shipwire.warehouse
Module shipwire.warehouse
API
Definitions
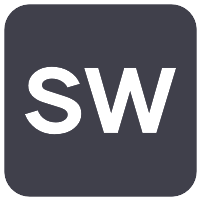
ballerinax/shipwire.warehouse Ballerina library
Overview
This is a generated connector for Shipwire Warehouses API v3.0 OpenAPI specification. The Warehouse API is used to manage Shipwire Anywhere warehouses and dropship locations. Use it to create a new warehouse, to update an existing warehouse, or to get information about already created warehouses.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create Shipwire account
- Obtain tokens by following this guide
Quickstart
To use the Shipwire Warehouses connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/shipwire.warehouse
module into the Ballerina project.
import ballerinax/shipwire.warehouse;
Step 2: Create a new connector instance
Create a warehouse:ClientConfig
with the token obtained, and initialize the connector with it.
warehouse:ClientConfig clientConfig = { auth: { token: <ACCESS_TOKEN> } }; warehouse:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to get a collection of warehouses using the connector.
Get a collection of warehouses
public function main() { warehouse:GetWarehousesResponse|error response = baseClient->getWarehouses(); if (response is warehouse:GetWarehousesResponse) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
shipwire.warehouse: Client
This is a generated connector for Shipwire Warehouses API v3.0 OpenAPI specification. The Warehouse API is used to manage Shipwire Anywhere warehouses and dropship locations. Use it to create a new warehouse, to update an existing warehouse, or to get information about already created warehouses.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Shipwire account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.shipwire.com/" - URL of the target service
getWarehouses
function getWarehouses(int? id, string? externalId, string? name, int? vendorId, string? vendorExternalId, string? country, string? generatesLabels, string? 'type) returns GetWarehousesResponse|error
Get warehouses
Parameters
- id int? (default ()) - Filter by warehouse ids, supports comma separated list
- externalId string? (default ()) - Filter by warehouse external ids, supports comma separated list
- name string? (default ()) - Filter by warehouse names, supports comma separated list
- vendorId int? (default ()) - Filter by associated vendor's id, supports comma separated list
- vendorExternalId string? (default ()) - Filter by associated vendor's external id, supports comma separated list
- country string? (default ()) - Filter by country, supports comma separated list
- generatesLabels string? (default ()) - Filter by warehouses that require label generation
- 'type string? (default ()) - Filter by type ("SHIPWIRE", "SHIPWIRE_ANYWHERE")
Return Type
postWarehouse
function postWarehouse(CreateANewWarehouseRequest payload) returns CreateANewWarehouseResponse|error
Create a new warehouse
Parameters
- payload CreateANewWarehouseRequest - Create a new warehouse request
Return Type
getWarehousesById
function getWarehousesById(string id) returns GetAWarehouseResponse|error
Get a warehouse
Parameters
- id string - The warehouse id or external id.
Return Type
putWarehousesById
function putWarehousesById(string id, UpdateAWarehouseRequest payload) returns CreateANewWarehouseResponse|error
Update a warehouse
Parameters
- id string - The warehouse's id or external id.
- payload UpdateAWarehouseRequest - Update a warehouse request
Return Type
- CreateANewWarehouseResponse|error - Create a new warehouse response
postWarehousesRetireById
function postWarehousesRetireById(string id) returns RetireAWarehouseResponse|error
Retire a warehouse
Parameters
- id string - Warehouse id or external id
Return Type
- RetireAWarehouseResponse|error - Retire a warehouse response
getWarehousesContainersById
function getWarehousesContainersById(string id) returns GetContainersAssociatedWithAWarehouseResponse|error
Get containers associated with a warehouse
Parameters
- id string - The warehouse id or external id.
Return Type
- GetContainersAssociatedWithAWarehouseResponse|error - Get containers associated with a warehouse response
getWarehousesCarriersById
function getWarehousesCarriersById(string id) returns GetCarriersResponse|error
Get carriers associated with a warehouse
Parameters
- id string - The warehouse id or external id.
Return Type
- GetCarriersResponse|error - Get carriers response
addCarriersByIdToWarehouse
function addCarriersByIdToWarehouse(string id, AddCarrierRequest payload) returns GetCarriersResponse|error
Add carriers to a warehouse
Return Type
- GetCarriersResponse|error - Get carriers response
removeCarriersByIdToWarehouse
function removeCarriersByIdToWarehouse(string id, RemoveCarrierRequest payload) returns GetCarriersResponse|error
Remove carriers to a warehouse
Parameters
- id string - Warehouse id or external id.
- payload RemoveCarrierRequest - Remove carrier request
Return Type
- GetCarriersResponse|error - Get carriers response
Records
shipwire.warehouse: AddCarrierRequest
Fields
- carrierCodes string[] - A string consisting of carrier code and service level.
shipwire.warehouse: CarrierResourceLocation
Carrier Resource Location
Fields
- resourceLocation string? - A URL that gives more information about the linked resource. A "null" value would mean that no further information is available for that resource.
- 'resource GetCarrierProperties? - Carrier properties for container
shipwire.warehouse: CarrierResources
Carrier Resources
Fields
- offset int? - This is an integer that matches the offset given in the request and dictates the number of items to skip while fetching results. For example, there is a preset limit of 20 results per page. If there are 30 total results and offset is set to 20, then the response will show the second "page" of results, with items 21 to 30.
- total int? - Total number of available items in the API response.
- previous string? - A link used to fetch the previous set or page of items in the API response.
- next string? - A link used to fetch the next set or page of items in the API response.
- items record {}[]? - Items
shipwire.warehouse: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
shipwire.warehouse: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth CredentialsConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
shipwire.warehouse: ContainerDimensions
Fields
- length float? - This is the length of the container based on its Unit of Measurement (lengthUnit)
- width float? - This is the width of the container based on its Unit of Measurement (widthUnit)
- height float? - This is the width of the container based on its Unit of Measurement (widthUnit)
- weight float? - This is the weight of the container based on its Unit of Measurement (weightUnit)
- maxWeight float? - This is the maximum weight that the container can hold based on its Unit of Measurement (maxWeightUnit)
- lengthUnit string? - This is the Unit of Measurement for Container length (e.g. inches)
- widthUnit string? - This is the Unit of Measurement for Container width (e.g. inches)
- heightUnit string? - This is the Unit of Measurement for Container height (e.g. inches)
- weightUnit string? - This is the Unit of Measurement for Container weight (e.g. pounds)
- maxWeightUnit string? - This is the Unit of Measurement for Container's max weight (e.g. pounds)
shipwire.warehouse: CostValues
Fields
- costValue string? - This is the cost value for the container. During cartonization (choosing packaging) phase for an order, Shipwire will first consider containers with a lower cost, even if chosing one will end up making the shipping cost comparatively higher. E.g. Shipwire will chose a container 1 with a $0 cost over a container 2 with a $1 cost, even if the shipping cost for using an overly large container 1 is $10 higher than for using a container 2.
- retailValue string? - This is the retail value for the container. Container retail value will be added to the shipping quote for the customer. If a merchant doesn't plan to charge customers for cartons/boxes, the container retail value should be set to $0.
- costValueCurrency string? - This is the currency used in determining cost value for the container.
- retailValueCurrency string? - This is the currency used in determining retail value for the container.
shipwire.warehouse: CreateANewWarehouseRequest
Create a New Shipwire Anywhere Warehouse
Fields
- Fields Included from *PostWarehouseRequest
shipwire.warehouse: CreateANewWarehouseResponse
Fields
- Fields Included from *WarehouseServerResponse
shipwire.warehouse: GetAWarehouseResponse
Fields
- Fields Included from *WarehouseServerResponse
shipwire.warehouse: GetCarrierProperties
Carrier properties for container
Fields
- code string? - A string consisting of carrier code and service level.
- serviceLevelCode string? - This is the service level code associated with the carrier. There are multiple levels for one type of service. Each level has a specific code. (e.g. For FDX the codes are 1D, 2D, GD, etc.)
- name string? - Name of the carrier
- isFreightCarrier int? - A boolean that indicates the carrier is a freight carrier. The value "1" (True) means this carrier supports heavy weight orders or large items.
- isRestrictedCarrier int? - A boolean that indicates whether or not the carrier is restricted. The value "1" (True) means this carrier is not available for general use. If required, contact customer care for possible enablement.
- isPostalCarrier int? - A boolean that indicates the carrier uses a national government postal service, either for aggregated or for last mile delivery.
- isTerritorySupported int? - A boolean that indicates if the carrier supports US territories. (e.g. Puerto Rico, Guam, etc.)
- isPoBoxSupported int? - A boolean that indicates if the carrier supports PO box delivery.
- isApoFpoSupported int? - A boolean that indicates the carrier supports delivery to military addresses (APO, FPO).
- isPickupRequired int? - A boolean that indicates the carrier requires pickup. A "0" (False) value would mean that the carrier supports delivery to doorstep. This feature is related to third party carriers.
- isSignatureRequired int? - A boolean that indicates the carrier requires a signature upon delivery of the order.
- isSelfInsured int? - A boolean that indicates the carrier is self-insured for damages that occur to an order while in transit.
- isDangerousGoodsSupported int? - A boolean that indicates the carrier can transport orders that include dangerous goods. (e.g. chemicals, etc.)
- isTrackable int? - A boolean that indicates orders transported by the carrier will receive a tracking ID so that it can be tracked after leaving the warehouse through to delivery.
- isMediaSupported int? - A boolean that indicates the carrier provides special services specific to the handling of media. (e.g. books, videotapes, DVDs, CDs, printed music and other sound recordings)
- minimumShippingDays int? - The minimum number of days the carrier takes for shipping the order.
- maximumShippingDays int? - The maximum number of days the carrier takes for shipping the order.
shipwire.warehouse: GetCarriersResponse
Fields
- Fields Included from *WarehouseServerResponse
shipwire.warehouse: GetContainersAssociatedWithAWarehouseResponse
Get list of containers associated with Warehouse. This contains information for both Shipwire and Shipwire anywhere Warehouse
Fields
- status int? - This is the HTTP status code.
- message string? - This is the HTTP status message.
- resourceLocation string? - A URL that gives more information about the linked resource. A "null" value would mean that no further information is available for that resource.
- 'resource GetcontainersassociatedwithawarehouseresponseResource? - GetContainersAssociatedWithAWarehouseResponse resource
shipwire.warehouse: GetcontainersassociatedwithawarehouseresponseResource
GetContainersAssociatedWithAWarehouseResponse resource
Fields
- items ResourceInWarehouseResponse[]? - ResourceInWarehouseResponse list
shipwire.warehouse: GetWarehouseResources
Warehouse Resources
Fields
- offset int? - This is an integer that matches the offset given in the request and dictates the number of items to skip while fetching results. For example, there is a preset limit of 20 results per page. If there are 30 total results and offset is set to 20, then the response will show the second "page" of results, with items 21 to 30.
- total int? - Total number of available items in the API response.
- previous string? - A link used to fetch the previous set or page of items in the API response.
- next string? - A link used to fetch the next set or page of items in the API response.
- items GetwarehouseresourcesItems[]? - GetWarehouseResources items
shipwire.warehouse: GetwarehouseresourcesItems
Fields
- resourceLocation string? - A URL that gives more information about the linked resource. A "null" value would mean that no further information is available for that resource.
- 'resource WarehouseInformation? - Warehouse resource information
shipwire.warehouse: GetWarehousesResponse
Fields
- Fields Included from *WarehouseServerResponse
shipwire.warehouse: PostWarehouseRequest
Sample Post request to create a new warehouse
Fields
- externalId string? - A unique user-provided identifier for the warehouse in an external system.
- name string - Name of the warehouse
- code string? - This is the code assigned to the warehouse for easy reference.
- vendorId int? - A unique ID assigned to each vendor in the Shipwire system. (See the Vendors API.)
- vendorExternalId string? - A unique user provided identifier for a vendor in an external system.
- isActive int - A boolean which indicates whether or not the warehouse is available to the customer for storage, shipping and receiving inventory.
- address record {} - Address
- latitude float? - Latitude of the warehouse. This is useful for routing purposes to find the nearest warehouse.
- longitude float? - Longitude of the warehouse. This is useful for routing purposes to find the nearest warehouse.
- isRoutable int? - A boolean which indicates whether orders may be routed to this warehouse by our routing engine.
- labelFormat string - This is the generic format of the label that the warehouse generates for each customer
- generatesLabels int? - A boolean which indicates whether or not the warehouse can generate shipping labels. These labels are stored on the server and can be given to the customer if required
- returnWarehouseId int? - This is the unique ID of the Shipwire warehouse used for RMA process and will store returned goods.
- returnWarehouseExternalId string? - This is the external ID of the Shipwire warehouse used for RMA process.
shipwire.warehouse: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
shipwire.warehouse: RemoveCarrierRequest
Fields
- carrierCodes string[] - A string consisting of carrier code and service level.
shipwire.warehouse: ResourceDimensionValues
Fields
- id int? - A unique auto-generated ID assigned to each new container added to the Shipwire Platform.
- externalId string? - A unique user-provided identifier for a container in an external system.
- name string? - A unique identifier for the container in the Shipwire system.
- 'type string? - This is the type of container based on the contents stored in it.
- isActive int? - A boolean which indicates whether or not the warehouse can use the container for a particular customer for packaging.
- warehouseId int? - A unique identifier for a Shipwire or Shipwire Anywhere warehouse.
- warehouseExternalId string? - A unique user-provided identifier for a Shipwire Anywhere warehouse in an external system.
- basis float? - Basis is a parameter that is used by Shipwire to prioritize the use of some boxes over the others depending on the product dimensions. The higher the box dimensions are the higher the basis will be. The highest basis for standard boxes is 1025. Pallets have the highest basis where as envelopes have the lowest basis. When setting up a merchant specific box, if you want to use this new box over some of the generic boxes that are bigger in volume, you need to assign a higher basis for the new box to ensure products rate into the box you create.
- serviceSpecificCarrierCode string? - It indicates that this container is used with a particular carrier service (eg. FDX 1D).
- dimensions ResourcedimensionvaluesDimensions? -
shipwire.warehouse: ResourcedimensionvaluesDimensions
Fields
- resourceLocation string? - A URL that gives more information about the linked resource. A "null" value would mean that no further information is available for that resource.
- 'resource ContainerDimensions? -
shipwire.warehouse: ResourceInWarehouseResponse
Fields
- resourceLocation string? - A URL that gives more information about the linked resource. A "null" value would mean that no further information is available for that resource
- 'resource ResourceinwarehouseresponseResource? -
shipwire.warehouse: ResourceinwarehouseresponseResource
Fields
shipwire.warehouse: ResourceinwarehouseresponseResourceValues
Fields
- resourceLocation string? - A URL that gives more information about the linked resource. A "null" value would mean that no further information is available for that resource.
- 'resource CostValues? -
shipwire.warehouse: RetireAWarehouse404Response
Fields
- status int? - This is the HTTP status code.
- message string? - This is the HTTP status message.
- resourceLocation string? - A URL that gives more information about the linked resource. A "null" value would mean that no further information is available for that resource.
shipwire.warehouse: RetireAWarehouseResponse
Delete Shipwire Anywhere Warehouse from the system
Fields
- status int? - This is the HTTP status code.
- message string? - This is the HTTP status message.
- resourceLocation string? - A URL that gives more information about the linked resource. A "null" value would mean that no further information is available for that resource.
shipwire.warehouse: UpdateAWarehouseRequest
Update Shipwire Warehouse Information
Fields
- externalId string? - A unique user-provided identifier for the warehouse in an external system.
- name string? - Name of the warehouse
- code string? - This is the code assigned to the warehouse for easy reference.
- vendorId int? - A unique ID assigned to each vendor in the Shipwire system. (See the Vendors API.)
- vendorExternalId string? - A unique user provided identifier for a vendor in an external system.
- isActive int? - A boolean which indicates whether or not the warehouse is available to the customer for storage, shipping and receiving inventory.
- address WarehouseAddress? - Shipwire Anywhere Warehouse Address
- latitude float? - Latitude of the warehouse. This is useful for routing purposes to find the nearest warehouse.
- longitude float? - Longitude of the warehouse. This is useful for routing purposes to find the nearest warehouse.
- isRoutable int? - A boolean which indicates whether orders may be routed to this warehouse by our routing engine.
- generatesLabels int? - A boolean which indicates whether or not the warehouse can generate shipping labels. These labels are stored on the server and can be given to the customer if required
- returnWarehouseId string? - This is the unique ID of the Shipwire warehouse used for RMA process and will store returned goods.
- returnWarehouseExternalId string? - This is the external ID of the Shipwire warehouse used for RMA process.
shipwire.warehouse: WarehouseAddress
Shipwire Anywhere Warehouse Address
Fields
- address1 string - This is the first line of the address of the warehouse.
- address2 string? - This is the second line of the address of the warehouse.
- address3 string? - This is the third line of the address of the warehouse.
- city string - City where the warehouse is located.
- state string - State where the warehouse is located.
- postalCode string - Postal code of the warehouse
- country string - 2 digit country code of the warehouse
- continent string? - Name of the continent where the warehouse is located.
- name string - Name of the warehouse
- email string? - Email address of contact at the warehouse for any communication
- phone string - Contact phone number of the warehouse for any communication
- fax string? - Contact fax number of the warehouse for any communication
shipwire.warehouse: WarehouseInformation
Warehouse resource information
Fields
- id int? - A unique auto-generated ID assigned to each new warehouse added to the Shipwire Platform.
- externalId string? - A unique user-provided identifier for the warehouse in an external system.
- name string? - Name of the warehouse
- code string? - This is the code assigned to the warehouse for easy reference.
- vendorId int? - A unique ID assigned to each vendor in the Shipwire system. (See the Vendors API.)
- vendorExternalId string? - A unique user provided identifier for a vendor in an external system.
- isActive int? - A boolean which indicates whether or not the warehouse is available to the customer for storage, shipping and receiving inventory.
- address WarehouseinformationAddress? - WarehouseInformation address
- latitude float? - Latitude of the warehouse. This is useful for routing purposes to find the nearest warehouse.
- longitude float? - Longitude of the warehouse. This is useful for routing purposes to find the nearest warehouse.
- isRoutable int? - A boolean which indicates whether orders may be routed to this warehouse by our routing engine.
- generatesLabels int? - A boolean which indicates whether or not the warehouse can generate shipping labels. These labels are stored on the server and can be given to the customer if required
- 'type string? - This is the type of the warehouse. It can be Shipwire or Shipwire Anywhere.
- labelFormat string? - This is the generic format of the label that the warehouse generates for each customer
- combineShippingDocuments boolean? - This warehouse property ensures all shipping labels generated are a combination of packing list and label.
- returnWarehouseId string? - This is the unique ID of the Shipwire warehouse used for RMA process and will store returned goods.
- returnWarehouseExternalId string? - This is the external ID of the Shipwire warehouse used for RMA process.
- physicalWarehouseId int? - A shipwire warehouse ID used to identify a specific physical warehouse that corresponds to a Shipwire Anywhere warehouse and is useful in consolidating inventory. If the SKU item is at a Shipwire warehouse, then this will be "null".
- containers WarehouseinformationContainers? - WarehouseInformation containers
- carriers string? - These are customizations to carrier settings (ex. FDX 1D) specific to a warehouse. May relate to destination/origin country & zip code, max shipment dimensions & carrier capabilities etc.
shipwire.warehouse: WarehouseinformationAddress
WarehouseInformation address
Fields
- resourceLocation string? - A URL that gives more information about the linked resource. A "null" value would mean that no further information is available for that resource.
- 'resource WarehouseAddress? - Shipwire Anywhere Warehouse Address
shipwire.warehouse: WarehouseinformationContainers
WarehouseInformation containers
Fields
- resourceLocation string? - A URL that gives more information about the linked resource. A "null" value would mean that no further information is available for that resource.
shipwire.warehouse: WarehouserPaginationResponse
Server Pagination Response
Fields
- offset int? - This is an integer that matches the offset given in the request and dictates the number of items to skip while fetching results. For example, there is a preset limit of 20 results per page. If there are 30 total results and offset is set to 20, then the response will show the second "page" of results, with items 21 to 30.
- total int? - Total number of available items in the API response.
- previous string? - A link used to fetch the previous set or page of items in the API response.
- next string? - A link used to fetch the next set or page of items in the API response.
shipwire.warehouse: WarehouseServerResponse
Get Warehouses Response based on the criteria provided in the URL
Fields
- status int? - This is the HTTP status code.
- message string? - This is the HTTP status message.
Import
import ballerinax/shipwire.warehouse;
Metadata
Released date: over 1 year ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 0
Current verison: 0
Weekly downloads
Keywords
Commerce/eCommerce
Cost/Freemium
Contributors