shipwire.receivings
Module shipwire.receivings
API
Definitions
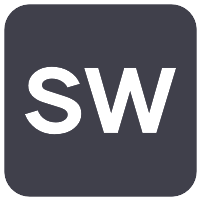
ballerinax/shipwire.receivings Ballerina library
Overview
This is a generated connector for Shipwire Receivings API v3.0 OpenAPI specification. Allows managing inventory at Shipwire and Shipwire Anywhere warehouses using receiving orders.
Prerequisites
Before using this connector in your Ballerina application, complete the following:
- Create Shipwire account
- Obtain tokens by following this guide
Quickstart
To use the Shipwire Receivings connector in your Ballerina application, update the .bal file as follows:
Step 1: Import connector
First, import the ballerinax/shipwire.receivings
module into the Ballerina project.
import ballerinax/shipwire.receivings;
Step 2: Create a new connector instance
Create a receivings:ClientConfig
with the token obtained, and initialize the connector with it.
receivings:ClientConfig clientConfig = { auth: { token: <ACCESS_TOKEN> } }; receivings:Client baseClient = check new Client(clientConfig);
Step 3: Invoke connector operation
-
Now you can use the operations available within the connector. Note that they are in the form of remote operations.
Following is an example on how to get list of advance ship notices using the connector.
Get list of advance ship notices
public function main() { receivings:GetListOfAdvanceShipNoticesResponse|error response = baseClient->getReceivings(); if (response is receivings:GetListOfAdvanceShipNoticesResponse) { log:printInfo(response.toString()); } else { log:printError(response.message()); } }
-
Use
bal run
command to compile and run the Ballerina program.
Clients
shipwire.receivings: Client
This is a generated connector for Shipwire Receivings API v3.0 OpenAPI specification. Allows managing inventory at Shipwire and Shipwire Anywhere warehouses using receiving orders.
Constructor
Gets invoked to initialize the connector
.
The connector initialization requires setting the API credentials.
Create a Shipwire account and obtain tokens by following this guide.
init (ConnectionConfig config, string serviceUrl)
- config ConnectionConfig - The configurations to be used when initializing the
connector
- serviceUrl string "https://api.shipwire.com/" - URL of the target service
getReceivings
function getReceivings(string[]? expand, string? commerceName, string? transactionId, string? externalId, string? orderId, string? orderNo, string? status, string? updatedAfter, string? warehouseId, string? warehouseExternalId, string? vendorId, string? vendorExternalId) returns GetListOfAdvanceShipNoticesResponse|error
Get list of advance ship notices
Parameters
- expand string[]? (default ()) - Expand receivings data in the response, instead of accessing directly via a URL (comma separated list). See resources
Holds
,Instruction Recipients
,Items
,Shipments
,labels
andTrackings
for information on the data model returned by this parameter.
- commerceName string? (default ()) - Filter by commerceName (comma separated list)
- transactionId string? (default ()) - Filter by transactionId (comma separated list)
- externalId string? (default ()) - Filter by externalId (comma separated list)
- orderId string? (default ()) - Filter by orderId (comma separated list)
- orderNo string? (default ()) - Filter by orderNo (comma separated list)
- status string? (default ()) - Filter by status (comma separated list)
- updatedAfter string? (default ()) - Only show resources updated after this date (ISO 8601 format, ex: "2014-05-30T13:08:29-07:00")
- warehouseId string? (default ()) - Filter by warehouseId (comma separated list)
- warehouseExternalId string? (default ()) - Filter by warehouseExternalId (comma separated list)
- vendorId string? (default ()) - Filter by vendorId
- vendorExternalId string? (default ()) - Filter by vendorExternalId
Return Type
postReceivings
function postReceivings(CreateAnAdvanceShipNoticeRequest payload, string[]? expand) returns PostListOfAdvanceShipNoticesResponse|error
Create an advance ship notice
Parameters
- payload CreateAnAdvanceShipNoticeRequest - CreateAnAdvanceShipNotice request
- expand string[]? (default ()) - Expand receivings data in the response, instead of accessing directly via a URL (comma separated list). See resources
Holds
,Instruction Recipients
,Items
,Shipments
,labels
andTrackings
for information on the data model returned by this parameter.
Return Type
getReceivingsById
function getReceivingsById(string id, string[]? expand) returns GetAdvanceShipNoticeResponse|error
Get advance ship notice
Parameters
- id string - The advance ship notice's ID.
- expand string[]? (default ()) - Expand receivings data in the response, instead of accessing directly via a URL (comma separated list). See resources
Holds
,Instruction Recipients
,Items
,Shipments
,labels
andTrackings
for information on the data model returned by this parameter.
Return Type
putReceivingsById
function putReceivingsById(string id, CreateAnAdvanceShipNoticeRequest payload, string[]? expand) returns GetAdvanceShipNoticeResponse|error
Update an advance ship notice
Parameters
- id string - The advance ship notice's ID.
- payload CreateAnAdvanceShipNoticeRequest - CreateAnAdvanceShipNotice request
- expand string[]? (default ()) - Expand receivings data in the response, instead of accessing directly via a URL (comma separated list). See resources
Holds
,Instruction Recipients
,Items
,Shipments
,labels
andTrackings
for information on the data model returned by this parameter.
Return Type
postReceivingsCancelById
function postReceivingsCancelById(string id) returns CancelAReceivingOrderResponse|error
Cancel a receiving order
Parameters
- id string - The advance ship notice's ID.
Return Type
postReceivingsLabelsCancelById
function postReceivingsLabelsCancelById(string id) returns CancelAReceivingLabelResponse|error
Cancel a receiving label
Parameters
- id string - The advance ship notice's ID.
Return Type
getReceivingsHoldsById
function getReceivingsHoldsById(string id, int? includeCleared) returns GetHoldDetailsResponse|error
Get holds detail
Parameters
- id string - The advance ship notice's ID.
- includeCleared int? (default ()) - When set to 1, response includes holds that have been cleared.
Return Type
getReceivingsInstructionsRecipientsById
function getReceivingsInstructionsRecipientsById(string id) returns GetInstructionsRecipientsDetailsResponse|error
Get instructions and the recipients contact details
Parameters
- id string - The advance ship notice's ID.
Return Type
getReceivingsExtendedAttributesById
function getReceivingsExtendedAttributesById(string id) returns GetReceivingExtendedAttributesResponse|error
Get receiving extended attributes
Parameters
- id string - The receiving's ID.
Return Type
getReceivingsItemsById
function getReceivingsItemsById(string id) returns GetItemsDetailResponse|error
Get items detail
Parameters
- id string - The advance ship notice's ID.
Return Type
getReceivingsShipmentsById
function getReceivingsShipmentsById(string id) returns GetShipmentDetailsResponse|error
Get shipment details
Parameters
- id string - The advance ship notice's ID.
Return Type
getReceivingsTrackingsById
function getReceivingsTrackingsById(string id) returns GetTrackingsDetailResponse|error
Get trackings detail
Parameters
- id string - The advance ship notice's ID.
Return Type
getReceivingsLabelsById
function getReceivingsLabelsById(string id) returns GetLabelsDetailResponse|error
Get labels detail
Parameters
- id string - The advance ship notice's ID.
Return Type
postReceivingsMarkCompleteById
function postReceivingsMarkCompleteById(string id) returns MarkTheReceivingCompletedResponse|error
Mark the receiving completed
Parameters
- id string - The advance ship notice's ID.
Return Type
Records
shipwire.receivings: Address
Shipping source information
Fields
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- 'resource AddressResource? - Address resource
shipwire.receivings: AddressResource
Address resource
Fields
- email string? - Email address of the shipper. i.e. the merchant sending inventory to the Shipwire warehouse.
- name string? - Name of the shipper.
- address1 string? - This is the first line of the address of the shipper.
- address2 string? - This is the second line of the address of the shipper.
- address3 string? - This is the third line of the address of the shipper.
- city string? - City from where the inventory is shipped.
- state string? - State from where the inventory is shipped.
- postalCode string? - Postal code of the area from where the inventory is shipped.
- country string? - Country from where the inventory is shipped.
- phone string? - Contact phone number of the shipper for any communication.
shipwire.receivings: AdvanceShippingModel
Fields
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- 'resource AdvanceshippingmodelResource? -
shipwire.receivings: AdvanceshippingmodelResource
Fields
- externalId string? - Unique ID of the Advance Shipping Notice in an external system.
- orderNo string? - Purchase Order number associated with the Advance Shipping Notice.
- id int? - Unique ID of the Advance Shipping Notice in an Shipwire system.
- transactionId string? - Transaction ID of the Advance Shipping Notice in Shipwire system.
- expectedDate string? - Date on which the Advance Shipping Notice is expected to arrive at the Shipwire warehouse.
- commerceName string? - This determines the medium through which the Advance Shipping Notice is created. If it is created through a shopping cart the commerce name will be Shoppify, Magento. If created through the platform it will be Shippwirewebapp and if created through API it will be Shippwire. If created through Excel sheet it will be Spreadsheet.
- lastUpdateDate string? - Date on which the Advance Shipping Notice was last updated.
- status string? - Status of the Advance Shipping Notice. (e.g. completed, held)
- vendorId int? - A unique ID assigned to each vendor in the Shipwire system. (See the Vendors API.)
- vendorExternalId string? - A unique user-provided identifier for a vendor in an external system.
- items ReceivingItems? - Items being shipped to Shipwire
- holds ReceivingHolds? -
- trackings Trackings? - Specifies shipment tracking details. See
trackings
resource.
- shipments Shipments? - Specifies goods details. See
shipment
resource.
- labels Labels? -
- instructionsRecipients InstructionsRecipients? - List of contacts and instructions to send to each of them
- options Options? - Specify the warehouse where items are being shipped
- arrangement Arrangement? - The arrangement type tells Shipwire what further actions to take once the advance ship notice is created.
- shipForm record {}? -
- routing Routing? - Package routing details
- events Events? - Events registered to this advance ship notice
- documents Documents? -
- extendedAttributes ReceivingextendedattributesResource? - ReceivingExtendedAttributes resource
shipwire.receivings: Arrangement
The arrangement type tells Shipwire what further actions to take once the advance ship notice is created.
Fields
- contact string? - Contact name of the person from Shipwire system responsible for coordinating with the carrier or supplier for arranging delivery if needed.
- phone string? - Contact phone number of the person from Shipwire system responsible for coordinating with the carrier or supplier for arranging delivery if needed.
- 'type string? - Type
shipwire.receivings: CancelAReceivingLabelResponse
Fields
- status int? - This is the HTTP status code.
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- message string? - This is the HTTP status message.
shipwire.receivings: CancelAReceivingOrderResponse
Fields
- status int? - This is the HTTP status code.
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- message string? - This is the HTTP status message.
shipwire.receivings: ClientHttp1Settings
Provides settings related to HTTP/1.x protocol.
Fields
- keepAlive KeepAlive(default http:KEEPALIVE_AUTO) - Specifies whether to reuse a connection for multiple requests
- chunking Chunking(default http:CHUNKING_AUTO) - The chunking behaviour of the request
- proxy ProxyConfig? - Proxy server related options
shipwire.receivings: ConnectionConfig
Provides a set of configurations for controlling the behaviours when communicating with a remote HTTP endpoint.
Fields
- auth CredentialsConfig - Configurations related to client authentication
- httpVersion HttpVersion(default http:HTTP_2_0) - The HTTP version understood by the client
- http1Settings ClientHttp1Settings? - Configurations related to HTTP/1.x protocol
- http2Settings ClientHttp2Settings? - Configurations related to HTTP/2 protocol
- timeout decimal(default 60) - The maximum time to wait (in seconds) for a response before closing the connection
- forwarded string(default "disable") - The choice of setting
forwarded
/x-forwarded
header
- poolConfig PoolConfiguration? - Configurations associated with request pooling
- cache CacheConfig? - HTTP caching related configurations
- compression Compression(default http:COMPRESSION_AUTO) - Specifies the way of handling compression (
accept-encoding
) header
- circuitBreaker CircuitBreakerConfig? - Configurations associated with the behaviour of the Circuit Breaker
- retryConfig RetryConfig? - Configurations associated with retrying
- responseLimits ResponseLimitConfigs? - Configurations associated with inbound response size limits
- secureSocket ClientSecureSocket? - SSL/TLS-related options
- proxy ProxyConfig? - Proxy server related options
- validation boolean(default true) - Enables the inbound payload validation functionality which provided by the constraint package. Enabled by default
shipwire.receivings: CreateAnAdvanceShipNoticeRequest
Fields
- externalId string? - Unique ID of the Advance Shipping Notice in an external system.
- orderNo string? - Purchase Order number associated with the Advance Shipping Notice.
- expectedDate string? - Date on which the Advance Shipping Notice is expected to arrive at the Shipwire warehouse.
- options OptionsResource - Options resource
- arrangement Arrangement - The arrangement type tells Shipwire what further actions to take once the advance ship notice is created.
- shipments Shipment - Shipment
- labels Label? -
- trackings Tracking[]? - Specifies shipment tracking details. See
trackings
resource.
- items CreateReceivingItem[] - Items being shipped to Shipwire
- shipFrom AddressResource - Address resource
- instructionsRecipients InstructionsRecipient[]? -
- extendedAttributes CreateAnAdvanceShipNoticeRequest? -
shipwire.receivings: CreateReceivingItem
Item being shipped to Shipwire
Fields
- sku string? - SKU (Stock Keeping Unit) is a unique name for your product and is required for both physical and virtual products in inventory.
- quantity int? - The quantity of total SKU items belonging to the particular lot.
- id int? - A unique auto-generated ID assigned to each new line item added to the Shipwire Platform.
- productId int? - A unique auto-generated ID assigned to each new product added to the Shipwire Product Catalog.
- productExternalId string? - A unique user-provided identifier for a product in an external system.
- orderId int? - This is the receving order ID in the shipwire system on which the hold is applied.
- orderExternalId string? - This is the receving order ID in the external system on which the hold is applied.
- expected int? - The total number of SKU items expected to be received at the warehouse as per the order.
- pending int? - The number of pending SKU items expected to be received at the warehouse.
- good int? - The number of SKU items ready for fulfillment in the warehouse.
- inReview int? - The number of SKU items currently under review within the warehouse. (i.e. items being reviewed to determine if they are in sellable condition or damaged, etc.)
- damaged int? - The number of SKU items found to be damaged (during receiving or otherwise) within the warehouse and unavailable for fulfillment.
shipwire.receivings: Documents
Fields
- resourceLocation string? - A URL for downloading the generic label format that the warehouse generates for each customer.
- 'resource DocumentsResource? -
shipwire.receivings: DocumentsResource
Fields
- genericLabel string? - Link to dowload a generic label document
- receivingGuide string? - Link to dowload a Shipwire Warehouse Receiving guide
shipwire.receivings: Events
Events registered to this advance ship notice
Fields
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- 'resource EventsResource? - Events resource
shipwire.receivings: EventsResource
Events resource
Fields
- createdDate string? - Date on which the Advance Shipping Notice is created by the merchant.
- pickedUpDate string? - Date on which the inventory is picked up from the Shipper's address.
- submittedDate string? - Date on which the Advance Shipping Notice is submitted to the Shipwire warehouse.
- processedDate string? - Date on which the Advance Shipping Notice is processed i.e. the details of inventory are entered in the Warehouse Management System.
- completedDate string? - Date on which the Advance Shipping Notice is completed by the merchant.
- expectedDate string? - Date on which the inventory is expected to arrive at the Shipwire warehouse.
- deliveredDate string? - Date on which the inventory is received at the Shipwire warehouse.
- cancelledDate string? - Date on which the Advance Shipping Notice is cancelled due to any reason.
- returnedDate string? - Date on which the partial/full inventory is returned to the Shipper's address.
- lastManualUpdateDate string? - Date on which the Advance Shipping Notice was last edited by the merchant manually.
shipwire.receivings: ExtendedAttributes
Extended attributes
Fields
- name string? - Name of the extended attribute i.e. any additional information as per the customer request to be added in the receiving order. (e.g. return instructions)
- value string? - Value of the extended attribute which depends on the customer request. (e.g. For return instructions the value can be mail in or store)
- 'type string? - Data type of the extended attribute value i.e. a string or integer
shipwire.receivings: GetAdvanceShipNoticeResponse
Fields
- status int? - This is the HTTP status code.
- message string? - This is the HTTP status message.
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- 'resource record {}? -
shipwire.receivings: GetHoldDetailsResponse
Get instructions recipients details response
Fields
- status int? - This is the HTTP status code.
- message string? - This is the HTTP status message.
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- 'resource GetholddetailsresponseResource? - GetHoldDetailsResponse resource
shipwire.receivings: GetholddetailsresponseResource
GetHoldDetailsResponse resource
Fields
- offset record {}? - This is an integer that matches the offset given in the request and dictates the number of items to skip while fetching results. For example, there is a preset limit of 20 results per page. If there are 30 total results and offset is set to 20, then the response will show the second "page" of results, with items 21 to 30. type: integer example: 0 total: description: Total number of available items in the API response. type: integer example: 1 previous: description: A link used to fetch the previous set or page of items in the API response. type: string example: null next: description: A link used to fetch the next set or page of items in the API response. type: string example: null items: type: array items: type: object properties: resourceLocation: example: null type: string resource: allOf: - $ref:
shipwire.receivings: GetInstructionsRecipientsDetailsResponse
Get instructions recipients details response
Fields
- status int? - This is the HTTP status code.
- message string? - This is the HTTP status message.
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- 'resource GetinstructionsrecipientsdetailsresponseResource? - GetInstructionsRecipientsDetailsResponse resource
shipwire.receivings: GetinstructionsrecipientsdetailsresponseResource
GetInstructionsRecipientsDetailsResponse resource
Fields
- offset int? - This is an integer that matches the offset given in the request and dictates the number of items to skip while fetching results. For example, there is a preset limit of 20 results per page. If there are 30 total results and offset is set to 20, then the response will show the second "page" of results, with items 21 to 30.
- total int? - Total number of available items in the API response.
- previous string? - A link used to fetch the previous set or page of items in the API response.
- next string? - A link used to fetch the next set or page of items in the API response.
- items GetinstructionsrecipientsdetailsresponseResourceItems[]? - GetInstructionsRecipientsDetailsResponse resource items
shipwire.receivings: GetinstructionsrecipientsdetailsresponseResourceItems
Fields
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- 'resource record {}? -
shipwire.receivings: GetItemsDetailResponse
Get items details response
Fields
- status int? - This is the HTTP status code.
- message string? - This is the HTTP status message.
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- 'resource GetinstructionsrecipientsdetailsresponseResource? - GetInstructionsRecipientsDetailsResponse resource
shipwire.receivings: GetLabelsDetailResponse
Get labels details response
Fields
- status int? - This is the HTTP status code.
- message string? - This is the HTTP status message.
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- 'resource GetlabelsdetailresponseResource? - GetLabelsDetailResponse resource
shipwire.receivings: GetlabelsdetailresponseResource
GetLabelsDetailResponse resource
Fields
- offset int? - This is an integer that matches the offset given in the request and dictates the number of items to skip while fetching results. For example, there is a preset limit of 20 results per page. If there are 30 total results and offset is set to 20, then the response will show the second "page" of results, with items 21 to 30.
- total int? - Total number of available items in the API response.
- previous string? - A link used to fetch the previous set or page of items in the API response.
- next string? - A link used to fetch the next set or page of items in the API response.
- items GetlabelsdetailresponseResourceItems[]? - GetLabelsDetailResponse resource items
shipwire.receivings: GetlabelsdetailresponseResourceItems
GetLabelsDetailResponse resource items
Fields
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- 'resource record {}? - Resource
shipwire.receivings: GetListOfAdvanceShipNoticesResponse
Fields
- Fields Included from *ReceivingServerResponse
- 'resource record {}? -
shipwire.receivings: GetReceivingExtendedAttributesResponse
Get receiving extended attributes response
Fields
- status int? - This is the HTTP status code.
- message string? - This is the HTTP status message.
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- 'resource GetinstructionsrecipientsdetailsresponseResource? - GetInstructionsRecipientsDetailsResponse resource
shipwire.receivings: GetShipmentDetailsResponse
Get shipment details response
Fields
- status int? - This is the HTTP status code.
- message string? - This is the HTTP status message.
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- 'resource GetshipmentdetailsresponseResource? - GetShipmentDetailsResponse resource
shipwire.receivings: GetshipmentdetailsresponseResource
GetShipmentDetailsResponse resource
Fields
- offset int? - This is an integer that matches the offset given in the request and dictates the number of items to skip while fetching results. For example, there is a preset limit of 20 results per page. If there are 30 total results and offset is set to 20, then the response will show the second "page" of results, with items 21 to 30.
- total int? - Total number of available items in the API response.
- previous string? - A link used to fetch the previous set or page of items in the API response.
- next string? - A link used to fetch the next set or page of items in the API response.
- items GetshipmentdetailsresponseResourceItems[]? - GetShipmentDetailsResponse resource items
shipwire.receivings: GetshipmentdetailsresponseResourceItems
Fields
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- 'resource record {}? -
shipwire.receivings: GetTrackingsDetailResponse
Get trackings details response
Fields
- status int? - This is the HTTP status code.
- message string? - This is the HTTP status message.
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- 'resource GettrackingsdetailresponseResource? - GetTrackingsDetailResponse resource
shipwire.receivings: GettrackingsdetailresponseResource
GetTrackingsDetailResponse resource
Fields
- offset int? - This is an integer that matches the offset given in the request and dictates the number of items to skip while fetching results. For example, there is a preset limit of 20 results per page. If there are 30 total results and offset is set to 20, then the response will show the second "page" of results, with items 21 to 30.
- total int? - Total number of available items in the API response.
- previous string? - A link used to fetch the previous set or page of items in the API response.
- next string? - A link used to fetch the next set or page of items in the API response.
- items GettrackingsdetailresponseResourceItems[]? - GetTrackingsDetailResponse resource items
shipwire.receivings: GettrackingsdetailresponseResourceItems
Fields
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- 'resource record {}? -
shipwire.receivings: InstructionsRecipient
Fields
- email string? - Email address of individual responsible for processing order/inventory at receiving facility.
- name string? - Name of individual responsible for processing order/inventory at receiving facility.
- note string? - May be used to provide instructions to recipient on how to process and store received inventory.
shipwire.receivings: InstructionsRecipients
List of contacts and instructions to send to each of them
Fields
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- 'resource record {}? - Object
shipwire.receivings: Label
Fields
- labelId int? - A unique ID assigned to each shipping label in the shipwire system.
- orderId int? - This is the receving order ID in the shipwire system on which the hold is applied.
- orderExternalId string? - This is the receving order ID in the external system on which the hold is applied.
shipwire.receivings: Labels
Fields
- 'resource LabelsResource? -
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
shipwire.receivings: LabelsResource
Fields
- offset int? - This is an integer that matches the offset given in the request and dictates the number of items to skip while fetching results. For example, there is a preset limit of 20 results per page. If there are 30 total results and offset is set to 20, then the response will show the second "page" of results, with items 21 to 30.
- total int? - Total number of available items in the API response.
- previous string? - A link used to fetch the previous set or page of items in the API response.
- next string? - A link used to fetch the next set or page of items in the API response.
- items LabelsResourceItems[]? -
shipwire.receivings: LabelsResourceItems
Fields
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- 'resource Label? -
shipwire.receivings: MarkTheReceivingCompletedResponse
Mark the receiving as completed
Fields
- status int? - This is the HTTP status code.
- message string? - This is the HTTP status message.
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
shipwire.receivings: Options
Specify the warehouse where items are being shipped
Fields
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- 'resource OptionsResource? - Options resource
shipwire.receivings: OptionsResource
Options resource
Fields
- warehouseExternalId string? - A unique user-provided identifier for a Shipwire Anywhere warehouse in an external system.
- warehouseId int? - A unique identifier for a Shipwire or Shipwire Anywhere warehouse.
- warehouseRegion string? - This is the place where the warehouse is located.
- doNotSendEmail int? - A boolean which indicates whether or not the warehouse sends ASN related emails on events such as creation, delivery. The value '1' (True) means that the emails will not be sent.
shipwire.receivings: PostListOfAdvanceShipNoticesResponse
Fields
- Fields Included from *ReceivingServerResponse
- 'resource ReceivingitemsResource? - ReceivingItems resource
shipwire.receivings: ProxyConfig
Proxy server configurations to be used with the HTTP client endpoint.
Fields
- host string(default "") - Host name of the proxy server
- port int(default 0) - Proxy server port
- userName string(default "") - Proxy server username
- password string(default "") - Proxy server password
shipwire.receivings: ReceivingextendedattributesResource
ReceivingExtendedAttributes resource
Fields
- offset int? - This is an integer that matches the offset given in the request and dictates the number of items to skip while fetching results. For example, there is a preset limit of 20 results per page. If there are 30 total results and offset is set to 20, then the response will show the second "page" of results, with items 21 to 30.
- total int? - Total number of available items in the API response.
- previous string? - A link used to fetch the previous set or page of items in the API response.
- next string? - A link used to fetch the next set or page of items in the API response.
- items ReceivingextendedattributesResourceItems[]? - ReceivingExtendedAttributes resource items
shipwire.receivings: ReceivingextendedattributesResourceItems
ReceivingExtendedAttributes resource items
Fields
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- 'resource ExtendedAttributes? - Extended attributes
shipwire.receivings: ReceivingHold
Holds that apply to this advance ship notice
Fields
- id int? - This is the unique ID assigned to a particular hold in the shipwire system.
- orderId int? - This is the receving order ID in the shipwire system on which the hold is applied.
- externalOrderId string? - This is the receving order ID in the external system on which the hold is applied.
- 'type string? - This is the type of hold due to which the order is stuck. (e.g. customer, admin, etc.)
- description string? - This is the description given for the hold in the Shipwire system.
- appliedDate string? - This is the date on which the hold is applied to the order.
- clearedDate string? - This is the date on which the hold is removed from the order.
shipwire.receivings: ReceivingHolds
Fields
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- 'resource ReceivingholdsResource? -
shipwire.receivings: ReceivingholdsResource
Fields
- offset int? - This is an integer that matches the offset given in the request and dictates the number of items to skip while fetching results. For example, there is a preset limit of 20 results per page. If there are 30 total results and offset is set to 20, then the response will show the second "page" of results, with items 21 to 30.
- total int? - Total number of available items in the API response.
- previous string? - A link used to fetch the previous set or page of items in the API response.
- next string? - A link used to fetch the next set or page of items in the API response.
- items ReceivingholdsResourceItems[]? -
shipwire.receivings: ReceivingholdsResourceItems
Fields
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- 'resource ReceivingHold? - Holds that apply to this advance ship notice
shipwire.receivings: ReceivingItem
Item being shipped to Shipwire
Fields
- sku string? - SKU (Stock Keeping Unit) is a unique name for your product and is required for both physical and virtual products in inventory.
- quantity int? - The quantity of total SKU items belonging to the particular lot.
- id int? - A unique auto-generated ID assigned to each new line item added to the Shipwire Platform.
- productId int? - A unique auto-generated ID assigned to each new product added to the Shipwire Product Catalog.
- productExternalId string? - A unique user-provided identifier for a product in an external system.
- orderId int? - This is the receving order ID in the shipwire system on which the hold is applied.
- orderExternalId string? - This is the receving order ID in the external system on which the hold is applied.
- expected int? - The total number of SKU items expected to be received at the warehouse as per the order.
- pending int? - The number of pending SKU items expected to be received at the warehouse.
- good int? - The number of SKU items ready for fulfillment in the warehouse.
- inReview int? - The number of SKU items currently under review within the warehouse. (i.e. items being reviewed to determine if they are in sellable condition or damaged, etc.)
- damaged int? - The number of SKU items found to be damaged (during receiving or otherwise) within the warehouse and unavailable for fulfillment.
- lotControl ReceivingitemLotcontrol? - ReceivingItem lotControl
- extendedAttributes ReceivingitemExtendedattributes? - ReceivingItem extended attributes
shipwire.receivings: ReceivingitemExtendedattributes
ReceivingItem extended attributes
Fields
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- 'resource ReceivingextendedattributesResource? - ReceivingExtendedAttributes resource
shipwire.receivings: ReceivingitemLotcontrol
ReceivingItem lotControl
Fields
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- 'resource ReceivinglotcontrolResource? - ReceivingLotControl resource
shipwire.receivings: ReceivingItems
Items being shipped to Shipwire
Fields
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- 'resource ReceivingitemsResource? - ReceivingItems resource
shipwire.receivings: ReceivingitemsResource
ReceivingItems resource
Fields
- offset int? - This is an integer that matches the offset given in the request and dictates the number of items to skip while fetching results. For example, there is a preset limit of 20 results per page. If there are 30 total results and offset is set to 20, then the response will show the second "page" of results, with items 21 to 30.
- total int? - Total number of available items in the API response.
- previous string? - A link used to fetch the previous set or page of items in the API response.
- next string? - A link used to fetch the next set or page of items in the API response.
- items ReceivingitemsResourceItems[]? - ReceivingItems resource items
shipwire.receivings: ReceivingitemsResourceItems
Fields
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- 'resource ReceivingItem? - Item being shipped to Shipwire
shipwire.receivings: ReceivingLotControl
Fields
- lotId string? - A unique ID assigned to a particular quantity or lot of material shipped from a siingle merchant.
- quantity int? - The quantity of total SKU items belonging to the particular lot.
- expirationDate string? - This is the expiry date of the product belonging to the particular lot.
shipwire.receivings: ReceivinglotcontrolResource
ReceivingLotControl resource
Fields
- offset int? - This is an integer that matches the offset given in the request and dictates the number of items to skip while fetching results. For example, there is a preset limit of 20 results per page. If there are 30 total results and offset is set to 20, then the response will show the second "page" of results, with items 21 to 30.
- total int? - Total number of available items in the API response.
- previous string? - A link used to fetch the previous set or page of items in the API response.
- next string? - A link used to fetch the next set or page of items in the API response.
- items ReceivinglotcontrolResourceItems[]? - ReceivingLotControl resource items
shipwire.receivings: ReceivinglotcontrolResourceItems
Fields
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- 'resource ReceivingLotControl? -
shipwire.receivings: ReceivingServerPaginationResponse
Receiving Server Pagination Response
Fields
- offset int? - This is an integer that matches the offset given in the request and dictates the number of items to skip while fetching results. For example, there is a preset limit of 20 results per page. If there are 30 total results and offset is set to 20, then the response will show the second "page" of results, with items 21 to 30.
- total int? - Total number of available items in the API response.
- previous string? - A link used to fetch the previous set or page of items in the API response.
- next string? - A link used to fetch the next set or page of items in the API response.
shipwire.receivings: ReceivingServerResponse
Receiving Server Response
Fields
- status int? - This is the HTTP status code.
- message string? - This is the HTTP status message.
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
shipwire.receivings: Routing
Package routing details
Fields
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- 'resource RoutingResource? - Routing resource
shipwire.receivings: RoutingResource
Routing resource
Fields
- originLatitude decimal? - Latitude of the warehouse. This is useful for routing purposes to find the nearest warehouse.
- originLongitude decimal? - Longitude of the warehouse. This is useful for routing purposes to find the nearest warehouse.
- warehouseExternalId string? - A unique user-provided identifier for a Shipwire Anywhere warehouse in an external system.
- warehouseId int? - A unique identifier for a Shipwire or Shipwire Anywhere warehouse.
- warehouseName string? - This is the name of the warehouse.
- warehouseRegion string? - This is the place where the warehouse is located.
shipwire.receivings: Shipment
Shipment
Fields
- shipmentId int? - A unique ID assigned to the shipment (container) in the shipwire system.
- 'type string? - Type of the shipment (container) based on the contents stored in it (e.g. a box, parcel, etc.)
- height decimal? - This is the height of the shipment (container) based on its Unit of Measurement
- length decimal? - This is the length of the shipment (container) based on its Unit of Measurement
- weight decimal? - This is the weight of the shipment (container) based on its Unit of Measurement
- width decimal? - This is the width of the shipment (container) based on its Unit of Measurement
shipwire.receivings: Shipments
Specifies goods details. See shipment
resource.
Fields
- 'resource ShipmentsResource? - Shipments resource
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
shipwire.receivings: ShipmentsResource
Shipments resource
Fields
- offset int? - This is an integer that matches the offset given in the request and dictates the number of items to skip while fetching results. For example, there is a preset limit of 20 results per page. If there are 30 total results and offset is set to 20, then the response will show the second "page" of results, with items 21 to 30.
- total int? - Total number of available items in the API response.
- previous string? - A link used to fetch the previous set or page of items in the API response.
- next string? - A link used to fetch the next set or page of items in the API response.
- items ShipmentsResourceItems[]? - Shipments resource items
shipwire.receivings: ShipmentsResourceItems
Shipments resource items
Fields
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- 'resource Shipment? - Shipment
shipwire.receivings: Tracking
Fields
- id int? - This is the unquie ID generated in the shipwire system for each tracking record.
- orderId int? - This is the receving order ID in the shipwire system on which the hold is applied.
- orderExternalId string? - This is the receving order ID in the external system on which the hold is applied.
- tracking string? - This is reference tracking number provided by the carrier for tracking purposes.
- carrier string? - This is the unique ID of the carrier used to deliver the order to the warehouse.
- contact string? - Name of person from the carrier company who can be contacted for any communication.
- phone string? - Phone number of the contact person from the carrier company for any communication.
- url string? - A URL that gives more information about the tracking record.
- summary string? - Summary added to the tracking record for tracking purposes.
- summaryDate string? - Date on which the summary is added to the tracking record.
- trackedDate string? -
- delivered1Date string? - Date on which the the inventory is received against the tracking record.
- firstScanDate string? - Date the order's associated shipping carrier scans the label when it receives the order.
- labelCreationDate string? - Date on which the shipping labels are created for the tracking record.
shipwire.receivings: Trackings
Specifies shipment tracking details. See trackings
resource.
Fields
- 'resource TrackingsResource? - Trackings resource
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
shipwire.receivings: TrackingsResource
Trackings resource
Fields
- offset int? - This is an integer that matches the offset given in the request and dictates the number of items to skip while fetching results. For example, there is a preset limit of 20 results per page. If there are 30 total results and offset is set to 20, then the response will show the second "page" of results, with items 21 to 30.
- total int? - Total number of available items in the API response.
- previous string? - A link used to fetch the previous set or page of items in the API response.
- next string? - A link used to fetch the next set or page of items in the API response.
- items TrackingsResourceItems[]? - Trackings resource items
shipwire.receivings: TrackingsResourceItems
Fields
- resourceLocation string? - A URL that gives more information about the linked resource. A 'null' value would mean that no further information is available for that resource.
- 'resource Tracking? -
Import
import ballerinax/shipwire.receivings;
Metadata
Released date: about 2 years ago
Version: 1.5.1
License: Apache-2.0
Compatibility
Platform: any
Ballerina version: 2201.4.1
GraalVM compatible: Yes
Pull count
Total: 5
Current verison: 5
Weekly downloads
Keywords
Commerce/eCommerce
Cost/Freemium
Contributors