spotto
Module spotto
API
Definitions
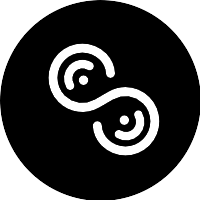
ballerinax/spotto Ballerina library
Overview
This is a generated connector for Spotto API v1.0.0 OpenAPI specification. The Spotto API provides a broad set of operations and resources that allow you to:
- Access and manage the Spotto registry of assets, locations and readers
- Control levels of access to Spotto applications and APIs across your account
- Query the Spotto event log to gain valuable insights into your historical data
- Setup event based hooks when something significant changes in Spotto.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Spotto account
- Obtain tokens by following this guide
Quickstart
To use the Spotto connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/spotto
module into the Ballerina project.
import ballerinax/spotto;
Step 2: Create a new connector instance
Create a spotto:ApiKeysConfig
with the API key obtained, and initialize the connector with it.
spotto:ApiKeysConfig config = { xApiKey: "<API_KEY>" } spotto:Client baseClient = check new Client(config);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to get the list of assets that belong to your account using the connector.
Get the list of assets that belong to your account
public function main() { spotto:GetAssetsResponse response = check baseClient->getAssets(); log:printInfo(response.toString()); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
spotto: Client
This is a generated connector for Spotto API v1.0.0 OpenAPI specification. The Spotto API provides a broad set of operations and resources that allow you to:
- Access and manage the Spotto registry of assets, locations and readers
- Control levels of access to Spotto applications and APIs across your account
- Query the Spotto event log to gain valuable insights into your historical data
- Setup event based hooks when something significant changes in Spotto.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Spotto account and obtain tokens by following this guide.
init (ApiKeysConfig apiKeyConfig, ConnectionConfig config, string serviceUrl)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.spotto.cloud" - URL of the target service
getAssets
function getAssets(float? page, float? 'limit, string[]? ids, string[]? tagIds, boolean? hasTags, boolean? archived, SearchableSortFields? sort, SortOrders? sortOrder, string? search, boolean? searchFuzzy, AssetSearchField[]? searchFields, AssetEmbedField[]? embed) returns GetAssetsResponse|error
Get the list of assets that belong to your account. This request will only return the first 20 assets,
unless otherwise specified using the pagination
parameters below. To enrich the response with
additional data such as tag identifiers, location state and created/updated timestamps, check out
the embed
parameter.
Parameters
- page float? (default ()) - Pagination: What page of results, assuming the limit (defaulting to 20) to start from. The default is page 0 (the first page of results). e.g. With a limit of 20, page 1 gets us results 20-39 (with zero based indexing).
- 'limit float? (default ()) - Pagination: Max number of results to return in the request. The default is 20.
- ids string[]? (default ()) - Lookup specific assets by passing an array of valid Spotto IDs.
- tagIds string[]? (default ()) - Lookup assets with specific tag IDs e.g. Beacon MAC addresses and RFID EPC codes.
- hasTags boolean? (default ()) - Only return assets that have tags registered.
- archived boolean? (default ()) - Return deleted assets, the default is false.
- sort SearchableSortFields? (default ()) - Sorting: Which field the results are to be sorted by. Use in combination with
sortOrder
, the default is ascending.
- sortOrder SortOrders? (default ()) - Sorting: Whether to sort the results in ascending or descending order. Use in combination with
sort
to determine the field this is acting on.
- search string? (default ()) - Search query string, for lucene based full text search.
- searchFuzzy boolean? (default ()) - Whether or not to run the search in fuzzy mode. The level of fuzziness depends on the character length of search terms: - 1-2: Must match exactly. - 3-5: Off by at most 1 character. - 6+: Off by at most 2 characters.
- searchFields AssetSearchField[]? (default ()) - Reduce the scope of fields to perform the search on. When left blank it will search all of these fields.
- embed AssetEmbedField[]? (default ()) - Attach additional asset information: - meta includes basic CRUD status information. - tags includes the tag IDs connected to this asset. - lastLocation includes the last known time and location the asset was seen.
Return Type
- GetAssetsResponse|error - 200 response
postAssets
function postAssets(PostAssetsRequest payload) returns PostAssetsResponse|error
Add one or more assets into the Spotto Asset registry. You can pass either a single object,
or an array of objects. If you already know the ID of one or more tags that will be used
to identify this asset, you can add them to the tagIds
array.
Parameters
- payload PostAssetsRequest - PostAssets request
Return Type
- PostAssetsResponse|error - 200 response
getAsset
function getAsset(string id) returns GetAssetResponse|error
Get the detailed information of a particular asset. Unlike the GetAssets request, this automatically attaches location state information, tag identifiers and created/updated timestamps.
Parameters
- id string - Spotto ID for this object, should look something like this:
6161acddc5a257c8c7d6b60e
Return Type
- GetAssetResponse|error - 200 response
updateAsset
function updateAsset(string id, UpdateAssetRequest payload) returns GetAssetResponse|error
To update asset information, we utilise a PATCH request to support partial updates. This enables any number of fields to be updated in a single request.
Parameters
- id string - Spotto ID for this object, should look something like this:
6161acddc5a257c8c7d6b60e
- payload UpdateAssetRequest - UpdateAsset request
Return Type
- GetAssetResponse|error - 200 response
getAssetLastLocation
function getAssetLastLocation(string id) returns GetAssetLastLocationResponse|error
Fetch the last location of a given asset. This is essentially the same as the GetAsset request, however is optimised to return faster without resolving the additional information.
Parameters
- id string - Spotto ID for this object, should look something like this:
6161acddc5a257c8c7d6b60e
Return Type
- GetAssetLastLocationResponse|error - 200 response
getEvents
function getEvents(float? page, float? 'limit, string[]? readers, string[]? locations, string[]? assets, EventType[]? 'type, float? timeFrom, float? timeTo, SortOrders? sortOrder) returns GetEventsResponse|error
Get the list of all events for your organisations. This request will only
return the first 20 events, unless otherwise specified using the pagination
parameters below. To enrich the response with the names of any connected entities,
check out the embed
parameter.
⚠️ Warning: Embedding the names of entities significantly impacts query time if the
limit
is adjusted to return large quantities of events.
Parameters
- page float? (default ()) - Pagination: What page of results, assuming the limit (defaulting to 20) to start from. The default is page 0 (the first page of results). e.g. With a limit of 20, page 1 gets us results 20-39 (with zero based indexing).
- 'limit float? (default ()) - Pagination: Max number of results to return in the request. The default is 20.
- readers string[]? (default ()) - Lookup events for specific readers by passing an array of valid Spotto IDs.
- locations string[]? (default ()) - Lookup events for specific locations by passing an array of valid Spotto IDs.
- assets string[]? (default ()) - Lookup events for specific assets by passing an array of valid Spotto IDs.
- 'type EventType[]? (default ()) - Lookup events for specific event types, e.g. only show Reader Online/Offline events.
- timeFrom float? (default ()) - Filter events from a given timestamp. It will yield events up until the current server time, unless otherwise specified by
timeTo
.
- timeTo float? (default ()) - Filter events until a given timestamp. It will yield all events prior to the specified time, unless otherwise specified by
timeFrom
.
- sortOrder SortOrders? (default ()) - Sorting: Whether to sort the results in ascending or descending order. Use in combination with
sort
to determine the field this is acting on.
Return Type
- GetEventsResponse|error - 200 response
getMQTTSettings
function getMQTTSettings() returns MQTTSettings|error
Get the credentials required to connect to the Spotto MQTT broker. There is only a single set of credentials for your account, however you may connect many MQTT clients by using the same username/password.
Your MQTT credentials should be enabled by default, but if for any reason the request returns without credentials, get in touch and we'll get you online.
Return Type
- MQTTSettings|error - 200 response
getLevels
function getLevels() returns GetLevelsResponse|error
Gets the list of levels connected to your organisation. Levels allow locations to be categorised and helps Readers better assign locations to assets, especially when readers between floors are competing for the best signal.
Return Type
- GetLevelsResponse|error - 200 response
putLevels
function putLevels(PutLevelsRequest payload) returns PutLevelsResponse|error
Updates the entire list of levels in your account. Levels allow locations to be categorised and helps Readers better assign locations to assets, especially when readers between floors are competing for the best signal.
⚠️ Warning: This will overwrite the existing list of Levels, so make sure that you're starting with the current list of Levels, using the GetLevels request.
Parameters
- payload PutLevelsRequest - PutLevels request
Return Type
- PutLevelsResponse|error - 200 response
postLevel
function postLevel(PostLevelRequest payload) returns PostLevelResponse|error
Adds a single level to your account. Levels allow locations to be categorised and helps Readers better assign locations to assets, especially when readers between floors are competing for the best signal.
Parameters
- payload PostLevelRequest - PostLevel request
Return Type
- PostLevelResponse|error - 201 response
getLocations
function getLocations(float? page, float? 'limit, string[]? ids, string[]? tagIds, string[]? levels, boolean? hasTags, boolean? hasReaders, boolean? setupComplete, boolean? archived, SearchableSortFields? sort, SortOrders? sortOrder, string? search, boolean? searchFuzzy, LocationSearchField[]? searchFields, LocationEmbedField[]? embed) returns GetLocationsResponse|error
Get the list of locations that belong to your account. This request will only return the first 20 locations,
unless otherwise specified using the pagination
parameters below. To enrich the response with
additional data such as tag identifiers, connected readers and created/updated timestamps, check out
the embed
parameter.
Parameters
- page float? (default ()) - Pagination: What page of results, assuming the limit (defaulting to 20) to start from. The default is page 0 (the first page of results). e.g. With a limit of 20, page 1 gets us results 20-39 (with zero based indexing).
- 'limit float? (default ()) - Pagination: Max number of results to return in the request. The default is 20.
- ids string[]? (default ()) - Lookup specific locations by passing an array of valid Spotto IDs.
- tagIds string[]? (default ()) - Lookup locations with specific tag IDs e.g. Beacon MAC addresses and RFID EPC codes.
- levels string[]? (default ()) - Lookup locations at specific floor levels, e.g. Ground and Level 1 ONLY.
- hasTags boolean? (default ()) - Only return locations that have tags registered.
- hasReaders boolean? (default ()) - Only return locations that have readers attached.
- setupComplete boolean? (default ()) - Only return locations that either have tags OR readers attached
- archived boolean? (default ()) - Return deleted locations, the default is false.
- sort SearchableSortFields? (default ()) - Sorting: Which field the results are to be sorted by. Use in combination with
sortOrder
, the default is ascending.
- sortOrder SortOrders? (default ()) - Sorting: Whether to sort the results in ascending or descending order. Use in combination with
sort
to determine the field this is acting on.
- search string? (default ()) - Search query string, for lucene based full text search.
- searchFuzzy boolean? (default ()) - Whether or not to run the search in fuzzy mode. The level of fuzziness depends on the character length of search terms: - 1-2: Must match exactly. - 3-5: Off by at most 1 character. - 6+: Off by at most 2 characters.
- searchFields LocationSearchField[]? (default ()) - Reduce the scope of fields to perform the search on. When left blank it will search all of these fields.
- embed LocationEmbedField[]? (default ()) - Attach additional location information: - meta includes basic CRUD status information. - tags includes the tag IDs connected to this location. - readers includes any readers configured at the given location.
Return Type
- GetLocationsResponse|error - 200 response
postLocations
function postLocations(PostLocationsRequest payload) returns PostLocationsResponse|error
Add one or more locations into the Spotto Location registry. You can pass either a single object,
or an array of objects. If you already know the ID of one or more tags that will be used
to identify this location, you can add them to the tagIds
array.
Parameters
- payload PostLocationsRequest - PostLocations request
Return Type
- PostLocationsResponse|error - 200 response
getLocation
function getLocation(string id) returns GetLocationResponse|error
Get the detailed information of a particular location. Unlike the GetLocations request, this automatically attaches tag identifiers, any connected readers and created/updated timestamps.
Parameters
- id string - Spotto ID for this object, should look something like this:
6161acddc5a257c8c7d6b60e
Return Type
- GetLocationResponse|error - 200 response
updateLocation
function updateLocation(string id, UpdateLocationRequest payload) returns GetLocationResponse|error
To update location information, we utilise a PATCH request to support partial updates. This enables any number of fields to be updated in a single request.
Parameters
- id string - Spotto ID for this object, should look something like this:
6161acddc5a257c8c7d6b60e
- payload UpdateLocationRequest - UpdateLocation request
Return Type
- GetLocationResponse|error - 200 response
getLocationInventory
Fetches the list of assets that were last seen at this location. Use the timestamps against each item in the response to determine if the asset is still being seen at this location.
Parameters
- id string - Spotto ID for this object, should look something like this:
6161acddc5a257c8c7d6b60e
Return Type
- GetLocationInventoryResponse|error - 200 response
getReaders
function getReaders(float? page, float? 'limit, string[]? ids, string[]? locationIds, string[]? deviceIds, ReaderType[]? types, DeviceType[]? deviceTypes, boolean? archived, SearchableSortFields? sort, SortOrders? sortOrder, string? search, boolean? searchFuzzy, ReaderSearchField[]? searchFields, ReaderEmbedField[]? embed) returns GetReadersResponse|error
Get the list of readers that belong to your account. This request will only return the first 20 readers,
unless otherwise specified using the pagination
parameters below. To enrich the response with
additional data such as reader state information and created/updated timestamps, check out
the embed
parameter.
Parameters
- page float? (default ()) - Pagination: What page of results, assuming the limit (defaulting to 20) to start from. The default is page 0 (the first page of results). e.g. With a limit of 20, page 1 gets us results 20-39 (with zero based indexing).
- 'limit float? (default ()) - Pagination: Max number of results to return in the request. The default is 20.
- ids string[]? (default ()) - Lookup specific readers by passing an array of valid Spotto IDs.
- locationIds string[]? (default ()) - Lookup readers that are positioned at specific location IDs
- deviceIds string[]? (default ()) - Lookup specific readers by passing an array of device IDs. These are typically the MAC address of the reader.
- types ReaderType[]? (default ()) - Filter based on the reader behavioural type.
- deviceTypes DeviceType[]? (default ()) - Lookup readers belonging to specific device types.
- archived boolean? (default ()) - Return deleted readers, the default is false.
- sort SearchableSortFields? (default ()) - Sorting: Which field the results are to be sorted by. Use in combination with
sortOrder
, the default is ascending.
- sortOrder SortOrders? (default ()) - Sorting: Whether to sort the results in ascending or descending order. Use in combination with
sort
to determine the field this is acting on.
- search string? (default ()) - Search query string, for Lucene based full text search.
- searchFuzzy boolean? (default ()) - Whether or not to run the search in fuzzy mode. The level of fuzziness depends on the character length of search terms: - 1-2: Must match exactly. - 3-5: Off by at most 1 character. - 6+: Off by at most 2 characters.
- searchFields ReaderSearchField[]? (default ()) - Reduce the scope of fields to perform the search on. When left blank it will search all of these fields.
- embed ReaderEmbedField[]? (default ()) - Attach additional reader information: - meta includes basic CRUD status information. - status includes dynamic status information about the reader such as the time it came online and when we last heard from it.
Return Type
- GetReadersResponse|error - 200 response
postReaders
function postReaders(PostReadersRequest payload) returns PostReadersResponse|error
Add one or more readers into the Spotto Reader registry. You can pass either a single object, or an array of objects. Adding readers into the system requires a bit more information than Assets or Locations. See below for more details.
Parameters
- payload PostReadersRequest - PostReaders request
Return Type
- PostReadersResponse|error - 200 response
getReader
function getReader(string id) returns GetReaderResponse|error
Get the detailed information of a particular reader. Unlike the GetReaders request, this automatically attaches reader state information and created/updated timestamps.
Parameters
- id string - Spotto ID for this object, should look something like this:
6161acddc5a257c8c7d6b60e
Return Type
- GetReaderResponse|error - 200 response
updateReader
function updateReader(string id, UpdateReaderRequest payload) returns GetReaderResponse|error
To update reader information, we utilise a PATCH request to support partial updates. This enables any number of fields to be updated in a single request.
Parameters
- id string - Spotto ID for this object, should look something like this:
6161acddc5a257c8c7d6b60e
- payload UpdateReaderRequest - UpdateReader request
Return Type
- GetReaderResponse|error - 200 response
getReaderInventory
Fetches the list of assets that were last seen by this reader. Use the timestamps against each item in the response to determine if the asset is still being seen by this reader. You can pass in an optional query to set the threshold for how recent assets must be seen to be included in the inventory.
Parameters
- id string - Spotto ID for this object, should look something like this:
6161acddc5a257c8c7d6b60e
- windowSize float? (default ()) - Time in seconds to consider assets to be within the readers inventory
Return Type
- GetReaderInventoryResponse|error - 200 response
getReaderStatus
function getReaderStatus(string id) returns GetReaderStatusResponse|error
Fetch the status of a given reader. This is essentially the same as the GetReader request, however is optimised to return faster without resolving the additional information.
Parameters
- id string - Spotto ID for this object, should look something like this:
6161acddc5a257c8c7d6b60e
Return Type
- GetReaderStatusResponse|error - 200 response
getRoles
function getRoles(StandardSortFields? sort, SortOrders? sortOrder, RoleEmbedField[]? embed) returns GetRolesResponse|error
Get the list of roles that belong to your account. This request will return all roles, without pagination.
It will typically only return the default roles that your account has access e.g. if you are 'Admin' it will
return 'Admin', 'Power User' and 'User'. If your account has custom roles it will return these instead.
Get in touch if you need custom fine grained access control for your account.
To enrich the response with additional data such as created/updated timestamps, check out the embed
parameter.
Parameters
- sort StandardSortFields? (default ()) - Sorting: Which field the results are to be sorted by. Use in combination with sortOrder, the default is ascending.
- sortOrder SortOrders? (default ()) - Sorting: Whether to sort the results in ascending or descending order. Use in combination with sort to determine the field this is acting on.
- embed RoleEmbedField[]? (default ()) - Attach additional user information: meta includes basic CRUD status information.
Return Type
- GetRolesResponse|error - 200 response
getTriggers
function getTriggers(float? page, float? 'limit, string[]? ids, boolean? enabled, TriggerType? 'type, StandardSortFields? sort, SortOrders? sortOrder, TriggerEmbedField[]? embed) returns GetTriggersResponse|error
Get the list of triggers that belong to your account. This request will only return the first 20 triggers,
unless otherwise specified using the pagination
parameters below. To enrich the response with
additional data such as the created/updated timestamps, check out the embed
parameter.
Parameters
- page float? (default ()) - Pagination: What page of results, assuming the limit (defaulting to 20) to start from. The default is page 0 (the first page of results). e.g. With a limit of 20, page 1 gets us results 20-39 (with zero based indexing).
- 'limit float? (default ()) - Pagination: Max number of results to return in the request. The default is 20.
- ids string[]? (default ()) - Lookup specific triggers by passing an array of valid Spotto IDs.
- enabled boolean? (default ()) - Lookup triggers that are only enabled or disabled.
- 'type TriggerType? (default ()) - Filter based on the trigger type.
- sort StandardSortFields? (default ()) - Sorting: Which field the results are to be sorted by. Use in combination with
sortOrder
, the default is ascending.
- sortOrder SortOrders? (default ()) - Sorting: Whether to sort the results in ascending or descending order. Use in combination with
sort
to determine the field this is acting on.
- embed TriggerEmbedField[]? (default ()) - Attach additional trigger information: - meta includes basic CRUD status information.
Return Type
- GetTriggersResponse|error - 200 response
postTriggers
function postTriggers(PostTriggersRequest payload) returns PostTriggersResponse|error
Add one or more triggers into the Spotto Trigger registry. You can pass either a single object, or an array of objects. Triggers allow people and systems outside of Spotto to be notified when something significant occurs in Spotto. We call these significant state changes Events (see GetEvents) that we can hook into using Triggers. Adding a trigger requires us to choose which Event type we want to respond to. Each event type will have a different set of rules we can apply. These are explained below:
- WentOffline: Spotto hasn't heard from a reader in a while so is transitioned to offline.
- WentOnline: Spotto heard from a reader that hadn't reported in a while so was transitioned to online.
- ArrivedAt: An asset moved to a specific location or group of locations.
- Moved: An asset moved to any location.
Each trigger will have subjectRules
which allow us to filter the subject of the event either by name or ID.
Matching on name uses a case insensitive regular expression so we can match on multiple objects that share
similar names e.g. 'chair' could match names 'Wheelchair', 'M1 Shower Chair'.
The subject of WentOffline and WentOnline events are always the Reader (that's turning online/offline).
The subject of ArrivedAt and Moved can either be an Asset, or a ROVING reader moving locations.
ArrivedAt triggers also call for locationRules
which allow us to specify either the name or ID of locations.
These operate in the same way as subjectRules
and allow us to filter the range of locations that cause the trigger to fire.
We must also specify actions
that fire when all the conditions are satisfied. We can add multiple actions to a trigger.
Parameters
- payload PostTriggersRequest - PostTriggers request
Return Type
- PostTriggersResponse|error - 200 response
getTrigger
function getTrigger(string id) returns GetTriggerResponse|error
Get the detailed information of a particular trigger. Unlike the GetTriggers request, this automatically attaches the created/updated timestamps.
Parameters
- id string - Spotto ID for this object, should look something like this:
6161acddc5a257c8c7d6b60e
Return Type
- GetTriggerResponse|error - 200 response
deleteTrigger
function deleteTrigger(string id) returns DeleteResponse|error
Unlike a number of the other requests, triggers does not have an impact on the event log so we allow the permanent deletion of triggers. It takes no query parameters or body, and returns with the standard REST deletion response of an empty object.
Parameters
- id string - Spotto ID for this object, should look something like this:
6161acddc5a257c8c7d6b60e
Return Type
- DeleteResponse|error - 200 response
updateTrigger
function updateTrigger(string id, UpdateTriggerRequest payload) returns GetTriggerResponse|error
To update trigger information, we utilise a PATCH request to support partial updates. This enables any number of fields to be updated in a single request.
Parameters
- id string - Spotto ID for this object, should look something like this:
6161acddc5a257c8c7d6b60e
- payload UpdateTriggerRequest - UpdateTrigger request
Return Type
- GetTriggerResponse|error - 200 response
getUsers
function getUsers(float? page, float? 'limit, string[]? ids, string[]? role, UserType? 'type, StandardSortFields? sort, SortOrders? sortOrder, UserEmbedField[]? embed) returns GetUsersResponse|error
Get the list of users that belong to your account. This request will only return the first 20 users,
unless otherwise specified using the pagination
parameters below. To enrich the response with
additional data such as the created/updated timestamps, check out the embed
parameter.
Parameters
- page float? (default ()) - Pagination: What page of results, assuming the limit (defaulting to 20) to start from. The default is page 0 (the first page of results). e.g. With a limit of 20, page 1 gets us results 20-39 (with zero based indexing).
- 'limit float? (default ()) - Pagination: Max number of results to return in the request. The default is 20.
- ids string[]? (default ()) - Lookup specific users by passing an array of valid Spotto IDs.
- role string[]? (default ()) - Lookup users belonging to specific roles by passing an array of valid Spotto IDs.
- 'type UserType? (default ()) - Lookup only internal or external API users.
- sort StandardSortFields? (default ()) - Sorting: Which field the results are to be sorted by. Use in combination with
sortOrder
, the default is ascending.
- sortOrder SortOrders? (default ()) - Sorting: Whether to sort the results in ascending or descending order. Use in combination with
sort
to determine the field this is acting on.
- embed UserEmbedField[]? (default ()) - Attach additional user information: - meta includes basic CRUD status information.
Return Type
- GetUsersResponse|error - 200 response
postUser
function postUser(PostUserRequest payload) returns GetUserResponse|error
Add one or more users into the Spotto User registry. You can only send a single object at a time as there a number of provisioning steps that must occur per user.
You can add EXTERNAL users to create additional API users of varying access levels by setting the role
.
EXTERNAL users cannot access Spotto applications and only have access to the Spotto REST API.
INTERNAL users have access to Spotto applications and require an email address for logging in. A random password will be generated and sent to the elected address, unless a custom password is specified. INTERNAL users cannot access the Spotto API, instead an EXTERNAL user must be created.
Parameters
- payload PostUserRequest - PostUser request
Return Type
- GetUserResponse|error - 200 response
getUser
function getUser(string id, boolean? withKey) returns GetUserResponse|error
Get the detailed information of a particular user. Unlike the GetUsers request, this automatically attaches the created/updated timestamps.
Parameters
- id string - Spotto ID for this object, should look something like this:
6161acddc5a257c8c7d6b60e
- withKey boolean? (default ()) - If you have the API admin permissions you can view the API key of external users.
Return Type
- GetUserResponse|error - 200 response
deleteUser
function deleteUser(string id) returns DeleteResponse|error
Unlike a number of the other requests, users do not have an impact on the event log so we allow the permanent deletion of users. It takes no query parameters or body, and returns with the standard REST deletion response of an empty object.
Parameters
- id string - Spotto ID for this object, should look something like this:
6161acddc5a257c8c7d6b60e
Return Type
- DeleteResponse|error - 200 response
updateUser
function updateUser(string id, UpdateUserRequest payload) returns GetUserResponse|error
To update user information, we utilise a PATCH request to support partial updates. This enables any number of fields to be updated in a single request.
Parameters
- id string - Spotto ID for this object, should look something like this:
6161acddc5a257c8c7d6b60e
- payload UpdateUserRequest - UpdateUser request
Return Type
- GetUserResponse|error - 200 response
getKey
function getKey(string id) returns GetKeyResponse|error
Access the API key for a specific EXTERNAL user. This request takes no query parameters.
Parameters
- id string - Spotto ID for this object, should look something like this:
6161acddc5a257c8c7d6b60e
Return Type
- GetKeyResponse|error - 200 response
Records
spotto: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- xApiKey string - To authenticate API requests, Spotto uses a header based API key scheme. To gain access to the Spotto REST API,
simply set the
x-api-key
header to an API Key associated with your account. If you don't have an API key, follow this guide to get one. Include the header in all API calls, otherwise you will receive a403 Forbidden
response.
spotto: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
spotto: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
spotto: DefaultError
Fields
- message string -
spotto: DefaultReaderRequest
Fields
- name string - Name of the new reader, try to be descriptive to make it easy for people find it when searching. <br> This field is optional for FIXED readers, see IFixedReaderRequest.
- 'type ReaderType - The reader behaviour type:
- FIXED - regular non-moving readers.
- ROVING - readers that move around and look for location tags, assigning nearby assets to the locations it sees.
- VIRTUAL - allow different antennas plugged into a reader to report as their own readers.
- PORTAL - similar to virtual but for differentiating 2 adjacent zones e.g. IN/OUT.
- deviceType DeviceType - The physical reader device type:
- B1 - Spotto B1 Bluetooth Reader.
- RF1A4 - Spotto RF1A4 4 Port RFID Reader.
- RF1F - Spotto RF1F FEIG Reader.
- HD1 - Spotto HD1 HID Reader.
- ZEBRA - Zebra RFID Reader.
- IMPINJ - Impinj RFID Reader.
- TURCK - Turck Handheld RFID Reader.
- OTHER - Custom Reader (BYO Device).
- APPLICATION - Spotto Detector Application.
- locationId string? - locationId is used for specifying an already existing location.
- location IUpsertedLocation? - If a location object is specified, a new location will be created and added to the locations list.
- deviceId string? - Reader Device ID. This will typically be a MAC address e.g. 00:11:22:33:44:55. <br> It is only applicable to IoTX3 readers.
- network INetworkRequest? - WiFi network credentials.
- saveNetwork boolean? - When this flag is true, it will save any provided WiFi details to the account. It will be ignored if no networks are present.
- antennas IAntennaRequest[]? - Additional antenna config. This is only applicable to VIRTUAL or PORTAL readers.
spotto: DeleteResponse
Standard deletion response is an empty object
spotto: EmailAction
Fields
- 'type string -
- address string - Email address of the trigger recipient.
spotto: GetArrivedAtTriggerResponse
Asset or Reader moved in relation to selected locations
Fields
- 'type string - Type
- locationRules Rule[] - Location rules filter the possible events that cause this trigger to fire.
- id string - Unique ID of the Trigger.
- name string - The human readable name of the trigger, for easily identifying the trigger and its purpose.
- enabled boolean - Status of the trigger, disabled triggers will not fire.
- actions Action[] - The list of actions that fire for this trigger.
- subjectRules Rule[] - Subject rules filter the possible events that cause this trigger to fire.
- meta IEntityMeta? - Metadata, holding important timestamps of this object's lifecycle.
spotto: GetAssetLastLocationResponse
Fields
- id string - Unique ID of this Asset.
- lastLocation ILastLocation -
spotto: GetAssetResponse
Fields
- id string - Unique ID of this Asset.
- name string - Human readable asset name NOTE: We do not enforce unique names for assets, instead we rely on the
id
for uniqueness.
- tagIds string[]? - List of connected tag identifiers, these will typically be BLE tag MAC addresses e.g. 00:11:22:33:44:55.
- score float? - Lucene based search score, added as an optional meta field when searching.
- meta IEntityMeta? - Metadata, holding important timestamps of this object's lifecycle.
- lastLocation ILastLocation? -
spotto: GetAssetsQuery
Fields
- page float? - Pagination: What page of results, assuming the limit (defaulting to 20) to start from. The default is page 0 (the first page of results). e.g. With a limit of 20, page 1 gets us results 20-39 (with zero based indexing).
- 'limit float? - Pagination: Max number of results to return in the request. The default is 20.
- ids string[]? - Lookup specific assets by passing an array of valid Spotto IDs.
- tagIds string[]? - Lookup assets with specific tag IDs e.g. Beacon MAC addresses and RFID EPC codes.
- hasTags boolean? - Only return assets that have tags registered.
- archived boolean? - Return deleted assets, the default is false.
- sort SearchableSortFields? -
- sortOrder SortOrders? - Sort orders - ascending and descending
- search string? - Search query string, for lucene based full text search.
- searchFuzzy boolean? - Whether or not to run the search in fuzzy mode. The level of fuzziness
depends on the character length of search terms:
- 1-2: Must match exactly.
- 3-5: Off by at most 1 character.
- 6+: Off by at most 2 characters.
- searchFields AssetSearchField[]? - Reduce the scope of fields to perform the search on. When left blank it will search all of these fields.
- embed AssetEmbedField[]? - Attach additional asset information:
- meta includes basic CRUD status information.
- tags includes the tag IDs connected to this asset.
- lastLocation includes the last known time and location the asset was seen.
spotto: GetAssetsResponse
Fields
- query GetAssetsQuery -
- total float - Total number of records that match the query (without pagination).
- items GetAssetResponse[] - This is the list of paginated results, which will be an array of 20 items or less, unless another limit was specified in the query params.
spotto: GetEventResponse
Fields
- id string - Unique ID of this Event.
- timestamp float - Unix numeric timestamp (milliseconds) of when the event was recorded.
- 'type EventType -
- asset IEmbeddedEntity? -
- location IEmbeddedEntity? -
- reader IEmbeddedEntity? -
spotto: GetEventsQuery
Fields
- page float? - Pagination: What page of results, assuming the limit (defaulting to 20) to start from. The default is page 0 (the first page of results). e.g. With a limit of 20, page 1 gets us results 20-39 (with zero based indexing).
- 'limit float? - Pagination: Max number of results to return in the request. The default is 20.
- readers string[]? - Lookup events for specific readers by passing an array of valid Spotto IDs.
- locations string[]? - Lookup events for specific locations by passing an array of valid Spotto IDs.
- assets string[]? - Lookup events for specific assets by passing an array of valid Spotto IDs.
- 'type EventType[]? - Lookup events for specific event types, e.g. only show Reader Online/Offline events.
- timeFrom float? - Filter events from a given timestamp. It will yield events up until the
current server time, unless otherwise specified by
timeTo
.
- timeTo float? - Filter events until a given timestamp. It will yield all events prior to the specified time,
unless otherwise specified by
timeFrom
.
- sortOrder SortOrders? - Sort orders - ascending and descending
spotto: GetEventsResponse
Fields
- query GetEventsQuery -
- total float - Total number of records that match the query (without pagination).
- items GetEventResponse[] - This is the list of paginated results, which will be an array of 20 items or less, unless another limit was specified in the query params.
spotto: GetExternalUserResponse
Fields
- 'type string - User type for identifying external API users from internal users.
- 'key string? - The API key is returned when creating new EXTERNAL users, or where requested.
- id string - Unique Spotto ID assigned to this User.
- name string - Human readable full name or identifier of the User.
NOTE: We do not enforce unique names, we instead rely on
id
for uniqueness.
- role IEmbeddedEntity -
- organisation IEmbeddedEntity -
- meta IEntityMeta? - Metadata, holding important timestamps of this object's lifecycle.
spotto: GetInternalUserResponse
Fields
- 'type string - User type for identifying internal users from external API users.
- email string - The User's unique email address.
- id string - Unique Spotto ID assigned to this User.
- name string - Human readable full name or identifier of the User.
NOTE: We do not enforce unique names, we instead rely on
id
for uniqueness.
- role IEmbeddedEntity -
- organisation IEmbeddedEntity -
- meta IEntityMeta? - Metadata, holding important timestamps of this object's lifecycle.
spotto: GetKeyResponse
Fields
- 'key string - Access key for using the Spotto REST API. Insert this key into the
x-api-key
header, then call any request in this spec, provided the user this key is associated with has the correct permissions.
spotto: GetLevelsResponse
Fields
- levels string[] - List of levels saved against the account.
spotto: GetLocationResponse
Fields
- id string - Unique ID of this Location.
- name string - Human readable location name NOTE: We do not enforce unique names, instead relying on
id
for uniqueness.
- tagIds string[]? - List of connected tag identifiers, these will typically be BLE tag MAC addresses e.g. 00:11:22:33:44:55.
- readers IEmbeddedEntity[]? - List of readers configured at this location.
- meta IEntityMeta? - Metadata, holding important timestamps of this object's lifecycle.
spotto: GetLocationsQuery
Fields
- page float? - Pagination: What page of results, assuming the limit (defaulting to 20) to start from. The default is page 0 (the first page of results). e.g. With a limit of 20, page 1 gets us results 20-39 (with zero based indexing).
- 'limit float? - Pagination: Max number of results to return in the request. The default is 20.
- ids string[]? - Lookup specific locations by passing an array of valid Spotto IDs.
- tagIds string[]? - Lookup locations with specific tag IDs e.g. Beacon MAC addresses and RFID EPC codes.
- levels string[]? - Lookup locations at specific floor levels, e.g. Ground and Level 1 ONLY.
- hasTags boolean? - Only return locations that have tags registered.
- hasReaders boolean? - Only return locations that have readers attached.
- setupComplete boolean? - Only return locations that either have tags OR readers attached
- archived boolean? - Return deleted locations, the default is false.
- sort SearchableSortFields? -
- sortOrder SortOrders? - Sort orders - ascending and descending
- search string? - Search query string, for lucene based full text search.
- searchFuzzy boolean? - Whether or not to run the search in fuzzy mode. The level of fuzziness
depends on the character length of search terms:
- 1-2: Must match exactly.
- 3-5: Off by at most 1 character.
- 6+: Off by at most 2 characters.
- searchFields LocationSearchField[]? - Reduce the scope of fields to perform the search on. When left blank it will search all of these fields.
- embed LocationEmbedField[]? - Attach additional location information:
- meta includes basic CRUD status information.
- tags includes the tag IDs connected to this location.
- readers includes any readers configured at the given location.
spotto: GetLocationsResponse
Fields
- query GetLocationsQuery -
- total float - Total number of records that match the query (without pagination).
- items GetLocationResponse[] - This is the list of paginated results, which will be an array of 20 items or less, unless another limit was specified in the query params.
spotto: GetMovedTriggerResponse
Asset or Reader moved anywhere (No location rules)
Fields
- 'type string - Type
- id string - Unique ID of the Trigger.
- name string - The human readable name of the trigger, for easily identifying the trigger and its purpose.
- enabled boolean - Status of the trigger, disabled triggers will not fire.
- actions Action[] - The list of actions that fire for this trigger.
- subjectRules Rule[] - Subject rules filter the possible events that cause this trigger to fire.
- meta IEntityMeta? - Metadata, holding important timestamps of this object's lifecycle.
spotto: GetReaderResponse
Fields
- id string - Unique ID of this Reader.
- name string - Human readable reader name NOTE: We do not enforce unique names, instead we rely on
id
for uniqueness.
- 'type ReaderType - The reader behaviour type:
- FIXED - regular non-moving readers.
- ROVING - readers that move around and look for location tags, assigning nearby assets to the locations it sees.
- VIRTUAL - allow different antennas plugged into a reader to report as their own readers.
- PORTAL - similar to virtual but for differentiating 2 adjacent zones e.g. IN/OUT.
- deviceType DeviceType - The physical reader device type:
- B1 - Spotto B1 Bluetooth Reader.
- RF1A4 - Spotto RF1A4 4 Port RFID Reader.
- RF1F - Spotto RF1F FEIG Reader.
- HD1 - Spotto HD1 HID Reader.
- ZEBRA - Zebra RFID Reader.
- IMPINJ - Impinj RFID Reader.
- TURCK - Turck Handheld RFID Reader.
- OTHER - Custom Reader (BYO Device).
- APPLICATION - Spotto Detector Application.
- deviceId string? - Reader Device ID. This will typically be a MAC address e.g. 00:11:22:33:44:55.
- endpoint string - Endpoint this reader will POST reads to. This is only needed when manually setting up a reader, or when using a BYO device.
- network string? - SSID of the network the reader is connected to, if any.
- location ReaderLocation? - Reader Locations can either be: FIXED: Are static and don't have a timestamp<br> ROVING: Are dynamic and have a lastSeen timestamp
- meta IEntityMeta? - Metadata, holding important timestamps of this object's lifecycle.
- status IReaderStatus? - Reader online status information. Note: Other IoT style status information will ultimately end up here e.g. Temperature, WiFi signal, Battery level (for portable readers).
spotto: GetReadersQuery
Fields
- page float? - Pagination: What page of results, assuming the limit (defaulting to 20) to start from. The default is page 0 (the first page of results). e.g. With a limit of 20, page 1 gets us results 20-39 (with zero based indexing).
- 'limit float? - Pagination: Max number of results to return in the request. The default is 20.
- ids string[]? - Lookup specific readers by passing an array of valid Spotto IDs.
- locationIds string[]? - Lookup readers that are positioned at specific location IDs
- deviceIds string[]? - Lookup specific readers by passing an array of device IDs. These are typically the MAC address of the reader.
- types ReaderType[]? - Filter based on the reader behavioural type.
- deviceTypes DeviceType[]? - Lookup readers belonging to specific device types.
- archived boolean? - Return deleted readers, the default is false.
- sort SearchableSortFields? -
- sortOrder SortOrders? - Sort orders - ascending and descending
- search string? - Search query string, for Lucene based full text search.
- searchFuzzy boolean? - Whether or not to run the search in fuzzy mode. The level of fuzziness
depends on the character length of search terms:
- 1-2: Must match exactly.
- 3-5: Off by at most 1 character.
- 6+: Off by at most 2 characters.
- searchFields ReaderSearchField[]? - Reduce the scope of fields to perform the search on. When left blank it will search all of these fields.
- embed ReaderEmbedField[]? - Attach additional reader information:
- meta includes basic CRUD status information.
- status includes dynamic status information about the reader such as the time it came online and when we last heard from it.
spotto: GetReadersResponse
Fields
- query GetReadersQuery -
- total float - Total number of records that match the query (without pagination).
- items GetReaderResponse[] - This is the list of paginated results, which will be an array of 20 items or less, unless another limit was specified in the query params.
spotto: GetReaderStatusResponse
Fields
- location IRovingReaderLocation? -
- status IReaderStatus - Reader online status information. Note: Other IoT style status information will ultimately end up here e.g. Temperature, WiFi signal, Battery level (for portable readers).
spotto: GetRoleResponse
Fields
- id string - Unique Spotto ID of the role.
- meta IEntityMeta? - Metadata, holding important timestamps of this object's lifecycle.
- name string - Human readable name of the role.
- description string - Short description of the role including the scope of access.
- permissions string[] - List of permissions this role has access to, following the entity:action pattern.
- default boolean? - Whether or not this role is a default system role, available to all organisations.
spotto: GetRolesQuery
Fields
- sort StandardSortFields? - Common sort fields across most GET endpoints
- sortOrder SortOrders? - Sort orders - ascending and descending
- embed RoleEmbedField[]? - Attach additional user information: meta includes basic CRUD status information.
spotto: GetRolesResponse
Fields
- query GetRolesQuery -
- total float - Total number of records that match the query (without pagination).
- items GetRoleResponse[] - This is the list of paginated results, which will be an array of 20 items or less, unless another limit was specified in the query params.
spotto: GetTriggersQuery
Fields
- page float? - Pagination: What page of results, assuming the limit (defaulting to 20) to start from. The default is page 0 (the first page of results). e.g. With a limit of 20, page 1 gets us results 20-39 (with zero based indexing).
- 'limit float? - Pagination: Max number of results to return in the request. The default is 20.
- ids string[]? - Lookup specific triggers by passing an array of valid Spotto IDs.
- enabled boolean? - Lookup triggers that are only enabled or disabled.
- 'type TriggerType? - ==== TRIGGER TYPES ====
- sort StandardSortFields? - Common sort fields across most GET endpoints
- sortOrder SortOrders? - Sort orders - ascending and descending
- embed TriggerEmbedField[]? - Attach additional trigger information:
- meta includes basic CRUD status information.
spotto: GetTriggersResponse
Fields
- query GetTriggersQuery -
- total float - Total number of records that match the query (without pagination).
- items GetTriggerResponse[] - This is the list of paginated results, which will be an array of 20 items or less, unless another limit was specified in the query params.
spotto: GetUsersQuery
Fields
- page float? - Pagination: What page of results, assuming the limit (defaulting to 20) to start from. The default is page 0 (the first page of results). e.g. With a limit of 20, page 1 gets us results 20-39 (with zero based indexing).
- 'limit float? - Pagination: Max number of results to return in the request. The default is 20.
- ids string[]? - Lookup specific users by passing an array of valid Spotto IDs.
- role string[]? - Lookup users belonging to specific roles by passing an array of valid Spotto IDs.
- 'type UserType? -
- sort StandardSortFields? - Common sort fields across most GET endpoints
- sortOrder SortOrders? - Sort orders - ascending and descending
- embed UserEmbedField[]? - Attach additional user information:
- meta includes basic CRUD status information.
spotto: GetUsersResponse
Fields
- query GetUsersQuery -
- total float - Total number of records that match the query (without pagination).
- items GetUserResponse[] - This is the list of paginated results, which will be an array of 20 items or less, unless another limit was specified in the query params.
spotto: GetWentOfflineTriggerResponse
Reader went online after a given threshold
Fields
- 'type string - Type
- id string - Unique ID of the Trigger.
- name string - The human readable name of the trigger, for easily identifying the trigger and its purpose.
- enabled boolean - Status of the trigger, disabled triggers will not fire.
- actions Action[] - The list of actions that fire for this trigger.
- subjectRules Rule[] - Subject rules filter the possible events that cause this trigger to fire.
- meta IEntityMeta? - Metadata, holding important timestamps of this object's lifecycle.
spotto: GetWentOnlineTriggerResponse
Reader went online after a given threshold
Fields
- 'type string - Type
- id string - Unique ID of the Trigger.
- name string - The human readable name of the trigger, for easily identifying the trigger and its purpose.
- enabled boolean - Status of the trigger, disabled triggers will not fire.
- actions Action[] - The list of actions that fire for this trigger.
- subjectRules Rule[] - Subject rules filter the possible events that cause this trigger to fire.
- meta IEntityMeta? - Metadata, holding important timestamps of this object's lifecycle.
spotto: IAntennaRequest
Fields
- name string? -
- port float -
- locationId string? -
- location IantennarequestLocation? -
spotto: IantennarequestLocation
Fields
- name string -
- level string? -
spotto: IEmbeddedEntity
Fields
- id string - Unique Spotto ID of this object.
- name string - Human readable name of this object.
spotto: IEntityMeta
Metadata, holding important timestamps of this object's lifecycle.
Fields
- created float - Created timestamp
- updated float? - Last updated timestamp
- deleted float? - Deletion timestamp
spotto: IFixedReaderLocation
Fields
- 'type string -
- id string - Unique Spotto ID of this object.
- name string - Human readable name of this object.
spotto: IFixedReaderRequest
Fields
- 'type string -
- name string? - Name is optional for FIXED reader. This field is appended to the location name separated by a ' - '.
- deviceType DeviceType - The physical reader device type:
- B1 - Spotto B1 Bluetooth Reader.
- RF1A4 - Spotto RF1A4 4 Port RFID Reader.
- RF1F - Spotto RF1F FEIG Reader.
- HD1 - Spotto HD1 HID Reader.
- ZEBRA - Zebra RFID Reader.
- IMPINJ - Impinj RFID Reader.
- TURCK - Turck Handheld RFID Reader.
- OTHER - Custom Reader (BYO Device).
- APPLICATION - Spotto Detector Application.
- locationId string? - locationId is used for specifying an already existing location.
- location IUpsertedLocation? - If a location object is specified, a new location will be created and added to the locations list.
- deviceId string? - Reader Device ID. This will typically be a MAC address e.g. 00:11:22:33:44:55. <br> It is only applicable to IoTX3 readers.
- network INetworkRequest? - WiFi network credentials.
- saveNetwork boolean? - When this flag is true, it will save any provided WiFi details to the account. It will be ignored if no networks are present.
- antennas IAntennaRequest[]? - Additional antenna config. This is only applicable to VIRTUAL or PORTAL readers.
spotto: IInventoryItem
Fields
- firstSeen float - When the asset was first seen at this location.
- lastSeen float - When the asset was last seen at this location.
- id string - Unique Spotto ID of this object.
- name string - Human readable name of this object.
spotto: ILastLocation
Fields
- firstSeen float - When the asset was first seen at this location.
- lastSeen float - When the asset was last seen at this location.
- id string - Unique Spotto ID of this object.
- name string - Human readable name of this object.
spotto: INetworkRequest
WiFi network credentials.
Fields
- name string - SSID of the network.
- username string? - Optional network username, this is only required for WPA2 Enterprise networks.
- password string? - Optional network password, this is not required for open networks.
spotto: IReaderStatus
Reader online status information.
Note: Other IoT style status information will ultimately end up here e.g. Temperature, WiFi signal, Battery level (for portable readers).
Fields
- online boolean - Whether or not the reader is currently online.
- onlineTime float - Unix numeric timestamp (milliseconds) of when the reader came online.
- lastReportedTime float - Unix numeric timestamp (milliseconds) of when the reader last reported.
spotto: IRovingReaderLocation
Fields
- 'type string -
- firstSeen float - First time the location was seen by this reader
- lastSeen float - Last time the location was seen by this reader
- id string - Unique Spotto ID of this object.
- name string - Human readable name of this object.
spotto: IUpsertedLocation
If a location object is specified, a new location will be created and added to the locations list.
Fields
- name string - Name
- level string? - Level
spotto: MatchNameRule
Fields
- 'type string -
- 'match string - Case insensitive name or substring to match on the event subject.
spotto: MQTTAction
Fields
- 'type string -
- topic string - Custom MQTT topic to publish the event payload to.
spotto: MQTTDisabled
==== INTEGRATIONS ====
Fields
- enabled boolean - Enabled
spotto: MQTTEnabledWithDetails
Extends the base shape returned in the integrations list with additional information about the integration.
Fields
- password string - Password for accessing the Spotto MQTT broker.
- host string - Host URL of Spotto MQTT broker.
- rootTopic string - MQTT Topic that will subscribe to all events for this organisation.
- enabled boolean - Enabled
- username string - Username for accessing the Spotto MQTT broker.
spotto: PostArrivedAtTriggerRequest
Asset or Reader moved in relation to selected locations
Fields
- 'type string - Type
- locationRules Rule[] - Location rules filter the possible events that cause this trigger to fire.
- name string - The name of the trigger, for easily identifying the trigger and its purpose.
- actions Action[] - The list of actions that fire for this trigger.
- subjectRules Rule[] - Subject rules filter the possible events that cause this trigger to fire.
spotto: PostAssetRequest
Fields
- name string - Name of the new asset, try to be descriptive to make it easy for people find it when searching.
- tagIds string[]? - Array of tag identifiers, these will typically be BLE tag MAC addresses e.g. 00:11:22:33:44:55.
spotto: PostAssetsError
Fields
- data TagErrorInList[] -
- message string -
spotto: PostAssetsResponse
Fields
- inserted float - Total number of records that were inserted.
- items GetAssetResponse[] - The list of inserted items.
spotto: PostExternalUserRequest
Fields
- 'type string - User type for identifying external API users.
- name string - The User's full name, or an identifier of the external API user.
spotto: PostInternalUserRequest
Fields
- 'type string - User type for identifying internal users.
- email string - The User's unique email address. We send a welcome email including their password.
- password string? - Optionally provide a custom password rather than have Spotto automatically generate one. This will be forwarded to the new User's email address so that they can log into Spotto.
- name string - The User's full name, or an identifier of the external API user.
spotto: PostLevelRequest
Fields
- level string - Name of the new level to be inserted.
spotto: PostLevelResponse
spotto: PostLocationsError
Fields
- data TagErrorInList[] -
- message string -
spotto: PostlocationsrequestInner
Fields
- name string - Name of the new location, try to be descriptive to make it easy for people find it when searching.
- tagIds string[]? - If this location can be sensed by a mobile reader, you can add identifiers so it can be seen. It accepts an array of tag identifiers, these will typically be BLE tag MAC addresses e.g. 00:11:22:33:44:55.
- address string? - ⚠️ Experimental - You can assign an address to a Location however this is not used in any Spotto interfaces
- geoLocation string? - ⚠️ Experimental - You can assign coordinates to a Location however this is not used in any Spotto interfaces. For brevity this option has been set to a string. Any valid GeoJSON object will be accepted for this field. Check https://geojson.org/ for details.
spotto: PostLocationsResponse
Fields
- inserted float - Total number of records that were inserted
- items GetLocationResponse[] - The list of inserted items
spotto: PostMovedTriggerRequest
Asset or Reader moved anywhere (No location rules).
Fields
- 'type string - Type
- name string - The name of the trigger, for easily identifying the trigger and its purpose.
- actions Action[] - The list of actions that fire for this trigger.
- subjectRules Rule[] - Subject rules filter the possible events that cause this trigger to fire.
spotto: PostReadersResponse
Fields
- inserted float - Total number of records that were inserted
- items GetReaderResponse[] - The list of inserted items
spotto: PostTriggersResponse
Fields
- inserted float - Total number of records that were inserted
- items GetTriggerResponse[] - The list of inserted items
spotto: PostWentOfflineTriggerRequest
Reader went online after a given threshold.
Fields
- 'type string - Type
- name string - The name of the trigger, for easily identifying the trigger and its purpose.
- actions Action[] - The list of actions that fire for this trigger.
- subjectRules Rule[] - Subject rules filter the possible events that cause this trigger to fire.
spotto: PostWentOnlineTriggerRequest
Reader went online after a given threshold.
Fields
- 'type string - Type
- name string - The name of the trigger, for easily identifying the trigger and its purpose.
- actions Action[] - The list of actions that fire for this trigger.
- subjectRules Rule[] - Subject rules filter the possible events that cause this trigger to fire.
spotto: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
spotto: PutLevelsRequest
Fields
- levels string[] - New list of levels to save against the account.
spotto: PutLevelsResponse
Fields
- levels string[] - The new list of levels for this organisation.
spotto: StrictMatchIdRule
Fields
- 'type string -
- 'match string - Case sensitive exact Spotto ID of the event subject.
spotto: TagErrorInList
Fields
- index float - Index within the request that errors occurred
- errors (TagsExistError|TagsDuplicatedError)[] - List of errors for the asset at the given index of the request body
spotto: TagsDuplicatedConflict
A single conflict with a new tag ID existing on a previous item in the request
Fields
- tagId string - Tag ID causing the conflict
- index float - Index in this request the tag ID was first found
spotto: TagsDuplicatedError
Error structure for revealing tag ID conflicts within the request
Fields
- 'type string - Type
- message string - Message
- conflicts TagsDuplicatedConflict[] - Conflicts
spotto: TagsExistConflict
A single conflict with a new tag ID existing on an existing entity
Fields
- tagId string - Tag ID causing the conflict
- 'type string - Type of entity the tag ID exists on
- id string - ID of the entity the tag ID exists on
spotto: TagsExistError
Error structure for revealing tag ID conflicts
Fields
- 'type string - Type
- message string - Message
- conflicts TagsExistConflict[] - Conflicts
spotto: UpdateAssetError
Fields
- data TagsExistError - Error structure for revealing tag ID conflicts
- message string -
spotto: UpdateAssetRequest
Fields
- name string? - Update the name of the asset, perhaps because of a typo or to be more descriptive.
- tagIds string[]? - New list of tag identifiers, perhaps adding a new tag if the original tag is low on battery.
spotto: UpdateLocationError
Fields
- data TagsExistError - Error structure for revealing tag ID conflicts
- message string -
spotto: UpdateLocationRequest
Fields
- name string? - Update the name of the location, perhaps because of a typo or to be more descriptive.
- tagIds string[]? - New list of tag identifiers, perhaps adding a new tag if the original tag is low on battery.
- archived boolean? - Setting the archived status to true hides the location from view, you can reveal archived assets by using the GetLocations request.
- address string? - ⚠️ Experimental - You can edit a Locations address however this is not used in any Spotto interfaces.
- geoLocation string? - ⚠️ Experimental - You can edit Location coordinates however this is not used in any Spotto interfaces. NOTE: For brevity this option has been set to a string. Any valid GeoJSON object will be accepted for this field. Check https://geojson.org/ for details.
spotto: UpdateReaderRequest
Fields
- name string? - Update the name of the reader, perhaps because of a typo or to be more descriptive.
- archived boolean? - Setting the archived status to true hides the reader from view, you can reveal archived readers by using the GetReaders request.
- locationId string? - locationId is used for specifying an already existing location.
- location IUpsertedLocation? - If a location object is specified, a new location will be created and added to the locations list.
- deviceId string? - Reader Device ID. This will typically be a MAC address e.g. 00:11:22:33:44:55. <br> It is only applicable to IoTX3 readers.
- network INetworkRequest? - WiFi network credentials.
- saveNetwork boolean? - When this flag is true, it will save any provided WiFi details to the account. It will be ignored if no networks are present.
- antennas IAntennaRequest[]? - Additional antenna config. This is only applicable to VIRTUAL or PORTAL readers.
spotto: UpdateTriggerRequest
Fields
- name string? - The human readable name of the trigger, for easily identifying the trigger and its purpose.
- enabled boolean? - Change the status of the trigger, disabled triggers will not fire.
- actions Action[]? - Change the actions that fire for this trigger, you must send the entire array as part of the request. Use the GetTrigger request to fetch the existing actions to prevent accidentally deleting any.
- subjectRules Rule[]? - Change the subject rules to filter the events that cause this trigger to fire, you must send the entire array as part of the request. Use the GetTrigger request to fetch the existing subjectRules to prevent accidentally deleting any.
- locationRules Rule[]? - Change the location rules to filter the events that cause this trigger to fire, you must send the entire array as part of the request. Use the GetTrigger request to fetch the existing actions to prevent accidentally deleting any. ⚠️ Warning: This is only allowed for ArrivedAt triggers.
spotto: UpdateUserRequest
Fields
- name string? - Change the User's full name, or name of the external API user.
- organisation string? - If you have super-admin permissions you can move users between organisations.
spotto: WebhookAction
Fields
- 'type string -
- endpoint string - Endpoint URL to POST the event payload to.
Union types
spotto: Action
Action
spotto: GetTriggerResponse
GetTriggerResponse
spotto: GetUserResponse
GetUserResponse
spotto: MQTTSettings
MQTTSettings
spotto: PostAssetsErrorResponse
PostAssetsErrorResponse
spotto: PostLocationsErrorResponse
PostLocationsErrorResponse
spotto: PostReaderRequest
PostReaderRequest
spotto: PostTriggerRequest
PostTriggerRequest
spotto: PostUserRequest
PostUserRequest
spotto: ReaderLocation
ReaderLocation
Reader Locations can either be:
FIXED: Are static and don't have a timestamp
ROVING: Are dynamic and have a lastSeen timestamp
spotto: Rule
Rule
spotto: SearchableSortFields
SearchableSortFields
spotto: UpdateAssetErrorResponse
UpdateAssetErrorResponse
spotto: UpdateLocationErrorResponse
UpdateLocationErrorResponse
String types
spotto: AssetEmbedField
AssetEmbedField
spotto: AssetSearchField
AssetSearchField
spotto: DeviceType
DeviceType
The physical reader device type:
- B1 - Spotto B1 Bluetooth Reader.
- RF1A4 - Spotto RF1A4 4 Port RFID Reader.
- RF1F - Spotto RF1F FEIG Reader.
- HD1 - Spotto HD1 HID Reader.
- ZEBRA - Zebra RFID Reader.
- IMPINJ - Impinj RFID Reader.
- TURCK - Turck Handheld RFID Reader.
- OTHER - Custom Reader (BYO Device).
- APPLICATION - Spotto Detector Application.
spotto: EventType
EventType
spotto: LocationEmbedField
LocationEmbedField
spotto: LocationSearchField
LocationSearchField
spotto: ReaderEmbedField
ReaderEmbedField
spotto: ReaderSearchField
ReaderSearchField
spotto: ReaderType
ReaderType
The reader behaviour type:
- FIXED - regular non-moving readers.
- ROVING - readers that move around and look for location tags, assigning nearby assets to the locations it sees.
- VIRTUAL - allow different antennas plugged into a reader to report as their own readers.
- PORTAL - similar to virtual but for differentiating 2 adjacent zones e.g. IN/OUT.
spotto: RoleEmbedField
RoleEmbedField
spotto: SortOrders
SortOrders
Sort orders - ascending and descending
spotto: StandardSortFields
StandardSortFields
Common sort fields across most GET endpoints
spotto: TriggerEmbedField
TriggerEmbedField
spotto: TriggerType
TriggerType
==== TRIGGER TYPES ====
spotto: UserEmbedField
UserEmbedField
spotto: UserType
UserType
Import
import ballerinax/spotto;
Metadata
Released date: almost 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 3
Current verison: 2
Weekly downloads
Keywords
Internet of Things/Device Management
Cost/Paid
Contributors
Dependencies