shortcut
Module shortcut
API
Definitions
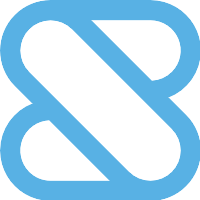
ballerinax/shortcut Ballerina library
Overview
This is a generated connector for Shortcut API v3.0 OpenAPI specification. The Shortcut API bring the flow to your software team's workflow. Plan, collaborate, build, and measure success with Shortcut. The Shortcut API provides a greater amount of control over your organization’s Shortcut data than what is possible using the Shortcut web app. You can use this API to build custom integrations or automate even more of your organization’s workflow.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Shortcut account
- Obtain tokens by following this guide
Quickstart
To use the Shortcut connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/shortcut
module into the Ballerina project.
import ballerinax/shortcut;
Step 2: Create a new connector instance
Create a shortcut:ApiKeysConfig
with the API key obtained, and initialize the connector with it.
shortcut:ApiKeysConfig config = { shortcutToken: "<API_KEY>" } shortcut:Client baseClient = check new Client(config);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to retrieve a list of categories using the connector.
List Categories
public function main() returns error? { shortcut:Category[] response = check baseClient->listCategories(); log:printInfo(response.toString()); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
shortcut: Client
This is a generated connector for Shortcut API v3.0 OpenAPI specification. The Shortcut API bring the flow to your software team's workflow. Plan, collaborate, build, and measure success with Shortcut. The Shortcut API provides a greater amount of control over your organization’s Shortcut data than what is possible using the Shortcut web app. You can use this API to build custom integrations or automate even more of your organization’s workflow.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Shortcut account and obtain tokens by following this guide.
init (ApiKeysConfig apiKeyConfig, ConnectionConfig config, string serviceUrl)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.app.shortcut.com/" - URL of the target service
listCategories
List Categories
createCategory
function createCategory(CreateCategory payload) returns Category|error
Create Category
Parameters
- payload CreateCategory - Create category data
getCategory
Get Category
Parameters
- categoryPublicId int - The unique ID of the Category.
updateCategory
function updateCategory(int categoryPublicId, UpdateCategory payload) returns Category|error
Update Category
Parameters
- categoryPublicId int - The unique ID of the Category you wish to update.
- payload UpdateCategory - Update category data
deleteCategory
Delete Category
Parameters
- categoryPublicId int - The unique ID of the Category.
listCategoryMilestones
List Category Milestones
Parameters
- categoryPublicId int - The unique ID of the Category.
listEntityTemplates
function listEntityTemplates() returns EntityTemplate[]|error
List Entity Templates
Return Type
- EntityTemplate[]|error - Resource
createEntityTemplate
function createEntityTemplate(CreateEntityTemplate payload) returns EntityTemplate|error
Create Entity Template
Parameters
- payload CreateEntityTemplate - Request paramaters for creating an entirely new entity template.
Return Type
- EntityTemplate|error - Resource
disableStoryTemplates
Disable Story Templates
enableStoryTemplates
Enable Story Templates
getEntityTemplate
function getEntityTemplate(string entityTemplatePublicId) returns EntityTemplate|error
Get Entity Template
Parameters
- entityTemplatePublicId string - The unique ID of the entity template.
Return Type
- EntityTemplate|error - Resource
updateEntityTemplate
function updateEntityTemplate(string entityTemplatePublicId, UpdateEntityTemplate payload) returns EntityTemplate|error
Update Entity Template
Parameters
- entityTemplatePublicId string - The unique ID of the template to be updated.
- payload UpdateEntityTemplate - Request parameters for changing either a template's name or any of
Return Type
- EntityTemplate|error - Resource
deleteEntityTemplate
Delete Entity Template
Parameters
- entityTemplatePublicId string - The unique ID of the entity template.
getEpicWorkflow
function getEpicWorkflow() returns EpicWorkflow|error
Get Epic Workflow
Return Type
- EpicWorkflow|error - Resource
listEpics
List Epics
createEpic
function createEpic(CreateEpic payload) returns Epic|error
Create Epic
Parameters
- payload CreateEpic - Create epic data
getEpic
Get Epic
Parameters
- epicPublicId int - The unique ID of the Epic.
updateEpic
function updateEpic(int epicPublicId, UpdateEpic payload) returns Epic|error
Update Epic
deleteEpic
Delete Epic
Parameters
- epicPublicId int - The unique ID of the Epic.
listEpicComments
function listEpicComments(int epicPublicId) returns ThreadedComment[]|error
List Epic Comments
Parameters
- epicPublicId int - The unique ID of the Epic.
Return Type
- ThreadedComment[]|error - Resource
createEpicComment
function createEpicComment(int epicPublicId, CreateEpicComment payload) returns ThreadedComment|error
Create Epic Comment
Parameters
- epicPublicId int - The ID of the associated Epic.
- payload CreateEpicComment - Create epic comment data
Return Type
- ThreadedComment|error - Resource
getEpicComment
function getEpicComment(int epicPublicId, int commentPublicId) returns ThreadedComment|error
Get Epic Comment
Parameters
- epicPublicId int - The ID of the associated Epic.
- commentPublicId int - The ID of the Comment.
Return Type
- ThreadedComment|error - Resource
updateEpicComment
function updateEpicComment(int epicPublicId, int commentPublicId, UpdateComment payload) returns ThreadedComment|error
Update Epic Comment
Parameters
- epicPublicId int - The ID of the associated Epic.
- commentPublicId int - The ID of the Comment.
- payload UpdateComment - Update epic comment data
Return Type
- ThreadedComment|error - Resource
createEpicCommentComment
function createEpicCommentComment(int epicPublicId, int commentPublicId, CreateCommentComment payload) returns ThreadedComment|error
Create Epic Comment Comment
Parameters
- epicPublicId int - The ID of the associated Epic.
- commentPublicId int - The ID of the parent Epic Comment.
- payload CreateCommentComment - Create epic comment comment data
Return Type
- ThreadedComment|error - Resource
deleteEpicComment
Delete Epic Comment
Parameters
- epicPublicId int - The ID of the associated Epic.
- commentPublicId int - The ID of the Comment.
listEpicStories
List Epic Stories
Parameters
- epicPublicId int - The unique ID of the Epic.
unlinkProductboardFromEpic
Unlink Productboard from Epic
Parameters
- epicPublicId int - The unique ID of the Epic.
getExternalLinkStories
Get External Link Stories
listFiles
function listFiles() returns UploadedFile[]|error
List Files
Return Type
- UploadedFile[]|error - Resource
getFile
function getFile(int filePublicId) returns UploadedFile|error
Get File
Parameters
- filePublicId int - The File’s unique ID.
Return Type
- UploadedFile|error - Resource
updateFile
function updateFile(int filePublicId, UpdateFile payload) returns UploadedFile|error
Update File
Parameters
- filePublicId int - The unique ID assigned to the file in Shortcut.
- payload UpdateFile - Update file data
Return Type
- UploadedFile|error - Resource
deleteFile
Delete File
Parameters
- filePublicId int - The File’s unique ID.
listGroups
List Groups
createGroup
function createGroup(CreateGroup payload) returns Group|error
Create Group
Parameters
- payload CreateGroup - Create group data
disableGroups
Disable Groups
enableGroups
Enable Groups
getGroup
Get Group
Parameters
- groupPublicId string - The unique ID of the Group.
updateGroup
function updateGroup(string groupPublicId, UpdateGroup payload) returns Group|error
Update Group
listGroupStories
List Group Stories
Parameters
- groupPublicId string - The unique ID of the Group.
listIterations
function listIterations() returns IterationSlim[]|error
List Iterations
Return Type
- IterationSlim[]|error - Resource
createIteration
function createIteration(CreateIteration payload) returns Iteration|error
Create Iteration
Parameters
- payload CreateIteration - Create iteration data
disableIterations
Disable Iterations
enableIterations
Enable Iterations
getIteration
Get Iteration
Parameters
- iterationPublicId int - The unique ID of the Iteration.
updateIteration
function updateIteration(int iterationPublicId, UpdateIteration payload) returns Iteration|error
Update Iteration
Parameters
- iterationPublicId int - The unique ID of the Iteration.
- payload UpdateIteration - Update iteration data
deleteIteration
Delete Iteration
Parameters
- iterationPublicId int - The unique ID of the Iteration.
listIterationStories
List Iteration Stories
Parameters
- iterationPublicId int - The unique ID of the Iteration.
listLabels
List Labels
createLabel
function createLabel(CreateLabelParams payload) returns Label|error
Create Label
Parameters
- payload CreateLabelParams - Request parameters for creating a Label on a Shortcut Story.
getLabel
Get Label
Parameters
- labelPublicId int - The unique ID of the Label.
updateLabel
function updateLabel(int labelPublicId, UpdateLabel payload) returns Label|error
Update Label
Parameters
- labelPublicId int - The unique ID of the Label you wish to update.
- payload UpdateLabel - Update label data
deleteLabel
Delete Label
Parameters
- labelPublicId int - The unique ID of the Label.
listLabelEpics
List Label Epics
Parameters
- labelPublicId int - The unique ID of the Label.
listLabelStories
List Label Stories
Parameters
- labelPublicId int - The unique ID of the Label.
listLinkedFiles
function listLinkedFiles() returns LinkedFile[]|error
List Linked Files
Return Type
- LinkedFile[]|error - Resource
createLinkedFile
function createLinkedFile(CreateLinkedFile payload) returns LinkedFile|error
Create Linked File
Parameters
- payload CreateLinkedFile - Create linked file data
Return Type
- LinkedFile|error - Resource
getLinkedFile
function getLinkedFile(int linkedFilePublicId) returns LinkedFile|error
Get Linked File
Parameters
- linkedFilePublicId int - The unique identifier of the linked file.
Return Type
- LinkedFile|error - Resource
updateLinkedFile
function updateLinkedFile(int linkedFilePublicId, UpdateLinkedFile payload) returns LinkedFile|error
Update Linked File
Parameters
- linkedFilePublicId int - The unique identifier of the linked file.
- payload UpdateLinkedFile - Update linked file data
Return Type
- LinkedFile|error - Resource
deleteLinkedFile
Delete Linked File
Parameters
- linkedFilePublicId int - The unique identifier of the linked file.
getCurrentMemberInfo
function getCurrentMemberInfo() returns MemberInfo|error
Get Current Member Info
Return Type
- MemberInfo|error - Resource
listMembers
List Members
getMember
Get Member
Parameters
- memberPublicId string - The Member's unique ID.
listMilestones
List Milestones
createMilestone
function createMilestone(CreateMilestone payload) returns Milestone|error
Create Milestone
Parameters
- payload CreateMilestone - Create milestone data
getMilestone
Get Milestone
Parameters
- milestonePublicId int - The ID of the Milestone.
updateMilestone
function updateMilestone(int milestonePublicId, UpdateMilestone payload) returns Milestone|error
Update Milestone
Parameters
- milestonePublicId int - The ID of the Milestone.
- payload UpdateMilestone - Update milestone data
deleteMilestone
Delete Milestone
Parameters
- milestonePublicId int - The ID of the Milestone.
listMilestoneEpics
List Milestone Epics
Parameters
- milestonePublicId int - The ID of the Milestone.
listProjects
List Projects
createProject
function createProject(CreateProject payload) returns Project|error
Create Project
Parameters
- payload CreateProject - Create project data
getProject
Get Project
Parameters
- projectPublicId int - The unique ID of the Project.
updateProject
function updateProject(int projectPublicId, UpdateProject payload) returns Project|error
Update Project
Parameters
- projectPublicId int - The unique ID of the Project.
- payload UpdateProject - Update project data
deleteProject
Delete Project
Parameters
- projectPublicId int - The unique ID of the Project.
listStories
List Stories
Parameters
- projectPublicId int - The unique ID of the Project.
listRepositories
function listRepositories() returns Repository[]|error
List Repositories
Return Type
- Repository[]|error - Resource
getRepository
function getRepository(int repoPublicId) returns Repository|error
Get Repository
Parameters
- repoPublicId int - The unique ID of the Repository.
Return Type
- Repository|error - Resource
search
function search() returns SearchResults|error
Search
Return Type
- SearchResults|error - Resource
searchEpics
function searchEpics() returns EpicSearchResults|error
Search Epics
Return Type
- EpicSearchResults|error - Resource
searchStories
function searchStories() returns StorySearchResults|error
Search Stories
Return Type
- StorySearchResults|error - Resource
createStory
function createStory(CreateStoryParams payload) returns Story|error
Create Story
Parameters
- payload CreateStoryParams - Request parameters for creating a story.
updateMultipleStories
function updateMultipleStories(UpdateStories payload) returns StorySlim[]|error
Update Multiple Stories
Parameters
- payload UpdateStories - Update multiple stories data
createMultipleStories
function createMultipleStories(CreateStories payload) returns StorySlim[]|error
Create Multiple Stories
Parameters
- payload CreateStories - Create multiple stories
deleteMultipleStories
Delete Multiple Stories
searchStoriesOld
function searchStoriesOld(SearchStories payload) returns StorySlim[]|error
Search Stories (Old)
Parameters
- payload SearchStories - Search stories (old) data
getStory
Get Story
Parameters
- storyPublicId int - The ID of the Story.
updateStory
function updateStory(int storyPublicId, UpdateStory payload) returns Story|error
Update Story
Parameters
- storyPublicId int - The unique identifier of this story.
- payload UpdateStory - Update story data
deleteStory
Delete Story
Parameters
- storyPublicId int - The ID of the Story.
createStoryComment
function createStoryComment(int storyPublicId, CreateStoryComment payload) returns StoryComment|error
Create Story Comment
Parameters
- storyPublicId int - The ID of the Story that the Comment is in.
- payload CreateStoryComment - Create story comment data
Return Type
- StoryComment|error - Resource
getStoryComment
function getStoryComment(int storyPublicId, int commentPublicId) returns StoryComment|error
Get Story Comment
Parameters
- storyPublicId int - The ID of the Story that the Comment is in.
- commentPublicId int - The ID of the Comment.
Return Type
- StoryComment|error - Resource
updateStoryComment
function updateStoryComment(int storyPublicId, int commentPublicId, UpdateStoryComment payload) returns StoryComment|error
Update Story Comment
Parameters
- storyPublicId int - The ID of the Story that the Comment is in.
- commentPublicId int - The ID of the Comment
- payload UpdateStoryComment - Update story comment data
Return Type
- StoryComment|error - Resource
deleteStoryComment
Delete Story Comment
Parameters
- storyPublicId int - The ID of the Story that the Comment is in.
- commentPublicId int - The ID of the Comment.
createStoryReaction
function createStoryReaction(int storyPublicId, int commentPublicId, CreateOrDeleteStoryReaction payload) returns StoryReaction[]|error
Create Story Reaction
Parameters
- storyPublicId int - The ID of the Story that the Comment is in.
- commentPublicId int - The ID of the Comment.
- payload CreateOrDeleteStoryReaction - Create story reaction data
Return Type
- StoryReaction[]|error - Resource
deleteStoryReaction
Delete Story Reaction
Parameters
- storyPublicId int - The ID of the Story that the Comment is in.
- commentPublicId int - The ID of the Comment.
storyHistory
Story History
Parameters
- storyPublicId int - The ID of the Story.
createTask
function createTask(int storyPublicId, CreateTask payload) returns Task|error
Create Task
Parameters
- storyPublicId int - The ID of the Story that the Task will be in.
- payload CreateTask - Create task data
getTask
Get Task
Parameters
- storyPublicId int - The unique ID of the Story this Task is associated with.
- taskPublicId int - The unique ID of the Task.
updateTask
function updateTask(int storyPublicId, int taskPublicId, UpdateTask payload) returns Task|error
Update Task
Parameters
- storyPublicId int - The unique identifier of the parent Story.
- taskPublicId int - The unique identifier of the Task you wish to update.
- payload UpdateTask - Update task data
deleteTask
Delete Task
Parameters
- storyPublicId int - The unique ID of the Story this Task is associated with.
- taskPublicId int - The unique ID of the Task.
createStoryLink
function createStoryLink(CreateStoryLink payload) returns StoryLink|error
Create Story Link
Parameters
- payload CreateStoryLink - Create story link data
getStoryLink
Get Story Link
Parameters
- storyLinkPublicId int - The unique ID of the Story Link.
updateStoryLink
function updateStoryLink(int storyLinkPublicId, UpdateStoryLink payload) returns StoryLink|error
Update Story Link
Parameters
- storyLinkPublicId int - The unique ID of the Story Link.
- payload UpdateStoryLink - Update story link data
deleteStoryLink
Delete Story Link
Parameters
- storyLinkPublicId int - The unique ID of the Story Link.
listWorkflows
List Workflows
getWorkflow
Get Workflow
Parameters
- workflowPublicId int - The ID of the Workflow.
Records
shortcut: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- shortcutToken string - Represents API Key
Shortcut-Token
shortcut: BasicWorkspaceInfo
Fields
- url_slug string -
- estimate_scale int[] -
shortcut: Branch
Branch refers to a VCS branch. Branches are feature branches associated with Shortcut Stories.
Fields
- entity_type string - A string description of this resource.
- deleted boolean - A true/false boolean indicating if the Branch has been deleted.
- name string - The name of the Branch.
- persistent boolean - A true/false boolean indicating if the Branch is persistent; e.g. master.
- updated_at string - The time/date the Branch was updated.
- pull_requests PullRequest[] - An array of PullRequests attached to the Branch (there is usually only one).
- merged_branch_ids int[] - The IDs of the Branches the Branch has been merged into.
- id int - The unique ID of the Branch.
- url string - The URL of the Branch.
- repository_id int - The ID of the Repository that contains the Branch.
- created_at string - The time/date the Branch was created.
shortcut: Category
A Category can be used to associate Milestones.
Fields
- archived boolean - A true/false boolean indicating if the Category has been archived.
- entity_type string - A string description of this resource.
- color string - The hex color to be displayed with the Category (for example, "#ff0000").
- name string - The name of the Category.
- 'type string - The type of entity this Category is associated with; currently Milestone is the only type of Category.
- updated_at string - The time/date that the Category was updated.
- external_id string - This field can be set to another unique ID. In the case that the Category has been imported from another tool, the ID in the other tool can be indicated here.
- id int - The unique ID of the Category.
- created_at string - The time/date that the Category was created.
shortcut: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
shortcut: Commit
Commit refers to a VCS commit and all associated details.
Fields
- entity_type string - A string description of this resource.
- author_id string - The ID of the Member that authored the Commit, if known.
- hash string - The Commit hash.
- updated_at string - The time/date the Commit was updated.
- merged_branch_ids int[] - The IDs of the Branches the Commit has been merged into.
- id int - The unique ID of the Commit.
- url string - The URL of the Commit.
- author_email string - The email address of the VCS user that authored the Commit.
- timestamp string - The time/date the Commit was pushed.
- author_identity Identity - The Identity of the VCS user that authored the Commit.
- repository_id int - The ID of the Repository that contains the Commit.
- created_at string - The time/date the Commit was created.
- message string - The Commit message.
shortcut: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
shortcut: CreateCategory
Fields
- name string - The name of the new Category.
- color string? - The hex color to be displayed with the Category (for example, "#ff0000").
- external_id string? - This field can be set to another unique ID. In the case that the Category has been imported from another tool, the ID in the other tool can be indicated here.
- 'type string - The type of entity this Category is associated with; currently Milestone is the only type of Category.
shortcut: CreateCategoryParams
Request parameters for creating a Category with a Milestone.
Fields
- name string - The name of the new Category.
- color string? - The hex color to be displayed with the Category (for example, "#ff0000").
- external_id string? - This field can be set to another unique ID. In the case that the Category has been imported from another tool, the ID in the other tool can be indicated here.
shortcut: CreateCommentComment
Fields
- text string - The comment text.
- author_id string? - The Member ID of the Comment's author. Defaults to the user identified by the API token.
- created_at string? - Defaults to the time/date the comment is created, but can be set to reflect another date.
- updated_at string? - Defaults to the time/date the comment is last updated, but can be set to reflect another date.
- external_id string? - This field can be set to another unique ID. In the case that the comment has been imported from another tool, the ID in the other tool can be indicated here.
shortcut: CreateEntityTemplate
Request paramaters for creating an entirely new entity template.
Fields
- name string - The name of the new entity template
- author_id string? - The id of the user creating this template.
- story_contents CreateStoryContents - A map of story attributes this template populates.
shortcut: CreateEpic
Fields
- description string? - The Epic's description.
- labels CreateLabelParams[]? - An array of Labels attached to the Epic.
- completed_at_override string? - A manual override for the time/date the Epic was completed.
- name string - The Epic's name.
- planned_start_date string? - The Epic's planned start date.
- state string? -
Deprecated
The Epic's state (to do, in progress, or done); will be ignored whenepic_state_id
is set.
- milestone_id int? - The ID of the Milestone this Epic is related to.
- requested_by_id string? - The ID of the member that requested the epic.
- epic_state_id int? - The ID of the Epic State.
- started_at_override string? - A manual override for the time/date the Epic was started.
- group_id string? - The ID of the group to associate with the epic.
- updated_at string? - Defaults to the time/date it is created but can be set to reflect another date.
- follower_ids string[]? - An array of UUIDs for any Members you want to add as Followers on this new Epic.
- owner_ids string[]? - An array of UUIDs for any members you want to add as Owners on this new Epic.
- external_id string? - This field can be set to another unique ID. In the case that the Epic has been imported from another tool, the ID in the other tool can be indicated here.
- deadline string? - The Epic's deadline.
- created_at string? - Defaults to the time/date it is created but can be set to reflect another date.
shortcut: CreateEpicComment
Fields
- text string - The comment text.
- author_id string? - The Member ID of the Comment's author. Defaults to the user identified by the API token.
- created_at string? - Defaults to the time/date the comment is created, but can be set to reflect another date.
- updated_at string? - Defaults to the time/date the comment is last updated, but can be set to reflect another date.
- external_id string? - This field can be set to another unique ID. In the case that the comment has been imported from another tool, the ID in the other tool can be indicated here.
shortcut: CreateGroup
Fields
- description string? - The description of the Group.
- member_ids string[]? - The Member ids to add to this Group.
- workflow_ids int[]? - The Workflow ids to add to the Group.
- name string - The name of this Group.
- mention_name string - The mention name of this Group.
- color string? - The color you wish to use for the Group in the system.
- color_key string? - The color key you wish to use for the Group in the system.
- display_icon_id string? - The Icon id for the avatar of this Group.
shortcut: CreateIteration
Fields
- follower_ids string[]? - An array of UUIDs for any Members you want to add as Followers.
- group_ids string[]? - An array of UUIDs for any Groups you want to add as Followers. Currently, only one Group association is presented in our web UI.
- labels CreateLabelParams[]? - An array of Labels attached to the Iteration.
- description string? - The description of the Iteration.
- name string - The name of this Iteration.
- start_date string - The date this Iteration begins, e.g. 2019-07-01.
- end_date string - The date this Iteration ends, e.g. 2019-07-01.
shortcut: CreateLabelParams
Request parameters for creating a Label on a Shortcut Story.
Fields
- name string - The name of the new Label.
- description string? - The description of the new Label.
- color string? - The hex color to be displayed with the Label (for example, "#ff0000").
- external_id string? - This field can be set to another unique ID. In the case that the Label has been imported from another tool, the ID in the other tool can be indicated here.
shortcut: CreateLinkedFile
Fields
- description string? - The description of the file.
- story_id int? - The ID of the linked story.
- name string - The name of the file.
- thumbnail_url string? - The URL of the thumbnail, if the integration provided it.
- 'type string - The integration type of the file (e.g. google, dropbox, box).
- size int? - The filesize, if the integration provided it.
- uploader_id string? - The UUID of the member that uploaded the file.
- content_type string? - The content type of the image (e.g. txt/plain).
- url string - The URL of linked file.
shortcut: CreateMilestone
Fields
- name string - The name of the Milestone.
- description string? - The Milestone's description.
- state string? - The workflow state that the Milestone is in.
- started_at_override string? - A manual override for the time/date the Milestone was started.
- completed_at_override string? - A manual override for the time/date the Milestone was completed.
- categories CreateCategoryParams[]? - An array of IDs of Categories attached to the Milestone.
shortcut: CreateOrDeleteStoryReaction
Fields
- emoji string - The emoji short-code to add / remove. E.g.
:thumbsup::skin-tone-4:
.
shortcut: CreateProject
Fields
- description string? - The Project description.
- color string? - The color you wish to use for the Project in the system.
- name string - The name of the Project.
- start_time string? - The date at which the Project was started.
- updated_at string? - Defaults to the time/date it is created but can be set to reflect another date.
- follower_ids string[]? - An array of UUIDs for any members you want to add as Owners on this new Epic.
- external_id string? - This field can be set to another unique ID. In the case that the Project has been imported from another tool, the ID in the other tool can be indicated here.
- team_id int - The ID of the team the project belongs to.
- iteration_length int? - The number of weeks per iteration in this Project.
- abbreviation string? - The Project abbreviation used in Story summaries. Should be kept to 3 characters at most.
- created_at string? - Defaults to the time/date it is created but can be set to reflect another date.
shortcut: CreateStories
Fields
- stories CreateStoryParams[] - An array of stories to be created.
shortcut: CreateStoryComment
Fields
- text string - The comment text.
- author_id string? - The Member ID of the Comment's author. Defaults to the user identified by the API token.
- created_at string? - Defaults to the time/date the comment is created, but can be set to reflect another date.
- updated_at string? - Defaults to the time/date the comment is last updated, but can be set to reflect another date.
- external_id string? - This field can be set to another unique ID. In the case that the comment has been imported from another tool, the ID in the other tool can be indicated here.
shortcut: CreateStoryCommentParams
Request parameters for creating a Comment on a Shortcut Story.
Fields
- text string - The comment text.
- author_id string? - The Member ID of the Comment's author. Defaults to the user identified by the API token.
- created_at string? - Defaults to the time/date the comment is created, but can be set to reflect another date.
- updated_at string? - Defaults to the time/date the comment is last updated, but can be set to reflect another date.
- external_id string? - This field can be set to another unique ID. In the case that the comment has been imported from another tool, the ID in the other tool can be indicated here.
shortcut: CreateStoryContents
A map of story attributes this template populates.
Fields
- description string? - The description of the story.
- entity_type string? - A string description of this resource.
- labels CreateLabelParams[]? - An array of labels to be populated by the template.
- story_type string? - The type of story (feature, bug, chore).
- linked_files LinkedFile[]? - An array of linked files attached to the story.
- file_ids int[]? - An array of the attached file IDs to be populated.
- name string? - The name of the story.
- epic_id int? - The ID of the epic the to be populated.
- external_links string[]? - An array of external links to be populated.
- iteration_id int? - The ID of the iteration the to be populated.
- tasks EntityTemplateTask[]? - An array of tasks to be populated by the template.
- label_ids int[]? - An array of label ids attached to the story.
- group_id string? - The ID of the group to be populated.
- workflow_state_id int? - The ID of the workflow state the story is currently in.
- follower_ids string[]? - An array of UUIDs for any Members listed as Followers.
- owner_ids string[]? - An array of UUIDs of the owners of this story.
- estimate int? - The numeric point estimate to be populated.
- files UploadedFile[]? - An array of files attached to the story.
- project_id int? - The ID of the project the story belongs to.
- linked_file_ids int[]? - An array of the linked file IDs to be populated.
- deadline string? - The due date of the story.
shortcut: CreateStoryLink
Fields
- verb string - The type of link.
- subject_id int - The ID of the subject Story.
- object_id int - The ID of the object Story.
shortcut: CreateStoryLinkParams
Request parameters for creating a Story Link within a Story.
Fields
- subject_id int? - The unique ID of the Story defined as subject.
- verb string - How the subject Story acts on the object Story. This can be "blocks", "duplicates", or "relates to".
- object_id int? - The unique ID of the Story defined as object.
shortcut: CreateStoryParams
Request parameters for creating a story.
Fields
- description string? - The description of the story.
- archived boolean? - Controls the story's archived state.
- story_links CreateStoryLinkParams[]? - An array of story links attached to the story.
- labels CreateLabelParams[]? - An array of labels attached to the story.
- story_type string? - The type of story (feature, bug, chore).
- file_ids int[]? - An array of IDs of files attached to the story.
- completed_at_override string? - A manual override for the time/date the Story was completed.
- name string - The name of the story.
- comments CreateStoryCommentParams[]? - An array of comments to add to the story.
- epic_id int? - The ID of the epic the story belongs to.
- story_template_id string? - The id of the story template used to create this story, if applicable.
- external_links string[]? - An array of External Links associated with this story.
- requested_by_id string? - The ID of the member that requested the story.
- iteration_id int? - The ID of the iteration the story belongs to.
- tasks CreateTaskParams[]? - An array of tasks connected to the story.
- started_at_override string? - A manual override for the time/date the Story was started.
- group_id string? - The id of the group to associate with this story.
- workflow_state_id int? - The ID of the workflow state the story will be in.
- updated_at string? - The time/date the Story was updated.
- follower_ids string[]? - An array of UUIDs of the followers of this story.
- owner_ids string[]? - An array of UUIDs of the owners of this story.
- external_id string? - This field can be set to another unique ID. In the case that the Story has been imported from another tool, the ID in the other tool can be indicated here.
- estimate int? - The numeric point estimate of the story. Can also be null, which means unestimated.
- project_id int? - The ID of the project the story belongs to.
- linked_file_ids int[]? - An array of IDs of linked files attached to the story.
- deadline string? - The due date of the story.
- created_at string? - The time/date the Story was created.
shortcut: CreateTask
Fields
- description string - The Task description.
- complete boolean? - True/false boolean indicating whether the Task is completed. Defaults to false.
- owner_ids string[]? - An array of UUIDs for any members you want to add as Owners on this new Task.
- created_at string? - Defaults to the time/date the Task is created but can be set to reflect another creation time/date.
- updated_at string? - Defaults to the time/date the Task is created in Shortcut but can be set to reflect another time/date.
- external_id string? - This field can be set to another unique ID. In the case that the Task has been imported from another tool, the ID in the other tool can be indicated here.
shortcut: CreateTaskParams
Request parameters for creating a Task on a Story.
Fields
- description string - The Task description.
- complete boolean? - True/false boolean indicating whether the Task is completed. Defaults to false.
- owner_ids string[]? - An array of UUIDs for any members you want to add as Owners on this new Task.
- created_at string? - Defaults to the time/date the Task is created but can be set to reflect another creation time/date.
- updated_at string? - Defaults to the time/date the Task is created in Shortcut but can be set to reflect another time/date.
- external_id string? - This field can be set to another unique ID. In the case that the Task has been imported from another tool, the ID in the other tool can be indicated here.
shortcut: DeleteStories
Fields
- story_ids int[] - An array of IDs of Stories to delete.
shortcut: EntityTemplate
An entity template can be used to prefill various fields when creating new stories.
Fields
- entity_type string - A string description of this resource.
- id string - The unique identifier for the entity template.
- created_at string - The time/date when the entity template was created.
- updated_at string - The time/date when the entity template was last updated.
- name string - The template's name.
- author_id string - The unique ID of the member who created the template.
- last_used_at string - The last time that someone created an entity using this template.
- story_contents StoryContents - A container entity for the attributes this template should populate.
shortcut: EntityTemplateTask
Request parameters for specifying how to pre-populate a task through a template.
Fields
- description string - The Task description.
- complete boolean? - True/false boolean indicating whether the Task is completed. Defaults to false.
- owner_ids string[]? - An array of UUIDs for any members you want to add as Owners on this new Task.
- external_id string? - This field can be set to another unique ID. In the case that the Task has been imported from another tool, the ID in the other tool can be indicated here.
shortcut: Epic
An Epic is a collection of stories that together might make up a release, a milestone, or some other large initiative that your organization is working on.
Fields
- app_url string - The Shortcut application url for the Epic.
- description string - The Epic's description.
- archived boolean - True/false boolean that indicates whether the Epic is archived or not.
- started boolean - A true/false boolean indicating if the Epic has been started.
- entity_type string - A string description of this resource.
- labels LabelSlim[] - An array of Labels attached to the Epic.
- mention_ids string[] - Deprecated: use member_mention_ids.
- member_mention_ids string[] - An array of Member IDs that have been mentioned in the Epic description.
- project_ids int[] - The IDs of Projects related to this Epic.
- stories_without_projects int - The number of stories in this epic which are not associated with a project.
- completed_at_override string - A manual override for the time/date the Epic was completed.
- productboard_plugin_id string - The ID of the associated productboard integration.
- started_at string - The time/date the Epic was started.
- completed_at string - The time/date the Epic was completed.
- name string - The name of the Epic.
- completed boolean - A true/false boolean indicating if the Epic has been completed.
- comments ThreadedComment[] - A nested array of threaded comments.
- productboard_url string - The URL of the associated productboard feature.
- planned_start_date string - The Epic's planned start date.
- state string -
Deprecated
The workflow state that the Epic is in.
- milestone_id int - The ID of the Milestone this Epic is related to.
- requested_by_id string - The ID of the Member that requested the epic.
- epic_state_id int - The ID of the Epic State.
- label_ids int[] - An array of Label ids attached to the Epic.
- started_at_override string - A manual override for the time/date the Epic was started.
- group_id string - Group ID
- updated_at string - The time/date the Epic was updated.
- group_mention_ids string[] - An array of Group IDs that have been mentioned in the Epic description.
- productboard_id string - The ID of the associated productboard feature.
- follower_ids string[] - An array of UUIDs for any Members you want to add as Followers on this Epic.
- owner_ids string[] - An array of UUIDs for any members you want to add as Owners on this new Epic.
- external_id string - This field can be set to another unique ID. In the case that the Epic has been imported from another tool, the ID in the other tool can be indicated here.
- id int - The unique ID of the Epic.
- position int - The Epic's relative position in the Epic workflow state.
- productboard_name string - The name of the associated productboard feature.
- deadline string - The Epic's deadline.
- stats EpicStats - A group of calculated values for this Epic.
- created_at string - The time/date the Epic was created.
shortcut: EpicSearchResults
The results of the Epic search query.
Fields
- total int - The total number of matches for the search query. The first 1000 matches can be paged through via the API.
- data Epic[] - A list of search results.
- next string - The URL path and query string for the next page of search results.
- cursors string[]? - Cursors
shortcut: EpicSlim
EpicSlim represents the same resource as an Epic but is more light-weight, including all Epic fields except the comments array. The description string can be optionally included. Use the Get Epic endpoint to fetch the unabridged payload for an Epic.
Fields
- app_url string - The Shortcut application url for the Epic.
- description string? - The Epic's description.
- archived boolean - True/false boolean that indicates whether the Epic is archived or not.
- started boolean - A true/false boolean indicating if the Epic has been started.
- entity_type string - A string description of this resource.
- labels LabelSlim[] - An array of Labels attached to the Epic.
- mention_ids string[] - Deprecated: use member_mention_ids.
- member_mention_ids string[] - An array of Member IDs that have been mentioned in the Epic description.
- project_ids int[] - The IDs of Projects related to this Epic.
- stories_without_projects int - The number of stories in this epic which are not associated with a project.
- completed_at_override string - A manual override for the time/date the Epic was completed.
- productboard_plugin_id string - The ID of the associated productboard integration.
- started_at string - The time/date the Epic was started.
- completed_at string - The time/date the Epic was completed.
- name string - The name of the Epic.
- completed boolean - A true/false boolean indicating if the Epic has been completed.
- productboard_url string - The URL of the associated productboard feature.
- planned_start_date string - The Epic's planned start date.
- state string -
Deprecated
The workflow state that the Epic is in.
- milestone_id int - The ID of the Milestone this Epic is related to.
- requested_by_id string - The ID of the Member that requested the epic.
- epic_state_id int - The ID of the Epic State.
- label_ids int[] - An array of Label ids attached to the Epic.
- started_at_override string - A manual override for the time/date the Epic was started.
- group_id string - Group ID
- updated_at string - The time/date the Epic was updated.
- group_mention_ids string[] - An array of Group IDs that have been mentioned in the Epic description.
- productboard_id string - The ID of the associated productboard feature.
- follower_ids string[] - An array of UUIDs for any Members you want to add as Followers on this Epic.
- owner_ids string[] - An array of UUIDs for any members you want to add as Owners on this new Epic.
- external_id string - This field can be set to another unique ID. In the case that the Epic has been imported from another tool, the ID in the other tool can be indicated here.
- id int - The unique ID of the Epic.
- position int - The Epic's relative position in the Epic workflow state.
- productboard_name string - The name of the associated productboard feature.
- deadline string - The Epic's deadline.
- stats EpicStats - A group of calculated values for this Epic.
- created_at string - The time/date the Epic was created.
shortcut: EpicState
Epic State is any of the at least 3 columns. Epic States correspond to one of 3 types: Unstarted, Started, or Done.
Fields
- description string - The description of what sort of Epics belong in that Epic State.
- entity_type string - A string description of this resource.
- color string? - The hex color for this Epic State.
- name string - The Epic State's name.
- 'type string - The type of Epic State (Unstarted, Started, or Done)
- updated_at string - When the Epic State was last updated.
- id int - The unique ID of the Epic State.
- position int - The position that the Epic State is in, starting with 0 at the left.
- created_at string - The time/date the Epic State was created.
shortcut: EpicStats
A group of calculated values for this Epic.
Fields
- num_points_done int - The total number of completed points in this Epic.
- num_related_documents int - The total number of documents associated with this Epic.
- average_cycle_time int? - The average cycle time (in seconds) of completed stories in this Epic.
- num_stories_unstarted int - The total number of unstarted Stories in this Epic.
- num_stories_total int - The total number of Stories in this Epic.
- last_story_update string - The date of the last update of a Story in this Epic.
- num_points_started int - The total number of started points in this Epic.
- num_points_unstarted int - The total number of unstarted points in this Epic.
- num_stories_started int - The total number of started Stories in this Epic.
- num_stories_unestimated int - The total number of Stories with no point estimate.
- average_lead_time int? - The average lead time (in seconds) of completed stories in this Epic.
- num_points int - The total number of points in this Epic.
- num_stories_done int - The total number of done Stories in this Epic.
shortcut: EpicWorkflow
Epic Workflow is the array of defined Epic States. Epic Workflow can be queried using the API but must be updated in the Shortcut UI.
Fields
- entity_type string - A string description of this resource.
- id int - The unique ID of the Epic Workflow.
- created_at string - The date the Epic Workflow was created.
- updated_at string - The date the Epic Workflow was updated.
- default_epic_state_id int - The unique ID of the default Epic State that new Epics are assigned by default.
- epic_states EpicState[] - A map of the Epic States in this Epic Workflow.
shortcut: GetEpicStories
Fields
- includes_description boolean? - A true/false boolean indicating whether to return Stories with their descriptions.
shortcut: GetExternalLinkStoriesParams
Fields
- external_link string - The external link associated with one or more stories.
shortcut: GetIterationStories
Fields
- includes_description boolean? - A true/false boolean indicating whether to return Stories with their descriptions.
shortcut: GetLabelStories
Fields
- includes_description boolean? - A true/false boolean indicating whether to return Stories with their descriptions.
shortcut: GetMember
Fields
- 'org\-public\-id string? - The unique ID of the Organization to limit the lookup to.
shortcut: GetProjectStories
Fields
- includes_description boolean? - A true/false boolean indicating whether to return Stories with their descriptions.
shortcut: Group
A Group.
Fields
- app_url string - The Shortcut application url for the Group.
- description string - The description of the Group.
- archived boolean - Whether or not the Group is archived.
- entity_type string - A string description of this resource.
- color string - The hex color to be displayed with the Group (for example, "#ff0000").
- num_stories_started int - The number of stories assigned to the group which are in a started workflow state.
- mention_name string - The mention name of the Group.
- name string - The name of the Group.
- color_key string - The color key to be displayed with the Group.
- num_stories int - The total number of stories assigned ot the group.
- num_epics_started int - The number of epics assigned to the group which are in the started workflow state.
- id string - The id of the Group.
- display_icon Icon - Icons are used to attach images to Organizations, Members, and Loading screens in the Shortcut web application.
- member_ids string[] - The Member IDs contain within the Group.
- story_workflow_ids int[] - The Workflow IDs which have stories assigned to the group.
- workflow_ids int[] - The Workflow IDs contained within the Group.
shortcut: History
A history item is a group of actions that represent a transactional change to a Story.
Fields
- changed_at string - The date when the change occurred.
- primary_id int? - The ID of the primary entity that has changed, if applicable.
- references (HistoryReferenceBranch|HistoryReferenceCommit|HistoryReferenceEpic|HistoryReferenceGroup|HistoryReferenceIteration|HistoryReferenceLabel|HistoryReferenceProject|HistoryReferenceStory|HistoryReferenceStoryTask|HistoryReferenceWorkflowState|HistoryReferenceGeneral)[]? - An array of objects affected by the change. Reference objects provide basic information for the entities reference in the history actions. Some have specific fields, but they always contain an id, entity_type, and a name.
- actions record {}[] - An array of actions that were performed for the change.
- member_id string? - The ID of the member who performed the change.
- external_id string? - The ID of the webhook that handled the change.
- id string - The ID representing the change for the story.
- 'version string - The version of the change format.
- webhook_id string? - The ID of the webhook that handled the change.
shortcut: HistoryActionBranchCreate
An action representing a VCS Branch being created.
Fields
- id int - The ID of the entity referenced.
- entity_type string - The type of entity referenced.
- name string - The name of the VCS Branch that was pushed
- url string - The URL from the provider of the VCS Branch that was pushed
- action string - The action of the entity referenced.
shortcut: HistoryActionBranchMerge
An action representing a VCS Branch being merged.
Fields
- id int - The ID of the entity referenced.
- entity_type string - The type of entity referenced.
- name string - The name of the VCS Branch that was pushed
- url string - The URL from the provider of the VCS Branch that was pushed
- action string - The action of the entity referenced.
shortcut: HistoryActionBranchPush
An action representing a VCS Branch being pushed.
Fields
- id int - The ID of the entity referenced.
- entity_type string - The type of entity referenced.
- name string - The name of the VCS Branch that was pushed
- url string - The URL from the provider of the VCS Branch that was pushed
- action string - The action of the entity referenced.
shortcut: HistoryActionLabelCreate
An action representing a Label being created.
Fields
- id int - The ID of the entity referenced.
- entity_type string - The type of entity referenced.
- action string - The action of the entity referenced.
- app_url string - The application URL of the Label.
- name string - The name of the Label.
shortcut: HistoryActionLabelDelete
An action representing a Label being deleted.
Fields
- id int - The ID of the entity referenced.
- entity_type string - The type of entity referenced.
- action string - The action of the entity referenced.
- name string - The name of the Label.
shortcut: HistoryActionLabelUpdate
An action representing a Label being updated.
Fields
- id int - The ID of the entity referenced.
- entity_type string - The type of entity referenced.
- action string - The action of the entity referenced.
shortcut: HistoryActionProjectUpdate
An action representing a Project being updated.
Fields
- id int - The ID of the entity referenced.
- entity_type string - The type of entity referenced.
- action string - The action of the entity referenced.
- app_url string - The application URL of the Project.
- name string - The name of the Project.
shortcut: HistoryActionPullRequest
An action representing various operations for a Pull Request.
Fields
- id int - The ID of the entity referenced.
- entity_type string - The type of entity referenced.
- action string - The action of the entity referenced.
- number int - The VCS Repository-specific ID for the Pull Request.
- title string - The title of the Pull Request.
- url string - The URL from the provider of the VCS Pull Request.
shortcut: HistoryActionStoryCommentCreate
An action representing a Story Comment being created.
Fields
- id int - The ID of the entity referenced.
- entity_type string - The type of entity referenced.
- action string - The action of the entity referenced.
- app_url string - The application URL of the Story Comment.
- text string - The text of the Story Comment.
- author_id string - The Member ID of who created the Story Comment.
shortcut: HistoryActionStoryCreate
An action representing a Story being created.
Fields
- app_url string - The application URL of the Story.
- description string? - The description of the Story.
- started boolean? - Whether or not the Story has been started.
- entity_type string - The type of entity referenced.
- task_ids int[]? - An array of Task IDs on this Story.
- story_type string - The type of Story; either feature, bug, or chore.
- name string - The name of the Story.
- completed boolean? - Whether or not the Story is completed.
- blocker boolean? - Whether or not the Story is blocking another Story.
- epic_id int? - The Epic ID for this Story.
- requested_by_id string? - The ID of the Member that requested the Story.
- iteration_id int? - The Iteration ID the Story is in.
- label_ids int[]? - An array of Labels IDs attached to the Story.
- workflow_state_id int? - An array of Workflow State IDs attached to the Story.
- object_story_link_ids int[]? - An array of Story IDs that are the object of a Story Link relationship.
- follower_ids string[]? - An array of Member IDs for the followers of the Story.
- owner_ids string[]? - An array of Member IDs that are the owners of the Story.
- id int - The ID of the entity referenced.
- estimate int? - The estimate (or point value) for the Story.
- subject_story_link_ids int[]? - An array of Story IDs that are the subject of a Story Link relationship.
- action string - The action of the entity referenced.
- blocked boolean? - Whether or not the Story is blocked by another Story.
- project_id int? - The Project ID of the Story is in.
- deadline string? - The timestamp representing the Story's deadline.
shortcut: HistoryActionStoryDelete
An action representing a Story being deleted.
Fields
- id int - The ID of the entity referenced.
- entity_type string - The type of entity referenced.
- action string - The action of the entity referenced.
- name string - The name of the Story.
- story_type string - The type of Story; either feature, bug, or chore.
shortcut: HistoryActionStoryLinkCreate
An action representing a Story Link being created.
Fields
- id int - The ID of the entity referenced.
- entity_type string - The type of entity referenced.
- action string - The action of the entity referenced.
- verb string - The verb describing the link's relationship.
- subject_id int - The Story ID of the subject Story.
- object_id int - The Story ID of the object Story.
shortcut: HistoryActionStoryLinkDelete
An action representing a Story Link being deleted.
Fields
- id int - The ID of the entity referenced.
- entity_type string - The type of entity referenced.
- action string - The action of the entity referenced.
- verb string - The verb describing the link's relationship.
- subject_id int - The Story ID of the subject Story.
- object_id int - The Story ID of the object Story.
shortcut: HistoryActionStoryLinkUpdate
An action representing a Story Link being updated.
Fields
- id int - The ID of the entity referenced.
- entity_type string - The type of entity referenced.
- action string - The action of the entity referenced.
- verb string - The verb describing the link's relationship.
- subject_id int - The Story ID of the subject Story.
- object_id int - The Story ID of the object Story.
- changes HistoryChangesStoryLink - The changes that have occurred as a result of the action.
shortcut: HistoryActionStoryUpdate
An action representing a Story being updated.
Fields
- id int - The ID of the entity referenced.
- entity_type string - The type of entity referenced.
- action string - The action of the entity referenced.
- app_url string - The application URL of the Story.
- changes HistoryChangesStory? - The changes that have occurred as a result of the action.
- name string - The name of the Story.
- story_type string - The type of Story; either feature, bug, or chore.
shortcut: HistoryActionTaskCreate
An action representing a Task being created.
Fields
- description string - The description of the Task.
- entity_type string - The type of entity referenced.
- mention_ids string[]? - An array of Member IDs that represent who has been mentioned in the Task.
- group_mention_ids string[]? - An array of Groups IDs that represent which have been mentioned in the Task.
- owner_ids string[]? - An array of Member IDs that represent the Task's owners.
- id int - The ID of the entity referenced.
- action string - The action of the entity referenced.
- complete boolean - Whether or not the Task is complete.
- deadline string? - A timestamp that represent's the Task's deadline.
shortcut: HistoryActionTaskDelete
An action representing a Task being deleted.
Fields
- id int - The ID of the entity referenced.
- entity_type string - The type of entity referenced.
- action string - The action of the entity referenced.
- description string - The description of the Task being deleted.
shortcut: HistoryActionTaskUpdate
An action representing a Task being updated.
Fields
- id int - The ID of the entity referenced.
- entity_type string - The type of entity referenced.
- action string - The action of the entity referenced.
- changes HistoryChangesTask - The changes that have occurred as a result of the action.
- complete boolean? - Whether or not the Task is complete.
- description string - The description of the Task.
- story_id int - The Story ID that contains the Task.
shortcut: HistoryChangesStory
The changes that have occurred as a result of the action.
Fields
- description StoryHistoryChangeOldNewStr? - A timestamp that represents the Story's deadline.
- archived StoryHistoryChangeOldNewBool? - True if the Story has archived, otherwise false.
- started StoryHistoryChangeOldNewBool? - True if the Story has archived, otherwise false.
- task_ids StoryHistoryChangeAddsRemovesInt? - Task IDs that have been added or removed from the Story.
- mention_ids StoryHistoryChangeAddsRemovesUuid? - Member IDs that have been added or removed as a owner of the Story.
- story_type StoryHistoryChangeOldNewStr? - A timestamp that represents the Story's deadline.
- name StoryHistoryChangeOldNewStr? - A timestamp that represents the Story's deadline.
- completed StoryHistoryChangeOldNewBool? - True if the Story has archived, otherwise false.
- blocker StoryHistoryChangeOldNewBool? - True if the Story has archived, otherwise false.
- epic_id StoryHistoryChangeOldNewInt? - The estimate value for the Story
- branch_ids StoryHistoryChangeAddsRemovesInt? - Task IDs that have been added or removed from the Story.
- commit_ids StoryHistoryChangeAddsRemovesInt? - Task IDs that have been added or removed from the Story.
- requested_by_id StoryHistoryChangeOldNewUuid? - The Member ID of the preson who requested the Story.
- iteration_id StoryHistoryChangeOldNewInt? - The estimate value for the Story
- label_ids StoryHistoryChangeAddsRemovesInt? - Task IDs that have been added or removed from the Story.
- workflow_state_id StoryHistoryChangeOldNewInt? - The estimate value for the Story
- object_story_link_ids StoryHistoryChangeAddsRemovesInt? - Task IDs that have been added or removed from the Story.
- follower_ids StoryHistoryChangeAddsRemovesUuid? - Member IDs that have been added or removed as a owner of the Story.
- owner_ids StoryHistoryChangeAddsRemovesUuid? - Member IDs that have been added or removed as a owner of the Story.
- estimate StoryHistoryChangeOldNewInt? - The estimate value for the Story
- subject_story_link_ids StoryHistoryChangeAddsRemovesInt? - Task IDs that have been added or removed from the Story.
- blocked StoryHistoryChangeOldNewBool? - True if the Story has archived, otherwise false.
- project_id StoryHistoryChangeOldNewInt? - The estimate value for the Story
- deadline StoryHistoryChangeOldNewStr? - A timestamp that represents the Story's deadline.
shortcut: HistoryChangesStoryLink
The changes that have occurred as a result of the action.
Fields
- verb StoryHistoryChangeOldNewStr? - A timestamp that represents the Story's deadline.
- object_id StoryHistoryChangeOldNewInt? - The estimate value for the Story
- subject_id StoryHistoryChangeOldNewInt? - The estimate value for the Story
shortcut: HistoryChangesTask
The changes that have occurred as a result of the action.
Fields
- complete StoryHistoryChangeOldNewBool? - True if the Story has archived, otherwise false.
- description StoryHistoryChangeOldNewStr? - A timestamp that represents the Story's deadline.
- mention_ids StoryHistoryChangeAddsRemovesUuid? - Member IDs that have been added or removed as a owner of the Story.
- owner_ids StoryHistoryChangeAddsRemovesUuid? - Member IDs that have been added or removed as a owner of the Story.
shortcut: HistoryReferenceBranch
A reference to a VCS Branch.
Fields
- id int - The ID of the entity referenced.
- entity_type string - The type of entity referenced.
- name string - The name of the entity referenced.
- url string - The external URL for the Branch.
shortcut: HistoryReferenceCommit
A reference to a VCS Commit.
Fields
- id int - The ID of the entity referenced.
- entity_type string - The type of entity referenced.
- message string - The message from the Commit.
- url string - The external URL for the Branch.
shortcut: HistoryReferenceEpic
A reference to an Epic.
Fields
- id int - The ID of the entity referenced.
- entity_type string - The type of entity referenced.
- app_url string - The application URL of the Epic.
- name string - The name of the entity referenced.
shortcut: HistoryReferenceGeneral
A default reference for entity types that don't have extra fields.
Fields
- id int - The ID of the entity referenced.
- entity_type string - The type of entity referenced.
- name string - The name of the entity referenced.
shortcut: HistoryReferenceGroup
A reference to a Group.
Fields
- id string - The ID of the entity referenced.
- entity_type string - The type of entity referenced.
- name string - The name of the entity referenced.
shortcut: HistoryReferenceIteration
A reference to an Iteration.
Fields
- id int - The ID of the entity referenced.
- entity_type string - The type of entity referenced.
- app_url string - The application URL of the Iteration.
- name string - The name of the entity referenced.
shortcut: HistoryReferenceLabel
A reference to an Label.
Fields
- id int - The ID of the entity referenced.
- entity_type string - The type of entity referenced.
- app_url string - The application URL of the Label.
- name string - The name of the entity referenced.
shortcut: HistoryReferenceProject
A reference to an Project.
Fields
- id int - The ID of the entity referenced.
- entity_type string - The type of entity referenced.
- app_url string - The application URL of the Project.
- name string - The name of the entity referenced.
shortcut: HistoryReferenceStory
A reference to a Story.
Fields
- id int - The ID of the entity referenced.
- entity_type string - The type of entity referenced.
- app_url string - The application URL of the Story.
- name string - The name of the entity referenced.
- story_type string - If the referenced entity is a Story, either "bug", "chore", or "feature".
shortcut: HistoryReferenceStoryTask
A reference to a Story Task.
Fields
- id int - The ID of the entity referenced.
- entity_type string - The type of entity referenced.
- description string - The description of the Story Task.
shortcut: HistoryReferenceWorkflowState
A references to a Story Workflow State.
Fields
- id int - The ID of the entity referenced.
- entity_type string - The type of entity referenced.
- 'type string - Either "unstarted", "started", or "done".
- name string - The name of the entity referenced.
shortcut: Icon
Icons are used to attach images to Organizations, Members, and Loading screens in the Shortcut web application.
Fields
- entity_type string - A string description of this resource.
- id string - The unique ID of the Icon.
- created_at string - The time/date that the Icon was created.
- updated_at string - The time/date that the Icon was updated.
- url string - The URL of the Icon.
shortcut: Identity
The Identity of the VCS user that authored the Commit.
Fields
- entity_type string - A string description of this resource.
- name string - This is your login in VCS.
- 'type string - The type of Identity; currently only type is github.
shortcut: Iteration
An Iteration is a defined, time-boxed period of development for a collection of Stories. See https://help.shortcut.com/hc/en-us/articles/360028953452-Iterations-Overview for more information.
Fields
- app_url string - The Shortcut application url for the Iteration.
- description string - The description of the iteration.
- entity_type string - A string description of this resource
- labels Label[] - An array of labels attached to the iteration.
- mention_ids string[] - Deprecated: use member_mention_ids.
- member_mention_ids string[] - An array of Member IDs that have been mentioned in the Story description.
- name string - The name of the iteration.
- label_ids int[] - An array of label ids attached to the iteration.
- updated_at string - The instant when this iteration was last updated.
- group_mention_ids string[] - An array of Group IDs that have been mentioned in the Story description.
- end_date string - The date this iteration begins.
- follower_ids string[] - An array of UUIDs for any Members listed as Followers.
- group_ids string[] - An array of UUIDs for any Groups you want to add as Followers. Currently, only one Group association is presented in our web UI.
- start_date string - The date this iteration begins.
- status string - The status of the iteration. Values are either "unstarted", "started", or "done".
- id int - The ID of the iteration.
- stats IterationStats - A group of calculated values for this Iteration.
- created_at string - The instant when this iteration was created.
shortcut: IterationSlim
IterationSlim represents the same resource as an Iteration, but is more light-weight. Use the Get Iteration endpoint to fetch the unabridged payload for an Iteration.
Fields
- app_url string - The Shortcut application url for the Iteration.
- entity_type string - A string description of this resource
- labels Label[] - An array of labels attached to the iteration.
- mention_ids string[] - Deprecated: use member_mention_ids.
- member_mention_ids string[] - An array of Member IDs that have been mentioned in the Story description.
- name string - The name of the iteration.
- label_ids int[] - An array of label ids attached to the iteration.
- updated_at string - The instant when this iteration was last updated.
- group_mention_ids string[] - An array of Group IDs that have been mentioned in the Story description.
- end_date string - The date this iteration begins.
- follower_ids string[] - An array of UUIDs for any Members listed as Followers.
- group_ids string[] - An array of UUIDs for any Groups you want to add as Followers. Currently, only one Group association is presented in our web UI.
- start_date string - The date this iteration begins.
- status string - The status of the iteration. Values are either "unstarted", "started", or "done".
- id int - The ID of the iteration.
- stats IterationStats - A group of calculated values for this Iteration.
- created_at string - The instant when this iteration was created.
shortcut: IterationStats
A group of calculated values for this Iteration.
Fields
- num_points_done int - The total number of completed points in this Iteration.
- num_related_documents int - The total number of documents related to an Iteration
- average_cycle_time int? - The average cycle time (in seconds) of completed stories in this Iteration.
- num_stories_unstarted int - The total number of unstarted Stories in this Iteration.
- num_points_started int - The total number of started points in this Iteration.
- num_points_unstarted int - The total number of unstarted points in this Iteration.
- num_stories_started int - The total number of started Stories in this Iteration.
- num_stories_unestimated int - The total number of Stories with no point estimate.
- average_lead_time int? - The average lead time (in seconds) of completed stories in this Iteration.
- num_points int - The total number of points in this Iteration.
- num_stories_done int - The total number of done Stories in this Iteration.
shortcut: Label
A Label can be used to associate and filter Stories and Epics, and also create new Workspaces.
Fields
- app_url string - The Shortcut application url for the Label.
- description string - The description of the Label.
- archived boolean - A true/false boolean indicating if the Label has been archived.
- entity_type string - A string description of this resource.
- color string - The hex color to be displayed with the Label (for example, "#ff0000").
- name string - The name of the Label.
- updated_at string - The time/date that the Label was updated.
- external_id string - This field can be set to another unique ID. In the case that the Label has been imported from another tool, the ID in the other tool can be indicated here.
- id int - The unique ID of the Label.
- stats LabelStats? - A group of calculated values for this Label. This is not included if the slim? flag is set to true for the List Labels endpoint.
- created_at string - The time/date that the Label was created.
shortcut: LabelSlim
A Label can be used to associate and filter Stories and Epics, and also create new Workspaces. A slim Label does not include aggregate stats. Fetch the Label using the labels endpoint to retrieve them.
Fields
- app_url string - The Shortcut application url for the Label.
- description string - The description of the Label.
- archived boolean - A true/false boolean indicating if the Label has been archived.
- entity_type string - A string description of this resource.
- color string - The hex color to be displayed with the Label (for example, "#ff0000").
- name string - The name of the Label.
- updated_at string - The time/date that the Label was updated.
- external_id string - This field can be set to another unique ID. In the case that the Label has been imported from another tool, the ID in the other tool can be indicated here.
- id int - The unique ID of the Label.
- created_at string - The time/date that the Label was created.
shortcut: LabelStats
A group of calculated values for this Label. This is not included if the slim? flag is set to true for the List Labels endpoint.
Fields
- num_related_documents int - The total number of Documents associated this Label.
- num_epics int - The total number of Epics with this Label.
- num_stories_unstarted int - The total number of stories unstarted Stories with this Label.
- num_stories_total int - The total number of Stories with this Label.
- num_epics_unstarted int - The number of unstarted epics assoicated with this label.
- num_epics_in_progress int - The number of in progress epics assoicated with this label.
- num_points_unstarted int - The total number of unstarted points with this Label.
- num_stories_unestimated int - The total number of Stories with no point estimate with this Label.
- num_points_in_progress int - The total number of in-progress points with this Label.
- num_epics_total int - The total number of Epics assoicated with this Label.
- num_stories_completed int - The total number of completed Stories with this Label.
- num_points_completed int - The total number of completed points with this Label.
- num_points_total int - The total number of points with this Label.
- num_stories_in_progress int - The total number of in-progress Stories with this Label.
- num_epics_completed int - The number of completed Epics assoicated with this Label.
shortcut: LinkedFile
Linked files are stored on a third-party website and linked to one or more Stories. Shortcut currently supports linking files from Google Drive, Dropbox, Box, and by URL.
Fields
- description string - The description of the file.
- entity_type string - A string description of this resource.
- story_ids int[] - The IDs of the stories this file is attached to.
- mention_ids string[] - Deprecated: use member_mention_ids.
- member_mention_ids string[] - The members that are mentioned in the description of the file.
- name string - The name of the linked file.
- thumbnail_url string - The URL of the file thumbnail, if the integration provided it.
- 'type string - The integration type (e.g. google, dropbox, box).
- size int - The filesize, if the integration provided it.
- uploader_id string - The UUID of the member that uploaded the file.
- content_type string - The content type of the image (e.g. txt/plain).
- updated_at string - The time/date the LinkedFile was updated.
- group_mention_ids string[] - The groups that are mentioned in the description of the file.
- id int - The unique identifier for the file.
- url string - The URL of the file.
- created_at string - The time/date the LinkedFile was created.
shortcut: ListEpics
Fields
- includes_description boolean? - A true/false boolean indicating whether to return Epics with their descriptions.
shortcut: ListGroupStories
Fields
- 'limit int? - The maximum number of results to return. (Defaults to 1000, max 1000)
- offset int? - The offset at which to begin returning results. (Defaults to 0)
shortcut: ListLabels
Fields
- slim boolean? - A true/false boolean indicating if the slim versions of the Label should be returned.
shortcut: ListMembers
Fields
- 'org\-public\-id string? - The unique ID of the Organization to limit the list to.
shortcut: MaxSearchResultsExceededError
Error returned when total maximum supported results have been reached.
Fields
- 'error string - The name for this type of error,
maximum-results-exceeded
- message string - An explanatory message: "A maximum of 1000 search results are supported."
- 'maximum\-results int - The maximum number of search results supported,
1000
shortcut: Member
Details about individual Shortcut user within the Shortcut organization that has issued the token.
Fields
- role string - The Member's role in the Shortcut organization.
- entity_type string - A string description of this resource.
- disabled boolean - True/false boolean indicating whether the Member has been disabled within this Organization.
- state string - The user state, one of partial, full, disabled, or imported. A partial user is disabled, has no means to log in, and is not an import user. A full user is enabled and has a means to log in. A disabled user is disabled and has a means to log in. An import user is disabled, has no means to log in, and is marked as an import user.
- updated_at string - The time/date the Member was last updated.
- created_without_invite boolean - Whether this member was created as a placeholder entity.
- group_ids string[] - The Member's group ids
- id string - The Member's ID in Shortcut.
- profile Profile - A group of Member profile details.
- created_at string - The time/date the Member was created.
- replaced_by string? - The id of the member that replaces this one when merged.
shortcut: MemberInfo
Fields
- id string -
- name string -
- mention_name string -
- workspace2 BasicWorkspaceInfo -
shortcut: Milestone
A Milestone is a collection of Epics that represent a release or some other large initiative that your organization is working on.
Fields
- app_url string - The Shortcut application url for the Milestone.
- description string - The Milestone's description.
- started boolean - A true/false boolean indicating if the Milestone has been started.
- entity_type string - A string description of this resource.
- completed_at_override string - A manual override for the time/date the Milestone was completed.
- started_at string - The time/date the Milestone was started.
- completed_at string - The time/date the Milestone was completed.
- name string - The name of the Milestone.
- completed boolean - A true/false boolean indicating if the Milestone has been completed.
- state string - The workflow state that the Milestone is in.
- started_at_override string - A manual override for the time/date the Milestone was started.
- updated_at string - The time/date the Milestone was updated.
- categories Category[] - An array of Categories attached to the Milestone.
- id int - The unique ID of the Milestone.
- position int - A number representing the position of the Milestone in relation to every other Milestone within the Organization.
- stats MilestoneStats? - A group of calculated values for this Milestone.
- created_at string - The time/date the Milestone was created.
shortcut: MilestoneStats
A group of calculated values for this Milestone.
Fields
- average_cycle_time int? - The average cycle time (in seconds) of completed stories in this Milestone.
- average_lead_time int? - The average lead time (in seconds) of completed stories in this Milestone.
- num_related_documents int - The number of related documents tp this Milestone.
shortcut: Profile
A group of Member profile details.
Fields
- entity_type string - A string description of this resource.
- deactivated boolean - A true/false boolean indicating whether the Member has been deactivated within Shortcut.
- two_factor_auth_activated boolean? - If Two Factor Authentication is activated for this User.
- mention_name string - The Member's username within the Organization.
- name string - The Member's name within the Organization.
- gravatar_hash string - This is the gravatar hash associated with email_address.
- id string - The unique identifier of the profile.
- display_icon Icon - Icons are used to attach images to Organizations, Members, and Loading screens in the Shortcut web application.
- email_address string - The primary email address of the Member with the Organization.
shortcut: Project
Projects typically map to teams (such as Frontend, Backend, Mobile, Devops, etc) but can represent any open-ended product, component, or initiative.
Fields
- app_url string - The Shortcut application url for the Project.
- description string - The description of the Project.
- archived boolean - True/false boolean indicating whether the Project is in an Archived state.
- entity_type string - A string description of this resource.
- days_to_thermometer int - The number of days before the thermometer appears in the Story summary.
- color string - The color associated with the Project in the Shortcut member interface.
- workflow_id int - The ID of the workflow the project belongs to.
- name string - The name of the Project
- start_time string - The date at which the Project was started.
- updated_at string - The time/date that the Project was last updated.
- follower_ids string[] - An array of UUIDs for any Members listed as Followers.
- external_id string - This field can be set to another unique ID. In the case that the Project has been imported from another tool, the ID in the other tool can be indicated here.
- id int - The unique ID of the Project.
- show_thermometer boolean - Configuration to enable or disable thermometers in the Story summary.
- team_id int - The ID of the team the project belongs to.
- iteration_length int - The number of weeks per iteration in this Project.
- abbreviation string - The Project abbreviation used in Story summaries. Should be kept to 3 characters at most.
- stats ProjectStats - A group of calculated values for this Project.
- created_at string - The time/date that the Project was created.
shortcut: ProjectStats
A group of calculated values for this Project.
Fields
- num_stories int - The total number of stories in this Project.
- num_points int - The total number of points in this Project.
- num_related_documents int - The total number of documents related to this Project
shortcut: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
shortcut: PullRequest
Corresponds to a VCS Pull Request attached to a Shortcut story.
Fields
- entity_type string - A string description of this resource.
- closed boolean - True/False boolean indicating whether the VCS pull request has been closed.
- merged boolean - True/False boolean indicating whether the VCS pull request has been merged.
- num_added int - Number of lines added in the pull request, according to VCS.
- branch_id int - The ID of the branch for the particular pull request.
- number int - The pull request's unique number ID in VCS.
- branch_name string - The name of the branch for the particular pull request.
- target_branch_name string - The name of the target branch for the particular pull request.
- num_commits int - The number of commits on the pull request.
- title string - The title of the pull request.
- updated_at string - The time/date the pull request was created.
- draft boolean - True/False boolean indicating whether the VCS pull request is in the draft state.
- id int - The unique ID associated with the pull request in Shortcut.
- vcs_labels PullRequestLabel[]? - An array of PullRequestLabels attached to the PullRequest.
- url string - The URL for the pull request.
- num_removed int - Number of lines removed in the pull request, according to VCS.
- review_status string? - The status of the review for the pull request.
- num_modified int - Number of lines modified in the pull request, according to VCS.
- build_status string? - The status of the Continuous Integration workflow for the pull request.
- target_branch_id int - The ID of the target branch for the particular pull request.
- repository_id int - The ID of the repository for the particular pull request.
- created_at string - The time/date the pull request was created.
shortcut: PullRequestLabel
Corresponds to a VCS Label associated with a Pull Request.
Fields
- entity_type string - A string description of this resource.
- id int - The unique ID of the VCS Label.
- color string - The color of the VCS label.
- description string? - The description of the VCS label.
- name string - The name of the VCS label.
shortcut: Repository
Repository refers to a VCS repository.
Fields
- entity_type string - A string description of this resource.
- name string - The shorthand name of the VCS repository.
- 'type string - The type of Repository. Currently this can only be "github".
- updated_at string - The time/date the Repository was updated.
- external_id string - The VCS unique identifier for the Repository.
- id int - The ID associated to the VCS repository in Shortcut.
- url string - The URL of the Repository.
- full_name string - The full name of the VCS repository.
- created_at string - The time/date the Repository was created.
shortcut: Search
Fields
- query string - See our help center article on search operators
- page_size int? - The number of search results to include in a page. Minimum of 1 and maximum of 25.
- next string? - The next page token.
- include string? -
shortcut: SearchResults
The results of the multi-entity search query.
Fields
- epics EpicSearchResults - The results of the Epic search query.
- stories StorySearchResults - The results of the Story search query.
shortcut: SearchStories
Fields
- archived boolean? - A true/false boolean indicating whether the Story is in archived state.
- owner_id string? - An array of UUIDs for any Users who may be Owners of the Stories.
- story_type string? - The type of Stories that you want returned.
- epic_ids int[]? - The Epic IDs that may be associated with the Stories.
- project_ids int[]? - The IDs for the Projects the Stories may be assigned to.
- updated_at_end string? - Stories should have been updated before this date.
- completed_at_end string? - Stories should have been completed before this date.
- workflow_state_types string[]? - The type of Workflow State the Stories may be in.
- deadline_end string? - Stories should have a deadline before this date.
- created_at_start string? - Stories should have been created after this date.
- epic_id int? - The Epic IDs that may be associated with the Stories.
- label_name string? - The name of any associated Labels.
- requested_by_id string? - The UUID of any Users who may have requested the Stories.
- iteration_id int? - The Iteration ID that may be associated with the Stories.
- label_ids int[]? - The Label IDs that may be associated with the Stories.
- group_id string? - The Group ID that is associated with the Stories
- workflow_state_id int? - The unique IDs of the specific Workflow States that the Stories should be in.
- iteration_ids int[]? - The Iteration IDs that may be associated with the Stories.
- created_at_end string? - Stories should have been created before this date.
- deadline_start string? - Stories should have a deadline after this date.
- group_ids string[]? - The Group IDs that are associated with the Stories
- owner_ids string[]? - An array of UUIDs for any Users who may be Owners of the Stories.
- external_id string? - An ID or URL that references an external resource. Useful during imports.
- includes_description boolean? - Whether to include the story description in the response.
- estimate int? - The number of estimate points associate with the Stories.
- project_id int? - The IDs for the Projects the Stories may be assigned to.
- completed_at_start string? - Stories should have been competed after this date.
- updated_at_start string? - Stories should have been updated after this date.
shortcut: Story
Stories are the standard unit of work in Shortcut and represent individual features, bugs, and chores.
Fields
- app_url string - The Shortcut application url for the Story.
- description string - The description of the story.
- archived boolean - True if the story has been archived or not.
- started boolean - A true/false boolean indicating if the Story has been started.
- story_links TypedStoryLink[] - An array of story links attached to the Story.
- entity_type string - A string description of this resource.
- labels LabelSlim[] - An array of labels attached to the story.
- mention_ids string[] - Deprecated: use member_mention_ids.
- member_mention_ids string[] - An array of Member IDs that have been mentioned in the Story description.
- story_type string - The type of story (feature, bug, chore).
- linked_files LinkedFile[] - An array of linked files attached to the story.
- workflow_id int - The ID of the workflow the story belongs to.
- completed_at_override string - A manual override for the time/date the Story was completed.
- started_at string - The time/date the Story was started.
- completed_at string - The time/date the Story was completed.
- name string - The name of the story.
- completed boolean - A true/false boolean indicating if the Story has been completed.
- comments StoryComment[] - An array of comments attached to the story.
- blocker boolean - A true/false boolean indicating if the Story is currently a blocker of another story.
- branches Branch[] - An array of Git branches attached to the story.
- epic_id int - The ID of the epic the story belongs to.
- story_template_id string - The ID of the story template used to create this story, or null if not created using a template.
- external_links string[] - An array of external links (strings) associated with a Story
- previous_iteration_ids int[] - The IDs of the iteration the story belongs to.
- requested_by_id string - The ID of the Member that requested the story.
- iteration_id int - The ID of the iteration the story belongs to.
- tasks Task[] - An array of tasks connected to the story.
- label_ids int[] - An array of label ids attached to the story.
- started_at_override string - A manual override for the time/date the Story was started.
- group_id string - The ID of the group associated with the story.
- workflow_state_id int - The ID of the workflow state the story is currently in.
- updated_at string - The time/date the Story was updated.
- pull_requests PullRequest[] - An array of Pull/Merge Requests attached to the story.
- group_mention_ids string[] - An array of Group IDs that have been mentioned in the Story description.
- follower_ids string[] - An array of UUIDs for any Members listed as Followers.
- owner_ids string[] - An array of UUIDs of the owners of this story.
- external_id string - This field can be set to another unique ID. In the case that the Story has been imported from another tool, the ID in the other tool can be indicated here.
- id int - The unique ID of the Story.
- lead_time int? - The lead time (in seconds) of this story when complete.
- estimate int - The numeric point estimate of the story. Can also be null, which means unestimated.
- commits Commit[] - An array of commits attached to the story.
- files UploadedFile[] - An array of files attached to the story.
- position int - A number representing the position of the story in relation to every other story in the current project.
- blocked boolean - A true/false boolean indicating if the Story is currently blocked.
- project_id int - The ID of the project the story belongs to.
- deadline string - The due date of the story.
- stats StoryStats - The stats object for Stories
- cycle_time int? - The cycle time (in seconds) of this story when complete.
- created_at string - The time/date the Story was created.
- moved_at string - The time/date the Story was last changed workflow-state.
shortcut: StoryComment
A Comment is any note added within the Comment field of a Story.
Fields
- app_url string - The Shortcut application url for the Comment.
- entity_type string - A string description of this resource.
- story_id int - The ID of the Story on which the Comment appears.
- mention_ids string[] - Deprecated: use member_mention_ids.
- author_id string - The unique ID of the Member who is the Comment's author.
- member_mention_ids string[] - The unique IDs of the Member who are mentioned in the Comment.
- updated_at string - The time/date when the Comment was updated.
- group_mention_ids string[] - The unique IDs of the Group who are mentioned in the Comment.
- external_id string - This field can be set to another unique ID. In the case that the Comment has been imported from another tool, the ID in the other tool can be indicated here.
- id int - The unique ID of the Comment.
- position int - The Comments numerical position in the list from oldest to newest.
- reactions StoryReaction[] - A set of Reactions to this Comment.
- created_at string - The time/date when the Comment was created.
- text string - The text of the Comment.
shortcut: StoryContents
A container entity for the attributes this template should populate.
Fields
- description string? - The description of the story.
- entity_type string? - A string description of this resource.
- labels LabelSlim[]? - An array of labels attached to the story.
- story_type string? - The type of story (feature, bug, chore).
- linked_files LinkedFile[]? - An array of linked files attached to the story.
- name string? - The name of the story.
- epic_id int? - The ID of the epic the story belongs to.
- external_links string[]? - An array of external links connected to the story.
- iteration_id int? - The ID of the iteration the story belongs to.
- tasks StoryContentsTask[]? - An array of tasks connected to the story.
- label_ids int[]? - An array of label ids attached to the story.
- group_id string? - The ID of the group to which the story is assigned.
- workflow_state_id int? - The ID of the workflow state the story is currently in.
- follower_ids string[]? - An array of UUIDs for any Members listed as Followers.
- owner_ids string[]? - An array of UUIDs of the owners of this story.
- estimate int? - The numeric point estimate of the story. Can also be null, which means unestimated.
- files UploadedFile[]? - An array of files attached to the story.
- project_id int? - The ID of the project the story belongs to.
- deadline string? - The due date of the story.
shortcut: StoryContentsTask
Fields
- description string - Full text of the Task.
- position int? - The number corresponding to the Task's position within a list of Tasks on a Story.
- complete boolean? - True/false boolean indicating whether the Task has been completed.
- owner_ids string[]? - An array of UUIDs of the Owners of this Task.
- external_id string? - This field can be set to another unique ID. In the case that the Task has been imported from another tool, the ID in the other tool can be indicated here.
shortcut: StoryHistoryChangeAddsRemovesInt
Task IDs that have been added or removed from the Story.
Fields
- adds int[]? - The values that have been added.
- removes int[]? - The values that have been removed
shortcut: StoryHistoryChangeAddsRemovesUuid
Member IDs that have been added or removed as a owner of the Story.
Fields
- adds string[]? - The values that have been added.
- removes string[]? - The values that have been removed
shortcut: StoryHistoryChangeOldNewBool
True if the Story has archived, otherwise false.
Fields
- old boolean? - The old value.
- 'new boolean? - The new value.
shortcut: StoryHistoryChangeOldNewInt
The estimate value for the Story
Fields
- old int? - The old value.
- 'new int? - The new value.
shortcut: StoryHistoryChangeOldNewStr
A timestamp that represents the Story's deadline.
Fields
- old string? - The old value.
- 'new string? - The new value.
shortcut: StoryHistoryChangeOldNewUuid
The Member ID of the preson who requested the Story.
Fields
- old string? - The old value.
- 'new string? - The new value.
shortcut: StoryLink
Story links allow you create semantic relationships between two stories. Relationship types are relates to, blocks / blocked by, and duplicates / is duplicated by. The format is subject -> link -> object
, or for example "story 5 blocks story 6".
Fields
- entity_type string - A string description of this resource.
- id int - The unique identifier of the Story Link.
- subject_id int - The ID of the subject Story.
- subject_workflow_state_id int - The workflow state of the "subject" story.
- verb string - How the subject Story acts on the object Story. This can be "blocks", "duplicates", or "relates to".
- object_id int - The ID of the object Story.
- created_at string - The time/date when the Story Link was created.
- updated_at string - The time/date when the Story Link was last updated.
shortcut: StoryReaction
Emoji reaction on a comment.
Fields
- emoji string - Emoji text of the reaction.
- permission_ids string[] - Permissions who have reacted with this.
shortcut: StorySearchResults
The results of the Story search query.
Fields
- total int - The total number of matches for the search query. The first 1000 matches can be paged through via the API.
- data Story[] - A list of search results.
- next string - The URL path and query string for the next page of search results.
- cursors string[]? - Cursors
shortcut: StorySlim
StorySlim represents the same resource as a Story, but is more light-weight. For certain fields it provides ids rather than full resources (e.g., comment_ids
and file_ids
) and it also excludes certain aggregate values (e.g., cycle_time
). The description
field can be optionally included. Use the Get Story endpoint to fetch the unabridged payload for a Story.
Fields
- app_url string - The Shortcut application url for the Story.
- description string? - The description of the Story.
- archived boolean - True if the story has been archived or not.
- started boolean - A true/false boolean indicating if the Story has been started.
- story_links TypedStoryLink[] - An array of story links attached to the Story.
- entity_type string - A string description of this resource.
- labels LabelSlim[] - An array of labels attached to the story.
- task_ids int[] - An array of IDs of Tasks attached to the story.
- mention_ids string[] - Deprecated: use member_mention_ids.
- member_mention_ids string[] - An array of Member IDs that have been mentioned in the Story description.
- story_type string - The type of story (feature, bug, chore).
- file_ids int[] - An array of IDs of Files attached to the story.
- num_tasks_completed int - The number of tasks on the story which are complete.
- workflow_id int - The ID of the workflow the story belongs to.
- completed_at_override string - A manual override for the time/date the Story was completed.
- started_at string - The time/date the Story was started.
- completed_at string - The time/date the Story was completed.
- name string - The name of the story.
- completed boolean - A true/false boolean indicating if the Story has been completed.
- blocker boolean - A true/false boolean indicating if the Story is currently a blocker of another story.
- epic_id int - The ID of the epic the story belongs to.
- story_template_id string - The ID of the story template used to create this story, or null if not created using a template.
- external_links string[] - An array of external links (strings) associated with a Story
- previous_iteration_ids int[] - The IDs of the iteration the story belongs to.
- requested_by_id string - The ID of the Member that requested the story.
- iteration_id int - The ID of the iteration the story belongs to.
- label_ids int[] - An array of label ids attached to the story.
- started_at_override string - A manual override for the time/date the Story was started.
- group_id string - The ID of the group associated with the story.
- workflow_state_id int - The ID of the workflow state the story is currently in.
- updated_at string - The time/date the Story was updated.
- group_mention_ids string[] - An array of Group IDs that have been mentioned in the Story description.
- follower_ids string[] - An array of UUIDs for any Members listed as Followers.
- owner_ids string[] - An array of UUIDs of the owners of this story.
- external_id string - This field can be set to another unique ID. In the case that the Story has been imported from another tool, the ID in the other tool can be indicated here.
- id int - The unique ID of the Story.
- lead_time int? - The lead time (in seconds) of this story when complete.
- estimate int - The numeric point estimate of the story. Can also be null, which means unestimated.
- position int - A number representing the position of the story in relation to every other story in the current project.
- blocked boolean - A true/false boolean indicating if the Story is currently blocked.
- project_id int - The ID of the project the story belongs to.
- linked_file_ids int[] - An array of IDs of LinkedFiles attached to the story.
- deadline string - The due date of the story.
- stats StoryStats - The stats object for Stories
- comment_ids int[] - An array of IDs of Comments attached to the story.
- cycle_time int? - The cycle time (in seconds) of this story when complete.
- created_at string - The time/date the Story was created.
- moved_at string - The time/date the Story was last changed workflow-state.
shortcut: StoryStats
The stats object for Stories
Fields
- num_related_documents int - The number of documents related to this Story.
shortcut: Task
Fields
- description string - Full text of the Task.
- entity_type string - A string description of this resource.
- story_id int - The unique identifier of the parent Story.
- mention_ids string[] - Deprecated: use member_mention_ids.
- member_mention_ids string[] - An array of UUIDs of Members mentioned in this Task.
- completed_at string - The time/date the Task was completed.
- updated_at string - The time/date the Task was updated.
- group_mention_ids string[] - An array of UUIDs of Groups mentioned in this Task.
- owner_ids string[] - An array of UUIDs of the Owners of this Task.
- external_id string - This field can be set to another unique ID. In the case that the Task has been imported from another tool, the ID in the other tool can be indicated here.
- id int - The unique ID of the Task.
- position int - The number corresponding to the Task's position within a list of Tasks on a Story.
- complete boolean - True/false boolean indicating whether the Task has been completed.
- created_at string - The time/date the Task was created.
shortcut: ThreadedComment
Comments associated with Epic Discussions.
Fields
- app_url string - The Shortcut application url for the Comment.
- entity_type string - A string description of this resource.
- deleted boolean - True/false boolean indicating whether the Comment is deleted.
- mention_ids string[] - Deprecated: use member_mention_ids.
- author_id string - The unique ID of the Member that authored the Comment.
- member_mention_ids string[] - An array of Member IDs that have been mentioned in this Comment.
- comments ThreadedComment[] - A nested array of threaded comments.
- updated_at string - The time/date the Comment was updated.
- group_mention_ids string[] - An array of Group IDs that have been mentioned in this Comment.
- external_id string - This field can be set to another unique ID. In the case that the Comment has been imported from another tool, the ID in the other tool can be indicated here.
- id int - The unique ID of the Comment.
- created_at string - The time/date the Comment was created.
- text string - The text of the Comment.
shortcut: TypedStoryLink
The type of Story Link. The string can be subject or object.
Fields
- entity_type string - A string description of this resource.
- object_id int - The ID of the object Story.
- verb string - How the subject Story acts on the object Story. This can be "blocks", "duplicates", or "relates to".
- 'type string - This indicates whether the Story is the subject or object in the Story Link.
- updated_at string - The time/date when the Story Link was last updated.
- id int - The unique identifier of the Story Link.
- subject_id int - The ID of the subject Story.
- subject_workflow_state_id int - The workflow state of the "subject" story.
- created_at string - The time/date when the Story Link was created.
shortcut: UnusableEntitlementError
Fields
- reason_tag string - The tag for violating an entitlement action.
- entitlement_tag string - Short tag describing the unusable entitlement action taken by the user.
- message string - Message displayed to the user on why their action failed.
shortcut: UpdateCategory
Fields
- name string? - The new name of the Category.
- color string? - The hex color to be displayed with the Category (for example, "#ff0000").
- archived boolean? - A true/false boolean indicating if the Category has been archived.
shortcut: UpdateComment
Fields
- text string - The updated comment text.
shortcut: UpdateEntityTemplate
Request parameters for changing either a template's name or any of the attributes it is designed to pre-populate.
Fields
- name string? - The updated template name.
- story_contents UpdateStoryContents? - Updated attributes for the template to populate.
shortcut: UpdateEpic
Fields
- description string? - The Epic's description.
- archived boolean? - A true/false boolean indicating whether the Epic is in archived state.
- labels CreateLabelParams[]? - An array of Labels attached to the Epic.
- completed_at_override string? - A manual override for the time/date the Epic was completed.
- name string? - The Epic's name.
- planned_start_date string? - The Epic's planned start date.
- state string? -
Deprecated
The Epic's state (to do, in progress, or done); will be ignored whenepic_state_id
is set.
- milestone_id int? - The ID of the Milestone this Epic is related to.
- requested_by_id string? - The ID of the member that requested the epic.
- epic_state_id int? - The ID of the Epic State.
- started_at_override string? - A manual override for the time/date the Epic was started.
- group_id string? - The ID of the group to associate with the epic.
- follower_ids string[]? - An array of UUIDs for any Members you want to add as Followers on this Epic.
- owner_ids string[]? - An array of UUIDs for any members you want to add as Owners on this Epic.
- before_id int? - The ID of the Epic we want to move this Epic before.
- after_id int? - The ID of the Epic we want to move this Epic after.
- deadline string? - The Epic's deadline.
shortcut: UpdateFile
Fields
- description string? - The description of the file.
- created_at string? - The time/date that the file was uploaded.
- updated_at string? - The time/date that the file was last updated.
- name string? - The name of the file.
- uploader_id string? - The unique ID assigned to the Member who uploaded the file to Shortcut.
- external_id string? - An additional ID that you may wish to assign to the file.
shortcut: UpdateGroup
Fields
- description string? - The description of this Group.
- archived boolean? - Whether or not this Group is archived.
- color string? - The color you wish to use for the Group in the system.
- display_icon_id string? - The Icon id for the avatar of this Group.
- mention_name string? - The mention name of this Group.
- name string? - The name of this Group.
- color_key string? - The color key you wish to use for the Group in the system.
- member_ids string[]? - The Member ids to add to this Group.
- workflow_ids int[]? - The Workflow ids to add to the Group.
shortcut: UpdateIteration
Fields
- follower_ids string[]? - An array of UUIDs for any Members you want to add as Followers.
- group_ids string[]? - An array of UUIDs for any Groups you want to add as Followers. Currently, only one Group association is presented in our web UI.
- labels CreateLabelParams[]? - An array of Labels attached to the Iteration.
- description string? - The description of the Iteration.
- name string? - The name of this Iteration
- start_date string? - The date this Iteration begins, e.g. 2019-07-01
- end_date string? - The date this Iteration ends, e.g. 2019-07-05.
shortcut: UpdateLabel
Fields
- name string? - The new name of the label.
- description string? - The new description of the label.
- color string? - The hex color to be displayed with the Label (for example, "#ff0000").
- archived boolean? - A true/false boolean indicating if the Label has been archived.
shortcut: UpdateLinkedFile
Fields
- description string? - The description of the file.
- story_id int? - The ID of the linked story.
- name string? - The name of the file.
- thumbnail_url string? - The URL of the thumbnail, if the integration provided it.
- 'type string? - The integration type of the file (e.g. google, dropbox, box).
- size int? - The filesize, if the integration provided it.
- uploader_id string? - The UUID of the member that uploaded the file.
- url string? - The URL of linked file.
shortcut: UpdateMilestone
Fields
- description string? - The Milestone's description.
- completed_at_override string? - A manual override for the time/date the Milestone was completed.
- name string? - The name of the Milestone.
- state string? - The workflow state that the Milestone is in.
- started_at_override string? - A manual override for the time/date the Milestone was started.
- categories CreateCategoryParams[]? - An array of IDs of Categories attached to the Milestone.
- before_id int? - The ID of the Milestone we want to move this Milestone before.
- after_id int? - The ID of the Milestone we want to move this Milestone after.
shortcut: UpdateProject
Fields
- description string? - The Project's description.
- archived boolean? - A true/false boolean indicating whether the Story is in archived state.
- days_to_thermometer int? - The number of days before the thermometer appears in the Story summary.
- color string? - The color that represents the Project in the UI.
- name string? - The Project's name.
- follower_ids string[]? - An array of UUIDs for any Members you want to add as Followers.
- show_thermometer boolean? - Configuration to enable or disable thermometers in the Story summary.
- team_id int? - The ID of the team the project belongs to.
- abbreviation string? - The Project abbreviation used in Story summaries. Should be kept to 3 characters at most.
shortcut: UpdateStories
Fields
- archived boolean? - If the Stories should be archived or not.
- story_ids int[] - The Ids of the Stories you wish to update.
- story_type string? - The type of story (feature, bug, chore).
- move_to string? - One of "first" or "last". This can be used to move the given story to the first or last position in the workflow state.
- follower_ids_add string[]? - The UUIDs of the new followers to be added.
- epic_id int? - The ID of the epic the story belongs to.
- external_links string[]? - An array of External Links associated with this story.
- follower_ids_remove string[]? - The UUIDs of the followers to be removed.
- requested_by_id string? - The ID of the member that requested the story.
- iteration_id int? - The ID of the iteration the story belongs to.
- labels_add CreateLabelParams[]? - An array of labels to be added.
- group_id string? - The Id of the Group the Stories should belong to.
- workflow_state_id int? - The ID of the workflow state to put the stories in.
- before_id int? - The ID of the story that the stories are to be moved before.
- estimate int? - The numeric point estimate of the story. Can also be null, which means unestimated.
- after_id int? - The ID of the story that the stories are to be moved below.
- owner_ids_remove string[]? - The UUIDs of the owners to be removed.
- project_id int? - The ID of the Project the Stories should belong to.
- labels_remove CreateLabelParams[]? - An array of labels to be removed.
- deadline string? - The due date of the story.
- owner_ids_add string[]? - The UUIDs of the new owners to be added.
shortcut: UpdateStory
Fields
- description string? - The description of the story.
- archived boolean? - True if the story is archived, otherwise false.
- labels CreateLabelParams[]? - An array of labels attached to the story.
- pull_request_ids int[]? - An array of IDs of Pull/Merge Requests attached to the story.
- story_type string? - The type of story (feature, bug, chore).
- move_to string? - One of "first" or "last". This can be used to move the given story to the first or last position in the workflow state.
- file_ids int[]? - An array of IDs of files attached to the story.
- completed_at_override string? - A manual override for the time/date the Story was completed.
- name string? - The title of the story.
- epic_id int? - The ID of the epic the story belongs to.
- external_links string[]? - An array of External Links associated with this story.
- branch_ids int[]? - An array of IDs of Branches attached to the story.
- commit_ids int[]? - An array of IDs of Commits attached to the story.
- requested_by_id string? - The ID of the member that requested the story.
- iteration_id int? - The ID of the iteration the story belongs to.
- started_at_override string? - A manual override for the time/date the Story was started.
- group_id string? - The ID of the group to associate with this story
- workflow_state_id int? - The ID of the workflow state to put the story in.
- follower_ids string[]? - An array of UUIDs of the followers of this story.
- owner_ids string[]? - An array of UUIDs of the owners of this story.
- before_id int? - The ID of the story we want to move this story before.
- estimate int? - The numeric point estimate of the story. Can also be null, which means unestimated.
- after_id int? - The ID of the story we want to move this story after.
- project_id int? - The ID of the project the story belongs to.
- linked_file_ids int[]? - An array of IDs of linked files attached to the story.
- deadline string? - The due date of the story.
shortcut: UpdateStoryComment
Fields
- text string - The updated comment text.
shortcut: UpdateStoryContents
Updated attributes for the template to populate.
Fields
- description string? - The description of the story.
- entity_type string? - A string description of this resource.
- labels CreateLabelParams[]? - An array of labels to be populated by the template.
- story_type string? - The type of story (feature, bug, chore).
- linked_files LinkedFile[]? - An array of linked files attached to the story.
- file_ids int[]? - An array of the attached file IDs to be populated.
- name string? - The name of the story.
- epic_id int? - The ID of the epic the to be populated.
- external_links string[]? - An array of external links to be populated.
- iteration_id int? - The ID of the iteration the to be populated.
- tasks EntityTemplateTask[]? - An array of tasks to be populated by the template.
- label_ids int[]? - An array of label ids attached to the story.
- group_id string? - The ID of the group to be populated.
- workflow_state_id int? - The ID of the workflow state the story is currently in.
- follower_ids string[]? - An array of UUIDs for any Members listed as Followers.
- owner_ids string[]? - An array of UUIDs of the owners of this story.
- estimate int? - The numeric point estimate to be populated.
- files UploadedFile[]? - An array of files attached to the story.
- project_id int? - The ID of the project the story belongs to.
- linked_file_ids int[]? - An array of the linked file IDs to be populated.
- deadline string? - The due date of the story.
shortcut: UpdateStoryLink
Fields
- verb string? - The type of link.
- subject_id int? - The ID of the subject Story.
- object_id int? - The ID of the object Story.
shortcut: UpdateTask
Fields
- description string? - The Task's description.
- owner_ids string[]? - An array of UUIDs of the owners of this story.
- complete boolean? - A true/false boolean indicating whether the task is complete.
- before_id int? - Move task before this task ID.
- after_id int? - Move task after this task ID.
shortcut: UploadedFile
An UploadedFile is any document uploaded to your Shortcut Workspace. Files attached from a third-party service are different: see the Linked Files endpoint.
Fields
- description string - The description of the file.
- entity_type string - A string description of this resource.
- story_ids int[] - The unique IDs of the Stories associated with this file.
- mention_ids string[] - Deprecated: use member_mention_ids.
- member_mention_ids string[] - The unique IDs of the Members who are mentioned in the file description.
- name string - The optional User-specified name of the file.
- thumbnail_url string - The url where the thumbnail of the file can be found in Shortcut.
- size int - The size of the file.
- uploader_id string - The unique ID of the Member who uploaded the file.
- content_type string - Free form string corresponding to a text or image file.
- updated_at string - The time/date that the file was updated.
- filename string - The name assigned to the file in Shortcut upon upload.
- group_mention_ids string[] - The unique IDs of the Groups who are mentioned in the file description.
- external_id string - This field can be set to another unique ID. In the case that the File has been imported from another tool, the ID in the other tool can be indicated here.
- id int - The unique ID for the file.
- url string - The URL for the file.
- created_at string - The time/date that the file was created.
shortcut: Workflow
Workflow is the array of defined Workflow States. Workflow can be queried using the API but must be updated in the Shortcut UI.
Fields
- description string - A description of the workflow.
- entity_type string - A string description of this resource.
- project_ids decimal[] - An array of IDs of projects within the Workflow.
- states WorkflowState[] - A map of the states in this Workflow.
- name string - The name of the workflow.
- updated_at string - The date the Workflow was updated.
- auto_assign_owner boolean - Indicates if an owner is automatically assigned when an unowned story is started.
- id int - The unique ID of the Workflow.
- team_id int - The ID of the team the workflow belongs to.
- created_at string - The date the Workflow was created.
- default_state_id int - The unique ID of the default state that new Stories are entered into.
shortcut: WorkflowState
Workflow State is any of the at least 3 columns. Workflow States correspond to one of 3 types: Unstarted, Started, or Done.
Fields
- description string - The description of what sort of Stories belong in that Workflow state.
- entity_type string - A string description of this resource.
- color string? - The hex color for this Workflow State.
- verb string - The verb that triggers a move to that Workflow State when making VCS commits.
- name string - The Workflow State's name.
- num_stories int - The number of Stories currently in that Workflow State.
- 'type string - The type of Workflow State (Unstarted, Started, or Finished)
- updated_at string - When the Workflow State was last updated.
- id int - The unique ID of the Workflow State.
- num_story_templates int - The number of Story Templates associated with that Workflow State.
- position int - The position that the Workflow State is in, starting with 0 at the left.
- created_at string - The time/date the Workflow State was created.
Import
import ballerinax/shortcut;
Metadata
Released date: about 1 year ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 0
Current verison: 0
Weekly downloads
Keywords
Productivity/Project Management
Cost/Freemium
Contributors