sinch.conversation
Module sinch.conversation
API
Definitions
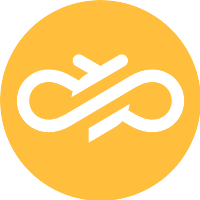
ballerinax/sinch.conversation Ballerina library
Overview
This is a generated connector for Sinch Conversation API v1.0 OpenAPI specification.
Send and receive messages globally over SMS, RCS, WhatsApp, Viber Business, Facebook messenger and other popular channels using the Sinch Conversation API. The Conversation API endpoint uses built-in transcoding to give you the power of conversation across all supported channels and, if required, full control over channel specific features.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Sinch account account
- Obtain tokens by following this guide.
Quickstart
To use the Sinch Conversation connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/sinch.conversation
module into the Ballerina project.
import ballerinax/sinch.conversation;
Step 2: Create a new connector instance
Create a conversation:ClientConfig
with the <USERNAME>
and <PASSWORD>
obtained, and initialize the connector with it.
conversation:ClientConfig clientConfig = { auth: { username: <USERNAME>, password: <PASSWORD> } }; conversation:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example to send message using the connector.
public function main() { string projectId = "{PROJECT_ID}"; conversation:SendMessageRequest request = { app_id: "{APP_ID}", message: {}, project_id: "{PROJECT_ID}", recipient: { contact_id: "{CONTACT_ID}", identified_by: { channel_identities: [ { channel: "WHATSAPP", identity: "string" } ] } } }; conversation:SendMessageResponse|error response = baseClient->messagesSendmessage(projectId, request); if (response is conversation:SendMessageResponse) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
sinch.conversation: Client
This is a generated connector for Sinch Conversation API v1.0 OpenAPI specification. Send and receive messages globally over SMS, RCS, WhatsApp, Viber Business, Facebook messenger and other popular channels using the Sinch Conversation API. The Conversation API endpoint uses built-in transcoding to give you the power of conversation across all supported channels and, if required, full control over channel specific features.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Sinch account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://eu.conversation.api.sinch.com" - URL of the target service
messagesSendmessage
function messagesSendmessage(string projectId, SendMessageRequest payload) returns SendMessageResponse|error
Send a message
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- payload SendMessageRequest - Send message request
Return Type
- SendMessageResponse|error - A successful response.
messagesGetmessage
function messagesGetmessage(string projectId, string messageId) returns ConversationMessage|error
Get a message
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- messageId string - The conversation message ID.
Return Type
- ConversationMessage|error - A successful response.
messagesDeletemessage
Deletes a message
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- messageId string - The conversation message ID.
Return Type
- json|error - A successful response.
appListapps
function appListapps(string projectId) returns ListAppsResponse|error
List all apps for a given project
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
Return Type
- ListAppsResponse|error - A successful response.
appCreateapp
Creates an app
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- payload App - The app to create.
appGetapp
Get an app
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- appId string - The unique ID of the app. You can find this on the Sinch Dashboard.
appDeleteapp
Delete an app
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- appId string - The unique ID of the app. You can find this on the Sinch Dashboard.
Return Type
- json|error - A successful response.
appUpdateapp
function appUpdateapp(string projectId, string appId, App payload, string[]? updateMaskPaths) returns App|error
Update an app
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- appId string - The unique ID of the app. You can find this on the Sinch Dashboard.
- payload App - The updated app.
- updateMaskPaths string[]? (default ()) - The set of field mask paths.
webhooksListwebhooks
function webhooksListwebhooks(string projectId, string appId) returns ListWebhooksResponse|error
List all webhooks for a given app
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- appId string - The unique ID of the app. You can find this on the Sinch Dashboard.
Return Type
- ListWebhooksResponse|error - A successful response.
capabilityQuerycapability
function capabilityQuerycapability(string projectId, QueryCapability payload) returns QueryCapabilityResponse|error
Capability lookup
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- payload QueryCapability - The query capability request.
Return Type
- QueryCapabilityResponse|error - A successful response.
contactListcontacts
function contactListcontacts(string projectId, int? pageSize, string? pageToken) returns ListContactsResponse|error
Get contacts
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- pageSize int? (default ()) - Optional. The maximum number of contacts to fetch. The default is 10 and the maximum is 20.
- pageToken string? (default ()) - Optional. Next page token previously returned if any.
Return Type
- ListContactsResponse|error - A successful response.
contactCreatecontact
Create a Contact
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- payload Contact - Required. The contact to be added.
contactGetcontact
Get a Contact
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- contactId string - The unique ID of the contact.
contactDeletecontact
Delete a Contact
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- contactId string - The unique ID of the contact.
Return Type
- json|error - A successful response.
contactUpdatecontact
function contactUpdatecontact(string projectId, string contactId, Contact payload, string[]? updateMaskPaths) returns Contact|error
Update a Contact
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- contactId string - The unique ID of the contact.
- payload Contact - The updated contact.
- updateMaskPaths string[]? (default ()) - The set of field mask paths.
contactMergecontact
function contactMergecontact(string projectId, string destinationId, MergeContactRequest payload) returns Contact|error
Merge two contacts
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- destinationId string - The unique ID of the contact that should be kept when merging two contacts.
- payload MergeContactRequest - Merge contact request.
conversationListconversations
function conversationListconversations(string projectId, boolean onlyActive, string? appId, string? contactId, int? pageSize, string? pageToken) returns ListConversationsResponse|error
List conversations
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- onlyActive boolean - Required. True if only active conversations should be listed.
- appId string? (default ()) - The ID of the app involved in the conversations.
- contactId string? (default ()) - Resource name (ID) of the contact.
- pageSize int? (default ()) - Optional. The maximum number of conversations to fetch. Defaults to 10 and the maximum is 20.
- pageToken string? (default ()) - Optional. Next page token previously returned if any.
Return Type
- ListConversationsResponse|error - A successful response.
conversationCreateconversation
function conversationCreateconversation(string projectId, Conversation payload) returns Conversation|error
Creates a conversation
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- payload Conversation - The conversation to create. ID will be generated for the conversation and any ID in the given conversation will be ignored.
Return Type
- Conversation|error - A successful response.
conversationGetconversation
function conversationGetconversation(string projectId, string conversationId) returns Conversation|error
Get a conversation
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- conversationId string - The unique ID of the conversation. This is generated by the system.
Return Type
- Conversation|error - A successful response.
conversationDeleteconversation
Deletes a conversation
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- conversationId string - The unique ID of the conversation. This is generated by the system.
Return Type
- json|error - A successful response.
conversationUpdateconversation
function conversationUpdateconversation(string projectId, string conversationId, Conversation payload, string[]? updateMaskPaths) returns Conversation|error
Update a conversation
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- conversationId string - The unique ID of the conversation. This is generated by the system.
- payload Conversation - The updated conversation.
- updateMaskPaths string[]? (default ()) - The set of field mask paths.
Return Type
- Conversation|error - A successful response.
conversationStopactiveconversation
function conversationStopactiveconversation(string projectId, string conversationId) returns json|error
Stop conversation
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- conversationId string - The unique ID of the conversation. This is generated by the system.
Return Type
- json|error - A successful response.
conversationInjectmessage
function conversationInjectmessage(string projectId, string messageConversationId, ConversationMessage payload) returns json|error
Inject messages
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- messageConversationId string - Required. The ID of the conversation.
- payload ConversationMessage - Message to be injected. ID field of the message is ignored and instead generated on the server.
Return Type
- json|error - A successful response.
eventsSendevent
function eventsSendevent(string projectId, SendEventRequest payload) returns SendEventResponse|error
Send an event
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- payload SendEventRequest - Send event request.
Return Type
- SendEventResponse|error - A successful response.
conversationListmessages
function conversationListmessages(string projectId, string? conversationId, string? contactId, int? pageSize, string? pageToken, ConversationMessagesView? view) returns ListMessagesResponse|error
List messages
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- conversationId string? (default ()) - Resource name (ID) of the conversation.
- contactId string? (default ()) - Resource name (ID) of the contact.
- pageSize int? (default ()) - Optional. Maximum number of messages to fetch. Defaults to 10 and the maximum is 20.
- pageToken string? (default ()) - Optional. Next page token previously returned if any.
- view ConversationMessagesView? (default ()) - Conversation message view.
Return Type
- ListMessagesResponse|error - A successful response.
transcodingTranscodemessage
function transcodingTranscodemessage(string projectId, TranscodeMessageRequest payload) returns TranscodeMessageResponse|error
Transcode a message
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- payload TranscodeMessageRequest - Transaction code message request.
Return Type
- TranscodeMessageResponse|error - A successful response.
optinRegisteroptin
function optinRegisteroptin(string projectId, OptIn payload, string? requestId) returns OptInResponse|error
Register an opt-in
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- payload OptIn - OptIn request.
- requestId string? (default ()) - ID for the asynchronous request, will be generated if not set. Currently this field is not used for idempotency but it will be added in v1.
Return Type
- OptInResponse|error - A successful response.
optinRegisteroptout
function optinRegisteroptout(string projectId, OptOut payload, string? requestId) returns OptOutResponse|error
Register an opt-out
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- payload OptOut - OptOut request
- requestId string? (default ()) - ID for the asynchronous request, will be generated if not set. Currently this field is not used for idempotency but it will be added in v1.
Return Type
- OptOutResponse|error - A successful response.
webhooksCreatewebhook
Create a new webhook
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- payload Webhook - Required. The Webhook to create
webhooksGetwebhook
function webhooksGetwebhook(string projectId, string webhookId, string[]? updateMaskPaths) returns Webhook|error
Get a webhook
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- webhookId string - The unique ID of the webhook.
- updateMaskPaths string[]? (default ()) - The set of field mask paths.
webhooksDeletewebhook
function webhooksDeletewebhook(string projectId, string webhookId, string[]? updateMaskPaths) returns json|error
Delete an existing webhook
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- webhookId string - The unique ID of the webhook.
- updateMaskPaths string[]? (default ()) - The set of field mask paths.
Return Type
- json|error - A successful response.
webhooksUpdatewebhook
function webhooksUpdatewebhook(string projectId, string webhookId, Webhook payload, string[]? updateMaskPaths) returns Webhook|error
Update an existing webhook
Parameters
- projectId string - The unique ID of the project. You can find this on the Sinch Dashboard.
- webhookId string - The unique ID of the webhook.
- payload Webhook - Required. The Webhook to update
- updateMaskPaths string[]? (default ()) - The set of field mask paths.
Records
sinch.conversation: App
The app corresponds to the API user and is a collection of channel credentials allowing access to the underlying messaging channels. The app is tied to a set of webhooks which define the destination for various events coming from the Conversation API.
Fields
- channel_credentials ConversationChannelCredential[] - Channel credentials. The order of the credentials defines the app channel priority.
- conversation_metadata_report_view ConversationMetadataReportView? - Conversation metadata report view.
- display_name string? - Human readable identifier of the app.
- id string - The ID of the app you can find this on the Sinch Dashboard.
- rate_limits RateLimits? - Rate limits
- retention_policy RetentionPolicy? - Retention policy for messages and conversations
sinch.conversation: AppEvent
Fields
- composing_event ComposingEvent? -
- comment_reply_event CommentReplyEvent? - Represents a response to a comment event.
sinch.conversation: AppMessage
Message originating from an app
Fields
- card_message CardMessage? - Message containing text, media and choices.
- carousel_message CarouselMessage? - Message containing a list of cards often rendered horizontally on supported channels. Supported types for media are only images, e.g. .png, .jpg, .jpeg extensions.
- choice_message ChoiceMessage? - Message containing choices/actions.
- explicit_channel_message record {}? - Optional. Channel specific messages, overriding any transcoding. The key in the map must point to a valid conversation channel as defined by the enum ConversationChannel.
- location_message LocationMessage? - Message with geo location
- media_message MediaMessage? - Message containing media
- template_message TemplateMessage? - Message referring to predefined template
- text_message TextMessage? - Message containing only text
sinch.conversation: BasicAuthCredential
It consists of a username and a password.
Fields
- password string - Basic auth password.
- username string - Basic auth username.
sinch.conversation: CallMessage
Message for triggering a call.
Fields
- phone_number string - Phone number in E.164 with leading +.
- title string - Title shown close to the phone number. The title is clickable in some cases.
sinch.conversation: CardMessage
Message containing text, media and choices.
Fields
- choices Choice[]? - Optional. The number of choices is limited to 3.
- description string? - Optional.
- height CardHeight? - Card height
- media_message MediaMessage? - Message containing media
- title string? - Required.
sinch.conversation: CarouselMessage
Message containing a list of cards often rendered horizontally on supported channels. Supported types for media are only images, e.g. .png, .jpg, .jpeg extensions.
Fields
- cards CardMessage[] - A list of up to 10 cards.
- choices Choice[]? - Optional. Outer choices on the carousel level. The number of outer choices is limited to 3.
sinch.conversation: ChannelIdentities
Channel identities.
Fields
- channel_identities ChannelRecipientIdentity[] - A list of specific channel identities. The API will use these identities when sending to specific channels.
sinch.conversation: ChannelIdentity
A unique identity of message recipient on a particular channel. For example, the channel identity on SMS, WHATSAPP or VIBERBM is a MSISDN phone number.
Fields
- app_id string? - Optional. The Conversation API's app ID if this is app-scoped channel identity. Currently, FB Messenger and Viber are using app-scoped channel identities which means contacts will have different channel identities for different apps. FB Messenger uses PSIDs (Page-Scoped IDs) as channel identities. The app_id is pointing to the app linked to the FB page for which this PSID is issued.
- channel ConversationChannel - Channel Identifier
- identity string - Required. The channel identity e.g., a phone number for SMS, WhatsApp and Viber Business.
sinch.conversation: ChannelRecipientIdentity
Fields
- channel ConversationChannel - Channel Identifier
- identity string - The channel recipient identity.
sinch.conversation: Choice
A choice is an action the user can take such as buttons for quick replies, call actions etc.
Fields
- call_message CallMessage? - Message for triggering a call.
- location_message LocationMessage? - Message with geo location
- postback_data string? - Optional. This data will be returned in the ChoiceResponseMessage. The default is message_id_{text, title}.
- text_message TextMessage? - Message containing only text
- url_message UrlMessage? - A generic URL message.
sinch.conversation: ChoiceMessage
Message containing choices/actions.
Fields
- choices Choice[] - The number of choices is limited to 3.
- text_message TextMessage? - Message containing only text
sinch.conversation: ChoiceResponseMessage
Represents a response to a choice message.
Fields
- message_id string - The message id containing the choice.
- postback_data string - The postback_data defined in the selected choice.
sinch.conversation: ClientCredentials
Client credentials
Fields
- client_id string - The Client ID that will be used in the Client Credentials flow.
- client_secret string - The Client Secret that will be used in the Client Credentials flow.
- endpoint string - The endpoint that will be used in the Client Credentials flow.
sinch.conversation: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
sinch.conversation: CommentReplyEvent
Represents a response to a comment event.
Fields
- text string - The text of the comment reply.
sinch.conversation: ComposingEvent
sinch.conversation: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth OAuth2ClientCredentialsGrantConfig|CredentialsConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
sinch.conversation: Contact
A participant in a conversation typically representing a person. It is associated with a collection of channel identities.
Fields
- channel_identities ChannelIdentity[]? - List of channel identities.
- channel_priority ConversationChannel[]? - List of channels defining the channel priority.
- display_name string? - Optional. The display name. A default 'Unknown' will be assigned if left empty.
- email string? - Optional. Email of the contact.
- external_id string? - Optional. Contact identifier in an external system.
- id string - The ID of the contact.
- language ContactLanguage - Contact language
- metadata string? - Optional. Metadata associated with the contact. Up to 1024 characters long.
sinch.conversation: ContactMessage
Message originating from a contact
Fields
- choice_response_message ChoiceResponseMessage? - Represents a response to a choice message.
- fallback_message FallbackMessage? - Fallback message, appears when original contact message can not be handled
- location_message LocationMessage? - Message with geo location
- media_card_message MediaCardMessage? - Contact Message containing media and caption
- media_message MediaMessage? - Message containing media
- reply_to ReplyTo? - If the contact message was a response to a previous App message then this field contains information about that.
- text_message TextMessage? - Message containing only text
sinch.conversation: Conversation
A collection of messages exchanged between a contact and an app. Conversations are normally created on the fly by Conversation API once a message is sent and there is no active conversation already. There can be only one active conversation at any given time between a particular contact and an app.
Fields
- active boolean? - Flag for whether this conversation is active.
- active_channel ConversationChannel? - Channel Identifier
- app_id string? - The ID of the participating app.
- contact_id string? - The ID of the participating contact.
- id string? - The ID of the conversation.
- last_received string? - Output only. The timestamp of the latest message in the conversation. The timestamp will be Thursday January 01, 1970 00:00:00 UTC if the conversation contains no messages.
- metadata string? - An arbitrary data set by the Conversation API clients. Up to 1024 characters long.
sinch.conversation: ConversationChannelCredential
Enables access to the underlying messaging channel.
Fields
- callback_secret string? - The secret used to verify the channel callbacks for channels which support callback verification. The callback verification is not needed for Sinch-managed channels because the callbacks are not leaving Sinch internal networks. Max length is 256 characters. Note: leaving channel_callback_secret empty for channels with callback verification will disable the verification.
- channel ConversationChannel - Channel Identifier
- mms_credentials MMSCredentials? - MMS channel credential
- static_bearer StaticBearerCredential? - It consists of claimed identity and a static token.
- static_token StaticTokenCredential? - Static Token Credential
sinch.conversation: ConversationMessage
A message on a particular channel.
Fields
- accept_time string? - Output only.
- app_message AppMessage? - Message originating from an app
- channel_identity ChannelIdentity? - A unique identity of message recipient on a particular channel. For example, the channel identity on SMS, WHATSAPP or VIBERBM is a MSISDN phone number.
- contact_id string? - Required. The ID of the contact.
- contact_message ContactMessage? - Message originating from a contact
- conversation_id string? - Required. The ID of the conversation.
- direction ConversationDirection? - Conversation direction
- id string? - Required. The ID of the message.
- metadata string? - Optional. Metadata associated with the contact. Up to 1024 characters long.
sinch.conversation: Coordinates
Geographic coordinates
Fields
- latitude float? - Required. The latitude.
- longitude float? - Required. The longitude.
sinch.conversation: FallbackMessage
Fallback message, appears when original contact message can not be handled
Fields
- raw_message string? - Optional. The raw fallback message if provided by the channel.
- reason Reason? - Reason
sinch.conversation: ListAppsResponse
Fields
- apps App[]? - List of apps belonging to a specific project ID.
sinch.conversation: ListContactsResponse
Fields
- contacts Contact[]? - List of contacts belonging to the specified project.
- next_page_token string? - Token that should be included in the next list contacts request to fetch the next page.
sinch.conversation: ListConversationsResponse
Fields
- conversations Conversation[]? - Output only. List of conversations matching the search query.
- next_page_token string? -
- total_size int? -
sinch.conversation: ListMessagesResponse
Fields
- messages ConversationMessage[]? - Output only. List of messages associated to the referenced conversation.
- next_page_token string? -
sinch.conversation: ListWebhooksResponse
Fields
- webhooks Webhook[]? -
sinch.conversation: LocationMessage
Message with geo location
Fields
- coordinates Coordinates? - Geographic coordinates
- label string? - Optional. Label or name for the position.
- title string? - Required. The title is shown close to the button or link that leads to a map showing the location. The title is clickable in some cases.
sinch.conversation: MediaCardMessage
Contact Message containing media and caption
Fields
- caption string? - Optional. Caption for the media on channels, where its supported.
- url string? - Required. Url to the file.
sinch.conversation: MediaMessage
Message containing media
Fields
- thumbnail_url string? - Optional. Will be used where it is natively supported
- url string? - Required. Url to the file.
sinch.conversation: MergeContactRequest
Fields
- destination_id string? - Required. The ID of the contact that should be kept.
- project_id string? - Required. The project ID.
- source_id string? - Required. The ID of the contact that should be removed.
- strategy ConversationMergeStrategy? -
sinch.conversation: MMSCredentials
MMS channel credential
Fields
- account_id string? - Required. MMS Account ID.
- api_key string? - Required. MMS API Key.
- basic_auth BasicAuthCredential? - It consists of a username and a password.
sinch.conversation: OAuth2ClientCredentialsGrantConfig
OAuth2 Client Credentials Grant Configs
Fields
- Fields Included from *OAuth2ClientCredentialsGrantConfig
- tokenUrl string(default "https://auth.sinch.com/oauth2/token") - Token URL
sinch.conversation: OptIn
An Opt-In contains the identity of the recipient which gave its consent to receive messages from Conversation API apps on a given channel.
Fields
- app_id string - Required. The app for which the Opt-In is being registered.
- channels ConversationChannel[] - Required. The channels covered by this Opt-In. The default are all the channels for a contact if recipient is contact_id or the channels in the channel_identities list.
- recipient Recipient? - Recipient information
sinch.conversation: OptInResponse
The response received for an Opt-In request.
Fields
- opt_in OptIn? - An Opt-In contains the identity of the recipient which gave its consent to receive messages from Conversation API apps on a given channel.
- request_id string? - ID for the asynchronous request, will be generated id if not set in request
sinch.conversation: OptOut
An Opt-Out contains the identity of the recipient which retract its consent to receive messages from Conversation API apps on a given channel.
Fields
- app_id string - The app for which the Opt-Out is being registered.
- channels ConversationChannel[] - The channels covered by this Opt-Out. The default are all the channels for a contact if recipient is contact_id or the channels in the channel_identities list.
- recipient Recipient? - Recipient information
sinch.conversation: OptOutResponse
The response received for an Opt-Out request.
Fields
- opt_out OptOut? - An Opt-Out contains the identity of the recipient which retract its consent to receive messages from Conversation API apps on a given channel.
- request_id string? - ID for the asynchronous request, will be generated id if not set in request
sinch.conversation: ProtobufAny
Fields
- type_url string? -
- value string? -
sinch.conversation: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
sinch.conversation: QueryCapability
Fields
- app_id string? - Required. The ID of the app to use for capability lookup.
- project_id string? - Required. The project ID.
- recipient Recipient? - Recipient information
- request_id string? -
sinch.conversation: QueryCapabilityResponse
An CapabilityResponse contains the identity of the recipient for which will be perform a capability lookup.
Fields
- app_id string? - Required. The ID of the app to use for capability lookup.
- recipient Recipient? - Recipient information
- request_id string? - ID for the asynchronous request, will be generated id if not set in request
sinch.conversation: RateLimits
Rate limits
Fields
- inbound int? - Output only. The number of inbound messages/events we process per second, from underlying channels to the app. The default rate limit is 25.
- outbound int? - Output only. The number of messages/events we process per second, from the app to the underlying channels. Note that underlying channels may have other rate limits. The default rate limit is 25.
- webhooks int? - Output only. The rate limit of callbacks sent to the webhooks registered for the app. Note that if you have multiple webhooks with shared triggers, multiple callbacks will be sent out for each triggering event. The default rate limit is 25.
sinch.conversation: Reason
Reason
Fields
- code ReasonCode? -
- UNKNOWN: UNKNOWN is used if no other code can be used to describe the encountered error.
- INTERNAL_ERROR: An internal error occurred. Please save the entire callback if you want to report an error.
- RATE_LIMITED: The message or event was not sent due to rate limiting.
- RECIPIENT_INVALID_CHANNEL_IDENTITY: The channel recipient identity was malformed.
- RECIPIENT_NOT_REACHABLE: It was not possible to reach the contact, or channel recipient identity, on the channel.
- RECIPIENT_NOT_OPTED_IN: The contact, or channel recipient identity, has not opt-ed in on the channel.
- OUTSIDE_ALLOWED_SENDING_WINDOW: The allowed sending window has expired. See the channel documentation for more information about how the sending window works for the different channels.
- CHANNEL_FAILURE: The channel failed to accept the message. The Conversation API performs multiple retries in case of transient errors
- CHANNEL_BAD_CONFIGURATION: The configuration of the channel for the used App is wrong. The bad configuration caused the channel to reject the message. Please see the channel support documentation page for how to set it up correctly.
- CHANNEL_CONFIGURATION_MISSING: The configuration of the channel is missing from the used App. Please see the channel support documentation page for how to set it up correctly.
- MEDIA_TYPE_UNSUPPORTED: Some of the referenced media files is of a unsupported media type. Please read the channel support documentation page to find out the limitations on media that the different channels impose.
- MEDIA_TOO_LARGE: Some of the referenced media files are too large. Please read the channel support documentation to find out the limitations on file size that the different channels impose.
- MEDIA_NOT_REACHABLE: The provided media link was not accessible from the Conversation API or from the underlying channels. Please make sure that the media file is accessible.
- NO_CHANNELS_LEFT: No channels to try to send the message to. This error will occur if one attempts to send a message to a channel with no channel identities or if all applicable channels have been attempted.
- TEMPLATE_NOT_FOUND: The referenced template was not found.
- TEMPLATE_INSUFFICIENT_PARAMETERS: Sufficient template parameters was not given. All parameters defined in the template must be provided when sending a template message
- TEMPLATE_NON_EXISTING_LANGUAGE_OR_VERSION: The selected language, or version, of the referenced template did not exist. Please check the available versions and languages of the template
- DELIVERY_TIMED_OUT: The message delivery, or event delivery, failed due to a channel-imposed timeout. See the channel support documentation page for further details about how the different channels behave.
- DELIVERY_REJECTED_DUE_TO_POLICY: The message or event was rejected by the channel due to a policy. Some channels have specific policies that must be met to send a message. See the channel support documentation page for more information about when this error will be triggered.
- CONTACT_NOT_FOUND: The provided Contact ID did not exist.
- BAD_REQUEST: Conversation API validates send requests in two different stages. The first stage is right before the message is enqueued. If this first validation fails the API responds with 400 Bad Request and the request is discarded immediately. The second validation kicks in during message processing and it normally contains channel specific validation rules. Failures during second request validation are delivered as callbacks to MESSAGE_DELIVERY (EVENT_DELIVERY) webhooks with ReasonCode BAD_REQUEST.
- UNKNOWN_APP: The used App is missing. This error may occur when the app is removed during message processing.
- NO_CHANNEL_IDENTITY_FOR_CONTACT: The contact has no channel identities setup, or the contact has no channels setup for the resolved channel priorities.
- CHANNEL_REJECT: Generic error for channel permanently rejecting a message. Only used if no other better matching error can be used
- NO_PERMISSION: No permission to perform action
- NO_PROFILE_AVAILABLE: No available profile data for user
- UNSUPPORTED_OPERATION: Generic error for channel unsupported operations.
- description string? - A textual description of the reason.
- sub_code ReasonSubCode? -
- UNSPECIFIED_SUB_CODE: UNSPECIFIED_SUB_CODE is used if no other sub code can be used to describe the encountered error.
- ATTACHMENT_REJECTED: The message attachment was rejected by the channel due to a policy. Some channels have specific policies that must be met to receive an attachment.
- MEDIA_TYPE_UNDETERMINED: The specified media urls media type could not be determined
- INACTIVE_SENDER: The used credentials for the underlying channel is inactivated and not allowed to send or receive messages.
sinch.conversation: Recipient
Recipient information
Fields
- contact_id string - The ID of the receiving contact.
- identified_by ChannelIdentities - Channel identities.
sinch.conversation: ReplyTo
If the contact message was a response to a previous App message then this field contains information about that.
Fields
- message_id string? - Required. The Id of the message that this is a response to
sinch.conversation: RetentionPolicy
Retention policy for messages and conversations
Fields
- retention_type RetentionPolicyType? -
- MESSAGE_EXPIRE_POLICY: The default retention policy where messages older than ttl_days are automatically deleted from Conversation API database.
- CONVERSATION_EXPIRE_POLICY: The conversation expire policy only considers the last message in a conversation. If the last message is older that ttl_days the entire conversation is deleted. The difference with MESSAGE_EXPIRE_POLICY is that messages with accept_time older than ttl_days are persisted as long as there is a newer message in the same conversation.
- PERSIST_RETENTION_POLICY: Persist policy does not delete old messages or conversations. Please note that message storage might be subject to additional charges in the future.
- ttl_days int? - Optional. The days before a message or conversation is eligible for deletion. Default value is 180. The ttl_days value has no effect when retention_type is PERSIST_RETENTION_POLICY. The valid values for this field are [1 - 3650]. Note that retention cleanup job runs once every twenty-four hours which can lead to delay i.e., messages and conversations are not deleted on the minute they become eligible for deletion.
sinch.conversation: RuntimeError
Fields
- code int? -
- details ProtobufAny[]? -
- 'error string? -
- message string? -
- status string? -
sinch.conversation: SendEventRequest
Fields
- app_id string? - Required. The ID of the app sending the event.
- callback_url string? - Overwrites the default callback url for delivery reports for this message The REST URL should be of the form: http://host[:port]/path
- channel_priority_order ConversationChannel[]? - Optional. Channel priority order that dictates on which channels the Conversation API should try to send the message on. The order provided here overrides any default.
- event AppEvent? -
- event_metadata string? - Optional. Eventual metadata that should be associated to the event.
- project_id string? - Required. The project ID.
- queue MessageQueue? - Message queue priority.
- recipient Recipient? - Recipient information
sinch.conversation: SendEventResponse
Fields
- accepted_time string? - Output only. Accepted timestamp.
- event_id string? - Output only. Event id.
sinch.conversation: SendMessageRequest
Send message request.
Fields
- app_id string - The ID of the app sending the message.
- callback_url string? - Overwrites the default callback url for delivery reports for this message The REST URL should be of the form: http://host[:port]/path
- channel_priority_order ConversationChannel[]? - Explicitly define the channels and order in which they are tried when sending the message. Note that collection can't contain 'CHANNEL_UNSPECIFIED' value. Which channels the API will try and their priority is defined by: 1. channel_priority_order if available. 2. recipient.identified_by.channel_identities if available. 3. When recipient is a contact_id:
- if a conversation with the contact exists: the active channel of the conversation is tried first.
- the existing channels for the contact are ordered by contact channel preferences if given.
- lastly the existing channels for the contact are ordered by the app priority.
- channel_properties record {}? - Channel-specific properties. The key in the map must point to a valid channel property key as defined by the enum ChannelPropertyKeys. The maximum allowed property value length is 1024 characters.
- message AppMessage - Message originating from an app
- message_metadata string? - Metadata that should be associated with the message.
- project_id string - The project ID.
- queue MessageQueue? - Message queue priority.
- recipient Recipient - Recipient information
- ttl string? - The timeout allotted for sending the message. Passed onto channels which have support for it and emulated by Conversation API for channels without ttl support but with message retract/unsend functionality. Channel failover will not be performed for messages with expired TTL.
sinch.conversation: SendMessageResponse
Fields
- accepted_time string? - Timestamp when the Conversation API accepted the message for delivery to the referenced contact.
- message_id string? - The ID of the message.
sinch.conversation: StaticBearerCredential
It consists of claimed identity and a static token.
Fields
- claimed_identity string? - Required. Claimed identity.
- token string? - Required. Static bearer token.
sinch.conversation: StaticTokenCredential
Static Token Credential
Fields
- token string? - Required. The static token.
sinch.conversation: TemplateMessage
Message referring to predefined template
Fields
- channel_template record {}? - Optional. Channel specific template reference with parameters per channel. The channel template if exists overrides the omnichannel template. At least one of channel_template or omni_template needs to be present. The key in the map must point to a valid conversation channel as defined by the enum ConversationChannel.
- omni_template TemplateReference? - The referenced template can be an omnichannel template stored in Conversation API Template Store as AppMessage or it can reference external channel-specific template such as WhatsApp Business Template.
sinch.conversation: TemplateReference
The referenced template can be an omnichannel template stored in Conversation API Template Store as AppMessage or it can reference external channel-specific template such as WhatsApp Business Template.
Fields
- language_code string? - Optional. The BCP-47 language code, such as "en-US" or "sr-Latn". For more information, see http://www.unicode.org/reports/tr35/#Unicode_locale_identifier. English is the default language_code.
- parameters record {}? - Optional. Required if the template has parameters. Concrete values must be present for all defined parameters in the template. Parameters can be different for different versions and/or languages of the template.
- template_id string? - Required. The ID of the template.
- 'version string? - Required. Used to specify what version of a template to use. This will be used in conjunction with language_code.
sinch.conversation: TextMessage
Message containing only text
Fields
- text string? - Required. The text to be sent.
sinch.conversation: TranscodeMessageRequest
Fields
- app_id string? - Required. The ID of the app used to transcode the message.
- app_message AppMessage? - Message originating from an app
- channels ConversationChannel[]? - Required. The list of channels for which the message shall be transcoded to.
- 'from string? - Optional.
- project_id string? - Required. The project ID.
- to string? - Optional.
sinch.conversation: TranscodeMessageResponse
Fields
- transcoded_message record {}? - Output only. The transcoded message for the different channels. The keys in the map correspond to channel names, as defined by the type ConversationChannel.
sinch.conversation: UrlMessage
A generic URL message.
Fields
- title string? - Required. The title shown close to the URL. The title will be clickable in some cases.
- url string? - Required. The url to show.
sinch.conversation: Webhook
Represents a destination for receiving callbacks from the Conversation API.
Fields
- app_id string - The app that this webhook belongs to.
- client_credentials ClientCredentials? - Client credentials
- id string? - Output only. The ID of the webhook.
- secret string? - Optional secret be used to sign contents of webhooks sent by the Conversation API. You can then use the secret to verify the signature.
- target string - The target url where events should be sent to. Maximum URL length is 742.
- target_type WebhookTargetType? - Webhook target-type
- triggers WebhookTrigger[] - An array of triggers that should trigger the webhook and result in a event being sent to the target url. -
UNSPECIFIED_TRIGGER
: Using this value will cause errors. -MESSAGE_DELIVERY
: Subscribe to delivery receipts for a message sent. -EVENT_DELIVERY
: Subscribe to delivery receipts for a event sent. -MESSAGE_INBOUND
: Subscribe to inbound messages from end users on the underlying channels. -EVENT_INBOUND
: Subscribe to inbound events from end users on the underlying channels. -CONVERSATION_START
: Subscribe to an event that is triggered when a new conversation has been started. -CONVERSATION_STOP
: Subscribe to an event that is triggered when a active conversation has been stopped. -CONTACT_CREATE
: Subscribe to an event that is triggered when a new contact has been created. -CONTACT_DELETE
: Subscribe to an event that is triggered when a contact has been deleted. -CONTACT_MERGE
: Subscribe to an event that is triggered when a two contacts are merged. -UNSUPPORTED
: Subscribe to callbacks that are not natively supported by the Conversation API. -OPT_IN
: Subscribe to opt_ins. -OPT_OUT
: Subscribe to opt_outs. -CAPABILITY
: Subscribe to see get capability results. -CONVERSATION_DELETE
: Subscribe to get an event when a conversation is deleted.
String types
sinch.conversation: CardHeight
CardHeight
Card height
sinch.conversation: ContactLanguage
ContactLanguage
Contact language
sinch.conversation: ConversationChannel
ConversationChannel
Channel Identifier
sinch.conversation: ConversationDirection
ConversationDirection
Conversation direction
sinch.conversation: ConversationMergeStrategy
ConversationMergeStrategy
sinch.conversation: ConversationMessagesView
ConversationMessagesView
Conversation message view.
sinch.conversation: ConversationMetadataReportView
ConversationMetadataReportView
Conversation metadata report view.
sinch.conversation: MessageQueue
MessageQueue
Message queue priority.
sinch.conversation: ReasonCode
ReasonCode
- UNKNOWN: UNKNOWN is used if no other code can be used to describe the encountered error.
- INTERNAL_ERROR: An internal error occurred. Please save the entire callback if you want to report an error.
- RATE_LIMITED: The message or event was not sent due to rate limiting.
- RECIPIENT_INVALID_CHANNEL_IDENTITY: The channel recipient identity was malformed.
- RECIPIENT_NOT_REACHABLE: It was not possible to reach the contact, or channel recipient identity, on the channel.
- RECIPIENT_NOT_OPTED_IN: The contact, or channel recipient identity, has not opt-ed in on the channel.
- OUTSIDE_ALLOWED_SENDING_WINDOW: The allowed sending window has expired. See the channel documentation for more information about how the sending window works for the different channels.
- CHANNEL_FAILURE: The channel failed to accept the message. The Conversation API performs multiple retries in case of transient errors
- CHANNEL_BAD_CONFIGURATION: The configuration of the channel for the used App is wrong. The bad configuration caused the channel to reject the message. Please see the channel support documentation page for how to set it up correctly.
- CHANNEL_CONFIGURATION_MISSING: The configuration of the channel is missing from the used App. Please see the channel support documentation page for how to set it up correctly.
- MEDIA_TYPE_UNSUPPORTED: Some of the referenced media files is of a unsupported media type. Please read the channel support documentation page to find out the limitations on media that the different channels impose.
- MEDIA_TOO_LARGE: Some of the referenced media files are too large. Please read the channel support documentation to find out the limitations on file size that the different channels impose.
- MEDIA_NOT_REACHABLE: The provided media link was not accessible from the Conversation API or from the underlying channels. Please make sure that the media file is accessible.
- NO_CHANNELS_LEFT: No channels to try to send the message to. This error will occur if one attempts to send a message to a channel with no channel identities or if all applicable channels have been attempted.
- TEMPLATE_NOT_FOUND: The referenced template was not found.
- TEMPLATE_INSUFFICIENT_PARAMETERS: Sufficient template parameters was not given. All parameters defined in the template must be provided when sending a template message
- TEMPLATE_NON_EXISTING_LANGUAGE_OR_VERSION: The selected language, or version, of the referenced template did not exist. Please check the available versions and languages of the template
- DELIVERY_TIMED_OUT: The message delivery, or event delivery, failed due to a channel-imposed timeout. See the channel support documentation page for further details about how the different channels behave.
- DELIVERY_REJECTED_DUE_TO_POLICY: The message or event was rejected by the channel due to a policy. Some channels have specific policies that must be met to send a message. See the channel support documentation page for more information about when this error will be triggered.
- CONTACT_NOT_FOUND: The provided Contact ID did not exist.
- BAD_REQUEST: Conversation API validates send requests in two different stages. The first stage is right before the message is enqueued. If this first validation fails the API responds with 400 Bad Request and the request is discarded immediately. The second validation kicks in during message processing and it normally contains channel specific validation rules. Failures during second request validation are delivered as callbacks to MESSAGE_DELIVERY (EVENT_DELIVERY) webhooks with ReasonCode BAD_REQUEST.
- UNKNOWN_APP: The used App is missing. This error may occur when the app is removed during message processing.
- NO_CHANNEL_IDENTITY_FOR_CONTACT: The contact has no channel identities setup, or the contact has no channels setup for the resolved channel priorities.
- CHANNEL_REJECT: Generic error for channel permanently rejecting a message. Only used if no other better matching error can be used
- NO_PERMISSION: No permission to perform action
- NO_PROFILE_AVAILABLE: No available profile data for user
- UNSUPPORTED_OPERATION: Generic error for channel unsupported operations.
sinch.conversation: ReasonSubCode
ReasonSubCode
- UNSPECIFIED_SUB_CODE: UNSPECIFIED_SUB_CODE is used if no other sub code can be used to describe the encountered error.
- ATTACHMENT_REJECTED: The message attachment was rejected by the channel due to a policy. Some channels have specific policies that must be met to receive an attachment.
- MEDIA_TYPE_UNDETERMINED: The specified media urls media type could not be determined
- INACTIVE_SENDER: The used credentials for the underlying channel is inactivated and not allowed to send or receive messages.
sinch.conversation: RetentionPolicyType
RetentionPolicyType
- MESSAGE_EXPIRE_POLICY: The default retention policy where messages older than ttl_days are automatically deleted from Conversation API database.
- CONVERSATION_EXPIRE_POLICY: The conversation expire policy only considers the last message in a conversation. If the last message is older that ttl_days the entire conversation is deleted. The difference with MESSAGE_EXPIRE_POLICY is that messages with accept_time older than ttl_days are persisted as long as there is a newer message in the same conversation.
- PERSIST_RETENTION_POLICY: Persist policy does not delete old messages or conversations. Please note that message storage might be subject to additional charges in the future.
sinch.conversation: WebhookTargetType
WebhookTargetType
Webhook target-type
sinch.conversation: WebhookTrigger
WebhookTrigger
UNSPECIFIED_TRIGGER
: Using this value will cause errors. -MESSAGE_DELIVERY
: Subscribe to delivery receipts for a message sent. -EVENT_DELIVERY
: Subscribe to delivery receipts for a event sent. -MESSAGE_INBOUND
: Subscribe to inbound messages from end users on the underlying channels. -EVENT_INBOUND
: Subscribe to inbound events from end users on the underlying channels. -CONVERSATION_START
: Subscribe to an event that is triggered when a new conversation has been started. -CONVERSATION_STOP
: Subscribe to an event that is triggered when a active conversation has been stopped. -CONTACT_CREATE
: Subscribe to an event that is triggered when a new contact has been created. -CONTACT_DELETE
: Subscribe to an event that is triggered when a contact has been deleted. -CONTACT_MERGE
: Subscribe to an event that is triggered when a two contacts are merged. -UNSUPPORTED
: Subscribe to callbacks that are not natively supported by the Conversation API. -OPT_IN
: Subscribe to opt_ins. -OPT_OUT
: Subscribe to opt_outs. -CAPABILITY
: Subscribe to see get capability results. -CONVERSATION_DELETE
: Subscribe to get an event when a conversation is deleted.
Import
import ballerinax/sinch.conversation;
Metadata
Released date: about 1 year ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 0
Current verison: 0
Weekly downloads
Keywords
Communication/Call & SMS
Cost/Paid
Contributors
Dependencies