sinch.sms
Module sinch.sms
API
Definitions
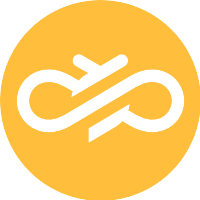
ballerinax/sinch.sms Ballerina library
Overview
This is a generated connector for Sinch SMS API v1 OpenAPI specification.
Sinch SMS API is the one of the easiest APIs we offer and enables you to add fast and reliable global SMS to your applications. It allows for sending single messages, scheduled batch sending, message templates and more.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Sinch account account
- Obtain tokens by following this guide.
Quickstart
To use the Sinch sms connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/sinch.sms
module into the Ballerina project.
import ballerinax/sinch.sms;
Step 2: Create a new connector instance
Create a sms:ClientConfig
with the <BEARER_TOKEN>
obtained, and initialize the connector with it.
sms:ClientConfig clientConfig = { auth : { token: <BEARER_TOKEN> } }; sms:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example to send sms using the connector.
public function main() { string servicePlanID = "jd63jf88477ll"; sms:SendBatchObject message = { 'from: "12345", to: [ "+15551231212" ], body: "Hello how are you" }; sms:SendBatchCreated|error response = baseClient->sendSMS(servicePlanID, message); if (response is sms:SendBatchCreated) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
sinch.sms: Client
This is a generated connector for Sinch SMS API v1 OpenAPI specification. Sinch SMS API is the one of the easiest APIs we offer and enables you to add fast and reliable global SMS to your applications. It allows for sending single messages, scheduled batch sending, message templates and "Only list messages sent from this sender number. Multiple originating numbers can be comma separated.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Sinch account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://us.sms.api.sinch.com" - URL of the target service
listBatches
function listBatches(string servicePlanId, int? page, int pageSize, string? 'from, string? startDate, string? endDate) returns InlineResponse200|error
List Batches
Parameters
- page int? (default ()) - The page number starting from 0.
- pageSize int (default 30) - Determines the size of a page. Default: 30 Constraints: max 100
- 'from string? (default ()) - Only list messages sent from this sender number. Multiple originating numbers can be comma separated.
Return Type
- InlineResponse200|error - OK
sendSMS
function sendSMS(string servicePlanId, SendBatchObject payload) returns SendBatchCreated|error
Send
Parameters
- payload SendBatchObject - Send batch object
Return Type
- SendBatchCreated|error - Created
listInboundMessages
function listInboundMessages(string servicePlanId, int page, int pageSize, string? to, string startDate, string? endDate) returns InboundResponseObject|error
List incoming messages
Parameters
- page int (default 0) - The page number starting from 0.
- pageSize int (default 30) - Determines the size of a page Constraints: Max 100 Default: 30
Return Type
retrieveInboundMessage
function retrieveInboundMessage(string servicePlanId, string inboundId) returns InboundResponseObject|error
Retrieve inbound message
Parameters
- inboundId string - The inbound ID found when listing inbound messages.
Return Type
dryRun
function dryRun(string servicePlanId, SendBatchObject payload, boolean? perRecipient, int numberOfRecipients) returns InlineResponse2001|error
Dry run
Parameters
- payload SendBatchObject - Send batch object
- perRecipient boolean? (default ()) - Whether to include per recipient details in the response
- numberOfRecipients int (default 100) - Max number of recipients to include per recipient details for in the response
Return Type
- InlineResponse2001|error - OK
getBatchMessage
function getBatchMessage(string servicePlanId, string batchId) returns SendBatchCreated|error
Get a batch message
Parameters
- batchId string - The batch ID you received from sending a message.
Return Type
- SendBatchCreated|error - OK
replaceBatch
function replaceBatch(string servicePlanId, string batchId, SendBatchObject payload) returns SendBatchCreated|error
Replace a batch
Parameters
- batchId string - The batch ID you received from sending a message.
- payload SendBatchObject - Send batch object
Return Type
- SendBatchCreated|error - OK
updateBatchMessage
function updateBatchMessage(string servicePlanId, string batchId, UpdateBatchReq payload) returns SendBatchCreated|error
Update a Batch message
Parameters
- batchId string - The batch ID you received from sending a message.
- payload UpdateBatchReq - Update batch request
Return Type
- SendBatchCreated|error - OK
cancelBatchMessage
function cancelBatchMessage(string servicePlanId, string batchId) returns SendBatchObject|error
Cancel a batch message
Parameters
- batchId string - The batch ID you received from sending a message.
Return Type
- SendBatchObject|error - Batch deleted
getDeliveryReportByBatchId
function getDeliveryReportByBatchId(string servicePlanId, string batchId, string 'type, string? status, string? code) returns RetrieveDeliveryResponseObj|error
Retrieve a delivery report
Parameters
- batchId string - The batch ID you received from sending a message.
- 'type string (default "summary") - The type of delivery report. Constraints: Must be summary or full Default: summary
- status string? (default ()) - Comma separated list of delivery_report_statuses to include
- code string? (default ()) - Comma separated list of delivery_receipt_error_codes to include
Return Type
getDeliveryReportByPhoneNumber
function getDeliveryReportByPhoneNumber(string servicePlanId, string batchId, string recipientMsisdn) returns RetrieveRecipientDeliveryResponseObj|error
Retrieve a recipient delivery report
Parameters
- batchId string - The batch ID you received from sending a message.
- recipientMsisdn string - Phone number for which you to want to search.
Return Type
listGroups
function listGroups(string servicePlanId, int page, int pageSize) returns InlineResponse2002|error
List Groups
Parameters
- page int (default 0) - The page number starting from 0 Constraints: 0 or larger Default: 0
- pageSize int (default 30) - Determines the size of a page Constraints: Max 100 Default: 30
Return Type
- InlineResponse2002|error - OK
createGroup
function createGroup(string servicePlanId, GroupObject payload) returns CreateGroupResponse|error
Create group
Parameters
- payload GroupObject - Group object
Return Type
- CreateGroupResponse|error - Created
retrieveGroup
function retrieveGroup(string servicePlanId, string groupId) returns CreateGroupResponse|error
Retrieve a group
Parameters
- groupId string - ID of a group that you are interested in
Return Type
- CreateGroupResponse|error - OK
replaceGroup
function replaceGroup(string servicePlanId, string groupId, GroupsGroupIdBody payload) returns CreateGroupResponse|error
Replace a group
Parameters
- groupId string - ID of a group that you are interested in
- payload GroupsGroupIdBody - Group information
Return Type
- CreateGroupResponse|error - OK
updateGroup
function updateGroup(string servicePlanId, string groupId, GroupsGroupIdBody1 payload) returns CreateGroupResponse|error
Update a group
Parameters
- groupId string - ID of a group that you are interested in
- payload GroupsGroupIdBody1 - Group information
Return Type
- CreateGroupResponse|error - OK
getMembers
Get phone numbers for a group
Parameters
- groupId string - ID of a group that you are interested in
Records
sinch.sms: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
sinch.sms: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth BearerTokenConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
sinch.sms: CreateGroupResponse
Fields
- id string? - The ID used to reference this group
- name string? - Name of group if set
- size int? - The number of members currently in the group
- created_at string? - Timestamp for when the group was created. ISO-8601 format
- modified_at string? - Timestamp for when the group was last modified. ISO-8601 format
sinch.sms: ErrorResponseObj
Fields
- code string? - The error code. See error codes.
- text string? - The human readable description of the error.
sinch.sms: GroupObject
Fields
- name string? - Name of the group
- members Msisdn[]? - Initial list of phone numbers in E.164 format MSISDNs for the group.
- child_groups string[]? - MSISDNs of child group will be included in this group. If present then this group will be auto populated. Constraints: Elements must be group IDs.
- auto_update GroupobjectAutoUpdate? -
sinch.sms: GroupobjectAutoUpdate
Fields
- to string? - Short code or long number addressed in MO. Constraints: Must be valid MSISDN or short code.
- add GroupobjectAutoUpdateAdd? -
- remove GroupobjectAutoUpdateRemove? - Keyword to be sent in MO to remove from a group.
sinch.sms: GroupobjectAutoUpdateAdd
Fields
- first_word string? - Keyword to be sent in MO to add MSISDN to a group Opt-in keyword like "JOIN" If auto_update.to is dedicated long/short number or unique brand keyword like "Sinch" if it is a shared short code. Constraints: Max length: 15 characters Must be one word.
- second_word string? - Opt-in keyword like "JOIN" if auto_update.to is shared short code. Constraints: Max length: 15 characters Must be one word.
sinch.sms: GroupobjectAutoUpdateRemove
Keyword to be sent in MO to remove from a group.
Fields
- first_word string? - Opt-out keyword like "LEAVE" If auto_update.to is dedicated long/short number or unique brand keyword like "Sinch" if it is a shared short code. Constraints: Max length: 15 characters Must be one word.
- second_word string? - Opt-out keyword like "LEAVE" if auto_update.to is shared short code. Constraints: Max length: 15 characters Must be one word.
sinch.sms: GroupsGroupIdBody
Fields
- name string? - Name of group. Constraints: Max 20 characters
sinch.sms: GroupsGroupIdBody1
Fields
- add Msisdn[]? - phone numbers to add as members. Constraints: Elements must be phone numbers. Max 10 000 elements.
- remove Msisdn[]? - phone numbers to remove from group. Constraints: Elements must be phone numbers. Max 10 000 elements.
- name string? - Name of group. Constraints: Max 20 characters
- add_from_group string? - Copy the members from the given group into this group. Constraints: Must be valid group ID
- remove_from_group string? - Remove members in the given group from group. Constraints: Must be valid group ID
sinch.sms: InboundResponseObject
Fields
- page int? - The requested page.
- page_size int? - The number of inbounds returned in this request.
- count int? - The total number of inbounds matching the given filters
- inbounds InboundsObject[]? - The page of inbounds matching the given filters
sinch.sms: InboundsObject
The response object of an inbound message. They can be retrieved and listed or sent via a callback.
Fields
- 'type string? - The object type. Either mo_text or mo_binary.
- id string? - The ID of this inbound message.
- 'from string? - The phone number that sent the message.
- to string? - The Sinch phone number or short code to which the message was sent.
- body string? - The message body. Base64 encoded if type is
mo_binary
.
- operator_id string? - The MCCMNC of the sender's operator if known.
- sent_at string? - When the message left the originating device. Only available if provided by operator. Formatted as ISO-8601.
- received_at string? - When the system received the message. Formatted as ISO-8601.
sinch.sms: InlineResponse200
Fields
- page int? - The requested page.
- count int? - The total number of batches matching the given filters.
- page_size int? - The number of batches returned in this request
- batches SendBatchObject[]? - The page of batches matching the given filters
sinch.sms: InlineResponse2001
Fields
- number_of_recipients int? - The number of recipients in the batch
- number_of_messages int? - The total number of SMS message parts to be sent in the batch
- per_recipient InlineResponse2001PerRecipient[]? - The recipient, the number of message parts to this recipient, the body of the message, and the encoding type of each message
sinch.sms: InlineResponse2001PerRecipient
Fields
- recipient string? -
- message_part string? -
- body string? -
- encoding string? -
sinch.sms: InlineResponse2002
Fields
- page int? -
- page_size int? -
- count int? -
- groups GroupObject[]? -
sinch.sms: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
sinch.sms: RetrieveDeliveryResponseObj
Fields
- 'type string? - The object type. Will always be delivery_report_sms
- batch_id string? - The ID of the batch this delivery report belongs to
- total_message_count string? - The total number of messages for the batch
- statuses RetrievedeliveryresponseobjStatuses[]? - Array with status objects. Only status codes with at least one recipient will be listed.
- client_reference string? - The client identifier of the batch this delivery report belongs to, if set when submitting batch.
sinch.sms: RetrievedeliveryresponseobjStatuses
Fields
- code int? - The detailed status code.
- status string? - The simplified status as described in Delivery Report Statuses
- count int? - The number of messages that currently has this code. Will always be at least 1
- recipients string[]? - Only for full report. A list of the phone number recipients which messages has this status code.
sinch.sms: RetrieveRecipientDeliveryResponseObj
Fields
- applied_originator string? - The default originator used for the recipient this delivery report belongs to, if default originator pool configured and no originator set when submitting batch.
- at string - A timestamp of when the Delivery Report was created in the Sinch service.
- batch_id string - The ID of the batch this delivery report belongs to
- client_reference string? - The client identifier of the batch this delivery report belongs to, if set when submitting batch.
- code int - The detailed status code.
- number_of_message_parts int? - The number of parts the message was split into. Present only if max_number_of_message_parts parameter was set.
- operator string? - The operator that was used for delivering the message to this recipient, if configured for the account.
- operator_status_at string? - A timestamp extracted from the Delivery Receipt from the originating SMSC.
- recipient string - Phone number that was queried for.
- status string - The simplified status as described in Delivery Report Statuses.
- 'type string? - The object type. Will always be recipient_delivery_report_sms.
sinch.sms: SendBatchCreated
Fields
- 'from string? - Sender number. Required if Automatic Default Originator not configured.
- 'type string? - Identifies the type of batch message. Default: mt_text is regular SMS, mt_binary is a binary format.
- body string? - The message content. Normal text string for mt_text and Base64 encoded for mt_binary. Max 1600 chars for mt_text and max 140 bytes together with udh for mt_binary.
- udh string? - The UDH header of a binary message. Max 140 bytes together with body. Required if type is mt_binary.
- delivery_report string? - Request delivery report callback. Note that delivery reports can be fetched from the API regardless of this setting. Valid types are none, summary, full and per_recipient
- send_at string? - If set in the future the message will be delayed until send_at occurs. Must be before expire_at. If set in the past messages will be sent immediately.
- expire_at string? - If set the system will stop trying to deliver the message at this point. Must be after send_at. Default is 3 days after send_at.
- callback_url string? - Override the default callback URL for this batch. Must be valid URL. Max 2048 characters long.
- flash_message boolean? - Shows message on screen without user interaction while not saving the message to the inbox. true or false.
- client_reference string? - The client identifier of batch message. If set, it will be added in the delivery report/callback of this batch Max 128 characters long
- max_number_of_message_parts int? - Message will be dispatched only if it is not split to more parts than Max Number of Message Parts Must be higher or equal 1
- id string? - Unique identifier for batch
- created_at string? - Timestamp for when batch was created. ISO-8601 format
- modified_at string? - Timestamp for when batch was last updated. ISO-8601 format
- canceled boolean? - Indicates if the batch has been canceled or not.
sinch.sms: SendBatchObject
Fields
- 'from string? - Sender number. Required if Automatic Default Originator not configured.
- 'type string(default "mt_text") - Identifies the type of batch message. Default: mt_text
- body string - The message content. Normal text string for mt_text and Base64 encoded for mt_binary. Max 1600 chars for mt_text and max 140 bytes together with udh for mt_binary.
- udh string? - The UDH header of a binary message. Max 140 bytes together with body. Required if type is mt_binary.
- delivery_report string? - Request delivery report callback. Note that delivery reports can be fetched from the API regardless of this setting. Valid types are none, summary, full and per_recipient
- send_at string? - If set in the future the message will be delayed until send_at occurs. Must be before expire_at. If set in the past messages will be sent immediately.
- expire_at string? - If set the system will stop trying to deliver the message at this point. Must be after send_at. Default is 3 days after send_at.
- callback_url string? - Override the default callback URL for this batch. Must be valid URL. Max 2048 characters long.
- flash_message boolean(default false) - Shows message on screen without user interaction while not saving the message to the inbox. true or false.
- client_reference string? - The client identifier of batch message. If set, it will be added in the delivery report/callback of this batch Max 128 characters long
- max_number_of_message_parts int? - Message will be dispatched only if it is not split to more parts than Max Number of Message Parts Must be higher or equal 1
sinch.sms: UpdateBatchReq
Fields
- toAdd string[]? - List of phone numbers and group IDs to add to the batch. Constraints: 1 to 100 elements.
- toRemove string[]? - List of phone numbers and group IDs to remove from the batch. constraints: 1 to 100 elements.
- 'from string? - Sender number. Default: Must be valid phone number, short code or alphanumeric.
- body string? - The message content. Normal text string for mt_text and Base64 encoded for mt_binary. Constraints: Max 1600 chars for mt_text and max 140 bytes together with udh for mt_binary
- delivery_report string? - Request delivery report callback. Note that delivery reports can be fetched from the API regardless of this setting. Constraints: Valid types are none, summary, full and per_recipient
- send_at string? - If set in the future the message will be delayed until send_at occurs. ISO-8601 format. Constraints: Must be before expire_at. If set in the past messages will be sent immediately.
- expire_at string? - If set the system will stop trying to deliver the message at this point. Constraints: Must be after send_at Default: 3 days after send_at
- callback_url string? - Override the default callback URL for this batch. Constraints: Must be valid URL. Max 2048 characters long.
String types
sinch.sms: Msisdn
Msisdn
A phone number in E.164 format.
Import
import ballerinax/sinch.sms;
Metadata
Released date: about 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 6
Current verison: 5
Weekly downloads
Keywords
Communication/Call & SMS
Cost/Paid
Contributors