supportbee
Module supportbee
API
Definitions
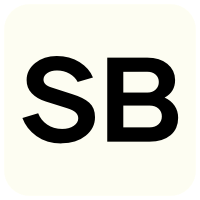
ballerinax/supportbee Ballerina library
Overview
This is a generated connector for SupportBee API v1 OpenAPI specification. SupportBee’s support ticket system enables teams to organize, prioritize and collaborate on customer support emails.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a SupportBee account
- Obtain tokens by following this guide
Quickstart
To use the SupportBee connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/supportbee
module into the Ballerina project.
import ballerinax/supportbee;
Step 2: Create a new connector instance
Create a supportbee:ApiKeysConfig
with the API key obtained, and initialize the connector with it.
supportbee:ApiKeysConfig config = { authToken: "<API_KEY>" } supportbee:Client baseClient = check new Client(config, serviceUrl = "https://{company}.supportbee.com");
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to create a ticket for the company using the connector.
Creates a ticket for the company
public function main() returns error? { supportbee:CreateTicketRequest requestData = { ticket: { subject: "Subject", requester_name: "John Doe", requester_email: "john@example.com", cc: [ "Test1 <test1@example.com>", "Test2 <test2@example.com>" ], bcc: [ "Test3 <test3@example.com>", "Test4 <test4@example.com>" ], content: { text: "Creating a ticket", html: "<p>Creating a ticket</p>" } } }; supportbee:CreatedTicket response = check baseClient->createTicket(requestData); log:printInfo(response.toJsonString()); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
supportbee: Client
This is a generated connector for SupportBee API v1 OpenAPI specification. SupportBee’s support ticket system enables teams to organize, prioritize and collaborate on customer support emails.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a SupportBee account and obtain tokens by following this guide.
init (ApiKeysConfig apiKeyConfig, string serviceUrl, ConnectionConfig config)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- serviceUrl string - URL of the target service
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
listTickets
function listTickets(string accept, int? perPage, int? page, string? archived, boolean? spam, boolean? trash, boolean? replies, int? maxReplies, string? assignedUser, string? assignedTeam, boolean? starred, string? label, string? since, string? until, string? sortBy, string? requesterEmails, boolean? totalOnly) returns Tickets|error
Returns 15 tickets of the company in the order of their last activity. Only tickets that are not archived are returned.
Parameters
- accept string (default "application/json") - All API requests must have a Accept header set to application/json.
- perPage int? (default ()) - Specifies the number of tickets to retrieve. Must be less than 100. Defaults to 15.
- page int? (default ()) - Specifies the page of tickets to retrieve. Defaults to 1.
- archived string? (default ()) - If true, retrieves only archived tickets. If false, it does not return any archived tickets. If any, includes archived tickets in the result. Defaults to false.
- spam boolean? (default ()) - If true, retrieved tickets contain tickets marked as spam. Defaults to false.
- trash boolean? (default ()) - If true, retrieved tickets contain tickets that are trashed. Defaults to false.
- replies boolean? (default ()) - If true, retrieved tickets contain only tickets with replies. If false, retrieved tickets contain only tickets without replies.
- maxReplies int? (default ()) - Specifies the number of replies that a ticket must have. This cannot be used with replies = false.
- assignedUser string? (default ()) - If me, retrieves only tickets assigned to the current user. If agent_id, retrieves only tickets assigned to the Agent with id agent_id. If any, retrieves tickets that are assigned to any user. If none, retrieves tickets that aren't assigned to any user.
- assignedTeam string? (default ()) - If mine, retrieves only tickets assigned to the teams of the current user. If team_id, retrieves only tickets assigned to the team with id team_id. If none, retrieves tickets that aren't assigned to any team.
- starred boolean? (default ()) - If true, retrieves only the starred tickets of the current user. If false, retrieves only the non starred tickets assigned to the current user.
- label string? (default ()) - Set to label_name, retrieves only the tickets with the label label_name.
- since string? (default ()) - Can be used to retrieve tickets whose last activity timestamp is greater than the time specified in this parameter. The last activity timestamp of a ticket is updated whenever there is a new reply or a new comment on the ticket. To retrieve tickets sorted by creation time, instead of last activity, send the sort_by parameter along with the since parameter.
- until string? (default ()) - Can be used to retrieve tickets whose last activity timestamp is lesser than the time specified in this parameter. The last activity timestamp of a ticket is updated whenever there is a new reply or a new comment on the ticket. To retrieve tickets sorted by creation time, instead of last activity, send the sort_by parameter along with the until parameter.
- sortBy string? (default ()) - If last_activity, retrieves tickets sorted by last activity. If creation_time, retrieves tickets sorted by creation time. Defaults to last_activity.
- requesterEmails string? (default ()) - Can be used to filter tickets by requester email addresses. Accepts a string of comma separated email addresses. For Example requester_emails=test1@example.com,test2@example.com
- totalOnly boolean? (default ()) - Can be used in conjunction with any other parameters to return only the total number of tickets. Accepts any truthy value. For example total_only=true. Defaults to false.
createTicket
function createTicket(CreateTicketRequest payload, string accept) returns CreatedTicket|error
Creates a ticket for the company.
Parameters
- payload CreateTicketRequest - The data required to create a ticket.
- accept string (default "application/json") - All API requests must have a Accept header set to application/json.
Return Type
- CreatedTicket|error - Created ticket
getTicket
function getTicket(string id, string accept) returns TicketObject|error
Retrieves the ticket specified by the id.
Parameters
- id string - The ticket ID
- accept string (default "application/json") - All API requests must have a Accept header set to application/json.
Return Type
- TicketObject|error - A ticket object
deleteTrashedTicket
Deletes a trashed ticket.
Parameters
- id string - The ticket ID
- accept string (default "application/json") - All API requests must have a Accept header set to application/json.
archiveTicket
Archives an unarchived ticket specified by ticket_id.
Parameters
- ticketId string - The ticket ID
- accept string (default "application/json") - All API requests must have a Accept header set to application/json.
trashTicket
Trashes' an un-trashed ticket specified by ticket_id.
Parameters
- ticketId string - The ticket ID
- accept string (default "application/json") - All API requests must have a Accept header set to application/json.
assignUser
function assignUser(string ticketId, AssignUserRequest payload, string accept) returns AssignedUserObject|error
Assign a ticket to a user. If the ticket is already assigned to a team, then the given user must be a member of that team.
Parameters
- ticketId string - The ticket ID
- payload AssignUserRequest - The data required to assign a ticket to a user.
- accept string (default "application/json") - All API requests must have a Accept header set to application/json.
Return Type
- AssignedUserObject|error - An object with a property user_assignment.
getUser
function getUser(string id, string accept, string? maxTickets) returns GetUserObject|error
Retrieves the user specified by id.
Parameters
- id string - The user ID
- accept string (default "application/json") - All API requests must have a Accept header set to application/json.
- maxTickets string? (default ()) - Specify the maximum number of recent tickets you want to retrieve that this user created. If false returns all tickets.
Return Type
- GetUserObject|error - A user object
listUsers
function listUsers(string accept, boolean? withInvited, string? withRoles, string? 'type) returns Users|error
Retrieves all users of the company.
Parameters
- accept string (default "application/json") - All API requests must have a Accept header set to application/json.
- withInvited boolean? (default ()) - If true, returns all the agents, including invited (unconfirmed) agents. Defaults to false.
- withRoles string? (default ()) - Specifies the role of the users you want to get. The role must be one of these: admin, agent, collaborator, customer. Several or all of these values can be used. Defaults to admin,agent,collaborator.
- 'type string? (default ()) - Specifies the type of the users you want to get. The type must be one of these: user, customer_group. Defaults to user.
listReplies
Retrieves all the replies of the ticket with id ticket_id.
Parameters
- ticketId string - The ticket ID
- accept string (default "application/json") - All API requests must have a Accept header set to application/json.
createReply
function createReply(string ticketId, CreateReplyRequest payload, string accept) returns CreatedReply|error
Posts a reply to the ticket with id ticket_id.
Parameters
- ticketId string - The ticket ID
- payload CreateReplyRequest - The data required to create a ticket.
- accept string (default "application/json") - All API requests must have a Accept header set to application/json.
Return Type
- CreatedReply|error - Created reply
getReply
function getReply(string ticketId, string id, string accept) returns ReplyObject|error
Retrieves the reply for ticket specified by the ticket_id with id.
Parameters
- ticketId string - The ticket ID
- id string - The reply ID
- accept string (default "application/json") - All API requests must have a Accept header set to application/json.
Return Type
- ReplyObject|error - A reply object
createLabel
function createLabel(string ticketId, string labelName, string accept) returns CreatedLabel|error
Adds the label with {label_name} to the ticket with id ticket_id
Parameters
- ticketId string - The ticket ID
- labelName string - The label name
- accept string (default "application/json") - All API requests must have a Accept header set to application/json.
Return Type
- CreatedLabel|error - Created reply
removeLabel
Removes label from a ticket.
Parameters
- ticketId string - The ticket ID
- labelName string - The label name
- accept string (default "application/json") - All API requests must have a Accept header set to application/json.
listLabels
Retrieves all the custom labels of a company.
Parameters
- accept string (default "application/json") - All API requests must have a Accept header set to application/json.
listComments
Retrieves all the comments of the ticket with id.
Parameters
- ticketId string - The ticket ID
- accept string (default "application/json") - All API requests must have a Accept header set to application/json.
Return Type
createComment
function createComment(string ticketId, CreateCommentRequest payload, string accept) returns CreatedComment|error
Posts a comment to the ticket with id.
Parameters
- ticketId string - The ticket ID
- payload CreateCommentRequest - The data required to create a comment.
- accept string (default "application/json") - All API requests must have a Accept header set to application/json.
Return Type
- CreatedComment|error - Created comment
Records
supportbee: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- authToken string - Represents API Key
auth_token
supportbee: AssignedUserObject
The assigned user object
Fields
- id int? - User assignment ID
- created_at string? - Created at
- ticket UserAssignmentTicket? - User assignment ticket object
- assignee Assignee? - Assignee object
supportbee: Assignee
Assignee object
Fields
- user User? - User object
supportbee: AssignUserRequest
The data required to assign a ticket to a user.
Fields
- user_assignment UserAssignmentRequest? - The data required to assign a ticket to a user.
supportbee: Attachment
Specifies the attachment object.
Fields
- id int? - Attachment ID
- created_at string? - Created at
- filename string? - Filename
- content_type string? - Content type
- url Url? - URL object
supportbee: BCC
BCC object
Fields
- id int? - Email ID
- email string? - Email address
- name string? - Name
- role string? - Role
- agent boolean? - Agent status
- picture Picture? - Picture object
supportbee: CC
CC object
Fields
- id int? - Email ID
- email string? - Email address
- name string? - Name
- role string? - Role
- agent boolean? - Agent status
- picture Picture? - Picture object
supportbee: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
supportbee: Comment
Comment object
Fields
- id int? - Comment ID
- created_at string? - Created at
- ticket Ticket? - Ticket object
- commenter Commenter? - Commenter object
- content CommentContent? - Specifies the content of the comment.
supportbee: CommentContent
Specifies the content of the comment.
Fields
- text string? - Specifies the text content of the comment.
- html string? - Specifies the html content of the comment.
- attachments Attachment[]? - Specifies the attachments of the comment.
supportbee: Commenter
Commenter object
Fields
- id int? - Replier ID
- email string? - Email address
- name string? - Name
- agent boolean? - Agent status
- picture Picture? - Picture object
supportbee: CommentRequest
The data required to create a comment.
Fields
- content CommentRequestContent? - Specifies the content of the comment. Either text or html must be present.
supportbee: CommentRequestContent
Specifies the content of the comment. Either text or html must be present.
Fields
- text string? - Specifies the text content of the comment.
- html string? - Specifies the html content of the comment.
- attachment_ids int[]? - Specifies the attachment IDs of the comment.
supportbee: Comments
An object with a property comments which is an array of Comment objects.
Fields
- comments Comment[]? - An array of Comment objects.
supportbee: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
supportbee: Content
Specifies the content of the ticket. Either text or html must be present.
Fields
- text string? - Specifies the text content of the ticket.
- html string? - Specifies the html content of the ticket.
- attachment_ids int[]? - Specifies the attachment IDs of the ticket.
supportbee: CreateCommentRequest
The data required to create a comment.
Fields
- comment CommentRequest? - The data required to create a comment.
supportbee: CreatedComment
Created comment.
Fields
- comment Comment? - Comment object
supportbee: CreatedLabel
Created label.
Fields
- label Label? - Label object.
supportbee: CreatedReply
Created reply.
Fields
- reply Reply? - Reply object
supportbee: CreatedTicket
Created ticket.
Fields
- ticket Ticket? - Ticket object
supportbee: CreateReplyRequest
The data required to create a reply.
Fields
- reply ReplyRequest? - The data required to create a reply.
supportbee: CreateTicketRequest
The data required to create a ticket.
Fields
- ticket TicketRequest? - The data required to create a ticket.
supportbee: CurrentTeamAssignee
Current team assignee
Fields
- team Team? - Team object
supportbee: CurrentUserAssignee
Current user assignee
Fields
- user User? - User object
supportbee: GetUserObject
Get user object
Fields
- user UserObject? - User object
supportbee: Label
Label object.
Fields
- id int? - Label ID.
- label string? - Label name.
- ticket int? - Ticket ID.
supportbee: LabelObject
Label object.
Fields
- name string? - Label name
- color string? - Label color
supportbee: Labels
An object with a property labels which is an array of Label objects.
Fields
- labels LabelObject[]? - An array of Label objects.
supportbee: Picture
Picture object
Fields
- thumb20 string? - Thumb 20
- thumb24 string? - Thumb 24
- thumb32 string? - Thumb 32
- thumb48 string? - Thumb 48
- thumb64 string? - Thumb 64
- thumb128 string? - Thumb 128
supportbee: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
supportbee: Replier
Replier object
Fields
- id int? - Replier ID
- email string? - Email address
- name string? - Name
- agent boolean? - Agent status
- picture Picture? - Picture object
supportbee: Replies
An object with a property replies which is an array of Reply objects.
Fields
- replies Reply[]? - An array of Reply objects.
supportbee: Reply
Reply object
Fields
- id int? - Reply ID
- created_at string? - Created at
- summary string? - Summary of the reply
- cc CC[]? - Specifies the CC email addresses included in the reply.
- bcc BCC[]? - Specifies the BCC email addresses included in the reply.
- ticket Ticket? - Ticket object
- replier Replier? - Replier object
- content ReplyContent? - Specifies the content of the reply.
supportbee: ReplyContent
Specifies the content of the reply.
Fields
- text string? - Specifies the text content of the reply.
- html string? - Specifies the html content of the reply.
- attachments Attachment[]? - Specifies the attachments of the reply.
supportbee: ReplyObject
A reply object.
Fields
- reply Reply? - Reply object
supportbee: ReplyRequest
The data required to create a reply.
Fields
- cc string[]? - Specifies the CC email addresses included in the reply. If there are multiple emails, pass them comma separated. Up to 25 addresses are allowed.
- bcc string[]? - Specifies the BCC email addresses included in the reply. If there are multiple emails, pass them comma separated. Up to 25 addresses are allowed.
- content ReplyRequestContent? - Specifies the content of the reply. Either text or html must be present.
supportbee: ReplyRequestContent
Specifies the content of the reply. Either text or html must be present.
Fields
- text string? - Specifies the text content of the ticket.
- html string? - Specifies the html content of the ticket.
- attachment_ids int[]? - Specifies the attachment IDs of the ticket.
supportbee: Requester
Requester object
Fields
- id int? - Email ID
- email string? - Email address
- name string? - Name
- role string? - Role
- agent boolean? - Agent status
- picture Picture? - Picture object
supportbee: Source
Source of the ticket
Fields
- email string? - Email address
supportbee: Team
Team object
Fields
- id int? - Email ID
- name string? - Name
- picture Picture? - Picture object
supportbee: Ticket
Ticket object
Fields
- id int? - Ticket ID
- subject string? - Subject of the ticket
- replies_count int? - Reply count for the ticket
- comments_count int? - Comments count for the ticket
- last_activity_at string? - Last activity at
- created_at string? - Created at
- unanswered boolean? - Unanswered status
- archived boolean? - Archived status
- spam boolean? - Spam status
- starred boolean? - Starred status
- summary string? - Summary of the ticket
- 'source Source? - Source of the ticket
- cc CC[]? - Specifies the CC email addresses included in the ticket.
- bcc BCC[]? - Specifies the BCC email addresses included in the ticket.
- current_user_assignee CurrentUserAssignee? - Current user assignee
- current_team_assignee CurrentTeamAssignee? - Current team assignee
- requester Requester? - Requester object
- content TicketContent? - Specifies the content of the ticket.
supportbee: TicketContent
Specifies the content of the ticket.
Fields
- text string? - Specifies the text content of the ticket.
- html string? - Specifies the html content of the ticket.
- attachments Attachment[]? - Specifies the attachments of the ticket.
supportbee: TicketObject
A ticket object.
Fields
- ticket Ticket? - Ticket object
supportbee: TicketRequest
The data required to create a ticket.
Fields
- subject string? - Specifies the subject of the ticket.
- requester_name string? - Specifies the name of the requester of the ticket.
- requester_email string? - Specifies the email of the requester of the ticket.
- cc string[]? - Specifies the CC email addresses included in the ticket. If there are multiple emails, pass them comma separated. Up to 25 addresses are allowed.
- bcc string[]? - Specifies the BCC email addresses included in the ticket. If there are multiple emails, pass them comma separated. Up to 25 addresses are allowed.
- notify_requester boolean? - If true, a copy of the ticket is sent to the requester and all email addresses in CC and BCC. An auto-response is not sent when this parameter is true (even if it's enabled in the settings). The sender name in this copy is taken from the forwarding address used to create the ticket.
- content Content? - Specifies the content of the ticket. Either text or html must be present.
- attachment_ids int[]? - Specifies the attachment IDs of the ticket.
- forwarding_address_id string? - This optional parameter lets you specify the email address/name to be used for sending out replies/auto-responses to the customer. You can find the forwarding address id by editing the desired forwarding address and copying the id from the URL once you are on the edit page (we are working on making this more straight forward). If you are using a SMTP server for delivering emails, it's important that you send this parameter and use the correct email address to avoid any delivery issues.
supportbee: Tickets
An object with a property tickets which is an array of Ticket objects.
Fields
- total int? - Total tickets
- current_page int? - Current page count
- per_page int? - Per page count
- total_pages int? - Total pages count
- tickets Ticket[]? - An array of Ticket objects.
supportbee: Url
URL object
Fields
- original string? - Original URL
- thumb string? - Thumbnail URL
supportbee: User
User object
Fields
- id int? - Email ID
- email string? - Email address
- name string? - Name
- agent boolean? - Agent status
- picture Picture? - Picture object
supportbee: UserAssignmentRequest
The data required to assign a ticket to a user.
Fields
- user_id int? - User ID
supportbee: UserAssignmentTicket
User assignment ticket object
Fields
- id int? - User assignment ticket ID
supportbee: UserObject
User object
Fields
- id int? - User ID
- 'type string? - User type
- email string? - Email address
- first_name string? - First name
- last_name string? - Last name
- name string? - Name
- role string? - Role
- agent boolean? - Agent status
- two_factor_authentication_enabled boolean? - Two factor authentication enabled
- picture Picture? - Picture object
- can_members_access_group_tickets boolean? - Can members access group tickets
- members_count int? - Members count
- active_tickets_count int? - Active tickets count
supportbee: Users
An object with a property users which is an array of User objects.
Fields
- users UserObject[]? - An array of User objects.
Import
import ballerinax/supportbee;
Metadata
Released date: about 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 5
Current verison: 5
Weekly downloads
Keywords
Support/Customer Support
Cost/Paid
Contributors
Dependencies