sugarcrm
Module sugarcrm
API
Definitions
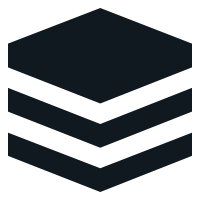
ballerinax/sugarcrm Ballerina library
Overview
This is a generated connector for SugarCRM REST API v12.0 OpenAPI Specification. SugarCRM REST API provides capabilities to effectively manage the customer lifecycle with a set of modules that support each stage of the funnel.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a SugarCRM account
- Obtain tokens by following this guide
Quickstart
To use the SugarCRM connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/sugarcrm
module into the Ballerina project.
import ballerinax/sugarcrm;
Step 2: Create a new connector instance
Create a sugarcrm:ApiKeysConfig
with the API key obtained, and initialize the connector with it.
sugarcrm:ClientConfig config = { auth: { tokenUrl: "<TOKEN_URL>" username: "<USERNAME>" password: "<PASSWORD>" clientId: "<CLIENT_ID>" clientSecret: "<CLIENT_SECRET>" } } sugarcrm:Client sugarCrmClient = check new Client(config, serviceUrl = "https://<site_url>/rest/v{version}/");
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to list set of records filtered by an expression using the connector.
Creates a ticket for the company
public function main() returns error? { string module = "Account"; string[] filter = [{"id":"1"}]; FilteredRecordDetails records = check sugarCrmClient -> listRecords(module, filter); io:println(records); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
sugarcrm: Client
This is a generated connector for SugarCRM REST API v12.0 OpenAPI Specification. SugarCRM REST API provides capabilities to effectively manage the customer lifecycle with a set of modules that support each stage of the funnel.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create SugarCRM account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string - URL of the target service
listRecords
function listRecords(string module, string[]? filter, string? filterId, string? q, string? maxNum, string? offset, string? fields, string? orderBy, boolean? deleted, string? view, boolean? nullsLast) returns FilteredRecordDetails|error
Return a set of records filtered by an expression.
Parameters
- module string - The name of the module.
- filter string[]? (default ()) - The [filter expression](https://support.sugarcrm.com/Documentation/Sugar_Developer/Sugar_Developer_Guide_11.3/Integration/Web_Services/REST_API/End
- filterId string? (default ()) - Identifier for a preexisting filter. If filter is also set, the two filters are joined with an AND.
- q string? (default ()) - A search expression, will search on this module. Cannot be used at the same time as a filter expression or id.
- maxNum string? (default ()) - A maximum number of records to return. The default is all records.
- offset string? (default ()) - The number of records to skip over before records are returned. The default is 0. The parameter will be ignored if the max_num parameter is missing.
- fields string? (default ()) - Comma delimited list of fields to return. The field date_modified will always be returned.
name,account_type,description
- orderBy string? (default ()) - How to sort the returned records, in a comma delimited list with the direction appended to the column name after a colon.
name:DESC,account_type:DESC,date_modified:ASC
- deleted boolean? (default ()) - Boolean to show deleted records in the result set.
- view string? (default ()) - Instead of defining the fields argument, the view argument can be used instead. The field list is constructed at the server side based on the view definition which is requested. This argument can be used in combination with the fields argument.
- nullsLast boolean? (default ()) - Boolean to return records with null values in order_by fields last in the result set.
Return Type
createRecord
Create a new record of the specified type
Return Type
- json|error - ok
listFilteredRecords
function listFilteredRecords(string module, FilterOptions payload) returns FilteredRecordDetails|error
Return a set of records filtered by an expression. The filter can be applied to multiple fields and have multiple and/or conditions in it.
Return Type
retrieveRecord
Returns a single record
Return Type
- json|error - ok
updateRecord
Update a record of the specified type
Parameters
- module string - The name of the module.
- 'record string - The record id.
- payload json - Record Details
Return Type
- json|error - ok
deleteRecord
function deleteRecord(string module, string 'record) returns SuccessResponseID|error
Delete a record of the specified type
Return Type
- SuccessResponseID|error - ok
viewChangeLog
function viewChangeLog(string module, string 'record, string? maxNum, string? offset) returns FilteredRecordDetails|error
View audit log in record view
Parameters
- module string - The name of the module.
- 'record string - The record id.
- maxNum string? (default ()) - A maximum number of records to return. The default is all records.
- offset string? (default ()) - The number of records to skip over before records are returned. The default is 0. The parameter will be ignored if the max_num parameter is missing.
Return Type
globalSearchElasticsearch
function globalSearchElasticsearch(SearchOptions payload) returns FilteredRecordDetails|error
Expose the global search capability using solely the Elasticsearch backend as an alternative to the /search endpoint.
Parameters
- payload SearchOptions - Filter options
Return Type
globalSearch
function globalSearch(string? q, string? maxNum, string? offset, string? fields, string? orderBy, boolean? favorites, boolean? myItems) returns FilteredRecordDetails|error
Globally search records
Parameters
- q string? (default ()) - A search expression, will search on this module. Cannot be used at the same time as a filter expression or id.
- maxNum string? (default ()) - A maximum number of records to return. The default is all records.
- offset string? (default ()) - The number of records to skip over before records are returned. The default is 0. The parameter will be ignored if the max_num parameter is missing.
- fields string? (default ()) - Comma delimited list of fields to return. The field date_modified will always be returned.
name,account_type,description
- orderBy string? (default ()) - How to sort the returned records, in a comma delimited list with the direction appended to the column name after a colon.
name:DESC,account_type:DESC,date_modified:ASC
- favorites boolean? (default ()) - Only fetch the current users favorited records.
- myItems boolean? (default ()) - Only fetch items assigned to the current user.
Return Type
createCallEvent
function createCallEvent(json payload) returns json|error
Create a single event or a series of event records of the specified type
Parameters
- payload json - The name value list of fields to populate.
Return Type
- json|error - ok
updateCallEvent
Update a calendar event record of the specified type.
Parameters
- 'record string - The record id.
- allRecurrences boolean? (default ()) - Flag to update all events in a series.
Return Type
- json|error - ok
deleteCallEvent
function deleteCallEvent(string 'record, boolean? allRecurrences) returns SuccessResponseID|error
Deletes either a single event record or a series of event records.
Parameters
- 'record string - The record id.
- allRecurrences boolean? (default ()) - Flag to delete all events in a series.
Return Type
- SuccessResponseID|error - ok
retrieveCommentlogParent
Returns a single parent record of the given commentlog
Parameters
- 'record string - CommentLog ID
Return Type
- json|error - ok
configSave
function configSave(json payload) returns json|error
Saves configuration changes in the database.
Parameters
- payload json - Update account payload
Return Type
- json|error - ok
getDefaultMetadata
Return the original metadata for the module.
Parameters
- modules string - Comma-separated module names
- 'type string - The type of the desired metadata. The following types are supported- 'filter', 'layout', 'menu', 'view'.
- name string - The name of the metadata. Examples- 'list', 'record', or 'multi-line-list'.
Return Type
- json|error - ok
getContactCases
function getContactCases(string 'record, string[]? filter, string? filterId, string? maxNum, string? offset, string? fields, string? orderBy, boolean? deleted) returns FilteredRecordDetails|error
Get a contact's related cases, filtered by an expression, which are accessible to a contact with portal visibility.
Parameters
- 'record string - Contact ID
- filter string[]? (default ()) - The [filter expression](https://support.sugarcrm.com/Documentation/Sugar_Developer/Sugar_Developer_Guide_11.3/Integration/Web_Services/REST_API/End
- filterId string? (default ()) - Identifier for a preexisting filter. If filter is also set, the two filters are joined with an AND.
- maxNum string? (default ()) - A maximum number of records to return. The default is all records.
- offset string? (default ()) - The number of records to skip over before records are returned. The default is 0. The parameter will be ignored if the max_num parameter is missing.
- fields string? (default ()) - Comma delimited list of fields to return. The field date_modified will always be returned.
name,account_type,description
- orderBy string? (default ()) - How to sort the returned records, in a comma delimited list with the direction appended to the column name after a colon.
name:DESC,account_type:DESC,date_modified:ASC
- deleted boolean? (default ()) - Boolean to show deleted records in the result set.
Return Type
getFreeBusySchedule
function getFreeBusySchedule(string 'record) returns FreeBusyData|error
Returns a list of time slots for which the specified person is busy.
Parameters
- 'record string - Contact ID
Return Type
- FreeBusyData|error - ok
getCurrencies
function getCurrencies() returns FilteredRecordDetails|error
Return the Currencies defined in the application
Return Type
getUsers
function getUsers(string[]? filter, string? filterId, string? q, string? maxNum, string? offset, string? fields, string? orderBy, boolean? deleted, string? view, boolean? nullsLast) returns FilteredRecordDetails|error
Return a set of User records filtered by an expression.
Parameters
- filter string[]? (default ()) - The [filter expression](https://support.sugarcrm.com/Documentation/Sugar_Developer/Sugar_Developer_Guide_11.3/Integration/Web_Services/REST_API/End
- filterId string? (default ()) - Identifier for a preexisting filter. If filter is also set, the two filters are joined with an AND.
- q string? (default ()) - A search expression, will search on this module. Cannot be used at the same time as a filter expression or id.
- maxNum string? (default ()) - A maximum number of records to return. The default is all records.
- offset string? (default ()) - The number of records to skip over before records are returned. The default is 0. The parameter will be ignored if the max_num parameter is missing.
- fields string? (default ()) - Comma delimited list of fields to return. The field date_modified will always be returned.
name,account_type,description
- orderBy string? (default ()) - How to sort the returned records, in a comma delimited list with the direction appended to the column name after a colon.
name:DESC,account_type:DESC,date_modified:ASC
- deleted boolean? (default ()) - Boolean to show deleted records in the result set.
- view string? (default ()) - Instead of defining the fields argument, the view argument can be used instead. The field list is constructed at the server side based on the view definition which is requested. This argument can be used in combination with the fields argument.
- nullsLast boolean? (default ()) - Boolean to return records with null values in order_by fields last in the result set.
Return Type
deleteUser
function deleteUser(string 'record) returns SuccessResponseID|error
Delete a User record
Parameters
- 'record string - User ID
Return Type
- SuccessResponseID|error - ok
getUserFreeBusySchedule
function getUserFreeBusySchedule(string 'record) returns FreeBusyData|error
Retrieve a list of calendar event start and end times for specified person
Parameters
- 'record string - User ID
Return Type
- FreeBusyData|error - ok
createCalendarEvent
function createCalendarEvent() returns json|error
Create a single event record or a series of event records
Return Type
- json|error - ok
updateCalendarEvent
Update a single event record or a series of event records
Parameters
- 'record string - Meeting ID
- allRecurrences boolean? (default ()) - Flag to update all events in a series.
Return Type
- json|error - ok
deleteCalendarEvent
function deleteCalendarEvent(string 'record, boolean? allRecurrences) returns SuccessResponseID|error
Delete a single event record or a series of event records
Parameters
- 'record string - Meeting ID
- allRecurrences boolean? (default ()) - Flag to delete all events in a series.
Return Type
- SuccessResponseID|error - ok
getExternalMeetingInfo
Retrieve info about launching an external meeting
Parameters
- 'record string - Meeting ID
Return Type
- json|error - ok
updateOpportunity
Update a record of the specified type
Parameters
- 'record string - Opportunity ID
Return Type
- json|error - ok
createLead
Create a Lead with the optional post-save actions for Convert Target/Prospect and Create Lead from Email operations
Parameters
- prospectId string - Pass a prospect id if Lead is being created as part of a Convert Target/Prospect process
- inboundEmailId string - Pass an inbound email id if Lead is being created from an Email
Return Type
- json|error - ok
convertLead
Convert Lead to a Contact and optionally link it to a new or existing module such as an Account or Opportunity
Parameters
- leadId string - Lead ID
- payload json - An object containing the Contacts module to be created as part of the conversion, along with (optionally) any modules that this new Contact record is to be related to.
Return Type
- json|error - ok
getLeadFreeBusySchedule
function getLeadFreeBusySchedule(string 'record) returns FreeBusyData|error
Retrieve a list of calendar event start and end times for specified person
Parameters
- 'record string - Lead ID
Return Type
- FreeBusyData|error - ok
createLeadRecord
function createLeadRecord() returns InlineResponse200|error
Create lead
Return Type
- InlineResponse200|error - ok
listFilteredRelatedRecords
function listFilteredRelatedRecords(string linkName, string 'record, string[]? filter, string? maxNum, string? offset, string? fields, string? orderBy, string? view) returns FilteredRecordDetails|error
Lists related filtered records.
Parameters
- linkName string - a link name
- 'record string - a record name
- filter string[]? (default ()) - The [filter expression](https://support.sugarcrm.com/Documentation/Sugar_Developer/Sugar_Developer_Guide_11.3/Integration/Web_Services/REST_API/End
- maxNum string? (default ()) - A maximum number of records to return. The default is all records.
- offset string? (default ()) - The number of records to skip over before records are returned. The default is 0. The parameter will be ignored if the max_num parameter is missing.
- fields string? (default ()) - Comma delimited list of fields to return. The field date_modified will always be returned.
name,account_type,description
- orderBy string? (default ()) - How to sort the returned records, in a comma delimited list with the direction appended to the column name after a colon.
name:DESC,account_type:DESC,date_modified:ASC
- view string? (default ()) - Instead of defining the fields argument, the view argument can be used instead. The field list is constructed at the server side based on the view definition which is requested. This argument can be used in combination with the fields argument.
Return Type
getHomeActivities
function getHomeActivities(string? maxNum, string? offset) returns FilteredRecordDetails|error
Get a filtered list of homepage activities for a user
Parameters
- maxNum string? (default ()) - A maximum number of records to return. The default is all records.
- offset string? (default ()) - The number of records to skip over before records are returned. The default is 0. The parameter will be ignored if the max_num parameter is missing.
Return Type
getAwsConfig
Get configuration values for Amazon Web Services. This is only available to administrators of Sugar Serve.
setAwsConfig
Set configuration values for Amazon Web Services. This is only available to administrators of Sugar Serve.
Parameters
- payload AWSConfig - Amazon Web Services configs
getConfig
Get configuration values for a given category. This is only available to administrators.
Parameters
- category string - The category
Return Type
- json|error - ok
setConfig
Set configuration values for a given category. This is only available to administrators.
Parameters
- category string - The category
Return Type
- json|error - ok
Records
sugarcrm: AWSConfig
Fields
- aws_connect_instance_name string? - The name of the AWS Connect instance
- aws_connect_region string? - The region assigned to the AWS Connect instance
sugarcrm: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
sugarcrm: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth OAuth2PasswordGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
sugarcrm: DashboardData
Fields
- dashboard_module string? - The module dashboard belongs to (e.g. Home, Cases, Accounts)
- dashboard string? - The dashboard name (e.g. portal-home)
sugarcrm: FileUploadResponse
Fields
- file_install string? -
- unFile string? -
sugarcrm: FilteredRecordDetails
Fields
- next_offset int? - Displays the next offset for retrieval of additional results. -1 will be returned when there are no more records.
- records anydata[]? - An array of results containing matched records
sugarcrm: FilterOptions
Fields
- filter record {}[]? - The filter expression.
- filter_id string? - Identifier for a preexisting filter. If filter is also set, the two filters are joined with an AND.
- max_num int? - A maximum number of records to return. Default is 20.
- offset int? - The number of records to skip over before records are returned. Default is 0.
- fields string? - Comma delimited list of fields to return. The field date_modified will always be returned. This argument can be combined with the view argument. Example
name,account_type,description
- view string? - Instead of defining the fields argument, the view argument can be used instead. The field list is constructed at the server side based on the view definition which is requested. This argument can be used in combination with the fields argument. Common views are "record" and "list". Example-
record
- order_by string? - How to sort the returned records, in a comma delimited list with the direction appended to the column name after a colon. Example-
name:DESC,account_type:DESC,date_modified:ASC
- q string? - A search expression, will search on this module. Cannot be used at the same time as a filter expression or id.
- deleted boolean? - Boolean to show deleted records in the result set.
sugarcrm: FreeBusyData
Fields
- id string? -
- module string? -
- freebusy FreeBusySchedule[]? -
sugarcrm: FreeBusySchedule
Fields
- 'start string? -
- end string? -
sugarcrm: InlineResponse200
Fields
- modules record {}? -
sugarcrm: LicenseLimitData
Fields
- default_limit int? -
- limit_enforced int? -
- default_license_type string? -
- seats LicenselimitdataSeats? -
- available_seats LicenselimitdataSeats? -
sugarcrm: LicenselimitdataSeats
Fields
- CURRENT int? -
- SUGAR_SERVE int? -
- SUGAR_SELL int? -
sugarcrm: OAuth2PasswordGrantConfig
OAuth2 Password Grant Configs
Fields
- Fields Included from *OAuth2PasswordGrantConfig
- tokenUrl string
- username string
- password string
- clientId string
- clientSecret string
- scopes string|string[]
- refreshConfig RefreshConfig|"INFER_REFRESH_CONFIG"
- defaultTokenExpTime decimal
- clockSkew decimal
- optionalParams map<string>
- credentialBearer CredentialBearer
- clientConfig ClientConfiguration
- tokenUrl string(default "https:/<site_url>/rest/<version>/oauth2/token") - Token URL
sugarcrm: Package
Fields
- name string? -
- 'version string? -
- 'type string? -
- published_date string? -
- description string? -
- uninstallable boolean? -
- file_install string? -
- file string? -
- enabled string? -
- id string? -
- installed boolean? -
- unFile string? -
sugarcrm: PackageManagerList
Fields
- packages Package[]? -
sugarcrm: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
sugarcrm: SearchBackendStatus
Fields
- available boolean? -
- enabled_modules string[]? -
sugarcrm: SearchOptions
Fields
- max_num int? - A maximum number of records to return. Default is 20.
- offset int? - The number of records to skip over before records are returned. Default is 0.
- q string? - A search expression, will search on this module. Cannot be used at the same time as a filter expression or id.
- sort anydata[]? - Wether or not to return highlighted results. Default is true.
- highlights boolean? - A search expression, will search on this module. Cannot be used at the same time as a filter expression or id.
- module_list string? - Comma delimited list of modules to search. If omitted, all search enabled modules will be queried.
sugarcrm: SuccessResponseID
Fields
- id string? -
sugarcrm: SuccessResponseStatus
Fields
- success boolean? - The success status
Import
import ballerinax/sugarcrm;
Metadata
Released date: about 2 years ago
Version: 1.4.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 6
Current verison: 6
Weekly downloads
Keywords
Sales & CRM
Customer Relationship Management
Contributors
Dependencies