Module twitter
API
Definitions
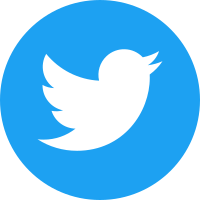
ballerinax/twitter Ballerina library
Overview
Twitter(X) is a widely-used social networking service provided by X Corp., enabling users to post and interact with messages known as "tweets".
The ballerinax/twitter
package offers APIs to connect and interact with Twitter(X) API endpoints, specifically based on Twitter(X) API v2.
Setup guide
To use the Twitter connector, you must have access to the Twitter API through a Twitter developer account and a project under it. If you do not have a Twitter Developer account, you can sign up for one here.
Step 1: Create a Twitter Developer Project
-
Open the Twitter Developer Portal.
-
Click on the "Projects & Apps" tab and select an existing project or create a new one for which you want API Keys and Authentication Tokens.
Step 2: Set up user authentication settings
-
Scroll down and Click on the Set up button to set up user authentication.
-
Complete the user authentication setup.
Step 3. Obtain Client Id and Client Secret.
-
After completing the setup, you will be provided with your client Id and client secret. Make sure to save the provided client Id and client secret.
Step 4: Setup OAuth 2.0 Flow
Before proceeding with the Quickstart, ensure you have obtained the Access Token using the following steps:
-
Create an authorization URL using the following format:
https://twitter.com/i/oauth2/authorize?response_type=code&client_id=<your_client_id>&redirect_uri=<your_redirect_uri>&scope=tweet.read%20tweet.write%20users.read%20follows.read&state=state&code_challenge=<your_code_challenge>&code_challenge_method=plain
Replace
<your_client_id>
,<your_redirect_uri>
, and<your_code_challenge>
with your specific values. Make sure to include the necessary scopes depending on your use case.Note: The "code verifier" is a randomly generated string used to verify the authorization code, and the "code challenge" is derived from the code verifier. These methods enhance security during the authorization process. In OAuth 2.0 PKCE, there are two methods for creating a "code challenge":
-
S256: The code challenge is a base64 URL-encoded SHA256 hash of a randomly generated string called the "code verifier".
-
plain: The code challenge is the plain code verifier string itself.
Example authorization URL:
https://twitter.com/i/oauth2/authorize?response_type=code&client_id=asdasASDas21Y0OGR4bnUxSzA4c0k6MTpjaQ&redirect_uri=http://example&scope=tweet.read%20tweet.write%20users.read%20follows.read&state=state&code_challenge=D601XXCSK57UineGq62gUnsoasdas1GfKUY8QWhOF9hiN_k&code_challenge_method=plain
Note: By default, the access token you create through the OAuth 2.0 Flow, as used here, will only remain valid for two hours. There is an alternative way that does not invalidate the access token after 2 hours. To do this, refer to Obtain access token under offline.access.
-
-
Copy and paste the generated URL into your browser. This will redirect you to the Twitter authorization page.
-
Once you authorize, you will be redirected to your specified redirect URI with an authorization code in the URL.
Example:
http://www.example.com/?state=state&code=QjAtYldxeTZITnd5N0FVN1B3MlliU29rb1hrdmFPUWNXSG5LX1hCRExaeFE3OjE3MTkzODMzNjkxNjQ6MTowOmFjOjE
Note: Store the authorization code and use it promptly as it expires quickly.
-
Use the obtained authorization code to run the following curl command, replacing
<your_client_id>
,<your_redirect_url>
,<your_code_verifier>
, and<your_authorization_code>
with your specific values:- Linux/MacOS:
curl --location "https://api.twitter.com/2/oauth2/token" \ --header "Content-Type: application/x-www-form-urlencoded" \ --data-urlencode "code=<your_authorization_code>" \ --data-urlencode "grant_type=authorization_code" \ --data-urlencode "client_id=<your_client_id>" \ --data-urlencode "redirect_uri=<your_redirect_url>" \ --data-urlencode "code_verifier=<your_code_verifier>"
- Windows:
curl --location "https://api.twitter.com/2/oauth2/token" ^ --header "Content-Type: application/x-www-form-urlencoded" ^ --data-urlencode "code=<your_authorization_code>" ^ --data-urlencode "grant_type=authorization_code" ^ --data-urlencode "client_id=<your_client_id>" ^ --data-urlencode "redirect_uri=<your_redirect_url>" ^ --data-urlencode "code_verifier=<your_code_verifier>"
This command will return the access token necessary for API calls.
{ "token_type":"bearer", "expires_in":7200, "access_token":"VWdEaEQ2eEdGdmVSbUJQV1U5LUdWREZuYndVT1JaNDddsdsfdsfdsxcvIZGMzblNjRGtvb3dGOjE3MTkzNzYwOTQ1MDQ6MTowOmF0Oj", "scope":"tweet.write users.read follows.read tweet.read" }
-
Store the access token securely for use in your application.
Note: We recommend using the OAuth 2.0 Authorization Code with PKCE method as used here, but there is another way using OAuth 2.0 App Only OAuth 2.0 App Only. Refer to this document to check which operations in Twitter API v2 are done using which method: API reference.
Quickstart
To use the Twitter
connector in your Ballerina application, update the .bal
file as follows:
Step 1: Import the module
Import the twitter
module.
import ballerinax/twitter;
Step 2: Instantiate a new connector
- Create a
Config.toml
file and, configure the obtained credentials in the above steps as follows:
token = "<Access Token>"
- Create a
twitter:ConnectionConfig
with the obtained access token and initialize the connector with it.
configurable string token = ?; final twitter:Client twitter = check new({ auth: { token } });
Step 3: Invoke the connector operation
Now, utilize the available connector operations.
Post a tweet
public function main() returns error? { twitter:TweetCreateResponse postTweet = check twitter->/tweets.post( payload = { text: "This is a sample tweet" } ); }
Step 4: Run the Ballerina application
bal run
Examples
The Twitter
connector provides practical examples illustrating usage in various scenarios. Explore these examples, covering the following use cases:
-
Direct message company mentions - Integrate Twitter to send direct messages to users who mention the company in tweets.
-
Tweet performance tracker - Analyze the performance of tweets posted by a user over the past month.
Clients
twitter: Client
Twitter API v2 available endpoints
Constructor
Gets invoked to initialize the connector
.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.twitter.com/2" - URL of the target service
delete dm_events/[DmEventId event_id]
function delete dm_events/[DmEventId event_id](map<string|string[]> headers) returns DeleteDmResponse|error
Delete Dm
Return Type
- DeleteDmResponse|error - The request has succeeded.
delete lists/[ListId id]
function delete lists/[ListId id](map<string|string[]> headers) returns ListDeleteResponse|error
Delete List
Return Type
- ListDeleteResponse|error - The request has succeeded.
delete lists/[ListId id]/members/[UserId user_id]
function delete lists/[ListId id]/members/[UserId user_id](map<string|string[]> headers) returns ListMutateResponse|error
Remove a List member
Return Type
- ListMutateResponse|error - The request has succeeded.
delete tweets/[TweetId id]
function delete tweets/[TweetId id](map<string|string[]> headers) returns TweetDeleteResponse|error
Post delete by Post ID
Return Type
- TweetDeleteResponse|error - The request has succeeded.
delete users/[UserIdMatchesAuthenticatedUser id]/bookmarks/[TweetId tweet_id]
function delete users/[UserIdMatchesAuthenticatedUser id]/bookmarks/[TweetId tweet_id](map<string|string[]> headers) returns BookmarkMutationResponse|error
Remove a bookmarked Post
Return Type
- BookmarkMutationResponse|error - The request has succeeded.
delete users/[UserIdMatchesAuthenticatedUser id]/followed_lists/[ListId list_id]
function delete users/[UserIdMatchesAuthenticatedUser id]/followed_lists/[ListId list_id](map<string|string[]> headers) returns ListFollowedResponse|error
Unfollow a List
Return Type
- ListFollowedResponse|error - The request has succeeded.
delete users/[UserIdMatchesAuthenticatedUser id]/likes/[TweetId tweet_id]
function delete users/[UserIdMatchesAuthenticatedUser id]/likes/[TweetId tweet_id](map<string|string[]> headers) returns UsersLikesDeleteResponse|error
Causes the User (in the path) to unlike the specified Post
Return Type
- UsersLikesDeleteResponse|error - The request has succeeded.
delete users/[UserIdMatchesAuthenticatedUser id]/pinned_lists/[ListId list_id]
function delete users/[UserIdMatchesAuthenticatedUser id]/pinned_lists/[ListId list_id](map<string|string[]> headers) returns ListUnpinResponse|error
Unpin a List
Return Type
- ListUnpinResponse|error - The request has succeeded.
delete users/[UserIdMatchesAuthenticatedUser id]/retweets/[TweetId source_tweet_id]
function delete users/[UserIdMatchesAuthenticatedUser id]/retweets/[TweetId source_tweet_id](map<string|string[]> headers) returns UsersRetweetsDeleteResponse|error
Causes the User (in the path) to unretweet the specified Post
Return Type
- UsersRetweetsDeleteResponse|error - The request has succeeded.
delete users/[UserIdMatchesAuthenticatedUser source_user_id]/following/[UserId target_user_id]
function delete users/[UserIdMatchesAuthenticatedUser source_user_id]/following/[UserId target_user_id](map<string|string[]> headers) returns UsersFollowingDeleteResponse|error
Unfollow User
Return Type
- UsersFollowingDeleteResponse|error - The request has succeeded.
delete users/[UserIdMatchesAuthenticatedUser source_user_id]/muting/[UserId target_user_id]
function delete users/[UserIdMatchesAuthenticatedUser source_user_id]/muting/[UserId target_user_id](map<string|string[]> headers) returns MuteUserMutationResponse|error
Unmute User by User ID
Return Type
- MuteUserMutationResponse|error - The request has succeeded.
get compliance/jobs
function get compliance/jobs(map<string|string[]> headers, *ListBatchComplianceJobsQueries queries) returns Get2ComplianceJobsResponse|error
List Compliance Jobs
Parameters
- queries *ListBatchComplianceJobsQueries - Queries to be sent with the request
Return Type
- Get2ComplianceJobsResponse|error - The request has succeeded.
get compliance/jobs/[JobId id]
function get compliance/jobs/[JobId id](map<string|string[]> headers, *GetBatchComplianceJobQueries queries) returns Get2ComplianceJobsIdResponse|error
Get Compliance Job
Parameters
- queries *GetBatchComplianceJobQueries - Queries to be sent with the request
Return Type
- Get2ComplianceJobsIdResponse|error - The request has succeeded.
get dm_conversations/[DmConversationId id]/dm_events
function get dm_conversations/[DmConversationId id]/dm_events(map<string|string[]> headers, *GetDmConversationsIdDmEventsQueries queries) returns Get2DmConversationsIdDmEventsResponse|error
Get DM Events for a DM Conversation
Parameters
- queries *GetDmConversationsIdDmEventsQueries - Queries to be sent with the request
Return Type
- Get2DmConversationsIdDmEventsResponse|error - The request has succeeded.
get dm_conversations/with/[UserId participant_id]/dm_events
function get dm_conversations/with/[UserId participant_id]/dm_events(map<string|string[]> headers, *GetDmConversationsWithParticipantIdDmEventsQueries queries) returns Get2DmConversationsWithParticipantIdDmEventsResponse|error
Get DM Events for a DM Conversation
Parameters
- queries *GetDmConversationsWithParticipantIdDmEventsQueries - Queries to be sent with the request
Return Type
- Get2DmConversationsWithParticipantIdDmEventsResponse|error - The request has succeeded.
get dm_events
function get dm_events(map<string|string[]> headers, *GetDmEventsQueries queries) returns Get2DmEventsResponse|error
Get recent DM Events
Parameters
- queries *GetDmEventsQueries - Queries to be sent with the request
Return Type
- Get2DmEventsResponse|error - The request has succeeded.
get dm_events/[DmEventId event_id]
function get dm_events/[DmEventId event_id](map<string|string[]> headers, *GetDmEventsByIdQueries queries) returns Get2DmEventsEventIdResponse|error
Get DM Events by id
Parameters
- queries *GetDmEventsByIdQueries - Queries to be sent with the request
Return Type
- Get2DmEventsEventIdResponse|error - The request has succeeded.
get likes/compliance/'stream
function get likes/compliance/'stream(map<string|string[]> headers, *GetLikesComplianceStreamQueries queries) returns LikesComplianceStreamResponse|error
get likes/firehose/'stream
function get likes/firehose/'stream(map<string|string[]> headers, *LikesFirehoseStreamQueries queries) returns StreamingLikeResponse|error
get likes/sample10/'stream
function get likes/sample10/'stream(map<string|string[]> headers, *LikesSample10StreamQueries queries) returns StreamingLikeResponse|error
get lists/[ListId id]
function get lists/[ListId id](map<string|string[]> headers, *ListIdGetQueries queries) returns Get2ListsIdResponse|error
List lookup by List ID.
Parameters
- queries *ListIdGetQueries - Queries to be sent with the request
Return Type
- Get2ListsIdResponse|error - The request has succeeded.
get lists/[ListId id]/followers
function get lists/[ListId id]/followers(map<string|string[]> headers, *ListGetFollowersQueries queries) returns Get2ListsIdFollowersResponse|error
Returns User objects that follow a List by the provided List ID
Parameters
- queries *ListGetFollowersQueries - Queries to be sent with the request
Return Type
- Get2ListsIdFollowersResponse|error - The request has succeeded.
get lists/[ListId id]/members
function get lists/[ListId id]/members(map<string|string[]> headers, *ListGetMembersQueries queries) returns Get2ListsIdMembersResponse|error
Returns User objects that are members of a List by the provided List ID.
Parameters
- queries *ListGetMembersQueries - Queries to be sent with the request
Return Type
- Get2ListsIdMembersResponse|error - The request has succeeded.
get lists/[ListId id]/tweets
function get lists/[ListId id]/tweets(map<string|string[]> headers, *ListsIdTweetsQueries queries) returns Get2ListsIdTweetsResponse|error
List Posts timeline by List ID.
Parameters
- queries *ListsIdTweetsQueries - Queries to be sent with the request
Return Type
- Get2ListsIdTweetsResponse|error - The request has succeeded.
get openapi.json
get spaces
function get spaces(map<string|string[]> headers, *FindSpacesByIdsQueries queries) returns Get2SpacesResponse|error
Space lookup up Space IDs
Parameters
- queries *FindSpacesByIdsQueries - Queries to be sent with the request
Return Type
- Get2SpacesResponse|error - The request has succeeded.
get spaces/'by/creator_ids
function get spaces/'by/creator_ids(map<string|string[]> headers, *FindSpacesByCreatorIdsQueries queries) returns Get2SpacesByCreatorIdsResponse|error
get spaces/[string id]
function get spaces/[string id](map<string|string[]> headers, *FindSpaceByIdQueries queries) returns Get2SpacesIdResponse|error
Space lookup by Space ID
Parameters
- queries *FindSpaceByIdQueries - Queries to be sent with the request
Return Type
- Get2SpacesIdResponse|error - The request has succeeded.
get spaces/[string id]/buyers
function get spaces/[string id]/buyers(map<string|string[]> headers, *SpaceBuyersQueries queries) returns Get2SpacesIdBuyersResponse|error
Retrieve the list of Users who purchased a ticket to the given space
Parameters
- queries *SpaceBuyersQueries - Queries to be sent with the request
Return Type
- Get2SpacesIdBuyersResponse|error - The request has succeeded.
get spaces/[string id]/tweets
function get spaces/[string id]/tweets(map<string|string[]> headers, *SpaceTweetsQueries queries) returns Get2SpacesIdTweetsResponse|error
Retrieve Posts from a Space.
Parameters
- queries *SpaceTweetsQueries - Queries to be sent with the request
Return Type
- Get2SpacesIdTweetsResponse|error - The request has succeeded.
get spaces/search
function get spaces/search(map<string|string[]> headers, *SearchSpacesQueries queries) returns Get2SpacesSearchResponse|error
Search for Spaces
Parameters
- queries *SearchSpacesQueries - Queries to be sent with the request
Return Type
- Get2SpacesSearchResponse|error - The request has succeeded.
get trends/'by/woeid/[int:Signed32 woeid]
function get trends/'by/woeid/[int:Signed32 woeid](map<string|string[]> headers, *GetTrendsQueries queries) returns Get2TrendsByWoeidWoeidResponse|error
get tweets
function get tweets(map<string|string[]> headers, *FindTweetsByIdQueries queries) returns Get2TweetsResponse|error
Post lookup by Post IDs
Parameters
- queries *FindTweetsByIdQueries - Queries to be sent with the request
Return Type
- Get2TweetsResponse|error - The request has succeeded.
get tweets/[TweetId id]
function get tweets/[TweetId id](map<string|string[]> headers, *FindTweetByIdQueries queries) returns Get2TweetsIdResponse|error
Post lookup by Post ID
Parameters
- queries *FindTweetByIdQueries - Queries to be sent with the request
Return Type
- Get2TweetsIdResponse|error - The request has succeeded.
get tweets/[TweetId id]/liking_users
function get tweets/[TweetId id]/liking_users(map<string|string[]> headers, *TweetsIdLikingUsersQueries queries) returns Get2TweetsIdLikingUsersResponse|error
Returns User objects that have liked the provided Post ID
Parameters
- queries *TweetsIdLikingUsersQueries - Queries to be sent with the request
Return Type
- Get2TweetsIdLikingUsersResponse|error - The request has succeeded.
get tweets/[TweetId id]/quote_tweets
function get tweets/[TweetId id]/quote_tweets(map<string|string[]> headers, *FindTweetsThatQuoteATweetQueries queries) returns Get2TweetsIdQuoteTweetsResponse|error
Retrieve Posts that quote a Post.
Parameters
- queries *FindTweetsThatQuoteATweetQueries - Queries to be sent with the request
Return Type
- Get2TweetsIdQuoteTweetsResponse|error - The request has succeeded.
get tweets/[TweetId id]/retweeted_by
function get tweets/[TweetId id]/retweeted_by(map<string|string[]> headers, *TweetsIdRetweetingUsersQueries queries) returns Get2TweetsIdRetweetedByResponse|error
Returns User objects that have retweeted the provided Post ID
Parameters
- queries *TweetsIdRetweetingUsersQueries - Queries to be sent with the request
Return Type
- Get2TweetsIdRetweetedByResponse|error - The request has succeeded.
get tweets/[TweetId id]/retweets
function get tweets/[TweetId id]/retweets(map<string|string[]> headers, *FindTweetsThatRetweetATweetQueries queries) returns Get2TweetsIdRetweetsResponse|error
Retrieve Posts that repost a Post.
Parameters
- queries *FindTweetsThatRetweetATweetQueries - Queries to be sent with the request
Return Type
- Get2TweetsIdRetweetsResponse|error - The request has succeeded.
get tweets/compliance/'stream
function get tweets/compliance/'stream(map<string|string[]> headers, *GetTweetsComplianceStreamQueries queries) returns TweetComplianceStreamResponse|error
get tweets/counts/all
function get tweets/counts/all(map<string|string[]> headers, *TweetCountsFullArchiveSearchQueries queries) returns Get2TweetsCountsAllResponse|error
Full archive search counts
Parameters
- queries *TweetCountsFullArchiveSearchQueries - Queries to be sent with the request
Return Type
- Get2TweetsCountsAllResponse|error - The request has succeeded.
get tweets/counts/recent
function get tweets/counts/recent(map<string|string[]> headers, *TweetCountsRecentSearchQueries queries) returns Get2TweetsCountsRecentResponse|error
Recent search counts
Parameters
- queries *TweetCountsRecentSearchQueries - Queries to be sent with the request
Return Type
- Get2TweetsCountsRecentResponse|error - The request has succeeded.
get tweets/firehose/'stream
function get tweets/firehose/'stream(map<string|string[]> headers, *GetTweetsFirehoseStreamQueries queries) returns StreamingTweetResponse|error
get tweets/firehose/'stream/lang/en
function get tweets/firehose/'stream/lang/en(map<string|string[]> headers, *GetTweetsFirehoseStreamLangEnQueries queries) returns StreamingTweetResponse|error
Parameters
- queries *GetTweetsFirehoseStreamLangEnQueries -
get tweets/firehose/'stream/lang/ja
function get tweets/firehose/'stream/lang/ja(map<string|string[]> headers, *GetTweetsFirehoseStreamLangJaQueries queries) returns StreamingTweetResponse|error
Parameters
- queries *GetTweetsFirehoseStreamLangJaQueries -
get tweets/firehose/'stream/lang/ko
function get tweets/firehose/'stream/lang/ko(map<string|string[]> headers, *GetTweetsFirehoseStreamLangKoQueries queries) returns StreamingTweetResponse|error
Parameters
- queries *GetTweetsFirehoseStreamLangKoQueries -
get tweets/firehose/'stream/lang/pt
function get tweets/firehose/'stream/lang/pt(map<string|string[]> headers, *GetTweetsFirehoseStreamLangPtQueries queries) returns StreamingTweetResponse|error
Parameters
- queries *GetTweetsFirehoseStreamLangPtQueries -
get tweets/label/'stream
function get tweets/label/'stream(map<string|string[]> headers, *GetTweetsLabelStreamQueries queries) returns TweetLabelStreamResponse|error
get tweets/sample/'stream
function get tweets/sample/'stream(map<string|string[]> headers, *SampleStreamQueries queries) returns StreamingTweetResponse|error
get tweets/sample10/'stream
function get tweets/sample10/'stream(map<string|string[]> headers, *GetTweetsSample10StreamQueries queries) returns Get2TweetsSample10StreamResponse|error
get tweets/search/'stream
function get tweets/search/'stream(map<string|string[]> headers, *SearchStreamQueries queries) returns FilteredStreamingTweetResponse|error
get tweets/search/'stream/rules
function get tweets/search/'stream/rules(map<string|string[]> headers, *GetRulesQueries queries) returns RulesLookupResponse|error
get tweets/search/'stream/rules/counts
function get tweets/search/'stream/rules/counts(map<string|string[]> headers, *GetRuleCountQueries queries) returns Get2TweetsSearchStreamRulesCountsResponse|error
get tweets/search/all
function get tweets/search/all(map<string|string[]> headers, *TweetsFullarchiveSearchQueries queries) returns Get2TweetsSearchAllResponse|error
Full-archive search
Parameters
- queries *TweetsFullarchiveSearchQueries - Queries to be sent with the request
Return Type
- Get2TweetsSearchAllResponse|error - The request has succeeded.
get tweets/search/recent
function get tweets/search/recent(map<string|string[]> headers, *TweetsRecentSearchQueries queries) returns Get2TweetsSearchRecentResponse|error
Recent search
Parameters
- queries *TweetsRecentSearchQueries - Queries to be sent with the request
Return Type
- Get2TweetsSearchRecentResponse|error - The request has succeeded.
get usage/tweets
function get usage/tweets(map<string|string[]> headers, *GetUsageTweetsQueries queries) returns Get2UsageTweetsResponse|error
Post Usage
Parameters
- queries *GetUsageTweetsQueries - Queries to be sent with the request
Return Type
- Get2UsageTweetsResponse|error - The request has succeeded.
get users
function get users(map<string|string[]> headers, *FindUsersByIdQueries queries) returns Get2UsersResponse|error
User lookup by IDs
Parameters
- queries *FindUsersByIdQueries - Queries to be sent with the request
Return Type
- Get2UsersResponse|error - The request has succeeded.
get users/'by
function get users/'by(map<string|string[]> headers, *FindUsersByUsernameQueries queries) returns Get2UsersByResponse|error
get users/'by/username/[string username]
function get users/'by/username/[string username](map<string|string[]> headers, *FindUserByUsernameQueries queries) returns Get2UsersByUsernameUsernameResponse|error
get users/[UserIdMatchesAuthenticatedUser id]/blocking
function get users/[UserIdMatchesAuthenticatedUser id]/blocking(map<string|string[]> headers, *UsersIdBlockingQueries queries) returns Get2UsersIdBlockingResponse|error
Returns User objects that are blocked by provided User ID
Parameters
- queries *UsersIdBlockingQueries - Queries to be sent with the request
Return Type
- Get2UsersIdBlockingResponse|error - The request has succeeded.
get users/[UserIdMatchesAuthenticatedUser id]/bookmarks
function get users/[UserIdMatchesAuthenticatedUser id]/bookmarks(map<string|string[]> headers, *GetUsersIdBookmarksQueries queries) returns Get2UsersIdBookmarksResponse|error
Bookmarks by User
Parameters
- queries *GetUsersIdBookmarksQueries - Queries to be sent with the request
Return Type
- Get2UsersIdBookmarksResponse|error - The request has succeeded.
get users/[UserIdMatchesAuthenticatedUser id]/muting
function get users/[UserIdMatchesAuthenticatedUser id]/muting(map<string|string[]> headers, *UsersIdMutingQueries queries) returns Get2UsersIdMutingResponse|error
Returns User objects that are muted by the provided User ID
Parameters
- queries *UsersIdMutingQueries - Queries to be sent with the request
Return Type
- Get2UsersIdMutingResponse|error - The request has succeeded.
get users/[UserIdMatchesAuthenticatedUser id]/pinned_lists
function get users/[UserIdMatchesAuthenticatedUser id]/pinned_lists(map<string|string[]> headers, *ListUserPinnedListsQueries queries) returns Get2UsersIdPinnedListsResponse|error
Get a User's Pinned Lists
Parameters
- queries *ListUserPinnedListsQueries - Queries to be sent with the request
Return Type
- Get2UsersIdPinnedListsResponse|error - The request has succeeded.
get users/[UserIdMatchesAuthenticatedUser id]/timelines/reverse_chronological
function get users/[UserIdMatchesAuthenticatedUser id]/timelines/reverse_chronological(map<string|string[]> headers, *UsersIdTimelineQueries queries) returns Get2UsersIdTimelinesReverseChronologicalResponse|error
User home timeline by User ID
Parameters
- queries *UsersIdTimelineQueries - Queries to be sent with the request
Return Type
- Get2UsersIdTimelinesReverseChronologicalResponse|error - The request has succeeded.
get users/[UserId id]
function get users/[UserId id](map<string|string[]> headers, *FindUserByIdQueries queries) returns Get2UsersIdResponse|error
User lookup by ID
Parameters
- queries *FindUserByIdQueries - Queries to be sent with the request
Return Type
- Get2UsersIdResponse|error - The request has succeeded.
get users/[UserId id]/followed_lists
function get users/[UserId id]/followed_lists(map<string|string[]> headers, *UserFollowedListsQueries queries) returns Get2UsersIdFollowedListsResponse|error
Get User's Followed Lists
Parameters
- queries *UserFollowedListsQueries - Queries to be sent with the request
Return Type
- Get2UsersIdFollowedListsResponse|error - The request has succeeded.
get users/[UserId id]/followers
function get users/[UserId id]/followers(map<string|string[]> headers, *UsersIdFollowersQueries queries) returns Get2UsersIdFollowersResponse|error
Followers by User ID
Parameters
- queries *UsersIdFollowersQueries - Queries to be sent with the request
Return Type
- Get2UsersIdFollowersResponse|error - The request has succeeded.
get users/[UserId id]/following
function get users/[UserId id]/following(map<string|string[]> headers, *UsersIdFollowingQueries queries) returns Get2UsersIdFollowingResponse|error
Following by User ID
Parameters
- queries *UsersIdFollowingQueries - Queries to be sent with the request
Return Type
- Get2UsersIdFollowingResponse|error - The request has succeeded.
get users/[UserId id]/liked_tweets
function get users/[UserId id]/liked_tweets(map<string|string[]> headers, *UsersIdLikedTweetsQueries queries) returns Get2UsersIdLikedTweetsResponse|error
Returns Post objects liked by the provided User ID
Parameters
- queries *UsersIdLikedTweetsQueries - Queries to be sent with the request
Return Type
- Get2UsersIdLikedTweetsResponse|error - The request has succeeded.
get users/[UserId id]/list_memberships
function get users/[UserId id]/list_memberships(map<string|string[]> headers, *GetUserListMembershipsQueries queries) returns Get2UsersIdListMembershipsResponse|error
Get a User's List Memberships
Parameters
- queries *GetUserListMembershipsQueries - Queries to be sent with the request
Return Type
- Get2UsersIdListMembershipsResponse|error - The request has succeeded.
get users/[UserId id]/mentions
function get users/[UserId id]/mentions(map<string|string[]> headers, *UsersIdMentionsQueries queries) returns Get2UsersIdMentionsResponse|error
User mention timeline by User ID
Parameters
- queries *UsersIdMentionsQueries - Queries to be sent with the request
Return Type
- Get2UsersIdMentionsResponse|error - The request has succeeded.
get users/[UserId id]/owned_lists
function get users/[UserId id]/owned_lists(map<string|string[]> headers, *ListUserOwnedListsQueries queries) returns Get2UsersIdOwnedListsResponse|error
Get a User's Owned Lists.
Parameters
- queries *ListUserOwnedListsQueries - Queries to be sent with the request
Return Type
- Get2UsersIdOwnedListsResponse|error - The request has succeeded.
get users/[UserId id]/tweets
function get users/[UserId id]/tweets(map<string|string[]> headers, *UsersIdTweetsQueries queries) returns Get2UsersIdTweetsResponse|error
User Posts timeline by User ID
Parameters
- queries *UsersIdTweetsQueries - Queries to be sent with the request
Return Type
- Get2UsersIdTweetsResponse|error - The request has succeeded.
get users/compliance/'stream
function get users/compliance/'stream(map<string|string[]> headers, *GetUsersComplianceStreamQueries queries) returns UserComplianceStreamResponse|error
get users/me
function get users/me(map<string|string[]> headers, *FindMyUserQueries queries) returns Get2UsersMeResponse|error
User lookup me
Parameters
- queries *FindMyUserQueries - Queries to be sent with the request
Return Type
- Get2UsersMeResponse|error - The request has succeeded.
get users/search
function get users/search(map<string|string[]> headers, *SearchUserByQueryQueries queries) returns Get2UsersSearchResponse|error
User search
Parameters
- queries *SearchUserByQueryQueries - Queries to be sent with the request
Return Type
- Get2UsersSearchResponse|error - The request has succeeded.
post compliance/jobs
function post compliance/jobs(CreateComplianceJobRequest payload, map<string|string[]> headers) returns CreateComplianceJobResponse|error
Create compliance job
Parameters
- payload CreateComplianceJobRequest -
Return Type
- CreateComplianceJobResponse|error - The request has succeeded.
post dm_conversations
function post dm_conversations(CreateDmConversationRequest payload, map<string|string[]> headers) returns CreateDmEventResponse|error
Create a new DM Conversation
Parameters
- payload CreateDmConversationRequest -
Return Type
- CreateDmEventResponse|error - The request has succeeded.
post dm_conversations/[string dm_conversation_id]/messages
function post dm_conversations/[string dm_conversation_id]/messages(CreateMessageRequest payload, map<string|string[]> headers) returns CreateDmEventResponse|error
Send a new message to a DM Conversation
Parameters
- payload CreateMessageRequest -
Return Type
- CreateDmEventResponse|error - The request has succeeded.
post dm_conversations/with/[UserId participant_id]/messages
function post dm_conversations/with/[UserId participant_id]/messages(CreateMessageRequest payload, map<string|string[]> headers) returns CreateDmEventResponse|error
Send a new message to a user
Parameters
- payload CreateMessageRequest -
Return Type
- CreateDmEventResponse|error - The request has succeeded.
post lists
function post lists(ListCreateRequest payload, map<string|string[]> headers) returns ListCreateResponse|error
Create List
Parameters
- payload ListCreateRequest -
Return Type
- ListCreateResponse|error - The request has succeeded.
post lists/[ListId id]/members
function post lists/[ListId id]/members(ListAddUserRequest payload, map<string|string[]> headers) returns ListMutateResponse|error
Add a List member
Parameters
- payload ListAddUserRequest -
Return Type
- ListMutateResponse|error - The request has succeeded.
post tweets
function post tweets(TweetCreateRequest payload, map<string|string[]> headers) returns TweetCreateResponse|error
Creation of a Post
Parameters
- payload TweetCreateRequest -
Return Type
- TweetCreateResponse|error - The request has succeeded.
post tweets/search/'stream/rules
function post tweets/search/'stream/rules(AddOrDeleteRulesRequest payload, map<string|string[]> headers, *AddOrDeleteRulesQueries queries) returns AddOrDeleteRulesResponse|error
post users/[UserIdMatchesAuthenticatedUser id]/bookmarks
function post users/[UserIdMatchesAuthenticatedUser id]/bookmarks(BookmarkAddRequest payload, map<string|string[]> headers) returns BookmarkMutationResponse|error
Add Post to Bookmarks
Parameters
- payload BookmarkAddRequest -
Return Type
- BookmarkMutationResponse|error - The request has succeeded.
post users/[UserIdMatchesAuthenticatedUser id]/followed_lists
function post users/[UserIdMatchesAuthenticatedUser id]/followed_lists(ListFollowedRequest payload, map<string|string[]> headers) returns ListFollowedResponse|error
Follow a List
Parameters
- payload ListFollowedRequest -
Return Type
- ListFollowedResponse|error - The request has succeeded.
post users/[UserIdMatchesAuthenticatedUser id]/following
function post users/[UserIdMatchesAuthenticatedUser id]/following(UsersFollowingCreateRequest payload, map<string|string[]> headers) returns UsersFollowingCreateResponse|error
Follow User
Parameters
- payload UsersFollowingCreateRequest -
Return Type
- UsersFollowingCreateResponse|error - The request has succeeded.
post users/[UserIdMatchesAuthenticatedUser id]/likes
function post users/[UserIdMatchesAuthenticatedUser id]/likes(UsersLikesCreateRequest payload, map<string|string[]> headers) returns UsersLikesCreateResponse|error
Causes the User (in the path) to like the specified Post
Parameters
- payload UsersLikesCreateRequest -
Return Type
- UsersLikesCreateResponse|error - The request has succeeded.
post users/[UserIdMatchesAuthenticatedUser id]/muting
function post users/[UserIdMatchesAuthenticatedUser id]/muting(MuteUserRequest payload, map<string|string[]> headers) returns MuteUserMutationResponse|error
Mute User by User ID.
Parameters
- payload MuteUserRequest -
Return Type
- MuteUserMutationResponse|error - The request has succeeded.
post users/[UserIdMatchesAuthenticatedUser id]/pinned_lists
function post users/[UserIdMatchesAuthenticatedUser id]/pinned_lists(ListPinnedRequest payload, map<string|string[]> headers) returns ListPinnedResponse|error
Pin a List
Parameters
- payload ListPinnedRequest -
Return Type
- ListPinnedResponse|error - The request has succeeded.
post users/[UserIdMatchesAuthenticatedUser id]/retweets
function post users/[UserIdMatchesAuthenticatedUser id]/retweets(UsersRetweetsCreateRequest payload, map<string|string[]> headers) returns UsersRetweetsCreateResponse|error
Causes the User (in the path) to repost the specified Post.
Parameters
- payload UsersRetweetsCreateRequest -
Return Type
- UsersRetweetsCreateResponse|error - The request has succeeded.
put lists/[ListId id]
function put lists/[ListId id](ListUpdateRequest payload, map<string|string[]> headers) returns ListUpdateResponse|error
Update List.
Parameters
- payload ListUpdateRequest -
Return Type
- ListUpdateResponse|error - The request has succeeded.
put tweets/[TweetId tweet_id]/hidden
function put tweets/[TweetId tweet_id]/hidden(TweetHideRequest payload, map<string|string[]> headers) returns TweetHideResponse|error
Hide replies
Parameters
- payload TweetHideRequest -
Return Type
- TweetHideResponse|error - The request has succeeded.
Records
twitter: AddOrDeleteRulesQueries
Represents the Queries record for the operation: addOrDeleteRules
Fields
- dry_run boolean? - Dry Run can be used with both the add and delete action, with the expected result given, but without actually taking any action in the system (meaning the end state will always be as it was when the request was submitted). This is particularly useful to validate rule changes.
- delete_all boolean? - Delete All can be used to delete all of the rules associated this client app, it should be specified with no other parameters. Once deleted, rules cannot be recovered.
twitter: AddOrDeleteRulesResponse
A response from modifying user-specified stream filtering rules.
Fields
- data Rule[]? - All user-specified stream filtering rules that were created.
- errors Problem[]? -
- meta RulesResponseMetadata -
twitter: AddRulesRequest
A request to add a user-specified stream filtering rule.
Fields
- add RuleNoId[] -
twitter: AppRulesCount
A count of user-provided stream filtering rules at the client application level.
Fields
- client_app_id ClientAppId? -
- rule_count Signed32? - Number of rules for client application
twitter: BookmarkAddRequest
Fields
- tweet_id TweetId -
twitter: BookmarkMutationResponse
Fields
- data BookmarkMutationResponse_data? -
- errors Problem[]? -
twitter: BookmarkMutationResponse_data
Fields
- bookmarked boolean? -
twitter: CashtagEntity
Fields
- Fields Included from *EntityIndicesInclusiveExclusive
- Fields Included from *CashtagFields
- tag string
- anydata...
twitter: CashtagFields
Represent the portion of text recognized as a Cashtag, and its start and end position within the text.
Fields
- tag string -
twitter: ClientAppUsage
Usage per client app
Fields
- client_app_id string? - The unique identifier for this project
- usage UsageFields[]? - The usage value
- usage_result_count Signed32? - The number of results returned
twitter: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
twitter: ComplianceJob
Fields
- created_at CreatedAt -
- download_expires_at DownloadExpiration -
- download_url DownloadUrl -
- id JobId -
- name ComplianceJobName? -
- status ComplianceJobStatus -
- 'type ComplianceJobType -
- upload_expires_at UploadExpiration -
- upload_url UploadUrl -
twitter: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
twitter: ContextAnnotation
Annotation inferred from the Tweet text.
Fields
- domain ContextAnnotationDomainFields -
- entity ContextAnnotationEntityFields -
twitter: ContextAnnotationDomainFields
Represents the data for the context annotation domain.
Fields
- description string? - Description of the context annotation domain.
- id string - The unique id for a context annotation domain.
- name string? - Name of the context annotation domain.
twitter: ContextAnnotationEntityFields
Represents the data for the context annotation entity.
Fields
- description string? - Description of the context annotation entity.
- id string - The unique id for a context annotation entity.
- name string? - Name of the context annotation entity.
twitter: CreateAttachmentsMessageRequest
Fields
- attachments DmAttachments -
- text string? - Text of the message.
twitter: CreateComplianceJobRequest
A request to create a new batch compliance job.
Fields
- name ComplianceJobName? -
- resumable boolean? - If true, this endpoint will return a pre-signed URL with resumable uploads enabled.
- 'type "tweets"|"users" - Type of compliance job to list.
twitter: CreateComplianceJobResponse
Fields
- data ComplianceJob? -
- errors Problem[]? -
twitter: CreateDmConversationRequest
Fields
- conversation_type "Group" - The conversation type that is being created.
- message CreateMessageRequest -
- participant_ids DmParticipants -
twitter: CreateDmEventResponse
Fields
- data CreateDmEventResponse_data? -
- errors Problem[]? -
twitter: CreateDmEventResponse_data
Fields
- dm_conversation_id DmConversationId -
- dm_event_id DmEventId -
twitter: CreateTextMessageRequest
Fields
- attachments DmAttachments? -
- text string - Text of the message.
twitter: DeleteDmResponse
Fields
- data DeleteDmResponse_data? -
- errors Problem[]? -
twitter: DeleteDmResponse_data
Fields
- deleted boolean? -
twitter: DeleteRulesRequest
A response from deleting user-specified stream filtering rules.
Fields
- delete DeleteRulesRequest_delete -
twitter: DeleteRulesRequest_delete
IDs and values of all deleted user-specified stream filtering rules.
Fields
- ids RuleId[]? - IDs of all deleted user-specified stream filtering rules.
- values RuleValue[]? - Values of all deleted user-specified stream filtering rules.
twitter: DmEvent
Fields
- attachments DmEvent_attachments? -
- cashtags CashtagEntity[]? -
- created_at string? -
- dm_conversation_id DmConversationId? -
- event_type string -
- hashtags HashtagEntity[]? -
- id DmEventId -
- mentions MentionEntity[]? -
- participant_ids UserId[]? - A list of participants for a ParticipantsJoin or ParticipantsLeave event_type.
- referenced_tweets DmEvent_referenced_tweets[]? - A list of Posts this DM refers to.
- sender_id UserId? -
- text string? -
- urls UrlEntityDm[]? -
twitter: DmEvent_attachments
Specifies the type of attachments (if any) present in this DM.
Fields
- card_ids string[]? - A list of card IDs (if cards are attached).
- media_keys MediaKey[]? - A list of Media Keys for each one of the media attachments (if media are attached).
twitter: DmEvent_referenced_tweets
Fields
- id TweetId -
twitter: DmMediaAttachment
Fields
- media_id MediaId -
twitter: EntityIndicesInclusiveExclusive
Represent a boundary range (start and end index) for a recognized entity (for example a hashtag or a mention). start
must be smaller than end
. The start index is inclusive, the end index is exclusive.
Fields
- end int - Index (zero-based) at which position this entity ends. The index is exclusive.
- 'start int - Index (zero-based) at which position this entity starts. The index is inclusive.
twitter: EntityIndicesInclusiveInclusive
Represent a boundary range (start and end index) for a recognized entity (for example a hashtag or a mention). start
must be smaller than end
. The start index is inclusive, the end index is inclusive.
Fields
- end int - Index (zero-based) at which position this entity ends. The index is inclusive.
- 'start int - Index (zero-based) at which position this entity starts. The index is inclusive.
twitter: Expansions
Fields
- media Media[]? -
- places Place[]? -
- polls Poll[]? -
- topics Topic[]? -
- tweets Tweet[]? -
- users User[]? -
twitter: FilteredStreamingTweetResponse
A Tweet or error that can be returned by the streaming Tweet API. The values returned with a successful streamed Tweet includes the user provided rules that the Tweet matched.
Fields
- data Tweet? -
- errors Problem[]? -
- includes Expansions? -
- matching_rules FilteredStreamingTweetResponse_matching_rules[]? - The list of rules which matched the Tweet
twitter: FilteredStreamingTweetResponse_matching_rules
Fields
- id RuleId -
- tag RuleTag? -
twitter: FindMyUserQueries
Represents the Queries record for the operation: findMyUser
Fields
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- expansions ("most_recent_tweet_id"|"pinned_tweet_id")[]? - A comma separated list of fields to expand.
twitter: FindSpaceByIdQueries
Represents the Queries record for the operation: findSpaceById
Fields
- space\.fields ("created_at"|"creator_id"|"ended_at"|"host_ids"|"id"|"invited_user_ids"|"is_ticketed"|"lang"|"participant_count"|"scheduled_start"|"speaker_ids"|"started_at"|"state"|"subscriber_count"|"title"|"topic_ids"|"updated_at")[]? - A comma separated list of Space fields to display.
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- topic\.fields ("description"|"id"|"name")[]? - A comma separated list of Topic fields to display.
- expansions ("creator_id"|"host_ids"|"invited_user_ids"|"speaker_ids"|"topic_ids")[]? - A comma separated list of fields to expand.
twitter: FindSpacesByCreatorIdsQueries
Represents the Queries record for the operation: findSpacesByCreatorIds
Fields
- space\.fields ("created_at"|"creator_id"|"ended_at"|"host_ids"|"id"|"invited_user_ids"|"is_ticketed"|"lang"|"participant_count"|"scheduled_start"|"speaker_ids"|"started_at"|"state"|"subscriber_count"|"title"|"topic_ids"|"updated_at")[]? - A comma separated list of Space fields to display.
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- user_ids UserId[] - The IDs of Users to search through.
- topic\.fields ("description"|"id"|"name")[]? - A comma separated list of Topic fields to display.
- expansions ("creator_id"|"host_ids"|"invited_user_ids"|"speaker_ids"|"topic_ids")[]? - A comma separated list of fields to expand.
twitter: FindSpacesByIdsQueries
Represents the Queries record for the operation: findSpacesByIds
Fields
- space\.fields ("created_at"|"creator_id"|"ended_at"|"host_ids"|"id"|"invited_user_ids"|"is_ticketed"|"lang"|"participant_count"|"scheduled_start"|"speaker_ids"|"started_at"|"state"|"subscriber_count"|"title"|"topic_ids"|"updated_at")[]? - A comma separated list of Space fields to display.
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- ids FindSpacesByIdsQueriesIdsItemsString[] - The list of Space IDs to return.
- topic\.fields ("description"|"id"|"name")[]? - A comma separated list of Topic fields to display.
- expansions ("creator_id"|"host_ids"|"invited_user_ids"|"speaker_ids"|"topic_ids")[]? - A comma separated list of fields to expand.
twitter: FindTweetByIdQueries
Represents the Queries record for the operation: findTweetById
Fields
- poll\.fields ("duration_minutes"|"end_datetime"|"id"|"options"|"voting_status")[]? - A comma separated list of Poll fields to display.
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- media\.fields ("alt_text"|"duration_ms"|"height"|"media_key"|"non_public_metrics"|"organic_metrics"|"preview_image_url"|"promoted_metrics"|"public_metrics"|"type"|"url"|"variants"|"width")[]? - A comma separated list of Media fields to display.
- place\.fields ("contained_within"|"country"|"country_code"|"full_name"|"geo"|"id"|"name"|"place_type")[]? - A comma separated list of Place fields to display.
- expansions ("attachments.media_keys"|"attachments.media_source_tweet"|"attachments.poll_ids"|"author_id"|"edit_history_tweet_ids"|"entities.mentions.username"|"geo.place_id"|"in_reply_to_user_id"|"entities.note.mentions.username"|"referenced_tweets.id"|"referenced_tweets.id.author_id"|"author_screen_name")[]? - A comma separated list of fields to expand.
twitter: FindTweetsByIdQueries
Represents the Queries record for the operation: findTweetsById
Fields
- poll\.fields ("duration_minutes"|"end_datetime"|"id"|"options"|"voting_status")[]? - A comma separated list of Poll fields to display.
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- media\.fields ("alt_text"|"duration_ms"|"height"|"media_key"|"non_public_metrics"|"organic_metrics"|"preview_image_url"|"promoted_metrics"|"public_metrics"|"type"|"url"|"variants"|"width")[]? - A comma separated list of Media fields to display.
- ids TweetId[] - A comma separated list of Post IDs. Up to 100 are allowed in a single request.
- place\.fields ("contained_within"|"country"|"country_code"|"full_name"|"geo"|"id"|"name"|"place_type")[]? - A comma separated list of Place fields to display.
- expansions ("attachments.media_keys"|"attachments.media_source_tweet"|"attachments.poll_ids"|"author_id"|"edit_history_tweet_ids"|"entities.mentions.username"|"geo.place_id"|"in_reply_to_user_id"|"entities.note.mentions.username"|"referenced_tweets.id"|"referenced_tweets.id.author_id"|"author_screen_name")[]? - A comma separated list of fields to expand.
twitter: FindTweetsThatQuoteATweetQueries
Represents the Queries record for the operation: findTweetsThatQuoteATweet
Fields
- poll\.fields ("duration_minutes"|"end_datetime"|"id"|"options"|"voting_status")[]? - A comma separated list of Poll fields to display.
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- pagination_token PaginationToken36? - This parameter is used to get a specified 'page' of results.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- media\.fields ("alt_text"|"duration_ms"|"height"|"media_key"|"non_public_metrics"|"organic_metrics"|"preview_image_url"|"promoted_metrics"|"public_metrics"|"type"|"url"|"variants"|"width")[]? - A comma separated list of Media fields to display.
- max_results Signed32(default 10) - The maximum number of results to be returned.
- place\.fields ("contained_within"|"country"|"country_code"|"full_name"|"geo"|"id"|"name"|"place_type")[]? - A comma separated list of Place fields to display.
- exclude ("replies"|"retweets")[]? - The set of entities to exclude (e.g. 'replies' or 'retweets').
- expansions ("attachments.media_keys"|"attachments.media_source_tweet"|"attachments.poll_ids"|"author_id"|"edit_history_tweet_ids"|"entities.mentions.username"|"geo.place_id"|"in_reply_to_user_id"|"entities.note.mentions.username"|"referenced_tweets.id"|"referenced_tweets.id.author_id"|"author_screen_name")[]? - A comma separated list of fields to expand.
twitter: FindTweetsThatRetweetATweetQueries
Represents the Queries record for the operation: findTweetsThatRetweetATweet
Fields
- poll\.fields ("duration_minutes"|"end_datetime"|"id"|"options"|"voting_status")[]? - A comma separated list of Poll fields to display.
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- pagination_token PaginationToken36? - This parameter is used to get the next 'page' of results.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- media\.fields ("alt_text"|"duration_ms"|"height"|"media_key"|"non_public_metrics"|"organic_metrics"|"preview_image_url"|"promoted_metrics"|"public_metrics"|"type"|"url"|"variants"|"width")[]? - A comma separated list of Media fields to display.
- max_results Signed32(default 100) - The maximum number of results.
- place\.fields ("contained_within"|"country"|"country_code"|"full_name"|"geo"|"id"|"name"|"place_type")[]? - A comma separated list of Place fields to display.
- expansions ("attachments.media_keys"|"attachments.media_source_tweet"|"attachments.poll_ids"|"author_id"|"edit_history_tweet_ids"|"entities.mentions.username"|"geo.place_id"|"in_reply_to_user_id"|"entities.note.mentions.username"|"referenced_tweets.id"|"referenced_tweets.id.author_id"|"author_screen_name")[]? - A comma separated list of fields to expand.
twitter: FindUserByIdQueries
Represents the Queries record for the operation: findUserById
Fields
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- expansions ("most_recent_tweet_id"|"pinned_tweet_id")[]? - A comma separated list of fields to expand.
twitter: FindUserByUsernameQueries
Represents the Queries record for the operation: findUserByUsername
Fields
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- expansions ("most_recent_tweet_id"|"pinned_tweet_id")[]? - A comma separated list of fields to expand.
twitter: FindUsersByIdQueries
Represents the Queries record for the operation: findUsersById
Fields
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- ids UserId[] - A list of User IDs, comma-separated. You can specify up to 100 IDs.
- expansions ("most_recent_tweet_id"|"pinned_tweet_id")[]? - A comma separated list of fields to expand.
twitter: FindUsersByUsernameQueries
Represents the Queries record for the operation: findUsersByUsername
Fields
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- usernames FindUsersByUsernameQueriesUsernamesItemsString[] - A list of usernames, comma-separated.
- expansions ("most_recent_tweet_id"|"pinned_tweet_id")[]? - A comma separated list of fields to expand.
twitter: FullTextEntities
Fields
- cashtags CashtagEntity[]? -
- hashtags HashtagEntity[]? -
- mentions MentionEntity[]? -
- urls UrlEntity[]? -
twitter: FullTextEntitiesAnnotationsItemsnull
Fields
- Fields Included from *EntityIndicesInclusiveInclusive
- normalized_text string? -
- probability decimal? -
- 'type string? -
twitter: Geo
Fields
- bbox GeoBboxItemsNumber[] -
- geometry Point? -
- properties record {} -
- 'type "Feature" -
twitter: Get2ComplianceJobsIdResponse
Fields
- data ComplianceJob? -
- errors Problem[]? -
twitter: Get2ComplianceJobsResponse
Fields
- data ComplianceJob[]? -
- errors Problem[]? -
- meta Get2ComplianceJobsResponse_meta? -
twitter: Get2ComplianceJobsResponse_meta
Fields
- result_count ResultCount? -
twitter: Get2DmConversationsIdDmEventsResponse
Fields
- data DmEvent[]? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2DmConversationsIdDmEventsResponse_meta
Fields
- next_token NextToken? -
- previous_token PreviousToken? -
- result_count ResultCount? -
twitter: Get2DmConversationsWithParticipantIdDmEventsResponse
Fields
- data DmEvent[]? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2DmEventsEventIdResponse
Fields
- data DmEvent? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2DmEventsResponse
Fields
- data DmEvent[]? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2ListsIdFollowersResponse
Fields
- data User[]? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2ListsIdMembersResponse
Fields
- data User[]? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2ListsIdResponse
Fields
- data List? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2ListsIdTweetsResponse
Fields
- data Tweet[]? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2SpacesByCreatorIdsResponse
Fields
- data Space[]? -
- errors Problem[]? -
- includes Expansions? -
- meta Get2ComplianceJobsResponse_meta? -
twitter: Get2SpacesIdBuyersResponse
Fields
- data User[]? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2SpacesIdResponse
Fields
- data Space? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2SpacesIdTweetsResponse
Fields
- data Tweet[]? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2SpacesResponse
Fields
- data Space[]? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2SpacesSearchResponse
Fields
- data Space[]? -
- errors Problem[]? -
- includes Expansions? -
- meta Get2ComplianceJobsResponse_meta? -
twitter: Get2TrendsByWoeidWoeidResponse
Fields
- data Trend[]? -
- errors Problem[]? -
twitter: Get2TweetsCountsAllResponse
Fields
- data SearchCount[]? -
- errors Problem[]? -
- meta Get2TweetsCountsAllResponse_meta? -
twitter: Get2TweetsCountsAllResponse_meta
Fields
- newest_id NewestId? -
- next_token NextToken? -
- oldest_id OldestId? -
- total_tweet_count Aggregate? -
twitter: Get2TweetsCountsRecentResponse
Fields
- data SearchCount[]? -
- errors Problem[]? -
- meta Get2TweetsCountsAllResponse_meta? -
twitter: Get2TweetsIdLikingUsersResponse
Fields
- data User[]? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2TweetsIdQuoteTweetsResponse
Fields
- data Tweet[]? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2TweetsIdQuoteTweetsResponse_meta
Fields
- next_token NextToken? -
- result_count ResultCount? -
twitter: Get2TweetsIdResponse
Fields
- data Tweet? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2TweetsIdRetweetedByResponse
Fields
- data User[]? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2TweetsIdRetweetsResponse
Fields
- data Tweet[]? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2TweetsResponse
Fields
- data Tweet[]? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2TweetsSample10StreamResponse
Fields
- data Tweet? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2TweetsSearchAllResponse
Fields
- data Tweet[]? -
- errors Problem[]? -
- includes Expansions? -
- meta Get2TweetsSearchAllResponse_meta? -
twitter: Get2TweetsSearchAllResponse_meta
Fields
- newest_id NewestId? -
- next_token NextToken? -
- oldest_id OldestId? -
- result_count ResultCount? -
twitter: Get2TweetsSearchRecentResponse
Fields
- data Tweet[]? -
- errors Problem[]? -
- includes Expansions? -
- meta Get2TweetsSearchAllResponse_meta? -
twitter: Get2TweetsSearchStreamRulesCountsResponse
Fields
- data RulesCount? -
- errors Problem[]? -
twitter: Get2UsageTweetsResponse
Fields
- data Usage? -
- errors Problem[]? -
twitter: Get2UsersByResponse
Fields
- data User[]? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2UsersByUsernameUsernameResponse
Fields
- data User? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2UsersIdBlockingResponse
Fields
- data User[]? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2UsersIdBookmarksResponse
Fields
- data Tweet[]? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2UsersIdFollowedListsResponse
Fields
- data List[]? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2UsersIdFollowersResponse
Fields
- data User[]? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2UsersIdFollowingResponse
Fields
- data User[]? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2UsersIdLikedTweetsResponse
Fields
- data Tweet[]? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2UsersIdListMembershipsResponse
Fields
- data List[]? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2UsersIdMentionsResponse
Fields
- data Tweet[]? -
- errors Problem[]? -
- includes Expansions? -
- meta Get2UsersIdMentionsResponse_meta? -
twitter: Get2UsersIdMentionsResponse_meta
Fields
- newest_id NewestId? -
- next_token NextToken? -
- oldest_id OldestId? -
- previous_token PreviousToken? -
- result_count ResultCount? -
twitter: Get2UsersIdMutingResponse
Fields
- data User[]? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2UsersIdOwnedListsResponse
Fields
- data List[]? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2UsersIdPinnedListsResponse
Fields
- data List[]? -
- errors Problem[]? -
- includes Expansions? -
- meta Get2ComplianceJobsResponse_meta? -
twitter: Get2UsersIdResponse
Fields
- data User? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2UsersIdTimelinesReverseChronologicalResponse
Fields
- data Tweet[]? -
- errors Problem[]? -
- includes Expansions? -
- meta Get2UsersIdMentionsResponse_meta? -
twitter: Get2UsersIdTweetsResponse
Fields
- data Tweet[]? -
- errors Problem[]? -
- includes Expansions? -
- meta Get2UsersIdMentionsResponse_meta? -
twitter: Get2UsersMeResponse
Fields
- data User? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2UsersResponse
Fields
- data User[]? -
- errors Problem[]? -
- includes Expansions? -
twitter: Get2UsersSearchResponse
Fields
- data User[]? -
- errors Problem[]? -
- includes Expansions? -
- meta Get2UsersSearchResponse_meta? -
twitter: Get2UsersSearchResponse_meta
Fields
- next_token NextToken? -
- previous_token PreviousToken? -
twitter: GetBatchComplianceJobQueries
Represents the Queries record for the operation: getBatchComplianceJob
Fields
- compliance_job\.fields ("created_at"|"download_expires_at"|"download_url"|"id"|"name"|"resumable"|"status"|"type"|"upload_expires_at"|"upload_url")[]? - A comma separated list of ComplianceJob fields to display.
twitter: GetDmConversationsIdDmEventsQueries
Represents the Queries record for the operation: getDmConversationsIdDmEvents
Fields
- dm_event\.fields ("attachments"|"created_at"|"dm_conversation_id"|"entities"|"event_type"|"id"|"participant_ids"|"referenced_tweets"|"sender_id"|"text")[]? - A comma separated list of DmEvent fields to display.
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- pagination_token PaginationToken32? - This parameter is used to get a specified 'page' of results.
- event_types ("MessageCreate"|"ParticipantsJoin"|"ParticipantsLeave")[](default ["MessageCreate","ParticipantsLeave","ParticipantsJoin"]) - The set of event_types to include in the results.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- media\.fields ("alt_text"|"duration_ms"|"height"|"media_key"|"non_public_metrics"|"organic_metrics"|"preview_image_url"|"promoted_metrics"|"public_metrics"|"type"|"url"|"variants"|"width")[]? - A comma separated list of Media fields to display.
- max_results Signed32(default 100) - The maximum number of results.
- expansions ("attachments.media_keys"|"participant_ids"|"referenced_tweets.id"|"sender_id")[]? - A comma separated list of fields to expand.
twitter: GetDmConversationsWithParticipantIdDmEventsQueries
Represents the Queries record for the operation: getDmConversationsWithParticipantIdDmEvents
Fields
- dm_event\.fields ("attachments"|"created_at"|"dm_conversation_id"|"entities"|"event_type"|"id"|"participant_ids"|"referenced_tweets"|"sender_id"|"text")[]? - A comma separated list of DmEvent fields to display.
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- pagination_token PaginationToken32? - This parameter is used to get a specified 'page' of results.
- event_types ("MessageCreate"|"ParticipantsJoin"|"ParticipantsLeave")[](default ["MessageCreate","ParticipantsLeave","ParticipantsJoin"]) - The set of event_types to include in the results.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- media\.fields ("alt_text"|"duration_ms"|"height"|"media_key"|"non_public_metrics"|"organic_metrics"|"preview_image_url"|"promoted_metrics"|"public_metrics"|"type"|"url"|"variants"|"width")[]? - A comma separated list of Media fields to display.
- max_results Signed32(default 100) - The maximum number of results.
- expansions ("attachments.media_keys"|"participant_ids"|"referenced_tweets.id"|"sender_id")[]? - A comma separated list of fields to expand.
twitter: GetDmEventsByIdQueries
Represents the Queries record for the operation: getDmEventsById
Fields
- dm_event\.fields ("attachments"|"created_at"|"dm_conversation_id"|"entities"|"event_type"|"id"|"participant_ids"|"referenced_tweets"|"sender_id"|"text")[]? - A comma separated list of DmEvent fields to display.
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- media\.fields ("alt_text"|"duration_ms"|"height"|"media_key"|"non_public_metrics"|"organic_metrics"|"preview_image_url"|"promoted_metrics"|"public_metrics"|"type"|"url"|"variants"|"width")[]? - A comma separated list of Media fields to display.
- expansions ("attachments.media_keys"|"participant_ids"|"referenced_tweets.id"|"sender_id")[]? - A comma separated list of fields to expand.
twitter: GetDmEventsQueries
Represents the Queries record for the operation: getDmEvents
Fields
- dm_event\.fields ("attachments"|"created_at"|"dm_conversation_id"|"entities"|"event_type"|"id"|"participant_ids"|"referenced_tweets"|"sender_id"|"text")[]? - A comma separated list of DmEvent fields to display.
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- pagination_token PaginationToken32? - This parameter is used to get a specified 'page' of results.
- event_types ("MessageCreate"|"ParticipantsJoin"|"ParticipantsLeave")[](default ["MessageCreate","ParticipantsLeave","ParticipantsJoin"]) - The set of event_types to include in the results.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- media\.fields ("alt_text"|"duration_ms"|"height"|"media_key"|"non_public_metrics"|"organic_metrics"|"preview_image_url"|"promoted_metrics"|"public_metrics"|"type"|"url"|"variants"|"width")[]? - A comma separated list of Media fields to display.
- max_results Signed32(default 100) - The maximum number of results.
- expansions ("attachments.media_keys"|"participant_ids"|"referenced_tweets.id"|"sender_id")[]? - A comma separated list of fields to expand.
twitter: GetLikesComplianceStreamQueries
Represents the Queries record for the operation: getLikesComplianceStream
Fields
- backfill_minutes Signed32? - The number of minutes of backfill requested.
- start_time string? - YYYY-MM-DDTHH:mm:ssZ. The earliest UTC timestamp from which the Likes Compliance events will be provided.
- end_time string? - YYYY-MM-DDTHH:mm:ssZ. The latest UTC timestamp from which the Likes Compliance events will be provided.
twitter: GetRuleCountQueries
Represents the Queries record for the operation: getRuleCount
Fields
- rules_count\.fields ("all_project_client_apps"|"cap_per_client_app"|"cap_per_project"|"client_app_rules_count"|"project_rules_count")[]? - A comma separated list of RulesCount fields to display.
twitter: GetRulesQueries
Represents the Queries record for the operation: getRules
Fields
- pagination_token string? - This value is populated by passing the 'next_token' returned in a request to paginate through results.
- ids RuleId[]? - A comma-separated list of Rule IDs.
- max_results Signed32(default 1000) - The maximum number of results.
twitter: GetTrendsQueries
Represents the Queries record for the operation: getTrends
Fields
- trend\.fields ("trend_name"|"tweet_count")[]? - A comma separated list of Trend fields to display.
twitter: GetTweetsComplianceStreamQueries
Represents the Queries record for the operation: getTweetsComplianceStream
Fields
- backfill_minutes Signed32? - The number of minutes of backfill requested.
- start_time string? - YYYY-MM-DDTHH:mm:ssZ. The earliest UTC timestamp from which the Post Compliance events will be provided.
- partition Signed32 - The partition number.
- end_time string? - YYYY-MM-DDTHH:mm:ssZ. The latest UTC timestamp to which the Post Compliance events will be provided.
twitter: GetTweetsFirehoseStreamLangEnQueries
Represents the Queries record for the operation: getTweetsFirehoseStreamLangEn
Fields
- poll\.fields ("duration_minutes"|"end_datetime"|"id"|"options"|"voting_status")[]? - A comma separated list of Poll fields to display.
- backfill_minutes Signed32? - The number of minutes of backfill requested.
- start_time string? - YYYY-MM-DDTHH:mm:ssZ. The earliest UTC timestamp to which the Posts will be provided.
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- partition Signed32 - The partition number.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- media\.fields ("alt_text"|"duration_ms"|"height"|"media_key"|"non_public_metrics"|"organic_metrics"|"preview_image_url"|"promoted_metrics"|"public_metrics"|"type"|"url"|"variants"|"width")[]? - A comma separated list of Media fields to display.
- end_time string? - YYYY-MM-DDTHH:mm:ssZ. The latest UTC timestamp to which the Posts will be provided.
- place\.fields ("contained_within"|"country"|"country_code"|"full_name"|"geo"|"id"|"name"|"place_type")[]? - A comma separated list of Place fields to display.
- expansions ("attachments.media_keys"|"attachments.media_source_tweet"|"attachments.poll_ids"|"author_id"|"edit_history_tweet_ids"|"entities.mentions.username"|"geo.place_id"|"in_reply_to_user_id"|"entities.note.mentions.username"|"referenced_tweets.id"|"referenced_tweets.id.author_id"|"author_screen_name")[]? - A comma separated list of fields to expand.
twitter: GetTweetsFirehoseStreamLangJaQueries
Represents the Queries record for the operation: getTweetsFirehoseStreamLangJa
Fields
- poll\.fields ("duration_minutes"|"end_datetime"|"id"|"options"|"voting_status")[]? - A comma separated list of Poll fields to display.
- backfill_minutes Signed32? - The number of minutes of backfill requested.
- start_time string? - YYYY-MM-DDTHH:mm:ssZ. The earliest UTC timestamp to which the Posts will be provided.
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- partition Signed32 - The partition number.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- media\.fields ("alt_text"|"duration_ms"|"height"|"media_key"|"non_public_metrics"|"organic_metrics"|"preview_image_url"|"promoted_metrics"|"public_metrics"|"type"|"url"|"variants"|"width")[]? - A comma separated list of Media fields to display.
- end_time string? - YYYY-MM-DDTHH:mm:ssZ. The latest UTC timestamp to which the Posts will be provided.
- place\.fields ("contained_within"|"country"|"country_code"|"full_name"|"geo"|"id"|"name"|"place_type")[]? - A comma separated list of Place fields to display.
- expansions ("attachments.media_keys"|"attachments.media_source_tweet"|"attachments.poll_ids"|"author_id"|"edit_history_tweet_ids"|"entities.mentions.username"|"geo.place_id"|"in_reply_to_user_id"|"entities.note.mentions.username"|"referenced_tweets.id"|"referenced_tweets.id.author_id"|"author_screen_name")[]? - A comma separated list of fields to expand.
twitter: GetTweetsFirehoseStreamLangKoQueries
Represents the Queries record for the operation: getTweetsFirehoseStreamLangKo
Fields
- poll\.fields ("duration_minutes"|"end_datetime"|"id"|"options"|"voting_status")[]? - A comma separated list of Poll fields to display.
- backfill_minutes Signed32? - The number of minutes of backfill requested.
- start_time string? - YYYY-MM-DDTHH:mm:ssZ. The earliest UTC timestamp to which the Posts will be provided.
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- partition Signed32 - The partition number.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- media\.fields ("alt_text"|"duration_ms"|"height"|"media_key"|"non_public_metrics"|"organic_metrics"|"preview_image_url"|"promoted_metrics"|"public_metrics"|"type"|"url"|"variants"|"width")[]? - A comma separated list of Media fields to display.
- end_time string? - YYYY-MM-DDTHH:mm:ssZ. The latest UTC timestamp to which the Posts will be provided.
- place\.fields ("contained_within"|"country"|"country_code"|"full_name"|"geo"|"id"|"name"|"place_type")[]? - A comma separated list of Place fields to display.
- expansions ("attachments.media_keys"|"attachments.media_source_tweet"|"attachments.poll_ids"|"author_id"|"edit_history_tweet_ids"|"entities.mentions.username"|"geo.place_id"|"in_reply_to_user_id"|"entities.note.mentions.username"|"referenced_tweets.id"|"referenced_tweets.id.author_id"|"author_screen_name")[]? - A comma separated list of fields to expand.
twitter: GetTweetsFirehoseStreamLangPtQueries
Represents the Queries record for the operation: getTweetsFirehoseStreamLangPt
Fields
- poll\.fields ("duration_minutes"|"end_datetime"|"id"|"options"|"voting_status")[]? - A comma separated list of Poll fields to display.
- backfill_minutes Signed32? - The number of minutes of backfill requested.
- start_time string? - YYYY-MM-DDTHH:mm:ssZ. The earliest UTC timestamp to which the Posts will be provided.
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- partition Signed32 - The partition number.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- media\.fields ("alt_text"|"duration_ms"|"height"|"media_key"|"non_public_metrics"|"organic_metrics"|"preview_image_url"|"promoted_metrics"|"public_metrics"|"type"|"url"|"variants"|"width")[]? - A comma separated list of Media fields to display.
- end_time string? - YYYY-MM-DDTHH:mm:ssZ. The latest UTC timestamp to which the Posts will be provided.
- place\.fields ("contained_within"|"country"|"country_code"|"full_name"|"geo"|"id"|"name"|"place_type")[]? - A comma separated list of Place fields to display.
- expansions ("attachments.media_keys"|"attachments.media_source_tweet"|"attachments.poll_ids"|"author_id"|"edit_history_tweet_ids"|"entities.mentions.username"|"geo.place_id"|"in_reply_to_user_id"|"entities.note.mentions.username"|"referenced_tweets.id"|"referenced_tweets.id.author_id"|"author_screen_name")[]? - A comma separated list of fields to expand.
twitter: GetTweetsFirehoseStreamQueries
Represents the Queries record for the operation: getTweetsFirehoseStream
Fields
- poll\.fields ("duration_minutes"|"end_datetime"|"id"|"options"|"voting_status")[]? - A comma separated list of Poll fields to display.
- backfill_minutes Signed32? - The number of minutes of backfill requested.
- start_time string? - YYYY-MM-DDTHH:mm:ssZ. The earliest UTC timestamp to which the Posts will be provided.
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- partition Signed32 - The partition number.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- media\.fields ("alt_text"|"duration_ms"|"height"|"media_key"|"non_public_metrics"|"organic_metrics"|"preview_image_url"|"promoted_metrics"|"public_metrics"|"type"|"url"|"variants"|"width")[]? - A comma separated list of Media fields to display.
- end_time string? - YYYY-MM-DDTHH:mm:ssZ. The latest UTC timestamp to which the Posts will be provided.
- place\.fields ("contained_within"|"country"|"country_code"|"full_name"|"geo"|"id"|"name"|"place_type")[]? - A comma separated list of Place fields to display.
- expansions ("attachments.media_keys"|"attachments.media_source_tweet"|"attachments.poll_ids"|"author_id"|"edit_history_tweet_ids"|"entities.mentions.username"|"geo.place_id"|"in_reply_to_user_id"|"entities.note.mentions.username"|"referenced_tweets.id"|"referenced_tweets.id.author_id"|"author_screen_name")[]? - A comma separated list of fields to expand.
twitter: GetTweetsLabelStreamQueries
Represents the Queries record for the operation: getTweetsLabelStream
Fields
- backfill_minutes Signed32? - The number of minutes of backfill requested.
- start_time string? - YYYY-MM-DDTHH:mm:ssZ. The earliest UTC timestamp from which the Post labels will be provided.
- end_time string? - YYYY-MM-DDTHH:mm:ssZ. The latest UTC timestamp from which the Post labels will be provided.
twitter: GetTweetsSample10StreamQueries
Represents the Queries record for the operation: getTweetsSample10Stream
Fields
- poll\.fields ("duration_minutes"|"end_datetime"|"id"|"options"|"voting_status")[]? - A comma separated list of Poll fields to display.
- backfill_minutes Signed32? - The number of minutes of backfill requested.
- start_time string? - YYYY-MM-DDTHH:mm:ssZ. The earliest UTC timestamp to which the Posts will be provided.
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- partition Signed32 - The partition number.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- media\.fields ("alt_text"|"duration_ms"|"height"|"media_key"|"non_public_metrics"|"organic_metrics"|"preview_image_url"|"promoted_metrics"|"public_metrics"|"type"|"url"|"variants"|"width")[]? - A comma separated list of Media fields to display.
- end_time string? - YYYY-MM-DDTHH:mm:ssZ. The latest UTC timestamp to which the Posts will be provided.
- place\.fields ("contained_within"|"country"|"country_code"|"full_name"|"geo"|"id"|"name"|"place_type")[]? - A comma separated list of Place fields to display.
- expansions ("attachments.media_keys"|"attachments.media_source_tweet"|"attachments.poll_ids"|"author_id"|"edit_history_tweet_ids"|"entities.mentions.username"|"geo.place_id"|"in_reply_to_user_id"|"entities.note.mentions.username"|"referenced_tweets.id"|"referenced_tweets.id.author_id"|"author_screen_name")[]? - A comma separated list of fields to expand.
twitter: GetUsageTweetsQueries
Represents the Queries record for the operation: getUsageTweets
Fields
- usage\.fields ("cap_reset_day"|"daily_client_app_usage"|"daily_project_usage"|"project_cap"|"project_id"|"project_usage")[]? - A comma separated list of Usage fields to display.
- days Signed32(default 7) - The number of days for which you need usage for.
twitter: GetUserListMembershipsQueries
Represents the Queries record for the operation: getUserListMemberships
Fields
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- pagination_token PaginationTokenLong? - This parameter is used to get a specified 'page' of results.
- max_results Signed32(default 100) - The maximum number of results.
- list\.fields ("created_at"|"description"|"follower_count"|"id"|"member_count"|"name"|"owner_id"|"private")[]? - A comma separated list of List fields to display.
- expansions ("owner_id")[]? - A comma separated list of fields to expand.
twitter: GetUsersComplianceStreamQueries
Represents the Queries record for the operation: getUsersComplianceStream
Fields
- backfill_minutes Signed32? - The number of minutes of backfill requested.
- start_time string? - YYYY-MM-DDTHH:mm:ssZ. The earliest UTC timestamp from which the User Compliance events will be provided.
- partition Signed32 - The partition number.
- end_time string? - YYYY-MM-DDTHH:mm:ssZ. The latest UTC timestamp from which the User Compliance events will be provided.
twitter: GetUsersIdBookmarksQueries
Represents the Queries record for the operation: getUsersIdBookmarks
Fields
- poll\.fields ("duration_minutes"|"end_datetime"|"id"|"options"|"voting_status")[]? - A comma separated list of Poll fields to display.
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- pagination_token PaginationToken36? - This parameter is used to get the next 'page' of results.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- media\.fields ("alt_text"|"duration_ms"|"height"|"media_key"|"non_public_metrics"|"organic_metrics"|"preview_image_url"|"promoted_metrics"|"public_metrics"|"type"|"url"|"variants"|"width")[]? - A comma separated list of Media fields to display.
- max_results Signed32? - The maximum number of results.
- place\.fields ("contained_within"|"country"|"country_code"|"full_name"|"geo"|"id"|"name"|"place_type")[]? - A comma separated list of Place fields to display.
- expansions ("attachments.media_keys"|"attachments.media_source_tweet"|"attachments.poll_ids"|"author_id"|"edit_history_tweet_ids"|"entities.mentions.username"|"geo.place_id"|"in_reply_to_user_id"|"entities.note.mentions.username"|"referenced_tweets.id"|"referenced_tweets.id.author_id"|"author_screen_name")[]? - A comma separated list of fields to expand.
twitter: HashtagEntity
Fields
- Fields Included from *EntityIndicesInclusiveExclusive
- Fields Included from *HashtagFields
- tag string
- anydata...
twitter: HashtagFields
Represent the portion of text recognized as a Hashtag, and its start and end position within the text.
Fields
- tag string - The text of the Hashtag.
twitter: Like
A Like event, with the liking user and the tweet being liked
Fields
- created_at string? - Creation time of the Tweet.
- id LikeId? -
- liked_tweet_id TweetId? -
- liking_user_id UserId? -
- timestamp_ms Signed32? - Timestamp in milliseconds of creation.
twitter: LikeComplianceSchema
Fields
- delete UnlikeComplianceSchema -
twitter: LikesFirehoseStreamQueries
Represents the Queries record for the operation: likesFirehoseStream
Fields
- like\.fields ("created_at"|"id"|"liked_tweet_id"|"timestamp_ms")[]? - A comma separated list of Like fields to display.
- backfill_minutes Signed32? - The number of minutes of backfill requested.
- start_time string? - YYYY-MM-DDTHH:mm:ssZ. The earliest UTC timestamp to which the Likes will be provided.
- partition Signed32 - The partition number.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- end_time string? - YYYY-MM-DDTHH:mm:ssZ. The latest UTC timestamp to which the Posts will be provided.
- expansions ("liked_tweet_id")[]? - A comma separated list of fields to expand.
twitter: LikesSample10StreamQueries
Represents the Queries record for the operation: likesSample10Stream
Fields
- like\.fields ("created_at"|"id"|"liked_tweet_id"|"timestamp_ms")[]? - A comma separated list of Like fields to display.
- backfill_minutes Signed32? - The number of minutes of backfill requested.
- start_time string? - YYYY-MM-DDTHH:mm:ssZ. The earliest UTC timestamp to which the Likes will be provided.
- partition Signed32 - The partition number.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- end_time string? - YYYY-MM-DDTHH:mm:ssZ. The latest UTC timestamp to which the Posts will be provided.
- expansions ("liked_tweet_id")[]? - A comma separated list of fields to expand.
twitter: List
A X List is a curated group of accounts.
Fields
- created_at string? -
- description string? -
- follower_count int? -
- id ListId -
- member_count int? -
- name string - The name of this List.
- owner_id UserId? -
- 'private boolean? -
twitter: ListAddUserRequest
Fields
- user_id UserId -
twitter: ListBatchComplianceJobsQueries
Represents the Queries record for the operation: listBatchComplianceJobs
Fields
- compliance_job\.fields ("created_at"|"download_expires_at"|"download_url"|"id"|"name"|"resumable"|"status"|"type"|"upload_expires_at"|"upload_url")[]? - A comma separated list of ComplianceJob fields to display.
- 'type "tweets"|"users" - Type of Compliance Job to list.
- status "created"|"in_progress"|"failed"|"complete" ? - Status of Compliance Job to list.
twitter: ListCreateRequest
Fields
- description string? -
- name string -
- 'private boolean(default false) -
twitter: ListCreateResponse
Fields
- data ListCreateResponse_data? -
- errors Problem[]? -
twitter: ListCreateResponse_data
A X List is a curated group of accounts.
Fields
- id ListId -
- name string - The name of this List.
twitter: ListDeleteResponse
Fields
- data DeleteDmResponse_data? -
- errors Problem[]? -
twitter: ListFollowedRequest
Fields
- list_id ListId -
twitter: ListFollowedResponse
Fields
- data ListFollowedResponse_data? -
- errors Problem[]? -
twitter: ListFollowedResponse_data
Fields
- following boolean? -
twitter: ListGetFollowersQueries
Represents the Queries record for the operation: listGetFollowers
Fields
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- pagination_token PaginationTokenLong? - This parameter is used to get a specified 'page' of results.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- max_results Signed32(default 100) - The maximum number of results.
- expansions ("most_recent_tweet_id"|"pinned_tweet_id")[]? - A comma separated list of fields to expand.
twitter: ListGetMembersQueries
Represents the Queries record for the operation: listGetMembers
Fields
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- pagination_token PaginationTokenLong? - This parameter is used to get a specified 'page' of results.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- max_results Signed32(default 100) - The maximum number of results.
- expansions ("most_recent_tweet_id"|"pinned_tweet_id")[]? - A comma separated list of fields to expand.
twitter: ListIdGetQueries
Represents the Queries record for the operation: listIdGet
Fields
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- list\.fields ("created_at"|"description"|"follower_count"|"id"|"member_count"|"name"|"owner_id"|"private")[]? - A comma separated list of List fields to display.
- expansions ("owner_id")[]? - A comma separated list of fields to expand.
twitter: ListMutateResponse
Fields
- data ListMutateResponse_data? -
- errors Problem[]? -
twitter: ListMutateResponse_data
Fields
- is_member boolean? -
twitter: ListPinnedRequest
Fields
- list_id ListId -
twitter: ListPinnedResponse
Fields
- data ListPinnedResponse_data? -
- errors Problem[]? -
twitter: ListPinnedResponse_data
Fields
- pinned boolean? -
twitter: ListsIdTweetsQueries
Represents the Queries record for the operation: listsIdTweets
Fields
- poll\.fields ("duration_minutes"|"end_datetime"|"id"|"options"|"voting_status")[]? - A comma separated list of Poll fields to display.
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- pagination_token PaginationToken36? - This parameter is used to get the next 'page' of results.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- media\.fields ("alt_text"|"duration_ms"|"height"|"media_key"|"non_public_metrics"|"organic_metrics"|"preview_image_url"|"promoted_metrics"|"public_metrics"|"type"|"url"|"variants"|"width")[]? - A comma separated list of Media fields to display.
- max_results Signed32(default 100) - The maximum number of results.
- place\.fields ("contained_within"|"country"|"country_code"|"full_name"|"geo"|"id"|"name"|"place_type")[]? - A comma separated list of Place fields to display.
- expansions ("attachments.media_keys"|"attachments.media_source_tweet"|"attachments.poll_ids"|"author_id"|"edit_history_tweet_ids"|"entities.mentions.username"|"geo.place_id"|"in_reply_to_user_id"|"entities.note.mentions.username"|"referenced_tweets.id"|"referenced_tweets.id.author_id"|"author_screen_name")[]? - A comma separated list of fields to expand.
twitter: ListUnpinResponse
Fields
- data ListPinnedResponse_data? -
- errors Problem[]? -
twitter: ListUpdateRequest
Fields
- description string? -
- name string? -
- 'private boolean? -
twitter: ListUpdateResponse
Fields
- data ListUpdateResponse_data? -
- errors Problem[]? -
twitter: ListUpdateResponse_data
Fields
- updated boolean? -
twitter: ListUserOwnedListsQueries
Represents the Queries record for the operation: listUserOwnedLists
Fields
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- pagination_token PaginationTokenLong? - This parameter is used to get a specified 'page' of results.
- max_results Signed32(default 100) - The maximum number of results.
- list\.fields ("created_at"|"description"|"follower_count"|"id"|"member_count"|"name"|"owner_id"|"private")[]? - A comma separated list of List fields to display.
- expansions ("owner_id")[]? - A comma separated list of fields to expand.
twitter: ListUserPinnedListsQueries
Represents the Queries record for the operation: listUserPinnedLists
Fields
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- list\.fields ("created_at"|"description"|"follower_count"|"id"|"member_count"|"name"|"owner_id"|"private")[]? - A comma separated list of List fields to display.
- expansions ("owner_id")[]? - A comma separated list of fields to expand.
twitter: Media
Fields
- height MediaHeight? -
- media_key MediaKey? -
- 'type string -
- width MediaWidth? -
twitter: MentionEntity
Fields
- Fields Included from *EntityIndicesInclusiveExclusive
- Fields Included from *MentionFields
twitter: MentionFields
Represent the portion of text recognized as a User mention, and its start and end position within the text.
Fields
- id UserId? -
- username UserName -
twitter: MuteUserMutationResponse
Fields
- data MuteUserMutationResponse_data? -
- errors Problem[]? -
twitter: MuteUserMutationResponse_data
Fields
- muting boolean? -
twitter: MuteUserRequest
Fields
- target_user_id UserId -
twitter: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://api.twitter.com/2/oauth2/token") - Refresh URL
twitter: Place
Fields
- contained_within PlaceId[]? -
- country string? - The full name of the county in which this place exists.
- country_code CountryCode? -
- full_name string - The full name of this place.
- geo Geo? -
- id PlaceId -
- name string? - The human readable name of this place.
- place_type PlaceType? -
twitter: Point
A GeoJson Point geometry object.
Fields
- coordinates Position -
- 'type "Point" -
twitter: Poll
Represent a Poll attached to a Tweet.
Fields
- duration_minutes Signed32? -
- end_datetime string? -
- id PollId -
- options PollOption[] -
- voting_status "open"|"closed" ? -
twitter: PollOption
Describes a choice in a Poll object.
Fields
- label PollOptionLabel -
- position int - Position of this choice in the poll.
- votes int - Number of users who voted for this choice.
twitter: Problem
An HTTP Problem Details object, as defined in IETF RFC 7807 (https://tools.ietf.org/html/rfc7807).
Fields
- detail string? -
- status int? -
- title string -
- 'type string -
twitter: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
twitter: Rule
A user-provided stream filtering rule.
Fields
- id RuleId? -
- tag RuleTag? -
- value RuleValue -
twitter: RuleNoId
A user-provided stream filtering rule.
Fields
- tag RuleTag? -
- value RuleValue -
twitter: RulesCount
A count of user-provided stream filtering rules at the application and project levels.
Fields
- all_project_client_apps AllProjectClientApps? -
- cap_per_client_app Signed32? - Cap of number of rules allowed per client application
- cap_per_project Signed32? - Cap of number of rules allowed per project
- client_app_rules_count AppRulesCount? -
- project_rules_count Signed32? - Number of rules for project
twitter: RulesLookupResponse
Fields
- data Rule[]? -
- meta RulesResponseMetadata -
twitter: RulesResponseMetadata
Fields
- next_token NextToken? -
- result_count Signed32? - Number of Rules in result set.
- sent string -
- summary RulesRequestSummary? -
twitter: SampleStreamQueries
Represents the Queries record for the operation: sampleStream
Fields
- poll\.fields ("duration_minutes"|"end_datetime"|"id"|"options"|"voting_status")[]? - A comma separated list of Poll fields to display.
- backfill_minutes Signed32? - The number of minutes of backfill requested.
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- media\.fields ("alt_text"|"duration_ms"|"height"|"media_key"|"non_public_metrics"|"organic_metrics"|"preview_image_url"|"promoted_metrics"|"public_metrics"|"type"|"url"|"variants"|"width")[]? - A comma separated list of Media fields to display.
- place\.fields ("contained_within"|"country"|"country_code"|"full_name"|"geo"|"id"|"name"|"place_type")[]? - A comma separated list of Place fields to display.
- expansions ("attachments.media_keys"|"attachments.media_source_tweet"|"attachments.poll_ids"|"author_id"|"edit_history_tweet_ids"|"entities.mentions.username"|"geo.place_id"|"in_reply_to_user_id"|"entities.note.mentions.username"|"referenced_tweets.id"|"referenced_tweets.id.author_id"|"author_screen_name")[]? - A comma separated list of fields to expand.
twitter: SearchCount
Represent a Search Count Result.
Fields
- end End -
- 'start Start -
- tweet_count TweetCount -
twitter: SearchSpacesQueries
Represents the Queries record for the operation: searchSpaces
Fields
- space\.fields ("created_at"|"creator_id"|"ended_at"|"host_ids"|"id"|"invited_user_ids"|"is_ticketed"|"lang"|"participant_count"|"scheduled_start"|"speaker_ids"|"started_at"|"state"|"subscriber_count"|"title"|"topic_ids"|"updated_at")[]? - A comma separated list of Space fields to display.
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- query string - The search query.
- max_results Signed32(default 100) - The number of results to return.
- state "live"|"scheduled"|"all" (default "all") - The state of Spaces to search for.
- topic\.fields ("description"|"id"|"name")[]? - A comma separated list of Topic fields to display.
- expansions ("creator_id"|"host_ids"|"invited_user_ids"|"speaker_ids"|"topic_ids")[]? - A comma separated list of fields to expand.
twitter: SearchStreamQueries
Represents the Queries record for the operation: searchStream
Fields
- poll\.fields ("duration_minutes"|"end_datetime"|"id"|"options"|"voting_status")[]? - A comma separated list of Poll fields to display.
- backfill_minutes Signed32? - The number of minutes of backfill requested.
- start_time string? - YYYY-MM-DDTHH:mm:ssZ. The earliest UTC timestamp from which the Posts will be provided.
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- media\.fields ("alt_text"|"duration_ms"|"height"|"media_key"|"non_public_metrics"|"organic_metrics"|"preview_image_url"|"promoted_metrics"|"public_metrics"|"type"|"url"|"variants"|"width")[]? - A comma separated list of Media fields to display.
- end_time string? - YYYY-MM-DDTHH:mm:ssZ. The latest UTC timestamp to which the Posts will be provided.
- place\.fields ("contained_within"|"country"|"country_code"|"full_name"|"geo"|"id"|"name"|"place_type")[]? - A comma separated list of Place fields to display.
- expansions ("attachments.media_keys"|"attachments.media_source_tweet"|"attachments.poll_ids"|"author_id"|"edit_history_tweet_ids"|"entities.mentions.username"|"geo.place_id"|"in_reply_to_user_id"|"entities.note.mentions.username"|"referenced_tweets.id"|"referenced_tweets.id.author_id"|"author_screen_name")[]? - A comma separated list of fields to expand.
twitter: SearchUserByQueryQueries
Represents the Queries record for the operation: searchUserByQuery
Fields
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- query UserSearchQuery - TThe the query string by which to query for users.
- max_results Signed32(default 100) - The maximum number of results.
- next_token PaginationToken36? - This parameter is used to get the next 'page' of results. The value used with the parameter is pulled directly from the response provided by the API, and should not be modified.
- expansions ("most_recent_tweet_id"|"pinned_tweet_id")[]? - A comma separated list of fields to expand.
twitter: Space
Fields
- created_at string? - Creation time of the Space.
- creator_id UserId? -
- ended_at string? - End time of the Space.
- host_ids UserId[]? - The user ids for the hosts of the Space.
- id SpaceId -
- invited_user_ids UserId[]? - An array of user ids for people who were invited to a Space.
- is_ticketed boolean? - Denotes if the Space is a ticketed Space.
- lang string? - The language of the Space.
- participant_count Signed32? - The number of participants in a Space.
- scheduled_start string? - A date time stamp for when a Space is scheduled to begin.
- speaker_ids UserId[]? - An array of user ids for people who were speakers in a Space.
- started_at string? - When the Space was started as a date string.
- state "live"|"scheduled"|"ended" - The current state of the Space.
- subscriber_count Signed32? - The number of people who have either purchased a ticket or set a reminder for this Space.
- title string? - The title of the Space.
- topics Space_topics[]? - The topics of a Space, as selected by its creator.
- updated_at string? - When the Space was last updated.
twitter: Space_topics
The X Topic object.
Fields
- description string? - The description of the given topic.
- id string - An ID suitable for use in the REST API.
- name string - The name of the given topic.
twitter: SpaceBuyersQueries
Represents the Queries record for the operation: spaceBuyers
Fields
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- pagination_token PaginationToken32? - This parameter is used to get a specified 'page' of results.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- max_results Signed32(default 100) - The maximum number of results.
- expansions ("most_recent_tweet_id"|"pinned_tweet_id")[]? - A comma separated list of fields to expand.
twitter: SpaceTweetsQueries
Represents the Queries record for the operation: spaceTweets
Fields
- poll\.fields ("duration_minutes"|"end_datetime"|"id"|"options"|"voting_status")[]? - A comma separated list of Poll fields to display.
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- media\.fields ("alt_text"|"duration_ms"|"height"|"media_key"|"non_public_metrics"|"organic_metrics"|"preview_image_url"|"promoted_metrics"|"public_metrics"|"type"|"url"|"variants"|"width")[]? - A comma separated list of Media fields to display.
- max_results Signed32(default 100) - The number of Posts to fetch from the provided space. If not provided, the value will default to the maximum of 100.
- place\.fields ("contained_within"|"country"|"country_code"|"full_name"|"geo"|"id"|"name"|"place_type")[]? - A comma separated list of Place fields to display.
- expansions ("attachments.media_keys"|"attachments.media_source_tweet"|"attachments.poll_ids"|"author_id"|"edit_history_tweet_ids"|"entities.mentions.username"|"geo.place_id"|"in_reply_to_user_id"|"entities.note.mentions.username"|"referenced_tweets.id"|"referenced_tweets.id.author_id"|"author_screen_name")[]? - A comma separated list of fields to expand.
twitter: StreamingLikeResponse
Fields
- data Like? -
- errors Problem[]? -
- includes Expansions? -
twitter: StreamingTweetResponse
Fields
- data Tweet? -
- errors Problem[]? -
- includes Expansions? -
twitter: Topic
The topic of a Space, as selected by its creator.
Fields
- description string? - The description of the given topic.
- id TopicId -
- name string - The name of the given topic.
twitter: Trend
A trend.
Fields
- trend_name string? - Name of the trend.
- tweet_count Signed32? - Number of Posts in this trend.
twitter: Tweet
Fields
- attachments Tweet_attachments? -
- author_id UserId? -
- context_annotations ContextAnnotation[]? -
- conversation_id TweetId? -
- created_at string? - Creation time of the Tweet.
- edit_controls Tweet_edit_controls? -
- edit_history_tweet_ids TweetId[]? - A list of Tweet Ids in this Tweet chain.
- entities FullTextEntities? -
- geo Tweet_geo? -
- id TweetId? -
- in_reply_to_user_id UserId? -
- lang string? - Language of the Tweet, if detected by X. Returned as a BCP47 language tag.
- non_public_metrics Tweet_non_public_metrics? -
- note_tweet Tweet_note_tweet? -
- organic_metrics Tweet_organic_metrics? -
- possibly_sensitive boolean? - Indicates if this Tweet contains URLs marked as sensitive, for example content suitable for mature audiences.
- promoted_metrics Tweet_promoted_metrics? -
- public_metrics Tweet_public_metrics? -
- referenced_tweets Tweet_referenced_tweets[]? - A list of Posts this Tweet refers to. For example, if the parent Tweet is a Retweet, a Quoted Tweet or a Reply, it will include the related Tweet referenced to by its parent.
- reply_settings ReplySettingsWithVerifiedUsers? -
- scopes Tweet_scopes? -
- 'source string? - This is deprecated.
- text TweetText? -
- username UserName? -
- withheld TweetWithheld? -
twitter: Tweet_attachments
Specifies the type of attachments (if any) present in this Tweet.
Fields
- media_keys MediaKey[]? - A list of Media Keys for each one of the media attachments (if media are attached).
- media_source_tweet_id TweetId[]? - A list of Posts the media on this Tweet was originally posted in. For example, if the media on a tweet is re-used in another Tweet, this refers to the original, source Tweet..
- poll_ids PollId[]? - A list of poll IDs (if polls are attached).
twitter: Tweet_edit_controls
Fields
- editable_until string - Time when Tweet is no longer editable.
- edits_remaining int - Number of times this Tweet can be edited.
- is_edit_eligible boolean - Indicates if this Tweet is eligible to be edited.
twitter: Tweet_geo
The location tagged on the Tweet, if the user provided one.
Fields
- coordinates Point? -
- place_id PlaceId? -
twitter: Tweet_non_public_metrics
Nonpublic engagement metrics for the Tweet at the time of the request.
Fields
- impression_count Signed32? - Number of times this Tweet has been viewed.
twitter: Tweet_note_tweet
The full-content of the Tweet, including text beyond 280 characters.
Fields
- entities Tweet_note_tweet_entities? -
- text NoteTweetText? -
twitter: Tweet_note_tweet_entities
Fields
- cashtags CashtagEntity[]? -
- hashtags HashtagEntity[]? -
- mentions MentionEntity[]? -
- urls UrlEntity[]? -
twitter: Tweet_organic_metrics
Organic nonpublic engagement metrics for the Tweet at the time of the request.
Fields
- impression_count int - Number of times this Tweet has been viewed.
- like_count int - Number of times this Tweet has been liked.
- reply_count int - Number of times this Tweet has been replied to.
- retweet_count int - Number of times this Tweet has been Retweeted.
twitter: Tweet_promoted_metrics
Promoted nonpublic engagement metrics for the Tweet at the time of the request.
Fields
- impression_count Signed32? - Number of times this Tweet has been viewed.
- like_count Signed32? - Number of times this Tweet has been liked.
- reply_count Signed32? - Number of times this Tweet has been replied to.
- retweet_count Signed32? - Number of times this Tweet has been Retweeted.
twitter: Tweet_public_metrics
Engagement metrics for the Tweet at the time of the request.
Fields
- bookmark_count Signed32 - Number of times this Tweet has been bookmarked.
- impression_count Signed32 - Number of times this Tweet has been viewed.
- like_count int - Number of times this Tweet has been liked.
- quote_count int? - Number of times this Tweet has been quoted.
- reply_count int - Number of times this Tweet has been replied to.
- retweet_count int - Number of times this Tweet has been Retweeted.
twitter: Tweet_referenced_tweets
Fields
- id TweetId -
- 'type "retweeted"|"quoted"|"replied_to" -
twitter: Tweet_scopes
The scopes for this tweet
Fields
- followers boolean? - Indicates if this Tweet is viewable by followers without the Tweet ID
twitter: TweetComplianceSchema
Fields
- event_at string - Event time.
- quote_tweet_id TweetId? -
- tweet TweetComplianceSchema_tweet -
twitter: TweetComplianceSchema_tweet
Fields
- author_id UserId -
- id TweetId -
twitter: TweetCountsFullArchiveSearchQueries
Represents the Queries record for the operation: tweetCountsFullArchiveSearch
Fields
- start_time string? - YYYY-MM-DDTHH:mm:ssZ. The oldest UTC timestamp (from most recent 7 days) from which the Posts will be provided. Timestamp is in second granularity and is inclusive (i.e. 12:00:01 includes the first second of the minute).
- search_count\.fields ("end"|"start"|"tweet_count")[]? - A comma separated list of SearchCount fields to display.
- pagination_token PaginationToken36? - This parameter is used to get the next 'page' of results. The value used with the parameter is pulled directly from the response provided by the API, and should not be modified.
- granularity "minute"|"hour"|"day" (default "hour") - The granularity for the search counts results.
- query string - One query/rule/filter for matching Posts. Refer to https://t.co/rulelength to identify the max query length.
- end_time string? - YYYY-MM-DDTHH:mm:ssZ. The newest, most recent UTC timestamp to which the Posts will be provided. Timestamp is in second granularity and is exclusive (i.e. 12:00:01 excludes the first second of the minute).
- since_id TweetId? - Returns results with a Post ID greater than (that is, more recent than) the specified ID.
- next_token PaginationToken36? - This parameter is used to get the next 'page' of results. The value used with the parameter is pulled directly from the response provided by the API, and should not be modified.
- until_id TweetId? - Returns results with a Post ID less than (that is, older than) the specified ID.
twitter: TweetCountsRecentSearchQueries
Represents the Queries record for the operation: tweetCountsRecentSearch
Fields
- start_time string? - YYYY-MM-DDTHH:mm:ssZ. The oldest UTC timestamp (from most recent 7 days) from which the Posts will be provided. Timestamp is in second granularity and is inclusive (i.e. 12:00:01 includes the first second of the minute).
- search_count\.fields ("end"|"start"|"tweet_count")[]? - A comma separated list of SearchCount fields to display.
- pagination_token PaginationToken36? - This parameter is used to get the next 'page' of results. The value used with the parameter is pulled directly from the response provided by the API, and should not be modified.
- granularity "minute"|"hour"|"day" (default "hour") - The granularity for the search counts results.
- query string - One query/rule/filter for matching Posts. Refer to https://t.co/rulelength to identify the max query length.
- end_time string? - YYYY-MM-DDTHH:mm:ssZ. The newest, most recent UTC timestamp to which the Posts will be provided. Timestamp is in second granularity and is exclusive (i.e. 12:00:01 excludes the first second of the minute).
- since_id TweetId? - Returns results with a Post ID greater than (that is, more recent than) the specified ID.
- next_token PaginationToken36? - This parameter is used to get the next 'page' of results. The value used with the parameter is pulled directly from the response provided by the API, and should not be modified.
- until_id TweetId? - Returns results with a Post ID less than (that is, older than) the specified ID.
twitter: TweetCreateRequest
Fields
- card_uri string? - Card Uri Parameter. This is mutually exclusive from Quote Tweet Id, Poll, Media, and Direct Message Deep Link.
- direct_message_deep_link string? - Link to take the conversation from the public timeline to a private Direct Message.
- for_super_followers_only boolean(default false) - Exclusive Tweet for super followers.
- geo TweetCreateRequest_geo? -
- media TweetCreateRequest_media? -
- nullcast boolean(default false) - Nullcasted (promoted-only) Posts do not appear in the public timeline and are not served to followers.
- poll TweetCreateRequest_poll? -
- quote_tweet_id TweetId? -
- reply TweetCreateRequest_reply? -
- reply_settings "following"|"mentionedUsers"|"subscribers" ? - Settings to indicate who can reply to the Tweet.
- text TweetText? -
twitter: TweetCreateRequest_geo
Place ID being attached to the Tweet for geo location.
Fields
- place_id string? -
twitter: TweetCreateRequest_media
Media information being attached to created Tweet. This is mutually exclusive from Quote Tweet Id, Poll, and Card URI.
Fields
- media_ids MediaId[] - A list of Media Ids to be attached to a created Tweet.
- tagged_user_ids UserId[]? - A list of User Ids to be tagged in the media for created Tweet.
twitter: TweetCreateRequest_poll
Poll options for a Tweet with a poll. This is mutually exclusive from Media, Quote Tweet Id, and Card URI.
Fields
- duration_minutes Signed32 - Duration of the poll in minutes.
- options TweetCreateRequest_pollOptionsItemsString[] -
- reply_settings "following"|"mentionedUsers" ? - Settings to indicate who can reply to the Tweet.
twitter: TweetCreateRequest_reply
Tweet information of the Tweet being replied to.
Fields
- exclude_reply_user_ids UserId[]? - A list of User Ids to be excluded from the reply Tweet.
- in_reply_to_tweet_id TweetId -
twitter: TweetCreateResponse
Fields
- data TweetCreateResponse_data? -
- errors Problem[]? -
twitter: TweetCreateResponse_data
Fields
- id TweetId -
- text TweetText -
twitter: TweetDeleteComplianceSchema
Fields
- delete TweetComplianceSchema -
twitter: TweetDeleteResponse
Fields
- data TweetDeleteResponse_data? -
- errors Problem[]? -
twitter: TweetDeleteResponse_data
Fields
- deleted boolean -
twitter: TweetDropComplianceSchema
Fields
- drop TweetComplianceSchema -
twitter: TweetEditComplianceObjectSchema
Fields
- edit_tweet_ids TweetId[] -
- event_at string - Event time.
- initial_tweet_id TweetId -
- tweet DmEvent_referenced_tweets -
twitter: TweetEditComplianceSchema
Fields
- tweet_edit TweetEditComplianceObjectSchema -
twitter: TweetHideRequest
Fields
- hidden boolean -
twitter: TweetHideResponse
Fields
- data TweetHideResponse_data? -
twitter: TweetHideResponse_data
Fields
- hidden boolean? -
twitter: TweetNotice
Fields
- application string - If the label is being applied or removed. Possible values are ‘apply’ or ‘remove’.
- details string? - Information shown on the Tweet label
- event_at string - Event time.
- event_type string - The type of label on the Tweet
- extended_details_url string? - Link to more information about this kind of label
- label_title string? - Title/header of the Tweet label
- tweet TweetComplianceSchema_tweet -
twitter: TweetNoticeSchema
Fields
- public_tweet_notice TweetNotice -
twitter: TweetsFullarchiveSearchQueries
Represents the Queries record for the operation: tweetsFullarchiveSearch
Fields
- poll\.fields ("duration_minutes"|"end_datetime"|"id"|"options"|"voting_status")[]? - A comma separated list of Poll fields to display.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- query string - One query/rule/filter for matching Posts. Refer to https://t.co/rulelength to identify the max query length.
- end_time string? - YYYY-MM-DDTHH:mm:ssZ. The newest, most recent UTC timestamp to which the Posts will be provided. Timestamp is in second granularity and is exclusive (i.e. 12:00:01 excludes the first second of the minute).
- since_id TweetId? - Returns results with a Post ID greater than (that is, more recent than) the specified ID.
- next_token PaginationToken36? - This parameter is used to get the next 'page' of results. The value used with the parameter is pulled directly from the response provided by the API, and should not be modified.
- start_time string? - YYYY-MM-DDTHH:mm:ssZ. The oldest UTC timestamp from which the Posts will be provided. Timestamp is in second granularity and is inclusive (i.e. 12:00:01 includes the first second of the minute).
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- pagination_token PaginationToken36? - This parameter is used to get the next 'page' of results. The value used with the parameter is pulled directly from the response provided by the API, and should not be modified.
- media\.fields ("alt_text"|"duration_ms"|"height"|"media_key"|"non_public_metrics"|"organic_metrics"|"preview_image_url"|"promoted_metrics"|"public_metrics"|"type"|"url"|"variants"|"width")[]? - A comma separated list of Media fields to display.
- max_results Signed32(default 10) - The maximum number of search results to be returned by a request.
- place\.fields ("contained_within"|"country"|"country_code"|"full_name"|"geo"|"id"|"name"|"place_type")[]? - A comma separated list of Place fields to display.
- expansions ("attachments.media_keys"|"attachments.media_source_tweet"|"attachments.poll_ids"|"author_id"|"edit_history_tweet_ids"|"entities.mentions.username"|"geo.place_id"|"in_reply_to_user_id"|"entities.note.mentions.username"|"referenced_tweets.id"|"referenced_tweets.id.author_id"|"author_screen_name")[]? - A comma separated list of fields to expand.
- sort_order "recency"|"relevancy" ? - This order in which to return results.
- until_id TweetId? - Returns results with a Post ID less than (that is, older than) the specified ID.
twitter: TweetsIdLikingUsersQueries
Represents the Queries record for the operation: tweetsIdLikingUsers
Fields
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- pagination_token PaginationToken36? - This parameter is used to get the next 'page' of results.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- max_results Signed32(default 100) - The maximum number of results.
- expansions ("most_recent_tweet_id"|"pinned_tweet_id")[]? - A comma separated list of fields to expand.
twitter: TweetsIdRetweetingUsersQueries
Represents the Queries record for the operation: tweetsIdRetweetingUsers
Fields
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- pagination_token PaginationToken36? - This parameter is used to get the next 'page' of results.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- max_results Signed32(default 100) - The maximum number of results.
- expansions ("most_recent_tweet_id"|"pinned_tweet_id")[]? - A comma separated list of fields to expand.
twitter: TweetsRecentSearchQueries
Represents the Queries record for the operation: tweetsRecentSearch
Fields
- poll\.fields ("duration_minutes"|"end_datetime"|"id"|"options"|"voting_status")[]? - A comma separated list of Poll fields to display.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- query string - One query/rule/filter for matching Posts. Refer to https://t.co/rulelength to identify the max query length.
- end_time string? - YYYY-MM-DDTHH:mm:ssZ. The newest, most recent UTC timestamp to which the Posts will be provided. Timestamp is in second granularity and is exclusive (i.e. 12:00:01 excludes the first second of the minute).
- since_id TweetId? - Returns results with a Post ID greater than (that is, more recent than) the specified ID.
- next_token PaginationToken36? - This parameter is used to get the next 'page' of results. The value used with the parameter is pulled directly from the response provided by the API, and should not be modified.
- start_time string? - YYYY-MM-DDTHH:mm:ssZ. The oldest UTC timestamp from which the Posts will be provided. Timestamp is in second granularity and is inclusive (i.e. 12:00:01 includes the first second of the minute).
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- pagination_token PaginationToken36? - This parameter is used to get the next 'page' of results. The value used with the parameter is pulled directly from the response provided by the API, and should not be modified.
- media\.fields ("alt_text"|"duration_ms"|"height"|"media_key"|"non_public_metrics"|"organic_metrics"|"preview_image_url"|"promoted_metrics"|"public_metrics"|"type"|"url"|"variants"|"width")[]? - A comma separated list of Media fields to display.
- max_results Signed32(default 10) - The maximum number of search results to be returned by a request.
- place\.fields ("contained_within"|"country"|"country_code"|"full_name"|"geo"|"id"|"name"|"place_type")[]? - A comma separated list of Place fields to display.
- expansions ("attachments.media_keys"|"attachments.media_source_tweet"|"attachments.poll_ids"|"author_id"|"edit_history_tweet_ids"|"entities.mentions.username"|"geo.place_id"|"in_reply_to_user_id"|"entities.note.mentions.username"|"referenced_tweets.id"|"referenced_tweets.id.author_id"|"author_screen_name")[]? - A comma separated list of fields to expand.
- sort_order "recency"|"relevancy" ? - This order in which to return results.
- until_id TweetId? - Returns results with a Post ID less than (that is, older than) the specified ID.
twitter: TweetTakedownComplianceSchema
Fields
- event_at string - Event time.
- quote_tweet_id TweetId? -
- tweet TweetComplianceSchema_tweet -
- withheld_in_countries CountryCode[] -
twitter: TweetUndropComplianceSchema
Fields
- undrop TweetComplianceSchema -
twitter: TweetUnviewable
Fields
- application string - If the label is being applied or removed. Possible values are ‘apply’ or ‘remove’.
- event_at string - Event time.
- tweet TweetComplianceSchema_tweet -
twitter: TweetUnviewableSchema
Fields
- public_tweet_unviewable TweetUnviewable -
twitter: TweetWithheld
Indicates withholding details for withheld content.
Fields
- copyright boolean - Indicates if the content is being withheld for on the basis of copyright infringement.
- country_codes CountryCode[] - Provides a list of countries where this content is not available.
- scope "tweet"|"user" ? - Indicates whether the content being withheld is the
tweet
or auser
.
twitter: TweetWithheldComplianceSchema
Fields
- withheld TweetTakedownComplianceSchema -
twitter: UnlikeComplianceSchema
Fields
- event_at string - Event time.
- favorite UnlikeComplianceSchema_favorite -
twitter: UnlikeComplianceSchema_favorite
Fields
- id TweetId -
- user_id UserId -
twitter: UrlEntity
Represent the portion of text recognized as a URL, and its start and end position within the text.
Fields
- Fields Included from *EntityIndicesInclusiveExclusive
- Fields Included from *UrlFields
twitter: UrlEntityDm
Represent the portion of text recognized as a URL, and its start and end position within the text.
Fields
- Fields Included from *EntityIndicesInclusiveExclusive
- Fields Included from *UrlFields
twitter: UrlFields
Represent the portion of text recognized as a URL.
Fields
- description string? - Description of the URL landing page.
- display_url string? - The URL as displayed in the X client.
- expanded_url Url? -
- images UrlImage[]? -
- media_key MediaKey? -
- status HttpStatusCode? -
- title string? - Title of the page the URL points to.
- unwound_url string? - Fully resolved url.
- url Url -
twitter: UrlImage
Represent the information for the URL image.
Fields
- height MediaHeight? -
- url Url? -
- width MediaWidth? -
twitter: Usage
Usage per client app
Fields
- cap_reset_day Signed32? - Number of days left for the Tweet cap to reset
- daily_client_app_usage ClientAppUsage[]? - The daily usage breakdown for each Client Application a project
- daily_project_usage Usage_daily_project_usage? -
- project_cap Signed32? - Total number of Posts that can be read in this project per month
- project_id string? - The unique identifier for this project
- project_usage Signed32? - The number of Posts read in this project
twitter: Usage_daily_project_usage
The daily usage breakdown for a project
Fields
- project_id Signed32? - The unique identifier for this project
- usage UsageFields[]? - The usage value
twitter: UsageFields
Represents the data for Usage
Fields
- date string? - The time period for the usage
- usage Signed32? - The usage value
twitter: User
The X User object.
Fields
- connection_status ("follow_request_received"|"follow_request_sent"|"blocking"|"followed_by"|"following"|"muting")[]? - Returns detailed information about the relationship between two users.
- created_at string? - Creation time of this User.
- description string? - The text of this User's profile description (also known as bio), if the User provided one.
- entities User_entities? -
- id UserId -
- location string? - The location specified in the User's profile, if the User provided one. As this is a freeform value, it may not indicate a valid location, but it may be fuzzily evaluated when performing searches with location queries.
- most_recent_tweet_id TweetId? -
- name string - The friendly name of this User, as shown on their profile.
- pinned_tweet_id TweetId? -
- profile_image_url string? - The URL to the profile image for this User.
- protected boolean? - Indicates if this User has chosen to protect their Posts (in other words, if this User's Posts are private).
- public_metrics User_public_metrics? -
- receives_your_dm boolean? - Indicates if you can send a DM to this User
- subscription_type "Basic"|"Premium"|"PremiumPlus"|"None" ? - The X Blue subscription type of the user, eg: Basic, Premium, PremiumPlus or None.
- url string? - The URL specified in the User's profile.
- username UserName -
- verified boolean? - Indicate if this User is a verified X User.
- verified_type "blue"|"government"|"business"|"none" ? - The X Blue verified type of the user, eg: blue, government, business or none.
- withheld UserWithheld? -
twitter: User_entities
A list of metadata found in the User's profile description.
Fields
- description FullTextEntities? -
- url User_entities_url? -
twitter: User_entities_url
Expanded details for the URL specified in the User's profile, with start and end indices.
Fields
- urls UrlEntity[]? -
twitter: User_public_metrics
A list of metrics for this User.
Fields
- followers_count int - Number of Users who are following this User.
- following_count int - Number of Users this User is following.
- like_count int? - The number of likes created by this User.
- listed_count int - The number of lists that include this User.
- tweet_count int - The number of Posts (including Retweets) posted by this User.
twitter: UserComplianceSchema
Fields
- event_at string - Event time.
- user UserComplianceSchema_user -
twitter: UserComplianceSchema_user
Fields
- id UserId -
twitter: UserDeleteComplianceSchema
Fields
- user_delete UserComplianceSchema -
twitter: UserFollowedListsQueries
Represents the Queries record for the operation: userFollowedLists
Fields
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- pagination_token PaginationTokenLong? - This parameter is used to get a specified 'page' of results.
- max_results Signed32(default 100) - The maximum number of results.
- list\.fields ("created_at"|"description"|"follower_count"|"id"|"member_count"|"name"|"owner_id"|"private")[]? - A comma separated list of List fields to display.
- expansions ("owner_id")[]? - A comma separated list of fields to expand.
twitter: UserProfileModificationComplianceSchema
Fields
- user_profile_modification UserProfileModificationObjectSchema -
twitter: UserProfileModificationObjectSchema
Fields
- event_at string - Event time.
- new_value string -
- profile_field string -
- user UserComplianceSchema_user -
twitter: UserProtectComplianceSchema
Fields
- user_protect UserComplianceSchema -
twitter: UserScrubGeoObjectSchema
Fields
- event_at string - Event time.
- up_to_tweet_id TweetId -
- user UserComplianceSchema_user -
twitter: UserScrubGeoSchema
Fields
- scrub_geo UserScrubGeoObjectSchema -
twitter: UsersFollowingCreateRequest
Fields
- target_user_id UserId -
twitter: UsersFollowingCreateResponse
Fields
- errors Problem[]? -
twitter: UsersFollowingCreateResponse_data
Fields
- following boolean? -
- pending_follow boolean? -
twitter: UsersFollowingDeleteResponse
Fields
- data ListFollowedResponse_data? -
- errors Problem[]? -
twitter: UsersIdBlockingQueries
Represents the Queries record for the operation: usersIdBlocking
Fields
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- pagination_token PaginationToken32? - This parameter is used to get a specified 'page' of results.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- max_results Signed32? - The maximum number of results.
- expansions ("most_recent_tweet_id"|"pinned_tweet_id")[]? - A comma separated list of fields to expand.
twitter: UsersIdFollowersQueries
Represents the Queries record for the operation: usersIdFollowers
Fields
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- pagination_token PaginationToken32? - This parameter is used to get a specified 'page' of results.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- max_results Signed32? - The maximum number of results.
- expansions ("most_recent_tweet_id"|"pinned_tweet_id")[]? - A comma separated list of fields to expand.
twitter: UsersIdFollowingQueries
Represents the Queries record for the operation: usersIdFollowing
Fields
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- pagination_token PaginationToken32? - This parameter is used to get a specified 'page' of results.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- max_results Signed32? - The maximum number of results.
- expansions ("most_recent_tweet_id"|"pinned_tweet_id")[]? - A comma separated list of fields to expand.
twitter: UsersIdLikedTweetsQueries
Represents the Queries record for the operation: usersIdLikedTweets
Fields
- poll\.fields ("duration_minutes"|"end_datetime"|"id"|"options"|"voting_status")[]? - A comma separated list of Poll fields to display.
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- pagination_token PaginationToken36? - This parameter is used to get the next 'page' of results.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- media\.fields ("alt_text"|"duration_ms"|"height"|"media_key"|"non_public_metrics"|"organic_metrics"|"preview_image_url"|"promoted_metrics"|"public_metrics"|"type"|"url"|"variants"|"width")[]? - A comma separated list of Media fields to display.
- max_results Signed32? - The maximum number of results.
- place\.fields ("contained_within"|"country"|"country_code"|"full_name"|"geo"|"id"|"name"|"place_type")[]? - A comma separated list of Place fields to display.
- expansions ("attachments.media_keys"|"attachments.media_source_tweet"|"attachments.poll_ids"|"author_id"|"edit_history_tweet_ids"|"entities.mentions.username"|"geo.place_id"|"in_reply_to_user_id"|"entities.note.mentions.username"|"referenced_tweets.id"|"referenced_tweets.id.author_id"|"author_screen_name")[]? - A comma separated list of fields to expand.
twitter: UsersIdMentionsQueries
Represents the Queries record for the operation: usersIdMentions
Fields
- poll\.fields ("duration_minutes"|"end_datetime"|"id"|"options"|"voting_status")[]? - A comma separated list of Poll fields to display.
- start_time string? - YYYY-MM-DDTHH:mm:ssZ. The earliest UTC timestamp from which the Posts will be provided. The since_id parameter takes precedence if it is also specified.
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- pagination_token PaginationToken36? - This parameter is used to get the next 'page' of results.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- media\.fields ("alt_text"|"duration_ms"|"height"|"media_key"|"non_public_metrics"|"organic_metrics"|"preview_image_url"|"promoted_metrics"|"public_metrics"|"type"|"url"|"variants"|"width")[]? - A comma separated list of Media fields to display.
- end_time string? - YYYY-MM-DDTHH:mm:ssZ. The latest UTC timestamp to which the Posts will be provided. The until_id parameter takes precedence if it is also specified.
- max_results Signed32? - The maximum number of results.
- place\.fields ("contained_within"|"country"|"country_code"|"full_name"|"geo"|"id"|"name"|"place_type")[]? - A comma separated list of Place fields to display.
- since_id TweetId? - The minimum Post ID to be included in the result set. This parameter takes precedence over start_time if both are specified.
- expansions ("attachments.media_keys"|"attachments.media_source_tweet"|"attachments.poll_ids"|"author_id"|"edit_history_tweet_ids"|"entities.mentions.username"|"geo.place_id"|"in_reply_to_user_id"|"entities.note.mentions.username"|"referenced_tweets.id"|"referenced_tweets.id.author_id"|"author_screen_name")[]? - A comma separated list of fields to expand.
- until_id TweetId? - The maximum Post ID to be included in the result set. This parameter takes precedence over end_time if both are specified.
twitter: UsersIdMutingQueries
Represents the Queries record for the operation: usersIdMuting
Fields
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- pagination_token PaginationTokenLong? - This parameter is used to get the next 'page' of results.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- max_results Signed32(default 100) - The maximum number of results.
- expansions ("most_recent_tweet_id"|"pinned_tweet_id")[]? - A comma separated list of fields to expand.
twitter: UsersIdTimelineQueries
Represents the Queries record for the operation: usersIdTimeline
Fields
- poll\.fields ("duration_minutes"|"end_datetime"|"id"|"options"|"voting_status")[]? - A comma separated list of Poll fields to display.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- end_time string? - YYYY-MM-DDTHH:mm:ssZ. The latest UTC timestamp to which the Posts will be provided. The until_id parameter takes precedence if it is also specified.
- since_id TweetId? - The minimum Post ID to be included in the result set. This parameter takes precedence over start_time if both are specified.
- start_time string? - YYYY-MM-DDTHH:mm:ssZ. The earliest UTC timestamp from which the Posts will be provided. The since_id parameter takes precedence if it is also specified.
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- pagination_token PaginationToken36? - This parameter is used to get the next 'page' of results.
- media\.fields ("alt_text"|"duration_ms"|"height"|"media_key"|"non_public_metrics"|"organic_metrics"|"preview_image_url"|"promoted_metrics"|"public_metrics"|"type"|"url"|"variants"|"width")[]? - A comma separated list of Media fields to display.
- max_results Signed32? - The maximum number of results.
- place\.fields ("contained_within"|"country"|"country_code"|"full_name"|"geo"|"id"|"name"|"place_type")[]? - A comma separated list of Place fields to display.
- exclude ("replies"|"retweets")[]? - The set of entities to exclude (e.g. 'replies' or 'retweets').
- expansions ("attachments.media_keys"|"attachments.media_source_tweet"|"attachments.poll_ids"|"author_id"|"edit_history_tweet_ids"|"entities.mentions.username"|"geo.place_id"|"in_reply_to_user_id"|"entities.note.mentions.username"|"referenced_tweets.id"|"referenced_tweets.id.author_id"|"author_screen_name")[]? - A comma separated list of fields to expand.
- until_id TweetId? - The maximum Post ID to be included in the result set. This parameter takes precedence over end_time if both are specified.
twitter: UsersIdTweetsQueries
Represents the Queries record for the operation: usersIdTweets
Fields
- poll\.fields ("duration_minutes"|"end_datetime"|"id"|"options"|"voting_status")[]? - A comma separated list of Poll fields to display.
- tweet\.fields ("attachments"|"author_id"|"card_uri"|"context_annotations"|"conversation_id"|"created_at"|"edit_controls"|"edit_history_tweet_ids"|"entities"|"geo"|"id"|"in_reply_to_user_id"|"lang"|"non_public_metrics"|"note_tweet"|"organic_metrics"|"possibly_sensitive"|"promoted_metrics"|"public_metrics"|"referenced_tweets"|"reply_settings"|"scopes"|"source"|"text"|"username"|"withheld")[]? - A comma separated list of Tweet fields to display.
- end_time string? - YYYY-MM-DDTHH:mm:ssZ. The latest UTC timestamp to which the Posts will be provided. The until_id parameter takes precedence if it is also specified.
- since_id TweetId? - The minimum Post ID to be included in the result set. This parameter takes precedence over start_time if both are specified.
- start_time string? - YYYY-MM-DDTHH:mm:ssZ. The earliest UTC timestamp from which the Posts will be provided. The since_id parameter takes precedence if it is also specified.
- user\.fields ("connection_status"|"created_at"|"description"|"entities"|"id"|"location"|"most_recent_tweet_id"|"name"|"pinned_tweet_id"|"profile_image_url"|"protected"|"public_metrics"|"receives_your_dm"|"subscription_type"|"url"|"username"|"verified"|"verified_type"|"withheld")[]? - A comma separated list of User fields to display.
- pagination_token PaginationToken36? - This parameter is used to get the next 'page' of results.
- media\.fields ("alt_text"|"duration_ms"|"height"|"media_key"|"non_public_metrics"|"organic_metrics"|"preview_image_url"|"promoted_metrics"|"public_metrics"|"type"|"url"|"variants"|"width")[]? - A comma separated list of Media fields to display.
- max_results Signed32? - The maximum number of results.
- place\.fields ("contained_within"|"country"|"country_code"|"full_name"|"geo"|"id"|"name"|"place_type")[]? - A comma separated list of Place fields to display.
- exclude ("replies"|"retweets")[]? - The set of entities to exclude (e.g. 'replies' or 'retweets').
- expansions ("attachments.media_keys"|"attachments.media_source_tweet"|"attachments.poll_ids"|"author_id"|"edit_history_tweet_ids"|"entities.mentions.username"|"geo.place_id"|"in_reply_to_user_id"|"entities.note.mentions.username"|"referenced_tweets.id"|"referenced_tweets.id.author_id"|"author_screen_name")[]? - A comma separated list of fields to expand.
- until_id TweetId? - The maximum Post ID to be included in the result set. This parameter takes precedence over end_time if both are specified.
twitter: UsersLikesCreateRequest
Fields
- tweet_id TweetId -
twitter: UsersLikesCreateResponse
Fields
- data UsersLikesCreateResponse_data? -
- errors Problem[]? -
twitter: UsersLikesCreateResponse_data
Fields
- liked boolean? -
twitter: UsersLikesDeleteResponse
Fields
- data UsersLikesCreateResponse_data? -
- errors Problem[]? -
twitter: UsersRetweetsCreateRequest
Fields
- tweet_id TweetId -
twitter: UsersRetweetsCreateResponse
Fields
- data UsersRetweetsCreateResponse_data? -
- errors Problem[]? -
twitter: UsersRetweetsCreateResponse_data
Fields
- id TweetId? -
- retweeted boolean? -
twitter: UsersRetweetsDeleteResponse
Fields
- data UsersRetweetsDeleteResponse_data? -
- errors Problem[]? -
twitter: UsersRetweetsDeleteResponse_data
Fields
- retweeted boolean? -
twitter: UserSuspendComplianceSchema
Fields
- user_suspend UserComplianceSchema -
twitter: UserTakedownComplianceSchema
Fields
- event_at string - Event time.
- user UserComplianceSchema_user -
- withheld_in_countries CountryCode[] -
twitter: UserUndeleteComplianceSchema
Fields
- user_undelete UserComplianceSchema -
twitter: UserUnprotectComplianceSchema
Fields
- user_unprotect UserComplianceSchema -
twitter: UserUnsuspendComplianceSchema
Fields
- user_unsuspend UserComplianceSchema -
twitter: UserWithheld
Indicates withholding details for withheld content.
Fields
- country_codes CountryCode[] - Provides a list of countries where this content is not available.
- scope "user" ? - Indicates that the content being withheld is a
user
.
twitter: UserWithheldComplianceSchema
Fields
- user_withheld UserTakedownComplianceSchema -
Union types
twitter: TweetLabelData
TweetLabelData
Tweet label data.
twitter: UserComplianceStreamResponse
UserComplianceStreamResponse
User compliance stream events.
twitter: PlaceType
PlaceType
twitter: ComplianceJobType
ComplianceJobType
Type of compliance job to list.
twitter: AddOrDeleteRulesRequest
AddOrDeleteRulesRequest
twitter: TweetComplianceStreamResponse
TweetComplianceStreamResponse
Tweet compliance stream events.
twitter: TweetComplianceData
TweetComplianceData
Tweet compliance data.
twitter: RulesRequestSummary
RulesRequestSummary
twitter: UserComplianceData
UserComplianceData
User compliance data.
twitter: ReplySettingsWithVerifiedUsers
ReplySettingsWithVerifiedUsers
Shows who can reply a Tweet. Fields returned are everyone, mentioned_users, subscribers, verified and following.
twitter: LikesComplianceStreamResponse
LikesComplianceStreamResponse
Likes compliance stream events.
twitter: CreateMessageRequest
CreateMessageRequest
twitter: ComplianceJobStatus
ComplianceJobStatus
Status of a compliance job.
twitter: TweetLabelStreamResponse
TweetLabelStreamResponse
Tweet label stream events.
Array types
twitter: AllProjectClientApps
AllProjectClientApps
Client App Rule Counts for all applications in the project
twitter: DmParticipants
DmParticipants
Participants for the DM Conversation.
twitter: Position
Position
A GeoJson Position in the format [longitude,latitude]
.
twitter: DmAttachments
DmAttachments
Attachments to a DM Event.
String types
twitter: PreviousToken
PreviousToken
The previous token.
twitter: CreatedAt
CreatedAt
Creation time of the compliance job.
twitter: DmEventId
DmEventId
Unique identifier of a DM Event.
twitter: UserIdMatchesAuthenticatedUser
UserIdMatchesAuthenticatedUser
Unique identifier of this User. The value must be the same as the authenticated user.
twitter: ComplianceJobName
ComplianceJobName
User-provided name for a compliance job.
twitter: Start
Start
The start time of the bucket.
twitter: PaginationToken32
PaginationToken32
A base32 pagination token.
twitter: ClientAppId
ClientAppId
The ID of the client application
twitter: LikeId
LikeId
The unique identifier of this Like.
twitter: DownloadUrl
DownloadUrl
URL from which the user will retrieve their compliance results.
twitter: TweetCreateRequest_pollOptionsItemsString
TweetCreateRequest_pollOptionsItemsString
twitter: MediaId
MediaId
The unique identifier of this Media.
twitter: End
End
The end time of the bucket.
twitter: UploadUrl
UploadUrl
URL to which the user will upload their Tweet or user IDs.
twitter: TweetText
TweetText
The content of the Tweet.
twitter: PollOptionLabel
PollOptionLabel
The text of a poll choice.
twitter: UserSearchQuery
UserSearchQuery
The the search string by which to query for users.
twitter: UserName
UserName
The X handle (screen name) of this user.
twitter: FindSpacesByIdsQueriesIdsItemsString
FindSpacesByIdsQueriesIdsItemsString
twitter: TweetId
TweetId
Unique identifier of this Tweet. This is returned as a string in order to avoid complications with languages and tools that cannot handle large integers.
twitter: DownloadExpiration
DownloadExpiration
Expiration time of the download URL.
twitter: NoteTweetText
NoteTweetText
The note content of the Tweet.
twitter: Url
Url
A validly formatted URL.
twitter: RuleTag
RuleTag
A tag meant for the labeling of user provided rules.
twitter: PaginationToken36
PaginationToken36
A base36 pagination token.
twitter: FindUsersByUsernameQueriesUsernamesItemsString
FindUsersByUsernameQueriesUsernamesItemsString
twitter: NewestId
NewestId
The newest id in this response.
twitter: PollId
PollId
Unique identifier of this poll.
twitter: RuleValue
RuleValue
The filterlang value of the rule.
twitter: DmConversationId
DmConversationId
Unique identifier of a DM conversation. This can either be a numeric string, or a pair of numeric strings separated by a '-' character in the case of one-on-one DM Conversations.
twitter: OldestId
OldestId
The oldest id in this response.
twitter: PlaceId
PlaceId
The identifier for this place.
twitter: PaginationTokenLong
PaginationTokenLong
A 'long' pagination token.
twitter: NextToken
NextToken
The next token.
twitter: RuleId
RuleId
Unique identifier of this rule.
twitter: MediaKey
MediaKey
The Media Key identifier for this attachment.
twitter: ListId
ListId
The unique identifier of this List.
twitter: TopicId
TopicId
Unique identifier of this Topic.
twitter: CountryCode
CountryCode
A two-letter ISO 3166-1 alpha-2 country code.
twitter: JobId
JobId
Compliance Job ID.
twitter: SpaceId
SpaceId
The unique identifier of this Space.
twitter: UploadExpiration
UploadExpiration
Expiration time of the upload URL.
twitter: UserId
UserId
Unique identifier of this User. This is returned as a string in order to avoid complications with languages and tools that cannot handle large integers.
Integer types
Decimal types
twitter: GeoBboxItemsNumber
GeoBboxItemsNumber
Simple name reference types
Import
import ballerinax/twitter;
Metadata
Released date: 5 months ago
Version: 4.0.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.9.0
GraalVM compatible: Yes
Pull count
Total: 95540
Current verison: 8050
Weekly downloads
Keywords
Marketing/Social Media Accounts
Cost/Freemium
Contributors