vonage.verify
Module vonage.verify
API
Definitions
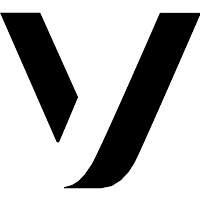
ballerinax/vonage.verify Ballerina library
Overview
This is a generated connector from Vonage Verify API v1.1.7 OpenAPI specification. The Verify API helps you to implement 2FA (two-factor authentication) in your applications. More information is available at https://developer.nexmo.com/verify/overview
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create Vonage account
- Obtain tokens
- Log into Vonage API Dashboard by visiting https://dashboard.nexmo.com/
- Obtain the
API Key
andAPI Secret
from the API Dashboard.
Quickstart
To use the Vonage Number Insight connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/vonage.verify
module into the Ballerina project.
import ballerinax/vonage.verify as vv;
Step 2: Create a new connector instance
Initialize the connector.
vv:Client baseClient = check new Client();
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to make a verification request using the connector. You can now provide the API key and API secret obtained from the Vonage API Dashboard. You can now generate and send a PIN to your user.
- Create a request to send a verification code to your user.
- Check the
status
field in the response to ensure that your request was successful (zero is success). - Use the
request_id
field in the response for the Verify check.
Request a Verification
public function main() { vv:VerifyRequest verifyRequest = { api_key: "7b.....4", api_secret: "Gd........4", number: "94......6", brand: "WSO2" }; vv:InlineResponse200|error response = baseClient->verifyRequest(verifyRequest); if (response is vv:InlineResponse200) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
Following is an example on how to verify check using the connector. You can now provide the API key and API secret obtained from the Vonage API Dashboard. Use verify check to confirm that the PIN you received from your user matches the one sent by Vonage in your verify request.
Verify Check
public function main() { vv:CheckRequest checkRequest = { api_key: "7b1.....4", api_secret: "Gd........4", request_id: "36...............443", code: "5..0" }; vv:InlineResponse200|error response = baseClient->verifyCheck(checkRequest); if (response is vv:InlineResponse200) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
vonage.verify: Client
This is a generated connector for Vonage Verify API v1.1.7 OpenAPI specification. The Verify API helps you to implement 2FA (two-factor authentication) in your applications. More information is available at https://developer.nexmo.com/verify/overview
Constructor
Gets invoked to initialize the connector
.
The connector initialization doesn't require setting the API credentials.
Create a Vonage account and obtain tokens by following this guide.
Some operations may require passing the token as a parameter.
init (ClientConfiguration clientConfig, string serviceUrl)
- clientConfig ClientConfiguration {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.nexmo.com/verify" - URL of the target service
verifyRequest
function verifyRequest(VerifyRequest payload) returns InlineResponse200|error
Request a Verification
Parameters
- payload VerifyRequest - Verify Request
Return Type
- InlineResponse200|error - OK
verifyCheck
function verifyCheck(CheckRequest payload) returns InlineResponse2001|error
Verify Check
Parameters
- payload CheckRequest - Check Request
Return Type
- InlineResponse2001|error - OK
verifySearch
function verifySearch(string apiKey, string apiSecret, string requestId, string[]? requestIds) returns InlineResponse2002|error
Verify Search
Parameters
- apiKey string - API Key
- apiSecret string - API Secret
- requestId string - The
request_id
you received in the Verify Request Response. Required ifrequest_ids
not provided.
- requestIds string[]? (default ()) - More than one
request_id
. Eachrequest_id
is a new parameter in the Verify Search request. Required ifrequest_id
not provided.
Return Type
- InlineResponse2002|error - OK
verifyControl
function verifyControl(ControlRequest payload) returns InlineResponse2003|error
Verify Control
Parameters
- payload ControlRequest - Control Request
Return Type
- InlineResponse2003|error - OK
verifyRequestWithPSD2
function verifyRequestWithPSD2(Psd2Request payload) returns InlineResponse200|error
PSD2 (Payment Services Directive 2) Request
Parameters
- payload Psd2Request - PSD2 (Payment Services Directive 2) Request
Return Type
- InlineResponse200|error - OK
Records
vonage.verify: CheckErrorResponse
Error
Fields
- request_id string? - The
request_id
that you received in the response to the Verify request and used in the Verify check request.
- status string? - Code | Text | Description
-- | -- | --
0 | Success | The request was successfully accepted by Vonage.
1 | Throttled | You are trying to send more than the maximum of 30 requests per second.
2 | Your request is incomplete and missing the mandatory parameter
$parameter
| The stated parameter is missing. 3 | Invalid value for parameter$parameter
| Invalid value for parameter. If you see Facility not allowed in the error text, check that you are using the correct Base URL in your request. 4 | Invalid credentials were provided | The supplied API key or secret in the request is either invalid or disabled. 5 | Internal Error | An error occurred processing this request in the Cloud Communications Platform. 6 | The Vonage platform was unable to process this message for the following reason:$reason
| The request could not be routed. 16 | The code inserted does not match the expected value | 17 | The wrong code was provided too many times | You can run Verify check on a specificrequest_id
up to three times unless a new verification code is generated. If you check a request more than three times, it is set to FAILED and you cannot check it again.
- error_text string? - If the
status
is non-zero, this explains the error encountered.
vonage.verify: CheckRequest
Fields
- api_key ApiKey - You can find your API key in your account dashboard
- api_secret ApiSecret - You can find your API secret in your account dashboard
- request_id string - The Verify request to check. This is the
request_id
you received in the response to the Verify request.
- code string - The verification code entered by your user.
- ip_address string? - (This field is no longer used)
vonage.verify: CheckResponse
Success
Fields
- request_id string? - The
request_id
that you received in the response to the Verify request and used in the Verify check request.
- event_id string? - The ID of the verification event, such as an SMS or TTS call.
- status string? - A value of
0
indicates that your user entered the correct code. If it is non-zero, check theerror_text
.
- price string? - The cost incurred for this request.
- currency string? - The currency code.
- estimated_price_messages_sent EstimatedPriceMessagesSent? - This field may not be present, depending on your pricing model.
vonage.verify: ControlErrorResponse
Error
Fields
- status string? - Code | Text | Description
-- | -- | --
0 | Success | The request was successfully accepted by Vonage.
1 | Throttled | You are trying to send more than the maximum of 30 requests per second.
2 | Your request is incomplete and missing the mandatory parameter
$parameter
| The stated parameter is missing. 3 | Invalid value for parameter$parameter
| Invalid value for parameter. If you see Facility not allowed in the error text, check that you are using the correct Base URL in your request. 4 | Invalid credentials were provided | The supplied API key or secret in the request is either invalid or disabled. 5 | Internal Error | An error occurred processing this request in the Cloud Communications Platform. 6 | The Vonage platform was unable to process this message for the following reason:$reason
| The request could not be routed. 8 | The api_key you supplied is for an account that has been barred from submitting messages. | 9 | Partner quota exceeded | Your account does not have sufficient credit to process this request. 19 | Forcancel
: Either you have not waited at least 30 secs after sending a Verify request before cancelling or Verify has made too many attempts to deliver the verification code for this request and you must now wait for the process to complete. Fortrigger_next_event
: All attempts to deliver the verification code for this request have completed and there are no remaining events to advance to.
- error_text string? - If the
status
is non-zero, this explains the error encountered.
vonage.verify: ControlRequest
Fields
- api_key ApiKey - You can find your API key in your account dashboard
- api_secret ApiSecret - You can find your API secret in your account dashboard
- request_id string - The
request_id
you received in the response to the Verify request.
- cmd string - The possible commands are
cancel
to request cancellation of the verification process, ortrigger_next_event
to advance to the next verification event (if any). Cancellation is only possible 30 seconds after the start of the verification request and before the second event (either TTS or SMS) has taken place.
vonage.verify: ControlResponse
Success
Fields
- status string? -
cmd
| Code | Description -- | -- | -- Any | 0 | Success
- command string? - The
cmd
you sent in the request.
vonage.verify: Psd2Request
Fields
- api_key ApiKey - You can find your API key in your account dashboard
- api_secret ApiSecret - You can find your API secret in your account dashboard
- country string? - If you do not provide
number
in international format or you are not sure ifnumber
is correctly formatted, specify the two-character country code incountry
. Verify will then format the number for you.
- payee string - An alphanumeric string to indicate to the user the name of the recipient that they are confirming a payment to.
- amount float - The decimal amount of the payment to be confirmed, in Euros
- code_length int? - The length of the verification code.
- lg string? - By default, the SMS or text-to-speech (TTS) message is generated in the locale that matches the
number
. For example, the text message or TTS message for a33*
number is sent in French. Use this parameter to explicitly control the language used. *Note: Voice calls in English forbg-bg
,ee-et
,ga-ie
,lv-lv
,lt-lt
,mt-mt
,sk-sk
,sk-si
- pin_expiry int? - How long the generated verification code is valid for, in seconds. When you specify both
pin_expiry
andnext_event_wait
thenpin_expiry
must be an integer multiple ofnext_event_wait
otherwisepin_expiry
is defaulted to equal next_event_wait. See changing the event timings.
- next_event_wait int? - Specifies the wait time in seconds between attempts to deliver the verification code.
- workflow_id int? - Selects the predefined sequence of SMS and TTS (Text To Speech) actions to use in order to convey the PIN to your user. For example, an id of 1 identifies the workflow SMS - TTS - TTS. For a list of all workflows and their associated ids, please visit the developer portal.
vonage.verify: RequestErrorResponse
Error
Fields
- request_id string? - The unique ID of the Verify request. This may be blank in an error situation
- status string? - Code | Text | Description
-- | -- | --
0 | Success | The request was successfully accepted by Vonage.
1 | Throttled | You are trying to send more than the maximum of 30 requests per second.
2 | Your request is incomplete and missing the mandatory parameter
$parameter
| The stated parameter is missing. 3 | Invalid value for parameter$parameter
| Invalid value for parameter. If you see Facility not allowed in the error text, check that you are using the correct Base URL in your request. 4 | Invalid credentials were provided | The supplied API key or secret in the request is either invalid or disabled. 5 | Internal Error | An error occurred processing this request in the Cloud Communications Platform. 6 | The Vonage platform was unable to process this message for the following reason:$reason
| The request could not be routed. 7 | The number you are trying to verify is blacklisted for verification. | 8 | The api_key you supplied is for an account that has been barred from submitting messages. | 9 | Partner quota exceeded | Your account does not have sufficient credit to process this request. 10 | Concurrent verifications to the same number are not allowed | 15 | The destination number is not in a supported network | The request has been rejected. Find out more about this error in the Knowledge Base 20 | This account does not support the parameter: pin_code. | Only certain accounts have the ability to set thepin_code
. Please contact your account manager for more information. 29 | Non-Permitted Destination | Your Vonage account is still in demo mode. While in demo mode you must add target numbers to the approved list for your account. Add funds to your account to remove this limitation.
- error_text string? - If
status
is non-zero, this explains the error encountered.
vonage.verify: RequestResponse
Success
Fields
- request_id string? - The unique ID of the Verify request. You need this
request_id
for the Verify check.
- status string? - Indicates the outcome of the request; zero is success
vonage.verify: SearchErrorResponse
Error
Fields
- request_id string? - The
request_id
that you received in the response to the Verify request and used in the Verify search request. May be empty in an error situation.
- status string? - Code | Description
-- | --
IN PROGRESS | The search is still in progress.
SUCCESS | Your user entered a correct verification code.
FAILED | Your user entered an incorrect code more than three times.
EXPIRED | Your user did not enter a code before the
pin_expiry
time elapsed. CANCELLED | The verification process was cancelled by a Verify control request. 101 | You supplied an invalidrequest_id
, or the data is not available. Note that for recently-completed requests, there can be a delay of up to 1 minute before the results are available in search.
- error_text string? - If
status
is notSUCCESS
, this message explains the issue encountered.
vonage.verify: SearchResponse
Success
Fields
- request_id string? - The
request_id
that you received in the response to the Verify request and used in the Verify search request.
- account_id string? - The Vonage account ID the request was for.
- status string? - Code | Description
-- | --
IN PROGRESS | The search is still in progress.
SUCCESS | Your user entered a correct verification code.
FAILED | Your user entered an incorrect code more than three times.
EXPIRED | Your user did not enter a code before the
pin_expiry
time elapsed. CANCELLED | The verification process was cancelled by a Verify control request.
- number string? - The phone number this verification request was used for.
- price string? - The cost incurred for this verification request.
- currency string? - The currency code.
- sender_id string? - The
sender_id
you provided in the Verify request.
- date_submitted string? - The date and time the verification request was submitted, in the following format YYYY-MM-DD HH:MM:SS.
- date_finalized string? - The date and time the verification request was completed. This response parameter is in the following format YYYY-MM-DD HH:MM:SS.
- first_event_date string? - The time the first verification attempt was made, in the following format YYYY-MM-DD HH:MM:SS.
- last_event_date string? - The time the last verification attempt was made, in the following format YYYY-MM-DD HH:MM:SS.
- checks SearchresponseChecks[]? - The list of checks made for this verification and their outcomes.
- events SearchresponseEvents[]? - The events that have taken place to verify this number, and their unique identifiers.
- estimated_price_messages_sent EstimatedPriceMessagesSent? - This field may not be present, depending on your pricing model.
vonage.verify: SearchresponseChecks
Fields
- date_received string? - The date and time this check was received (in the format YYYY-MM-DD HH:MM:SS)
- code string? - The code supplied with this check request
- status string? -
- ip_address string? - The IP address, if available (this field is no longer used).
vonage.verify: SearchresponseEvents
Fields
- 'type string? -
- id string? -
vonage.verify: VerifyRequest
Fields
- api_key ApiKey - You can find your API key in your account dashboard
- api_secret ApiSecret - You can find your API secret in your account dashboard
- country string? - If you do not provide
number
in international format or you are not sure ifnumber
is correctly formatted, specify the two-character country code incountry
. Verify will then format the number for you.
- brand string - An 18-character alphanumeric string you can use to personalize the verification request SMS body, to help users identify your company or application name. For example: "Your
Acme Inc
PIN is ..."
- sender_id string? - An 11-character alphanumeric string that represents the identity of the sender of the verification request. Depending on the destination of the phone number you are sending the verification SMS to, restrictions might apply.
- code_length int? - The length of the verification code.
- lg string? - By default, the SMS or text-to-speech (TTS) message is generated in the locale that matches the
number
. For example, the text message or TTS message for a33*
number is sent in French. Use this parameter to explicitly control the language used for the Verify request. A list of languages is available: https://developer.nexmo.com/verify/guides/verify-languages
- pin_expiry int? - How long the generated verification code is valid for, in seconds. When you specify both
pin_expiry
andnext_event_wait
thenpin_expiry
must be an integer multiple ofnext_event_wait
otherwisepin_expiry
is defaulted to equal next_event_wait. See changing the event timings.
- next_event_wait int? - Specifies the wait time in seconds between attempts to deliver the verification code.
- workflow_id int? - Selects the predefined sequence of SMS and TTS (Text To Speech) actions to use in order to convey the PIN to your user. For example, an id of 1 identifies the workflow SMS - TTS - TTS. For a list of all workflows and their associated ids, please visit the developer portal.
Union types
vonage.verify: InlineResponse200
InlineResponse200
vonage.verify: InlineResponse2001
InlineResponse2001
vonage.verify: InlineResponse2002
InlineResponse2002
vonage.verify: InlineResponse2003
InlineResponse2003
String types
vonage.verify: ApiKey
ApiKey
You can find your API key in your account dashboard
vonage.verify: ApiSecret
ApiSecret
You can find your API secret in your account dashboard
vonage.verify: EstimatedPriceMessagesSent
EstimatedPriceMessagesSent
This field may not be present, depending on your pricing model.
Import
import ballerinax/vonage.verify;
Metadata
Released date: over 2 years ago
Version: 1.2.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.0.0
GraalVM compatible: Yes
Pull count
Total: 0
Current verison: 0
Weekly downloads
Keywords
Communication/Call & SMS
Cost/Paid
Contributors
Dependencies