themoviedb
Module themoviedb
API
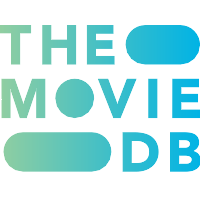
ballerinax/themoviedb Ballerina library
Overview
This is a generated connector for The Movie Database (TMDB) API v3 OpenAPI specification.
The Movie Database (TMDB) API provide data about movies and tv shows around the world.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create TMDB Account
- Obtaining tokens
- Log into TMDB Account
- Click the
Settings
- Click the
API
tab in the left sidebar - Click
Create
orclick here
on the API page
Quickstart
Step 1: Import connector
Import the ballerinax/themoviedb module into the Ballerina project.
import ballerinax/themoviedb;
Step 2: Create a new connector instance
themoviedb:ApiKeysConfig config = { apiKey : "<your appid>" } themoviedb:Client myclient = check new themoviedb:Client(config);
Step 3: Invoke connector operation
- You can get list of upcoming movies as follows with
getUpcomingMovies
method. Successful creation returns the createdGetUpcomingMoviesResponse
and the error cases returns anerror
object.themoviedb:GetUpcomingMoviesResponse result = check myclient->getUpcomingMovies();
- Use
bal run
command to compile and run the Ballerina program.
Clients
themoviedb: Client
This is a generated connector for The Movie Database (TMDB) API v3 OpenAPI specification. The Movie Database (TMDB) API provide data about movies and tv shows around the world.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Request a TMDB Account and obtain tokens following this guide.
init (ApiKeysConfig apiKeyConfig, ConnectionConfig config, string serviceUrl)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.themoviedb.org/3" - URL of the target service
getPopularMovies
function getPopularMovies() returns InlineResponse200|error
Get Popular Movies
Return Type
- InlineResponse200|error - Get popular movies response
getUpcomingMovies
function getUpcomingMovies() returns InlineResponse2001|error
Get Upcoming Movies
Return Type
- InlineResponse2001|error - Get upcoming movies response
getMovieByMovieId
function getMovieByMovieId(int movieId) returns InlineResponse2002|error
Get Movie Details
Parameters
- movieId int - Movie ID
Return Type
- InlineResponse2002|error - Get movie by movie ID response
getTopRatedTvShow
function getTopRatedTvShow() returns InlineResponse2003|error
Get Top Rated
Return Type
- InlineResponse2003|error - Get top rated TV show response
getTvShowEpisode
function getTvShowEpisode(int tvId, int seasonNumber, int episodeNumber) returns InlineResponse2004|error
Get Details
Parameters
- tvId int - TV show ID
- seasonNumber int - TV show season number
- episodeNumber int - TV show episode number
Return Type
- InlineResponse2004|error - Get TV show by details response
searchMovie
function searchMovie(string query, int? year) returns InlineResponse200|error
Search Movies
Return Type
- InlineResponse200|error - Search movie response
searchTvShow
function searchTvShow(string query, int? firstAirDateYear) returns InlineResponse2003|error
Search TV Shows
Return Type
- InlineResponse2003|error - Search TV show response
Records
themoviedb: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- apiKey string - All requests on the TMDB API needs to include an API key. The API key can be provided as part of the query string or as a request header. The name of the API key needs to be
api_key
themoviedb: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
themoviedb: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
themoviedb: Crew
Crew details of TV show
Fields
- id int - Crew ID
- credit_id string? - Credit ID of crew
- name string - Name of the crew
- department string? - Crew department
- job string? - Job of the crew
- profile_path string? - Profile image path
themoviedb: Genre
Genere of movie
Fields
- id int - Genre ID
- name string - Genre name
themoviedb: GuestStar
Guest star details of TV show
Fields
- id int - Guest star ID
- name string - Name of the guest star
- credit_id string? - Credit ID of guest star
- character string? - Character of guest star
- 'order string? - Order of guest star
- profile_path string? - Profile image path
themoviedb: InlineResponse200
Fields
- page int - Specify which page to query
- results MovieListObject[] - Movie List Object
- total_results int - Total number of results
- total_pages int - Total number of pages
themoviedb: InlineResponse2001
Fields
- page int - Specify which page to query
- results MovieListObject[] - Movie List Object
- dates ReleaseDateRange - Release date range
- total_pages int - Total number of pages
- total_results anydata - Total number of results
themoviedb: InlineResponse2002
Fields
- adult boolean? - Adult content or not
- backdrop_path string? - Backdrop image path
- budget int? - Budget of movie
- genres Genre[]? - Genres of movie
- id int - Movie ID
- original_language string? - Original language of movie
- original_title string? - Original title of movie
- popularity decimal? - Popularity of movie
- poster_path string? - Poster image path
- production_companies ProductionCompany[]? - Movie production companies
- production_countries ProductionCountry[]? - Countries where movie produced
- release_date string? - Release date of movie
- revenue int? - Revenue genereted by movie
- spoken_languages SpokenLanguage[]? - Languages spoken in movie
- status string? - Status of movie
- title string - Title of movie
- video boolean? - Contain videos or not
- vote_average decimal? - Vote average
- vote_count int? - Vote count
themoviedb: InlineResponse2003
Fields
- page int - Specify which page to query
- results TvListObject[] - TV List Object
- total_results int - Total number of results
- total_pages int - Total number of pages
themoviedb: InlineResponse2004
Fields
- air_date string? - Air date of TV show
- crew Crew[] - Crew details of TV show
- episode_number int - Episode number
- guest_stars GuestStar[]? - Guest stars in TV show
- name string - Crew details of TV show
- overview string - Overview of TV show
- id int - TV show ID
- season_number int - Season number
- still_path string? - Still path
- vote_average decimal? - Vote average
- vote_count int? - Vote count
themoviedb: InlineResponse401
Fields
- status_message string? -
- status_code int? -
themoviedb: MovieListObject
Movie list object
Fields
- poster_path string? - Poster image path
- adult boolean? - Adult content or not
- overview string - Overview of movie
- release_date string? - Release date
- genre_ids int[]? - Genre IDs of movie
- id int - Movie ID
- original_title string? - Original title
- original_language string? - Original language
- title string - Title of movie
- backdrop_path string? - Backdrop image path
- popularity decimal? - Popularity of movie
- vote_count int? - Vote count
- video boolean? - Video available or not
- vote_average decimal? - Vote average
themoviedb: ProductionCompany
Production company
Fields
- name string - Production company name
- id int - Production company ID
- origin_country string? - Country of production company
themoviedb: ProductionCountry
Production country
Fields
- iso_3166_1 string - Standard for representation of country names
- name string - Production country name
themoviedb: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
themoviedb: ReleaseDateRange
Release date range
Fields
- maximum string - Maximum date of release
- minimum string - Minimum date of release
themoviedb: SpokenLanguage
Spoken language
Fields
- iso_639_1 string - Standard for representation of languages
- name string - Spoken language name
themoviedb: TvListObject
TV list object
Fields
- poster_path string? - Poster image path
- popularity decimal? - Popularity of TV show
- id int - TV show ID
- backdrop_path string? - Backdrop image path
- vote_average decimal? - Vote average
- overview string - Overview of TV show
- first_air_date string? - First air date of TV show
- origin_country string[]? - Origin country
- genre_ids int[]? - Genre IDs of TV show
- original_language string? - Original language
- vote_count int? - Vote count
- name string - Name
- original_name string? - Original name of TV show
Import
import ballerinax/themoviedb;
Metadata
Released date: about 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 2096
Current verison: 294
Weekly downloads
Keywords
Content & Files/Video & Audio
Cost/Free
Contributors
Dependencies