slack
Module slack
Definitions
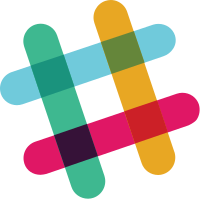
ballerinax/slack Ballerina library
Overview
Slack is a collaboration platform for teams, offering real-time messaging, file sharing, and integrations with various tools. It helps streamline communication and enhance productivity through organized channels and direct messaging.
Setup guide
Step 1: Sign in to Slack
-
To use the Slack Connector you need to be signed in to Slack. If you haven't created an account already, you can create it here.
Step 2: Create a new Slack application
-
Navigate to your apps in Slack API and create a new Slack app.
-
Provide an app name and choose a workspace of your choice.
-
Click on the "Create App" button.
Step 3: Add scopes to the token
-
Once the application is created, go to the "Add Features and Functionality" section and click on "Permissions" to set the token scopes.
-
In the User Token Scopes section set the following token scopes.
-
Install the application to workspace.
-
Copy the OAuth token that is generated upon installation.
Quickstart
To use the slack
connector in your Ballerina application, modify the .bal
file as follows:
Step 1: Import the module
Import the slack
module.
import ballerinax/slack;
Step 2: Instantiate a new connector
Assign the OAuth token obtained to the variable token, and then initialize a new instance of the slack client by passing the token.
configurable string token = ?; slack:Client slack = check new({ auth: { token } });
Step 3: Invoke the connector operation
Now, utilize the available connector operations.
Send a Text Message to General Channel
slack:ChatPostMessageResponse postMessageResponse = check slack->/chat\.postMessage.post({channel: "general", text: "hello"});
Step 4: Run the Ballerina application
bal run
Examples
The Slack
connector provides practical examples illustrating usage in various scenarios. Explore these examples, covering the following use cases:
-
Automated Summary Report - This use case demonstrates how the Slack API can be utilized to generate a summarized report of daily stand up chats in the general channel.
-
Survey Feedback Analysis - This use case demonstrates how the Slack API can be utilized to perform a company-wide survey by creating a dedicated channel to receive and track feedback replies.
Clients
slack: Client
One way to interact with the Slack platform is its HTTP RPC-based Web API, a collection of methods requiring OAuth 2.0-based user, bot, or workspace tokens blessed with related OAuth scopes.
Constructor
Gets invoked to initialize the connector
.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://slack.com/api" - URL of the target service
get admin.apps.approved.list
function get admin\.apps\.approved\.list(map<string|string[]> headers, *Admin_apps_approved_listQueries queries) returns DefaultSuccessResponse|error
get admin.apps.requests.list
function get admin\.apps\.requests\.list(map<string|string[]> headers, *Admin_apps_requests_listQueries queries) returns DefaultSuccessResponse|error
get admin.apps.restricted.list
function get admin\.apps\.restricted\.list(map<string|string[]> headers, *Admin_apps_restricted_listQueries queries) returns DefaultSuccessResponse|error
get admin.conversations.ekm.listOriginalConnectedChannelInfo
function get admin\.conversations\.ekm\.listOriginalConnectedChannelInfo(map<string|string[]> headers, *Admin_conversations_ekm_listOriginalConnectedChannelInfoQueries queries) returns DefaultSuccessResponse|error
Parameters
get admin.conversations.getConversationPrefs
function get admin\.conversations\.getConversationPrefs(map<string|string[]> headers, *Admin_conversations_getConversationPrefsQueries queries) returns AdminConversationsGetConversationPrefsResponse|error
Parameters
get admin.conversations.getTeams
function get admin\.conversations\.getTeams(map<string|string[]> headers, *Admin_conversations_getTeamsQueries queries) returns AdminConversationsGetTeamsResponse|error
Parameters
- queries *Admin_conversations_getTeamsQueries -
get admin.conversations.restrictAccess.listGroups
function get admin\.conversations\.restrictAccess\.listGroups(map<string|string[]> headers, *Admin_conversations_restrictAccess_listGroupsQueries queries) returns DefaultSuccessResponse|error
Parameters
get admin.conversations.search
function get admin\.conversations\.search(map<string|string[]> headers, *Admin_conversations_searchQueries queries) returns AdminConversationsSearchResponse|error
get admin.emoji.list
function get admin\.emoji\.list(map<string|string[]> headers, *Admin_emoji_listQueries queries) returns DefaultSuccessResponse|error
get admin.inviteRequests.approved.list
function get admin\.inviteRequests\.approved\.list(map<string|string[]> headers, *Admin_inviteRequests_approved_listQueries queries) returns DefaultSuccessResponse|error
Parameters
- queries *Admin_inviteRequests_approved_listQueries -
get admin.inviteRequests.denied.list
function get admin\.inviteRequests\.denied\.list(map<string|string[]> headers, *Admin_inviteRequests_denied_listQueries queries) returns DefaultSuccessResponse|error
Parameters
- queries *Admin_inviteRequests_denied_listQueries -
get admin.inviteRequests.list
function get admin\.inviteRequests\.list(map<string|string[]> headers, *Admin_inviteRequests_listQueries queries) returns DefaultSuccessResponse|error
get admin.teams.admins.list
function get admin\.teams\.admins\.list(map<string|string[]> headers, *Admin_teams_admins_listQueries queries) returns DefaultSuccessResponse|error
get admin.teams.list
function get admin\.teams\.list(map<string|string[]> headers, *Admin_teams_listQueries queries) returns DefaultSuccessResponse|error
get admin.teams.owners.list
function get admin\.teams\.owners\.list(map<string|string[]> headers, *Admin_teams_owners_listQueries queries) returns DefaultSuccessResponse|error
get admin.teams.settings.info
function get admin\.teams\.settings\.info(map<string|string[]> headers, *Admin_teams_settings_infoQueries queries) returns DefaultSuccessResponse|error
get admin.usergroups.listChannels
function get admin\.usergroups\.listChannels(map<string|string[]> headers, *Admin_usergroups_listChannelsQueries queries) returns DefaultSuccessResponse|error
Parameters
- queries *Admin_usergroups_listChannelsQueries -
get admin.users.list
function get admin\.users\.list(map<string|string[]> headers, *Admin_users_listQueries queries) returns DefaultSuccessResponse|error
get api.test
function get api\.test(map<string|string[]> headers, *Api_testQueries queries) returns ApiTestResponse|error
get apps.event.authorizations.list
function get apps\.event\.authorizations\.list(map<string|string[]> headers, *Apps_event_authorizations_listQueries queries) returns DefaultSuccessResponse|error
Parameters
- queries *Apps_event_authorizations_listQueries -
get apps.permissions.info
function get apps\.permissions\.info(map<string|string[]> headers) returns AppsPermissionsInfoResponse|error
get apps.permissions.request
function get apps\.permissions\.request(map<string|string[]> headers, *Apps_permissions_requestQueries queries) returns AppsPermissionsRequestResponse|error
get apps.permissions.resources.list
function get apps\.permissions\.resources\.list(map<string|string[]> headers, *Apps_permissions_resources_listQueries queries) returns AppsPermissionsResourcesListResponse|error
Parameters
- queries *Apps_permissions_resources_listQueries -
get apps.permissions.scopes.list
function get apps\.permissions\.scopes\.list(map<string|string[]> headers) returns ApiPermissionsScopesListResponse|error
get apps.permissions.users.list
function get apps\.permissions\.users\.list(map<string|string[]> headers, *Apps_permissions_users_listQueries queries) returns DefaultSuccessResponse|error
get apps.permissions.users.request
function get apps\.permissions\.users\.request(map<string|string[]> headers, *Apps_permissions_users_requestQueries queries) returns DefaultSuccessResponse|error
Parameters
- queries *Apps_permissions_users_requestQueries -
get apps.uninstall
function get apps\.uninstall(map<string|string[]> headers, *Apps_uninstallQueries queries) returns AppsUninstallResponse|error
get auth.revoke
function get auth\.revoke(map<string|string[]> headers, *Auth_revokeQueries queries) returns AuthRevokeResponse|error
get auth.test
function get auth\.test(map<string|string[]> headers) returns AuthTestResponse|error
get bots.info
function get bots\.info(map<string|string[]> headers, *Bots_infoQueries queries) returns BotsInfoResponse|error
get calls.info
function get calls\.info(map<string|string[]> headers, *Calls_infoQueries queries) returns DefaultSuccessResponse|error
get chat.getPermalink
function get chat\.getPermalink(map<string|string[]> headers, *Chat_getPermalinkQueries queries) returns ChatGetPermalinkResponse|error
get chat.scheduledMessages.list
function get chat\.scheduledMessages\.list(map<string|string[]> headers, *Chat_scheduledMessages_listQueries queries) returns ChatScheduledMessagesListResponse|error
get conversations.history
function get conversations\.history(map<string|string[]> headers, *Conversations_historyQueries queries) returns ConversationsHistoryResponse|error
get conversations.info
function get conversations\.info(map<string|string[]> headers, *Conversations_infoQueries queries) returns ConversationsInfoResponse|error
get conversations.list
function get conversations\.list(map<string|string[]> headers, *Conversations_listQueries queries) returns ConversationsListResponse|error
get conversations.members
function get conversations\.members(map<string|string[]> headers, *Conversations_membersQueries queries) returns ConversationsMembersResponse|error
get conversations.replies
function get conversations\.replies(map<string|string[]> headers, *Conversations_repliesQueries queries) returns ConversationsRepliesResponse|error
get dialog.open
function get dialog\.open(map<string|string[]> headers, *Dialog_openQueries queries) returns DialogOpenResponse|error
get dnd.info
function get dnd\.info(map<string|string[]> headers, *Dnd_infoQueries queries) returns DndInfoResponse|error
get dnd.teamInfo
function get dnd\.teamInfo(map<string|string[]> headers, *Dnd_teamInfoQueries queries) returns DefaultSuccessResponse|error
get emoji.list
function get emoji\.list(map<string|string[]> headers) returns DefaultSuccessResponse|error
get files.info
function get files\.info(map<string|string[]> headers, *Files_infoQueries queries) returns FilesInfoResponse|error
get files.list
function get files\.list(map<string|string[]> headers, *Files_listQueries queries) returns FilesListResponse|error
get files.remote.info
function get files\.remote\.info(map<string|string[]> headers, *Files_remote_infoQueries queries) returns DefaultSuccessResponse|error
get files.remote.list
function get files\.remote\.list(map<string|string[]> headers, *Files_remote_listQueries queries) returns DefaultSuccessResponse|error
get files.remote.share
function get files\.remote\.share(map<string|string[]> headers, *Files_remote_shareQueries queries) returns DefaultSuccessResponse|error
get migration.exchange
function get migration\.exchange(map<string|string[]> headers, *Migration_exchangeQueries queries) returns MigrationExchangeResponse|error
get oauth.access
function get oauth\.access(map<string|string[]> headers, *Oauth_accessQueries queries) returns DefaultSuccessResponse|error
get oauth.token
function get oauth\.token(map<string|string[]> headers, *Oauth_tokenQueries queries) returns DefaultSuccessResponse|error
get oauth.v2.access
function get oauth\.v2\.access(map<string|string[]> headers, *Oauth_v2_accessQueries queries) returns DefaultSuccessResponse|error
get pins.list
function get pins\.list(map<string|string[]> headers, *Pins_listQueries queries) returns record {| items (record {| created int, created_by UserIdDef, file FileObj, 'type "file" |}|record {| channel ChannelDef, created int, created_by UserIdDef, message MessageObj, 'type "message" |})[], ok OkTrueDef |}|record {| count int, ok OkTrueDef |}[]|error
get reactions.get
function get reactions\.get(map<string|string[]> headers, *Reactions_getQueries queries) returns record {| channel ChannelDef, message MessageObj, ok OkTrueDef, 'type "message" |}|record {| file FileObj, ok OkTrueDef, 'type "file" |}|record {| comment CommentObj, file FileObj, ok OkTrueDef, 'type "file_comment" |}[]|error
get reactions.list
function get reactions\.list(map<string|string[]> headers, *Reactions_listQueries queries) returns ReactionsListResponse|error
get reminders.info
function get reminders\.info(map<string|string[]> headers, *Reminders_infoQueries queries) returns RemindersInfoResponse|error
get reminders.list
function get reminders\.list(map<string|string[]> headers) returns RemindersListResponse|error
get rtm.connect
function get rtm\.connect(map<string|string[]> headers, *Rtm_connectQueries queries) returns RtmConnectResponse|error
get search.messages
function get search\.messages(map<string|string[]> headers, *Search_messagesQueries queries) returns DefaultSuccessResponse|error
get stars.list
function get stars\.list(map<string|string[]> headers, *Stars_listQueries queries) returns StarsListResponse|error
get team.accessLogs
function get team\.accessLogs(map<string|string[]> headers, *Team_accessLogsQueries queries) returns TeamAccessLogsResponse|error
get team.billableInfo
function get team\.billableInfo(map<string|string[]> headers, *Team_billableInfoQueries queries) returns DefaultSuccessResponse|error
get team.info
function get team\.info(map<string|string[]> headers, *Team_infoQueries queries) returns TeamInfoResponse|error
get team.integrationLogs
function get team\.integrationLogs(map<string|string[]> headers, *Team_integrationLogsQueries queries) returns TeamIntegrationLogsResponse|error
get team.profile.get
function get team\.profile\.get(map<string|string[]> headers, *Team_profile_getQueries queries) returns TeamProfileGetResponse|error
get usergroups.list
function get usergroups\.list(map<string|string[]> headers, *Usergroups_listQueries queries) returns UsergroupsListResponse|error
get usergroups.users.list
function get usergroups\.users\.list(map<string|string[]> headers, *Usergroups_users_listQueries queries) returns UsergroupsUsersListResponse|error
get users.conversations
function get users\.conversations(map<string|string[]> headers, *Users_conversationsQueries queries) returns UsersConversationsResponse|error
get users.getPresence
function get users\.getPresence(map<string|string[]> headers, *Users_getPresenceQueries queries) returns APIMethodUsersGetPresence|error
get users.identity
function get users\.identity(map<string|string[]> headers) returns record {| ok OkTrueDef, team record {| id TeamDef |}, user record {| id UserIdDef, name string |} |}|record {| ok OkTrueDef, team record {| id TeamDef |}, user record {| email string, id UserIdDef, name string |} |}|record {| ok OkTrueDef, team record {| id TeamDef |}, user record {| id UserIdDef, image_192 string, image_24 string, image_32 string, image_48 string, image_512 string, image_72 string, name string |} |}|record {| ok OkTrueDef, team record {| domain string, id TeamDef, image_102 string, image_132 string, image_230 string, image_34 string, image_44 string, image_68 string, image_88 string, image_default boolean, name string |}, user record {| id UserIdDef, name string |} |}[]|error
get users.info
function get users\.info(map<string|string[]> headers, *Users_infoQueries queries) returns UsersInfoResponse|error
get users.list
function get users\.list(map<string|string[]> headers, *Users_listQueries queries) returns UsersListResponse|error
get users.lookupByEmail
function get users\.lookupByEmail(map<string|string[]> headers, *Users_lookupByEmailQueries queries) returns UsersLookupByEmailResponse|error
get users.profile.get
function get users\.profile\.get(map<string|string[]> headers, *Users_profile_getQueries queries) returns UsersProfileGetResponse|error
get views.open
function get views\.open(map<string|string[]> headers, *Views_openQueries queries) returns DefaultSuccessResponse|error
get views.publish
function get views\.publish(map<string|string[]> headers, *Views_publishQueries queries) returns DefaultSuccessResponse|error
get views.push
function get views\.push(map<string|string[]> headers, *Views_pushQueries queries) returns DefaultSuccessResponse|error
get views.update
function get views\.update(map<string|string[]> headers, *Views_updateQueries queries) returns DefaultSuccessResponse|error
get workflows.stepCompleted
function get workflows\.stepCompleted(map<string|string[]> headers, *Workflows_stepCompletedQueries queries) returns DefaultSuccessResponse|error
get workflows.stepFailed
function get workflows\.stepFailed(map<string|string[]> headers, *Workflows_stepFailedQueries queries) returns DefaultSuccessResponse|error
get workflows.updateStep
function get workflows\.updateStep(map<string|string[]> headers, *Workflows_updateStepQueries queries) returns DefaultSuccessResponse|error
post admin.apps.approve
function post admin\.apps\.approve(admin_apps_approve_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
post admin.apps.restrict
function post admin\.apps\.restrict(admin_apps_restrict_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
post admin.conversations.archive
function post admin\.conversations\.archive(admin_conversations_archive_body payload, map<string|string[]> headers) returns AdminConversationsArchiveResponse|error
post admin.conversations.convertToPrivate
function post admin\.conversations\.convertToPrivate(admin_conversations_convertToPrivate_body payload, map<string|string[]> headers) returns AdminConversationsConvertToPrivateResponse|error
Parameters
post admin.conversations.create
function post admin\.conversations\.create(admin_conversations_create_body payload, map<string|string[]> headers) returns AdminConversationsCreateResponse|error
post admin.conversations.delete
function post admin\.conversations\.delete(admin_conversations_delete_body payload, map<string|string[]> headers) returns AdminConversationsDeleteResponse|error
post admin.conversations.disconnectShared
function post admin\.conversations\.disconnectShared(admin_conversations_disconnectShared_body payload, map<string|string[]> headers) returns AdminConversationsRenameResponse|error
Parameters
post admin.conversations.invite
function post admin\.conversations\.invite(admin_conversations_invite_body payload, map<string|string[]> headers) returns AdminConversationsInviteResponse|error
post admin.conversations.rename
function post admin\.conversations\.rename(admin_conversations_rename_body payload, map<string|string[]> headers) returns AdminConversationsRenameResponse_1|error
post admin.conversations.restrictAccess.addGroup
function post admin\.conversations\.restrictAccess\.addGroup(admin_conversations_restrictAccess_addGroup_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
Parameters
post admin.conversations.restrictAccess.removeGroup
function post admin\.conversations\.restrictAccess\.removeGroup(admin_conversations_restrictAccess_removeGroup_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
Parameters
post admin.conversations.setConversationPrefs
function post admin\.conversations\.setConversationPrefs(admin_conversations_setConversationPrefs_body payload, map<string|string[]> headers) returns AdminConversationsSetConversationPrefsResponse|error
Parameters
post admin.conversations.setTeams
function post admin\.conversations\.setTeams(admin_conversations_setTeams_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
post admin.conversations.unarchive
function post admin\.conversations\.unarchive(admin_conversations_unarchive_body payload, map<string|string[]> headers) returns AdminConversationsUnarchiveResponse|error
post admin.emoji.add
function post admin\.emoji\.add(admin_emoji_add_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
post admin.emoji.addAlias
function post admin\.emoji\.addAlias(admin_emoji_addAlias_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
post admin.emoji.remove
function post admin\.emoji\.remove(admin_emoji_remove_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
post admin.emoji.rename
function post admin\.emoji\.rename(admin_emoji_rename_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
post admin.inviteRequests.approve
function post admin\.inviteRequests\.approve(record { invite_request_id string, team_id string } payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
Parameters
post admin.inviteRequests.deny
function post admin\.inviteRequests\.deny(record { invite_request_id string, team_id string } payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
Parameters
post admin.teams.create
function post admin\.teams\.create(admin_teams_create_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
post admin.teams.settings.setDefaultChannels
function post admin\.teams\.settings\.setDefaultChannels(admin_teams_settings_setDefaultChannels_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
Parameters
post admin.teams.settings.setDescription
function post admin\.teams\.settings\.setDescription(admin_teams_settings_setDescription_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
Parameters
- payload admin_teams_settings_setDescription_body -
post admin.teams.settings.setDiscoverability
function post admin\.teams\.settings\.setDiscoverability(admin_teams_settings_setDiscoverability_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
Parameters
post admin.teams.settings.setIcon
function post admin\.teams\.settings\.setIcon(admin_teams_settings_setIcon_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
post admin.teams.settings.setName
function post admin\.teams\.settings\.setName(admin_teams_settings_setName_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
post admin.usergroups.addChannels
function post admin\.usergroups\.addChannels(admin_usergroups_addChannels_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
post admin.usergroups.addTeams
function post admin\.usergroups\.addTeams(admin_usergroups_addTeams_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
post admin.usergroups.removeChannels
function post admin\.usergroups\.removeChannels(admin_usergroups_removeChannels_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
Parameters
- payload admin_usergroups_removeChannels_body -
post admin.users.assign
function post admin\.users\.assign(admin_users_assign_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
post admin.users.invite
function post admin\.users\.invite(admin_users_invite_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
post admin.users.remove
function post admin\.users\.remove(admin_users_remove_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
post admin.users.session.invalidate
function post admin\.users\.session\.invalidate(admin_users_session_invalidate_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
post admin.users.session.reset
function post admin\.users\.session\.reset(admin_users_session_reset_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
post admin.users.setAdmin
function post admin\.users\.setAdmin(admin_users_setAdmin_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
post admin.users.setExpiration
function post admin\.users\.setExpiration(admin_users_setExpiration_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
post admin.users.setOwner
function post admin\.users\.setOwner(admin_users_setOwner_body payload, Admin_users_setOwnerHeaders headers) returns DefaultSuccessResponse|error
post admin.users.setRegular
function post admin\.users\.setRegular(admin_users_setRegular_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
post calls.add
function post calls\.add(calls_add_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
post calls.end
function post calls\.end(calls_end_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
post calls.participants.add
function post calls\.participants\.add(calls_participants_add_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
post calls.participants.remove
function post calls\.participants\.remove(calls_participants_remove_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
post calls.update
function post calls\.update(calls_update_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
post chat.delete
function post chat\.delete(chat_delete_body payload, map<string|string[]> headers) returns ChatDeleteResponse|error
post chat.deleteScheduledMessage
function post chat\.deleteScheduledMessage(chat_deleteScheduledMessage_body payload, map<string|string[]> headers) returns ChatDeleteScheduledMessageResponse|error
post chat.meMessage
function post chat\.meMessage(chat_meMessage_body payload, map<string|string[]> headers) returns ChatMeMessageResponse|error
post chat.postEphemeral
function post chat\.postEphemeral(chat_postEphemeral_body payload, map<string|string[]> headers) returns ChatPostEphemeralResponse|error
post chat.postMessage
function post chat\.postMessage(chat_postMessage_body payload, map<string|string[]> headers) returns ChatPostMessageResponse|error
post chat.scheduleMessage
function post chat\.scheduleMessage(chat_scheduleMessage_body payload, map<string|string[]> headers) returns ChatScheduleMessageResponse|error
post chat.unfurl
function post chat\.unfurl(chat_unfurl_body payload, map<string|string[]> headers) returns ChatUnfurlResponse|error
post chat.update
function post chat\.update(chat_update_body payload, map<string|string[]> headers) returns ChatUpdateResponse|error
post conversations.archive
function post conversations\.archive(conversations_archive_body payload, map<string|string[]> headers) returns ConversationsArchiveResponse|error
post conversations.close
function post conversations\.close(conversations_close_body payload, map<string|string[]> headers) returns ConversationsCloseResponse|error
post conversations.create
function post conversations\.create(conversations_create_body payload, map<string|string[]> headers) returns ConversationsCreateResponse|error
post conversations.invite
function post conversations\.invite(conversations_invite_body payload, map<string|string[]> headers) returns ConversationsInviteErrorResponse|error
post conversations.join
function post conversations\.join(conversations_join_body payload, map<string|string[]> headers) returns ConversationsJoinResponse|error
post conversations.kick
function post conversations\.kick(conversations_kick_body payload, map<string|string[]> headers) returns ConversationsKickResponse|error
post conversations.leave
function post conversations\.leave(conversations_leave_body payload, map<string|string[]> headers) returns ConversationsLeaveResponse|error
post conversations.mark
function post conversations\.mark(conversations_mark_body payload, map<string|string[]> headers) returns ConversationsMarkResponse|error
post conversations.open
function post conversations\.open(conversations_open_body payload, map<string|string[]> headers) returns ConversationsOpenResponse|error
post conversations.rename
function post conversations\.rename(conversations_rename_body payload, map<string|string[]> headers) returns ConversationsRenameResponse|error
post conversations.setPurpose
function post conversations\.setPurpose(conversations_setPurpose_body payload, map<string|string[]> headers) returns ConversationsSetPurposeResponse|error
post conversations.setTopic
function post conversations\.setTopic(conversations_setTopic_body payload, map<string|string[]> headers) returns ConversationsSetTopicResponse|error
post conversations.unarchive
function post conversations\.unarchive(conversations_unarchive_body payload, map<string|string[]> headers) returns ConversationsUnarchiveResponse|error
post dnd.endDnd
function post dnd\.endDnd(map<string|string[]> headers) returns DndEndDndResponse|error
post dnd.endSnooze
function post dnd\.endSnooze(map<string|string[]> headers) returns DndEndSnoozeResponse|error
post dnd.setSnooze
function post dnd\.setSnooze(dnd_setSnooze_body payload, map<string|string[]> headers) returns DndSetSnoozeResponse|error
post files.comments.delete
function post files\.comments\.delete(files_comments_delete_body payload, map<string|string[]> headers) returns FilesCommentsDeleteResponse|error
post files.delete
function post files\.delete(files_delete_body payload, map<string|string[]> headers) returns FilesDeleteResponse|error
post files.remote.add
function post files\.remote\.add(files_remote_add_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
post files.remote.remove
function post files\.remote\.remove(files_remote_remove_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
post files.remote.update
function post files\.remote\.update(files_remote_update_body payload, map<string|string[]> headers) returns DefaultSuccessResponse|error
post files.revokePublicURL
function post files\.revokePublicURL(files_revokePublicURL_body payload, map<string|string[]> headers) returns FilesRevokePublicURLResponse|error
post files.sharedPublicURL
function post files\.sharedPublicURL(files_sharedPublicURL_body payload, map<string|string[]> headers) returns FilesSharedPublicURLResponse|error
post files.upload
function post files\.upload(files_upload_body payload, map<string|string[]> headers) returns FilesUploadResponse|error
post pins.add
function post pins\.add(pins_add_body payload, map<string|string[]> headers) returns PinsAddResponse|error
post pins.remove
function post pins\.remove(pins_remove_body payload, map<string|string[]> headers) returns PinsRemoveResponse|error
post reactions.add
function post reactions\.add(reactions_add_body payload, map<string|string[]> headers) returns ReactionsAddResponse|error
post reactions.remove
function post reactions\.remove(reactions_remove_body payload, map<string|string[]> headers) returns ReactionsRemoveResponse|error
post reminders.add
function post reminders\.add(reminders_add_body payload, map<string|string[]> headers) returns RemindersAddResponse|error
post reminders.complete
function post reminders\.complete(reminders_complete_body payload, map<string|string[]> headers) returns RemindersCompleteResponse|error
post reminders.delete
function post reminders\.delete(reminders_delete_body payload, map<string|string[]> headers) returns RemindersDeleteResponse|error
post stars.add
function post stars\.add(stars_add_body payload, map<string|string[]> headers) returns StarsAddResponse|error
post stars.remove
function post stars\.remove(stars_remove_body payload, map<string|string[]> headers) returns StarsRemoveResponse|error
post usergroups.create
function post usergroups\.create(usergroups_create_body payload, map<string|string[]> headers) returns UsergroupsCreateResponse|error
post usergroups.disable
function post usergroups\.disable(usergroups_disable_body payload, map<string|string[]> headers) returns UsergroupsDisableResponse|error
post usergroups.enable
function post usergroups\.enable(usergroups_enable_body payload, map<string|string[]> headers) returns UsergroupsEnableResponse|error
post usergroups.update
function post usergroups\.update(usergroups_update_body payload, map<string|string[]> headers) returns UsergroupsUpdateResponse|error
post usergroups.users.update
function post usergroups\.users\.update(usergroups_users_update_body payload, map<string|string[]> headers) returns UsergroupsUsersUpdateResponse|error
post users.deletePhoto
function post users\.deletePhoto(users_deletePhoto_body payload, map<string|string[]> headers) returns UsersDeletePhotoResponse|error
post users.profile.set
function post users\.profile\.set(users_profile_set_body payload, map<string|string[]> headers) returns UsersProfileSetResponse|error
post users.setActive
function post users\.setActive(map<string|string[]> headers) returns UsersSetActiveResponse|error
post users.setPhoto
function post users\.setPhoto(users_setPhoto_body payload, map<string|string[]> headers) returns UsersSetPhotoResponse|error
post users.setPresence
function post users\.setPresence(users_setPresence_body payload, map<string|string[]> headers) returns UsersSetPresenceResponse|error
Records
slack: admin_apps_approve_body
Fields
- app_id? string - The id of the app to approve.
- request_id? string - The id of the request to approve.
- team_id? string -
slack: Admin_apps_approved_listQueries
Represents the Queries record for the operation: admin_apps_approved_list
Fields
- cursor? string - Set
cursor
tonext_cursor
returned by the previous call to list items in the next page
- 'limit? int - The maximum number of items to return. Must be between 1 - 1000 both inclusive.
- team_id? string -
- enterprise_id? string -
slack: Admin_apps_requests_listQueries
Represents the Queries record for the operation: admin_apps_requests_list
Fields
- cursor? string - Set
cursor
tonext_cursor
returned by the previous call to list items in the next page
- 'limit? int - The maximum number of items to return. Must be between 1 - 1000 both inclusive.
- team_id? string -
slack: admin_apps_restrict_body
Fields
- app_id? string - The id of the app to restrict.
- request_id? string - The id of the request to restrict.
- team_id? string -
slack: Admin_apps_restricted_listQueries
Represents the Queries record for the operation: admin_apps_restricted_list
Fields
- cursor? string - Set
cursor
tonext_cursor
returned by the previous call to list items in the next page
- 'limit? int - The maximum number of items to return. Must be between 1 - 1000 both inclusive.
- team_id? string -
- enterprise_id? string -
slack: admin_conversations_archive_body
Fields
- channel_id string - The channel to archive.
slack: admin_conversations_convertToPrivate_body
Fields
- channel_id string - The channel to convert to private.
slack: admin_conversations_create_body
Fields
- description? string - Description of the public or private channel to create.
- is_private boolean - When
true
, creates a private channel instead of a public channel
- name string - Name of the public or private channel to create.
- org_wide? boolean - When
true
, the channel will be available org-wide. Note: if the channel is notorg_wide=true
, you must specify ateam_id
for this channel
- team_id? string - The workspace to create the channel in. Note: this argument is required unless you set
org_wide=true
.
slack: admin_conversations_delete_body
Fields
- channel_id string - The channel to delete.
slack: admin_conversations_disconnectShared_body
Fields
- channel_id string - The channel to be disconnected from some workspaces.
- leaving_team_ids? string - The team to be removed from the channel. Currently only a single team id can be specified.
slack: Admin_conversations_ekm_listOriginalConnectedChannelInfoQueries
Represents the Queries record for the operation: admin_conversations_ekm_listOriginalConnectedChannelInfo
Fields
- channel_ids? string - A comma-separated list of channels to filter to.
- cursor? string - Set
cursor
tonext_cursor
returned by the previous call to list items in the next page.
- team_ids? string - A comma-separated list of the workspaces to which the channels you would like returned belong.
- 'limit? int - The maximum number of items to return. Must be between 1 - 1000 both inclusive.
slack: Admin_conversations_getConversationPrefsQueries
Represents the Queries record for the operation: admin_conversations_getConversationPrefs
Fields
- channel_id string - The channel to get preferences for.
slack: Admin_conversations_getTeamsQueries
Represents the Queries record for the operation: admin_conversations_getTeams
Fields
- cursor? string - Set
cursor
tonext_cursor
returned by the previous call to list items in the next page
- 'limit? int - The maximum number of items to return. Must be between 1 - 1000 both inclusive.
- channel_id string - The channel to determine connected workspaces within the organization for.
slack: admin_conversations_invite_body
Fields
- channel_id string - The channel that the users will be invited to.
- user_ids string - The users to invite.
slack: admin_conversations_rename_body
Fields
- channel_id string - The channel to rename.
- name string -
slack: admin_conversations_restrictAccess_addGroup_body
Fields
- channel_id string - The channel to link this group to.
- team_id? string - The workspace where the channel exists. This argument is required for channels only tied to one workspace, and optional for channels that are shared across an organization.
- token string - Authentication token. Requires scope:
admin.conversations:write
slack: Admin_conversations_restrictAccess_listGroupsQueries
Represents the Queries record for the operation: admin_conversations_restrictAccess_listGroups
Fields
- team_id? string - The workspace where the channel exists. This argument is required for channels only tied to one workspace, and optional for channels that are shared across an organization.
- channel_id string -
slack: admin_conversations_restrictAccess_removeGroup_body
Fields
- channel_id string - The channel to remove the linked group from.
- team_id string - The workspace where the channel exists. This argument is required for channels only tied to one workspace, and optional for channels that are shared across an organization.
- token string - Authentication token. Requires scope:
admin.conversations:write
slack: Admin_conversations_searchQueries
Represents the Queries record for the operation: admin_conversations_search
Fields
- cursor? string - Set
cursor
tonext_cursor
returned by the previous call to list items in the next page.
- search_channel_types? string - The type of channel to include or exclude in the search. For example
private
will search private channels, whileprivate_exclude
will exclude them. For a full list of types, check the Types section.
- team_ids? string - Comma separated string of team IDs, signifying the workspaces to search through.
- query? string - Name of the the channel to query by.
- 'limit? int - Maximum number of items to be returned. Must be between 1 - 20 both inclusive. Default is 10.
- sort? string - Possible values are
relevant
(search ranking based on what we think is closest),name
(alphabetical),member_count
(number of users in the channel), andcreated
(date channel was created). You can optionally pair this with thesort_dir
arg to change how it is sorted
- sort_dir? string - Sort direction. Possible values are
asc
for ascending order like (1, 2, 3) or (a, b, c), anddesc
for descending order like (3, 2, 1) or (c, b, a)
slack: admin_conversations_setConversationPrefs_body
Fields
- channel_id string - The channel to set the prefs for
- prefs string - The prefs for this channel in a stringified JSON format.
slack: admin_conversations_setTeams_body
Fields
- channel_id string - The encoded
channel_id
to add or remove to workspaces.
- org_channel? boolean - True if channel has to be converted to an org channel
- target_team_ids? string - A comma-separated list of workspaces to which the channel should be shared. Not required if the channel is being shared org-wide.
- team_id? string - The workspace to which the channel belongs. Omit this argument if the channel is a cross-workspace shared channel.
slack: admin_conversations_unarchive_body
Fields
- channel_id string - The channel to unarchive.
slack: admin_emoji_add_body
Fields
- name string - The name of the emoji to be removed. Colons (
:myemoji:
) around the value are not required, although they may be included.
- token string - Authentication token. Requires scope:
admin.teams:write
- url string - The URL of a file to use as an image for the emoji. Square images under 128KB and with transparent backgrounds work best.
slack: admin_emoji_addAlias_body
Fields
- alias_for string - The alias of the emoji.
- name string - The name of the emoji to be aliased. Colons (
:myemoji:
) around the value are not required, although they may be included.
- token string - Authentication token. Requires scope:
admin.teams:write
slack: Admin_emoji_listQueries
Represents the Queries record for the operation: admin_emoji_list
Fields
- cursor? string - Set
cursor
tonext_cursor
returned by the previous call to list items in the next page
- 'limit? int - The maximum number of items to return. Must be between 1 - 1000 both inclusive.
slack: admin_emoji_remove_body
Fields
- name string - The name of the emoji to be removed. Colons (
:myemoji:
) around the value are not required, although they may be included.
- token string - Authentication token. Requires scope:
admin.teams:write
slack: admin_emoji_rename_body
Fields
- name string - The name of the emoji to be renamed. Colons (
:myemoji:
) around the value are not required, although they may be included.
- new_name string - The new name of the emoji.
- token string - Authentication token. Requires scope:
admin.teams:write
slack: Admin_inviteRequests_approved_listQueries
Represents the Queries record for the operation: admin_inviteRequests_approved_list
Fields
- cursor? string - Value of the
next_cursor
field sent as part of the previous API response
- 'limit? int - The number of results that will be returned by the API on each invocation. Must be between 1 - 1000, both inclusive
- team_id? string - ID for the workspace where the invite requests were made.
slack: Admin_inviteRequests_denied_listQueries
Represents the Queries record for the operation: admin_inviteRequests_denied_list
Fields
- cursor? string - Value of the
next_cursor
field sent as part of the previous api response
- 'limit? int - The number of results that will be returned by the API on each invocation. Must be between 1 - 1000 both inclusive
- team_id? string - ID for the workspace where the invite requests were made.
slack: Admin_inviteRequests_listQueries
Represents the Queries record for the operation: admin_inviteRequests_list
Fields
- cursor? string - Value of the
next_cursor
field sent as part of the previous API response
- 'limit? int - The number of results that will be returned by the API on each invocation. Must be between 1 - 1000, both inclusive
- team_id? string - ID for the workspace where the invite requests were made.
slack: Admin_teams_admins_listQueries
Represents the Queries record for the operation: admin_teams_admins_list
Fields
- cursor? string - Set
cursor
tonext_cursor
returned by the previous call to list items in the next page.
- 'limit? int - The maximum number of items to return.
- team_id string -
slack: admin_teams_create_body
Fields
- team_description? string - Description for the team.
- team_discoverability? string - Who can join the team. A team's discoverability can be
open
,closed
,invite_only
, orunlisted
.
- team_domain string - Team domain (for example, slacksoftballteam).
- team_name string - Team name (for example, Slack Softball Team).
slack: Admin_teams_listQueries
Represents the Queries record for the operation: admin_teams_list
Fields
- cursor? string - Set
cursor
tonext_cursor
returned by the previous call to list items in the next page.
- 'limit? int - The maximum number of items to return. Must be between 1 - 100 both inclusive.
slack: Admin_teams_owners_listQueries
Represents the Queries record for the operation: admin_teams_owners_list
Fields
- cursor? string - Set
cursor
tonext_cursor
returned by the previous call to list items in the next page.
- 'limit? int - The maximum number of items to return. Must be between 1 - 1000 both inclusive.
- team_id string -
slack: Admin_teams_settings_infoQueries
Represents the Queries record for the operation: admin_teams_settings_info
Fields
- team_id string -
slack: admin_teams_settings_setDefaultChannels_body
Fields
- channel_ids string - An array of channel IDs.
- team_id string - ID for the workspace to set the default channel for.
- token string - Authentication token. Requires scope:
admin.teams:write
slack: admin_teams_settings_setDescription_body
Fields
- description string - The new description for the workspace.
- team_id string - ID for the workspace to set the description for.
slack: admin_teams_settings_setDiscoverability_body
Fields
- discoverability string - This workspace's discovery setting. It must be set to one of
open
,invite_only
,closed
, orunlisted
.
- team_id string - The ID of the workspace to set discoverability on.
slack: admin_teams_settings_setIcon_body
Fields
- image_url string - Image URL for the icon
- team_id string - ID for the workspace to set the icon for.
- token string - Authentication token. Requires scope:
admin.teams:write
slack: admin_teams_settings_setName_body
Fields
- name string - The new name of the workspace.
- team_id string - ID for the workspace to set the name for.
slack: admin_usergroups_addChannels_body
Fields
- channel_ids string - Comma separated string of channel IDs.
- team_id? string - The workspace to add default channels in.
- usergroup_id string - ID of the IDP group to add default channels for.
slack: admin_usergroups_addTeams_body
Fields
- auto_provision? boolean - When
true
, this method automatically creates new workspace accounts for the IDP group members.
- team_ids string - A comma separated list of encoded team (workspace) IDs. Each workspace MUST belong to the organization associated with the token.
- usergroup_id string - An encoded usergroup (IDP Group) ID.
slack: Admin_usergroups_listChannelsQueries
Represents the Queries record for the operation: admin_usergroups_listChannels
Fields
- include_num_members? boolean - Flag to include or exclude the count of members per channel.
- usergroup_id string - ID of the IDP group to list default channels for.
- team_id? string - ID of the the workspace.
slack: admin_usergroups_removeChannels_body
Fields
- channel_ids string - Comma-separated string of channel IDs
- usergroup_id string - ID of the IDP Group
slack: admin_users_assign_body
Fields
- channel_ids? string - Comma separated values of channel IDs to add user in the new workspace.
- is_restricted? boolean - True if user should be added to the workspace as a guest.
- is_ultra_restricted? boolean - True if user should be added to the workspace as a single-channel guest.
- team_id string - The ID (
T1234
) of the workspace.
- user_id string - The ID of the user to add to the workspace.
slack: admin_users_invite_body
Fields
- channel_ids string - A comma-separated list of
channel_id
s for this user to join. At least one channel is required.
- custom_message? string - An optional message to send to the user in the invite email.
- email string - The email address of the person to invite.
- guest_expiration_ts? string - Timestamp when guest account should be disabled. Only include this timestamp if you are inviting a guest user and you want their account to expire on a certain date.
- is_restricted? boolean - Is this user a multi-channel guest user? (default: false)
- is_ultra_restricted? boolean - Is this user a single channel guest user? (default: false)
- real_name? string - Full name of the user.
- resend? boolean - Allow this invite to be resent in the future if a user has not signed up yet. (default: false)
- team_id string - The ID (
T1234
) of the workspace.
slack: Admin_users_listQueries
Represents the Queries record for the operation: admin_users_list
Fields
- cursor? string - Set
cursor
tonext_cursor
returned by the previous call to list items in the next page.
- 'limit? int - Limit for how many users to be retrieved per page
- team_id string - The ID (
T1234
) of the workspace.
slack: admin_users_remove_body
Fields
- team_id string - The ID (
T1234
) of the workspace.
- user_id string - The ID of the user to remove.
slack: admin_users_session_invalidate_body
Fields
- session_id int -
- team_id string - ID of the team that the session belongs to
slack: admin_users_session_reset_body
Fields
- mobile_only? boolean - Only expire mobile sessions (default: false)
- user_id string - The ID of the user to wipe sessions for
- web_only? boolean - Only expire web sessions (default: false)
slack: admin_users_setAdmin_body
Fields
- team_id string - The ID (
T1234
) of the workspace.
- user_id string - The ID of the user to designate as an admin.
slack: admin_users_setExpiration_body
Fields
- expiration_ts int - Timestamp when guest account should be disabled.
- team_id string - The ID (
T1234
) of the workspace.
- user_id string - The ID of the user to set an expiration for.
slack: admin_users_setOwner_body
Fields
- team_id string - The ID (
T1234
) of the workspace.
- user_id string - Id of the user to promote to owner.
slack: Admin_users_setOwnerHeaders
Represents the Headers record for the operation: admin_users_setOwner
Fields
- token string - Authentication token. Requires scope:
admin.users:write
slack: admin_users_setRegular_body
Fields
- team_id string - The ID (
T1234
) of the workspace.
- user_id string - The ID of the user to designate as a regular user.
slack: AdminConversationsArchiveResponse
Schema for successful response of admin.conversations.archive
Fields
- ok OkTrueDef -
slack: AdminConversationsConvertToPrivateResponse
Schema for successful response of admin.conversations.convertToPrivate
Fields
- ok OkTrueDef -
slack: AdminConversationsCreateResponse
Schema for successful response of admin.conversations.create
Fields
- channel_id? ChannelIdDef -
- ok OkTrueDef -
slack: AdminConversationsDeleteResponse
Schema for successful response of admin.conversations.delete
Fields
- ok OkTrueDef -
slack: AdminConversationsGetConversationPrefsResponse
Schema for successful response of admin.conversations.getConversationPrefs
Fields
- ok OkTrueDef -
slack: AdminConversationsGetConversationPrefsResponse_prefs
Fields
- who_can_post? AdminConversationsGetConversationPrefsResponse_prefs_can_thread -
slack: AdminConversationsGetConversationPrefsResponse_prefs_can_thread
Fields
- 'type? string[] -
- user? string[] -
slack: AdminConversationsGetTeamsResponse
Schema for successful response of admin.conversations.getTeams
Fields
- ok OkTrueDef -
- response_metadata? AdminConversationsGetTeamsResponse_response_metadata -
- team_ids TeamDef[] -
slack: AdminConversationsGetTeamsResponse_response_metadata
Fields
- next_cursor string -
slack: AdminConversationsInviteResponse
Schema for successful response of admin.conversations.invite
Fields
- ok OkTrueDef -
slack: AdminConversationsRenameResponse
Schema for successful response of admin.conversations.disconnectShared
Fields
- ok OkTrueDef -
slack: AdminConversationsRenameResponse_1
Schema for successful response of admin.conversations.rename
Fields
- ok OkTrueDef -
slack: AdminConversationsSearchResponse
Schema for successful response of admin.conversations.search
Fields
- channels ChannelObj[] -
- next_cursor string -
slack: AdminConversationsSetConversationPrefsResponse
Schema for successful response of admin.conversations.setConversationPrefs
Fields
- ok OkTrueDef -
slack: AdminConversationsUnarchiveResponse
Schema for successful response of admin.conversations.unarchive
Fields
- ok OkTrueDef -
slack: Api_testQueries
Represents the Queries record for the operation: api_test
Fields
- foo? string - example property to return
slack: APIMethodUsersGetPresence
Generated from users.getPresence with shasum e7251aec575d8863f9e0eb38663ae9dc26655f65
Fields
- auto_away? boolean -
- connection_count? int -
- last_activity? int -
- manual_away? boolean -
- ok OkTrueDef -
- online? boolean -
- presence string -
slack: ApiPermissionsScopesListResponse
Schema for successful response api.permissions.scopes.list method
Fields
- ok OkTrueDef -
slack: ApiPermissionsScopesListResponse_scopes
Fields
- app_home? ScopesObj -
- channel? ScopesObj -
- group? ScopesObj -
- im? ScopesObj -
- mpim? ScopesObj -
- team? ScopesObj -
- user? ScopesObj -
slack: ApiTestResponse
Schema for successful response api.test method
Fields
- ok OkTrueDef -
- record {}... - Rest field
slack: Apps_event_authorizations_listQueries
Represents the Queries record for the operation: apps_event_authorizations_list
Fields
- cursor? string -
- 'limit? int -
- event_context string -
slack: Apps_permissions_requestQueries
Represents the Queries record for the operation: apps_permissions_request
Fields
- trigger_id string - Token used to trigger the permissions API
- scopes string - A comma separated list of scopes to request for
slack: Apps_permissions_resources_listQueries
Represents the Queries record for the operation: apps_permissions_resources_list
Fields
- cursor? string - Paginate through collections of data by setting the
cursor
parameter to anext_cursor
attribute returned by a previous request'sresponse_metadata
. Default value fetches the first "page" of the collection. See pagination for more detail.
- 'limit? int - The maximum number of items to return.
slack: Apps_permissions_users_listQueries
Represents the Queries record for the operation: apps_permissions_users_list
Fields
- cursor? string - Paginate through collections of data by setting the
cursor
parameter to anext_cursor
attribute returned by a previous request'sresponse_metadata
. Default value fetches the first "page" of the collection. See pagination for more detail.
- 'limit? int - The maximum number of items to return.
slack: Apps_permissions_users_requestQueries
Represents the Queries record for the operation: apps_permissions_users_request
Fields
- trigger_id string - Token used to trigger the request
- scopes string - A comma separated list of user scopes to request for
- user string - The user this scope is being requested for
slack: Apps_uninstallQueries
Represents the Queries record for the operation: apps_uninstall
Fields
- client_secret? string - Issued when you created your application.
- client_id? string - Issued when you created your application.
slack: AppsPermissionsInfoResponse
Schema for successful response from apps.permissions.info method
Fields
- ok OkTrueDef -
slack: AppsPermissionsInfoResponse_info
Fields
- app_home AppsPermissionsInfoResponse_info_app_home -
slack: AppsPermissionsInfoResponse_info_app_home
Fields
- resources? ResourcesObj -
- scopes? ScopesObj -
slack: AppsPermissionsInfoResponse_info_team
Fields
- resources ResourcesObj -
- scopes ScopesObj -
slack: AppsPermissionsRequestResponse
Schema for successful response from apps.permissions.request method
Fields
- ok OkTrueDef -
slack: AppsPermissionsResourcesListResponse
Schema for successful response apps.permissions.resources.list method
Fields
- ok OkTrueDef -
- resources AppsPermissionsResourcesListResponse_resources[] -
- response_metadata? AppsPermissionsResourcesListResponse_response_metadata -
slack: AppsPermissionsResourcesListResponse_resources
Fields
- id? string -
- 'type? string -
slack: AppsPermissionsResourcesListResponse_response_metadata
Fields
- next_cursor string -
slack: AppsUninstallResponse
Schema for successful response from apps.uninstall method
Fields
- ok OkTrueDef -
slack: Auth_revokeQueries
Represents the Queries record for the operation: auth_revoke
Fields
- test? boolean - Setting this parameter to
1
triggers a testing mode where the specified token will not actually be revoked.
slack: AuthRevokeResponse
Schema for successful response from auth.revoke method
Fields
- ok OkTrueDef -
- revoked boolean -
slack: AuthTestResponse
Schema for successful response auth.test method
Fields
- bot_id? BotIdDef -
- is_enterprise_install? boolean -
- ok OkTrueDef -
- team string -
- team_id TeamDef -
- url string -
- user string -
- user_id UserIdDef -
slack: blocks_inner
Fields
- 'type string -
slack: BotProfileObj
Fields
- app_id AppIdDef -
- deleted boolean -
- icons BotProfileObj_icons -
- id BotIdDef -
- name string -
- team_id TeamDef -
- updated int -
slack: BotProfileObj_icons
Fields
- image_36 string -
- image_48 string -
- image_72 string -
slack: Bots_infoQueries
Represents the Queries record for the operation: bots_info
Fields
- bot? string - Bot user to get info on
slack: BotsInfoResponse
Schema for successful response from bots.info method
Fields
- bot BotsInfoResponse_bot -
- ok OkTrueDef -
slack: BotsInfoResponse_bot
Fields
- app_id AppIdDef -
- deleted boolean -
- icons BotProfileObj_icons -
- id BotIdDef -
- name string -
- updated int -
- user_id? UserIdDef -
slack: calls_add_body
Fields
- created_by? string - The valid Slack user ID of the user who created this Call. When this method is called with a user token, the
created_by
field is optional and defaults to the authed user of the token. Otherwise, the field is required.
- date_start? int - Call start time in UTC UNIX timestamp format
- desktop_app_join_url? string - When supplied, available Slack clients will attempt to directly launch the 3rd-party Call with this URL.
- external_display_id? string - An optional, human-readable ID supplied by the 3rd-party Call provider. If supplied, this ID will be displayed in the Call object.
- external_unique_id string - An ID supplied by the 3rd-party Call provider. It must be unique across all Calls from that service.
- join_url string - The URL required for a client to join the Call.
- title? string - The name of the Call.
- users? string - The list of users to register as participants in the Call. Read more on how to specify users here.
slack: calls_end_body
Fields
- duration? int - Call duration in seconds
slack: Calls_infoQueries
Represents the Queries record for the operation: calls_info
Fields
slack: calls_participants_add_body
Fields
- users string - The list of users to add as participants in the Call. Read more on how to specify users here.
slack: calls_participants_remove_body
Fields
- users string - The list of users to remove as participants in the Call. Read more on how to specify users here.
slack: calls_update_body
Fields
- desktop_app_join_url? string - When supplied, available Slack clients will attempt to directly launch the 3rd-party Call with this URL.
- join_url? string - The URL required for a client to join the Call.
- title? string - The name of the Call.
slack: ChannelObj
Fields
- accepted_user? UserIdDef -
- created int -
- creator UserIdDef -
- id ChannelIdDef -
- is_archived? boolean -
- is_channel boolean -
- is_frozen? boolean -
- is_general? boolean -
- is_member? boolean -
- is_moved? int -
- is_mpim boolean -
- is_non_threadable? boolean -
- is_org_shared boolean -
- is_pending_ext_shared? boolean -
- is_private boolean -
- is_read_only? boolean -
- is_shared boolean -
- is_thread_only? boolean -
- last_read? TsDef -
- latest? (anydata)[] -
- members UserIdDef[] -
- name string -
- name_normalized string -
- num_members? int -
- pending_shared? TeamDef[] -
- previous_names? ChannelNameDef[] -
- priority? decimal -
- purpose ChannelObj_purpose -
- topic ChannelObj_purpose -
- unlinked? int -
- unread_count? int -
- unread_count_display? int -
slack: ChannelObj_purpose
Fields
- creator TopicPurposeCreatorDef -
- last_set int -
- value string -
slack: chat_delete_body
Fields
- channel? string - Channel containing the message to be deleted.
- ts? decimal - Timestamp of the message to be deleted.
slack: chat_deleteScheduledMessage_body
Fields
- channel string - The channel the scheduled_message is posting to
- scheduled_message_id string -
scheduled_message_id
returned from call to chat.scheduleMessage
slack: Chat_getPermalinkQueries
Represents the Queries record for the operation: chat_getPermalink
Fields
- channel string - The ID of the conversation or channel containing the message
- message_ts string - A message's
ts
value, uniquely identifying it within a channel
slack: chat_meMessage_body
Fields
- channel? string - Channel to send message to. Can be a public channel, private group or IM channel. Can be an encoded ID, or a name.
- text? string - Text of the message to send.
slack: chat_postEphemeral_body
Fields
- as_user? boolean - Pass true to post the message as the authed user. Defaults to true if the chat:write:bot scope is not included. Otherwise, defaults to false.
- attachments? string - A JSON-based array of structured attachments, presented as a URL-encoded string.
- blocks? string - A JSON-based array of structured blocks, presented as a URL-encoded string.
- channel string - Channel, private group, or IM channel to send message to. Can be an encoded ID, or a name.
- icon_emoji? string - Emoji to use as the icon for this message. Overrides
icon_url
. Must be used in conjunction withas_user
set tofalse
, otherwise ignored. See authorship below.
- icon_url? string - URL to an image to use as the icon for this message. Must be used in conjunction with
as_user
set to false, otherwise ignored. See authorship below.
- link_names? boolean - Find and link channel names and usernames.
- thread_ts? string - Provide another message's
ts
value to post this message in a thread. Avoid using a reply'sts
value; use its parent's value instead. Ephemeral messages in threads are only shown if there is already an active thread.
- user string -
id
of the user who will receive the ephemeral message. The user should be in the channel specified by thechannel
argument.
- username? string - Set your bot's user name. Must be used in conjunction with
as_user
set to false, otherwise ignored. See authorship below.
slack: chat_postMessage_body
Fields
- as_user? string - Pass true to post the message as the authed user, instead of as a bot. Defaults to false. See authorship below.
- attachments? string - A JSON-based array of structured attachments, presented as a URL-encoded string.
- blocks? string - A JSON-based array of structured blocks, presented as a URL-encoded string.
- icon_emoji? string - Emoji to use as the icon for this message. Overrides
icon_url
. Must be used in conjunction withas_user
set tofalse
, otherwise ignored. See authorship below.
- icon_url? string - URL to an image to use as the icon for this message. Must be used in conjunction with
as_user
set to false, otherwise ignored. See authorship below.
- link_names? boolean - Find and link channel names and usernames.
- mrkdwn? boolean - Disable Slack markup parsing by setting to
false
. Enabled by default.
- reply_broadcast? boolean - Used in conjunction with
thread_ts
and indicates whether reply should be made visible to everyone in the channel or conversation. Defaults tofalse
.
- thread_ts? string - Provide another message's
ts
value to make this message a reply. Avoid using a reply'sts
value; use its parent instead.
- unfurl_links? boolean - Pass true to enable unfurling of primarily text-based content.
- unfurl_media? boolean - Pass false to disable unfurling of media content.
- username? string - Set your bot's user name. Must be used in conjunction with
as_user
set to false, otherwise ignored. See authorship below.
slack: Chat_scheduledMessages_listQueries
Represents the Queries record for the operation: chat_scheduledMessages_list
Fields
- cursor? string - For pagination purposes, this is the
cursor
value returned from a previous call tochat.scheduledmessages.list
indicating where you want to start this call from.
- oldest? decimal - A UNIX timestamp of the oldest value in the time range
- channel? string - The channel of the scheduled messages
- 'limit? int - Maximum number of original entries to return.
- latest? decimal - A UNIX timestamp of the latest value in the time range
slack: chat_scheduleMessage_body
Fields
- as_user? boolean - Pass true to post the message as the authed user, instead of as a bot. Defaults to false. See chat.postMessage.
- attachments? string - A JSON-based array of structured attachments, presented as a URL-encoded string.
- blocks? string - A JSON-based array of structured blocks, presented as a URL-encoded string.
- link_names? boolean - Find and link channel names and usernames.
- parse? string - Change how messages are treated. Defaults to
none
. See chat.postMessage.
- post_at? string - Unix EPOCH timestamp of time in future to send the message.
- reply_broadcast? boolean - Used in conjunction with
thread_ts
and indicates whether reply should be made visible to everyone in the channel or conversation. Defaults tofalse
.
- thread_ts? decimal - Provide another message's
ts
value to make this message a reply. Avoid using a reply'sts
value; use its parent instead.
- unfurl_links? boolean - Pass true to enable unfurling of primarily text-based content.
- unfurl_media? boolean - Pass false to disable unfurling of media content.
slack: chat_unfurl_body
Fields
- channel string - Channel ID of the message
- ts string - Timestamp of the message to add unfurl behavior to.
- unfurls? string - URL-encoded JSON map with keys set to URLs featured in the the message, pointing to their unfurl blocks or message attachments.
- user_auth_message? string - Provide a simply-formatted string to send as an ephemeral message to the user as invitation to authenticate further and enable full unfurling behavior
- user_auth_required? boolean - Set to
true
or1
to indicate the user must install your Slack app to trigger unfurls for this domain
- user_auth_url? string - Send users to this custom URL where they will complete authentication in your app to fully trigger unfurling. Value should be properly URL-encoded.
slack: chat_update_body
Fields
- attachments? string - A JSON-based array of structured attachments, presented as a URL-encoded string. This field is required when not presenting
text
. If you don't include this field, the message's previousattachments
will be retained. To remove previousattachments
, include an empty array for this field.
- blocks? string - A JSON-based array of structured blocks, presented as a URL-encoded string. If you don't include this field, the message's previous
blocks
will be retained. To remove previousblocks
, include an empty array for this field.
- channel string - Channel containing the message to be updated.
- link_names? string - Find and link channel names and usernames. Defaults to
none
. If you do not specify a value for this field, the original value set for the message will be overwritten with the default,none
.
- parse? string - Change how messages are treated. Defaults to
client
, unlikechat.postMessage
. Accepts eithernone
orfull
. If you do not specify a value for this field, the original value set for the message will be overwritten with the default,client
.
- text? string - New text for the message, using the default formatting rules. It's not required when presenting
blocks
orattachments
.
- ts string - Timestamp of the message to be updated.
slack: ChatDeleteResponse
Schema for successful response of chat.delete method
Fields
- channel ChannelDef -
- ok OkTrueDef -
- ts TsDef -
slack: ChatDeleteScheduledMessageResponse
Schema for successful response from chat.deleteScheduledMessage method
Fields
- ok OkTrueDef -
slack: ChatGetPermalinkResponse
Schema for successful response chat.getPermalink
Fields
- channel ChannelDef -
- ok OkTrueDef -
- permalink string -
slack: ChatMeMessageResponse
Schema for successful response from chat.meMessage method
Fields
- channel? ChannelDef -
- ok OkTrueDef -
- ts? TsDef -
slack: ChatPostEphemeralResponse
Schema for successful response from chat.postEphemeral method
Fields
- message_ts TsDef -
- ok OkTrueDef -
slack: ChatPostMessageResponse
Schema for successful response of chat.postMessage method
Fields
- channel ChannelDef -
- message MessageObj -
- ok OkTrueDef -
- ts TsDef -
slack: ChatScheduledMessagesListResponse
Schema for successful response from chat.scheduledMessages.list method
Fields
- ok OkTrueDef -
- response_metadata AdminConversationsGetTeamsResponse_response_metadata -
- scheduled_messages ChatScheduledMessagesListResponse_scheduled_messages[] -
slack: ChatScheduledMessagesListResponse_scheduled_messages
Fields
- channel_id ChannelIdDef -
- date_created int -
- id string -
- post_at int -
- text? string -
slack: ChatScheduleMessageResponse
Schema for successful response of chat.scheduleMessage method
Fields
- channel ChannelDef -
- message ChatScheduleMessageResponse_message -
- ok OkTrueDef -
- post_at int -
- scheduled_message_id string -
slack: ChatScheduleMessageResponse_message
Fields
- bot_id BotIdDef -
- bot_profile? BotProfileObj -
- team TeamDef -
- text string -
- 'type string -
- user UserIdDef -
- username? string -
slack: ChatUnfurlResponse
Schema for successful response from chat.unfurl method
Fields
- ok OkTrueDef -
slack: ChatUpdateResponse
Schema for successful response of chat.update method
Fields
- channel string -
- message Message\ object -
- ok OkTrueDef -
- text string -
- ts string -
slack: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy? ProxyConfig - Proxy server related options
slack: CommentObj
Fields
- comment string -
- created int -
- id CommentIdDef -
- is_intro boolean -
- is_starred? boolean -
- num_stars? int -
- pinned_info? PinnedInfoDef -
- pinned_to? ChannelDef[] -
- reactions? ReactionObj[] -
- timestamp int -
- user UserIdDef -
slack: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig|OAuth2RefreshTokenGrantConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings? ClientHttp1Settings - Configurations related to HTTP/1.x protocol
- http2Settings? ClientHttp2Settings - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig? PoolConfiguration - Configurations associated with request pooling
- cache? CacheConfig - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker? CircuitBreakerConfig - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig? RetryConfig - Configurations associated with retrying
- responseLimits? ResponseLimitConfigs - Configurations associated with inbound response size limits
- secureSocket? ClientSecureSocket - SSL/TLS-related options
- proxy? ProxyConfig - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
slack: conversations_archive_body
Fields
- channel? string - ID of conversation to archive
slack: conversations_close_body
Fields
- channel? string - Conversation to close.
slack: conversations_create_body
Fields
- is_private? boolean - Create a private channel instead of a public one
- name? string - Name of the public or private channel to create
slack: Conversations_historyQueries
Represents the Queries record for the operation: conversations_history
Fields
- cursor? string - Paginate through collections of data by setting the
cursor
parameter to anext_cursor
attribute returned by a previous request'sresponse_metadata
. Default value fetches the first "page" of the collection. See pagination for more detail.
- inclusive? boolean - Include messages with latest or oldest timestamp in results only when either timestamp is specified.
- oldest? decimal - Start of time range of messages to include in results.
- channel? string - Conversation ID to fetch history for.
- 'limit? int - The maximum number of items to return. Fewer than the requested number of items may be returned, even if the end of the users list hasn't been reached.
- latest? decimal - End of time range of messages to include in results.
slack: Conversations_infoQueries
Represents the Queries record for the operation: conversations_info
Fields
- include_num_members? boolean - Set to
true
to include the member count for the specified conversation. Defaults tofalse
- channel? string - Conversation ID to learn more about
- include_locale? boolean - Set this to
true
to receive the locale for this conversation. Defaults tofalse
slack: conversations_invite_body
Fields
- channel? string - The ID of the public or private channel to invite user(s) to.
- users? string - A comma separated list of user IDs. Up to 1000 users may be listed.
slack: conversations_join_body
Fields
- channel? string - ID of conversation to join
slack: conversations_kick_body
Fields
- channel? string - ID of conversation to remove user from.
- user? string - User ID to be removed.
slack: conversations_leave_body
Fields
- channel? string - Conversation to leave
slack: Conversations_listQueries
Represents the Queries record for the operation: conversations_list
Fields
- cursor? string - Paginate through collections of data by setting the
cursor
parameter to anext_cursor
attribute returned by a previous request'sresponse_metadata
. Default value fetches the first "page" of the collection. See pagination for more detail.
- types? string - Mix and match channel types by providing a comma-separated list of any combination of
public_channel
,private_channel
,mpim
,im
- 'limit? int - The maximum number of items to return. Fewer than the requested number of items may be returned, even if the end of the list hasn't been reached. Must be an integer no larger than 1000.
- exclude_archived? boolean - Set to
true
to exclude archived channels from the list
slack: conversations_mark_body
Fields
- channel? string - Channel or conversation to set the read cursor for.
- ts? decimal - Unique identifier of message you want marked as most recently seen in this conversation.
slack: Conversations_membersQueries
Represents the Queries record for the operation: conversations_members
Fields
- cursor? string - Paginate through collections of data by setting the
cursor
parameter to anext_cursor
attribute returned by a previous request'sresponse_metadata
. Default value fetches the first "page" of the collection. See pagination for more detail.
- channel? string - ID of the conversation to retrieve members for
- 'limit? int - The maximum number of items to return. Fewer than the requested number of items may be returned, even if the end of the users list hasn't been reached.
slack: conversations_open_body
Fields
- channel? string - Resume a conversation by supplying an
im
ormpim
's ID. Or provide theusers
field instead.
- return_im? boolean - Boolean, indicates you want the full IM channel definition in the response.
- users? string - Comma separated lists of users. If only one user is included, this creates a 1:1 DM. The ordering of the users is preserved whenever a multi-person direct message is returned. Supply a
channel
when not supplyingusers
.
slack: conversations_rename_body
Fields
- channel? string - ID of conversation to rename
- name? string - New name for conversation.
slack: Conversations_repliesQueries
Represents the Queries record for the operation: conversations_replies
Fields
- cursor? string - Paginate through collections of data by setting the
cursor
parameter to anext_cursor
attribute returned by a previous request'sresponse_metadata
. Default value fetches the first "page" of the collection. See pagination for more detail.
- inclusive? boolean - Include messages with latest or oldest timestamp in results only when either timestamp is specified.
- oldest? decimal - Start of time range of messages to include in results.
- channel? string - Conversation ID to fetch thread from.
- 'limit? int - The maximum number of items to return. Fewer than the requested number of items may be returned, even if the end of the users list hasn't been reached.
- ts? decimal - Unique identifier of a thread's parent message.
ts
must be the timestamp of an existing message with 0 or more replies. If there are no replies then just the single message referenced byts
will return - it is just an ordinary, unthreaded message.
- latest? decimal - End of time range of messages to include in results.
slack: conversations_setPurpose_body
Fields
- channel? string - Conversation to set the purpose of
- purpose? string - A new, specialer purpose
slack: conversations_setTopic_body
Fields
- channel? string - Conversation to set the topic of
- topic? string - The new topic string. Does not support formatting or linkification.
slack: conversations_unarchive_body
Fields
- channel? string - ID of conversation to unarchive
slack: ConversationsArchiveResponse
Schema for successful response conversations.archive method
Fields
- ok OkTrueDef -
slack: ConversationsCloseResponse
Schema for successful response conversations.close method
Fields
- already_closed? boolean -
- no_op? boolean -
- ok OkTrueDef -
slack: ConversationsCreateResponse
Schema for successful response conversations.create method
Fields
- channel ConversationObj -
- ok OkTrueDef -
slack: ConversationsHistoryResponse
Schema for successful response from conversations.history method
Fields
- channel_actions_count int -
- channel_actions_ts (anydata)[] -
- has_more boolean -
- messages MessageObj[] -
- ok OkTrueDef -
- pin_count int -
slack: ConversationsInfoResponse
Schema for successful response conversations.info
Fields
- channel ConversationObj -
- ok OkTrueDef -
slack: ConversationsInviteErrorResponse
Schema for successful response from conversations.invite method
Fields
- channel ConversationObj -
- ok OkTrueDef -
slack: ConversationsJoinResponse
Schema for successful response from conversations.join method
Fields
- channel ConversationObj -
- ok OkTrueDef -
- response_metadata? Response\ metadata -
- warning? string -
slack: ConversationsKickResponse
Schema for successful response conversations.kick method
Fields
- ok OkTrueDef -
slack: ConversationsLeaveResponse
Schema for successful response from conversations.leave method
Fields
- not_in_channel? true -
- ok OkTrueDef -
slack: ConversationsListResponse
Schema for successful response from conversations.list method
Fields
- channels ConversationObj[] -
- ok OkTrueDef -
- response_metadata? AdminConversationsGetTeamsResponse_response_metadata -
slack: ConversationsMarkResponse
Schema for successful response conversations.mark method
Fields
- ok OkTrueDef -
slack: ConversationsMembersResponse
Schema for successful response conversations.members method
Fields
- members UserIdDef[] -
- ok OkTrueDef -
- response_metadata AdminConversationsGetTeamsResponse_response_metadata -
slack: ConversationsOpenResponse
Schema for successful response from conversations.open method when opening channels, ims, mpims
Fields
- already_open? boolean -
- channel (ConversationObj|record {| created string, id DmIdDef, is_im boolean, is_open boolean, last_read TsDef, latest MessageObj, unread_count decimal, unread_count_display decimal, user UserIdDef |})[] -
- no_op? boolean -
- ok OkTrueDef -
slack: ConversationsRenameResponse
Schema for successful response from conversations.rename method
Fields
- channel ConversationObj -
- ok OkTrueDef -
slack: ConversationsRepliesResponse
Schema for successful response from conversations.replies method
Fields
- has_more? boolean -
- messages (record {| last_read TsDef, latest_reply TsDef, reply_count int, reply_users UserIdDef[], reply_users_count int, source_team TeamDef, subscribed boolean, team TeamDef, text string, thread_ts TsDef, ts TsDef, 'type string, unread_count int, user UserIdDef, user_profile UserProfileShortObj, user_team TeamDef |}|record {| is_starred boolean, parent_user_id UserIdDef, source_team TeamDef, team TeamDef, text string, thread_ts TsDef, ts TsDef, 'type string, user UserIdDef, user_profile UserProfileShortObj, user_team TeamDef |})[][] -
- ok OkTrueDef -
slack: ConversationsSetPurposeResponse
Schema for successful response from conversations.setPurpose method
Fields
- channel ConversationObj -
- ok OkTrueDef -
slack: ConversationsSetTopicResponse
Schema for successful response from conversations.setTopic method
Fields
- channel ConversationObj -
- ok OkTrueDef -
slack: ConversationsUnarchiveResponse
Schema for successful response from conversations.unarchive method
Fields
- ok OkTrueDef -
slack: DefaultSuccessResponse
This method either only returns a brief OK response or a verbose schema is not available for this method.
Fields
- ok OkTrueDef -
slack: Dialog_openQueries
Represents the Queries record for the operation: dialog_open
Fields
- dialog string - The dialog definition. This must be a JSON-encoded string.
- trigger_id string - Exchange a trigger to post to the user.
slack: DialogOpenResponse
Schema for successful response from dialog.open method
Fields
- ok OkTrueDef -
slack: Dnd_infoQueries
Represents the Queries record for the operation: dnd_info
Fields
- user? string - User to fetch status for (defaults to current user)
slack: dnd_setSnooze_body
Fields
- num_minutes string - Number of minutes, from now, to snooze until.
- token string - Authentication token. Requires scope:
dnd:write
slack: Dnd_teamInfoQueries
Represents the Queries record for the operation: dnd_teamInfo
Fields
- users? string - Comma-separated list of users to fetch Do Not Disturb status for
slack: DndEndDndResponse
Schema for successful response from dnd.endDnd method
Fields
- ok OkTrueDef -
slack: DndEndSnoozeResponse
Schema for successful response from dnd.endSnooze method
Fields
- dnd_enabled boolean -
- next_dnd_end_ts int -
- next_dnd_start_ts int -
- ok OkTrueDef -
- snooze_enabled boolean -
slack: DndInfoResponse
Schema for successful response from dnd.info method
Fields
- dnd_enabled boolean -
- next_dnd_end_ts int -
- next_dnd_start_ts int -
- ok OkTrueDef -
- snooze_enabled? boolean -
- snooze_endtime? int -
- snooze_remaining? int -
slack: DndSetSnoozeResponse
Schema for successful response from dnd.setSnooze method
Fields
- ok OkTrueDef -
- snooze_enabled boolean -
- snooze_endtime int -
- snooze_remaining int -
slack: EnterpriseUserObj
Fields
- enterprise_id EnterpriseIdDef -
- enterprise_name EnterpriseNameDef -
- id EnterpriseUserIdDef -
- is_admin boolean -
- is_owner boolean -
- teams TeamDef[] -
slack: ExternalOrgMigrationsObj
Fields
- current ExternalOrgMigrationsObj_current[] -
- date_updated int -
slack: ExternalOrgMigrationsObj_current
Fields
- date_started int -
- team_id string -
slack: FileObj
Fields
- channels? ChannelIdDef[] -
- comments_count? int -
- created? int -
- date_delete? int -
- display_as_bot? boolean -
- editable? boolean -
- editor? UserIdDef -
- external_id? string -
- external_type? string -
- external_url? string -
- filetype? string -
- groups? GroupIdDef[] -
- has_rich_preview? boolean -
- id? FileIdDef -
- image_exif_rotation? int -
- ims? DmIdDef[] -
- is_external? boolean -
- is_public? boolean -
- is_starred? boolean -
- is_tombstoned? boolean -
- last_editor? UserIdDef -
- mimetype? string -
- mode? string -
- name? string -
- non_owner_editable? boolean -
- num_stars? int -
- original_h? int -
- original_w? int -
- permalink? string -
- permalink_public? string -
- pinned_info? PinnedInfoDef -
- pinned_to? ChannelDef[] -
- pretty_type? string -
- preview? string -
- public_url_shared? boolean -
- reactions? ReactionObj[] -
- shares? FileObj_shares -
- size? int -
- source_team? TeamDef -
- state? string -
- thumb_1024? string -
- thumb_1024_h? int -
- thumb_1024_w? int -
- thumb_160? string -
- thumb_360? string -
- thumb_360_h? int -
- thumb_360_w? int -
- thumb_480? string -
- thumb_480_h? int -
- thumb_480_w? int -
- thumb_64? string -
- thumb_720? string -
- thumb_720_h? int -
- thumb_720_w? int -
- thumb_80? string -
- thumb_800? string -
- thumb_800_h? int -
- thumb_800_w? int -
- thumb_960? string -
- thumb_960_h? int -
- thumb_960_w? int -
- thumb_tiny? string -
- timestamp? int -
- title? string -
- updated? int -
- url_private? string -
- url_private_download? string -
- user? string -
- user_team? TeamDef -
- username? string -
slack: FileObj_shares
Fields
- 'private? record {||} -
- 'public? record {||} -
slack: files_comments_delete_body
Fields
- file? string - File to delete a comment from.
- id? string - The comment to delete.
slack: files_delete_body
Fields
- file? string - ID of file to delete.
slack: Files_infoQueries
Represents the Queries record for the operation: files_info
Fields
- cursor? string - Parameter for pagination. File comments are paginated for a single file. Set
cursor
equal to thenext_cursor
attribute returned by the previous request'sresponse_metadata
. This parameter is optional, but pagination is mandatory: the default value simply fetches the first "page" of the collection of comments. See pagination for more details.
- file? string - Specify a file by providing its ID.
- count? string -
- 'limit? int - The maximum number of items to return. Fewer than the requested number of items may be returned, even if the end of the list hasn't been reached.
- page? string -
slack: Files_listQueries
Represents the Queries record for the operation: files_list
Fields
- ts_from? decimal - Filter files created after this timestamp (inclusive).
- show_files_hidden_by_limit? boolean - Show truncated file info for files hidden due to being too old, and the team who owns the file being over the file limit.
- ts_to? decimal - Filter files created before this timestamp (inclusive).
- channel? string - Filter files appearing in a specific channel, indicated by its ID.
- count? string -
- page? string -
- user? string - Filter files created by a single user.
slack: files_remote_add_body
Fields
- external_id? string - Creator defined GUID for the file.
- external_url? string - URL of the remote file.
- filetype? string - type of file
- indexable_file_contents? string - A text file (txt, pdf, doc, etc.) containing textual search terms that are used to improve discovery of the remote file.
- preview_image? string - Preview of the document via
multipart/form-data
.
- title? string - Title of the file being shared.
- token? string - Authentication token. Requires scope:
remote_files:write
slack: Files_remote_infoQueries
Represents the Queries record for the operation: files_remote_info
Fields
- file? string - Specify a file by providing its ID.
- external_id? string - Creator defined GUID for the file.
slack: Files_remote_listQueries
Represents the Queries record for the operation: files_remote_list
Fields
- ts_from? decimal - Filter files created after this timestamp (inclusive).
- cursor? string - Paginate through collections of data by setting the
cursor
parameter to anext_cursor
attribute returned by a previous request'sresponse_metadata
. Default value fetches the first "page" of the collection. See pagination for more detail.
- ts_to? decimal - Filter files created before this timestamp (inclusive).
- channel? string - Filter files appearing in a specific channel, indicated by its ID.
- 'limit? int - The maximum number of items to return.
slack: files_remote_remove_body
Fields
- external_id? string - Creator defined GUID for the file.
- file? string - Specify a file by providing its ID.
- token? string - Authentication token. Requires scope:
remote_files:write
slack: Files_remote_shareQueries
Represents the Queries record for the operation: files_remote_share
Fields
- file? string - Specify a file registered with Slack by providing its ID. Either this field or
external_id
or both are required.
- channels? string - Comma-separated list of channel IDs where the file will be shared.
- external_id? string - The globally unique identifier (GUID) for the file, as set by the app registering the file with Slack. Either this field or
file
or both are required.
slack: files_remote_update_body
Fields
- external_id? string - Creator defined GUID for the file.
- external_url? string - URL of the remote file.
- file? string - Specify a file by providing its ID.
- filetype? string - type of file
- indexable_file_contents? string - File containing contents that can be used to improve searchability for the remote file.
- preview_image? string - Preview of the document via
multipart/form-data
.
- title? string - Title of the file being shared.
- token? string - Authentication token. Requires scope:
remote_files:write
slack: files_revokePublicURL_body
Fields
- file? string - File to revoke
slack: files_sharedPublicURL_body
Fields
- file? string - File to share
slack: files_upload_body
Fields
- channels? string - Comma-separated list of channel names or IDs where the file will be shared.
- content? string - File contents via a POST variable. If omitting this parameter, you must provide a
file
.
- file? string - File contents via
multipart/form-data
. If omitting this parameter, you must submitcontent
.
- filename? string - Filename of file.
- initial_comment? string - The message text introducing the file in specified
channels
.
- thread_ts? decimal - Provide another message's
ts
value to upload this file as a reply. Never use a reply'sts
value; use its parent instead.
- title? string - Title of file.
- token? string - Authentication token. Requires scope:
files:write:user
slack: FilesCommentsDeleteResponse
Schema for successful response files.comments.delete method
Fields
- ok OkTrueDef -
slack: FilesDeleteResponse
Schema for successful response files.delete method
Fields
- ok OkTrueDef -
slack: FilesInfoResponse
Schema for successful response from files.info method
Fields
- comments CommentsObj -
- content_html? anydata? -
- editor? UserIdDef -
- file FileObj -
- ok OkTrueDef -
- paging? PagingObj -
- response_metadata? ResponseMetadataObj -
slack: FilesListResponse
Schema for successful response from files.list method
Fields
- files FileObj[] -
- ok OkTrueDef -
- paging PagingObj -
slack: FilesRevokePublicURLResponse
Schema for successful response from files.revokePublicURL method
Fields
- file FileObj -
- ok OkTrueDef -
slack: FilesSharedPublicURLResponse
Schema for successful response from files.sharedPublicURL method
Fields
- file FileObj -
- ok OkTrueDef -
slack: FilesUploadResponse
Schema for successful response files.upload method
Fields
- file FileObj -
- ok OkTrueDef -
slack: IconObj
Fields
- image_102? string -
- image_132? string -
- image_230? string -
- image_34? string -
- image_44? string -
- image_68? string -
- image_88? string -
- image_default? boolean -
slack: Message\ object
Fields
- attachments? record {}[] -
- blocks? record {} -
- text string -
slack: MessageObj
Fields
- attachments? MessageObj_attachments[] -
- blocks? blocks -
- bot_id? (anydata)[] -
- bot_profile? BotProfileObj -
- client_msg_id? string -
- comment? CommentObj -
- display_as_bot? boolean -
- file? FileObj -
- files? FileObj[] -
- icons? MessageObj_icons -
- inviter? UserIdDef -
- is_delayed_message? boolean -
- is_intro? boolean -
- is_starred? boolean -
- last_read? TsDef -
- latest_reply? TsDef -
- name? string -
- old_name? string -
- parent_user_id? UserIdDef -
- permalink? string -
- pinned_to? ChannelDef[] -
- purpose? string -
- reactions? ReactionObj[] -
- reply_count? int -
- reply_users? UserIdDef[] -
- reply_users_count? int -
- source_team? WorkspaceIdDef -
- subscribed? boolean -
- subtype? string -
- team? WorkspaceIdDef -
- text string -
- thread_ts? TsDef -
- topic? string -
- ts TsDef -
- 'type string -
- unread_count? int -
- upload? boolean -
- user? UserIdDef -
- user_profile? UserProfileShortObj -
- user_team? WorkspaceIdDef -
- username? string -
slack: MessageObj_attachments
Fields
- fallback? string -
- id int -
- image_bytes? int -
- image_height? int -
- image_url? string -
- image_width? int -
slack: MessageObj_icons
Fields
- emoji? string -
- image_64? string -
slack: Migration_exchangeQueries
Represents the Queries record for the operation: migration_exchange
Fields
- to_old? boolean - Specify
true
to convertW
global user IDs to workspace-specificU
IDs. Defaults tofalse
.
- team_id? string - Specify team_id starts with
T
in case of Org Token
- users string - A comma-separated list of user ids, up to 400 per request
slack: MigrationExchangeResponse
Schema for successful response from migration.exchange method
Fields
- enterprise_id string -
- invalid_user_ids? string[] -
- ok OkTrueDef -
- team_id TeamDef -
- user_id_map? record {} -
slack: OAuth2RefreshTokenGrantConfig
OAuth2 Refresh Token Grant Configs
Fields
- Fields Included from *OAuth2RefreshTokenGrantConfig
- refreshUrl string(default "https://slack.com/api/oauth.access") - Refresh URL
slack: Oauth_accessQueries
Represents the Queries record for the operation: oauth_access
Fields
- single_channel? boolean - Request the user to add your app only to a single channel. Only valid with a legacy workspace app.
- code? string - The
code
param returned via the OAuth callback.
- client_secret? string - Issued when you created your application.
- redirect_uri? string - This must match the originally submitted URI (if one was sent).
- client_id? string - Issued when you created your application.
slack: Oauth_tokenQueries
Represents the Queries record for the operation: oauth_token
Fields
- single_channel? boolean - Request the user to add your app only to a single channel.
- code? string - The
code
param returned via the OAuth callback.
- client_secret? string - Issued when you created your application.
- redirect_uri? string - This must match the originally submitted URI (if one was sent).
- client_id? string - Issued when you created your application.
slack: Oauth_v2_accessQueries
Represents the Queries record for the operation: oauth_v2_access
Fields
- code string - The
code
param returned via the OAuth callback.
- client_secret? string - Issued when you created your application.
- redirect_uri? string - This must match the originally submitted URI (if one was sent).
- client_id? string - Issued when you created your application.
slack: PagingObj
Fields
- count? int -
- page int -
- pages? int -
- per_page? int -
- spill? int -
- total int -
slack: PinnedInfoDef
slack: pins_add_body
Fields
- channel string - Channel to pin the item in.
- timestamp? string - Timestamp of the message to pin.
slack: Pins_listQueries
Represents the Queries record for the operation: pins_list
Fields
- channel string - Channel to get pinned items for.
slack: pins_remove_body
Fields
- channel string - Channel where the item is pinned to.
- timestamp? string - Timestamp of the message to un-pin.
slack: PinsAddResponse
Schema for successful response from pins.add method
Fields
- ok OkTrueDef -
slack: PinsRemoveResponse
Schema for successful response from pins.remove method
Fields
- ok OkTrueDef -
slack: PrimaryOwnerObj
Fields
- email string -
- id string -
slack: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
slack: ReactionObj
Fields
- count int -
- name string -
- users UserIdDef[] -
slack: reactions_add_body
Fields
- channel string - Channel where the message to add reaction to was posted.
- name string - Reaction (emoji) name.
- timestamp string - Timestamp of the message to add reaction to.
slack: Reactions_getQueries
Represents the Queries record for the operation: reactions_get
Fields
- file? string - File to get reactions for.
- channel? string - Channel where the message to get reactions for was posted.
- file_comment? string - File comment to get reactions for.
- full? boolean - If true always return the complete reaction list.
- timestamp? string - Timestamp of the message to get reactions for.
slack: Reactions_listQueries
Represents the Queries record for the operation: reactions_list
Fields
- cursor? string - Parameter for pagination. Set
cursor
equal to thenext_cursor
attribute returned by the previous request'sresponse_metadata
. This parameter is optional, but pagination is mandatory: the default value simply fetches the first "page" of the collection. See pagination for more details.
- count? int -
- 'limit? int - The maximum number of items to return. Fewer than the requested number of items may be returned, even if the end of the list hasn't been reached.
- page? int -
- user? string - Show reactions made by this user. Defaults to the authed user.
- full? boolean - If true always return the complete reaction list.
slack: reactions_remove_body
Fields
- channel? string - Channel where the message to remove reaction from was posted.
- file? string - File to remove reaction from.
- file_comment? string - File comment to remove reaction from.
- name string - Reaction (emoji) name.
- timestamp? string - Timestamp of the message to remove reaction from.
slack: ReactionsAddResponse
Schema for successful response from reactions.add method
Fields
- ok OkTrueDef -
slack: ReactionsListResponse
Schema for successful response from reactions.list method
Fields
- items (record {| channel ChannelDef, message MessageObj, 'type "message" |}|record {| file FileObj, 'type "file" |}|record {| comment CommentObj, file FileObj, 'type "file_comment" |})[][] -
- ok OkTrueDef -
- paging? PagingObj -
- response_metadata? ResponseMetadataObj -
slack: ReactionsRemoveResponse
Schema for successful response from reactions.remove method
Fields
- ok OkTrueDef -
slack: ReminderObj
Fields
- complete_ts? int -
- creator UserIdDef -
- id ReminderIdDef -
- recurring boolean -
- text string -
- time? int -
- user UserIdDef -
slack: reminders_add_body
Fields
- text string - The content of the reminder
- time string - When this reminder should happen: the Unix timestamp (up to five years from now), the number of seconds until the reminder (if within 24 hours), or a natural language description (Ex. "in 15 minutes," or "every Thursday")
- user? string - The user who will receive the reminder. If no user is specified, the reminder will go to user who created it.
slack: reminders_complete_body
Fields
- reminder? string - The ID of the reminder to be marked as complete
slack: reminders_delete_body
Fields
- reminder? string - The ID of the reminder
slack: Reminders_infoQueries
Represents the Queries record for the operation: reminders_info
Fields
- reminder? string - The ID of the reminder
slack: RemindersAddResponse
Schema for successful response from reminders.add method
Fields
- ok OkTrueDef -
- reminder ReminderObj -
slack: RemindersCompleteResponse
Schema for successful response from reminders.complete method
Fields
- ok OkTrueDef -
slack: RemindersDeleteResponse
Schema for successful response from reminders.delete method
Fields
- ok OkTrueDef -
slack: RemindersInfoResponse
Schema for successful response from reminders.info method
Fields
- ok OkTrueDef -
- reminder ReminderObj -
slack: RemindersListResponse
Schema for successful response from reminders.list method
Fields
- ok OkTrueDef -
- reminders ReminderObj[] -
slack: ResourcesObj
Fields
- excluded_ids? (ChannelDef|TeamDef)[][] -
- ids (ChannelDef|TeamDef)[][] -
- wildcard? boolean -
slack: Response\ metadata
Fields
- warnings? string[] -
slack: Rtm_connectQueries
Represents the Queries record for the operation: rtm_connect
Fields
- batch_presence_aware? boolean - Batch presence deliveries via subscription. Enabling changes the shape of
presence_change
events. See batch presence.
- presence_sub? boolean - Only deliver presence events when requested by subscription. See presence subscriptions.
slack: RtmConnectResponse
Schema for successful response from rtm.connect method
Fields
- ok OkTrueDef -
- self RtmConnectResponse_self -
- team RtmConnectResponse_team -
- url string -
slack: RtmConnectResponse_self
Fields
- id UserIdDef -
- name string -
slack: RtmConnectResponse_team
Fields
- domain string -
- id TeamDef -
- name string -
slack: Search_messagesQueries
Represents the Queries record for the operation: search_messages
Fields
- highlight? boolean - Pass a value of
true
to enable query highlight markers (see below).
- query string - Search query.
- count? int - Pass the number of results you want per "page". Maximum of
100
.
- page? int -
- sort? string - Return matches sorted by either
score
ortimestamp
.
- sort_dir? string - Change sort direction to ascending (
asc
) or descending (desc
).
slack: stars_add_body
Fields
- channel? string - Channel to add star to, or channel where the message to add star to was posted (used with
timestamp
).
- file? string - File to add star to.
- file_comment? string - File comment to add star to.
- timestamp? string - Timestamp of the message to add star to.
slack: Stars_listQueries
Represents the Queries record for the operation: stars_list
Fields
- cursor? string - Parameter for pagination. Set
cursor
equal to thenext_cursor
attribute returned by the previous request'sresponse_metadata
. This parameter is optional, but pagination is mandatory: the default value simply fetches the first "page" of the collection. See pagination for more details.
- count? string -
- 'limit? int - The maximum number of items to return. Fewer than the requested number of items may be returned, even if the end of the list hasn't been reached.
- page? string -
slack: stars_remove_body
Fields
- channel? string - Channel to remove star from, or channel where the message to remove star from was posted (used with
timestamp
).
- file? string - File to remove star from.
- file_comment? string - File comment to remove star from.
- timestamp? string - Timestamp of the message to remove star from.
slack: StarsAddResponse
Schema for successful response from stars.add method
Fields
- ok OkTrueDef -
slack: StarsListResponse
Schema for successful response from stars.list method
Fields
- items (record {| channel ChannelDef, date_create int, message MessageObj, 'type "message" |}|record {| date_create int, file FileObj, 'type "file" |}|record {| comment CommentObj, date_create int, file FileObj, 'type "file_comment" |}|record {| channel ChannelDef, date_create int, 'type "channel" |}|record {| channel DmIdDef, date_create int, 'type "im" |}|record {| channel GroupIdDef, date_create int, 'type "group" |})[][] -
- ok OkTrueDef -
- paging? PagingObj -
slack: StarsRemoveResponse
Schema for successful response from stars.remove method
Fields
- ok OkTrueDef -
slack: SubteamObj
Fields
- auto_provision boolean -
- auto_type (anydata)[] -
- channel_count? int -
- created_by UserIdDef -
- date_create int -
- date_delete int -
- date_update int -
- deleted_by (anydata)[] -
- description string -
- enterprise_subteam_id string -
- 'handle string -
- id SubteamIdDef -
- is_external boolean -
- is_subteam boolean -
- is_usergroup boolean -
- name string -
- prefs SubteamObj_prefs -
- team_id TeamDef -
- updated_by UserIdDef -
- user_count? int -
- users? UserIdDef[] -
slack: SubteamObj_prefs
Fields
- channels ChannelIdDef[] -
- groups GroupIdDef[] -
slack: Team_accessLogsQueries
Represents the Queries record for the operation: team_accessLogs
Fields
- before? string - End of time range of logs to include in results (inclusive).
- count? string -
- page? string -
slack: Team_billableInfoQueries
Represents the Queries record for the operation: team_billableInfo
Fields
- user? string - A user to retrieve the billable information for. Defaults to all users.
slack: Team_infoQueries
Represents the Queries record for the operation: team_info
Fields
- team? string - Team to get info on, if omitted, will return information about the current team. Will only return team that the authenticated token is allowed to see through external shared channels
slack: Team_integrationLogsQueries
Represents the Queries record for the operation: team_integrationLogs
Fields
- service_id? string - Filter logs to this service. Defaults to all logs.
- count? string -
- change_type? string - Filter logs with this change type. Defaults to all logs.
- page? string -
- app_id? string - Filter logs to this Slack app. Defaults to all logs.
- user? string - Filter logs generated by this user’s actions. Defaults to all logs.
slack: Team_profile_getQueries
Represents the Queries record for the operation: team_profile_get
Fields
- visibility? string - Filter by visibility.
slack: TeamAccessLogsResponse
Schema for successful response from team.accessLogs method
Fields
- logins TeamAccessLogsResponse_logins[] -
- ok OkTrueDef -
- paging PagingObj -
slack: TeamAccessLogsResponse_logins
Fields
- count int -
- country string? -
- date_first int -
- date_last int -
- ip string? -
- isp string? -
- region string? -
- user_agent string -
- user_id UserIdDef -
- username string -
slack: TeamInfoResponse
Schema for successful response from team.info method
Fields
- ok OkTrueDef -
- team TeamObj -
slack: TeamIntegrationLogsResponse
Schema for successful response from team.integrationLogs method
Fields
- logs TeamIntegrationLogsResponse_logs[] -
- ok OkTrueDef -
- paging PagingObj -
slack: TeamIntegrationLogsResponse_logs
Fields
- admin_app_id? AppIdDef -
- app_id AppIdDef -
- app_type string -
- change_type string -
- channel? ChannelDef -
- date string -
- scope string -
- service_id? string -
- service_type? string -
- user_id UserIdDef -
- user_name string -
slack: TeamObj
Fields
- archived? boolean -
- avatar_base_url? string -
- created? int -
- date_create? int -
- deleted? boolean -
- description? string? -
- discoverable? (anydata)[] -
- domain string -
- email_domain string -
- enterprise_id? EnterpriseIdDef -
- enterprise_name? EnterpriseNameDef -
- external_org_migrations? ExternalOrgMigrationsObj -
- has_compliance_export? boolean -
- icon IconObj -
- id WorkspaceIdDef -
- is_assigned? boolean -
- is_enterprise? int -
- is_over_storage_limit? boolean -
- limit_ts? int -
- locale? string -
- messages_count? int -
- msg_edit_window_mins? int -
- name string -
- over_integrations_limit? boolean -
- over_storage_limit? boolean -
- pay_prod_cur? string -
- plan? ""|"std"|"plus"|"compliance"|"enterprise" -
- primary_owner? PrimaryOwnerObj -
- sso_provider? TeamObj_sso_provider -
slack: TeamObj_sso_provider
Fields
- label? string -
- name? string -
- 'type? string -
slack: TeamProfileFieldObj
Fields
- field_name? string? -
- hint string -
- id string -
- is_hidden? boolean -
- label string -
- options? (anydata)[] -
- ordering decimal -
- possible_values? string[]? -
- 'type "text"|"date"|"link"|"mailto"|"options_list"|"user" -
slack: TeamProfileFieldOptionObj
Fields
- is_custom? boolean? -
- is_multiple_entry? boolean? -
- is_protected? boolean? -
- is_scim? boolean? -
slack: TeamProfileGetResponse
Schema for successful response from team.profile.get method
Fields
- ok OkTrueDef -
- profile TeamProfileGetResponse_profile -
slack: TeamProfileGetResponse_profile
Fields
- fields TeamProfileFieldObj[] -
slack: usergroups_create_body
Fields
- channels? string - A comma separated string of encoded channel IDs for which the User Group uses as a default.
- description? string - A short description of the User Group.
- 'handle? string - A mention handle. Must be unique among channels, users and User Groups.
- include_count? boolean - Include the number of users in each User Group.
- name string - A name for the User Group. Must be unique among User Groups.
slack: usergroups_disable_body
Fields
- include_count? boolean - Include the number of users in the User Group.
- usergroup string - The encoded ID of the User Group to disable.
slack: usergroups_enable_body
Fields
- include_count? boolean - Include the number of users in the User Group.
- usergroup string - The encoded ID of the User Group to enable.
slack: Usergroups_listQueries
Represents the Queries record for the operation: usergroups_list
Fields
- include_disabled? boolean - Include disabled User Groups.
- include_users? boolean - Include the list of users for each User Group.
- include_count? boolean - Include the number of users in each User Group.
slack: usergroups_update_body
Fields
- channels? string - A comma separated string of encoded channel IDs for which the User Group uses as a default.
- description? string - A short description of the User Group.
- 'handle? string - A mention handle. Must be unique among channels, users and User Groups.
- include_count? boolean - Include the number of users in the User Group.
- name? string - A name for the User Group. Must be unique among User Groups.
- usergroup string - The encoded ID of the User Group to update.
slack: Usergroups_users_listQueries
Represents the Queries record for the operation: usergroups_users_list
Fields
- include_disabled? boolean - Allow results that involve disabled User Groups.
- usergroup string - The encoded ID of the User Group to update.
slack: usergroups_users_update_body
Fields
- include_count? boolean - Include the number of users in the User Group.
- usergroup string - The encoded ID of the User Group to update.
- users string - A comma separated string of encoded user IDs that represent the entire list of users for the User Group.
slack: UsergroupsCreateResponse
Schema for successful response from usergroups.create method
Fields
- ok OkTrueDef -
- usergroup SubteamObj -
slack: UsergroupsDisableResponse
Schema for successful response from usergroups.disable method
Fields
- ok OkTrueDef -
- usergroup SubteamObj -
slack: UsergroupsEnableResponse
Schema for successful response from usergroups.enable method
Fields
- ok OkTrueDef -
- usergroup SubteamObj -
slack: UsergroupsListResponse
Schema for successful response from usergroups.list method
Fields
- ok OkTrueDef -
- usergroups SubteamObj[] -
slack: UsergroupsUpdateResponse
Schema for successful response from usergroups.update method
Fields
- ok OkTrueDef -
- usergroup SubteamObj -
slack: UsergroupsUsersListResponse
Schema for successful response from usergroups.users.list method
Fields
- ok OkTrueDef -
- users UserIdDef[] -
slack: UsergroupsUsersUpdateResponse
Schema for successful response from usergroups.users.update method
Fields
- ok OkTrueDef -
- usergroup SubteamObj -
slack: UserProfileObj
Fields
- always_active? boolean -
- api_app_id? OptionalAppIdDef -
- avatar_hash string -
- bot_id? BotIdDef -
- display_name string -
- display_name_normalized string -
- email? string? -
- fields record {}[]? -
- first_name? string? -
- guest_expiration_ts? int? -
- guest_invited_by? string? -
- image_1024? string? -
- image_192? string? -
- image_24? string? -
- image_32? string? -
- image_48? string? -
- image_512? string? -
- image_72? string? -
- image_original? string? -
- is_app_user? boolean -
- is_custom_image? boolean -
- is_restricted? boolean? -
- is_ultra_restricted? boolean? -
- last_avatar_image_hash? string -
- last_name? string? -
- memberships_count? int -
- name? string? -
- phone string -
- pronouns? string -
- real_name string -
- real_name_normalized string -
- skype string -
- status_default_emoji? string -
- status_default_text? string -
- status_default_text_canonical? string? -
- status_emoji string -
- status_expiration? int -
- status_text string -
- status_text_canonical? string? -
- team? WorkspaceIdDef -
- title string -
- updated? int -
- user_id? string -
- username? string? -
slack: UserProfileShortObj
Fields
- avatar_hash string -
- display_name string -
- display_name_normalized? string -
- first_name string? -
- image_72 string -
- is_restricted boolean -
- is_ultra_restricted boolean -
- name string -
- real_name string -
- real_name_normalized? string -
- team WorkspaceIdDef -
slack: Users_conversationsQueries
Represents the Queries record for the operation: users_conversations
Fields
- cursor? string - Paginate through collections of data by setting the
cursor
parameter to anext_cursor
attribute returned by a previous request'sresponse_metadata
. Default value fetches the first "page" of the collection. See pagination for more detail.
- types? string - Mix and match channel types by providing a comma-separated list of any combination of
public_channel
,private_channel
,mpim
,im
- 'limit? int - The maximum number of items to return. Fewer than the requested number of items may be returned, even if the end of the list hasn't been reached. Must be an integer no larger than 1000.
- user? string - Browse conversations by a specific user ID's membership. Non-public channels are restricted to those where the calling user shares membership.
- exclude_archived? boolean - Set to
true
to exclude archived channels from the list
slack: users_deletePhoto_body
Fields
- token string - Authentication token. Requires scope:
users.profile:write
slack: Users_getPresenceQueries
Represents the Queries record for the operation: users_getPresence
Fields
- user? string - User to get presence info on. Defaults to the authed user.
slack: Users_infoQueries
Represents the Queries record for the operation: users_info
Fields
- include_locale? boolean - Set this to
true
to receive the locale for this user. Defaults tofalse
- user? string - User to get info on
slack: Users_listQueries
Represents the Queries record for the operation: users_list
Fields
- cursor? string - Paginate through collections of data by setting the
cursor
parameter to anext_cursor
attribute returned by a previous request'sresponse_metadata
. Default value fetches the first "page" of the collection. See pagination for more detail.
- 'limit? int - The maximum number of items to return. Fewer than the requested number of items may be returned, even if the end of the users list hasn't been reached. Providing no
limit
value will result in Slack attempting to deliver you the entire result set. If the collection is too large you may experiencelimit_required
or HTTP 500 errors.
- include_locale? boolean - Set this to
true
to receive the locale for users. Defaults tofalse
slack: Users_lookupByEmailQueries
Represents the Queries record for the operation: users_lookupByEmail
Fields
- email string - An email address belonging to a user in the workspace
slack: Users_profile_getQueries
Represents the Queries record for the operation: users_profile_get
Fields
- include_labels? boolean - Include labels for each ID in custom profile fields
- user? string - User to retrieve profile info for
slack: users_profile_set_body
Fields
- name? string - Name of a single key to set. Usable only if
profile
is not passed.
- profile? string - Collection of key:value pairs presented as a URL-encoded JSON hash. At most 50 fields may be set. Each field name is limited to 255 characters.
- user? string - ID of user to change. This argument may only be specified by team admins on paid teams.
- value? string - Value to set a single key to. Usable only if
profile
is not passed.
slack: users_setPhoto_body
Fields
- crop_w? string - Width/height of crop box (always square)
- crop_x? string - X coordinate of top-left corner of crop box
- crop_y? string - Y coordinate of top-left corner of crop box
- image? string - File contents via
multipart/form-data
.
- token string - Authentication token. Requires scope:
users.profile:write
slack: users_setPresence_body
Fields
- presence string - Either
auto
oraway
slack: UsersConversationsResponse
Schema for successful response from users.conversations method. Returned conversation objects do not include num_members
or is_member
Fields
- channels ConversationObj[] -
- ok OkTrueDef -
- response_metadata? AdminConversationsGetTeamsResponse_response_metadata -
slack: UsersDeletePhotoResponse
Schema for successful response from users.deletePhoto method
Fields
- ok OkTrueDef -
slack: UsersInfoResponse
Schema for successful response from users.info method
Fields
- ok OkTrueDef -
- user UserObj -
slack: UsersListResponse
Schema for successful response from users.list method
Fields
- cache_ts int -
- members UserObj[] -
- ok OkTrueDef -
- response_metadata? ResponseMetadataObj -
slack: UsersLookupByEmailResponse
Schema for successful response from users.lookupByEmail method
Fields
- ok OkTrueDef -
- user UserObj -
slack: UsersProfileGetResponse
Schema for successful response from users.profile.get method
Fields
- ok OkTrueDef -
- profile UserProfileObj -
slack: UsersProfileSetResponse
Schema for successful response from users.profile.set method
Fields
- email_pending? string -
- ok OkTrueDef -
- profile UserProfileObj -
- username string -
slack: UsersSetActiveResponse
Schema for successful response from users.setActive method
Fields
- ok OkTrueDef -
slack: UsersSetPhotoResponse
Schema for successful response from users.setPhoto method
Fields
- ok OkTrueDef -
- profile UsersSetPhotoResponse_profile -
slack: UsersSetPhotoResponse_profile
Fields
- avatar_hash string -
- image_1024 string -
- image_192 string -
- image_24 string -
- image_32 string -
- image_48 string -
- image_512 string -
- image_72 string -
- image_original string -
slack: UsersSetPresenceResponse
Schema for successful response from users.setPresence method
Fields
- ok OkTrueDef -
slack: Views_openQueries
Represents the Queries record for the operation: views_open
Fields
- view string - A view payload. This must be a JSON-encoded string.
- trigger_id string - Exchange a trigger to post to the user.
slack: Views_publishQueries
Represents the Queries record for the operation: views_publish
Fields
- view string - A view payload. This must be a JSON-encoded string.
- user_id string -
id
of the user you want publish a view to.
- hash? string - A string that represents view state to protect against possible race conditions.
slack: Views_pushQueries
Represents the Queries record for the operation: views_push
Fields
- view string - A view payload. This must be a JSON-encoded string.
- trigger_id string - Exchange a trigger to post to the user.
slack: Views_updateQueries
Represents the Queries record for the operation: views_update
Fields
- view? string - A view object. This must be a JSON-encoded string.
- view_id? string - A unique identifier of the view to be updated. Either
view_id
orexternal_id
is required.
- external_id? string - A unique identifier of the view set by the developer. Must be unique for all views on a team. Max length of 255 characters. Either
view_id
orexternal_id
is required.
- hash? string - A string that represents view state to protect against possible race conditions.
slack: Workflows_stepCompletedQueries
Represents the Queries record for the operation: workflows_stepCompleted
Fields
- workflow_step_execute_id string - Context identifier that maps to the correct workflow step execution.
slack: Workflows_stepFailedQueries
Represents the Queries record for the operation: workflows_stepFailed
Fields
- workflow_step_execute_id string - Context identifier that maps to the correct workflow step execution.
- 'error string - A JSON-based object with a
message
property that should contain a human readable error message.
slack: Workflows_updateStepQueries
Represents the Queries record for the operation: workflows_updateStep
Fields
- outputs? string - An JSON array of output objects used during step execution. This is the data your app agrees to provide when your workflow step was executed.
- inputs? string - A JSON key-value map of inputs required from a user during configuration. This is the data your app expects to receive when the workflow step starts. Please note: the embedded variable format is set and replaced by the workflow system. You cannot create custom variables that will be replaced at runtime. Read more about variables in workflow steps here.
- step_name? string - An optional field that can be used to override the step name that is shown in the Workflow Builder.
- step_image_url? string - An optional field that can be used to override app image that is shown in the Workflow Builder.
- workflow_step_edit_id string - A context identifier provided with
view_submission
payloads used to call back toworkflows.updateStep
.
Array types
slack: CommentsObj
CommentsObj
slack: ConversationObj
ConversationObj
slack: blocks
blocks
This is a very loose definition, in the future, we'll populate this with deeper schema in this definition namespace.
slack: ResponseMetadataObj
ResponseMetadataObj
slack: ScopesObj
ScopesObj
slack: UserObj
UserObj
Anydata types
slack: UserObjItemsnull
UserObjItemsnull
slack: ConversationObjItemsnull
ConversationObjItemsnull
String types
slack: TopicPurposeCreatorDef
TopicPurposeCreatorDef
slack: ReminderIdDef
ReminderIdDef
slack: CommentIdDef
CommentIdDef
slack: TsDef
TsDef
slack: EnterpriseIdDef
EnterpriseIdDef
slack: EnterpriseNameDef
EnterpriseNameDef
slack: ChannelIdDef
ChannelIdDef
slack: DmIdDef
DmIdDef
slack: FileIdDef
FileIdDef
slack: TeamDef
TeamDef
slack: AppIdDef
AppIdDef
slack: ChannelNameDef
ChannelNameDef
slack: EnterpriseUserIdDef
EnterpriseUserIdDef
slack: BotIdDef
BotIdDef
slack: ChannelDef
ChannelDef
slack: OptionalAppIdDef
OptionalAppIdDef
slack: WorkspaceIdDef
WorkspaceIdDef
slack: UserIdDef
UserIdDef
slack: GroupIdDef
GroupIdDef
slack: SubteamIdDef
SubteamIdDef
Import
import ballerinax/slack;
Metadata
Released date: 11 months ago
Version: 4.0.0
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.9.3
GraalVM compatible: Yes
Pull count
Total: 6543
Current verison: 92
Weekly downloads
Keywords
Communication/Team Chat
Cost/Freemium
Contributors