your own machine. Vonage will call you and read out a text-to-speech message.
Create an outbound call
public function main() { vv:EndpointPhoneTo endpointPhoneTo = { 'type: "phone", number: "9477.....96" }; vv:EndpointPhoneFrom endpointPhoneFrom = { 'type: "phone", number: "9477.....96" }; vv:NCCO ncco = { action: "talk", text: "This is a text to speech call from Vonage" }; vv:CreateCallRequestNcco payload = { to: [endpointPhoneTo], 'from: endpointPhoneFrom, ncco: [ncco] }; vv:CreateCallResponse|error response = baseClient->createCall(payload); if (response is vv:CreateCallResponse) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
Following is an example on how to get details of your calls using the connector.
Get details of your calls
public function main() { vv:GetCallsResponse|error response = baseClient->getCalls(); if (response is vv:CreateCallResponse) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
vonage.voice: Client
This is a generated connector for Vonage Voice API v1.3.6 OpenAPI specification. The Voice API lets you create outbound calls, control in-progress calls and get information about historical calls. More information about the Voice API can be found at https://developer.nexmo.com/voice/voice-api/overview.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Vonage account and obtain tokens following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.nexmo.com/v1/calls" - URL of the target service
getCalls
function getCalls(string? status, string? dateStart, string? dateEnd, int pageSize, int recordIndex, string 'order, string? conversationUuid) returns GetCallsResponse|error
Get details of your calls
Parameters
- status string? (default ()) - Filter by call status
- dateStart string? (default ()) - Return the records that occurred after this point in time
- dateEnd string? (default ()) - Return the records that occurred before this point in time
- pageSize int (default 10) - Return this amount of records in the response
- recordIndex int (default 0) - Return calls from this index in the response
- 'order string (default "asc") - Either ascending or descending order.
- conversationUuid string? (default ()) - Return all the records associated with a specific conversation.
Return Type
- GetCallsResponse|error - OK
createCall
function createCall(Body payload) returns CreateCallResponse|error
Create an outbound call
Parameters
- payload Body - Call Details
Return Type
- CreateCallResponse|error - Created
getCall
function getCall(string uuid) returns GetCallResponse|error
Get detail of a specific call
Parameters
- uuid string - UUID of the Call
Return Type
- GetCallResponse|error - Ok
updateCall
Modify an in progress call
startStream
function startStream(string uuid, StartStreamRequest payload) returns StartStreamResponse|error
Play an audio file into a call
Return Type
- StartStreamResponse|error - Ok
stopStream
function stopStream(string uuid) returns StopStreamResponse|error
Stop playing an audio file into a call
Parameters
- uuid string - UUID of the Call Leg
Return Type
- StopStreamResponse|error - Ok
startTalk
function startTalk(string uuid, StartTalkRequest payload) returns StartTalkResponse|error
Play text to speech into a call
Return Type
- StartTalkResponse|error - Ok
stopTalk
function stopTalk(string uuid) returns StopTalkResponse|error
Stop text to speech in a call
Parameters
- uuid string - UUID of the Call Leg
Return Type
- StopTalkResponse|error - Ok
startDTMF
function startDTMF(string uuid, DTMFRequest payload) returns DTMFResponse|error
Play DTMF tones into a call
Return Type
- DTMFResponse|error - Ok
Records
vonage.voice: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
vonage.voice: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
vonage.voice: CreateCallRequestAnswerUrl
Fields
- answer_url string[]? - The webhook endpoint where you provide the Nexmo Call Control Object that governs this call.
- answer_method string? - The HTTP method used to send event information to answer_url.
- Fields Included from *CreateCallRequestBase
- to EndpointPhoneTo|EndpointSip|EndpointWebsocket|EndpointVBCExtension[]
- from EndpointPhoneFrom
- event_url string[]
- event_method string
- machine_detection string
- length_timer int
- ringing_timer int
- anydata...
vonage.voice: CreateCallRequestBase
Fields
- 'from EndpointPhoneFrom - Connect to a Phone (PSTN) number
- event_url string[]? - Required unless
event_url
is configured at the application level, see Create an Application The webhook endpoint where call progress events are sent to. For more information about the values sent, see Event webhook.
- event_method string(default "POST") - The HTTP method used to send event information to
event_url
.
- machine_detection string? - Configure the behavior when Vonage detects that the call is answered by voicemail. If
continue
, Vonage sends an HTTP request toevent_url
with the Call event machine. Ifhangup
, Vonage ends the call.
- length_timer int(default 7200) - Set the number of seconds that elapse before Vonage hangs up after the call state changes to answered.
- ringing_timer int(default 60) - Set the number of seconds that elapse before Vonage hangs up after the call state changes to ‘ringing’.
vonage.voice: CreateCallRequestNcco
Fields
- ncco record {}[]? - The Nexmo Call Control Object to use for this call.
- Fields Included from *CreateCallRequestBase
- to EndpointPhoneTo|EndpointSip|EndpointWebsocket|EndpointVBCExtension[]
- from EndpointPhoneFrom
- event_url string[]
- event_method string
- machine_detection string
- length_timer int
- ringing_timer int
- anydata...
vonage.voice: CreateCallResponse
Fields
- uuid Uuid? - The unique identifier for this call leg. The UUID is created when your call request is accepted by Vonage. You use the UUID in all requests for individual live calls
- status Status? - The status of the call. See possible values
- direction Direction? - Possible values are
outbound
orinbound
- conversation_uuid ConversationUuid? - The unique identifier for the conversation this call leg is part of.
vonage.voice: DTMFRequest
Fields
- digits string? - The digits to send
vonage.voice: DTMFResponse
Fields
- message string? - Description of the action taken
- uuid Uuid? - The unique identifier for this call leg. The UUID is created when your call request is accepted by Vonage. You use the UUID in all requests for individual live calls
vonage.voice: EndpointApp
Connect to an App User
Fields
- 'type string - The type of connection. Must be
app
- user string - The username to connect to
vonage.voice: EndpointPhoneFrom
Connect to a Phone (PSTN) number
Fields
- 'type string - The type of connection. Must be
phone
- number AddressE164 - The phone number to connect to
vonage.voice: EndpointPhoneTo
Connect to a Phone (PSTN) number
Fields
- 'type string - The type of connection. Must be
phone
- number AddressE164 - The phone number to connect to
- dtmfAnswer string? - Provide DTMF digits to send when the call is answered
vonage.voice: EndpointSip
Connect to a SIP Endpoint
Fields
- 'type string - The type of connection. Must be
sip
- uri AddressSip? - The SIP URI to connect to
vonage.voice: EndpointVBCExtension
Connect to a VBC extension
Fields
- 'type string - The type of connection. Must be
vbc
- extension string - Extension
vonage.voice: EndpointWebsocket
Connect to a Websocket
Fields
- 'type string - The type of connection. Must be
websocket
- uri AddressWebsocket? - Websocket URI
- contentType string? - Content Type
- headers EndpointwebsocketHeaders? - Details of the Websocket you want to connect to
vonage.voice: EndpointwebsocketHeaders
Details of the Websocket you want to connect to
Fields
- customer_id string? - This is an example header. You can provide any headers you may need
vonage.voice: From
The endpoint you called from. Possible values are the same as to
.
Fields
- 'type string? - The type of Endpoint that made the call
- number string? - The number that made the call
vonage.voice: GetCallResponse
Fields
- _links GetcallresponseLinks? -
- uuid Uuid? - The unique identifier for this call leg. The UUID is created when your call request is accepted by Vonage. You use the UUID in all requests for individual live calls
- conversation_uuid ConversationUuid? - The unique identifier for the conversation this call leg is part of.
- to To? - The single or mixed collection of endpoint types you connected to
- 'from From? - The endpoint you called from. Possible values are the same as
to
.
- status Status? - The status of the call. See possible values
- direction Direction? - Possible values are
outbound
orinbound
- rate Rate? - The price per minute for this call. This is only sent if
status
iscompleted
.
- price Price? - The total price charged for this call. This is only sent if
status
iscompleted
.
- duration Duration? - The time elapsed for the call to take place in seconds. This is only sent if
status
iscompleted
.
- start_time StartTime? - The time the call started in the following format:
YYYY-MM-DD HH:MM:SS
. For example,2020-01-01 12:00:00
. This is only sent ifstatus
iscompleted
.
- end_time EndTime? - The time the call started in the following format:
YYYY-MM-DD HH:MM:SS
. For xample,2020-01-01 12:00:00
. This is only sent ifstatus
iscompleted
.
vonage.voice: GetcallresponseLinks
Fields
- self GetcallresponseLinksSelf? -
vonage.voice: GetcallresponseLinksSelf
Fields
- href string? -
vonage.voice: GetCallsResponse
Fields
- count int? -
- page_size int? -
- record_index int? -
- _links GetcallsresponseLinks? -
- _embedded GetcallsresponseEmbedded? - A list of call objects. See the get details of a specific call response fields for a description of the nested objects
vonage.voice: GetcallsresponseEmbedded
A list of call objects. See the get details of a specific call response fields for a description of the nested objects
Fields
- calls GetCallResponse[]? - Array of Get Call Response
vonage.voice: GetcallsresponseLinks
Fields
- self GetcallsresponseLinksSelf? -
vonage.voice: GetcallsresponseLinksSelf
Fields
- href string? -
vonage.voice: NCCO
Fields
- action string? - Action
- text string? - Text
vonage.voice: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
vonage.voice: StartStreamRequest
Fields
- stream_url string[] -
- loop int(default 1) - the number of times to play the file, 0 for infinite
- level string(default "0") - Set the audio level of the stream in the range
-1 >= level <= 1
with a precision of 0.1. The default value is 0.
vonage.voice: StartStreamResponse
Fields
- message string? - Description of the action taken
- uuid Uuid? - The unique identifier for this call leg. The UUID is created when your call request is accepted by Vonage. You use the UUID in all requests for individual live calls
vonage.voice: StartTalkRequest
Fields
- text string - The text to read
- language Language? - The language to use
- style Style? - The vocal style (vocal range, tessitura, and timbre) to use
- voice_name VoiceName? - <strong>DEPRECATED</strong> The voice & language to use
- loop int(default 1) - The number of times to repeat the text the file, 0 for infinite
- level string(default "0") - The volume level that the speech is played. This can be any value between
-1
to1
in0.1
increments, with0
being the default.
vonage.voice: StartTalkResponse
Fields
- message string? - Description of the action taken
- uuid Uuid? - The unique identifier for this call leg. The UUID is created when your call request is accepted by Vonage. You use the UUID in all requests for individual live calls
vonage.voice: StopStreamResponse
Fields
- message string? - Description of the action taken
- uuid Uuid? - The unique identifier for this call leg. The UUID is created when your call request is accepted by Vonage. You use the UUID in all requests for individual live calls
vonage.voice: StopTalkResponse
Fields
- message string? - Description of the action taken
- uuid Uuid? - The unique identifier for this call leg. The UUID is created when your call request is accepted by Vonage. You use the UUID in all requests for individual live calls
vonage.voice: To
The single or mixed collection of endpoint types you connected to
Fields
- 'type string? - The Type of Endpoint Called
- number string? - The number of the endpoint called
vonage.voice: UpdateCallRequestEarmuff
Fields
- action string? - Prevent the specified UUID from hearing audio
vonage.voice: UpdateCallRequestHangup
Fields
- action string? - End the call for the specified UUID
vonage.voice: UpdateCallRequestMute
Fields
- action string? - Mute the specified UUID
vonage.voice: UpdateCallRequestTransferAnswerUrl
Fields
- action RequestTransferActionParam - Transfer the call to a new NCCO
- destination UpdatecallrequesttransferanswerurlDestination -
vonage.voice: UpdatecallrequesttransferanswerurlDestination
Fields
- 'type string - Always
ncco
- url string[] - The URL that Vonage makes a request to. Must return an NCCO.
vonage.voice: UpdateCallRequestTransferNcco
Fields
- action RequestTransferActionParam - Transfer the call to a new NCCO
- destination UpdatecallrequesttransfernccoDestination -
vonage.voice: UpdatecallrequesttransfernccoDestination
Fields
- 'type string - Always
ncco
- ncco record {}[] - Refer to the NCCO Guide for a description of possible NCCO parameters.
vonage.voice: UpdateCallRequestUnearmuff
Fields
- action string? - Allow the specified UUID to hear audio
vonage.voice: UpdateCallRequestUnmute
Fields
- action string? - Unmute the specified UUID
Union types
vonage.voice: Body
Body
String types
vonage.voice: AddressE164
AddressE164
The phone number to connect to
vonage.voice: AddressSip
AddressSip
The SIP URI to connect to
vonage.voice: AddressWebsocket
AddressWebsocket
Websocket URI
vonage.voice: ConversationUuid
ConversationUuid
The unique identifier for the conversation this call leg is part of.
vonage.voice: Direction
Direction
Possible values are outbound
or inbound
vonage.voice: Duration
Duration
The time elapsed for the call to take place in seconds. This is only sent if status
is completed
.
vonage.voice: EndTime
EndTime
The time the call started in the following format: YYYY-MM-DD HH:MM:SS
. For xample, 2020-01-01 12:00:00
. This is only sent if status
is completed
.
vonage.voice: Language
Language
The language to use
vonage.voice: Network
Network
The Mobile Country Code Mobile Network Code (MCCMNC) for the carrier network used to make this call.
vonage.voice: Price
Price
The total price charged for this call. This is only sent if status
is completed
.
vonage.voice: Rate
Rate
The price per minute for this call. This is only sent if status
is completed
.
vonage.voice: RequestTransferActionParam
RequestTransferActionParam
Transfer the call to a new NCCO
vonage.voice: StartTime
StartTime
The time the call started in the following format: YYYY-MM-DD HH:MM:SS
. For example, 2020-01-01 12:00:00
. This is only sent if status
is completed
.
vonage.voice: Status
Status
The status of the call. See possible values
vonage.voice: Uuid
Uuid
The unique identifier for this call leg. The UUID is created when your call request is accepted by Vonage. You use the UUID in all requests for individual live calls
vonage.voice: VoiceName
DeprecatedVoiceName
DEPRECATED The voice & language to use
Deprecated
Integer types
vonage.voice: Style
Style
The vocal style (vocal range, tessitura, and timbre) to use
Import
import ballerinax/vonage.voice;
Metadata
Released date: about 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 5
Current verison: 5
Weekly downloads
Keywords
Communication/Call & SMS
Cost/Paid
Contributors