sinch.voice
Module sinch.voice
API
Definitions
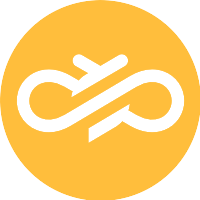
ballerinax/sinch.voice Ballerina library
Overview
This is a generated connector for Sinch Voice API v1.0.0 OpenAPI specification.
The Calling API exposes calling- and conference-related functionality in the Sinch Voice Platform.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Sinch account account
- Obtain tokens by following this guide.
Quickstart
To use the Sinch Voice connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/sinch.voice
module into the Ballerina project.
import ballerinax/sinch.voice;
Step 2: Create a new connector instance
Create a voice:ClientConfig
with the <USERNAME>
and <PASSWORD>
obtained, and initialize the connector with it.
voice:ClientConfig clientConfig = { auth: { username: <USERNAME>, password: <PASSWORD> } }; voice:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example to send callout using the connector.
public function main() { voice:CalloutRequest request = { method: "ttsCallout", ttsCallout: { cli: "+14045001000", destination: { 'type: "number", endpoint: "+14045005000" } } }; voice:GetCalloutResponseObj|error response = baseClient->callouts(request); if (response is voice:GetCalloutResponseObj) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
sinch.voice: Client
This is a generated connector for Sinch Voice API v1.0.0 OpenAPI specification. The Calling API exposes calling- and conference-related functionality in the Sinch Voice Platform.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Sinch account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://calling.api.sinch.com" - URL of the target service
callouts
function callouts(CalloutRequest payload) returns GetCalloutResponseObj|error
Callout Request
Parameters
- payload CalloutRequest - Callout request payload.
Return Type
callingGetcallresult
function callingGetcallresult(string callid) returns GetCallResponseObj|error
Get information about a call
Parameters
- callid string - The unique identifier of the call. This value is generated by the system.
Return Type
- GetCallResponseObj|error - GetCallResultResponse
callingUpdatecall
function callingUpdatecall(string callid, SVAMLRequestBody payload) returns json|error
Update a call in progress
Parameters
- callid string - The unique identifier of the call. This value is generated by the system.
- payload SVAMLRequestBody - Update call request payload.
Return Type
- json|error - Successful response
callingManagecallwithcallleg
function callingManagecallwithcallleg(string callid, string callLeg, SVAMLRequestBody payload) returns json|error
Manage Call with callLeg
Parameters
- callid string - The unique identifier of the call. This value is generated by the system.
- callLeg string - Specifies which part of the call will be managed. This option is used only by the
PlayFiles
andSay
instructions to indicate which channel the sound will be played on. Valid options arecaller
,callee
orboth
. If not specified, the default value iscaller
.</br><Warning>ThecallLeg
identifier is ignored for calls that are part of a conference and calls initiated using the Callout API.</Warning>
- payload SVAMLRequestBody - Manage Call with call leg payload.
Return Type
- json|error - Successful response
callingGetconferenceinfo
function callingGetconferenceinfo(string conferenceid) returns GetConferenceInfoResponse|error
Get Conference Info
Parameters
- conferenceid string - The unique identifier of the conference. The user sets this value.
Return Type
callingKickconferenceall
Kick Conference All (cloud)
Parameters
- conferenceid string - The unique identifier of the conference. The user sets this value.
Return Type
- json|error - No content
callingKickconferenceparticipant
Kick Conference Participant (cloud)
Parameters
- callid string - The unique identifier of the call. This value is generated by the system.
- conferenceid string - The unique identifier of the conference. The user sets this value.
Return Type
- json|error - Successful response
muteUnmuteConferenceParticipant
function muteUnmuteConferenceParticipant(string callid, string conferenceid, json payload) returns json|error
Mute / Unmute Conference participant (cloud)
Parameters
- callid string - The unique identifier of the call. This value is generated by the system.
- conferenceid string - The unique identifier of the conference. The user sets this value.
- payload json - Mute conference participant payload
Return Type
- json|error - Successful response
configurationGetnumbers
function configurationGetnumbers() returns GetNumbersResponseObj|error
Get Numbers
Return Type
configurationUpdatenumbers
function configurationUpdatenumbers(UpdateNumbers payload) returns json|error
Update Numbers
Parameters
- payload UpdateNumbers - Update numbers payload.
Return Type
- json|error - Successful response
configurationUnassignnumber
function configurationUnassignnumber() returns json|error
Un-assign number
Return Type
- json|error - No content
configurationGetcallbackurls
function configurationGetcallbackurls(string applicationkey) returns GetCallbacks|error
Get Callback URLs
Parameters
- applicationkey string - The unique identifying key of the application.
Return Type
- GetCallbacks|error - OK
configurationUpdatecallbackurls
function configurationUpdatecallbackurls(string applicationkey, UpdateCallbacks payload) returns json|error
Update Callbacks
Parameters
- applicationkey string - The unique identifying key of the application.
- payload UpdateCallbacks - Update callbacks payload.
Return Type
- json|error - No content
callingQuerynumber
function callingQuerynumber(string number) returns GetQueryNumber|error
Query number
Parameters
- number string - The phone number you want to query.
Return Type
- GetQueryNumber|error - Successful response
Records
sinch.voice: CalloutRequest
Currently three types of callouts are supported: conference callouts, text-to-speech callouts and custom callouts. The custom callout is the most flexible, but text-to-speech and conferece callouts are more convenient.
Fields
- method string - Sets the type of callout.
- conferenceCallout ConferenceCalloutRequest? - The conference callout calls a phone number or a user. When the call is answered, it's connected to a conference room.
- ttsCallout TtsCalloutRequest? - The text-to-speech callout calls a phone number and plays a synthesized text messages or pre-recorded sound files.
- customCallout CustomCalloutRequest? - The custom callout, the server initiates a call from the servers that can be controlled by specifying how the call should progress at each call event.
sinch.voice: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
sinch.voice: ConferenceCalloutRequest
The conference callout calls a phone number or a user. When the call is answered, it's connected to a conference room.
Fields
- destination Destination - The type of device and number or endpoint to call.
- conferenceId string - The conferenceId of the conference to which you want the callee to join. If the conferenceId doesn't exist a conference room will be created.
- dtmf string? - When the destination picks up, this DTMF tones will be played to the callee. Valid characters in the string are "0"-"9", "#" and "w". A "w" will render a 500 ms pause. Example: "ww1234#w#" will render a 1s pause, the DTMF tones "1", "2", "3", "4" and "#" followed by a 0.5s pause and finally the DTMF tone for "#". This can be used if the callout destination for instance require a conference PIN code or an extension to be entered.
- maxDuration string? - Maximum duration.
- enableAce boolean(default false) - If
enableAce
is set to true and the application has a callback URL specified, you will receive an ACE callback when the call is answered. When the callback is received, your platform must respond with a svamlet, containing the connectcon action in order to add the call to a conference or create the conference if it’s the first call. If it's set to false, no ACE event will be sent to your backend.
- enableDice boolean(default false) - If enableDice is set to true and the application has a callback URL specified, you will receive a DiCE callback when the call is disconnected. If it's set to false, no DiCE event will be sent to your backend.
- enablePie boolean(default false) - If enablePie is set to true and the application has a callback URL specified, you will receive a PIE callback after a runMenu action, with the information of the action that the user took. If it's set to false, no PIE event will be sent to your backend.
- greeting string? - The text that will be spoken as a greeting.
- mohClass string? - Means "music-on-hold." It's an optional parameter that specifies what the first participant should listen to while they're alone in the conference, waiting for other participants to join. It can take one of these pre-defined values:<ul><li>
ring
(progress tone)</li><li>music1
(music file)</li><li>music2
(music file)</li><li>music3
(music file)</li></ul></br>If no music-on-hold is specified, the user will only hear silence.
- custom string? - Used to input custom data.
- domain string? - can be either pstn for PSTN endpoint or mxp for data (app or web) clients.
sinch.voice: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth CredentialsConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
sinch.voice: CustomCalloutRequest
The custom callout, the server initiates a call from the servers that can be controlled by specifying how the call should progress at each call event.
Fields
- destination Destination? - The type of device and number or endpoint to call.
- dtmf string? - When the destination picks up, this DTMF tones will be played to the callee. Valid characters in the string are "0"-"9", "#", and "w". A "w" will render a 500 ms pause. For example, "ww1234#w#" will render a 1s pause, the DTMF tones "1", "2", "3", "4" and "#" followed by a 0.5s pause and finally the DTMF tone for "#". This can be used if the callout destination for instance require a conference PIN code or an extension to be entered.
- maxDuration int? - The maximum amount of time in seconds that the call will last.
- ice string? - Incoming Call Event (ICE) is when a call is placed / made.
- ace string? - Answered Call Event (ACE) is when a call is answered.
- pie string? - Input Event Callback (PIE) triggered from either a user pressing a number of DTMF digits, or by the “return” command.
- dice string? - Disconnected Call Event (DiCE) is when a call is disconnected.
sinch.voice: Destination
The type of device and number or endpoint to call.
Fields
- 'type string - Can be of
number
for PSTN endpoints or ofusername
for data endpoints.
- endpoint string - If the type is
number
the value of the endpoint is a phone number. If the type isusername
the value is the username for a data endpoint.
sinch.voice: Error
Fields
- status string? - A summary of the HTTP error code and error type.
- errorCode string? - The HTTP error code.
- message string? - A simple description of the cause of the error.
- reference string? - If applicable, a reference ID for support to use with diagnosing the error.
sinch.voice: GetCallbacks
Fields
- url GetcallbacksUrl[]? - Gets primary and if configured fallback callback URLs
sinch.voice: GetcallbacksUrl
Fields
- primary string? - Your primary callback URL
- fallback string? - Your fallback callback URL (returned if configured). It is used only if Sinch platform gets a timeout or error from your primary callback URL.
sinch.voice: GetCalloutResponseObj
The returned call ID.
Fields
- callid string? - The returned call identifier.
sinch.voice: GetCallResponseObj
Fields
- 'from string? - Contains the caller information.
- to string? - Contains the callee information.
- domain string? - Can be either
pstn
for PSTN endpoint ormxp
for data (app or web) clients.
- callid string? - The unique identifier of the call.
- duration int? - The duration of the call in seconds.
- status string? - The status of the call. Either
ONGOING
orFINAL
- result string? - Contains the result of a call.
- reason string? - Contains the reason why a call ended.
- timestamp string? - The date and time of the call.
- custom record {}? -
- userRate string? -
- debit string? -
sinch.voice: GetConferenceInfoResponse
Information about the participants in the conference.
Fields
- participants GetconferenceinforesponseParticipants[]? - Array containing information about the participants in the conference.
sinch.voice: GetconferenceinforesponseParticipants
Fields
- cli string? - The phone number of the PSTN participant that was connected in the conference, or whatever was passed as CLI for data originated/terminated calls.
- id string? - The callId of the call leg that the participant joined the conference.
- duration int? - The number of seconds that the participant has been connected to the conference.
- muted boolean? -
sinch.voice: GetNumbersResponseObj
Fields
- numbers GetnumbersresponseobjNumbers[]? - The object type. Will always be list of numbers, associated applicationkeys and capabilities
sinch.voice: GetnumbersresponseobjNumbers
Fields
- number string? - Numbers that you own in E.164 format.
- applicationkey string? - Indicates the application where the number(s) will be assigned. If no number is assigned the applicationkey will not be returned.
- capability string? - indicates the DID capability that needs to be assigned to the chosen application. Valid values are 'voice' and 'sms'. Please note that the DID needs to support the selected capability.
sinch.voice: GetQueryNumber
Fields
- countryId string? - The ISO 3166-1 formatted country code.
- numberType string? - The type of the number.
- normalizedNumber string? - The number in E.164 format.
- restricted boolean? - Concerns whether the call is restricted or not.
- rate GetquerynumberRate? - The cost per minute to call the destination number.
sinch.voice: GetquerynumberRate
The cost per minute to call the destination number.
Fields
- currencyId string? - The currency in which the call is charged.
- amount decimal? - The amount.
sinch.voice: GetRecordingFileInfo
Fields
- 'key string? -
- url string? -
- createdOn string? -
- expiresOn string? -
- headers record {}? -
sinch.voice: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
sinch.voice: SVAMLRequestBody
SVAML is a call control markup language. When a server receives a callback event from the Sinch platform, it can respond with a SVAML object to control the voice call. The following is an example of a SVAML object type and its contents.
Fields
- instructions SvamlrequestbodyInstructions[]? - The collection of instructions that can perform various tasks during the call. You can include as many instructions as necessary.
- action record {}? - The action that will control the call. Each SVAML object can only include one action.
sinch.voice: SvamlrequestbodyInstructions
Fields
- instruction record {}? - Instructions allow an application to play a message or file, start recording, and various other tasks. For more information about instructions, see the SVAML documentation.
sinch.voice: TtsCalloutRequest
The text-to-speech callout calls a phone number and plays a synthesized text messages or pre-recorded sound files.
Fields
- destination Destination - The type of device and number or endpoint to call.
- dtmf string? - When the destination picks up, this DTMF tones will be played to the callee. Valid characters in the string are "0"-"9", "#", and "w". A "w" will render a 500 ms pause. For example, "ww1234#w#" will render a 1s pause, the DTMF tones "1", "2", "3", "4" and "#" followed by a 0.5s pause and finally the DTMF tone for "#". This can be used if the callout destination for instance require a conference PIN code or an extension to be entered.
- domain string? - Can be either
pstn
for PSTN endpoint ormxp
for data (app or web) clients.
- custom string? - Can be used to input custom data.
- text string? - The text that will be spoken in the text-to-speech message. Every application's default maximum characters allowed in text-to-speech is 600 characters. Contact support if you wish this limit to be changed.
- prompts string? - An advanced alternative to using text. You can then supply a , -separated list of prompts. Either prompt can be the name of a pre-recorded file or a text-to-speech string specified as “#tts[my text]. To upload and use pre-recorded files, you need to contact Sinch for support. Every application's default maximum characters allowed per 'prompts'-command text-to-speech is 600 characters. Contact support if you wish this limit to be changed.
- enableAce boolean(default false) - If enableAce is set to true and the application has a callback URL specified, you will receive an ACE callback when the call is answered. When the callback is received, your platform must respond with a svamlet, containing the connectcon action in order to add the call to a conference or create the conference if it’s the first call. If it's set to false, no ACE event will be sent to your backend.
- enableDice boolean(default false) - If enableDice is set to true and the application has a callback URL specified, you will receive a DiCE callback when the call is disconnected. If it's set to false, no DiCE event will be sent to your backend.
sinch.voice: UnassignNumbers
Fields
- number string? - The phone number in E.164 format (https://en.wikipedia.org/wiki/E.164)
- applicationkey string? - Indicates the application where the number(s) was assigned. dIf empty, the application key that is used to sign the request will be used.
- capability string? - (optional) indicates the DID capability that was assigned to the chosen application. Please note that the DID needs to support the selected capability.
sinch.voice: UpdateCallbacks
Fields
- url UpdatecallbacksUrl[]? - Contains primary and or fallback callback URLs
sinch.voice: UpdatecallbacksUrl
Fields
- primary string? - Your primary callback URL
- fallback string? - Your fallback callback URL
sinch.voice: UpdateNumbers
Fields
- numbers string[]? - The phone number or list of numbers in E.164 format.
- applicationkey string? - indicates the application where the number(s) will be assigned. If empty, the application key that is used to sign the request will be used.
- capability string? - indicates the DID capability that needs to be assigned to the chosen application. Valid values are 'voice' and 'sms'. Please note that the DID needs to support the selected capability.
Import
import ballerinax/sinch.voice;
Metadata
Released date: over 1 year ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 0
Current verison: 0
Weekly downloads
Keywords
Communication/Call & SMS
Cost/Paid
Contributors