slack
Modules
slack
slack.listenerModule slack
API
Declarations
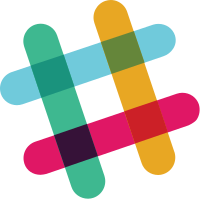
ballerinax/slack Ballerina library
Overview
This module allows you to access the Slack Web API through Ballerina. This module provides the capability to query information and perform some actions in a Slack workspace.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Slack account.
- Visit https://slack.com/get-started#/createnew and create a Slack account.
- Obtain Slack User OAuth Token.
- Visit https://api.slack.com/apps and create a Slack App.
- In the
Add features and functionality
section, ClickPermissions
. - Go to the
Scopes
section and add necessary OAuth scopes inUser Token Scopes
section. ("channels:history", "channels:read", "channels:write", "chat:write", "emoji:read", files:read", "files:write", "groups:read", "reactions:read", "users:read", "users:read.email") - Go back to
Basic Information
section of your Slack App. Then go toInstall your app
section and install the app to the workspace by clickingInstall to Workspace
button. - Get your User OAuth token from the
OAuth & Permissions
section of your Slack App.
Quickstart
To use the Slack connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
Import the ballerinax/slack
module as shown below.
import ballerinax/slack;
Step 2: Create a new connector instance
Create a slack:ConnectionConfig
using your Slack User Oauth Token
and initialize the connector with it.
slack:ConnectionConfig slackConfig = { auth: { token: "SLACK_USER_OAUTH_TOKEN" } }; slack:Client slackClient = check new(slackConfig);
Step 3: Invoke connector operation
- Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to post a message using the connector.
public function main() returns error? { slack:Message messageParams = { channelName: "channelName", text: "Hello" }; string threadTs = slackClient->postMessage(messageParams); }
- Use
bal run
command to compile and run the Ballerina program.
Clients
slack: Client
Ballerina Slack connector provides the capabiliy to access Slack Web API. This connector lets you access the Slack Web API using a Slack User Oath token.
Constructor
Initializes the connector. During initialization, you have to pass a Slack User Oauth Token.
Visit https://api.slack.com/apps and create a Slack App.
Obtain your User OAuth token from the OAuth & Permissions
section of your Slack App.
init (ConnectionConfig config)
- config ConnectionConfig - Configuration required to initialize the
Client
endpoint
createConversation
Creates a channel.
Parameters
- name string - Name of the channel to be created
- isPrivate boolean (default false) -
true
if a private channel,false
if a public channel
archiveConversation
Archives a channel.
Parameters
- channelName string - Name of the channel to archive
Return Type
- error? - An error if it is a failure or
nil
if it is a success
unArchiveConversation
Unarchives a channel.
Parameters
- channelName string - Name of the channel to unarchive
Return Type
- error? - An error if it is a failure or
nil
if it is a success
renameConversation
Renames a channel.
listConversations
function listConversations() returns Conversations|error
Lists all the channels in a slack team.
Return Type
- Conversations|error - An error if it is a failure or the
Conversations
record if it is a success
leaveConversation
Leaves a channel.
Parameters
- channelName string - Name of the channel
Return Type
- error? - An error if it is a failure or 'nil' if it is a success
getConversationInfo
function getConversationInfo(string channelName, boolean includeLocale, boolean memberCount) returns Channel|error
Gets information about a channel.
Parameters
- channelName string - Name of the channel
- includeLocale boolean (default false) - Set this to
true
to receive the locale for this conversation. Defaults tofalse
- memberCount boolean (default false) - Set to
true
to include the member count for the specified conversation. Defaults tofalse
removeUserFromConversation
Removes a user from a channel.
Return Type
- error? - An error if it is a failure or
nil
if it is a success
joinConversation
Joins an existing channel.
Parameters
- channelName string - Name of the channel
Return Type
- error? - An error if it is a failure or 'nil' if it is a success
inviteUsersToConversation
Invites users to a channel.
getConversationHistory
function getConversationHistory(string channelName, string? startOfTimeRange, string? endOfTimeRange) returns stream<MessageInfo, error?>|error
Gets message history of a channel.
Parameters
- channelName string - Name of the channel
- startOfTimeRange string? (default ()) - Start of time range as epoch
- endOfTimeRange string? (default ()) - End of time range as epoch
Return Type
- stream<MessageInfo, error?>|error - Stream of MessageInfo if it is a success or an error if it is a failure
getConversationMembers
Gets userId of all the members of a channel.
Parameters
- channelName string - Name of the channel
Return Type
getUserInfoByUsername
Gets information about a user by username.
Parameters
- username string - Slack username of the user
getUserInfoByUserId
Gets information about a user by userId.
Parameters
- userId string - Slack userId of the user
listUserConversations
function listUserConversations(boolean excludeArchived, int? noOfItems, string? types, string? user) returns Conversations|error
Lists channels which can be accessed by a user.
Parameters
- excludeArchived boolean (default false) - Set to
true
to exclude archived channels from the list
- noOfItems int? (default ()) - Maximum number of items to return
- types string? (default ()) - A comma-separated list of any combination of
public_channel
,private_channel
- user string? (default ()) - Username
Return Type
- Conversations|error - An error if it is a failure or the
Conversations
record if it is a success
lookupUserByEmail
Retrieves a single user by looking them up by their registered email address.
Parameters
- email string - An email address belonging to a user in the workspace
postMessage
Sends a message to a channel.
Parameters
- message Message - Message parameters to be posted on Slack
updateMessage
function updateMessage(UpdateMessage message) returns string|error
Updates a message.
Parameters
- message UpdateMessage - Message parameters to be updated on Slack
deleteMessage
Deletes a message.
Parameters
- channelName string - Name of the channel
- threadTs string - Timestamp of the message to be deleted
Return Type
- error? - An error if it is a failure or 'nil' if it is a success
deleteFile
Deletes a file.
Parameters
- fileId string - Id of the file to be deleted
Return Type
- error? - An error if it is a failure or 'nil' if it is a success
getFileInfo
Gets information of a file.
Parameters
- fileId string - ID of the file
listFiles
function listFiles(string? channelName, int? count, string? tsFrom, string? tsTo, string? types, string? user) returns FileInfo[]|error
Lists files.
Parameters
- channelName string? (default ()) - Name of the channel
- count int? (default ()) - Number of items to return per page
- tsFrom string? (default ()) - Filter files created after this timestamp (inclusive)
- tsTo string? (default ()) - Filter files created before this timestamp (inclusive)
- types string? (default ()) - Type to filter files (ex: types=spaces,snippets)
- user string? (default ()) - Username to filter files created by a single user
Return Type
uploadFile
function uploadFile(string filePath, string? channelName, string? title, string? initialComment, string? threadTs) returns FileInfo|error
Uploads or creates a file.
Enums
slack: Parse
Represents values for Parse
Members
Records
slack: Channel
Contains information about the channel.
Fields
- id string - ID of the channel
- name string - Name of the channel
- isChannel boolean -
true
if a channel or elsefalse
- isGroup boolean -
true
if a group conversation or elsefalse
- isArchived boolean -
true
if the channel is archived
- isGeneral boolean -
true
if the channel is the "general" channel, which includes all regular members
- nameNormalized string - Normalized name
- parentConversation string - Name of the parent conversation if there is one
- creator string - The user ID of the member who created the channel
- isMember boolean? -
true
if the calling member is part of the channel
- isPrivate boolean -
true
if a private channel
- topic Topic - Contains information about the channel topic
- purpose Purpose - Contains information about the channel purpose
slack: ConnectionConfig
Holds the parameters used to create a Client
.
Fields
- auth BearerTokenConfig - Configurations related to client authentication
- httpVersion string(default "1.1") - The HTTP version understood by the client
- http1Settings ClientHttp1Settings(default {}) - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings(default {}) - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- followRedirects FollowRedirects?(default ()) - Configurations associated with Redirection
- poolConfig PoolConfiguration?(default ()) - Configurations associated with request pooling
- cache CacheConfig(default {}) - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig?(default ()) - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig?(default ()) - Configurations associated with retrying
- cookieConfig CookieConfig?(default ()) - Configurations associated with cookies
- responseLimits ResponseLimitConfigs(default {}) - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket?(default ()) - SSL/TLS-related options
slack: Conversations
Contains information about conversations.
Fields
- channels Channel[] - An array of channels
- responseMetadata ResponseMetadata - Response meta data
slack: FileInfo
Contains information about the file.
Fields
- id string - File ID
- created float - Timestamp representing when the file was created
- name string - File name
- title string - File title
- user string - The user ID of the user who uploaded the file
- editable boolean -
true
if the file is stored as editable
- size int - File size in bytes
- mode string - Contains one of hosted, external, snippet, or post
- isExternal boolean -
true
if the master copy of the file is stored within the system otherwisefalse
- externalType string - Type of the external file (e.g., Dropbox or GDoc)
- isPublic boolean -
true
if the file is public
- publicUrlShared boolean -
true
if the file's public URL has been shared
- urlPrivate string - Points to a URL for the file content
- urlPrivateDownload string - Includes headers to force a browser download
- permalink string - The URL pointing to a single page for the file containing details, comments, and a download link
- permalinkPublic string - Points to a public file itself
- lines int - The total count of the lines shown in the snippet
- linesMore int - The count of the lines not shown in the preview
- commentsCount int - The number of comments attached to the file
slack: Message
Parameters associated with posting messages in Slack.
Fields
- channelName string - Channel name
- text string - Text message
- threadTs string? - Provide another message's
ts
value to make this message a reply
- attachments json[]? - A JSON-based array of structured attachments
- blocks json[]? - A JSON-based array of structured blocks
- iconEmoji string? - Emoji to use as the icon for this message
- iconUrl string? - URL to an image to use as the icon for this message
- linkNames boolean? - Set to
true
to find and link channel names and usernames
- mrkdwn boolean? - Set to
true
to enable Slack markup parsing
- parse Parse? - Refers how messages should be treated:
none
, which is the default value orfull
- replyBroadcast boolean? - Used in conjunction with
threadTs
and indicates whether the reply should be made visible to everyone in the channel or conversation
- unfurlLinks boolean? -
true
to enable unfurling of primarily text-based content or otherwisefalse
- unfurlMedia boolean? -
false
to disable unfurling of media content
- asUser boolean? -
true
to post the message as the authed user, instead of as a bot
- username string? - Bot's user name. This must be used in conjunction with
asUser
set tofalse
or otherwise ignored
slack: MessageInfo
Contains information about messages in a Channel.
Fields
- user string - UserId of the author
- text string - Message text
- 'type string -
- ts string - Time stamp of the message
slack: Profile
Contains information about the user profile.
Fields
- title string - Title added in the user profile
- phone string - Phone number
- skype string - Skype ID
- realName string - The real name, which the users specified in their workspace profile
- realNameNormalized string - The
real_name
field with any non-Latin characters filtered out
- displayName string - The display name, which the users have chosen to identify themselves by in their workspace profile
- displayNameNormalized string - The
display_name
field with any non-Latin characters filtered out
slack: Purpose
Contains information about the channel purpose.
Fields
- value string - Value of the purpose
- creator string - The user ID of the member who created the channel
slack: ResponseMetadata
Contains information about the response meta data.
Fields
- nextCursor string - Cursor in the second request
slack: Topic
Contains information about the channel topic.
Fields
- value string - Value of the topic
- creator string - The user ID of the member who created the topic
slack: UpdateMessage
Parameters associated with updating a message.
Fields
- channelName string - Channel name containing the message to be updated
- text string - Text message
- ts string - Timestamp of the message to be updated
- attachments json[]? - A JSON-based array of structured attachments
- blocks json[]? - A JSON-based array of structured blocks
- linkNames boolean? - Set to
true
to find and link channel names and usernames
- parse Parse? - Refers how messages should be treated
- replyBroadcast boolean? - Broadcast an existing thread reply to make it visible to everyone in the channel
slack: User
Contains information about the user.
Fields
- id string - User ID
- teamId string - Team ID
- name string - Name of the user
- deleted boolean -
true
if the user has been deactivated or elsefalse
- realName string - The real name that the users specified in their workspace profile
- tz string - The time zone
- tzLabel string - The commonly-used name of the
tz
timezone
- profile Profile - Contains information about the user's workspace profile
- isAdmin boolean -
true
if the user is an admin of the current workspace
- isOwner boolean -
true
if the user is an owner of the current workspace
- isPrimaryOwner boolean -
true
if the user is the primary owner of the current workspace
- isRestricted boolean -
true
if the user is a guest user
- isBot boolean -
true
if the user is a bot user
- isAppUser boolean -
true
if the user is an authorized user of the calling app
- has2fa boolean -
true
if the user has two factor authentication
Import
import ballerinax/slack;
Metadata
Released date: over 3 years ago
Version: 2.1.0
License: Apache-2.0
Compatibility
Platform: java11
Ballerina version: slbeta6
Pull count
Total: 6496
Current verison: 567
Weekly downloads
Keywords
Communication/Team Chat
Cost/Freemium
Contributors