shopify.admin
Module shopify.admin
API
Definitions
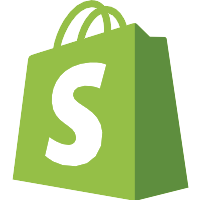
ballerinax/shopify.admin Ballerina library
Overview
This is a generated connector for Shopify Admin API v2021-10 OpenAPI specification. The Admin API lets you build apps and integrations that extend and enhance the Shopify admin. As the primary way that apps and services interact with Shopify, the Admin API allows you to add your own features to the Shopify user experience. Read and write Shopify store information, including products, inventory, orders, shipping, and more.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create a Shopify account
- Obtain tokens by following this guide
Quickstart
To use the Shopify Admin connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/shopify.admin
module into the Ballerina project.
import ballerinax/shopify.admin;
Step 2: Create a new connector instance
Create a admin:ApiKeysConfig
with the Shopify access token obtained, and initialize the connector with it.
admin:ApiKeysConfig config = { xShopifyAccessToken: "<ACCESS_TOKEN>" } admin:Client baseClient = check new (config, "https://<shopify_store_name>.myshopify.com");
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to retrieve a list of categories using the connector.
List Categories
public function main() returns error? { admin:CustomerList response = check baseClient->getCustomers(); log:printInfo(response.toJsonString()); }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
shopify.admin: Client
This is a generated connector for Shopify Admin API v2021-10 OpenAPI specification. The Admin API lets you build apps and integrations that extend and enhance the Shopify admin. As the primary way that apps and services interact with Shopify, the Admin API allows you to add your own features to the Shopify user experience. Read and write Shopify store information, including products, inventory, orders, shipping, and more.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Shopify account and obtain tokens by following this guide.
init (ApiKeysConfig apiKeyConfig, string serviceUrl, ConnectionConfig config)
- apiKeyConfig ApiKeysConfig - API keys for authorization
- serviceUrl string - URL of the target service
- config ConnectionConfig {} - The configurations to be used when initializing the
connector
getCustomers
function getCustomers(string? ids, string? sinceId, string? createdAtMin, string? createdAtMax, string? updatedAtMin, string? updatedAtMax, int? 'limit, string? fields) returns CustomerList|error
Retrieves a list of customers.
Parameters
- ids string? (default ()) - Restrict results to customers specified by a comma-separated list of IDs.
- sinceId string? (default ()) - Restrict results to those after the specified ID.
- createdAtMin string? (default ()) - Show customers created after a specified date.(format: 2014-04-25T16:15:47-04:00)
- createdAtMax string? (default ()) - Show customers created before a specified date.(format: 2014-04-25T16:15:47-04:00)
- updatedAtMin string? (default ()) - Show customers last updated after a specified date.(format: 2014-04-25T16:15:47-04:00)
- updatedAtMax string? (default ()) - Show customers last updated before a specified date.(format: 2014-04-25T16:15:47-04:00)
- 'limit int? (default ()) - The maximum number of results to show.(default: 50, maximum: 250)
- fields string? (default ()) - Show only certain fields, specified by a comma-separated list of field names.
Return Type
- CustomerList|error - List of customers
createCustomer
function createCustomer(CreateCustomer payload) returns CustomerObject|error
Creates a customer.
Parameters
- payload CreateCustomer - The Customer object to be created.
Return Type
- CustomerObject|error - Created customer
getCustomer
function getCustomer(string customerId, string? fields) returns CustomerObject|error
Retrieves a single customer.
Parameters
- customerId string - Customer ID
- fields string? (default ()) - Show only certain fields, specified by a comma-separated list of field names.
Return Type
- CustomerObject|error - Requested customer
updateCustomer
function updateCustomer(string customerId, UpdateCustomer payload) returns CustomerObject|error
Updates a customer.
Parameters
- customerId string - Customer ID
- payload UpdateCustomer - The Customer object to be updated.
Return Type
- CustomerObject|error - Updated customer
searchCustomers
function searchCustomers(string? query, string? 'order, int? 'limit, string? fields) returns CustomerList|error
Searches for customers that match a supplied query.
Parameters
- query string? (default ()) - Text to search for in the shop's customer data.
- 'order string? (default ()) - Set the field and direction by which to order results. (default: last_order_date DESC)
- 'limit int? (default ()) - The maximum number of results to show. (default: 50, maximum: 250)
- fields string? (default ()) - Show only certain fields, specified by a comma-separated list of field names.
Return Type
- CustomerList|error - List of customers
getProducts
function getProducts(string? ids, int? 'limit, string? sinceId, string? title, string? vendor, string? 'handle, string? productType, string? status, string? collectionId, string? createdAtMin, string? createdAtMax, string? updatedAtMin, string? updatedAtMax, string? publishedAtMin, string? publishedAtMax, string? publishedStatus, string? fields, string? presentmentCurrencies) returns ProductList|error
Retrieves a list of products.
Parameters
- ids string? (default ()) - Return only products specified by a comma-separated list of product IDs.
- 'limit int? (default ()) - Return up to this many results per page. (default: 50, maximum: 250)
- sinceId string? (default ()) - Restrict results to after the specified ID.
- title string? (default ()) - Filter results by product title.
- vendor string? (default ()) - Filter results by product vendor.
- 'handle string? (default ()) - Filter results by product handle.
- productType string? (default ()) - Filter results by product type.
- status string? (default ()) - Return products by their status. (default: active) active: Show only active products. archived: Show only archived products. draft: Show only draft products.
- collectionId string? (default ()) - Filter results by product collection ID.
- createdAtMin string? (default ()) - Show products created after date. (format: 2014-04-25T16:15:47-04:00)
- createdAtMax string? (default ()) - Show products created before date. (format: 2014-04-25T16:15:47-04:00)
- updatedAtMin string? (default ()) - Show products last updated after date. (format: 2014-04-25T16:15:47-04:00)
- updatedAtMax string? (default ()) - Show products last updated before date. (format: 2014-04-25T16:15:47-04:00)
- publishedAtMin string? (default ()) - Show products published after date. (format: 2014-04-25T16:15:47-04:00)
- publishedAtMax string? (default ()) - Show products published before date. (format: 2014-04-25T16:15:47-04:00)
- publishedStatus string? (default ()) - Return products by their published status (default: any) published: Show only published products. unpublished: Show only unpublished products. any: Show all products.
- fields string? (default ()) - Show only certain fields, specified by a comma-separated list of field names.
- presentmentCurrencies string? (default ()) - Return presentment prices in only certain currencies, specified by a comma-separated list of ISO 4217 currency codes.
Return Type
- ProductList|error - List of products
createProduct
function createProduct(CreateProduct payload) returns ProductObject|error
Creates a new product.
Parameters
- payload CreateProduct - The Product object to be created.
Return Type
- ProductObject|error - Created product
getProduct
function getProduct(string productId, string? fields) returns ProductObject|error
Retrieves a single product.
Parameters
- productId string - Product ID
- fields string? (default ()) - A comma-separated list of fields to include in the response.
Return Type
- ProductObject|error - Requested product
updateProduct
function updateProduct(string productId, UpdateProduct payload) returns ProductObject|error
Updates a product and its variants and images.
Return Type
- ProductObject|error - Updated product
getProductVariants
function getProductVariants(string productId, string? fields, int? 'limit, string? presentmentCurrencies, string? sinceId) returns ProductVariantList|error
Retrieves a list of product variants.
Parameters
- productId string - Product ID
- fields string? (default ()) - A comma-separated list of fields to include in the response
- 'limit int? (default ()) - Return up to this many results per page
- presentmentCurrencies string? (default ()) - Return presentment prices in only certain currencies, specified by a comma-separated list of ISO 4217 currency codes.
- sinceId string? (default ()) - Restrict results to after the specified ID
Return Type
- ProductVariantList|error - List of product variants
createProductVariant
function createProductVariant(string productId, CreateProductVariant payload) returns ProductVariantObject|error
Creates a new product variant.
Parameters
- productId string - Product ID
- payload CreateProductVariant - The Product variant object to be created.
Return Type
- ProductVariantObject|error - Created product variant
getProductVariant
function getProductVariant(string variantId, string? fields) returns ProductVariantObject|error
Retrieves a single product variant by ID.
Parameters
- variantId string - Variant ID
- fields string? (default ()) - A comma-separated list of fields to include in the response
Return Type
- ProductVariantObject|error - Requested product variant
updateProductVariant
function updateProductVariant(string variantId, UpdateProductVariant payload) returns ProductVariantObject|error
Updates an existing product variant.
Parameters
- variantId string - Variant ID
- payload UpdateProductVariant - The Product variant object to be updated.
Return Type
- ProductVariantObject|error - Updated product variant
getOrders
function getOrders(string? ids, int? 'limit, int? sinceId, string? createdAtMin, string? createdAtMax, string? updatedAtMin, string? updatedAtMax, string? processedAtMin, string? processedAtMax, string? attributionAppId, string? status, string? financialStatus, string? fulfillmentStatus, string? fields) returns OrderList|error
Retrieves a list of orders that meet the specified criteria.
Parameters
- ids string? (default ()) - Retrieve only orders specified by a comma-separated list of order IDs.
- 'limit int? (default ()) - The maximum number of results to show on a page. (default: 50, maximum: 250)
- sinceId int? (default ()) - Show orders after the specified ID.
- createdAtMin string? (default ()) - Show orders created at or after date (format: 2014-04-25T16:15:47-04:00).
- createdAtMax string? (default ()) - Show orders created at or before date (format: 2014-04-25T16:15:47-04:00).
- updatedAtMin string? (default ()) - Show orders last updated at or after date (format: 2014-04-25T16:15:47-04:00).
- updatedAtMax string? (default ()) - Show orders last updated at or before date (format: 2014-04-25T16:15:47-04:00).
- processedAtMin string? (default ()) - Show orders imported at or after date (format: 2014-04-25T16:15:47-04:00).
- processedAtMax string? (default ()) - Show orders imported at or before date (format: 2014-04-25T16:15:47-04:00).
- attributionAppId string? (default ()) - Show orders attributed to a certain app, specified by the app ID. Set as current to show orders for the app currently consuming the API.
- status string? (default ()) - Filter orders by their status. default: open) open: Show only open orders. closed: Show only closed orders. cancelled: Show only canceled orders. any: Show orders of any status, including archived orders.
- financialStatus string? (default ()) - Filter orders by their financial status. (default: any) authorized: Show only authorized orders pending: Show only pending orders paid: Show only paid orders partially_paid: Show only partially paid orders refunded: Show only refunded orders voided: Show only voided orders partially_refunded: Show only partially refunded orders any: Show orders of any financial status. unpaid: Show authorized and partially paid orders.
- fulfillmentStatus string? (default ()) - Filter orders by their fulfillment status. (default: any) shipped: Show orders that have been shipped. Returns orders with fulfillment_status of fulfilled. partial: Show partially shipped orders. unshipped: Show orders that have not yet been shipped. Returns orders with fulfillment_status of null. any: Show orders of any fulfillment status. unfulfilled: Returns orders with fulfillment_status of null or partial.
- fields string? (default ()) - Retrieve only certain fields, specified by a comma-separated list of fields names.
createOrder
function createOrder(CreateOrder payload) returns OrderObject|error
Creates an order. By default, product inventory is not claimed.
Parameters
- payload CreateOrder - The Order object to be created.
Return Type
- OrderObject|error - Created order
getOrder
function getOrder(string orderId, string? fields) returns OrderObject|error
Retrieves a specific order.
Parameters
- orderId string - Order ID
- fields string? (default ()) - A comma-separated list of fields to include in the response.
Return Type
- OrderObject|error - Requested order
updateOrder
function updateOrder(string orderId, UpdateOrder payload) returns OrderObject|error
Updates an existing order.
Return Type
- OrderObject|error - Updated order
getOrderFulfillments
function getOrderFulfillments(string orderId, string? createdAtMax, string? createdAtMin, string? fields, int? 'limit, string? sinceId, string? updatedAtMax, string? updatedAtMin) returns OrderFulfillmentsList|error
Retrieves fulfillments associated with an order.
Parameters
- orderId string - Order ID
- createdAtMax string? (default ()) - Show fulfillments created before date (format: 2014-04-25T16:15:47-04:00).
- createdAtMin string? (default ()) - Show fulfillments created after date (format: 2014-04-25T16:15:47-04:00).
- fields string? (default ()) - A comma-separated list of fields to include in the response.
- 'limit int? (default ()) - Limit the amount of results. (default: 50, maximum: 250)
- sinceId string? (default ()) - Restrict results to after the specified ID.
- updatedAtMax string? (default ()) - Show fulfillments last updated before date (format: 2014-04-25T16:15:47-04:00).
- updatedAtMin string? (default ()) - Show fulfillments last updated after date (format: 2014-04-25T16:15:47-04:00).
Return Type
- OrderFulfillmentsList|error - List of order fulfillments
createOrderFulfillment
function createOrderFulfillment(string orderId, CreateOrderFulfillment payload) returns OrderFulfillmentObject|error
Create a fulfillment for the specified order and line items. The fulfillment's status depends on the line items in the order: If the line items in the fulfillment use a manual or custom fulfillment service, then the status of the returned fulfillment will be set immediately. If the line items use an external fulfillment service, then they will be queued for fulfillment and the status will be set to pending until the external fulfillment service has been invoked. A fulfillment might then transition to open, which implies it is being processed by the service, before transitioning to success when the items have shipped. If you don't specify line item IDs, then all unfulfilled and partially fulfilled line items for the order will be fulfilled. However, if an order is refunded or if any of its individual line items are refunded, then the order can't be fulfilled. All line items being fulfilled must have the same fulfillment service. Note If you are using this endpoint with a Partner development store or a trial store, then you can create no more than 5 new fulfillments per minute. About tracking urls If you're creating a fulfillment for a supported carrier, then you can send the tracking_company and tracking_numbers fields, and Shopify will generate the tracking_url for you. If you're creating a fulfillment for an unsupported carrier (not in the tracking_company list), then send the tracking_company, tracking_numbers, and tracking_urls fields. Note If you send an unsupported carrier without a tracking URL, then Shopify will still try to generate a valid tracking URL by using pattern matching on the tracking number. However, Shopify does not validate the tracking URL, so you should make sure that your tracking URL is correct for the order and fulfillment.
Parameters
- orderId string - Order ID
- payload CreateOrderFulfillment - The Order fulfillment object to be created.
Return Type
- OrderFulfillmentObject|error - Created order fulfillment
createDraftOrder
function createDraftOrder(CreateDraftOrder payload, string? customerId, boolean? useCustomerDefaultAddress) returns DraftOrderObject|error
Creates a draft order.
Parameters
- payload CreateDraftOrder - The Draft order object to be created.
- customerId string? (default ()) - Used to load the customer. When a customer is loaded, the customer’s email address is also associated.
- useCustomerDefaultAddress boolean? (default ()) - An optional boolean that you can send as part of a draft order object to load customer shipping information. Valid values: true or false.
Return Type
- DraftOrderObject|error - Created draft order.
createTransactionForOrder
function createTransactionForOrder(string orderId, CreateTransaction payload, string? 'source) returns TransactionObject|error
Creates a transaction for an order.
Parameters
- orderId string - Order ID
- payload CreateTransaction - The Transaction object to be created.
- 'source string? (default ()) - The origin of the transaction. Set to external to create a cash transaction for the associated order.
Return Type
- TransactionObject|error - Created transaction.
createRefundForOrder
function createRefundForOrder(string orderId, CreateRefund payload) returns RefundObject|error
Creates a refund.
Return Type
- RefundObject|error - Created refund.
getOrderRisks
function getOrderRisks(string orderId) returns OrderRiskList|error
Retrieves a list of all order risks for an order.
Parameters
- orderId string - Order ID
Return Type
- OrderRiskList|error - List of all order risks for an order
createOrderRisk
function createOrderRisk(string orderId, CreateOrderRisk payload) returns OrderRiskObject|error
Creates an order risk for an order.
Return Type
- OrderRiskObject|error - Created order risk
getOrderRisk
function getOrderRisk(string orderId, string riskId) returns OrderRiskObject|error
Retrieves a single order risk by its ID.
Return Type
- OrderRiskObject|error - Requested order risk
updateOrderRisk
function updateOrderRisk(string orderId, string riskId, UpdateOrderRisk payload) returns OrderRiskObject|error
Updates an order risk.
Parameters
- orderId string - Order ID
- riskId string - Order Risk ID
- payload UpdateOrderRisk - The order risk object to be updated.
Return Type
- OrderRiskObject|error - Updated order risk
getWebhooks
function getWebhooks(string? address, string? createdAtMax, string? createdAtMin, string? fields, int? 'limit, string? sinceId, string? topic, string? updatedAtMax, string? updatedAtMin) returns WebhookList|error
Retrieves a list of webhooks.
Parameters
- address string? (default ()) - Retrieve webhook subscriptions that send the POST request to this URI.
- createdAtMax string? (default ()) - Retrieve webhook subscriptions that were created before a given date and time (format: 2014-04-25T16:15:47-04:00).
- createdAtMin string? (default ()) - Retrieve webhook subscriptions that were created after a given date and time (format: 2014-04-25T16:15:47-04:00).
- fields string? (default ()) - Comma-separated list of the properties you want returned for each item in the result list. Use this parameter to restrict the returned list of items to only those properties you specify.
- 'limit int? (default ()) - Maximum number of webhook subscriptions that should be returned. Setting this parameter outside the maximum range will return an error. (default: 50, maximum: 250)
- sinceId string? (default ()) - Restrict the returned list to webhook subscriptions whose id is greater than the specified since_id.
- topic string? (default ()) - Show webhook subscriptions with a given topic. For a list of valid values, refer to the topic property (https://shopify.dev/api/admin-rest/2021-10/resources/webhook).
- updatedAtMax string? (default ()) - Retrieve webhooks that were updated after a given date and time (format: 2014-04-25T16:15:47-04:00).
- updatedAtMin string? (default ()) - Retrieve webhooks that were updated before a given date and time (format: 2014-04-25T16:15:47-04:00).
Return Type
- WebhookList|error - List of all webhook subscriptions for your shop
createWebhook
function createWebhook(CreateWebhook payload) returns WebhookObject|error
Create a new webhook subscription by specifying both an address and a topic.
Parameters
- payload CreateWebhook - The webhook subscription object to be created.
Return Type
- WebhookObject|error - Created webhook subscription
getWebhook
function getWebhook(string webhookId, string? fields) returns WebhookObject|error
Retrieves a single order risk by its ID.
Parameters
- webhookId string - Webhook ID
- fields string? (default ()) - Comma-separated list of the properties you want returned for each item in the result list. Use this parameter to restrict the returned list of items to only those properties you specify.
Return Type
- WebhookObject|error - Requested webhook subscription
updateWebhook
function updateWebhook(string webhookId, UpdateWebhook payload) returns WebhookObject|error
Update a webhook subscription's topic or address URIs.
Return Type
- WebhookObject|error - Updated webhook
getWebhookCount
function getWebhookCount(string? address, string? topic) returns WebhookCountObject|error
Retrieves a count of existing webhook subscriptions. The results can be filtered by address or by topic.
Parameters
- address string? (default ()) - Retrieve webhook subscriptions that send the POST request to this URI.
- topic string? (default ()) - The topic of the webhook subscriptions. For a list of valid values, refer to the topic property (https://shopify.dev/api/admin-rest/2021-10/resources/webhook).
Return Type
- WebhookCountObject|error - Requested webhook subscription
Records
shopify.admin: Address
Address
Fields
- address1 string? - The customer's mailing address.
- address2 string? - An additional field for the customer's mailing address.
- city string? - The customer's city, town, or village.
- company string? - The customer's company.
- country string? - The customer's country.
- country_code string? - The two-letter country code corresponding to the customer's country.
- country_name string? - The customer's normalized country name.
- customer_id int? - A unique identifier for the customer.
- default boolean? - Returns true for each default address.
- first_name string? - The customer's first name.
- id int? - A unique identifier for the address.
- last_name string? - The customer's last name.
- name string? - The customer's first and last names.
- phone string? - The customer's phone number at this address.
- province string? - The customer's region name. Typically a province, a state, or a prefecture.
- province_code string? - The two-letter code for the customer's region.
- zip string? - The customer's postal code, also known as zip, postcode, Eircode, etc.
shopify.admin: AmountSet
The discount amount allocated to the line item in shop and presentment currencies.
Fields
- shop_money Price? - The price object
- presentment_money Price? - The price object
shopify.admin: ApiKeysConfig
Provides API key configurations needed when communicating with a remote HTTP endpoint.
Fields
- xShopifyAccessToken string - Represents API Key
X-Shopify-Access-Token
shopify.admin: AppliedDiscount
The discount applied to the line item or the draft order object. Each draft order object can have one applied_discount object and each draft order line item can have its own applied_discount.
Fields
- title string? - Title of the discount.
- description string? - Reason for the discount.
- value string? - The value of the discount. If the type of discount is fixed_amount, then it corresponds to a fixed dollar amount. If the type is percentage, then it corresponds to percentage.
- value_type string? - The type of discount. Valid values are percentage, fixed_amount.
- amount string? - The applied amount of the discount, based on the setting of value_type. For more information, see Applying discounts.
shopify.admin: ClientDetails
Information about the browser that the customer used when they placed their order
Fields
- accept_language string? - The languages and locales that the browser understands.
- browser_height int? - The browser screen height in pixels, if available.
- browser_ip string? - The browser IP address.
- browser_width int? - The browser screen width in pixels, if available.
- session_hash string? - A hash of the session.
- user_agent string? - Details of the browsing client, including software and operating versions.
shopify.admin: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
shopify.admin: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
shopify.admin: CreateCustomer
The Customer object to be created.
Fields
- customer Customer? - The Customer resource stores information about a shop's customers, such as their contact details, their order history, and whether they've agreed to receive email marketing.
shopify.admin: CreateDraftOrder
The Draft order object to be created.
Fields
- draft_order DraftOrder? - An order is a customer's request to purchase one or more products from a shop. You can create, retrieve, update, and delete orders using the Order resource.
shopify.admin: CreateOrder
The Order object to be created.
Fields
- 'order Order? - An order is a customer's request to purchase one or more products from a shop. You can create, retrieve, update, and delete orders using the Order resource.
shopify.admin: CreateOrderFulfillment
The Order fulfillment object to be created.
Fields
- fulfillment Fulfillment? - You can use the Fulfillment resource to view, create, modify, or delete an order's or fulfillment order's fulfillments. A fulfillment order represents a group of one or more items in an order that are to be fulfilled from the same location. A fulfillment represents work that is completed as part of a fulfillment order and can include one or more items. You can use the Fulfillment resource to manage fulfillments for both orders and fulfillment orders.
shopify.admin: CreateOrderRisk
The order risk object to be created.
Fields
- risk OrderRisk? - The order risk for an order.
shopify.admin: CreateProduct
Fields
- product Product? - The Product resource lets you update and create products in a merchant's store.
shopify.admin: CreateProductVariant
Fields
- variant ProductVariant? - A variant can be added to a Product resource to represent one version of a product with several options. The Product resource will have a variant for every possible combination of its options. Each product can have a maximum of three options and a maximum of 100 variants.
shopify.admin: CreateRefund
The Refund object to be created.
Fields
- refund RefundRequest? - Schedule associated to the payment terms
shopify.admin: CreateTransaction
The Transaction object to be created.
Fields
- 'transaction Transaction? - Transactions are created for every order that results in an exchange of money. There are five types of transactions. (Authorization: An amount reserved against the cardholder's funding source. Money does not change hands until the authorization is captured, Sale: An authorization and capture performed together in a single step, Capture: A transfer of the money that was reserved during the authorization stage, Void: A cancellation of a pending authorization or capture, Refund: A partial or full return of captured funds to the cardholder. A refund can happen only after a capture is processed.)
shopify.admin: CreateWebhook
The webhook object to be created.
Fields
- webhook Webhook? - The webhook resource.
shopify.admin: CurrencyExchangeAdjustment
An adjustment on the transaction showing the amount lost or gained due to fluctuations in the currency exchange rate. Requires the header X-Shopify-Api-Features = include-currency-exchange-adjustments.
Fields
- ID string? - The ID of the adjustment.
- adjustment string? - The difference between the amounts on the associated transaction and the parent transaction.
- original_amount string? - The amount of the parent transaction in the shop currency.
- final_amount string? - The amount of the associated transaction in the shop currency.
- currency string? - The shop currency.
shopify.admin: CurrentSubtotalPriceSet
The current subtotal price of the order in shop and presentment currencies. The amount values associated with this field reflect order edits, returns, and refunds.
Fields
- shop_money Price? - The price object
- presentment_money Price? - The price object
shopify.admin: CurrentTotalDiscountsSet
The current total discounts on the order in shop and presentment currencies. The amount values associated with this field reflect order edits, returns, and refunds.
Fields
- shop_money Price? - The price object
- presentment_money Price? - The price object
shopify.admin: CurrentTotalDutiesSet
The current total duties charged on the order in shop and presentment currencies. The amount values associated with this field reflect order edits, returns, and refunds.
Fields
- shop_money Price? - The price object
- presentment_money Price? - The price object
shopify.admin: CurrentTotalPriceSet
The current total price of the order in shop and presentment currencies. The amount values associated with this field reflect order edits, returns, and refunds.
Fields
- shop_money Price? - The price object
- presentment_money Price? - The price object
shopify.admin: CurrentTotalTaxSet
The current total taxes charged on the order in shop and presentment currencies. The amount values associated with this field reflect order edits, returns, and refunds.
Fields
- shop_money Price? - The price object
- presentment_money Price? - The price object
shopify.admin: Customer
The Customer resource stores information about a shop's customers, such as their contact details, their order history, and whether they've agreed to receive email marketing.
Fields
- accepts_marketing boolean? - Whether the customer has consented to receive marketing material via email.
- accepts_marketing_updated_at string? - The date and time (ISO 8601 format) when the customer consented or objected to receiving marketing material by email. Set this value whenever the customer consents or objects to marketing materials.
- addresses Address[]? - A list of the ten most recently updated addresses for the customer.
- currency string? - The three-letter code (ISO 4217 format) for the currency that the customer used when they paid for their last order. Defaults to the shop currency. Returns the shop currency for test orders.
- created_at string? - The date and time (ISO 8601 format) when the customer was created.
- first_name string? - The customer's first name.
- email string? - The unique email address of the customer. Attempting to assign the same email address to multiple customers returns an error.
- default_address Address? - Address
- id int? - A unique identifier for the customer.
- last_name string? - The customer's last name.
- last_order_id int? - The ID of the customer's last order.
- last_order_name string? - The name of the customer's last order. This is directly related to the name field on the Order resource.
- metafield Metafield? - Attaches additional metadata to a shop's resources
- marketing_opt_in_level string? - The marketing subscription opt-in level (as described by the M3AAWG best practices guideline) that the customer gave when they consented to receive marketing material by email. If the customer does not accept email marketing, then this property will be set to null.
- multipass_identifier string? - A unique identifier for the customer that's used with Multipass login.
- note string? - A note about the customer.
- orders_count int? - The number of orders associated with this customer.
- phone string? - The unique phone number (E.164 format) for this customer. Attempting to assign the same phone number to multiple customers returns an error. The property can be set using different formats, but each format must represent a number that can be dialed from anywhere in the world.
- sms_marketing_consent SmsMarketingConsent? - The marketing consent information when the customer consented to receiving marketing material by SMS. The phone property is required to create a customer with SMS consent information and to perform an SMS update on a customer that doesn't have a phone number recorded. The customer must have a unique phone number associated to the record.
- state string? - The state of the customer's account with a shop. Default value is disabled.
- tags string? - Tags that the shop owner has attached to the customer, formatted as a string of comma-separated values. A customer can have up to 250 tags. Each tag can have up to 255 characters.
- tax_exempt boolean? - Whether the customer is exempt from paying taxes on their order. If true, then taxes won't be applied to an order at checkout. If false, then taxes will be applied at checkout.
- tax_exemptions string[]? - Whether the customer is exempt from paying specific taxes on their order. Canadian taxes only.
- total_spent string? - The total amount of money that the customer has spent across their order history.
- updated_at string? - The date and time (ISO 8601 format) when the customer information was last updated.
- verified_email boolean? - Whether the customer has verified their email address.
shopify.admin: CustomerAddress
The mailing address associated with the payment method. This address is an optional field that won't be available on orders that do not require a payment method.
Fields
- address1 string? - The street address of the billing address.
- address2 string? - An optional additional field for the street address of the billing address.
- city string? - The city, town, or village of the billing address.
- company string? - The company of the person associated with the billing address.
- country string? - The name of the country of the billing address.
- country_code string? - The two-letter code (ISO 3166-1 format) for the country of the billing address.
- first_name string? - The first name of the person associated with the payment method.
- last_name string? - The last name of the person associated with the payment method.
- latitude string? - The latitude of the billing address.
- longitude string? - The longitude of the billing address.
- name string? - The full name of the person associated with the payment method.
- phone string? - The phone number at the billing address.
- province string? - The name of the region (for example, province, state, or prefecture) of the billing address.
- province_code string? - The two-letter abbreviation of the region of the billing address.
- zip string? - The postal code (for example, zip, postcode, or Eircode) of the billing address.
shopify.admin: CustomerList
A list of customers
Fields
- customers Customer[]? - A list of customers
shopify.admin: CustomerObject
The Customer object.
Fields
- customer Customer? - The Customer resource stores information about a shop's customers, such as their contact details, their order history, and whether they've agreed to receive email marketing.
shopify.admin: DiscountAllocations
An ordered list of amounts allocated by discount applications. Each discount allocation is associated with a particular discount application.
Fields
- amount string? - The discount amount allocated to the line in the shop currency.
- discount_application_index int? - The index of the associated discount application in the order's
- amount_set AmountSet? - The discount amount allocated to the line item in shop and presentment currencies.
shopify.admin: DiscountApplication
Stacked discount application
Fields
- allocation_method string? - The method by which the discount application value has been allocated to entitled lines. = ['across', 'each', 'one']
- code string? - The discount code that was used to apply the discount.Available only for discount code applications
- description string? - The description of the discount application, as defined by the merchant or the Shopify Script. Available only for manual and script discount applications
- target_selection string? - The lines on the order, of the type defined by target_type, that the discount is allocated over = ['all', 'entitled', 'explicit']
- target_type string? - The type of line on the order that the discount is applicable on = ['line_item', 'shipping_line']
- title string? - The title of the discount application, as defined by the merchant. Available only for manual discount applications
- 'type string? - The discount application type.Valid values:manual The discount was manually applied by the merchant (for example, by using an app or creating a draft order).script: The discount was applied by a Shopify Script.discount_code: The discount was applied by a discount code. = ['discount_code', 'manual', 'script']
- value string? - The value of the discount application as a decimal. This represents the intention of the discount application
- value_type string? - The type of the value = ['percentage', 'fixed_amount']
shopify.admin: DiscountCode
Discounts applied to the order
Fields
- amount string? - The value of the discount deducted from the order total. The type field determines how this value is calculated
- code string? - The discount code
- 'type string? - The type of discount. Can be one of: percentage, shipping, fixed_amount (default) = ['fixed_amount', 'percentage', 'shipping']
shopify.admin: DiscountedPriceSet
The price of the shipping method in both shop and presentment currencies after line-level discounts have been applied.
Fields
- shop_money Price? - The price object
- presentment_money Price? - The price object
shopify.admin: DraftOrder
An order is a customer's request to purchase one or more products from a shop. You can create, retrieve, update, and delete orders using the Order resource.
Fields
- id int? - The ID of the draft order.
- order_id DraftOrderID? - The ID of the order that 's created and associated with the draft order after the draft order is completed.
- name string? - Name of the draft order.
- customer Customer? - The Customer resource stores information about a shop's customers, such as their contact details, their order history, and whether they've agreed to receive email marketing.
- shipping_address CustomerAddress? - The mailing address associated with the payment method. This address is an optional field that won't be available on orders that do not require a payment method.
- billing_address CustomerAddress? - The mailing address associated with the payment method. This address is an optional field that won't be available on orders that do not require a payment method.
- note string? - The text of an optional note that a shop owner can attach to the draft order.
- note_attributes NoteAttribute[]? - Extra information that is added to the order. Appears in the Additional details section of an order details page. Each array entry must contain a hash with name and value keys.
- email string? - The customer's email address.
- currency string? - The three letter code (ISO 4217 format) for the currency used for the payment.
- invoice_sent_at string? - The date and time (ISO 8601 format) when the invoice was emailed to the customer.
- invoice_url string? - The URL for the invoice.
- line_items LineItem[]? - Product variant line item or custom line item associated to the draft order. Each draft order must include at least one line_item.
- payment_terms PaymentTerms? - The terms and conditions under which a payment should be processed.
- shipping_line DraftOrderShippingLine? - A shipping_line object, which details the shipping method used.
- tags string? - A comma-seperated list of additional short descriptors, commonly used for filtering and searching. Each individual tag is limited to 40 characters in length. For example, tags are "tag1","tag2","tag3".
- tax_exempt boolean? - Whether taxes are exempt for the draft order. If set to false, then Shopify refers to the taxable field for each line_item. If a customer is applied to the draft order, then Shopify uses the customer's tax_exempt field instead.
- tax_exemptions string[]? - Whether the customer is exempt from paying specific taxes on their order. Canadian taxes only.
- tax_lines TaxLine[]? - An array of tax line objects, each of which details a tax applicable to the order. When creating an order through the API, tax lines can be specified on the order or the line items but not both. Tax lines specified on the order are split across the taxable line items in the created order.
- applied_discount AppliedDiscount? - The discount applied to the line item or the draft order object. Each draft order object can have one applied_discount object and each draft order line item can have its own applied_discount.
- taxes_included boolean? - Whether taxes are included in the order subtotal. Valid values are true or false.
- total_tax string? - The sum of all the taxes applied to the order.
- subtotal_price string? - The price of the order before shipping and taxes.
- total_price string? - The sum of all the prices of all the items in the order, taxes and discounts included.
- completed_at string? - The date and time (ISO 8601 format) when the order is created and the draft order is completed.
- created_at string? - The date and time (ISO 8601 format) when the order was created in Shopify.
- updated_at string? - The date and time (ISO 8601 format) when the order was last modified.
- status string? - The status of a draft order as it transitions into an order. When a draft order is created it is set to open status. The invoice can then be sent to the customer, and status changes to invoice_sent. The draft order can then be paid, set to pending, or paid by credit card. In each case, the draft order is set to completed and an order is created. After a draft order is set to completed the only further modifications that can be made are adding tags or metafields.
shopify.admin: DraftOrderID
The ID of the order that 's created and associated with the draft order after the draft order is completed.
Fields
- id int? - The ID of the order that 's created and associated with the draft order after the draft order is completed.
shopify.admin: DraftOrderObject
The Draft order object.
Fields
- draft_order DraftOrder? - An order is a customer's request to purchase one or more products from a shop. You can create, retrieve, update, and delete orders using the Order resource.
shopify.admin: DraftOrderShippingLine
A shipping_line object, which details the shipping method used.
Fields
- 'handle string? - The handle of the shipping rate which was selected and applied. Required for regular shipping lines.
- title string? - The title of the shipping method. Required for custom shipping lines. (maximum 255 characters)
- price decimal? - The price of the shipping method. Required for custom shipping lines.
shopify.admin: ExtendedAuthorizationAttributes
The attributes associated with a Shopify Payments extended authorization period.
Fields
- standard_authorization_expires_at string? - The date and time (ISO 8601 format) when the standard authorization period expires. After expiry, an extended authorization fee is applied upon capturing the payment.
- extended_authorization_expires_at string? - The date and time (ISO 8601 format) when the extended authorization period expires. After expiry, the merchant can't capture the payment.
shopify.admin: Fulfillment
You can use the Fulfillment resource to view, create, modify, or delete an order's or fulfillment order's fulfillments. A fulfillment order represents a group of one or more items in an order that are to be fulfilled from the same location. A fulfillment represents work that is completed as part of a fulfillment order and can include one or more items. You can use the Fulfillment resource to manage fulfillments for both orders and fulfillment orders.
Fields
- created_at string? - The date and time when the fulfillment was created. The API returns this value in ISO 8601 format
- id int? - The ID for the fulfillment.
- line_items LineItem[]? - A historical record of each item in the fulfillment.
- location_id int? - The unique identifier of the location that the fulfillment should be processed for. To find the ID of the location, use the Location resource.
- name string? - The uniquely identifying fulfillment name, consisting of two parts separated by a .. The first part represents the order name and the second part represents the fulfillment number. The fulfillment number automatically increments depending on how many fulfillments are in an order (e.g. #1001.1, #1001.2).
- notify_customer string? - Whether the customer should be notified. If set to true, then an email will be sent when the fulfillment is created or updated. For orders that were initially created using the API, the default value is false. For all other orders, the default value is true.
- order_id int? - The unique numeric identifier for the order.
- origin_address Address? - Address
- receipt Receipt? - A text field that provides information about the receipt
- 'service string? - The type of service used.
- shipment_status string? - The current shipment status of the fulfillment.
- status string? - The status of the fulfillment.
- tracking_company string? - The name of the tracking company.
- tracking_numbers string[]? - A list of tracking numbers, provided by the shipping company.
- tracking_urls string[]? - The URLs of tracking pages for the fulfillment.
- updated_at string? - The date and time (ISO 8601 format) when the fulfillment was last modified.
- variant_inventory_management string? - The name of the inventory management service.
shopify.admin: LineItem
A historical record of each item in the fulfillment.
Fields
- id int? - The ID of the line item within the fulfillment.
- variant_id int? - The ID of the product variant being fulfilled.
- title string? - The title of the product.
- quantity int? - The number of items in the fulfillment.
- price string? - The price of the item.
- price_set PriceSet? - The price of the line item in shop and presentment currencies.
- grams int? - The weight of the item in grams.
- sku string? - The unique identifier of the item in the fulfillment.
- variant_title string? - The title of the product variant being fulfilled.
- vendor string? - The name of the supplier of the item.
- fulfillment_service string? - The service provider who is doing the fulfillment.
- product_id int? - The unique numeric identifier for the product in the fulfillment.
- requires_shipping boolean? - Whether a customer needs to provide a shipping address when placing an order for this product variant.
- taxable boolean? - Whether the line item is taxable.
- gift_card boolean? - Whether the line item is a gift card
- name string? - The name of the product variant.
- variant_inventory_management string? - The name of the inventory management system.
- properties Property[]? - Any additional properties associated with the line item.
- product_exists boolean? - Whether the product exists.
- fulfillable_quantity int? - The amount available to fulfill. This is the quantity - max (refunded_quantity, fulfilled_quantity) - pending_fulfilled_quantity - open_fulfilled_quantity.
- total_discount string? - The total of any discounts applied to the line item.
- fulfillment_status string? - The status of an order in terms of the line items being fulfilled. Valid values are fulfilled, null, or partial
- fulfillment_line_item_id int? - A unique identifier for a quantity of items within a single fulfillment. An order can have multiple fulfillment line items.
- tax_lines TaxLine[]? - A list of tax line objects, each of which details a tax applied to the item.
- tip_payment_gateway string? - The payment gateway used to tender the tip, such as shopify_payments. Present only on tips
- tip_payment_method string? - The payment method used to tender the tip, such as Visa. Present only on tips.
- total_discount_set TotalDiscountSet? - The total amount allocated to the line item in the presentment currency. Instead of using this field, Shopify recommends using discount_allocations, which provides the same information.
- discount_allocations DiscountAllocations[]? - An ordered list of amounts allocated by discount applications. Each discount allocation is associated with a particular discount application.
- origin_location Address? - Address
shopify.admin: LocationID
The ID of the physical location where the transaction was processed.
Fields
- id int? - The ID of the physical location where the transaction was processed.
shopify.admin: Metafield
Attaches additional metadata to a shop's resources
Fields
- 'key string - An identifier for the metafield (maximum of 30 characters).
- namespace string - A container for a set of metadata (maximum of 20 characters). Namespaces help distinguish between metadata that you created and metadata created by another individual with a similar namespace.
- value string - Information to be stored as metadata.
- value_type string - The value type. Valid values are string and integer.
- description string? - Additional information about the metafield.
shopify.admin: NoteAttribute
Extra information that is added to the order. Appears in the Additional details section of an order details page. Each array entry must contain a hash with name and value keys.
Fields
- name string? - Name
- value string? - Value
shopify.admin: Option
The custom properties that a shop owner uses to define product variants. You can define three options for a product variant are option1, option2, option3. Default value is Default Title. The title field is a concatenation of the option1, option2, and option3 fields. Updating the option fields updates the title field.
Fields
- option1 string? - Option 1
- option2 string? - Option 2
- option3 string? - Option 3
shopify.admin: Order
An order is a customer's request to purchase one or more products from a shop. You can create, retrieve, update, and delete orders using the Order resource.
Fields
- app_id int? - The ID of the app that created the order.
- billing_address CustomerAddress? - The mailing address associated with the payment method. This address is an optional field that won't be available on orders that do not require a payment method.
- browser_ip string? - The IP address of the browser used by the customer when they placed the order. Both IPv4 and IPv6 are supported.
- buyer_accepts_marketing boolean? - Whether the customer consented to receive email updates from the shop.
- cancel_reason string? - The reason why the order was canceled.
- cancelled_at string? - The date and time when the order was canceled. Returns null if the order isn't canceled.
- cart_token string? - A unique value when referencing the cart that's associated with the order.
- checkout_token string? - A unique value when referencing the checkout that's associated with the order.
- client_details ClientDetails? - Information about the browser that the customer used when they placed their order
- closed_at string? - The date and time (ISO 8601 format) when the order was closed. Returns null if the order isn't closed.
- created_at string? - The autogenerated date and time (ISO 8601 format) when the order was created in Shopify. The value for this property cannot be changed.
- currency string? - The three-letter code (ISO 4217 format) for the shop currency.
- current_total_discounts string? - The current total discounts on the order in the shop currency. The value of this field reflects order edits, returns, and refunds.
- current_total_discounts_set CurrentTotalDiscountsSet? - The current total discounts on the order in shop and presentment currencies. The amount values associated with this field reflect order edits, returns, and refunds.
- current_total_duties_set CurrentTotalDutiesSet? - The current total duties charged on the order in shop and presentment currencies. The amount values associated with this field reflect order edits, returns, and refunds.
- current_total_price string? - The current total price of the order in the shop currency. The value of this field reflects order edits, returns, and refunds.
- current_total_price_set CurrentTotalPriceSet? - The current total price of the order in shop and presentment currencies. The amount values associated with this field reflect order edits, returns, and refunds.
- current_subtotal_price string? - The current subtotal price of the order in the shop currency. The value of this field reflects order edits, returns, and refunds.
- current_subtotal_price_set CurrentSubtotalPriceSet? - The current subtotal price of the order in shop and presentment currencies. The amount values associated with this field reflect order edits, returns, and refunds.
- current_total_tax string? - The current total taxes charged on the order in the shop currency. The value of this field reflects order edits, returns, or refunds.
- current_total_tax_set CurrentTotalTaxSet? - The current total taxes charged on the order in shop and presentment currencies. The amount values associated with this field reflect order edits, returns, and refunds.
- customer Customer? - The Customer resource stores information about a shop's customers, such as their contact details, their order history, and whether they've agreed to receive email marketing.
- customer_locale string? - The two or three-letter language code, optionally followed by a region modifier.
- discount_applications DiscountApplication[]? - An ordered list of stacked discount applications.
- discount_codes DiscountCode[]? - A list of discounts applied to the order.
- email string? - The customer's email address.
- estimated_taxes boolean? - Whether taxes on the order are estimated. Many factors can change between the time a customer places an order and the time the order is shipped, which could affect the calculation of taxes. This property returns false when taxes on the order are finalized and aren't subject to any changes.
- financial_status string? - The status of payments associated with the order. Can only be set when the order is created.
- fulfillments Fulfillment[]? - An array of fulfillments associated with the order. For more information, see the Fulfillment API.
- fulfillment_status string? - The order's status in terms of fulfilled line items.
- gateway string? - The payment gateway used.
- id int? - The ID of the order, used for API purposes. This is different from the order_number property, which is the ID used by the shop owner and customer.'
- landing_site string? - The URL for the page where the buyer landed when they entered the shop.
- line_items LineItem[]? - A list of line item objects, each containing information about an item in the order.
- location_id int? - The ID of the physical location where the order was processed. If you need to reference the location against an order, then use the FulfillmentOrder resource.
- name string? - The order name, generated by combining the order_number property with the order prefix and suffix that are set in the merchant's general settings. This is different from the id property, which is the ID of the order used by the API. This field can also be set by the API to be any string value.
- note string? - An optional note that a shop owner can attach to the order.
- note_attributes NoteAttribute[]? - Extra information that is added to the order. Appears in the Additional details section of an order details page. Each array entry must contain a hash with name and value keys.
- number int? - The order's position in the shop's count of orders. Numbers are sequential and start at 1.
- order_number int? - The order 's position in the shop's count of orders starting at 1001. Order numbers are sequential and start at 1001.
- original_total_duties_set OriginalTotalDutiesSet? - The original total duties charged on the order in shop and presentment currencies.
- payment_details string? - An object containing information about the payment.
- payment_terms PaymentTerms? - The terms and conditions under which a payment should be processed.
- payment_gateway_names string[]? - The list of payment gateways used for the order.
- phone string? - The customer's phone number for receiving SMS notifications.
- presentment_currency string? - The presentment currency that was used to display prices to the customer.
- processed_at string? - The date and time (ISO 8601 format) when an order was processed. This value is the date that appears on your orders and that's used in the analytic reports. If you're importing orders from an app or another platform, then you can set processed_at to a date and time in the past to match when the original order was created.
- processing_method string? - How the payment was processed. It has the following valid values
- referring_site string? - The website where the customer clicked a link to the shop.
- refunds Refund[]? - A list of refunds applied to the order. For more information, see the Refund API.
- shipping_address CustomerAddress? - The mailing address associated with the payment method. This address is an optional field that won't be available on orders that do not require a payment method.
- shipping_lines ShippingLine[]? - An array of objects, each of which details a shipping method used.
- source_name string? - Where the order originated. Can be set only during order creation, and is not writeable afterwards. Values for Shopify channels are protected and cannot be assigned by other API clients: web, pos, shopify_draft_order, iphone, and android. Orders created via the API can be assigned any other string of your choice. If unspecified, then new orders are assigned the value of your app's ID.
- subtotal_price string? - The price of the order in the shop currency after discounts but before shipping, duties, taxes, and tips.
- subtotal_price_set SubtotalPriceSet? - The subtotal of the order in shop and presentment currencies after discounts but before shipping, duties, taxes, and tips.
- tags string? - Tags attached to the order, formatted as a string of comma-separated values. Tags are additional short descriptors, commonly used for filtering and searching. Each individual tag is limited to 40 characters in length.
- tax_lines TaxLine[]? - An array of tax line objects, each of which details a tax applicable to the order. When creating an order through the API, tax lines can be specified on the order or the line items but not both. Tax lines specified on the order are split across the taxable line items in the created order.
- taxes_included boolean? - Whether taxes are included in the order subtotal.
- test boolean? - Whether this is a test order.
- token string? - A unique value when referencing the order.
- total_discounts string? - The total discounts applied to the price of the order in the shop currency.
- total_discounts_set TotalDiscountsSet? - The total discounts applied to the price of the order in shop and presentment currencies.
- total_line_items_price string? - The sum of all line item prices in the shop currency.
- total_line_items_price_set TotalLineItemsPriceSet? - The total of all line item prices in shop and presentment currencies.
- total_outstanding string? - The total outstanding amount of the order in the shop currency.
- total_price string? - The sum of all line item prices, discounts, shipping, taxes, and tips in the shop currency. Must be positive.
- total_price_set TotalPriceSet? - The total price of the order in shop and presentment currencies.
- total_shipping_price_set TotalShippingPriceSet? - The total shipping price of the order, excluding discounts and returns, in shop and presentment currencies. If taxes_included is set to true, then total_shipping_price_set includes taxes.
- total_tax string? - The sum of all the taxes applied to the order in the shop currency. Must be positive.
- total_tax_set TotalTaxSet? - The total tax applied to the order in shop and presentment currencies.
- total_tip_received string? - The sum of all the tips in the order in the shop currency.
- total_weight decimal? - The sum of all line item weights in grams. The sum is not adjusted as items are removed from the order.
- updated_at string? - The date and time (ISO 8601 format) when the order was last modified. Filtering orders by updated_at is not an effective method for fetching orders because its value can change when no visible fields of an order have been updated. Use the Webhook and Event APIs to subscribe to order events instead.
- user_id int? - The ID of the user logged into Shopify POS who processed the order, if applicable.
- order_status_url string? - The URL pointing to the order status web page, if applicable.
shopify.admin: OrderAdjustment
Order adjustment attached to the refund.
Fields
- id int? - The unique identifier for the order adjustment.
- order_id int? - The unique identifier for the order that the order adjustment is associated with.
- refund_id int? - The unique identifier for the refund that the order adjustment is associated with.
- amount int? - The value of the discrepancy between the calculated refund and the actual refund. If the kind property's value is shipping_refund, then amount returns the value of shipping charges refunded to the customer.
- tax_amount string? - The taxes that are added to amount, such as applicable shipping taxes added to a shipping refund.
- kind string? - The order adjustment type. Valid values are shipping_refund and refund_discrepancy.
- reason string? - The reason for the order adjustment. To set this value, include discrepancy_reason when you create a refund.
shopify.admin: OrderFulfillmentObject
The Order fulfillment object.
Fields
- fulfillment Fulfillment? - You can use the Fulfillment resource to view, create, modify, or delete an order's or fulfillment order's fulfillments. A fulfillment order represents a group of one or more items in an order that are to be fulfilled from the same location. A fulfillment represents work that is completed as part of a fulfillment order and can include one or more items. You can use the Fulfillment resource to manage fulfillments for both orders and fulfillment orders.
shopify.admin: OrderFulfillmentsList
A list of order fulfillments
Fields
- fulfillments Fulfillment[]? - A list of order fulfillments
shopify.admin: OrderList
A list of orders
Fields
- orders Order[]? - A list of orders
shopify.admin: OrderObject
Fields
- 'order Order? - An order is a customer's request to purchase one or more products from a shop. You can create, retrieve, update, and delete orders using the Order resource.
shopify.admin: OrderRisk
The order risk for an order.
Fields
- cause_cancel boolean? - Whether this order risk is severe enough to force the cancellation of the order. If true, then this order risk is included in the Order canceled message that's shown on the details page of the canceled order.
- checkout_id int? - The ID of the checkout that the order risk belongs to.
- display boolean? - Whether the order risk is displayed on the order details page in the Shopify admin. If false, then this order risk is ignored when Shopify determines your app's overall risk level for the order.
- id int? - A unique numeric identifier for the order risk.
- merchant_message string? - The message that's displayed to the merchant to indicate the results of the fraud check. The message is displayed only if display is set to true.
- message string? - The message that's displayed to the merchant to indicate the results of the fraud check. The message is displayed only if display is set to true.
- order_id int? - The ID of the order that the order risk belongs to.
- recommendation string? - The recommended action given to the merchant. Valid values are,
cancel
- There is a high level of risk that this order is fraudulent. The merchant should cancel the order.investigate
- There is a medium level of risk that this order is fraudulent. The merchant should investigate the order.accept
- There is a low level of risk that this order is fraudulent. The order risk found no indication of fraud.
- score string? - A number between 0 and 1 that's assigned to the order. The closer the score is to 1, the more likely it is that the order is fraudulent.
- 'source string? - The source of the order risk.
shopify.admin: OrderRiskList
The object representation of a list of order risks
Fields
- risks OrderRisk[]? - A list of order risks
shopify.admin: OrderRiskObject
The order risk object for an order.
Fields
- risk OrderRisk? - The order risk for an order.
shopify.admin: OriginalTotalDutiesSet
The original total duties charged on the order in shop and presentment currencies.
Fields
- shop_money Price? - The price object
- presentment_money Price? - The price object
shopify.admin: PaymentDetails
Information about the credit card used for this transaction.
Fields
- credit_card_bin string? - The issuer identification number (IIN), formerly known as bank identification number (BIN) of the customer's credit card. This is made up of the first few digits of the credit card number.
- avs_result_code string? - The response code from the address verification system. The code is always a single letter.
- cvv_result_code string? - The response code from the credit card company indicating whether the customer entered the card security code, or card verification value, correctly. The code is a single letter or empty string.
- credit_card_number string? - The customer's credit card number, with most of the leading digits redacted.
- credit_card_company string? - The name of the company that issued the customer's credit card.
shopify.admin: PaymentSchedule
Schedule associated to the payment terms
Fields
- amount int? - The amount that is owed according to the payment terms.
- currency string? - The presentment currency for the payment.
- issued_at string? - The date and time when the payment terms were initiated.
- due_at string? - The date and time when the payment is due. Calculated based on issued_at and due_in_days or a customized fixed date if the type is fixed.
- completed_at string? - The date and time when the purchase is completed. Returns null initially and updates when the payment is captured.
- expected_payment_method string? - The name of the payment method gateway.
shopify.admin: PaymentsRefundAttributes
The attributes associated with a Shopify Payments refund.
Fields
- status string? - The current status of the refund. Valid values are pending, failure, success, and error.
- acquirer_reference_number string? - A unique number associated with the transaction that can be used to track the refund. This property has a value only for transactions completed with Visa or Mastercard.
shopify.admin: PaymentTerms
The terms and conditions under which a payment should be processed.
Fields
- amount int? - The amount that is owed according to the payment terms.
- currency string? - The presentment currency for the payment.
- payment_terms_name string? - The name of the selected payment terms template for the order.
- payment_terms_type string? - The type of selected payment terms template for the order.
- due_in_days int? - The number of days between the invoice date and due date that is defined in the selected payment terms template.
- payment_schedules PaymentSchedule[]? - An array of schedules associated to the payment terms.
shopify.admin: PresentmentPrice
The variant's presentment prices and compare-at prices in each of the shop's enabled presentment currencies.
Fields
- price Price? - The price object
- compare_at_price Price? - The price object
shopify.admin: PresentmentPrices
The variant's presentment prices and compare-at prices in each of the shop's enabled presentment currencies.
Fields
- presentment_prices PresentmentPrice[]? - A list of the variant's presentment prices and compare-at prices in each of the shop's enabled presentment currencies.
shopify.admin: Price
The price object
Fields
- amount string? - The variant's price or compare-at price in the presentment currency.
- currency_code string? - The three-letter code (ISO 4217 format) for one of the shop's enabled presentment currencies.
shopify.admin: PriceSet
The price of the line item in shop and presentment currencies.
Fields
- shop_money Price? - The price object
- presentment_money Price? - The price object
shopify.admin: Product
The Product resource lets you update and create products in a merchant's store.
Fields
- id int? - An unsigned 64-bit integer that's used as a unique identifier for the product. Each id is unique across the Shopify system. No two products will have the same id, even if they're from different shops.
- created_at string? - The date and time (ISO 8601 format) when the product was created.
- 'handle string? - A unique human-friendly string for the product. Automatically generated from the product's title. Used by the Liquid templating language to refer to objects.
- body_html string? - A description of the product. Supports HTML formatting.
- images ProductImage[]? - A list of product image objects, each one representing an image associated with the product.
- options ProductOption[]? - The custom product properties. For example, Size, Color, and Material. Each product can have up to 3 options and each option value can be up to 255 characters. Product variants are made of up combinations of option values. Options cannot be created without values. To create new options, a variant with an associated option value also needs to be created.
- product_type string? - A categorization for the product used for filtering and searching products.
- published_at string? - The date and time (ISO 8601 format) when the product was published. Can be set to null to unpublish the product from the Online Store channel.
- published_scope string? - Whether the product is published to the Point of Sale channel.
- status string? - The status of the product.
- tags string? - A string of comma-separated tags that are used for filtering and search. A product can have up to 250 tags. Each tag can have up to 255 characters.
- template_suffix string? - The suffix of the Liquid template used for the product page. If this property is specified, then the product page uses a template called "product.suffix.liquid", where "suffix" is the value of this property. If this property is "" or null, then the product page uses the default template "product.liquid". (default is null)
- title string? - The name of the product.
- updated_at string? - The date and time (ISO 8601 format) when the product was last modified. A product's updated_at value can change for different reasons. For example, if an order is placed for a product that has inventory tracking set up, then the inventory adjustment is counted as an update.
- variants ProductVariant[]? - An array of product variants, each representing a different version of the product. The position property is read-only. The position of variants is indicated by the order in which they are listed.
- vendor string? - The name of the product's vendor.
shopify.admin: ProductImage
Products are easier to sell if customers can see pictures of them, which is why there are product images.
Fields
- created_at string? - The date and time when the product image was created. The API returns this value in ISO 8601 formatting.
- id int? - A unique numeric identifier for the product image.
- position int? - The order of the product image in the list. The first product image is at position 1 and is the "main" image for the product.
- product_id int? - The id of the product associated with the image.
- variant_ids int[]? - An array of variant ids associated with the image.
- src string? - Specifies the location of the product image. This parameter supports URL filters that you can use to retrieve modified copies of the image. For example, add _small, to the filename to retrieve a scaled copy of the image at 100 x 100 px (for example, ipod-nano_small.png), or add _2048x2048 to retrieve a copy of the image constrained at 2048 x 2048 px resolution (for example, ipod-nano_2048x2048.png).
- width int? - Width dimension of the image which is determined on upload.
- height int? - Height dimension of the image which is determined on upload.
- updated_at string? - The date and time when the product image was last modified. The API returns this value in ISO 8601 format.
shopify.admin: ProductList
A list of products
Fields
- customers Product[]? - A list of products
shopify.admin: ProductObject
Fields
- product Product? - The Product resource lets you update and create products in a merchant's store.
shopify.admin: ProductOption
The custom product properties. For example, Size, Color, and Material. Each product can have up to 3 options and each option value can be up to 255 characters.
Fields
- id int? - Product option ID
- name string? - Product option name
- position int? - Product option position
- product_id int? - Product option product ID
- values string[]? - Product option values
shopify.admin: ProductVariant
A variant can be added to a Product resource to represent one version of a product with several options. The Product resource will have a variant for every possible combination of its options. Each product can have a maximum of three options and a maximum of 100 variants.
Fields
- barcode string? - The barcode, UPC, or ISBN number for the product.
- compare_at_price string? - The original price of the item before an adjustment or a sale.
- created_at string? - The date and time (ISO 8601 format) when the product variant was created.
- fulfillment_service string? - The fulfillment service associated with the product variant. Valid values are manual or the handle of a fulfillment service.
- grams int? - The weight of the product variant in grams.
- id int? - The unique numeric identifier for the product variant.
- image_id int? - The unique numeric identifier for a product's image. The image must be associated to the same product as the variant.
- inventory_item_id int? - The unique identifier for the inventory item, which is used in the Inventory API to query for inventory information.
- inventory_management string? - The fulfillment service that tracks the number of items in stock for the product variant.
- inventory_policy string? - Whether customers are allowed to place an order for the product variant when it's out of stock.
- inventory_quantity int? - An aggregate of inventory across all locations. To adjust inventory at a specific location, use the InventoryLevel resource.
- old_inventory_quantity int? - This property is deprecated. Use the InventoryLevel resource instead.
- inventory_quantity_adjustment int? - This property is deprecated. Use the InventoryLevel resource instead.
- option Option? - The custom properties that a shop owner uses to define product variants. You can define three options for a product variant are option1, option2, option3. Default value is Default Title. The title field is a concatenation of the option1, option2, and option3 fields. Updating the option fields updates the title field.
- presentment_prices PresentmentPrices? - The variant's presentment prices and compare-at prices in each of the shop's enabled presentment currencies.
- position int? - The order of the product variant in the list of product variants. The first position in the list is 1. The position of variants is indicated by the order in which they are listed.
- price string? - The price of the product variant.
- product_id int? - The unique numeric identifier for the product.
- requires_shipping boolean? - This property is deprecated. Use the
requires_shipping
property on the InventoryItem resource instead.
- sku string? - A unique identifier for the product variant in the shop. Required in order to connect to a FulfillmentService.
- taxable boolean? - Whether a tax is charged when the product variant is sold.
- tax_code string? - This parameter applies only to the stores that have the Avalara AvaTax app installed. Specifies the Avalara tax code for the product variant.
- title string? - The title of the product variant. The title field is a concatenation of the option1, option2, and option3 fields. You can only update title indirectly using the option fields.
- updated_at string? - The date and time when the product variant was last modified. Gets returned in ISO 8601 formatting.
- weight int? - The weight of the product variant in the unit system specified with weight_unit.
- weight_unit string? - The unit of measurement that applies to the product variant's weight. If you don't specify a value for weight_unit, then the shop's default unit of measurement is applied. Valid values are g, kg, oz, and lb.
shopify.admin: ProductVariantList
A list of products
Fields
- variants ProductVariant[]? - A list of product variants
shopify.admin: ProductVariantObject
Fields
- variant ProductVariant? - A variant can be added to a Product resource to represent one version of a product with several options. The Product resource will have a variant for every possible combination of its options. Each product can have a maximum of three options and a maximum of 100 variants.
shopify.admin: Property
Properties
Fields
- name string? - Property name
- value string? - Property value
shopify.admin: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
shopify.admin: Receipt
A text field that provides information about the receipt
Fields
- testcase boolean? - Whether the fulfillment was a testcase.
- authorization string? - The authorization code.
shopify.admin: Refund
Schedule associated to the payment terms
Fields
- created_at string? - The date and time (ISO 8601 format) when the refund was created.
- id int? - The unique identifier for the refund.
- note string? - An optional note attached to a refund.
- order_adjustments OrderAdjustment[]? - A list of order adjustments attached to the refund. Order adjustments are generated to account for refunded shipping costs and differences between calculated and actual refund amounts.
- processed_at string? - The date and time (ISO 8601 format) when the refund was imported. This value can be set to a date in the past when importing from other systems. If no value is provided, then it will be auto-generated as the current time in Shopify. Public apps need to be granted permission by Shopify to import orders with the processed_at timestamp set to a value earlier the created_at timestamp. Private apps can't be granted permission by Shopify.
- refund_line_items RefundLineItem[]? - A list of refunded line items.
- user_id int? - The unique identifier of the user who performed the refund.
shopify.admin: RefundLineItem
Refunded line item
Fields
- id int? - The unique identifier of the line item in the refund.
- line_item_id int? - The ID of the related line item in the order.
- quantity int? - The quantity of the associated line item that was returned.
- restock_type string? - How this refund line item affects inventory levels.
- location_id int? - The unique identifier of the location where the items will be restocked. Required when restock_type has the value return or cancel.
- subtotal decimal? - The subtotal of the refund line item.
- total_tax decimal? - The total tax on the refund line item.
shopify.admin: RefundLineItemObject
Line item IDs, quantities to refund, and restock instructions.
Fields
- quantity int? - The quantity to refund.
- location_id int? - The ID of the location where the items should be restocked. This is required when the value of restock_type is return or cancel. If the item is not already stocked at the location, then the item is connected to the location. An error is returned when the item is connected to a fulfillment service location and a different location is provided.
- line_item_id int? - The ID of a line item to refund.
- restock_type string? - How this refund line item affects inventory levels. Valid values are no_restock, cancel, and return.
shopify.admin: RefundObject
The Refund object.
Fields
- refund Refund? - Schedule associated to the payment terms
shopify.admin: RefundRequest
Schedule associated to the payment terms
Fields
- currency string? - The three-letter code (ISO 4217 format) for the currency used for the refund.
- discrepancy_reason string? - An optional comment that explains a discrepancy between calculated and actual refund amounts. Used to populate the reason property of the resulting order adjustment object attached to the refund. Valid values are restock, damage, customer, and other.
- note string? - An optional note attached to a refund.
- notify boolean? - Whether to send a refund notification to the customer.
- refund_line_items RefundLineItemObject[]? - A list of line item IDs, quantities to refund, and restock instructions.
- shipping Shipping? - Specify how much shipping to refund.
- transactions Transaction[]? - A list of transactions to process as refunds.
shopify.admin: Shipping
Specify how much shipping to refund.
Fields
- amount decimal? - Set a specific amount to refund for shipping. Takes precedence over full_refund.
- full_refund boolean? - Whether to refund all remaining shipping.
shopify.admin: ShippingLine
An object, which details a shipping method used.
Fields
- code string? - A reference to the shipping method.
- discounted_price string? - The price of the shipping method after line-level discounts have been applied. Doesn't reflect cart-level or order-level discounts.
- discounted_price_set DiscountedPriceSet? - The price of the shipping method in both shop and presentment currencies after line-level discounts have been applied.
- price string? - The price of this shipping method in the shop currency. Can't be negative.
- price_set PriceSet? - The price of the line item in shop and presentment currencies.
- 'source string? - The source of the shipping method.
- title string? - The title of the shipping method.
- carrier_identifier string? - A reference to the carrier service that provided the rate. Present when the rate was computed by a third-party carrier service.
- requested_fulfillment_service_id string? - A reference to the fulfillment service that is being requested for the shipping method. Present if the shipping method requires processing by a third party fulfillment service; null otherwise.
shopify.admin: SmsMarketingConsent
The marketing consent information when the customer consented to receiving marketing material by SMS. The phone property is required to create a customer with SMS consent information and to perform an SMS update on a customer that doesn't have a phone number recorded. The customer must have a unique phone number associated to the record.
Fields
- state string? - The current SMS marketing state for the customer.
- opt_in_level string? - The marketing subscription opt-in level, as described by the M3AAWG best practices guidelines, that the customer gave when they consented to receive marketing material by SMS.
- consent_updated_at string? - The date and time at which the customer consented to receive marketing material by SMS. The customer's consent state reflects the consent record with the most recent last_consent_updated_at date. If no date is provided, then the date and time at which the consent information was sent is used.
- consent_collected_from string? - The source for whether the customer has consented to receive marketing material by SMS.
shopify.admin: SubtotalPriceSet
The subtotal of the order in shop and presentment currencies after discounts but before shipping, duties, taxes, and tips.
Fields
- shop_money Price? - The price object
- presentment_money Price? - The price object
shopify.admin: TaxLine
Tax line object, which details a tax applicable to the order.
Fields
- price string? - The amount of tax to be charged in the shop currency.
- rate decimal? - The rate of tax to be applied.
- title string? - The name of the tax.
- channel_liable boolean? - Whether the channel that submitted the tax line is liable for remitting. A value of null indicates unknown liability for the tax line.
shopify.admin: TotalDiscountSet
The total amount allocated to the line item in the presentment currency. Instead of using this field, Shopify recommends using discount_allocations, which provides the same information.
Fields
- shop_money Price? - The price object
- presentment_money Price? - The price object
shopify.admin: TotalDiscountsSet
The total discounts applied to the price of the order in shop and presentment currencies.
Fields
- shop_money Price? - The price object
- presentment_money Price? - The price object
shopify.admin: TotalLineItemsPriceSet
The total of all line item prices in shop and presentment currencies.
Fields
- shop_money Price? - The price object
- presentment_money Price? - The price object
shopify.admin: TotalPriceSet
The total price of the order in shop and presentment currencies.
Fields
- shop_money Price? - The price object
- presentment_money Price? - The price object
shopify.admin: TotalShippingPriceSet
The total shipping price of the order, excluding discounts and returns, in shop and presentment currencies. If taxes_included is set to true, then total_shipping_price_set includes taxes.
Fields
- shop_money Price? - The price object
- presentment_money Price? - The price object
shopify.admin: TotalTaxSet
The total tax applied to the order in shop and presentment currencies.
Fields
- shop_money Price? - The price object
- presentment_money Price? - The price object
shopify.admin: Transaction
Transactions are created for every order that results in an exchange of money. There are five types of transactions. (Authorization: An amount reserved against the cardholder's funding source. Money does not change hands until the authorization is captured, Sale: An authorization and capture performed together in a single step, Capture: A transfer of the money that was reserved during the authorization stage, Void: A cancellation of a pending authorization or capture, Refund: A partial or full return of captured funds to the cardholder. A refund can happen only after a capture is processed.)
Fields
- amount string? - The amount of money included in the transaction. If you don't provide a value for
amount
, then it defaults to the total cost of the order (even if a previous transaction has been made towards it).
- authorization string? - The authorization code associated with the transaction.
- authorization_expires_at string? - The date and time (ISO 8601 format) when the Shopify Payments authorization expires.
- created_at string? - The date and time (ISO 8601 format) when the transaction was created.
- currency string? - The three-letter code (ISO 4217 format) for the currency used for the payment.
- device_id int? - The ID for the device.
- error_code string? - A standardized error code, independent of the payment provider.
- extended_authorization_attributes ExtendedAuthorizationAttributes? - The attributes associated with a Shopify Payments extended authorization period.
- gateway string? - The name of the gateway the transaction was issued through. A list of gateways can be found on Shopify's payment gateways page.
- id int? - The ID for the transaction.
- kind string? - The transaction's type.
- location_id LocationID? - The ID of the physical location where the transaction was processed.
- message string? - A string generated by the payment provider with additional information about why the transaction succeeded or failed.
- order_id int? - The ID for the order that the transaction is associated with.
- payment_details PaymentDetails? - Information about the credit card used for this transaction.
- parent_id int? - The ID of an associated transaction.
- payments_refund_attributes PaymentsRefundAttributes? - The attributes associated with a Shopify Payments refund.
- processed_at string? - The date and time (ISO 8601 format) when a transaction was processed. This value is the date that's used in the analytic reports. By default, it matches the created_at value. If you're importing transactions from an app or another platform, then you can set processed_at to a date and time in the past to match when the original transaction was processed.
- source_name string? - The origin of the transaction. This is set by Shopify and can't be overridden. Example values are web, pos, iphone, and android.
- status string? - The status of the transaction. Valid values are pending, failure, success, and error.
- test boolean? - Whether the transaction is a test transaction.
- user_id int? - The ID for the user who was logged into the Shopify POS device when the order was processed, if applicable.
- currency_exchange_adjustment CurrencyExchangeAdjustment? - An adjustment on the transaction showing the amount lost or gained due to fluctuations in the currency exchange rate. Requires the header X-Shopify-Api-Features = include-currency-exchange-adjustments.
shopify.admin: TransactionObject
The Transaction object.
Fields
- 'transaction Transaction? - Transactions are created for every order that results in an exchange of money. There are five types of transactions. (Authorization: An amount reserved against the cardholder's funding source. Money does not change hands until the authorization is captured, Sale: An authorization and capture performed together in a single step, Capture: A transfer of the money that was reserved during the authorization stage, Void: A cancellation of a pending authorization or capture, Refund: A partial or full return of captured funds to the cardholder. A refund can happen only after a capture is processed.)
shopify.admin: UpdateCustomer
The Customer object to be updated.
Fields
- customer Customer? - The Customer resource stores information about a shop's customers, such as their contact details, their order history, and whether they've agreed to receive email marketing.
shopify.admin: UpdateOrder
The Order object to be updated.
Fields
- 'order Order? - An order is a customer's request to purchase one or more products from a shop. You can create, retrieve, update, and delete orders using the Order resource.
shopify.admin: UpdateOrderRisk
The order risk object to be updated.
Fields
- risk OrderRisk? - The order risk for an order.
shopify.admin: UpdateProduct
Fields
- product Product? - The Product resource lets you update and create products in a merchant's store.
shopify.admin: UpdateProductVariant
Fields
- variant ProductVariant? - A variant can be added to a Product resource to represent one version of a product with several options. The Product resource will have a variant for every possible combination of its options. Each product can have a maximum of three options and a maximum of 100 variants.
shopify.admin: UpdateWebhook
The webhook object to be updated.
Fields
- webhook Webhook? - The webhook resource.
shopify.admin: Webhook
The webhook resource.
Fields
- address string? - Destination URI to which the webhook subscription should send the POST request when an event occurs.
- api_version string? - The Admin API version that Shopify uses to serialize webhook events. This value is inherited from the app that created the webhook subscription.
- created_at string? - Date and time when the webhook subscription was created. The API returns this value in ISO 8601 format.
- fields string[]? - An optional array of top-level resource fields that should be serialized and sent in the POST request. If absent, all fields will be sent.
- format string? - Format in which the webhook subscription should send the data. Valid values are JSON and XML. Defaults to JSON.
- id int? - Unique numeric identifier for the webhook subscription.
- metafield_namespaces string[]? - Optional array of namespaces for any metafields that should be included with each webhook.
- private_metafield_namespaces string[]? - Optional array of namespaces for any private metafields that should be included with each webhook.
- topic string? - Event that triggers the webhook. Valid values are app/uninstalled, bulk_operations/finish, carts/create, carts/update, checkouts/create, checkouts/delete, checkouts/update, collection_listings/add, collection_listings/remove, collection_listings/update, collections/create, collections/delete, collections/update, customer_groups/create, customer_groups/delete, customer_groups/update, customer_payment_methods/create, customer_payment_methods/revoke, customer_payment_methods/update, customers/create, customers/delete, customers/disable, customers/enable, customers/update, customers_marketing_consent/update, disputes/create, disputes/update, domains/create, domains/destroy, domains/update, draft_orders/create, draft_orders/delete, draft_orders/update, fulfillment_events/create, fulfillment_events/delete, fulfillments/create, fulfillments/update, inventory_items/create, inventory_items/delete, inventory_items/update, inventory_levels/connect, inventory_levels/disconnect, inventory_levels/update, locales/create, locales/update, locations/create, locations/delete, locations/update, order_transactions/create, orders/cancelled, orders/create, orders/delete, orders/edited, orders/fulfilled, orders/paid, orders/partially_fulfilled, orders/updated, product_listings/add, product_listings/remove, product_listings/update, products/create, products/delete, products/update, profiles/create, profiles/delete, profiles/update, refunds/create, selling_plan_groups/create, selling_plan_groups/delete, selling_plan_groups/update, shop/update, subscription_billing_attempts/challenged, subscription_billing_attempts/failure, subscription_billing_attempts/success, subscription_contracts/create, subscription_contracts/update, tender_transactions/create, themes/create, themes/delete, themes/publish, themes/update
- updated_at string? - Date and time when the webhook subscription was updated. The API returns this value in ISO 8601 format.
shopify.admin: WebhookCountObject
The webhook subscription count object.
Fields
- count int? - Webhook subscription count.
shopify.admin: WebhookList
The object representation of a list of webhook subscriptions.
Fields
- webhooks Webhook[]? - A list of webhook subscriptions
shopify.admin: WebhookObject
The webhook object.
Fields
- webhook Webhook? - The webhook resource.
Import
import ballerinax/shopify.admin;
Metadata
Released date: about 2 years ago
Version: 2.4.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 2615
Current verison: 286
Weekly downloads
Keywords
Commerce/eCommerce
Cost/Paid
Contributors
Dependencies