thinkific
Module thinkific
API
Definitions
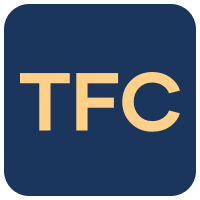
ballerinax/thinkific Ballerina library
Overview
This is the generated connector for Thinkific API v1 OpenAPI specification.
The Thinkific APIs allow developers to extend Thinkific's functionality in a variety of different ways by accessing site data.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
Clients
thinkific: Client
Thinkific's public API can be used to integrate your application with your Thinkific site.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
For private apps login to your Thinkific account and obtain API Key and Subdomain using the guide here
For public apps create a Thinkific Partner Account and register an App to access the credentials. Follow the guide here for more details.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.thinkific.com/api/public/v1" - URL of the target service
getBundleByID
function getBundleByID(decimal id) returns BundleResponse|error
List a bundle
Parameters
- id decimal - ID of the Bundle in the form of an integer
Return Type
- BundleResponse|error - Bundle Response
listCoursesByBundleId
function listCoursesByBundleId(decimal id, decimal page, decimal 'limit) returns GetCoursesResponse|error
List Courses by bundle ID
Parameters
- id decimal - ID of the Bundle in the form of an integer
- page decimal (default 1.0) - The page within the collection to fetch
- 'limit decimal (default 25.0) - The number of items to be returned
Return Type
- GetCoursesResponse|error - Courses Response
getBundleEnrollments
function getBundleEnrollments(decimal id, decimal page, decimal 'limit, decimal? queryUserId, string? queryEmail, string? queryCompleted, string? queryExpired, string? queryCreatedAfter, string? queryCreatedBefore, string? queryCreatedOn, string? queryCreatedOnOrAfter, string? queryCreatedOnOrBefore, string? queryUpdatedAfter, string? queryUpdatedBefore, string? queryUpdatedOn, string? queryUpdatedOnOrAfter, string? queryUpdatedOnOrBefore) returns GetEnrollmentsResponse|error
List a enrollments in a bundle
Parameters
- id decimal - ID of the Bundle in the form of an integer
- page decimal (default 1.0) - The page within the collection to fetch
- 'limit decimal (default 25.0) - The number of items to be returned
- queryUserId decimal? (default ()) - Search Bundle Enrollments by User ID.
- queryEmail string? (default ()) - Search Bundle Enrollments by User email
- queryCompleted string? (default ()) - Filter for only completed Bundle Enrollments when set to true.
- queryExpired string? (default ()) - Filter for only expired Bundle Enrollments when set to true.
- queryCreatedAfter string? (default ()) - Filter for only Enrollments created after the date specified.
- queryCreatedBefore string? (default ()) - Filter for only Enrollments created before the date specified.
- queryCreatedOn string? (default ()) - Filter for only Enrollments created on the date specified.
- queryCreatedOnOrAfter string? (default ()) - Filter for only Enrollments created on or after the date specified.
- queryCreatedOnOrBefore string? (default ()) - Filter for only Enrollments created on or before the date specified.
- queryUpdatedAfter string? (default ()) - Filter for only Enrollments updated after the date specified.
- queryUpdatedBefore string? (default ()) - Filter for only Enrollments updated before the date specified.
- queryUpdatedOn string? (default ()) - Filter for only Enrollments updated on the date specified.
- queryUpdatedOnOrAfter string? (default ()) - Filter for only Enrollments created on or after the date specified.
- queryUpdatedOnOrBefore string? (default ()) - Filter for only Enrollments updated on or before the date specified.
Return Type
- GetEnrollmentsResponse|error - Bundle Response
updateEnrollmentsInBundle
function updateEnrollmentsInBundle(decimal id, IdEnrollmentsBody payload) returns Response|error
Update Enrollments in a Bundle
Parameters
- id decimal - ID of the bundle in a form of integer
- payload IdEnrollmentsBody - New enrollment attributes
createEnrollmentInBundle
function createEnrollmentInBundle(decimal id, IdEnrollmentsBody1 payload) returns Response|error
Create an Enrollment in a Bundle of Courses
Parameters
- id decimal - ID of the bundle in a form of integer
- payload IdEnrollmentsBody1 - New enrollment attributes
getChapterByID
function getChapterByID(decimal id) returns ChapterResponse|error
Get Chapters
Parameters
- id decimal - ID of the Chapter in the form of an integer.
Return Type
- ChapterResponse|error - Chapters response
getContentsByID
function getContentsByID(decimal id, decimal page, decimal 'limit) returns GetContentsResponse|error
Get Contents by Chapter id
Parameters
- id decimal - ID of the Chapter in the form of an integer
- page decimal (default 1.0) - The page within the collection to fetch.
- 'limit decimal (default 25.0) - The number of items to be returned.
Return Type
- GetContentsResponse|error - Chapters response
getCollections
function getCollections(decimal page, decimal 'limit) returns GetCollectionsResponse|error
Get Collections
Parameters
- page decimal (default 1.0) - The page within the collection to fetch
- 'limit decimal (default 25.0) - The number of items to be returned
Return Type
- GetCollectionsResponse|error - Collections response
crateCollection
function crateCollection(CollectionRequest payload) returns CollectionResponse|error
Create a new Category
Parameters
- payload CollectionRequest - New collections attributes
Return Type
- CollectionResponse|error - Collection response
getCollectionbyID
function getCollectionbyID(decimal id) returns CollectionResponse|error
Get Collections by Id
Parameters
- id decimal - ID of the Category in the form of an integer
Return Type
- CollectionResponse|error - Collection response
updateCollectioByID
function updateCollectioByID(decimal id, CollectionRequest payload) returns Response|error
Update collection by ID
Parameters
- id decimal - ID of the Category in the form of an integer
- payload CollectionRequest - Collections attributes
deleteCollectionByID
Delete collection by ID
Parameters
- id decimal - ID of the Category in the form of an integer
getProductsbyID
function getProductsbyID(decimal id, decimal page, decimal 'limit) returns GetProductsResponse|error
Get products by Collections id
Parameters
- id decimal - ID of the Chapter in the form of an integer
- page decimal (default 1.0) - The page within the collection to fetch
- 'limit decimal (default 25.0) - The number of items to be returned
Return Type
- GetProductsResponse|error - Products response
getCoupons
function getCoupons(decimal promotionId, decimal page, decimal 'limit) returns GetCouponResponse|error
Get Coupons
Parameters
- promotionId decimal - The ID of the Promotion for which to get the Coupons in the form of an integer.
- page decimal (default 1.0) - The page within the collection to fetch.
- 'limit decimal (default 25.0) - The number of items to be returned
Return Type
- GetCouponResponse|error - Coupon Response
createCoupon
function createCoupon(decimal promotionId, CreateCouponRequest payload) returns CouponResponse|error
Create a Coupon
Parameters
- promotionId decimal - ID of the Promotion to add the Coupon to in the form of an integer.
- payload CreateCouponRequest - New Coupon attributes
Return Type
- CouponResponse|error - Create Coupon Response
bulkCreateCoupons
function bulkCreateCoupons(decimal promotionId, BulkCreateCouponRequest payload) returns CreateBulkCouponResponse|error
Bulk Create Coupons
Parameters
- promotionId decimal - The ID of the Promotion for which to bulk create the Coupons.
- payload BulkCreateCouponRequest - New Coupon attributes
Return Type
- CreateBulkCouponResponse|error - Create Coupon Response
getCouponByID
function getCouponByID(decimal id) returns CouponResponse|error
Get a Coupon by ID
Parameters
- id decimal - The ID of the Coupon in the form of an integer.
Return Type
- CouponResponse|error - Coupon Response
updateCoupon
function updateCoupon(decimal id, UpdateCoupon payload) returns Response|error
Update a Coupon
Parameters
- id decimal - The ID of the Coupon in the form of an integer.
- payload UpdateCoupon - Update an existing coupon
deleteCouponByID
Delete Coupon by ID
Parameters
- id decimal - The ID of the Coupon in the form of an integer.
getContentByID
function getContentByID(decimal id) returns ContentResponse|error
Get Contents by Id
Parameters
- id decimal - ID of the Content in the form of an integer
Return Type
- ContentResponse|error - Content response
getCourses
function getCourses(decimal page, decimal 'limit) returns GetCoursesResponse|error
Get Courses
Parameters
- page decimal (default 1.0) - The page within the collection to fetch.
- 'limit decimal (default 25.0) - The number of items to be returned
Return Type
- GetCoursesResponse|error - Courses Response
getCourseByID
function getCourseByID(decimal id) returns CourseResponse|error
Get courses by ID
Parameters
- id decimal - ID of the Course in the form of an integer.
Return Type
- CourseResponse|error - Courses response
getChapterOfCourseByID
function getChapterOfCourseByID(decimal id, decimal page, decimal 'limit) returns GetChaptersResponse|error
Get Chapters by Course ID
Parameters
- id decimal - ID of the Chapter in the form of an integer.
- page decimal (default 1.0) - The page within the collection to fetch.
- 'limit decimal (default 25.0) - The number of items to be returned.
Return Type
- GetChaptersResponse|error - Chapter of a course response
getCustomProfileFields
function getCustomProfileFields(decimal page, decimal 'limit) returns GetCustomProfileFieldDefinitions|error
Get Custom profile field definition
Parameters
- page decimal (default 1.0) - The page within the collection to fetch.
- 'limit decimal (default 25.0) - The number of items to be returned.
Return Type
- GetCustomProfileFieldDefinitions|error - Custom Profile Field Definitions
getEnrollments
function getEnrollments(decimal page, decimal 'limit, decimal? queryUserId, decimal? queryCourseId, string? queryEmail, boolean? queryFreeTrial, boolean? queryFull, boolean? queryCompleted, boolean? queryExpired, string? queryCreatedOn, string? queryCreatedBefore, string? queryCreatedOnOrBefore, string? queryCreatedAfter, string? queryCreatedOnOrAfter, string? queryUpdatedOn, string? queryUpdatedBefore, string? queryUpdatedOnOrBefore, string? queryUpdatedAfter) returns GetEnrollmentsResponse|error
Get Enrollments
Parameters
- page decimal (default 1.0) - The page within the collection to fetch.
- 'limit decimal (default 25.0) - The number of items to be returned.
- queryUserId decimal? (default ()) - Search Enrollments by User ID.
- queryCourseId decimal? (default ()) - Search Enrollments by Course ID.
- queryEmail string? (default ()) - Search Enrollments by User email.
- queryFreeTrial boolean? (default ()) - Filter for only Free Trial Enrollments when set to true.
- queryFull boolean? (default ()) - Filter for only full Enrollments when set to true.
- queryCompleted boolean? (default ()) - Filter for only completed Enrollments when set to true.
- queryExpired boolean? (default ()) - Filter for only expired Enrollments when set to true.
- queryCreatedOn string? (default ()) - Filter for only Enrollments created on the date specified.
- queryCreatedBefore string? (default ()) - Filter for only Enrollments created before the date specified.
- queryCreatedOnOrBefore string? (default ()) - Filter for only Enrollments created on or before the date specified.
- queryCreatedAfter string? (default ()) - Filter for only Enrollments created after the date specified.
- queryCreatedOnOrAfter string? (default ()) - Filter for only Enrollments created on or after the date specified.
- queryUpdatedOn string? (default ()) - Filter for only Enrollments updated on the date specified.
- queryUpdatedBefore string? (default ()) - Filter for only Enrollments updated before the date specified.
- queryUpdatedOnOrBefore string? (default ()) - Filter for only Enrollments updated on or before the date specified.
- queryUpdatedAfter string? (default ()) - Filter for only Enrollments updated after the date specified.
Return Type
- GetEnrollmentsResponse|error - Enrollments response
createEnrollment
function createEnrollment(CreateEnrollmentRequest payload) returns EnrollmentResponse|error
Create enrollment
Parameters
- payload CreateEnrollmentRequest - New enrollment attributes
Return Type
- EnrollmentResponse|error - Enrollment response
getEnrollmentsByID
function getEnrollmentsByID(decimal id) returns EnrollmentResponse|error
Get enrollments by ID
Parameters
- id decimal - ID of the Enrollment in the form of an integer.
Return Type
- EnrollmentResponse|error - Enrollment response
updateEnrollment
function updateEnrollment(decimal id, UpdateEnrollmentRequest payload) returns Response|error
Update enrollment
Parameters
- id decimal - ID of the Enrollment in the form of an integer.
- payload UpdateEnrollmentRequest - New enrollment attributes
createExternalOrder
function createExternalOrder(ExternalOrderRequest payload) returns InlineResponse201|error
Create a new external order
Parameters
- payload ExternalOrderRequest - External Order request
Return Type
- InlineResponse201|error - External Order created
createTransactionRefund
function createTransactionRefund(decimal id, ExternalOrderTransaction payload) returns Response|error
refund transaction
createTransactionPurchase
function createTransactionPurchase(string id, ExternalOrderTransaction payload) returns Response|error
purchase transaction
getGroups
function getGroups(decimal page, decimal 'limit) returns GetGroupsResponse|error
Get Groups
Parameters
- page decimal (default 1.0) - The page within the collection to fetch.
- 'limit decimal (default 25.0) - The number of items to be returned.
Return Type
- GetGroupsResponse|error - Groups response
createGroup
function createGroup(GroupRequest payload) returns GroupResponse|error
Create Group
Parameters
- payload GroupRequest - Group
Return Type
- GroupResponse|error - Group response
getGroup
function getGroup(decimal id) returns GroupResponse|error
Get Group
Parameters
- id decimal - The ID of the Group to fetch.
Return Type
- GroupResponse|error - Group response
getGroupAnalysts
function getGroupAnalysts(decimal groupId) returns GetGroupAnalystsResponse|error
Get Group Analysts
Parameters
- groupId decimal - ID of the Group in the form of an integer.
Return Type
- GetGroupAnalystsResponse|error - Groups response
postGroupAnalysts
function postGroupAnalysts(decimal groupId, GroupAddAnalystRequest payload) returns Response|error
Assign Analysts to a Group
Parameters
- groupId decimal - ID of the Group in the form of an integer.
- payload GroupAddAnalystRequest - Group Analyst
removeAnalystFromGroup
Delete Analyst
Parameters
- groupId decimal - ID of the Group in the form of an integer.
- userId decimal - ID of the User in the form of an integer.
addGroupToAnalyst
function addGroupToAnalyst(decimal userId, GroupAnalystsAddGroupRequest payload) returns Response|error
Add Analyst to Groups
Parameters
- userId decimal - ID of the User in the form of an integer.
- payload GroupAnalystsAddGroupRequest - Group Analyst
removeGroupFromAnalyst
Delete Analyst
Parameters
- userId decimal - ID of the User in the form of an integer.
- groupId decimal - ID of the Group in the form of an integer.
getInstructors
function getInstructors(decimal page, decimal 'limit) returns GetInstructorsResponse|error
Get Instructors
Parameters
- page decimal (default 1.0) - The page within the collection to fetch.
- 'limit decimal (default 25.0) - The number of items to be returned.
Return Type
- GetInstructorsResponse|error - Instructors response
createInstructor
function createInstructor(InstructorRequest payload) returns InstructorResponse|error
Create instructor
Parameters
- payload InstructorRequest - New instructor attributes
Return Type
- InstructorResponse|error - Instructor response
getInstructorByID
function getInstructorByID(decimal id) returns InstructorResponse|error
Get Instructor by ID
Parameters
- id decimal - ID of the Instructor in the form of an integer.
Return Type
- InstructorResponse|error - Instructor response
updateInstructor
function updateInstructor(decimal id, InstructorRequest payload) returns Response|error
Update instructor
Parameters
- id decimal - ID of the Instructor in the form of an integer
- payload InstructorRequest - New instructor attributes
deleteInstructorByID
Delete instructor
Parameters
- id decimal - ID of the Instructor in the form of an integer
getOrders
function getOrders(decimal page, decimal 'limit) returns GetOrdersResponse|error
Get Orders
Parameters
- page decimal (default 1.0) - The page within the collection to fetch.
- 'limit decimal (default 25.0) - The number of items to be returned.
Return Type
- GetOrdersResponse|error - Orders
getOrderByID
function getOrderByID(decimal id) returns OrderResponse|error
Get orders by ID
Parameters
- id decimal - ID of the Orders in the form of an integer.
Return Type
- OrderResponse|error - Orders response
createGroupUsers
function createGroupUsers(CreateGroupUsersRequest payload) returns Response|error
Create a User to existing Groups
Parameters
- payload CreateGroupUsersRequest - Group Users attributes
getProductPublishRequests
function getProductPublishRequests(decimal page, decimal 'limit) returns GetProductPublishResponse|error
Get List of product publish requests
Parameters
- page decimal (default 1.0) - The page within the collection to fetch
- 'limit decimal (default 25.0) - The number of items to be returned
Return Type
- GetProductPublishResponse|error - Product Publish Requests response
getProductPublishRequestByID
function getProductPublishRequestByID(decimal id) returns ProductPublishRequest|error
Get product publish request by ID
Parameters
- id decimal - ID of the Product Publish Request in the form of an integer.
Return Type
- ProductPublishRequest|error - Product Publish Request success response
approveProductPublishRequest
Approves Product Publish Request
Parameters
- id decimal - ID of the Product Publish Request in the form of an integer.
denyProductPublishRequest
Denies Product Publish Request
Parameters
- id decimal - ID of the Product Publish Request in the form of an integer.
getProducts
function getProducts(decimal page, decimal 'limit) returns GetProductsResponse|error
List products
Parameters
- page decimal (default 1.0) - The page within the collection to fetch
- 'limit decimal (default 25.0) - The number of items to be returned
Return Type
- GetProductsResponse|error - Products response
getProductByID
function getProductByID(decimal id) returns ProductResponse|error
Get product by ID
Parameters
- id decimal - Id of the product in the form of an integer
Return Type
- ProductResponse|error - Products response
getRelatedProductByProductID
function getRelatedProductByProductID(decimal id, decimal page, decimal 'limit) returns GetProductsResponse|error
Get related products
Parameters
- id decimal - Id of the product in the form of an integer
- page decimal (default 1.0) - The page within the collection to fetch
- 'limit decimal (default 25.0) - The number of items to be returned
Return Type
- GetProductsResponse|error - Products response
getPromotions
function getPromotions(decimal page, decimal 'limit) returns GetPromotionsResponse|error
List promotions
Parameters
- page decimal (default 1.0) - The page within the collection to fetch
- 'limit decimal (default 25.0) - The number of items to be returned
Return Type
- GetPromotionsResponse|error - Promotions response
createPromotion
function createPromotion(PromotionRequest payload) returns PromotionResponse|error
Create a new promotion
Parameters
- payload PromotionRequest - New Promotion parameters
Return Type
- PromotionResponse|error - Promotions response
getPromotionByID
function getPromotionByID(decimal id) returns PromotionResponse|error
Get promotion by provided ID
Parameters
- id decimal - Id of the promotion in the form of an integer
Return Type
- PromotionResponse|error - Promotions response
updatePromotionByID
function updatePromotionByID(decimal id, PromotionRequest payload) returns Response|error
Update promotion by provided ID
Parameters
- id decimal - Id of the promotion in the form of an integer
- payload PromotionRequest - Promotion attributes
deletePromotionByID
Delete promotion by provided ID
Parameters
- id decimal - Id of the promotion in the form of an integer
getPromotionByCouponCode
function getPromotionByCouponCode(decimal productId, string couponCode) returns PromotionResponse|error
Get Promotion associated with the provided Coupon code.
Parameters
- productId decimal - The id of the Product to which the promotion applies.
- couponCode string - The Coupon code to be used as search criteria.
Return Type
- PromotionResponse|error - Promotions response
getCourseReviews
function getCourseReviews(decimal courseId, decimal page, decimal 'limit, boolean? approved) returns GetCourseReviewsResponse|error
Get Course Reviews
Parameters
- courseId decimal - ID of the Course in the form of an integer.
- page decimal (default 1.0) - The page within the collection to fetch.
- 'limit decimal (default 25.0) - The number of items to be returned.
- approved boolean? (default ()) - If true, returns only approved Course Reviews.
Return Type
- GetCourseReviewsResponse|error - CourseReviews
createCourseReview
function createCourseReview(decimal courseId, CreateCourseReviewRequest payload) returns CourseReviewResponse|error
Create a course review
Parameters
- courseId decimal - ID of the Course for which the review needs to be created for.
- payload CreateCourseReviewRequest - Course Review Attributes
Return Type
- CourseReviewResponse|error - Course Review Created
getCourseReviewByID
function getCourseReviewByID(decimal id) returns CourseReviewResponse|error
Get Course Reviews By ID
Parameters
- id decimal - ID of the Course Review in the form of an integer.
Return Type
- CourseReviewResponse|error - CourseReviewByID
getUsers
function getUsers(decimal page, decimal 'limit, string? queryEmail, string? queryRole, string? queryExternalSource, string? queryCustomProfileFieldLabel, string? queryCustomProfileFieldValue, decimal? queryGroupId) returns GetUsersResponse|error
List Users
Parameters
- page decimal (default 1.0) - The page within the collection to fetch
- 'limit decimal (default 25.0) - The number of items to be returned
- queryEmail string? (default ()) - Search Users by email.
- queryRole string? (default ()) - Search Users by role.
- queryExternalSource string? (default ()) - Search Users by external source.
- queryCustomProfileFieldLabel string? (default ()) - Search by custom profile field label (must be combined with custom_profile_field_value)
- queryCustomProfileFieldValue string? (default ()) - Search by custom profile field value (must be combined with custom_profile_field_label)
- queryGroupId decimal? (default ()) - Search by group id.
Return Type
- GetUsersResponse|error - Users response
createUser
function createUser(CreateUserRequest payload) returns UserResponse|error
Create user
Parameters
- payload CreateUserRequest - Create user request body
Return Type
- UserResponse|error - User Created
getUserByID
function getUserByID(string id, string? provider) returns UserResponse|error
List Users by ID
Parameters
- id string - Accepts a Thinkific generated ID (in the form of an integer), or an External ID (as a string) when accompanied by the "provider" parameter.
- provider string? (default ()) - Provider from which the user's External Id is associated (Required if using External Id as id parameter)
Return Type
- UserResponse|error - User Response
updateUserByID
function updateUserByID(string id, UpdateUserRequest payload, string? provider) returns Response|error
Update user by ID
Parameters
- id string - Accepts a Thinkific generated ID (in the form of an integer), or an External ID (as a string) when accompanied by the "provider" parameter.
- payload UpdateUserRequest - User details
- provider string? (default ()) - Provider from which the user's External Id is associated (Required if using External Id as id parameter)
deleteUserByID
Delete user by ID
Parameters
- id string - Accepts a Thinkific generated ID (in the form of an integer), or an External ID (as a string) when accompanied by the "provider" parameter.
- provider string? (default ()) - Provider from which the user's External Id is associated (Required if using External Id as id parameter)
getSiteScripts
function getSiteScripts(decimal page, decimal 'limit) returns GetSiteScriptsResponse|error
List Site Scripts
Parameters
- page decimal (default 1.0) - The page within the collection to fetch
- 'limit decimal (default 25.0) - The number of items to be returned
Return Type
- GetSiteScriptsResponse|error - Site Scripts response
createSiteScript
function createSiteScript(SiteScriptRequest payload) returns SiteScriptResponse|error
Create Site Script
Parameters
- payload SiteScriptRequest - Create Site Script request body
Return Type
- SiteScriptResponse|error - Site Script created
getSiteScriptByID
function getSiteScriptByID(string id) returns SiteScriptResponse|error
Get Site Script by ID
Parameters
- id string - ID of the Site Script in the form of a string
Return Type
- SiteScriptResponse|error - Site Script Response
updateSiteScriptByID
function updateSiteScriptByID(string id, SiteScriptRequest payload) returns Response|error
Update Site Script by ID
Parameters
- id string - ID of the Site Script in the form of a string
- payload SiteScriptRequest - Update Site Script request body
deleteSiteScriptByID
Delete Site Script by ID
Parameters
- id string - ID of the Site Script in the form of a string
Records
thinkific: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- xAuthApiKey string - Used together with ApiKeySubdomain
- xAuthSubdomain string - Used together with ApiKey
thinkific: BulkCreateCouponRequest
Fields
- bulk_quantity_per_coupon decimal? - The number of times each of the autogenerated Coupons can be used. Defaults to allowing unlimited use.
- bulk_coupon_code_length decimal? - The length of the Coupon code. The minimum length is 6.
- bulk_quantity decimal? - The number of unique Coupons to create. Maximum value is 1000.
thinkific: BundleResponse
Fields
- id decimal - The ID of the Bundle
- name string - The name of the Bundle
- description string? - The description on the Bundle
- tagline string? - The tagline for the Bundle (Deprecated)
- banner_image_url string? - Deprecated - use 'bundle_card_image_url'* - The banner image url for the Bundle.
- course_ids decimal[] -
- bundle_card_image_url string? - The card image url for the Bundle
thinkific: ChapterResponse
Fields
- id decimal - The ID of the Chapter as an integer
- name string - The name of the Chapter
- position decimal - The position of the Chapter within the Course
- description string? - The description of the Chapter
- duration_in_seconds decimal? - The duration of the Chapter in seconds. This is the sum of the length of the video lessons in the Chapter
- content_ids decimal[] -
thinkific: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
thinkific: CollectionRequest
Fields
- name string - The name of the Category
- description string - The description of the Category
- slug string - The slug of the Category.
thinkific: CollectionResponse
Fields
- id decimal - The ID of the Category as an integer
- name string - The name of the Category
- description string - The description of the Category
- slug string? - courses (string, required) - The slug of the Category
- created_at string - The date and time when the Category was created
- product_ids decimal[] -
- default boolean - A boolean indicating whether the Category is the default
thinkific: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|ApiKeysConfig - Provides Auth configurations needed when communicating with a remote HTTP endpoint.
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
thinkific: ContentResponse
Fields
- id decimal - The ID of the Content as an integer
- name string - The name of the Content
- position decimal - The position of the Content within a Chapter.
- chapter_id decimal - The ID of the Chapter that the Content is in as an integer
- contentable_type string - The type of the Content
- free boolean - A boolean indicating whether the Content is free
- take_url string - The url of the Content in the Course Player
thinkific: CouponResponse
Fields
- id decimal - The ID of the Coupon as an integer.
- code string - The Coupon code.
- note string - A note associated with the Coupon. NOTE: this will be deprecated in future versions of the API.
- quantity_used decimal - The number of times the Coupon has been used.
- quantity decimal - The number of times the Coupon can be used. If this value if not set, the Coupon can be used an unlimited number of times.
- promotion_id decimal - The ID of the associated Promotion as an integer.
- created_at string - The data and time the Coupon was created.
thinkific: CourseResponse
Fields
- id decimal - The ID of the course
- name string - The name of the course
- slug string? - URL-friendly version of the course name. Used to construct URL for course Landing Pages & Course Player.
- subtitle string? - Deprecated - Used in legacy themes to display the subtitle of the Course.
- product_id decimal - The Course's Product ID
- description string? - The description of the Course(V2 themes currently don't have that info)
- course_card_text string? - Deprecated - use 'description'.* - Used in legacy themes to populate the text for a Course card.
- intro_video_youtube string? - Deprecated - Used in legacy themes to designate an intro video from Youtube.
- contact_information string? - Deprecated - The contact information of the Course.
- keywords string? - The keywords of the Course
- duration string? - Deprecated - Used in legacy themes to display the duration of the Course.
- banner_image_url string? - Deprecated - use 'course_card_image_url'* - The landing page banner image url of the Course.
- course_card_image_url string? - The card image url of the Course
- intro_video_wistia_identifier string? - Deprecated - Used in legacy themes to designate an intro video from Wistia.
- administrator_user_ids decimal[]? -
- chapter_ids decimal[] -
- reviews_enabled boolean - A boolean indicating whether reviews are enabled for the Course
- user_id decimal? - The ID of the User that created the Course
- instructor_id decimal - The ID of the Instructor of the Course
thinkific: CourseReviewResponse
Fields
- id decimal - The ID of the Course Review as an integer.
- rating decimal - The rating, out of 5, of the Course Review.
- title string - The Course Review title.
- review_text string - The body of the Course Review.
- user_id decimal - The ID of the User writing the Course Review as an integer.
- course_id decimal - The ID of the Course being reviewed as an integer.
- approved boolean - A boolean indicating whether the Course Review has been approved.
- created_at string? - The date and time the Course Review was created.
thinkific: CreateBulkCouponResponse
Fields
- items CouponResponse[]? -
thinkific: CreateCouponRequest
Fields
- code string - The Coupon code.
- note string? - A note associated with the Coupon. NOTE: this will be deprecated in future versions of the API.
- quantity decimal? - The number of times the Coupon can be used. If this value if not set, the Coupon can be used an unlimited number of times.
thinkific: CreateCourseReviewRequest
Fields
- rating decimal - The rating, out of 5, of the Course Review.
- title string - The Course Review title.
- review_text string - The body of the Course Review.
- user_id decimal - The ID of the User writing the Course Review as an integer.
- approved boolean - A boolean indicating whether the Course Review has been approved.
thinkific: CreateEnrollmentRequest
Fields
- course_id decimal? - The ID of the Course as an integer.
- user_id decimal? - The ID of the User owning the Enrollment as an integer.
- activated_at string? - The date/time at which the Enrollment is activated. If not provided, the Enrollment is a free trial.
- expiry_date string? - The date/time at which the Enrollment expired. If not provided, the Enrollment does not expire.
thinkific: CreateGroupUsersRequest
Fields
- user_id decimal - The ID of the User to add to Groups
- group_names string[] - The list of Group names to add selected User to
thinkific: CreateUserRequest
Fields
- first_name string - User's first name
- last_name string - User's last name
- email string - User's email
- password string? - The password of the User. If not included, the Express Sign In Link becomes activated for the User.
- roles string[]? - User's roles
- bio string? - User's bio
- company string? - User's Company Name
- headline string? - User's headline
- affiliate_code string? - User's affiliate code
- affiliate_commission decimal? - Required only if the User is an affiliate. This should be greater than 0 and less than or equal to 100 if the type is percentage or lower than 9999.99 if is a fixed type.
- affiliate_commission_type string? - The affiliate payout type, it can be either % (percentage, default) or $ (fixed amount). Required only if the User is an affiliate.
- affiliate_payout_email string? - The email of the User. Required only if the user is an affiliate. Used to pay the User out.
- custom_profile_fields CustomProfileFieldRequest[]? - Custom profile fields for the User.
- skip_custom_fields_validation boolean(default false) - Option to skip required custom profile fields validation.
- send_welcome_email boolean(default false) - Option to send the Site Welcome email to the User.
thinkific: CustomProfileField
Fields
- id decimal? - Custom profile field ID
- value string? - Custom profile field value
- label string? - Custom profile field label
- custom_profile_field_definition_id decimal? - Custom profile field definition ID
thinkific: CustomProfileFieldDefinitionsResponse
Fields
- id decimal - The ID of the Custom Profile Field Definition as an integer.
- label string - The label of the Custom Profile Field Definition.
- field_type string - The Course's Product ID as an integer.
- required boolean - A boolean indicating whether the Custom Profile Field Definition is required.
thinkific: CustomProfileFieldRequest
Fields
- value string? - Custom profile field value
- custom_profile_field_definition_id decimal - Custom profile field definition id
thinkific: EnrollmentResponse
Fields
- id decimal - The ID of the Enrollment as an integer.
- user_email string - The email of the User owning the Enrollment.
- user_name string - The full name of the User owning the Enrollment.
- user_id decimal - The ID of the User owning the Enrollment as an integer.
- course_name string - The name of the Course.
- course_id decimal - The ID of the Course as an integer.
- percentage_completed decimal - The percentage complete of the Enrollment. A number between 0.0 and 1.0. For example, to represent a percentage complete of 75%, this value would be 0.75.
- expired boolean - A boolean indicating whether the Enrollment is expired.
- is_free_trial boolean - A boolean indicating whether the Enrollment is a free trial.
- completed boolean - A boolean indicating whether the Enrollment is complete.
- started_at string - The date/time that the Enrollment started.
- activated_at string - The date/time that the Enrollment was activated.
- completed_at string - The date/time that the Enrollment was completed.
- updated_at string - The date/time that the Enrollment was updated last.
- expiry_date string - The date/time that the Enrollment expires.
thinkific: ErrorBadRequest
Fields
- 'error string? - Malformed request error
thinkific: ErrorForbiddenAppsNotAvailable
Fields
- 'error string? - access forbidden
thinkific: ErrorForbiddenInsufficientScope
Fields
- 'error string? - access forbidden
thinkific: ErrorNotFound
Fields
- 'error string? - item not found error
thinkific: ExternalOrderRequest
External Order Request
Fields
- payment_provider string - An identifier to external provider
- user_id decimal - The ID of the user as an integer
- product_id decimal - The ID of the product as an integer
- order_type string - type of the order
- 'transaction ExternalOrderTransaction? - External Order Transaction
thinkific: ExternalOrderTransaction
External Order Transaction
Fields
- amount decimal - the order amount in currency's smallest unit
- currency string - the order currency
- reference string? - reference number provided by external payment provider
- action string? - Action of the transaction
thinkific: GetChaptersResponse
Fields
- items ChapterResponse[] -
- meta Meta -
thinkific: GetCollectionsResponse
Fields
- items CollectionResponse[] -
- meta Meta -
thinkific: GetContentsResponse
Fields
- items ContentResponse[] -
- meta Meta -
thinkific: GetCouponResponse
Fields
- items CouponResponse[]? -
- meta Meta? -
thinkific: GetCourseReviewsResponse
Fields
- items CourseReviewResponse[] -
- meta Meta -
thinkific: GetCoursesResponse
Fields
- items CourseResponse[]? -
- meta Meta? -
thinkific: GetCustomProfileFieldDefinitions
Fields
- items CustomProfileFieldDefinitionsResponse[] -
- meta Meta -
thinkific: GetEnrollmentsResponse
Fields
- items EnrollmentResponse[] -
- meta Meta -
thinkific: GetGroupAnalystsResponse
Fields
- group_analysts decimal[] - The list of User IDs to add as analysts to the Group
thinkific: GetGroupsResponse
Fields
- items GroupResponse[] -
- meta Meta -
thinkific: GetInstructorsResponse
Fields
- items InstructorResponse[] -
- meta Meta -
thinkific: GetOrdersResponse
Fields
- items OrderResponse[] -
- meta Meta -
thinkific: GetProductPublishResponse
Fields
- items PromotionResponse[] -
- meta Meta -
thinkific: GetProductsResponse
Fields
- items ProductResponse[] -
- meta Meta -
thinkific: GetPromotionsResponse
Fields
- items PromotionResponse[] -
- meta Meta -
thinkific: GetSiteScriptsResponse
Fields
- items SiteScriptResponse[] -
- meta Meta -
thinkific: GetUsersResponse
Fields
- items UserResponse[] -
- meta Meta -
thinkific: GroupAddAnalystRequest
Fields
- user_ids decimal[] - The list of User IDs to add as analysts to the Group
thinkific: GroupAnalystsAddGroupRequest
Fields
- group_ids decimal[] - The list of Group IDs to add the analyst
thinkific: GroupRequest
Fields
- name string - The name of the Group
thinkific: GroupResponse
Fields
- id decimal - The ID of the Group
- name string - The name of the Group
- token string - The 8-character unique identifier for the Group
- created_at string - The date/time the Group was created
thinkific: IdEnrollmentsBody
Fields
- user_id decimal -
- activated_at string? - The date/time at which the Enrollment is to be activated. If not provided, the Enrollment will only provide access to free preview content within the Courses. Provide full access to courses by setting this value to the current date/time.
- expiry_date string? - The date/time at which the Enrollment should be expired. If not provided, the Enrollment does not expire.
thinkific: IdEnrollmentsBody1
Fields
- user_id decimal -
- activated_at string? - The date/time at which the Enrollment is to be activated. If not provided, the Enrollment will only provide access to free preview content within the Courses. Provide full access to courses by setting this value to the current date/time.
- expiry_date string? - The date/time at which the Enrollment should be expired. If not provided, the Enrollment does not expire.
thinkific: InlineResponse201
Fields
- id decimal? -
thinkific: InstructorRequest
Fields
- user_id decimal? - The ID of the User owning the Instructor
- title string? - The title of the Instructor
- first_name string - The first name of the Instructor
- last_name string - The last name of the Instructor
- bio string? - The bio of the Instructor
- slug string - The slug of the Instructor NOTE: This will be removed in future API versions
- avatar_url string? - The fully-qualified url of the Instructor's avatar
- email string? - The email of the Instructor
thinkific: InstructorResponse
Fields
- id decimal - The ID of the Instructor as an integer.
- user_id decimal? - The ID of the User owning the Instructor
- title string? - The title of the Instructor
- first_name string - The first name of the Instructor.
- last_name string - The last name of the Instructor.
- bio string? - The bio of the Instructor
- slug string? - The slug of the Instructor NOTE: This will be removed in future API versions
- avatar_url string? - The fully-qualified url of the Instructor's avatar
- email string? - The email of the Instructor
- created_at string - The date/time that the Instructor was created.
thinkific: Item
Fields
- product_id decimal? - Product ID
- product_name string? - Product name
- amount_dollars decimal? - Product price in dollars
- amount_cents decimal? - Product price in cents
thinkific: MembershipsRequest
Fields
- product_ids decimal[] -
thinkific: Meta
Fields
- pagination Pagination? - Pagination metadata
thinkific: OrderResponse
Fields
- user_id decimal - The ID of the User owning the Order as an integer.
- user_email string - The Email ID of the User.
- user_name string - The full name of the User owning the Order.
- product_name string - The name of the Product purchased.
- product_id decimal - The ID of the Product purchased as an integer.
- amount_dollars decimal - The Order amount in dollars.
- amount_cents decimal - The Order amount in cents.
- subscription boolean - A boolean indicating whether the Order was for a subscription.
- coupon_code string? - The Coupon code used on the Order.
- coupon_id decimal? - The ID of the Coupon used on the Order as an integer.
- items Item[]? - Products included in the Order.
- affiliate_referral_code string? - The Affiliate referral code used on the Order.
- status string - The order status.
- 'created\ at string? - The date/time that the Order was created.
- id decimal - The ID of the Order as an integer.
thinkific: Pagination
Pagination metadata
Fields
- current_page decimal? - Current page number
- next_page decimal? - Next page number
- prev_page decimal? - Previous page number
- total_pages decimal? - Number of total pages
- total_items decimal? - Number of total items
- entries_info string? - Entries info
thinkific: ProductPriceResponse
Fields
- id decimal? - The ID of the Product Price as an integer.
- is_primary boolean? - A boolean indicating whether the Product Price is the default.
- payment_type string? - The type of payment. Must be one of 'free', 'one-time', 'subscription', or 'payment-plan'.
- label string? - The description for the Product Price. This value is required when is_primary is false.
- price string? - The amount in dollars to be charged.
- days_until_expiry string? - The number of days, after purchase, that a student will be enrolled in the course.
- pay_button_text string? - This text that is displayed on the Buy Button on the course card and the course landing page.
- number_of_payments string? - The number of recurring payments for a Product Price with payment_type of 'payment-plan'.
- interval string? - The billing frequency. Must be one of 'month' or 'year'.
- interval_count string? - The number of intervals between billings. For example, if interval is 'month' and interval_count is '1', the billing frequency is once every month.
- trial_interval string? - The interval for the trial period. Must be one of 'day' or 'month'.
- trial_interval_count string? - The number of intervals for the trial period. For example, if trial_interval is 'month' and trial_interval_count is '6', the free trial will end (and regular payments will begin) in 6 months.
- custom_first_price string? - Students pay this amount for the first payment period of the subscription.
- price_name string? - The name of the Product Price. If is_primary is true, returns the name of the product, otherwise returns "{Product name} - {Product Price label}".
- currency string? - The three-letter ISO currency code of the Product Price. Must be a supported currency.
thinkific: ProductPublishRequest
Fields
- product_id decimal - The ID pf the Product as an integer.
- status string - The approval status of the Product Publish Request. Can be either 'approved' or 'denied'.
- response_text string? - The text of the response provided by the approver.
- requesting_user_id decimal - The ID of the requesting User as an integer.
- responding_user_id decimal? - The ID of the responding User as an integer.
- completed_at string? - The date/time the Product Publish Request was completed.
- created_at string? - The date/time the Product Publish Request was created.
- updated_at string? - The date/time the Product Publish Request was last updated.
thinkific: ProductResponse
Fields
- id decimal? - The ID of the Product as an integer.
- created_at string? - The date and time that the Product was created.
- productable_id decimal? - The ID of the Course or Bundle that is represented by the Product.
- productable_type string? - The type of item that the Product represents.
- price decimal? - The price of the Product. ** NOTE: price will be deprecated in future versions of the API **
- position decimal? - The position of the Product within the site. Used for ordering.
- status string? - The status of the Product. Can be either 'published' or 'draft'.
- name string? - The name of the Product.
- 'private boolean? - A boolean indicating whether the Product is private. When private, a Product cannot be purchased. A user must be enrolled manually.
- hidden boolean? - A boolean indicating whether the Product is hidden. When hidden, the Product will not appear on any site pages. It's landing page will be still be accessible via a link, however.
- subscription boolean? - A boolean indicating whether the Product is a subscription or not.
- days_until_expiry decimal? - If access to a Product can end, this value controls how many days access is granted for from the date of enrollment.
- has_certificate boolean? - A boolean indicating whether the Product has a certificate.
- keywords string? - Keywords associated with the Product.
- seo_title string? - The SEO title of the Product.
- seo_description string? - The SEO description of the Product.
- collection_ids decimal[]? - The IDs of the Categories to which this Product belongs as integers.
- related_product_ids decimal[]? - The IDs of any related Products as integers.
- description string? - The description of the product (course or bundle)
- card_image_url string? - The card image URL for the product.
- slug string? - The slug of the product
- product_prices ProductPriceResponse[]? - The Product Price objects for the Product.
thinkific: PromotionRequest
Fields
- name string - The name of the Promotion.
- description string? - A description for the Promotion.
- starts_at string? - The date and time when the Promotion begins.
- expires_at string? - The date and time when the Promotion ends.
- discount_type string - The type of discount. Must be either 'fixed' or 'percentage'.
- amount decimal - The amount of the discount. If 'fixed', must be a dollar value. If 'percentage', must be a number greater than 0 and less than or equal to 100.
- product_ids decimal[]? - The Products to apply the Promotion to. If none are specified, the Promotion is applicable to all Products.
- coupon_ids decimal[]? - The coupon ids related to the promotion.
- duration decimal? - The duration for which the Promotion is applied. ** This value only applies to Promotions set on Products that are set as subscriptions or Payment Plans. **
thinkific: PromotionResponse
Fields
- id decimal? - The ID of the Promotion as an integer.
- name string? - The name of the Promotion.
- description string? - A description for the Promotion.
- starts_at string? - The date and time when the Promotion begins.
- expires_at string? - The date and time when the Promotion ends.
- discount_type string? - The type of discount. Must be either 'fixed' or 'percentage'.
- amount decimal? - The amount of the discount. If 'fixed', must be a dollar value. If 'percentage', must be a number greater than 0 and less than or equal to 100.
- coupon_ids decimal[]? - The coupon ids related to the promotion.
- duration decimal? - The duration for which the Promotion is applied. ** This value only applies to Promotions set on Products that are set as subscriptions or Payment Plans. **
thinkific: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
thinkific: SiteScriptRequest
Fields
- src string? - A URL that points to where the script is hosted. Required if
content
is not provided. Note the TLD of the src url must match the app's url.
- content string? - A string containing HTML/Javascript. Required if
src
is not provided. When providing the script using content, the header HMAC-SHA256-SIGNATURE is also require to be sent. Generate the signature using the app's client secret and the value.
- name string - A short and user-friendly string to identify the script.
- description string - A user-friendly explanation of the script's purpose and what it does.
- page_scopes string[] - An array of page and domain identifiers that the Site Script should be injected onto.
page_scopes
can contain identifiers that are groupings of pages and/or the pages themselves. The majority of the page identifiers correspond to Liquid pages. For more details, take a look at our Liquid API docs. Groupings and Page Identifiers: | Page Grouping | Page Identifiers Included | | ------- | --------| | landing_pages | home_landing_page, course_landing_page, collections_landing_page, bundle_landing_page, coming_soon_page, page_template (used for custom pages) | | checkout | checkout_thankyou_page | | learning_experience | student_dashboard, course_player | | all | all page identifiers listed above |
- location string(default "footer") - Where in the HTML the script should be injected. Accepted values are: head or footer
- load_method string(default "async") - How the Site Script will be loaded into the page. Accepted values are: async, defer, and default
- category string - Describes the purpose of the script. Accepted values are: functional, analytics, marketing
thinkific: SiteScriptResponse
Fields
- id string? - The Site Script's ID.
- content string? - A string containing HTML/Javascript. Required if
src
is not provided.
- src string? - A URL that points to where the script is hosted. Required if
content
is not provided.
- name string? - A short and user-friendly string to identify the script.
- description string? - A user-friendly explanation of the script's purpose and what it does.
- page_scopes string[]? - An array of page and domain identifiers that the Site Script should be injected onto.
page_scopes
can contain identifiers that are groupings of pages and/or the pages themselves. The majority of the page identifiers correspond to Liquid pages. For more details, take a look at our Liquid API docs. Groupings and Page Identifiers: | Page Grouping | Page Identifiers Included | | ------- | --------| | landing_pages | home_landing_page, course_landing_page, collections_landing_page, bundle_landing_page, coming_soon_page, page_template (used for custom pages) | | checkout | checkout_thankyou_page | | learning_experience | student_dashboard, course_player | | all | all page identifiers listed above |
- location string? - Where in the HTML the script should be injected. Accepted values are: head or footer
- load_method string? - How the Site Script will be loaded into the page. Accepted values are: async, defer, and default
- category string? - Describes the purpose of the script. Accepted values are: functional, analytics, marketing
- created_at string? - Site Script's created date
- updated_at string? - Site Script's updated date
thinkific: UnprocessableEntityError
Fields
- errors ValidationError[]? -
thinkific: UpdateCoupon
Fields
- code string - The Coupon code.
- note string? - A note associated with the Coupon. NOTE: this will be deprecated in future versions of the API.
- quantity_used string? - The number of times the Coupon has been used.
- quantity decimal? - The number of times the Coupon can be used. If this value if not set, the Coupon can be used an unlimited number of times.
thinkific: UpdateEnrollmentRequest
Fields
- activated_at string? - The date/time at which the Enrollment is activated. If not provided, the Enrollment is a free trial.
- expiry_date string? - The date/time at which the Enrollment expired. If not provided, the Enrollment does not expire.
thinkific: UpdateUserRequest
Fields
- first_name string? - User's first name
- last_name string? - User's last name
- email string? - User's email
- password string? - The password of the User.
- roles string[]? - User's roles
- avatar_url string? - The fully-qualified avatar url of the User.
- bio string? - User's bio
- company string? - User's Company Name
- headline string? - User's headline
- external_source string? - User's external source
- affiliate_code string? - User's affiliate code
- affiliate_commission decimal? - Required only if the User is an affiliate. This should be greater than 0 and less than or equal to 100 if the type is percentage or lower than 9999.99 if is a fixed type.
- affiliate_commission_type string? - The affiliate payout type, it can be either % (percentage, default) or $ (fixed amount). Required only if the User is an affiliate.
- affiliate_payout_email string? - The email of the User. Required only if the user is an affiliate. Used to pay the User out.
- custom_profile_fields CustomProfileField[]? - Custom profile fields for the User.
thinkific: UserResponse
Fields
- id decimal? - User's ID
- created_at string? - User's created date
- first_name string? - The first name of the User.
- last_name string? - The last name of the User.
- full_name string? - The email of the User.
- company string? - The company of the User.
- email string? - User's email
- roles string[]? - Any specific roles that the User should be placed in. Possible roles are: affiliate, course_admin, group_analyst, site_admin.
- avatar_url string? - The fully-qualified avatar url of the User.
- bio string? - The bio of the User.
- headline string? - The headline/title of the User.
- affiliate_code string? - The affiliate code of the User. ** Required only if the User is an affiliate. **
- external_source string? - User's external source
- affiliate_commission decimal? - The affiliate commission % of the User. ** Required only if the User is an affiliate. This should be greater than 0 and less than or equal to 100. **
- affiliate_commission_type string? - The affiliate payout type, it can be either % (percentage, default) or $ (fixed amount). ** Required only if the User is an affiliate. **
- affiliate_payout_email string? - The email of the User. ** Required only if the user is an affiliate. Used to pay the User out. **
- administered_course_ids decimal[]? - User's administered course ids
- custom_profile_fields CustomProfileField[]? - Custom profile fields for the User.
thinkific: ValidationError
Fields
- field_name string? - validation error
Import
import ballerinax/thinkific;
Metadata
Released date: about 2 years ago
Version: 1.3.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 5
Current verison: 5
Weekly downloads
Keywords
Website & App Building/Website Builders
Cost/Freemium
Contributors